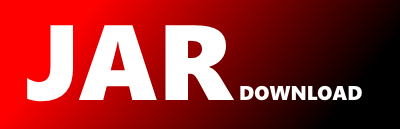
io.strimzi.api.kafka.model.EntityOperatorSpecFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import io.strimzi.api.kafka.model.template.EntityOperatorTemplateBuilder;
import io.strimzi.api.kafka.model.template.EntityOperatorTemplateFluentImpl;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import io.fabric8.kubernetes.api.builder.Predicate;
import java.lang.Deprecated;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.List;
import java.lang.Boolean;
import io.strimzi.api.kafka.model.template.EntityOperatorTemplate;
import io.fabric8.kubernetes.api.model.Toleration;
import java.lang.Long;
import io.fabric8.kubernetes.api.model.Affinity;
import java.util.Collection;
import java.lang.Object;
public class EntityOperatorSpecFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements EntityOperatorSpecFluent{
private EntityTopicOperatorSpecBuilder topicOperator;
private EntityUserOperatorSpecBuilder userOperator;
private Affinity affinity;
private List tolerations;
private TlsSidecarBuilder tlsSidecar;
private EntityOperatorTemplateBuilder template;
public EntityOperatorSpecFluentImpl(){
}
public EntityOperatorSpecFluentImpl(EntityOperatorSpec instance){
this.withTopicOperator(instance.getTopicOperator());
this.withUserOperator(instance.getUserOperator());
this.withAffinity(instance.getAffinity());
this.withTolerations(instance.getTolerations());
this.withTlsSidecar(instance.getTlsSidecar());
this.withTemplate(instance.getTemplate());
}
/**
* This method has been deprecated, please use method buildTopicOperator instead.
* @return The buildable object.
*/
@Deprecated public EntityTopicOperatorSpec getTopicOperator(){
return this.topicOperator!=null?this.topicOperator.build():null;
}
public EntityTopicOperatorSpec buildTopicOperator(){
return this.topicOperator!=null?this.topicOperator.build():null;
}
public A withTopicOperator(EntityTopicOperatorSpec topicOperator){
_visitables.get("topicOperator").remove(this.topicOperator);
if (topicOperator!=null){ this.topicOperator= new EntityTopicOperatorSpecBuilder(topicOperator); _visitables.get("topicOperator").add(this.topicOperator);} return (A) this;
}
public Boolean hasTopicOperator(){
return this.topicOperator != null;
}
public EntityOperatorSpecFluent.TopicOperatorNested withNewTopicOperator(){
return new TopicOperatorNestedImpl();
}
public EntityOperatorSpecFluent.TopicOperatorNested withNewTopicOperatorLike(EntityTopicOperatorSpec item){
return new TopicOperatorNestedImpl(item);
}
public EntityOperatorSpecFluent.TopicOperatorNested editTopicOperator(){
return withNewTopicOperatorLike(getTopicOperator());
}
public EntityOperatorSpecFluent.TopicOperatorNested editOrNewTopicOperator(){
return withNewTopicOperatorLike(getTopicOperator() != null ? getTopicOperator(): new EntityTopicOperatorSpecBuilder().build());
}
public EntityOperatorSpecFluent.TopicOperatorNested editOrNewTopicOperatorLike(EntityTopicOperatorSpec item){
return withNewTopicOperatorLike(getTopicOperator() != null ? getTopicOperator(): item);
}
/**
* This method has been deprecated, please use method buildUserOperator instead.
* @return The buildable object.
*/
@Deprecated public EntityUserOperatorSpec getUserOperator(){
return this.userOperator!=null?this.userOperator.build():null;
}
public EntityUserOperatorSpec buildUserOperator(){
return this.userOperator!=null?this.userOperator.build():null;
}
public A withUserOperator(EntityUserOperatorSpec userOperator){
_visitables.get("userOperator").remove(this.userOperator);
if (userOperator!=null){ this.userOperator= new EntityUserOperatorSpecBuilder(userOperator); _visitables.get("userOperator").add(this.userOperator);} return (A) this;
}
public Boolean hasUserOperator(){
return this.userOperator != null;
}
public EntityOperatorSpecFluent.UserOperatorNested withNewUserOperator(){
return new UserOperatorNestedImpl();
}
public EntityOperatorSpecFluent.UserOperatorNested withNewUserOperatorLike(EntityUserOperatorSpec item){
return new UserOperatorNestedImpl(item);
}
public EntityOperatorSpecFluent.UserOperatorNested editUserOperator(){
return withNewUserOperatorLike(getUserOperator());
}
public EntityOperatorSpecFluent.UserOperatorNested editOrNewUserOperator(){
return withNewUserOperatorLike(getUserOperator() != null ? getUserOperator(): new EntityUserOperatorSpecBuilder().build());
}
public EntityOperatorSpecFluent.UserOperatorNested editOrNewUserOperatorLike(EntityUserOperatorSpec item){
return withNewUserOperatorLike(getUserOperator() != null ? getUserOperator(): item);
}
public Affinity getAffinity(){
return this.affinity;
}
public A withAffinity(Affinity affinity){
this.affinity=affinity; return (A) this;
}
public Boolean hasAffinity(){
return this.affinity != null;
}
public A addToTolerations(int index,Toleration item){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
this.tolerations.add(index, item);
return (A)this;
}
public A setToTolerations(int index,Toleration item){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
this.tolerations.set(index, item); return (A)this;
}
public A addToTolerations(Toleration... items){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
for (Toleration item : items) {this.tolerations.add(item);} return (A)this;
}
public A addAllToTolerations(Collection items){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
for (Toleration item : items) {this.tolerations.add(item);} return (A)this;
}
public A removeFromTolerations(Toleration... items){
for (Toleration item : items) {if (this.tolerations!= null){ this.tolerations.remove(item);}} return (A)this;
}
public A removeAllFromTolerations(Collection items){
for (Toleration item : items) {if (this.tolerations!= null){ this.tolerations.remove(item);}} return (A)this;
}
public List getTolerations(){
return this.tolerations;
}
public Toleration getToleration(int index){
return this.tolerations.get(index);
}
public Toleration getFirstToleration(){
return this.tolerations.get(0);
}
public Toleration getLastToleration(){
return this.tolerations.get(tolerations.size() - 1);
}
public Toleration getMatchingToleration(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (Toleration item: tolerations) { if(predicate.apply(item)){ return item;} } return null;
}
public Boolean hasMatchingToleration(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (Toleration item: tolerations) { if(predicate.apply(item)){ return true;} } return false;
}
public A withTolerations(List tolerations){
if (this.tolerations != null) { _visitables.get("tolerations").removeAll(this.tolerations);}
if (tolerations != null) {this.tolerations = new ArrayList(); for (Toleration item : tolerations){this.addToTolerations(item);}} else { this.tolerations = null;} return (A) this;
}
public A withTolerations(Toleration... tolerations){
if (this.tolerations != null) {this.tolerations.clear();}
if (tolerations != null) {for (Toleration item :tolerations){ this.addToTolerations(item);}} return (A) this;
}
public Boolean hasTolerations(){
return tolerations != null && !tolerations.isEmpty();
}
public A addNewToleration(String effect,String key,String operator,Long tolerationSeconds,String value){
return (A)addToTolerations(new Toleration(effect, key, operator, tolerationSeconds, value));
}
/**
* This method has been deprecated, please use method buildTlsSidecar instead.
* @return The buildable object.
*/
@Deprecated public TlsSidecar getTlsSidecar(){
return this.tlsSidecar!=null?this.tlsSidecar.build():null;
}
public TlsSidecar buildTlsSidecar(){
return this.tlsSidecar!=null?this.tlsSidecar.build():null;
}
public A withTlsSidecar(TlsSidecar tlsSidecar){
_visitables.get("tlsSidecar").remove(this.tlsSidecar);
if (tlsSidecar!=null){ this.tlsSidecar= new TlsSidecarBuilder(tlsSidecar); _visitables.get("tlsSidecar").add(this.tlsSidecar);} return (A) this;
}
public Boolean hasTlsSidecar(){
return this.tlsSidecar != null;
}
public EntityOperatorSpecFluent.TlsSidecarNested withNewTlsSidecar(){
return new TlsSidecarNestedImpl();
}
public EntityOperatorSpecFluent.TlsSidecarNested withNewTlsSidecarLike(TlsSidecar item){
return new TlsSidecarNestedImpl(item);
}
public EntityOperatorSpecFluent.TlsSidecarNested editTlsSidecar(){
return withNewTlsSidecarLike(getTlsSidecar());
}
public EntityOperatorSpecFluent.TlsSidecarNested editOrNewTlsSidecar(){
return withNewTlsSidecarLike(getTlsSidecar() != null ? getTlsSidecar(): new TlsSidecarBuilder().build());
}
public EntityOperatorSpecFluent.TlsSidecarNested editOrNewTlsSidecarLike(TlsSidecar item){
return withNewTlsSidecarLike(getTlsSidecar() != null ? getTlsSidecar(): item);
}
/**
* This method has been deprecated, please use method buildTemplate instead.
* @return The buildable object.
*/
@Deprecated public EntityOperatorTemplate getTemplate(){
return this.template!=null?this.template.build():null;
}
public EntityOperatorTemplate buildTemplate(){
return this.template!=null?this.template.build():null;
}
public A withTemplate(EntityOperatorTemplate template){
_visitables.get("template").remove(this.template);
if (template!=null){ this.template= new EntityOperatorTemplateBuilder(template); _visitables.get("template").add(this.template);} return (A) this;
}
public Boolean hasTemplate(){
return this.template != null;
}
public EntityOperatorSpecFluent.TemplateNested withNewTemplate(){
return new TemplateNestedImpl();
}
public EntityOperatorSpecFluent.TemplateNested withNewTemplateLike(EntityOperatorTemplate item){
return new TemplateNestedImpl(item);
}
public EntityOperatorSpecFluent.TemplateNested editTemplate(){
return withNewTemplateLike(getTemplate());
}
public EntityOperatorSpecFluent.TemplateNested editOrNewTemplate(){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): new EntityOperatorTemplateBuilder().build());
}
public EntityOperatorSpecFluent.TemplateNested editOrNewTemplateLike(EntityOperatorTemplate item){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EntityOperatorSpecFluentImpl that = (EntityOperatorSpecFluentImpl) o;
if (topicOperator != null ? !topicOperator.equals(that.topicOperator) :that.topicOperator != null) return false;
if (userOperator != null ? !userOperator.equals(that.userOperator) :that.userOperator != null) return false;
if (affinity != null ? !affinity.equals(that.affinity) :that.affinity != null) return false;
if (tolerations != null ? !tolerations.equals(that.tolerations) :that.tolerations != null) return false;
if (tlsSidecar != null ? !tlsSidecar.equals(that.tlsSidecar) :that.tlsSidecar != null) return false;
if (template != null ? !template.equals(that.template) :that.template != null) return false;
return true;
}
public class TopicOperatorNestedImpl extends EntityTopicOperatorSpecFluentImpl> implements EntityOperatorSpecFluent.TopicOperatorNested,io.fabric8.kubernetes.api.builder.Nested{
private final EntityTopicOperatorSpecBuilder builder;
TopicOperatorNestedImpl(EntityTopicOperatorSpec item){
this.builder = new EntityTopicOperatorSpecBuilder(this, item);
}
TopicOperatorNestedImpl(){
this.builder = new EntityTopicOperatorSpecBuilder(this);
}
public N and(){
return (N) EntityOperatorSpecFluentImpl.this.withTopicOperator(builder.build());
}
public N endTopicOperator(){
return and();
}
}
public class UserOperatorNestedImpl extends EntityUserOperatorSpecFluentImpl> implements EntityOperatorSpecFluent.UserOperatorNested,io.fabric8.kubernetes.api.builder.Nested{
private final EntityUserOperatorSpecBuilder builder;
UserOperatorNestedImpl(EntityUserOperatorSpec item){
this.builder = new EntityUserOperatorSpecBuilder(this, item);
}
UserOperatorNestedImpl(){
this.builder = new EntityUserOperatorSpecBuilder(this);
}
public N and(){
return (N) EntityOperatorSpecFluentImpl.this.withUserOperator(builder.build());
}
public N endUserOperator(){
return and();
}
}
public class TlsSidecarNestedImpl extends TlsSidecarFluentImpl> implements EntityOperatorSpecFluent.TlsSidecarNested,io.fabric8.kubernetes.api.builder.Nested{
private final TlsSidecarBuilder builder;
TlsSidecarNestedImpl(TlsSidecar item){
this.builder = new TlsSidecarBuilder(this, item);
}
TlsSidecarNestedImpl(){
this.builder = new TlsSidecarBuilder(this);
}
public N and(){
return (N) EntityOperatorSpecFluentImpl.this.withTlsSidecar(builder.build());
}
public N endTlsSidecar(){
return and();
}
}
public class TemplateNestedImpl extends EntityOperatorTemplateFluentImpl> implements EntityOperatorSpecFluent.TemplateNested,io.fabric8.kubernetes.api.builder.Nested{
private final EntityOperatorTemplateBuilder builder;
TemplateNestedImpl(EntityOperatorTemplate item){
this.builder = new EntityOperatorTemplateBuilder(this, item);
}
TemplateNestedImpl(){
this.builder = new EntityOperatorTemplateBuilder(this);
}
public N and(){
return (N) EntityOperatorSpecFluentImpl.this.withTemplate(builder.build());
}
public N endTemplate(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy