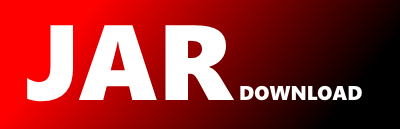
io.streamthoughts.jikkou.kafka.internals.consumer.DefaultConsumerFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jikkou-provider-kafka Show documentation
Show all versions of jikkou-provider-kafka Show documentation
Integration between Apache Kafka and Jikkou
The newest version!
/*
* SPDX-License-Identifier: Apache-2.0
* Copyright (c) The original authors
*
* Licensed under the Apache Software License version 2.0, available at http://www.apache.org/licenses/LICENSE-2.0
*/
package io.streamthoughts.jikkou.kafka.internals.consumer;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import org.apache.kafka.clients.consumer.Consumer;
import org.apache.kafka.clients.consumer.ConsumerConfig;
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.common.serialization.Deserializer;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Default factory to create Consumer instances.
*
* @param the key type.
* @param the value type.
*/
public class DefaultConsumerFactory implements ConsumerFactory {
private static final Logger LOG = LoggerFactory.getLogger(DefaultConsumerFactory.class);
private final Map clientProperties;
private Deserializer keyDeserializer = null;
private Deserializer valueDeserializer = null;
public DefaultConsumerFactory(@NotNull Map clientProperties) {
this(clientProperties, null, null);
}
public DefaultConsumerFactory setKeyDeserializer(Deserializer keyDeserializer) {
this.keyDeserializer = keyDeserializer;
return this;
}
public DefaultConsumerFactory setValueDeserializer(Deserializer valueDeserializer) {
this.valueDeserializer = valueDeserializer;
return this;
}
public DefaultConsumerFactory(@NotNull Map clientProperties,
@Nullable Deserializer keyDeserializer,
@Nullable Deserializer valueDeserializer) {
this.clientProperties = Collections.unmodifiableMap(clientProperties);
this.keyDeserializer = keyDeserializer;
this.valueDeserializer = valueDeserializer;
}
/** {@inheritDoc} **/
@Override
public Consumer createConsumer() {
LOG.debug("Creating consumer");
return new KafkaConsumer<>(clientProperties, keyDeserializer, valueDeserializer);
}
/** {@inheritDoc} **/
@Override
public Consumer createConsumer(String clientId) {
LOG.debug("Creating consumer with client.id={}", clientId);
Map props = new HashMap<>(clientProperties);
props.put(ConsumerConfig.CLIENT_ID_CONFIG, clientId);
return new KafkaConsumer<>(props, keyDeserializer, valueDeserializer);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy