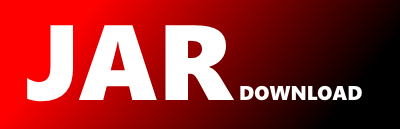
io.statusmachina.core.api.MachineDefinition Maven / Gradle / Ivy
/*
*
* Copyright 2019 <---> Present Status Machina Contributors (https://github.com/entzik/status-machina/graphs/contributors)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* This software is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
*/
package io.statusmachina.core.api;
import java.util.Optional;
import java.util.Set;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* Defines a state machine in terms of states, events and transitions.
*
* States are of a configurable type. The definition must provide all states, including exactly one initial state and at least one terminal state.
* The inital state cannot be a terminal state.
*
*Transitions determine how a machine will translate from one state to the other. There are two types of transitions:
*
* - STP (straight through processing
* - Event based
*
*
*
* An STP transition configured from state A to state B triggers an automatic translation to state B as soon as the machine enters state A.
* In the current version there can only be one STP transition configured from any state.
*
*
* An event transition from state A to state B, on event E will cause the machine in state A to translate to state B when it reveives event E
*
*
* Events are passive items delivered the machine reacts to
*
* @param - the type of the states the machine can find itself in
* @param - the type of events the machine can react to
*/
public interface MachineDefinition {
/**
* @return all possible states the machine can find itself in. This must inclue the initial and terminal states
*/
Set getAllStates();
/**
* @return the state a machine of this type will find itself in when first initialized.
*/
S getInitialState();
/**
* @return set of terminal states. Once the machine reaches one of this states it is considered to be completed. it is not possible to
* configure transitions out of a terminal state
*/
Set getTerminalStates();
/**
* @return the set of event the machine can react to
*/
Set getEvents();
/**
* @return the set of transitions that governs the state machine
*/
Set> getTransitions();
/**
* @param state the state out of which we ae looking for an STP transition
* @return an STP transition, if any
*/
Optional> findStpTransition(S state);
/**
* @param state the state out of which we ae looking for a transition that matches the event
* @param event the event that triggers the transition
* @return an STP transition, if any
*/
Optional> findEventTransion(S state, E event);
/**
* Provides a handler to be invoked when the machine enters an error state. An error state is reached when an
* error occurs during a transition
*
* @return the error handler
*/
Consumer> getErrorHandler();
/**
* @return the name of this state machine
*/
String getName();
/**
* @return a handler that converts a state into its string representation
*/
Function getStateToString();
/**
* @return a handler that converts a state's string representation into the state itself
*/
Function getStringToState();
/**
* @deprecated
* @return a handler that converts an event into its string representation
*/
Function getEventToString();
/**
* @return a handler that convers an event's string representation into the event itself
*/
Function getStringToEvent();
}