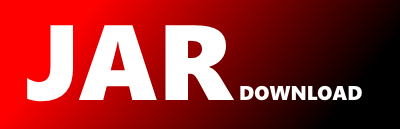
io.streamthoughts.azkarra.api.config.MapConf Maven / Gradle / Ivy
/*
* Copyright 2019-2020 StreamThoughts.
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.streamthoughts.azkarra.api.config;
import io.streamthoughts.azkarra.api.errors.InvalidConfException;
import io.streamthoughts.azkarra.api.errors.MissingConfException;
import io.streamthoughts.azkarra.api.monad.Tuple;
import io.streamthoughts.azkarra.api.util.TypeConverter;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Properties;
import java.util.Set;
import java.util.function.BiFunction;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* A simple {@link Conf} implementation which is backed by a {@link java.util.HashMap}.
*/
public class MapConf extends AbstractConf {
private final Map parameters;
private final Conf fallback;
/**
* Static helper that can be used to creates a new empty {@link MapConf} instance.
*
* @return a new {@link MapConf} instance.
*/
static MapConf empty() {
return new MapConf(Collections.emptyMap());
}
/**
* Static helper that can be used to creates a new single key-pair {@link MapConf} instance.
*
* @return a new {@link MapConf} instance.
*/
static MapConf singletonConf(final String key, final Object value) {
return new MapConf(Collections.singletonMap(key, value));
}
/**
* Creates a new {@link MapConf} instance.
*
* @param parameters the parameters configuration.
*/
MapConf(final Map parameters) {
this(parameters, null, true);
}
/**
* Creates a new {@link MapConf} instance.
*
* @param parameters the parameters configuration.
*/
MapConf(final Map parameters,
final boolean explode) {
this(parameters, null, explode);
}
private MapConf(final Map parameters,
final Conf fallback,
final boolean explode) {
Objects.requireNonNull(parameters, "parameters cannot be null");
this.parameters = explode ? explode(parameters).unwrap() : parameters;
this.fallback = fallback;
}
/**
* {@inheritDoc}
*/
@Override
public Set keySet() {
final HashSet keySet = new HashSet<>(parameters.keySet());
keySet.addAll(fallback.keySet());
return keySet;
}
/**
* {@inheritDoc}
*/
@Override
public Object getValue(final String path) {
return findForPathOrThrow(path, Conf::getValue);
}
/**
* {@inheritDoc}
*/
@Override
public String getString(final String path) {
final Object o = findForPathOrThrow(path, Conf::getString);
try {
return TypeConverter.getString(o);
} catch (final IllegalArgumentException e) {
throw new InvalidConfException(
"Type mismatch for path '" + path + "': " + o.getClass().getSimpleName() + "<> String");
}
}
/**
* {@inheritDoc}
*/
@Override
public long getLong(final String path) {
final Object o = findForPathOrThrow(path, Conf::getLong);
try {
return TypeConverter.getLong(o);
} catch (final IllegalArgumentException e) {
throw new InvalidConfException(
"Type mismatch for path '" + path + "': " + o.getClass().getSimpleName() + "<> Long");
}
}
/**
* {@inheritDoc}
*/
@Override
public int getInt(final String path) {
final Object o = findForPathOrThrow(path, Conf::getInt);
try {
return TypeConverter.getInt(o);
} catch (final IllegalArgumentException e) {
throw new InvalidConfException(
"Type mismatch for path '" + path + "': " + o.getClass().getSimpleName() + "<> Integer");
}
}
/**
* {@inheritDoc}
*/
@Override
public boolean getBoolean(final String path) {
final Object o = findForPathOrThrow(path, Conf::getBoolean);
try {
return TypeConverter.getBool(o);
} catch (final IllegalArgumentException e) {
throw new InvalidConfException(
"Type mismatch for path '" + path + "': " + o.getClass().getSimpleName() + "<> Boolean");
}
}
/**
* {@inheritDoc}
*/
@Override
public double getDouble(final String path) {
final Object o = findForPathOrThrow(path, Conf::getDouble);
try {
return TypeConverter.getDouble(o);
} catch (final IllegalArgumentException e) {
throw new InvalidConfException(
"Type mismatch for path '" + path + "': " + o.getClass().getSimpleName() + "<> Double");
}
}
/**
* {@inheritDoc}
*/
@Override
@SuppressWarnings("unchecked")
public List getStringList(final String path) {
Object o = findForPathOrThrow(path, Conf::getStringList);
try {
return (List) TypeConverter.getList(o);
} catch (final IllegalArgumentException e) {
throw new InvalidConfException(
"Type mismatch for path '" + path + "': " + o.getClass().getSimpleName() + "<> List");
}
}
/**
* {@inheritDoc}
*/
@Override
public Conf getSubConf(final String path) {
Object o = findForPathOrThrow(path, Conf::getSubConf);
Conf conf;
if(o instanceof Map)
conf = Conf.of((Map)o) ;
else if (o instanceof Conf)
conf = (Conf)o;
else {
throw new InvalidConfException(
"Type mismatch for path '" + path + "': " + o.getClass().getSimpleName() + "<> [Map, Conf]");
}
if (fallback != null && fallback.hasPath(path)) {
conf = conf.withFallback(fallback.getSubConf(path));
}
return conf;
}
/**
* {@inheritDoc}
*/
@Override
@SuppressWarnings("unchecked")
public List getSubConfList(final String path) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy