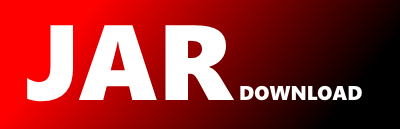
Java.libraries.resttemplate.ApiClient.mustache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stackgen Show documentation
Show all versions of stackgen Show documentation
Starter StackGen CORE Service Generator
package {{invokerPackage}};
{{#withXml}}
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.ser.ToXmlGenerator;
{{/withXml}}
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpRequest;
import org.springframework.http.HttpStatus;
import org.springframework.http.InvalidMediaTypeException;
import org.springframework.http.MediaType;
import org.springframework.http.RequestEntity;
import org.springframework.http.RequestEntity.BodyBuilder;
import org.springframework.http.ResponseEntity;
import org.springframework.http.client.BufferingClientHttpRequestFactory;
import org.springframework.http.client.ClientHttpRequestExecution;
import org.springframework.http.client.ClientHttpRequestInterceptor;
import org.springframework.http.client.ClientHttpResponse;
{{#withXml}}
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.http.converter.xml.MappingJackson2XmlHttpMessageConverter;
{{/withXml}}
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.util.StringUtils;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.RestTemplate;
import org.springframework.web.util.UriComponentsBuilder;
{{#threetenbp}}
import org.threeten.bp.*;
import com.fasterxml.jackson.datatype.threetenbp.ThreeTenModule;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.http.converter.json.AbstractJackson2HttpMessageConverter;
import com.fasterxml.jackson.databind.ObjectMapper;
{{/threetenbp}}
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import java.nio.charset.StandardCharsets;
import java.text.DateFormat;
import java.text.ParseException;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.TimeZone;
import {{invokerPackage}}.auth.Authentication;
import {{invokerPackage}}.auth.HttpBasicAuth;
import {{invokerPackage}}.auth.ApiKeyAuth;
import {{invokerPackage}}.auth.OAuth;
{{>generatedAnnotation}}
@Component("{{invokerPackage}}.ApiClient")
public class ApiClient {
public enum CollectionFormat {
CSV(","), TSV("\t"), SSV(" "), PIPES("|"), MULTI(null);
private final String separator;
private CollectionFormat(String separator) {
this.separator = separator;
}
private String collectionToString(Collection extends CharSequence> collection) {
return StringUtils.collectionToDelimitedString(collection, separator);
}
}
private boolean debugging = false;
private HttpHeaders defaultHeaders = new HttpHeaders();
private String basePath = "{{basePath}}";
private RestTemplate restTemplate;
private Map authentications;
private HttpStatus statusCode;
private MultiValueMap responseHeaders;
private DateFormat dateFormat;
public ApiClient() {
this.restTemplate = buildRestTemplate();
init();
}
@Autowired
public ApiClient(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
init();
}
protected void init() {
// Use RFC3339 format for date and datetime.
// See http://xml2rfc.ietf.org/public/rfc/html/rfc3339.html#anchor14
this.dateFormat = new RFC3339DateFormat();
// Use UTC as the default time zone.
this.dateFormat.setTimeZone(TimeZone.getTimeZone("UTC"));
// Set default User-Agent.
setUserAgent("Java-SDK");
// Setup authentications (key: authentication name, value: authentication).
authentications = new HashMap();{{#authMethods}}{{#isBasic}}
authentications.put("{{name}}", new HttpBasicAuth());{{/isBasic}}{{#isApiKey}}
authentications.put("{{name}}", new ApiKeyAuth({{#isKeyInHeader}}"header"{{/isKeyInHeader}}{{^isKeyInHeader}}"query"{{/isKeyInHeader}}, "{{keyParamName}}"));{{/isApiKey}}{{#isOAuth}}
authentications.put("{{name}}", new OAuth());{{/isOAuth}}{{/authMethods}}
// Prevent the authentications from being modified.
authentications = Collections.unmodifiableMap(authentications);
}
/**
* Get the current base path
* @return String the base path
*/
public String getBasePath() {
return basePath;
}
/**
* Set the base path, which should include the host
* @param basePath the base path
* @return ApiClient this client
*/
public ApiClient setBasePath(String basePath) {
this.basePath = basePath;
return this;
}
/**
* Gets the status code of the previous request
* @return HttpStatus the status code
*/
public HttpStatus getStatusCode() {
return statusCode;
}
/**
* Gets the response headers of the previous request
* @return MultiValueMap a map of response headers
*/
public MultiValueMap getResponseHeaders() {
return responseHeaders;
}
/**
* Get authentications (key: authentication name, value: authentication).
* @return Map the currently configured authentication types
*/
public Map getAuthentications() {
return authentications;
}
/**
* Get authentication for the given name.
*
* @param authName The authentication name
* @return The authentication, null if not found
*/
public Authentication getAuthentication(String authName) {
return authentications.get(authName);
}
/**
* Helper method to set username for the first HTTP basic authentication.
* @param username the username
*/
public void setUsername(String username) {
for (Authentication auth : authentications.values()) {
if (auth instanceof HttpBasicAuth) {
((HttpBasicAuth) auth).setUsername(username);
return;
}
}
throw new RuntimeException("No HTTP basic authentication configured!");
}
/**
* Helper method to set password for the first HTTP basic authentication.
* @param password the password
*/
public void setPassword(String password) {
for (Authentication auth : authentications.values()) {
if (auth instanceof HttpBasicAuth) {
((HttpBasicAuth) auth).setPassword(password);
return;
}
}
throw new RuntimeException("No HTTP basic authentication configured!");
}
/**
* Helper method to set API key value for the first API key authentication.
* @param apiKey the API key
*/
public void setApiKey(String apiKey) {
for (Authentication auth : authentications.values()) {
if (auth instanceof ApiKeyAuth) {
((ApiKeyAuth) auth).setApiKey(apiKey);
return;
}
}
throw new RuntimeException("No API key authentication configured!");
}
/**
* Helper method to set API key prefix for the first API key authentication.
* @param apiKeyPrefix the API key prefix
*/
public void setApiKeyPrefix(String apiKeyPrefix) {
for (Authentication auth : authentications.values()) {
if (auth instanceof ApiKeyAuth) {
((ApiKeyAuth) auth).setApiKeyPrefix(apiKeyPrefix);
return;
}
}
throw new RuntimeException("No API key authentication configured!");
}
/**
* Helper method to set access token for the first OAuth2 authentication.
* @param accessToken the access token
*/
public void setAccessToken(String accessToken) {
for (Authentication auth : authentications.values()) {
if (auth instanceof OAuth) {
((OAuth) auth).setAccessToken(accessToken);
return;
}
}
throw new RuntimeException("No OAuth2 authentication configured!");
}
/**
* Set the User-Agent header's value (by adding to the default header map).
* @param userAgent the user agent string
* @return ApiClient this client
*/
public ApiClient setUserAgent(String userAgent) {
addDefaultHeader("User-Agent", userAgent);
return this;
}
/**
* Add a default header.
*
* @param name The header's name
* @param value The header's value
* @return ApiClient this client
*/
public ApiClient addDefaultHeader(String name, String value) {
if (defaultHeaders.containsKey(name)) {
defaultHeaders.remove(name);
}
defaultHeaders.add(name, value);
return this;
}
public void setDebugging(boolean debugging) {
List currentInterceptors = this.restTemplate.getInterceptors();
if(debugging) {
if (currentInterceptors == null) {
currentInterceptors = new ArrayList();
}
ClientHttpRequestInterceptor interceptor = new ApiClientHttpRequestInterceptor();
currentInterceptors.add(interceptor);
this.restTemplate.setInterceptors(currentInterceptors);
} else {
if (currentInterceptors != null && !currentInterceptors.isEmpty()) {
Iterator iter = currentInterceptors.iterator();
while (iter.hasNext()) {
ClientHttpRequestInterceptor interceptor = iter.next();
if (interceptor instanceof ApiClientHttpRequestInterceptor) {
iter.remove();
}
}
this.restTemplate.setInterceptors(currentInterceptors);
}
}
this.debugging = debugging;
}
/**
* Check that whether debugging is enabled for this API client.
* @return boolean true if this client is enabled for debugging, false otherwise
*/
public boolean isDebugging() {
return debugging;
}
/**
* Get the date format used to parse/format date parameters.
* @return DateFormat format
*/
public DateFormat getDateFormat() {
return dateFormat;
}
/**
* Set the date format used to parse/format date parameters.
* @param dateFormat Date format
* @return API client
*/
public ApiClient setDateFormat(DateFormat dateFormat) {
this.dateFormat = dateFormat;
{{#threetenbp}}
for(HttpMessageConverter converter:restTemplate.getMessageConverters()){
if(converter instanceof AbstractJackson2HttpMessageConverter){
ObjectMapper mapper = ((AbstractJackson2HttpMessageConverter)converter).getObjectMapper();
mapper.setDateFormat(dateFormat);
}
}
{{/threetenbp}}
return this;
}
/**
* Parse the given string into Date object.
*/
public Date parseDate(String str) {
try {
return dateFormat.parse(str);
} catch (ParseException e) {
throw new RuntimeException(e);
}
}
/**
* Format the given Date object into string.
*/
public String formatDate(Date date) {
return dateFormat.format(date);
}
/**
* Format the given parameter object into string.
* @param param the object to convert
* @return String the parameter represented as a String
*/
public String parameterToString(Object param) {
if (param == null) {
return "";
} else if (param instanceof Date) {
return formatDate( (Date) param);
} else if (param instanceof Collection) {
StringBuilder b = new StringBuilder();
for(Object o : (Collection>) param) {
if(b.length() > 0) {
b.append(",");
}
b.append(String.valueOf(o));
}
return b.toString();
} else {
return String.valueOf(param);
}
}
/**
* Converts a parameter to a {@link MultiValueMap} for use in REST requests
* @param collectionFormat The format to convert to
* @param name The name of the parameter
* @param value The parameter's value
* @return a Map containing the String value(s) of the input parameter
*/
public MultiValueMap parameterToMultiValueMap(CollectionFormat collectionFormat, String name, Object value) {
final MultiValueMap params = new LinkedMultiValueMap();
if (name == null || name.isEmpty() || value == null) {
return params;
}
if(collectionFormat == null) {
collectionFormat = CollectionFormat.CSV;
}
Collection> valueCollection = null;
if (value instanceof Collection) {
valueCollection = (Collection>) value;
} else {
params.add(name, parameterToString(value));
return params;
}
if (valueCollection.isEmpty()){
return params;
}
if (collectionFormat.equals(CollectionFormat.MULTI)) {
for (Object item : valueCollection) {
params.add(name, parameterToString(item));
}
return params;
}
List values = new ArrayList();
for(Object o : valueCollection) {
values.add(parameterToString(o));
}
params.add(name, collectionFormat.collectionToString(values));
return params;
}
/**
* Check if the given {@code String} is a JSON MIME.
* @param mediaType the input MediaType
* @return boolean true if the MediaType represents JSON, false otherwise
*/
public boolean isJsonMime(String mediaType) {
// "* / *" is default to JSON
if ("*/*".equals(mediaType)) {
return true;
}
try {
return isJsonMime(MediaType.parseMediaType(mediaType));
} catch (InvalidMediaTypeException e) {
}
return false;
}
/**
* Check if the given MIME is a JSON MIME.
* JSON MIME examples:
* application/json
* application/json; charset=UTF8
* APPLICATION/JSON
* @param mediaType the input MediaType
* @return boolean true if the MediaType represents JSON, false otherwise
*/
public boolean isJsonMime(MediaType mediaType) {
return mediaType != null && (MediaType.APPLICATION_JSON.isCompatibleWith(mediaType) || mediaType.getSubtype().matches("^.*\\+json[;]?\\s*$"));
}
/**
* Select the Accept header's value from the given accepts array:
* if JSON exists in the given array, use it;
* otherwise use all of them (joining into a string)
*
* @param accepts The accepts array to select from
* @return List The list of MediaTypes to use for the Accept header
*/
public List selectHeaderAccept(String[] accepts) {
if (accepts.length == 0) {
return null;
}
for (String accept : accepts) {
MediaType mediaType = MediaType.parseMediaType(accept);
if (isJsonMime(mediaType)) {
return Collections.singletonList(mediaType);
}
}
return MediaType.parseMediaTypes(StringUtils.arrayToCommaDelimitedString(accepts));
}
/**
* Select the Content-Type header's value from the given array:
* if JSON exists in the given array, use it;
* otherwise use the first one of the array.
*
* @param contentTypes The Content-Type array to select from
* @return MediaType The Content-Type header to use. If the given array is empty, JSON will be used.
*/
public MediaType selectHeaderContentType(String[] contentTypes) {
if (contentTypes.length == 0) {
return MediaType.APPLICATION_JSON;
}
for (String contentType : contentTypes) {
MediaType mediaType = MediaType.parseMediaType(contentType);
if (isJsonMime(mediaType)) {
return mediaType;
}
}
return MediaType.parseMediaType(contentTypes[0]);
}
/**
* Select the body to use for the request
* @param obj the body object
* @param formParams the form parameters
* @param contentType the content type of the request
* @return Object the selected body
*/
protected Object selectBody(Object obj, MultiValueMap formParams, MediaType contentType) {
boolean isForm = MediaType.MULTIPART_FORM_DATA.isCompatibleWith(contentType) || MediaType.APPLICATION_FORM_URLENCODED.isCompatibleWith(contentType);
return isForm ? formParams : obj;
}
/**
* Invoke API by sending HTTP request with the given options.
*
* @param the return type to use
* @param path The sub-path of the HTTP URL
* @param method The request method
* @param queryParams The query parameters
* @param body The request body object
* @param headerParams The header parameters
* @param formParams The form parameters
* @param accept The request's Accept header
* @param contentType The request's Content-Type header
* @param authNames The authentications to apply
* @param returnType The return type into which to deserialize the response
* @return The response body in chosen type
*/
public T invokeAPI(String path, HttpMethod method, MultiValueMap queryParams, Object body, HttpHeaders headerParams, MultiValueMap formParams, List accept, MediaType contentType, String[] authNames, ParameterizedTypeReference returnType) throws RestClientException {
updateParamsForAuth(authNames, queryParams, headerParams);
final UriComponentsBuilder builder = UriComponentsBuilder.fromHttpUrl(basePath).path(path);
if (queryParams != null) {
builder.queryParams(queryParams);
}
final BodyBuilder requestBuilder = RequestEntity.method(method, builder.build().toUri());
if(accept != null) {
requestBuilder.accept(accept.toArray(new MediaType[accept.size()]));
}
if(contentType != null) {
requestBuilder.contentType(contentType);
}
addHeadersToRequest(headerParams, requestBuilder);
addHeadersToRequest(defaultHeaders, requestBuilder);
RequestEntity
© 2015 - 2024 Weber Informatics LLC | Privacy Policy