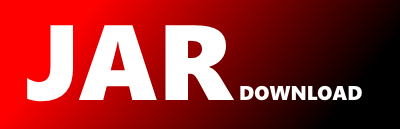
io.starter.ignite.generator.swagger.StackModelRelationGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stackgen Show documentation
Show all versions of stackgen Show documentation
Starter StackGen CORE Service Generator
package io.starter.ignite.generator.swagger;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import io.starter.ignite.generator.MyBatisJoin;
import io.swagger.models.Model;
import io.swagger.models.Swagger;
import io.swagger.models.properties.Property;
import io.swagger.models.properties.RefProperty;
/**
*
* * StackGen Model support for ONE-TO-MANY and MANY-TO-MANY relationships
* can be expressed as a many-to-many with a unique constraint on the "ONE" field
*
*
* 2 stages:
* - create "fake" swagger objects for IDX tables, let MyBatis and DML go forth
* - do 2nd pass on MyBatis XML output and add the proper many-to-many hydration mappings
*
* - when we encounter a FK_ID field in the Swagger ,we need to implement a many-to-many IDX table
* - when we encounter a one-to many "xxxx_id" relationship -- ie: owner_id
*
*
*
...
*
* @author john
*
*/
public class StackModelRelationGenerator {
protected final Logger logger = LoggerFactory
.getLogger(StackModelRelationGenerator.class);
public List generate(Swagger swagger) {
logger.warn("DISABLED: Generating Stack Model Relationships for : "
+ swagger.getInfo().getDescription());
List refs = new ArrayList();
if (true) // TODO: enable!
return refs;
// for each model, iterate the props
// if it is an ARRAY value,
Map models = swagger.getDefinitions();
fixTitles(models);
for (Model mdx : models.values()) {
Map props = mdx.getProperties();
fixNames(props);
/*
* ref String "#/definitions/CalendarEvent" (id=241) String
* simpleRef String "CalendarEvent" (id=242) String 242
* type RefType RefType (id=243) RefType 243 4
* name String "events" (id=194) String 194 13440
* required boolean false boolean
* type String "ref" (id=196) String 196 13440
*/
for (Property prop : props.values()) {
// then we need an IDX table
String field = prop.getName();
String[] tables = { prop.getName() };
String pt = prop.getType();
// it is a one-many foreign key ie: OWNER_ID
if (pt.equals("integer")
&& prop.getName().toLowerCase().endsWith("_id")) {
// TODO: handle the one-to-many ID issue
// MyBatisJoin j = new MyBatisJoin(field, tables);
} else if (pt.equals("ref")) {
RefProperty rp = (RefProperty) prop;
String sr = rp.getSimpleRef();
String sr1 = rp.get$ref();
if (sr1 != null)
sr1 = sr1.substring(sr1.lastIndexOf("/") + 1, sr1
.length());
Model rpm = models.get(sr);
// create IDX table for array types
// like shared multiple resources GROUPS or ROLES
if (rpm != null) {
if (rpm.getTitle() == null) {
rpm.setTitle(sr1);
}
MyBatisJoin j = new MyBatisJoin(field, mdx, rpm);
// creates the *Ref XML to inject into the Main Object
// Mapping
refs.add(j);
}
}
}
}
logger.info("Done Generating Stack Model Relationships.");
return refs;
}
private void fixTitles(Map models) {
for (String key : models.keySet()) {
Model p = models.get(key);
p.setTitle(key);
}
}
private void fixNames(Map props) {
for (String key : props.keySet()) {
Property p = props.get(key);
p.setName(key);
}
}
/*
*
* String t1 = "customer";
* String t2 = "order";
* String t3 = "order_detail";
*
* String f1 = "customer_id";
* String f2 = "order_id";
*
* String[] tables = { t1, t2, t3 };
* String[] fields = { f1, f2 };
*
* for (String field : fields) {
* MyBatisJoin j = new MyBatisJoin(field, tables);
* System.out.println(j.getXMLFragment());
* System.out.println(j.getDML());
* }
*/
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy