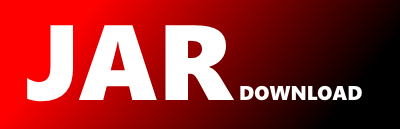
io.strimzi.api.kafka.model.EntityTopicOperatorSpecFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.String;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import java.lang.Deprecated;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Boolean;
import java.lang.StringBuffer;
import java.lang.Object;
public class EntityTopicOperatorSpecFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements EntityTopicOperatorSpecFluent{
private String watchedNamespace;
private String image;
private int reconciliationIntervalSeconds;
private int zookeeperSessionTimeoutSeconds;
private int topicMetadataMaxAttempts;
private ProbeBuilder livenessProbe;
private ProbeBuilder readinessProbe;
private ResourceRequirements resources;
private VisitableBuilder extends Logging,?> logging;
private EntityOperatorJvmOptionsBuilder jvmOptions;
public EntityTopicOperatorSpecFluentImpl(){
}
public EntityTopicOperatorSpecFluentImpl(EntityTopicOperatorSpec instance){
this.withWatchedNamespace(instance.getWatchedNamespace());
this.withImage(instance.getImage());
this.withReconciliationIntervalSeconds(instance.getReconciliationIntervalSeconds());
this.withZookeeperSessionTimeoutSeconds(instance.getZookeeperSessionTimeoutSeconds());
this.withTopicMetadataMaxAttempts(instance.getTopicMetadataMaxAttempts());
this.withLivenessProbe(instance.getLivenessProbe());
this.withReadinessProbe(instance.getReadinessProbe());
this.withResources(instance.getResources());
this.withLogging(instance.getLogging());
this.withJvmOptions(instance.getJvmOptions());
}
public String getWatchedNamespace(){
return this.watchedNamespace;
}
public A withWatchedNamespace(String watchedNamespace){
this.watchedNamespace=watchedNamespace; return (A) this;
}
public Boolean hasWatchedNamespace(){
return this.watchedNamespace != null;
}
public A withNewWatchedNamespace(String arg1){
return (A)withWatchedNamespace(new String(arg1));
}
public A withNewWatchedNamespace(StringBuilder arg1){
return (A)withWatchedNamespace(new String(arg1));
}
public A withNewWatchedNamespace(StringBuffer arg1){
return (A)withWatchedNamespace(new String(arg1));
}
public String getImage(){
return this.image;
}
public A withImage(String image){
this.image=image; return (A) this;
}
public Boolean hasImage(){
return this.image != null;
}
public A withNewImage(String arg1){
return (A)withImage(new String(arg1));
}
public A withNewImage(StringBuilder arg1){
return (A)withImage(new String(arg1));
}
public A withNewImage(StringBuffer arg1){
return (A)withImage(new String(arg1));
}
public int getReconciliationIntervalSeconds(){
return this.reconciliationIntervalSeconds;
}
public A withReconciliationIntervalSeconds(int reconciliationIntervalSeconds){
this.reconciliationIntervalSeconds=reconciliationIntervalSeconds; return (A) this;
}
public Boolean hasReconciliationIntervalSeconds(){
return true;
}
public int getZookeeperSessionTimeoutSeconds(){
return this.zookeeperSessionTimeoutSeconds;
}
public A withZookeeperSessionTimeoutSeconds(int zookeeperSessionTimeoutSeconds){
this.zookeeperSessionTimeoutSeconds=zookeeperSessionTimeoutSeconds; return (A) this;
}
public Boolean hasZookeeperSessionTimeoutSeconds(){
return true;
}
public int getTopicMetadataMaxAttempts(){
return this.topicMetadataMaxAttempts;
}
public A withTopicMetadataMaxAttempts(int topicMetadataMaxAttempts){
this.topicMetadataMaxAttempts=topicMetadataMaxAttempts; return (A) this;
}
public Boolean hasTopicMetadataMaxAttempts(){
return true;
}
/**
* This method has been deprecated, please use method buildLivenessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public Probe buildLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public A withLivenessProbe(Probe livenessProbe){
_visitables.get("livenessProbe").remove(this.livenessProbe);
if (livenessProbe!=null){ this.livenessProbe= new ProbeBuilder(livenessProbe); _visitables.get("livenessProbe").add(this.livenessProbe);} return (A) this;
}
public Boolean hasLivenessProbe(){
return this.livenessProbe != null;
}
public A withNewLivenessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withLivenessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public EntityTopicOperatorSpecFluent.LivenessProbeNested withNewLivenessProbe(){
return new LivenessProbeNestedImpl();
}
public EntityTopicOperatorSpecFluent.LivenessProbeNested withNewLivenessProbeLike(Probe item){
return new LivenessProbeNestedImpl(item);
}
public EntityTopicOperatorSpecFluent.LivenessProbeNested editLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe());
}
public EntityTopicOperatorSpecFluent.LivenessProbeNested editOrNewLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): new ProbeBuilder().build());
}
public EntityTopicOperatorSpecFluent.LivenessProbeNested editOrNewLivenessProbeLike(Probe item){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): item);
}
/**
* This method has been deprecated, please use method buildReadinessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public Probe buildReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public A withReadinessProbe(Probe readinessProbe){
_visitables.get("readinessProbe").remove(this.readinessProbe);
if (readinessProbe!=null){ this.readinessProbe= new ProbeBuilder(readinessProbe); _visitables.get("readinessProbe").add(this.readinessProbe);} return (A) this;
}
public Boolean hasReadinessProbe(){
return this.readinessProbe != null;
}
public A withNewReadinessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withReadinessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public EntityTopicOperatorSpecFluent.ReadinessProbeNested withNewReadinessProbe(){
return new ReadinessProbeNestedImpl();
}
public EntityTopicOperatorSpecFluent.ReadinessProbeNested withNewReadinessProbeLike(Probe item){
return new ReadinessProbeNestedImpl(item);
}
public EntityTopicOperatorSpecFluent.ReadinessProbeNested editReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe());
}
public EntityTopicOperatorSpecFluent.ReadinessProbeNested editOrNewReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): new ProbeBuilder().build());
}
public EntityTopicOperatorSpecFluent.ReadinessProbeNested editOrNewReadinessProbeLike(Probe item){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): item);
}
public ResourceRequirements getResources(){
return this.resources;
}
public A withResources(ResourceRequirements resources){
this.resources=resources; return (A) this;
}
public Boolean hasResources(){
return this.resources != null;
}
/**
* This method has been deprecated, please use method buildLogging instead.
* @return The buildable object.
*/
@Deprecated public Logging getLogging(){
return this.logging!=null?this.logging.build():null;
}
public Logging buildLogging(){
return this.logging!=null?this.logging.build():null;
}
public A withLogging(Logging logging){
if (logging instanceof ExternalLogging){ this.logging= new ExternalLoggingBuilder((ExternalLogging)logging); _visitables.get("logging").add(this.logging);}
if (logging instanceof InlineLogging){ this.logging= new InlineLoggingBuilder((InlineLogging)logging); _visitables.get("logging").add(this.logging);}
return (A) this;
}
public Boolean hasLogging(){
return this.logging != null;
}
public A withExternalLogging(ExternalLogging externalLogging){
_visitables.get("logging").remove(this.logging);
if (externalLogging!=null){ this.logging= new ExternalLoggingBuilder(externalLogging); _visitables.get("logging").add(this.logging);} return (A) this;
}
public EntityTopicOperatorSpecFluent.ExternalLoggingNested withNewExternalLogging(){
return new ExternalLoggingNestedImpl();
}
public EntityTopicOperatorSpecFluent.ExternalLoggingNested withNewExternalLoggingLike(ExternalLogging item){
return new ExternalLoggingNestedImpl(item);
}
public A withInlineLogging(InlineLogging inlineLogging){
_visitables.get("logging").remove(this.logging);
if (inlineLogging!=null){ this.logging= new InlineLoggingBuilder(inlineLogging); _visitables.get("logging").add(this.logging);} return (A) this;
}
public EntityTopicOperatorSpecFluent.InlineLoggingNested withNewInlineLogging(){
return new InlineLoggingNestedImpl();
}
public EntityTopicOperatorSpecFluent.InlineLoggingNested withNewInlineLoggingLike(InlineLogging item){
return new InlineLoggingNestedImpl(item);
}
/**
* This method has been deprecated, please use method buildJvmOptions instead.
* @return The buildable object.
*/
@Deprecated public EntityOperatorJvmOptions getJvmOptions(){
return this.jvmOptions!=null?this.jvmOptions.build():null;
}
public EntityOperatorJvmOptions buildJvmOptions(){
return this.jvmOptions!=null?this.jvmOptions.build():null;
}
public A withJvmOptions(EntityOperatorJvmOptions jvmOptions){
_visitables.get("jvmOptions").remove(this.jvmOptions);
if (jvmOptions!=null){ this.jvmOptions= new EntityOperatorJvmOptionsBuilder(jvmOptions); _visitables.get("jvmOptions").add(this.jvmOptions);} return (A) this;
}
public Boolean hasJvmOptions(){
return this.jvmOptions != null;
}
public EntityTopicOperatorSpecFluent.JvmOptionsNested withNewJvmOptions(){
return new JvmOptionsNestedImpl();
}
public EntityTopicOperatorSpecFluent.JvmOptionsNested withNewJvmOptionsLike(EntityOperatorJvmOptions item){
return new JvmOptionsNestedImpl(item);
}
public EntityTopicOperatorSpecFluent.JvmOptionsNested editJvmOptions(){
return withNewJvmOptionsLike(getJvmOptions());
}
public EntityTopicOperatorSpecFluent.JvmOptionsNested editOrNewJvmOptions(){
return withNewJvmOptionsLike(getJvmOptions() != null ? getJvmOptions(): new EntityOperatorJvmOptionsBuilder().build());
}
public EntityTopicOperatorSpecFluent.JvmOptionsNested editOrNewJvmOptionsLike(EntityOperatorJvmOptions item){
return withNewJvmOptionsLike(getJvmOptions() != null ? getJvmOptions(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EntityTopicOperatorSpecFluentImpl that = (EntityTopicOperatorSpecFluentImpl) o;
if (watchedNamespace != null ? !watchedNamespace.equals(that.watchedNamespace) :that.watchedNamespace != null) return false;
if (image != null ? !image.equals(that.image) :that.image != null) return false;
if (reconciliationIntervalSeconds != that.reconciliationIntervalSeconds) return false;
if (zookeeperSessionTimeoutSeconds != that.zookeeperSessionTimeoutSeconds) return false;
if (topicMetadataMaxAttempts != that.topicMetadataMaxAttempts) return false;
if (livenessProbe != null ? !livenessProbe.equals(that.livenessProbe) :that.livenessProbe != null) return false;
if (readinessProbe != null ? !readinessProbe.equals(that.readinessProbe) :that.readinessProbe != null) return false;
if (resources != null ? !resources.equals(that.resources) :that.resources != null) return false;
if (logging != null ? !logging.equals(that.logging) :that.logging != null) return false;
if (jvmOptions != null ? !jvmOptions.equals(that.jvmOptions) :that.jvmOptions != null) return false;
return true;
}
public class LivenessProbeNestedImpl extends ProbeFluentImpl> implements EntityTopicOperatorSpecFluent.LivenessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
LivenessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
LivenessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) EntityTopicOperatorSpecFluentImpl.this.withLivenessProbe(builder.build());
}
public N endLivenessProbe(){
return and();
}
}
public class ReadinessProbeNestedImpl extends ProbeFluentImpl> implements EntityTopicOperatorSpecFluent.ReadinessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
ReadinessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
ReadinessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) EntityTopicOperatorSpecFluentImpl.this.withReadinessProbe(builder.build());
}
public N endReadinessProbe(){
return and();
}
}
public class ExternalLoggingNestedImpl extends ExternalLoggingFluentImpl> implements EntityTopicOperatorSpecFluent.ExternalLoggingNested,io.fabric8.kubernetes.api.builder.Nested{
private final ExternalLoggingBuilder builder;
ExternalLoggingNestedImpl(ExternalLogging item){
this.builder = new ExternalLoggingBuilder(this, item);
}
ExternalLoggingNestedImpl(){
this.builder = new ExternalLoggingBuilder(this);
}
public N and(){
return (N) EntityTopicOperatorSpecFluentImpl.this.withExternalLogging(builder.build());
}
public N endExternalLogging(){
return and();
}
}
public class InlineLoggingNestedImpl extends InlineLoggingFluentImpl> implements EntityTopicOperatorSpecFluent.InlineLoggingNested,io.fabric8.kubernetes.api.builder.Nested{
private final InlineLoggingBuilder builder;
InlineLoggingNestedImpl(InlineLogging item){
this.builder = new InlineLoggingBuilder(this, item);
}
InlineLoggingNestedImpl(){
this.builder = new InlineLoggingBuilder(this);
}
public N and(){
return (N) EntityTopicOperatorSpecFluentImpl.this.withInlineLogging(builder.build());
}
public N endInlineLogging(){
return and();
}
}
public class JvmOptionsNestedImpl extends EntityOperatorJvmOptionsFluentImpl> implements EntityTopicOperatorSpecFluent.JvmOptionsNested,io.fabric8.kubernetes.api.builder.Nested{
private final EntityOperatorJvmOptionsBuilder builder;
JvmOptionsNestedImpl(EntityOperatorJvmOptions item){
this.builder = new EntityOperatorJvmOptionsBuilder(this, item);
}
JvmOptionsNestedImpl(){
this.builder = new EntityOperatorJvmOptionsBuilder(this);
}
public N and(){
return (N) EntityTopicOperatorSpecFluentImpl.this.withJvmOptions(builder.build());
}
public N endJvmOptions(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy