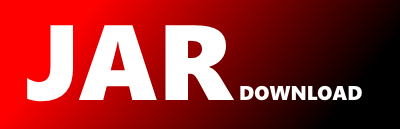
org.bookkeeper.tests.proto.rpc.PingPongServiceGrpc Maven / Gradle / Ivy
package org.bookkeeper.tests.proto.rpc;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.42.1)",
comments = "Source: rpc.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class PingPongServiceGrpc {
private PingPongServiceGrpc() {}
public static final String SERVICE_NAME = "bookkeeper.tests.proto.rpc.PingPongService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getPingPongMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "PingPong",
requestType = org.bookkeeper.tests.proto.rpc.PingRequest.class,
responseType = org.bookkeeper.tests.proto.rpc.PongResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPingPongMethod() {
io.grpc.MethodDescriptor getPingPongMethod;
if ((getPingPongMethod = PingPongServiceGrpc.getPingPongMethod) == null) {
synchronized (PingPongServiceGrpc.class) {
if ((getPingPongMethod = PingPongServiceGrpc.getPingPongMethod) == null) {
PingPongServiceGrpc.getPingPongMethod = getPingPongMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "PingPong"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PongResponse.getDefaultInstance()))
.setSchemaDescriptor(new PingPongServiceMethodDescriptorSupplier("PingPong"))
.build();
}
}
}
return getPingPongMethod;
}
private static volatile io.grpc.MethodDescriptor getLotsOfPingsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "LotsOfPings",
requestType = org.bookkeeper.tests.proto.rpc.PingRequest.class,
responseType = org.bookkeeper.tests.proto.rpc.PongResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
public static io.grpc.MethodDescriptor getLotsOfPingsMethod() {
io.grpc.MethodDescriptor getLotsOfPingsMethod;
if ((getLotsOfPingsMethod = PingPongServiceGrpc.getLotsOfPingsMethod) == null) {
synchronized (PingPongServiceGrpc.class) {
if ((getLotsOfPingsMethod = PingPongServiceGrpc.getLotsOfPingsMethod) == null) {
PingPongServiceGrpc.getLotsOfPingsMethod = getLotsOfPingsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.CLIENT_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "LotsOfPings"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PongResponse.getDefaultInstance()))
.setSchemaDescriptor(new PingPongServiceMethodDescriptorSupplier("LotsOfPings"))
.build();
}
}
}
return getLotsOfPingsMethod;
}
private static volatile io.grpc.MethodDescriptor getLotsOfPongsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "LotsOfPongs",
requestType = org.bookkeeper.tests.proto.rpc.PingRequest.class,
responseType = org.bookkeeper.tests.proto.rpc.PongResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getLotsOfPongsMethod() {
io.grpc.MethodDescriptor getLotsOfPongsMethod;
if ((getLotsOfPongsMethod = PingPongServiceGrpc.getLotsOfPongsMethod) == null) {
synchronized (PingPongServiceGrpc.class) {
if ((getLotsOfPongsMethod = PingPongServiceGrpc.getLotsOfPongsMethod) == null) {
PingPongServiceGrpc.getLotsOfPongsMethod = getLotsOfPongsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "LotsOfPongs"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PongResponse.getDefaultInstance()))
.setSchemaDescriptor(new PingPongServiceMethodDescriptorSupplier("LotsOfPongs"))
.build();
}
}
}
return getLotsOfPongsMethod;
}
private static volatile io.grpc.MethodDescriptor getBidiPingPongMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "BidiPingPong",
requestType = org.bookkeeper.tests.proto.rpc.PingRequest.class,
responseType = org.bookkeeper.tests.proto.rpc.PongResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
public static io.grpc.MethodDescriptor getBidiPingPongMethod() {
io.grpc.MethodDescriptor getBidiPingPongMethod;
if ((getBidiPingPongMethod = PingPongServiceGrpc.getBidiPingPongMethod) == null) {
synchronized (PingPongServiceGrpc.class) {
if ((getBidiPingPongMethod = PingPongServiceGrpc.getBidiPingPongMethod) == null) {
PingPongServiceGrpc.getBidiPingPongMethod = getBidiPingPongMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "BidiPingPong"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.bookkeeper.tests.proto.rpc.PongResponse.getDefaultInstance()))
.setSchemaDescriptor(new PingPongServiceMethodDescriptorSupplier("BidiPingPong"))
.build();
}
}
}
return getBidiPingPongMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static PingPongServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public PingPongServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PingPongServiceStub(channel, callOptions);
}
};
return PingPongServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static PingPongServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public PingPongServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PingPongServiceBlockingStub(channel, callOptions);
}
};
return PingPongServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static PingPongServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public PingPongServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PingPongServiceFutureStub(channel, callOptions);
}
};
return PingPongServiceFutureStub.newStub(factory, channel);
}
/**
*/
public static abstract class PingPongServiceImplBase implements io.grpc.BindableService {
/**
*/
public void pingPong(org.bookkeeper.tests.proto.rpc.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPingPongMethod(), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver lotsOfPings(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall(getLotsOfPingsMethod(), responseObserver);
