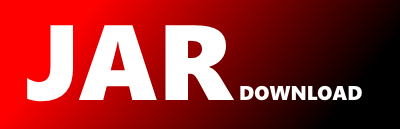
io.streamthoughts.jikkou.extension.aiven.reconciler.AivenSchemaRegistryAclEntryController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jikkou-provider-aiven Show documentation
Show all versions of jikkou-provider-aiven Show documentation
Integration between Aiven for Apache Kafka and Jikkou
The newest version!
/*
* SPDX-License-Identifier: Apache-2.0
* Copyright (c) The original authors
*
* Licensed under the Apache Software License version 2.0, available at http://www.apache.org/licenses/LICENSE-2.0
*/
package io.streamthoughts.jikkou.extension.aiven.reconciler;
import static io.streamthoughts.jikkou.core.ReconciliationMode.CREATE;
import static io.streamthoughts.jikkou.core.ReconciliationMode.DELETE;
import static io.streamthoughts.jikkou.core.ReconciliationMode.FULL;
import io.streamthoughts.jikkou.core.ReconciliationContext;
import io.streamthoughts.jikkou.core.annotation.SupportedResource;
import io.streamthoughts.jikkou.core.config.ConfigProperty;
import io.streamthoughts.jikkou.core.extension.ExtensionContext;
import io.streamthoughts.jikkou.core.models.change.ResourceChange;
import io.streamthoughts.jikkou.core.reconciler.ChangeExecutor;
import io.streamthoughts.jikkou.core.reconciler.ChangeHandler;
import io.streamthoughts.jikkou.core.reconciler.ChangeResult;
import io.streamthoughts.jikkou.core.reconciler.Controller;
import io.streamthoughts.jikkou.core.reconciler.annotations.ControllerConfiguration;
import io.streamthoughts.jikkou.core.selector.Selectors;
import io.streamthoughts.jikkou.extension.aiven.ApiVersions;
import io.streamthoughts.jikkou.extension.aiven.api.AivenApiClient;
import io.streamthoughts.jikkou.extension.aiven.api.AivenApiClientConfig;
import io.streamthoughts.jikkou.extension.aiven.api.AivenApiClientFactory;
import io.streamthoughts.jikkou.extension.aiven.change.schema.SchemaRegistryAclEntryChangeComputer;
import io.streamthoughts.jikkou.extension.aiven.change.schema.SchemaRegistryAclEntryChangeHandler;
import io.streamthoughts.jikkou.extension.aiven.models.V1SchemaRegistryAclEntry;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.atomic.AtomicBoolean;
import org.jetbrains.annotations.NotNull;
@ControllerConfiguration(
supportedModes = {CREATE, DELETE, FULL}
)
@SupportedResource(type = V1SchemaRegistryAclEntry.class)
@SupportedResource(
apiVersion = ApiVersions.KAFKA_AIVEN_V1BETA1,
kind = "SchemaRegistryAclEntryChange"
)
public final class AivenSchemaRegistryAclEntryController implements Controller {
public static final ConfigProperty DELETE_ORPHANS_OPTIONS = ConfigProperty
.ofBoolean("delete-orphans")
.orElse(false);
private final AtomicBoolean initialized = new AtomicBoolean(false);
private AivenApiClientConfig config;
private AivenSchemaRegistryAclEntryCollector collector;
/**
* Creates a new {@link AivenSchemaRegistryAclEntryController} instance.
*/
public AivenSchemaRegistryAclEntryController() {
}
/**
* Creates a new {@link AivenSchemaRegistryAclEntryController} instance.
*
* @param config the schema registry client configuration.
*/
public AivenSchemaRegistryAclEntryController(@NotNull AivenApiClientConfig config) {
init(config);
}
/**
* {@inheritDoc}
**/
@Override
public void init(@NotNull final ExtensionContext context) {
init(new AivenApiClientConfig(context.appConfiguration()));
}
private void init(@NotNull AivenApiClientConfig config) {
if (this.initialized.compareAndSet(false, true)) {
this.config = config;
this.collector = new AivenSchemaRegistryAclEntryCollector(config);
}
}
/**
* {@inheritDoc}
**/
@Override
public List execute(@NotNull ChangeExecutor executor,
@NotNull ReconciliationContext context) {
AivenApiClient api = AivenApiClientFactory.create(config);
try {
List> handlers = List.of(
new SchemaRegistryAclEntryChangeHandler.Create(api),
new SchemaRegistryAclEntryChangeHandler.Delete(api),
new SchemaRegistryAclEntryChangeHandler.None()
);
return executor.applyChanges(handlers);
} finally {
api.close();
}
}
/**
* {@inheritDoc}
**/
@Override
public List plan(
@NotNull Collection resources,
@NotNull ReconciliationContext context) {
// Get existing resources from the environment.
List actualResources = collector.listAll(context.configuration(), Selectors.NO_SELECTOR)
.stream()
.filter(context.selector()::apply)
.toList();
// Get expected resources which are candidates for this reconciliation.
List expectedResources = resources
.stream()
.filter(context.selector()::apply)
.toList();
Boolean deleteOrphans = DELETE_ORPHANS_OPTIONS.get(context.configuration());
SchemaRegistryAclEntryChangeComputer computer = new SchemaRegistryAclEntryChangeComputer(deleteOrphans);
return computer.computeChanges(actualResources, expectedResources);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy