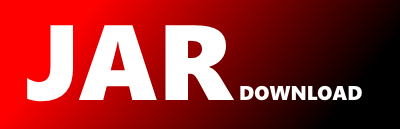
io.strati.functional.LazyTry Maven / Gradle / Ivy
Show all versions of strati-functional Show documentation
/*
* Copyright 2016 WalmartLabs
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.strati.functional;
import io.strati.functional.function.*;
import java.util.function.Function;
/**
* @author WalmartLabs
* @author Georgi Khomeriki [[email protected]]
*/
/**
* This is a lazy version of {@code io.strati.functional.Try} that supports lazy composition
* of potentially failing operations. The same compositional operators that are available for
* {@code Try} are also available for {@code LazyTry}. The key difference is that with
* {@code LazyTry} the operations don't actually get executed until the {@code run()} method
* is invoked. This allows {@code LazyTry} expressions to be chained without actually executing
* them. This is not directly possible with {@code Try} since it evaluates eagerly.
*
* Other than being lazy, all methods have the same semantics on {@code LazyTry} as they have
* on {@code Try}. For more information regarding specific methods please refer to their
* {@code Try} counterparts.
*/
public class LazyTry {
private TrySupplier> supplier;
private LazyTry(final TrySupplier> supplier) {
this.supplier = supplier;
}
public static LazyTry ofTry(final TrySupplier> s) {
return new LazyTry<>(s);
}
public static LazyTry ofFailable(final TrySupplier s) {
return new LazyTry<>(() -> Try.ofFailable(s));
}
public static LazyTry ofFailable(final TryRunnable r) {
return new LazyTry<>(() -> Try.ofFailable(r));
}
public LazyTry ifSuccess(final TryConsumer f) {
return new LazyTry<>(() -> supplier.get().ifSuccess(f));
}
public LazyTry ifSuccess(final TryRunnable r) {
return new LazyTry<>(() -> supplier.get().ifSuccess(r));
}
public LazyTry ifFailure(final TryConsumer c) {
return new LazyTry<>(() -> supplier.get().ifFailure(c));
}
public LazyTry ifFailure(final Class e, final TryConsumer c) {
return new LazyTry<>(() -> supplier.get().ifFailure(e, c));
}
public LazyTry flatMap(final TryFunction super T, ? extends Try extends U>> f) {
return new LazyTry<>(() -> supplier.get().flatMap(t -> f.apply(t)));
}
public LazyTry flatMap(final TrySupplier> f) {
return new LazyTry<>(() -> supplier.get().flatMap(f::get));
}
public LazyTry map(final TryFunction super T, ? extends U> f) {
return new LazyTry<>(() -> supplier.get().map(f));
}
public LazyTry map(final TrySupplier extends U> f) {
return new LazyTry<>(() -> supplier.get().map(f));
}
public LazyTry filter(final TryPredicate super T> predicate) {
return new LazyTry<>(() -> supplier.get().filter(predicate));
}
public LazyTry filter(final TryPredicate super T> predicate, final Throwable t) {
return new LazyTry<>(() -> supplier.get().filter(predicate, t));
}
public LazyTry filter(final TryPredicate super T> predicate, final TryFunction super T, Try extends T>> orElse) {
return new LazyTry<>(() -> supplier.get().filter(predicate, orElse));
}
public LazyTry filter(final TryPredicate super T> predicate, final TrySupplier> orElse) {
return new LazyTry<>(() -> supplier.get().filter(predicate, orElse));
}
public LazyTry recoverWith(final TryFunction> f) {
return new LazyTry<>(() -> supplier.get().recoverWith(f));
}
public LazyTry recoverWith(final Class e, final TryFunction> f) {
return new LazyTry<>(() -> supplier.get().recoverWith(e, f));
}
public LazyTry recover(final TryFunction f) {
return new LazyTry<>(() -> supplier.get().recover(f));
}
public LazyTry recover(final Class e, final TryFunction f) {
return new LazyTry<>(() -> supplier.get().recover(e, f));
}
public Try run() {
try {
return supplier.get();
} catch (final Exception e) {
return Try.failure(e);
}
}
public U apply(final Function, ? extends U> f) {
return f.apply(this);
}
}