}
/**
*/
public void lotsOfPongs(org.bookkeeper.tests.proto.rpc.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getLotsOfPongsMethod(), responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver bidiPingPong(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall(getBidiPingPongMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getPingPongMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
org.bookkeeper.tests.proto.rpc.PingRequest,
org.bookkeeper.tests.proto.rpc.PongResponse>(
this, METHODID_PING_PONG)))
.addMethod(
getLotsOfPingsMethod(),
io.grpc.stub.ServerCalls.asyncClientStreamingCall(
new MethodHandlers<
org.bookkeeper.tests.proto.rpc.PingRequest,
org.bookkeeper.tests.proto.rpc.PongResponse>(
this, METHODID_LOTS_OF_PINGS)))
.addMethod(
getLotsOfPongsMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
org.bookkeeper.tests.proto.rpc.PingRequest,
org.bookkeeper.tests.proto.rpc.PongResponse>(
this, METHODID_LOTS_OF_PONGS)))
.addMethod(
getBidiPingPongMethod(),
io.grpc.stub.ServerCalls.asyncBidiStreamingCall(
new MethodHandlers<
org.bookkeeper.tests.proto.rpc.PingRequest,
org.bookkeeper.tests.proto.rpc.PongResponse>(
this, METHODID_BIDI_PING_PONG)))
.build();
}
}
/**
*/
public static final class PingPongServiceStub extends io.grpc.stub.AbstractAsyncStub {
private PingPongServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected PingPongServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PingPongServiceStub(channel, callOptions);
}
/**
*/
public void pingPong(org.bookkeeper.tests.proto.rpc.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPingPongMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver lotsOfPings(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ClientCalls.asyncClientStreamingCall(
getChannel().newCall(getLotsOfPingsMethod(), getCallOptions()), responseObserver);
}
/**
*/
public void lotsOfPongs(org.bookkeeper.tests.proto.rpc.PingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getLotsOfPongsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public io.grpc.stub.StreamObserver bidiPingPong(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ClientCalls.asyncBidiStreamingCall(
getChannel().newCall(getBidiPingPongMethod(), getCallOptions()), responseObserver);
}
}
/**
*/
public static final class PingPongServiceBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private PingPongServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected PingPongServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PingPongServiceBlockingStub(channel, callOptions);
}
/**
*/
public org.bookkeeper.tests.proto.rpc.PongResponse pingPong(org.bookkeeper.tests.proto.rpc.PingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPingPongMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator lotsOfPongs(
org.bookkeeper.tests.proto.rpc.PingRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getLotsOfPongsMethod(), getCallOptions(), request);
}
}
/**
*/
public static final class PingPongServiceFutureStub extends io.grpc.stub.AbstractFutureStub {
private PingPongServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected PingPongServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PingPongServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture pingPong(
org.bookkeeper.tests.proto.rpc.PingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPingPongMethod(), getCallOptions()), request);
}
}
private static final int METHODID_PING_PONG = 0;
private static final int METHODID_LOTS_OF_PONGS = 1;
private static final int METHODID_LOTS_OF_PINGS = 2;
private static final int METHODID_BIDI_PING_PONG = 3;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final PingPongServiceImplBase serviceImpl;
private final int methodId;
MethodHandlers(PingPongServiceImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_PING_PONG:
serviceImpl.pingPong((org.bookkeeper.tests.proto.rpc.PingRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LOTS_OF_PONGS:
serviceImpl.lotsOfPongs((org.bookkeeper.tests.proto.rpc.PingRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_LOTS_OF_PINGS:
return (io.grpc.stub.StreamObserver) serviceImpl.lotsOfPings(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_BIDI_PING_PONG:
return (io.grpc.stub.StreamObserver) serviceImpl.bidiPingPong(
(io.grpc.stub.StreamObserver) responseObserver);
default:
throw new AssertionError();
}
}
}
private static abstract class PingPongServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
PingPongServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return org.bookkeeper.tests.proto.rpc.Rpc.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("PingPongService");
}
}
private static final class PingPongServiceFileDescriptorSupplier
extends PingPongServiceBaseDescriptorSupplier {
PingPongServiceFileDescriptorSupplier() {}
}
private static final class PingPongServiceMethodDescriptorSupplier
extends PingPongServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
PingPongServiceMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (PingPongServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new PingPongServiceFileDescriptorSupplier())
.addMethod(getPingPongMethod())
.addMethod(getLotsOfPingsMethod())
.addMethod(getLotsOfPongsMethod())
.addMethod(getBidiPingPongMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy