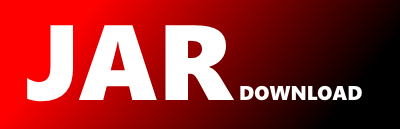
org.apache.pulsar.broker.resources.BaseResources Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.pulsar.broker.resources;
import com.fasterxml.jackson.core.type.TypeReference;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.function.Function;
import lombok.Getter;
import org.apache.pulsar.metadata.api.MetadataCache;
import org.apache.pulsar.metadata.api.MetadataStoreException;
import org.apache.pulsar.metadata.api.extended.MetadataStoreExtended;
/**
* Base class for all configuration resources to access configurations from metadata-store.
*
* @param
* type of configuration-resources.
*/
public class BaseResources {
@Getter
private final MetadataStoreExtended store;
@Getter
private final MetadataCache cache;
private int operationTimeoutSec;
public BaseResources(MetadataStoreExtended store, Class clazz, int operationTimeoutSec) {
this.store = store;
this.cache = store.getMetadataCache(clazz);
this.operationTimeoutSec = operationTimeoutSec;
}
public BaseResources(MetadataStoreExtended store, TypeReference typeRef, int operationTimeoutSec) {
this.store = store;
this.cache = store.getMetadataCache(typeRef);
this.operationTimeoutSec = operationTimeoutSec;
}
public List getChildren(String path) throws MetadataStoreException {
try {
return getChildrenAsync(path).get(operationTimeoutSec, TimeUnit.SECONDS);
} catch (ExecutionException e) {
throw (e.getCause() instanceof MetadataStoreException) ? (MetadataStoreException) e.getCause()
: new MetadataStoreException(e.getCause());
} catch (Exception e) {
throw new MetadataStoreException("Failed to get childeren of " + path, e);
}
}
public CompletableFuture> getChildrenAsync(String path) {
return cache.getChildren(path);
}
public Optional get(String path) throws MetadataStoreException {
try {
return getAsync(path).get(operationTimeoutSec, TimeUnit.SECONDS);
} catch (ExecutionException e) {
throw (e.getCause() instanceof MetadataStoreException) ? (MetadataStoreException) e.getCause()
: new MetadataStoreException(e.getCause());
} catch (Exception e) {
throw new MetadataStoreException("Failed to get data from " + path, e);
}
}
public CompletableFuture> getAsync(String path) {
return cache.get(path);
}
public void set(String path, Function modifyFunction) throws MetadataStoreException {
try {
setAsync(path, modifyFunction).get(operationTimeoutSec, TimeUnit.SECONDS);
} catch (ExecutionException e) {
throw (e.getCause() instanceof MetadataStoreException) ? (MetadataStoreException) e.getCause()
: new MetadataStoreException(e.getCause());
} catch (Exception e) {
throw new MetadataStoreException("Failed to set data for " + path, e);
}
}
public CompletableFuture setAsync(String path, Function modifyFunction) {
return cache.readModifyUpdate(path, modifyFunction).thenApply(__ -> null);
}
public void setWithCreate(String path, Function, T> createFunction) throws MetadataStoreException {
try {
setWithCreateAsync(path, createFunction).get(operationTimeoutSec, TimeUnit.SECONDS);
} catch (ExecutionException e) {
throw (e.getCause() instanceof MetadataStoreException) ? (MetadataStoreException) e.getCause()
: new MetadataStoreException(e.getCause());
} catch (Exception e) {
throw new MetadataStoreException("Failed to set/create " + path, e);
}
}
public CompletableFuture setWithCreateAsync(String path, Function, T> createFunction) {
return cache.readModifyUpdateOrCreate(path, createFunction).thenApply(__ -> null);
}
public void create(String path, T data) throws MetadataStoreException {
try {
createAsync(path, data).get(operationTimeoutSec, TimeUnit.SECONDS);
} catch (ExecutionException e) {
throw (e.getCause() instanceof MetadataStoreException) ? (MetadataStoreException) e.getCause()
: new MetadataStoreException(e.getCause());
} catch (Exception e) {
throw new MetadataStoreException("Failed to create " + path, e);
}
}
public CompletableFuture createAsync(String path, T data) {
return cache.create(path, data);
}
public void delete(String path) throws MetadataStoreException {
try {
deleteAsync(path).get(operationTimeoutSec, TimeUnit.SECONDS);
} catch (ExecutionException e) {
throw (e.getCause() instanceof MetadataStoreException) ? (MetadataStoreException) e.getCause()
: new MetadataStoreException(e.getCause());
} catch (Exception e) {
throw new MetadataStoreException("Failed to delete " + path, e);
}
}
public CompletableFuture deleteAsync(String path) {
return cache.delete(path);
}
public boolean exists(String path) throws MetadataStoreException {
try {
return existsAsync(path).get(operationTimeoutSec, TimeUnit.SECONDS);
} catch (ExecutionException e) {
throw (e.getCause() instanceof MetadataStoreException) ? (MetadataStoreException) e.getCause()
: new MetadataStoreException(e.getCause());
} catch (Exception e) {
throw new MetadataStoreException("Failed to check exist " + path, e);
}
}
public int getOperationTimeoutSec() {
return operationTimeoutSec;
}
public CompletableFuture existsAsync(String path) {
return cache.exists(path);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy