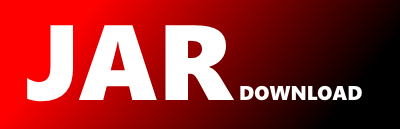
JavaSpring.swaggerDocumentationConfig.mustache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stackgen Show documentation
Show all versions of stackgen Show documentation
Starter StackGen CORE Service Generator
package {{configPackage}};
import java.util.Arrays;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import org.springframework.context.annotation.PropertySources;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.web.authentication.SavedRequestAwareAuthenticationSuccessHandler;
import org.springframework.web.cors.CorsConfiguration;
import org.springframework.web.cors.CorsConfigurationSource;
import org.springframework.web.cors.UrlBasedCorsConfigurationSource;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
{{#useOptional}}
import java.util.Optional;
{{/useOptional}}
{{>generatedAnnotation}}
@Configuration
@PropertySources({ @PropertySource("classpath:application.properties") })
public class SwaggerDocumentationConfig extends WebSecurityConfigurerAdapter {
private final String adminContextPath = "";
protected static final Logger logger = LoggerFactory
.getLogger(SwaggerDocumentationConfig.class);
@Value("${io.starter.stackgen.CORSOrigins:localhost}")
public String CORSOrigins;
@Value("${io.starter.stackgen.CORSMapping:/**}")
public String CORSMapping;
/**
* the CORS configuration for the REST api
*
* @return
*/
@Bean
CorsConfigurationSource corsConfigurationSource() {
logger.warn("Initializing CORS Config Origins: CORSOrigins "
+ CORSOrigins);
logger.warn("Initializing CORS Config Mapping: CORSMapping "
+ CORSMapping);
final CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList(CORSOrigins));
configuration.setAllowedMethods(Arrays
.asList("GET", "POST", "INSERT", "DELETE", "HEAD", "OPTIONS"));
final UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration(CORSMapping, configuration);
return source;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
// @formatter:off
final SavedRequestAwareAuthenticationSuccessHandler successHandler = new SavedRequestAwareAuthenticationSuccessHandler();
successHandler.setTargetUrlParameter("redirectTo");
// TODO: CHANGE THIS TO ENABLE AUTH
http.csrf().disable().cors();
/*
http.authorizeRequests()
.antMatchers(adminContextPath + "/assets/**").permitAll()
.antMatchers(adminContextPath + "/login**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().loginPage(adminContextPath + "/login").successHandler(successHandler).loginProcessingUrl("/login").and()
.logout().logoutUrl(adminContextPath + "/logout").and()
.httpBasic().and()
.csrf().disable();
*/
// @formatter:on
}
ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("{{appName}}")
.description("{{{appDescription}}}")
.license("{{licenseInfo}}")
.licenseUrl("{{licenseUrl}}")
.termsOfServiceUrl("{{infoUrl}}")
.version("{{appVersion}}")
.contact(new Contact("","", "{{infoEmail}}"))
.build();
}
@Bean
public Docket customImplementation(){
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("{{apiPackage}}"))
.build()
{{#java8}}
.directModelSubstitute(java.time.LocalDate.class, java.sql.Date.class)
.directModelSubstitute(java.time.OffsetDateTime.class, java.util.Date.class)
{{/java8}}
{{#joda}}
.directModelSubstitute(org.joda.time.LocalDate.class, java.sql.Date.class)
.directModelSubstitute(org.joda.time.DateTime.class, java.util.Date.class)
{{/joda}}
{{#threetenbp}}
.directModelSubstitute(org.threeten.bp.LocalDate.class, java.sql.Date.class)
.directModelSubstitute(org.threeten.bp.OffsetDateTime.class, java.util.Date.class)
{{/threetenbp}}
{{#useOptional}}
.genericModelSubstitutes(Optional.class)
{{/useOptional}}
.apiInfo(apiInfo());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy