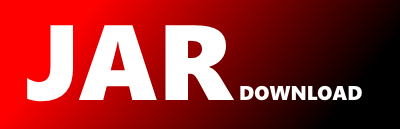
io.streamthoughts.kafka.specs.command.topic.subcommands.internal.DescribeTopics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jikkou Show documentation
Show all versions of jikkou Show documentation
A command-line tool to help you automate the management of the configurations that live on your Apache Kafka clusters.
/*
* Copyright 2020 StreamThoughts.
*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.streamthoughts.kafka.specs.command.topic.subcommands.internal;
import io.streamthoughts.kafka.specs.internal.ConfigsBuilder;
import io.streamthoughts.kafka.specs.operation.DescribeOperationOptions;
import io.streamthoughts.kafka.specs.resources.Configs;
import io.streamthoughts.kafka.specs.model.V1TopicObject;
import org.apache.kafka.clients.admin.AdminClient;
import org.apache.kafka.clients.admin.Config;
import org.apache.kafka.clients.admin.ConfigEntry;
import org.apache.kafka.clients.admin.DescribeConfigsResult;
import org.apache.kafka.clients.admin.DescribeTopicsResult;
import org.apache.kafka.clients.admin.TopicDescription;
import org.apache.kafka.common.TopicPartitionInfo;
import org.apache.kafka.common.config.ConfigResource;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.function.Predicate;
import java.util.stream.Collectors;
/**
* Function to list all topics on Kafka Cluster matching a given predicate.
*/
public final class DescribeTopics {
private final AdminClient client;
private Predicate configEntryPredicate;
/**
* Creates a new {@link DescribeTopics} instance.
*
* @param client the {@link AdminClient}.
* @param options the {@link DescribeOperationOptions}.
*/
public DescribeTopics(final AdminClient client,
final DescribeOperationOptions options) {
this.client = client;
this.configEntryPredicate = entry -> !entry.isDefault() || options.describeDefaultConfigs();
}
public void addConfigEntryPredicate(final Predicate configEntryPredicate) {
this.configEntryPredicate = this.configEntryPredicate.and(configEntryPredicate) ;
}
public List describe(final Predicate topicPredicate) {
final Collection topicNames;
try {
topicNames = client.listTopics().names().get()
.stream()
.filter(topicPredicate)
.collect(Collectors.toList());
} catch (Exception e) {
throw new RuntimeException(e);
}
final CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy