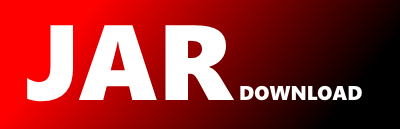
io.strimzi.api.kafka.model.KafkaConnectSpecFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationOAuthBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationScramSha512FluentImpl;
import java.util.ArrayList;
import java.lang.String;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import io.fabric8.kubernetes.api.builder.Predicate;
import java.util.LinkedHashMap;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.List;
import java.lang.Boolean;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationPlainFluentImpl;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationTls;
import io.strimzi.api.kafka.model.connect.ExternalConfigurationBuilder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthentication;
import io.fabric8.kubernetes.api.model.Toleration;
import java.lang.Long;
import io.fabric8.kubernetes.api.model.Affinity;
import java.util.Collection;
import java.lang.Object;
import io.strimzi.api.kafka.model.tracing.Tracing;
import io.strimzi.api.kafka.model.connect.ExternalConfigurationFluentImpl;
import java.util.Map;
import io.strimzi.api.kafka.model.template.KafkaConnectTemplateBuilder;
import io.strimzi.api.kafka.model.tracing.JaegerTracingBuilder;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationScramSha512Builder;
import io.strimzi.api.kafka.model.template.KafkaConnectTemplate;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationOAuth;
import java.lang.Deprecated;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationTlsFluentImpl;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationPlainBuilder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationTlsBuilder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationPlain;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationScramSha512;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationOAuthFluentImpl;
import io.strimzi.api.kafka.model.template.KafkaConnectTemplateFluentImpl;
import io.strimzi.api.kafka.model.tracing.JaegerTracing;
import java.lang.Integer;
import java.lang.StringBuffer;
import io.strimzi.api.kafka.model.connect.ExternalConfiguration;
import io.strimzi.api.kafka.model.tracing.JaegerTracingFluentImpl;
public class KafkaConnectSpecFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements KafkaConnectSpecFluent{
private Map config;
private VisitableBuilder extends Logging,?> logging;
private Integer replicas;
private String version;
private String image;
private ResourceRequirements resources;
private ProbeBuilder livenessProbe;
private ProbeBuilder readinessProbe;
private JvmOptionsBuilder jvmOptions;
private Map metrics;
private VisitableBuilder extends Tracing,?> tracing;
private Affinity affinity;
private List tolerations;
private String bootstrapServers;
private KafkaConnectTlsBuilder tls;
private VisitableBuilder extends KafkaClientAuthentication,?> authentication;
private KafkaConnectTemplateBuilder template;
private ExternalConfigurationBuilder externalConfiguration;
public KafkaConnectSpecFluentImpl(){
}
public KafkaConnectSpecFluentImpl(KafkaConnectSpec instance){
this.withConfig(instance.getConfig());
this.withLogging(instance.getLogging());
this.withReplicas(instance.getReplicas());
this.withVersion(instance.getVersion());
this.withImage(instance.getImage());
this.withResources(instance.getResources());
this.withLivenessProbe(instance.getLivenessProbe());
this.withReadinessProbe(instance.getReadinessProbe());
this.withJvmOptions(instance.getJvmOptions());
this.withMetrics(instance.getMetrics());
this.withTracing(instance.getTracing());
this.withAffinity(instance.getAffinity());
this.withTolerations(instance.getTolerations());
this.withBootstrapServers(instance.getBootstrapServers());
this.withTls(instance.getTls());
this.withAuthentication(instance.getAuthentication());
this.withTemplate(instance.getTemplate());
this.withExternalConfiguration(instance.getExternalConfiguration());
}
public A addToConfig(String key,Object value){
if(this.config == null && key != null && value != null) { this.config = new LinkedHashMap(); }
if(key != null && value != null) {this.config.put(key, value);} return (A)this;
}
public A addToConfig(Map map){
if(this.config == null && map != null) { this.config = new LinkedHashMap(); }
if(map != null) { this.config.putAll(map);} return (A)this;
}
public A removeFromConfig(String key){
if(this.config == null) { return (A) this; }
if(key != null && this.config != null) {this.config.remove(key);} return (A)this;
}
public A removeFromConfig(Map map){
if(this.config == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.config != null){this.config.remove(key);}}} return (A)this;
}
public Map getConfig(){
return this.config;
}
public A withConfig(Map config){
if (config == null) { this.config = null;} else {this.config = new LinkedHashMap(config);} return (A) this;
}
public Boolean hasConfig(){
return this.config != null;
}
/**
* This method has been deprecated, please use method buildLogging instead.
* @return The buildable object.
*/
@Deprecated public Logging getLogging(){
return this.logging!=null?this.logging.build():null;
}
public Logging buildLogging(){
return this.logging!=null?this.logging.build():null;
}
public A withLogging(Logging logging){
if (logging instanceof ExternalLogging){ this.logging= new ExternalLoggingBuilder((ExternalLogging)logging); _visitables.get("logging").add(this.logging);}
if (logging instanceof InlineLogging){ this.logging= new InlineLoggingBuilder((InlineLogging)logging); _visitables.get("logging").add(this.logging);}
return (A) this;
}
public Boolean hasLogging(){
return this.logging != null;
}
public A withExternalLogging(ExternalLogging externalLogging){
_visitables.get("logging").remove(this.logging);
if (externalLogging!=null){ this.logging= new ExternalLoggingBuilder(externalLogging); _visitables.get("logging").add(this.logging);} return (A) this;
}
public KafkaConnectSpecFluent.ExternalLoggingNested withNewExternalLogging(){
return new ExternalLoggingNestedImpl();
}
public KafkaConnectSpecFluent.ExternalLoggingNested withNewExternalLoggingLike(ExternalLogging item){
return new ExternalLoggingNestedImpl(item);
}
public A withInlineLogging(InlineLogging inlineLogging){
_visitables.get("logging").remove(this.logging);
if (inlineLogging!=null){ this.logging= new InlineLoggingBuilder(inlineLogging); _visitables.get("logging").add(this.logging);} return (A) this;
}
public KafkaConnectSpecFluent.InlineLoggingNested withNewInlineLogging(){
return new InlineLoggingNestedImpl();
}
public KafkaConnectSpecFluent.InlineLoggingNested withNewInlineLoggingLike(InlineLogging item){
return new InlineLoggingNestedImpl(item);
}
public Integer getReplicas(){
return this.replicas;
}
public A withReplicas(Integer replicas){
this.replicas=replicas; return (A) this;
}
public Boolean hasReplicas(){
return this.replicas != null;
}
public String getVersion(){
return this.version;
}
public A withVersion(String version){
this.version=version; return (A) this;
}
public Boolean hasVersion(){
return this.version != null;
}
public A withNewVersion(String arg1){
return (A)withVersion(new String(arg1));
}
public A withNewVersion(StringBuilder arg1){
return (A)withVersion(new String(arg1));
}
public A withNewVersion(StringBuffer arg1){
return (A)withVersion(new String(arg1));
}
public String getImage(){
return this.image;
}
public A withImage(String image){
this.image=image; return (A) this;
}
public Boolean hasImage(){
return this.image != null;
}
public A withNewImage(String arg1){
return (A)withImage(new String(arg1));
}
public A withNewImage(StringBuilder arg1){
return (A)withImage(new String(arg1));
}
public A withNewImage(StringBuffer arg1){
return (A)withImage(new String(arg1));
}
public ResourceRequirements getResources(){
return this.resources;
}
public A withResources(ResourceRequirements resources){
this.resources=resources; return (A) this;
}
public Boolean hasResources(){
return this.resources != null;
}
/**
* This method has been deprecated, please use method buildLivenessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public Probe buildLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public A withLivenessProbe(Probe livenessProbe){
_visitables.get("livenessProbe").remove(this.livenessProbe);
if (livenessProbe!=null){ this.livenessProbe= new ProbeBuilder(livenessProbe); _visitables.get("livenessProbe").add(this.livenessProbe);} return (A) this;
}
public Boolean hasLivenessProbe(){
return this.livenessProbe != null;
}
public A withNewLivenessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withLivenessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public KafkaConnectSpecFluent.LivenessProbeNested withNewLivenessProbe(){
return new LivenessProbeNestedImpl();
}
public KafkaConnectSpecFluent.LivenessProbeNested withNewLivenessProbeLike(Probe item){
return new LivenessProbeNestedImpl(item);
}
public KafkaConnectSpecFluent.LivenessProbeNested editLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe());
}
public KafkaConnectSpecFluent.LivenessProbeNested editOrNewLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): new ProbeBuilder().build());
}
public KafkaConnectSpecFluent.LivenessProbeNested editOrNewLivenessProbeLike(Probe item){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): item);
}
/**
* This method has been deprecated, please use method buildReadinessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public Probe buildReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public A withReadinessProbe(Probe readinessProbe){
_visitables.get("readinessProbe").remove(this.readinessProbe);
if (readinessProbe!=null){ this.readinessProbe= new ProbeBuilder(readinessProbe); _visitables.get("readinessProbe").add(this.readinessProbe);} return (A) this;
}
public Boolean hasReadinessProbe(){
return this.readinessProbe != null;
}
public A withNewReadinessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withReadinessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public KafkaConnectSpecFluent.ReadinessProbeNested withNewReadinessProbe(){
return new ReadinessProbeNestedImpl();
}
public KafkaConnectSpecFluent.ReadinessProbeNested withNewReadinessProbeLike(Probe item){
return new ReadinessProbeNestedImpl(item);
}
public KafkaConnectSpecFluent.ReadinessProbeNested editReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe());
}
public KafkaConnectSpecFluent.ReadinessProbeNested editOrNewReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): new ProbeBuilder().build());
}
public KafkaConnectSpecFluent.ReadinessProbeNested editOrNewReadinessProbeLike(Probe item){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): item);
}
/**
* This method has been deprecated, please use method buildJvmOptions instead.
* @return The buildable object.
*/
@Deprecated public JvmOptions getJvmOptions(){
return this.jvmOptions!=null?this.jvmOptions.build():null;
}
public JvmOptions buildJvmOptions(){
return this.jvmOptions!=null?this.jvmOptions.build():null;
}
public A withJvmOptions(JvmOptions jvmOptions){
_visitables.get("jvmOptions").remove(this.jvmOptions);
if (jvmOptions!=null){ this.jvmOptions= new JvmOptionsBuilder(jvmOptions); _visitables.get("jvmOptions").add(this.jvmOptions);} return (A) this;
}
public Boolean hasJvmOptions(){
return this.jvmOptions != null;
}
public KafkaConnectSpecFluent.JvmOptionsNested withNewJvmOptions(){
return new JvmOptionsNestedImpl();
}
public KafkaConnectSpecFluent.JvmOptionsNested withNewJvmOptionsLike(JvmOptions item){
return new JvmOptionsNestedImpl(item);
}
public KafkaConnectSpecFluent.JvmOptionsNested editJvmOptions(){
return withNewJvmOptionsLike(getJvmOptions());
}
public KafkaConnectSpecFluent.JvmOptionsNested editOrNewJvmOptions(){
return withNewJvmOptionsLike(getJvmOptions() != null ? getJvmOptions(): new JvmOptionsBuilder().build());
}
public KafkaConnectSpecFluent.JvmOptionsNested editOrNewJvmOptionsLike(JvmOptions item){
return withNewJvmOptionsLike(getJvmOptions() != null ? getJvmOptions(): item);
}
public A addToMetrics(String key,Object value){
if(this.metrics == null && key != null && value != null) { this.metrics = new LinkedHashMap(); }
if(key != null && value != null) {this.metrics.put(key, value);} return (A)this;
}
public A addToMetrics(Map map){
if(this.metrics == null && map != null) { this.metrics = new LinkedHashMap(); }
if(map != null) { this.metrics.putAll(map);} return (A)this;
}
public A removeFromMetrics(String key){
if(this.metrics == null) { return (A) this; }
if(key != null && this.metrics != null) {this.metrics.remove(key);} return (A)this;
}
public A removeFromMetrics(Map map){
if(this.metrics == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.metrics != null){this.metrics.remove(key);}}} return (A)this;
}
public Map getMetrics(){
return this.metrics;
}
public A withMetrics(Map metrics){
if (metrics == null) { this.metrics = null;} else {this.metrics = new LinkedHashMap(metrics);} return (A) this;
}
public Boolean hasMetrics(){
return this.metrics != null;
}
/**
* This method has been deprecated, please use method buildTracing instead.
* @return The buildable object.
*/
@Deprecated public Tracing getTracing(){
return this.tracing!=null?this.tracing.build():null;
}
public Tracing buildTracing(){
return this.tracing!=null?this.tracing.build():null;
}
public A withTracing(Tracing tracing){
if (tracing instanceof JaegerTracing){ this.tracing= new JaegerTracingBuilder((JaegerTracing)tracing); _visitables.get("tracing").add(this.tracing);}
return (A) this;
}
public Boolean hasTracing(){
return this.tracing != null;
}
public A withJaegerTracing(JaegerTracing jaegerTracing){
_visitables.get("tracing").remove(this.tracing);
if (jaegerTracing!=null){ this.tracing= new JaegerTracingBuilder(jaegerTracing); _visitables.get("tracing").add(this.tracing);} return (A) this;
}
public KafkaConnectSpecFluent.JaegerTracingNested withNewJaegerTracing(){
return new JaegerTracingNestedImpl();
}
public KafkaConnectSpecFluent.JaegerTracingNested withNewJaegerTracingLike(JaegerTracing item){
return new JaegerTracingNestedImpl(item);
}
public Affinity getAffinity(){
return this.affinity;
}
public A withAffinity(Affinity affinity){
this.affinity=affinity; return (A) this;
}
public Boolean hasAffinity(){
return this.affinity != null;
}
public A addToTolerations(int index,Toleration item){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
this.tolerations.add(index, item);
return (A)this;
}
public A setToTolerations(int index,Toleration item){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
this.tolerations.set(index, item); return (A)this;
}
public A addToTolerations(Toleration... items){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
for (Toleration item : items) {this.tolerations.add(item);} return (A)this;
}
public A addAllToTolerations(Collection items){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
for (Toleration item : items) {this.tolerations.add(item);} return (A)this;
}
public A removeFromTolerations(Toleration... items){
for (Toleration item : items) {if (this.tolerations!= null){ this.tolerations.remove(item);}} return (A)this;
}
public A removeAllFromTolerations(Collection items){
for (Toleration item : items) {if (this.tolerations!= null){ this.tolerations.remove(item);}} return (A)this;
}
public List getTolerations(){
return this.tolerations;
}
public Toleration getToleration(int index){
return this.tolerations.get(index);
}
public Toleration getFirstToleration(){
return this.tolerations.get(0);
}
public Toleration getLastToleration(){
return this.tolerations.get(tolerations.size() - 1);
}
public Toleration getMatchingToleration(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (Toleration item: tolerations) { if(predicate.apply(item)){ return item;} } return null;
}
public Boolean hasMatchingToleration(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (Toleration item: tolerations) { if(predicate.apply(item)){ return true;} } return false;
}
public A withTolerations(List tolerations){
if (this.tolerations != null) { _visitables.get("tolerations").removeAll(this.tolerations);}
if (tolerations != null) {this.tolerations = new ArrayList(); for (Toleration item : tolerations){this.addToTolerations(item);}} else { this.tolerations = null;} return (A) this;
}
public A withTolerations(Toleration... tolerations){
if (this.tolerations != null) {this.tolerations.clear();}
if (tolerations != null) {for (Toleration item :tolerations){ this.addToTolerations(item);}} return (A) this;
}
public Boolean hasTolerations(){
return tolerations != null && !tolerations.isEmpty();
}
public A addNewToleration(String effect,String key,String operator,Long tolerationSeconds,String value){
return (A)addToTolerations(new Toleration(effect, key, operator, tolerationSeconds, value));
}
public String getBootstrapServers(){
return this.bootstrapServers;
}
public A withBootstrapServers(String bootstrapServers){
this.bootstrapServers=bootstrapServers; return (A) this;
}
public Boolean hasBootstrapServers(){
return this.bootstrapServers != null;
}
public A withNewBootstrapServers(String arg1){
return (A)withBootstrapServers(new String(arg1));
}
public A withNewBootstrapServers(StringBuilder arg1){
return (A)withBootstrapServers(new String(arg1));
}
public A withNewBootstrapServers(StringBuffer arg1){
return (A)withBootstrapServers(new String(arg1));
}
/**
* This method has been deprecated, please use method buildTls instead.
* @return The buildable object.
*/
@Deprecated public KafkaConnectTls getTls(){
return this.tls!=null?this.tls.build():null;
}
public KafkaConnectTls buildTls(){
return this.tls!=null?this.tls.build():null;
}
public A withTls(KafkaConnectTls tls){
_visitables.get("tls").remove(this.tls);
if (tls!=null){ this.tls= new KafkaConnectTlsBuilder(tls); _visitables.get("tls").add(this.tls);} return (A) this;
}
public Boolean hasTls(){
return this.tls != null;
}
public KafkaConnectSpecFluent.TlsNested withNewTls(){
return new TlsNestedImpl();
}
public KafkaConnectSpecFluent.TlsNested withNewTlsLike(KafkaConnectTls item){
return new TlsNestedImpl(item);
}
public KafkaConnectSpecFluent.TlsNested editTls(){
return withNewTlsLike(getTls());
}
public KafkaConnectSpecFluent.TlsNested editOrNewTls(){
return withNewTlsLike(getTls() != null ? getTls(): new KafkaConnectTlsBuilder().build());
}
public KafkaConnectSpecFluent.TlsNested editOrNewTlsLike(KafkaConnectTls item){
return withNewTlsLike(getTls() != null ? getTls(): item);
}
/**
* This method has been deprecated, please use method buildAuthentication instead.
* @return The buildable object.
*/
@Deprecated public KafkaClientAuthentication getAuthentication(){
return this.authentication!=null?this.authentication.build():null;
}
public KafkaClientAuthentication buildAuthentication(){
return this.authentication!=null?this.authentication.build():null;
}
public A withAuthentication(KafkaClientAuthentication authentication){
if (authentication instanceof KafkaClientAuthenticationScramSha512){ this.authentication= new KafkaClientAuthenticationScramSha512Builder((KafkaClientAuthenticationScramSha512)authentication); _visitables.get("authentication").add(this.authentication);}
if (authentication instanceof KafkaClientAuthenticationPlain){ this.authentication= new KafkaClientAuthenticationPlainBuilder((KafkaClientAuthenticationPlain)authentication); _visitables.get("authentication").add(this.authentication);}
if (authentication instanceof KafkaClientAuthenticationOAuth){ this.authentication= new KafkaClientAuthenticationOAuthBuilder((KafkaClientAuthenticationOAuth)authentication); _visitables.get("authentication").add(this.authentication);}
if (authentication instanceof KafkaClientAuthenticationTls){ this.authentication= new KafkaClientAuthenticationTlsBuilder((KafkaClientAuthenticationTls)authentication); _visitables.get("authentication").add(this.authentication);}
return (A) this;
}
public Boolean hasAuthentication(){
return this.authentication != null;
}
public A withKafkaClientAuthenticationScramSha512(KafkaClientAuthenticationScramSha512 kafkaClientAuthenticationScramSha512){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationScramSha512!=null){ this.authentication= new KafkaClientAuthenticationScramSha512Builder(kafkaClientAuthenticationScramSha512); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationScramSha512Nested withNewKafkaClientAuthenticationScramSha512(){
return new KafkaClientAuthenticationScramSha512NestedImpl();
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationScramSha512Nested withNewKafkaClientAuthenticationScramSha512Like(KafkaClientAuthenticationScramSha512 item){
return new KafkaClientAuthenticationScramSha512NestedImpl(item);
}
public A withKafkaClientAuthenticationPlain(KafkaClientAuthenticationPlain kafkaClientAuthenticationPlain){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationPlain!=null){ this.authentication= new KafkaClientAuthenticationPlainBuilder(kafkaClientAuthenticationPlain); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationPlainNested withNewKafkaClientAuthenticationPlain(){
return new KafkaClientAuthenticationPlainNestedImpl();
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationPlainNested withNewKafkaClientAuthenticationPlainLike(KafkaClientAuthenticationPlain item){
return new KafkaClientAuthenticationPlainNestedImpl(item);
}
public A withKafkaClientAuthenticationOAuth(KafkaClientAuthenticationOAuth kafkaClientAuthenticationOAuth){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationOAuth!=null){ this.authentication= new KafkaClientAuthenticationOAuthBuilder(kafkaClientAuthenticationOAuth); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationOAuthNested withNewKafkaClientAuthenticationOAuth(){
return new KafkaClientAuthenticationOAuthNestedImpl();
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationOAuthNested withNewKafkaClientAuthenticationOAuthLike(KafkaClientAuthenticationOAuth item){
return new KafkaClientAuthenticationOAuthNestedImpl(item);
}
public A withKafkaClientAuthenticationTls(KafkaClientAuthenticationTls kafkaClientAuthenticationTls){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationTls!=null){ this.authentication= new KafkaClientAuthenticationTlsBuilder(kafkaClientAuthenticationTls); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationTlsNested withNewKafkaClientAuthenticationTls(){
return new KafkaClientAuthenticationTlsNestedImpl();
}
public KafkaConnectSpecFluent.KafkaClientAuthenticationTlsNested withNewKafkaClientAuthenticationTlsLike(KafkaClientAuthenticationTls item){
return new KafkaClientAuthenticationTlsNestedImpl(item);
}
/**
* This method has been deprecated, please use method buildTemplate instead.
* @return The buildable object.
*/
@Deprecated public KafkaConnectTemplate getTemplate(){
return this.template!=null?this.template.build():null;
}
public KafkaConnectTemplate buildTemplate(){
return this.template!=null?this.template.build():null;
}
public A withTemplate(KafkaConnectTemplate template){
_visitables.get("template").remove(this.template);
if (template!=null){ this.template= new KafkaConnectTemplateBuilder(template); _visitables.get("template").add(this.template);} return (A) this;
}
public Boolean hasTemplate(){
return this.template != null;
}
public KafkaConnectSpecFluent.TemplateNested withNewTemplate(){
return new TemplateNestedImpl();
}
public KafkaConnectSpecFluent.TemplateNested withNewTemplateLike(KafkaConnectTemplate item){
return new TemplateNestedImpl(item);
}
public KafkaConnectSpecFluent.TemplateNested editTemplate(){
return withNewTemplateLike(getTemplate());
}
public KafkaConnectSpecFluent.TemplateNested editOrNewTemplate(){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): new KafkaConnectTemplateBuilder().build());
}
public KafkaConnectSpecFluent.TemplateNested editOrNewTemplateLike(KafkaConnectTemplate item){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): item);
}
/**
* This method has been deprecated, please use method buildExternalConfiguration instead.
* @return The buildable object.
*/
@Deprecated public ExternalConfiguration getExternalConfiguration(){
return this.externalConfiguration!=null?this.externalConfiguration.build():null;
}
public ExternalConfiguration buildExternalConfiguration(){
return this.externalConfiguration!=null?this.externalConfiguration.build():null;
}
public A withExternalConfiguration(ExternalConfiguration externalConfiguration){
_visitables.get("externalConfiguration").remove(this.externalConfiguration);
if (externalConfiguration!=null){ this.externalConfiguration= new ExternalConfigurationBuilder(externalConfiguration); _visitables.get("externalConfiguration").add(this.externalConfiguration);} return (A) this;
}
public Boolean hasExternalConfiguration(){
return this.externalConfiguration != null;
}
public KafkaConnectSpecFluent.ExternalConfigurationNested withNewExternalConfiguration(){
return new ExternalConfigurationNestedImpl();
}
public KafkaConnectSpecFluent.ExternalConfigurationNested withNewExternalConfigurationLike(ExternalConfiguration item){
return new ExternalConfigurationNestedImpl(item);
}
public KafkaConnectSpecFluent.ExternalConfigurationNested editExternalConfiguration(){
return withNewExternalConfigurationLike(getExternalConfiguration());
}
public KafkaConnectSpecFluent.ExternalConfigurationNested editOrNewExternalConfiguration(){
return withNewExternalConfigurationLike(getExternalConfiguration() != null ? getExternalConfiguration(): new ExternalConfigurationBuilder().build());
}
public KafkaConnectSpecFluent.ExternalConfigurationNested editOrNewExternalConfigurationLike(ExternalConfiguration item){
return withNewExternalConfigurationLike(getExternalConfiguration() != null ? getExternalConfiguration(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
KafkaConnectSpecFluentImpl that = (KafkaConnectSpecFluentImpl) o;
if (config != null ? !config.equals(that.config) :that.config != null) return false;
if (logging != null ? !logging.equals(that.logging) :that.logging != null) return false;
if (replicas != null ? !replicas.equals(that.replicas) :that.replicas != null) return false;
if (version != null ? !version.equals(that.version) :that.version != null) return false;
if (image != null ? !image.equals(that.image) :that.image != null) return false;
if (resources != null ? !resources.equals(that.resources) :that.resources != null) return false;
if (livenessProbe != null ? !livenessProbe.equals(that.livenessProbe) :that.livenessProbe != null) return false;
if (readinessProbe != null ? !readinessProbe.equals(that.readinessProbe) :that.readinessProbe != null) return false;
if (jvmOptions != null ? !jvmOptions.equals(that.jvmOptions) :that.jvmOptions != null) return false;
if (metrics != null ? !metrics.equals(that.metrics) :that.metrics != null) return false;
if (tracing != null ? !tracing.equals(that.tracing) :that.tracing != null) return false;
if (affinity != null ? !affinity.equals(that.affinity) :that.affinity != null) return false;
if (tolerations != null ? !tolerations.equals(that.tolerations) :that.tolerations != null) return false;
if (bootstrapServers != null ? !bootstrapServers.equals(that.bootstrapServers) :that.bootstrapServers != null) return false;
if (tls != null ? !tls.equals(that.tls) :that.tls != null) return false;
if (authentication != null ? !authentication.equals(that.authentication) :that.authentication != null) return false;
if (template != null ? !template.equals(that.template) :that.template != null) return false;
if (externalConfiguration != null ? !externalConfiguration.equals(that.externalConfiguration) :that.externalConfiguration != null) return false;
return true;
}
public class ExternalLoggingNestedImpl extends ExternalLoggingFluentImpl> implements KafkaConnectSpecFluent.ExternalLoggingNested,io.fabric8.kubernetes.api.builder.Nested{
private final ExternalLoggingBuilder builder;
ExternalLoggingNestedImpl(ExternalLogging item){
this.builder = new ExternalLoggingBuilder(this, item);
}
ExternalLoggingNestedImpl(){
this.builder = new ExternalLoggingBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withExternalLogging(builder.build());
}
public N endExternalLogging(){
return and();
}
}
public class InlineLoggingNestedImpl extends InlineLoggingFluentImpl> implements KafkaConnectSpecFluent.InlineLoggingNested,io.fabric8.kubernetes.api.builder.Nested{
private final InlineLoggingBuilder builder;
InlineLoggingNestedImpl(InlineLogging item){
this.builder = new InlineLoggingBuilder(this, item);
}
InlineLoggingNestedImpl(){
this.builder = new InlineLoggingBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withInlineLogging(builder.build());
}
public N endInlineLogging(){
return and();
}
}
public class LivenessProbeNestedImpl extends ProbeFluentImpl> implements KafkaConnectSpecFluent.LivenessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
LivenessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
LivenessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withLivenessProbe(builder.build());
}
public N endLivenessProbe(){
return and();
}
}
public class ReadinessProbeNestedImpl extends ProbeFluentImpl> implements KafkaConnectSpecFluent.ReadinessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
ReadinessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
ReadinessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withReadinessProbe(builder.build());
}
public N endReadinessProbe(){
return and();
}
}
public class JvmOptionsNestedImpl extends JvmOptionsFluentImpl> implements KafkaConnectSpecFluent.JvmOptionsNested,io.fabric8.kubernetes.api.builder.Nested{
private final JvmOptionsBuilder builder;
JvmOptionsNestedImpl(JvmOptions item){
this.builder = new JvmOptionsBuilder(this, item);
}
JvmOptionsNestedImpl(){
this.builder = new JvmOptionsBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withJvmOptions(builder.build());
}
public N endJvmOptions(){
return and();
}
}
public class JaegerTracingNestedImpl extends JaegerTracingFluentImpl> implements KafkaConnectSpecFluent.JaegerTracingNested,io.fabric8.kubernetes.api.builder.Nested{
private final JaegerTracingBuilder builder;
JaegerTracingNestedImpl(JaegerTracing item){
this.builder = new JaegerTracingBuilder(this, item);
}
JaegerTracingNestedImpl(){
this.builder = new JaegerTracingBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withJaegerTracing(builder.build());
}
public N endJaegerTracing(){
return and();
}
}
public class TlsNestedImpl extends KafkaConnectTlsFluentImpl> implements KafkaConnectSpecFluent.TlsNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaConnectTlsBuilder builder;
TlsNestedImpl(KafkaConnectTls item){
this.builder = new KafkaConnectTlsBuilder(this, item);
}
TlsNestedImpl(){
this.builder = new KafkaConnectTlsBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withTls(builder.build());
}
public N endTls(){
return and();
}
}
public class KafkaClientAuthenticationScramSha512NestedImpl extends KafkaClientAuthenticationScramSha512FluentImpl> implements KafkaConnectSpecFluent.KafkaClientAuthenticationScramSha512Nested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationScramSha512Builder builder;
KafkaClientAuthenticationScramSha512NestedImpl(KafkaClientAuthenticationScramSha512 item){
this.builder = new KafkaClientAuthenticationScramSha512Builder(this, item);
}
KafkaClientAuthenticationScramSha512NestedImpl(){
this.builder = new KafkaClientAuthenticationScramSha512Builder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withKafkaClientAuthenticationScramSha512(builder.build());
}
public N endKafkaClientAuthenticationScramSha512(){
return and();
}
}
public class KafkaClientAuthenticationPlainNestedImpl extends KafkaClientAuthenticationPlainFluentImpl> implements KafkaConnectSpecFluent.KafkaClientAuthenticationPlainNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationPlainBuilder builder;
KafkaClientAuthenticationPlainNestedImpl(KafkaClientAuthenticationPlain item){
this.builder = new KafkaClientAuthenticationPlainBuilder(this, item);
}
KafkaClientAuthenticationPlainNestedImpl(){
this.builder = new KafkaClientAuthenticationPlainBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withKafkaClientAuthenticationPlain(builder.build());
}
public N endKafkaClientAuthenticationPlain(){
return and();
}
}
public class KafkaClientAuthenticationOAuthNestedImpl extends KafkaClientAuthenticationOAuthFluentImpl> implements KafkaConnectSpecFluent.KafkaClientAuthenticationOAuthNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationOAuthBuilder builder;
KafkaClientAuthenticationOAuthNestedImpl(KafkaClientAuthenticationOAuth item){
this.builder = new KafkaClientAuthenticationOAuthBuilder(this, item);
}
KafkaClientAuthenticationOAuthNestedImpl(){
this.builder = new KafkaClientAuthenticationOAuthBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withKafkaClientAuthenticationOAuth(builder.build());
}
public N endKafkaClientAuthenticationOAuth(){
return and();
}
}
public class KafkaClientAuthenticationTlsNestedImpl extends KafkaClientAuthenticationTlsFluentImpl> implements KafkaConnectSpecFluent.KafkaClientAuthenticationTlsNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationTlsBuilder builder;
KafkaClientAuthenticationTlsNestedImpl(KafkaClientAuthenticationTls item){
this.builder = new KafkaClientAuthenticationTlsBuilder(this, item);
}
KafkaClientAuthenticationTlsNestedImpl(){
this.builder = new KafkaClientAuthenticationTlsBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withKafkaClientAuthenticationTls(builder.build());
}
public N endKafkaClientAuthenticationTls(){
return and();
}
}
public class TemplateNestedImpl extends KafkaConnectTemplateFluentImpl> implements KafkaConnectSpecFluent.TemplateNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaConnectTemplateBuilder builder;
TemplateNestedImpl(KafkaConnectTemplate item){
this.builder = new KafkaConnectTemplateBuilder(this, item);
}
TemplateNestedImpl(){
this.builder = new KafkaConnectTemplateBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withTemplate(builder.build());
}
public N endTemplate(){
return and();
}
}
public class ExternalConfigurationNestedImpl extends ExternalConfigurationFluentImpl> implements KafkaConnectSpecFluent.ExternalConfigurationNested,io.fabric8.kubernetes.api.builder.Nested{
private final ExternalConfigurationBuilder builder;
ExternalConfigurationNestedImpl(ExternalConfiguration item){
this.builder = new ExternalConfigurationBuilder(this, item);
}
ExternalConfigurationNestedImpl(){
this.builder = new ExternalConfigurationBuilder(this);
}
public N and(){
return (N) KafkaConnectSpecFluentImpl.this.withExternalConfiguration(builder.build());
}
public N endExternalConfiguration(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy