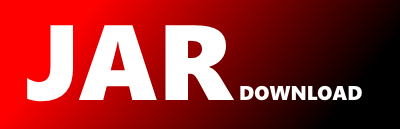
io.strimzi.api.kafka.model.KafkaExporterSpecFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import io.strimzi.api.kafka.model.template.KafkaExporterTemplate;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import io.strimzi.api.kafka.model.template.KafkaExporterTemplateBuilder;
import java.lang.String;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import java.lang.StringBuffer;
import java.lang.Deprecated;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Object;
import io.strimzi.api.kafka.model.template.KafkaExporterTemplateFluentImpl;
import java.lang.Boolean;
public class KafkaExporterSpecFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements KafkaExporterSpecFluent{
private String image;
private String groupRegex;
private String topicRegex;
private ResourceRequirements resources;
private ProbeBuilder livenessProbe;
private ProbeBuilder readinessProbe;
private String logging;
private boolean enableSaramaLogging;
private KafkaExporterTemplateBuilder template;
public KafkaExporterSpecFluentImpl(){
}
public KafkaExporterSpecFluentImpl(KafkaExporterSpec instance){
this.withImage(instance.getImage());
this.withGroupRegex(instance.getGroupRegex());
this.withTopicRegex(instance.getTopicRegex());
this.withResources(instance.getResources());
this.withLivenessProbe(instance.getLivenessProbe());
this.withReadinessProbe(instance.getReadinessProbe());
this.withLogging(instance.getLogging());
this.withEnableSaramaLogging(instance.getEnableSaramaLogging());
this.withTemplate(instance.getTemplate());
}
public String getImage(){
return this.image;
}
public A withImage(String image){
this.image=image; return (A) this;
}
public Boolean hasImage(){
return this.image != null;
}
public A withNewImage(String arg1){
return (A)withImage(new String(arg1));
}
public A withNewImage(StringBuilder arg1){
return (A)withImage(new String(arg1));
}
public A withNewImage(StringBuffer arg1){
return (A)withImage(new String(arg1));
}
public String getGroupRegex(){
return this.groupRegex;
}
public A withGroupRegex(String groupRegex){
this.groupRegex=groupRegex; return (A) this;
}
public Boolean hasGroupRegex(){
return this.groupRegex != null;
}
public A withNewGroupRegex(String arg1){
return (A)withGroupRegex(new String(arg1));
}
public A withNewGroupRegex(StringBuilder arg1){
return (A)withGroupRegex(new String(arg1));
}
public A withNewGroupRegex(StringBuffer arg1){
return (A)withGroupRegex(new String(arg1));
}
public String getTopicRegex(){
return this.topicRegex;
}
public A withTopicRegex(String topicRegex){
this.topicRegex=topicRegex; return (A) this;
}
public Boolean hasTopicRegex(){
return this.topicRegex != null;
}
public A withNewTopicRegex(String arg1){
return (A)withTopicRegex(new String(arg1));
}
public A withNewTopicRegex(StringBuilder arg1){
return (A)withTopicRegex(new String(arg1));
}
public A withNewTopicRegex(StringBuffer arg1){
return (A)withTopicRegex(new String(arg1));
}
public ResourceRequirements getResources(){
return this.resources;
}
public A withResources(ResourceRequirements resources){
this.resources=resources; return (A) this;
}
public Boolean hasResources(){
return this.resources != null;
}
/**
* This method has been deprecated, please use method buildLivenessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public Probe buildLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public A withLivenessProbe(Probe livenessProbe){
_visitables.get("livenessProbe").remove(this.livenessProbe);
if (livenessProbe!=null){ this.livenessProbe= new ProbeBuilder(livenessProbe); _visitables.get("livenessProbe").add(this.livenessProbe);} return (A) this;
}
public Boolean hasLivenessProbe(){
return this.livenessProbe != null;
}
public A withNewLivenessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withLivenessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public KafkaExporterSpecFluent.LivenessProbeNested withNewLivenessProbe(){
return new LivenessProbeNestedImpl();
}
public KafkaExporterSpecFluent.LivenessProbeNested withNewLivenessProbeLike(Probe item){
return new LivenessProbeNestedImpl(item);
}
public KafkaExporterSpecFluent.LivenessProbeNested editLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe());
}
public KafkaExporterSpecFluent.LivenessProbeNested editOrNewLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): new ProbeBuilder().build());
}
public KafkaExporterSpecFluent.LivenessProbeNested editOrNewLivenessProbeLike(Probe item){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): item);
}
/**
* This method has been deprecated, please use method buildReadinessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public Probe buildReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public A withReadinessProbe(Probe readinessProbe){
_visitables.get("readinessProbe").remove(this.readinessProbe);
if (readinessProbe!=null){ this.readinessProbe= new ProbeBuilder(readinessProbe); _visitables.get("readinessProbe").add(this.readinessProbe);} return (A) this;
}
public Boolean hasReadinessProbe(){
return this.readinessProbe != null;
}
public A withNewReadinessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withReadinessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public KafkaExporterSpecFluent.ReadinessProbeNested withNewReadinessProbe(){
return new ReadinessProbeNestedImpl();
}
public KafkaExporterSpecFluent.ReadinessProbeNested withNewReadinessProbeLike(Probe item){
return new ReadinessProbeNestedImpl(item);
}
public KafkaExporterSpecFluent.ReadinessProbeNested editReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe());
}
public KafkaExporterSpecFluent.ReadinessProbeNested editOrNewReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): new ProbeBuilder().build());
}
public KafkaExporterSpecFluent.ReadinessProbeNested editOrNewReadinessProbeLike(Probe item){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): item);
}
public String getLogging(){
return this.logging;
}
public A withLogging(String logging){
this.logging=logging; return (A) this;
}
public Boolean hasLogging(){
return this.logging != null;
}
public A withNewLogging(String arg1){
return (A)withLogging(new String(arg1));
}
public A withNewLogging(StringBuilder arg1){
return (A)withLogging(new String(arg1));
}
public A withNewLogging(StringBuffer arg1){
return (A)withLogging(new String(arg1));
}
public boolean isEnableSaramaLogging(){
return this.enableSaramaLogging;
}
public A withEnableSaramaLogging(boolean enableSaramaLogging){
this.enableSaramaLogging=enableSaramaLogging; return (A) this;
}
public Boolean hasEnableSaramaLogging(){
return true;
}
/**
* This method has been deprecated, please use method buildTemplate instead.
* @return The buildable object.
*/
@Deprecated public KafkaExporterTemplate getTemplate(){
return this.template!=null?this.template.build():null;
}
public KafkaExporterTemplate buildTemplate(){
return this.template!=null?this.template.build():null;
}
public A withTemplate(KafkaExporterTemplate template){
_visitables.get("template").remove(this.template);
if (template!=null){ this.template= new KafkaExporterTemplateBuilder(template); _visitables.get("template").add(this.template);} return (A) this;
}
public Boolean hasTemplate(){
return this.template != null;
}
public KafkaExporterSpecFluent.TemplateNested withNewTemplate(){
return new TemplateNestedImpl();
}
public KafkaExporterSpecFluent.TemplateNested withNewTemplateLike(KafkaExporterTemplate item){
return new TemplateNestedImpl(item);
}
public KafkaExporterSpecFluent.TemplateNested editTemplate(){
return withNewTemplateLike(getTemplate());
}
public KafkaExporterSpecFluent.TemplateNested editOrNewTemplate(){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): new KafkaExporterTemplateBuilder().build());
}
public KafkaExporterSpecFluent.TemplateNested editOrNewTemplateLike(KafkaExporterTemplate item){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
KafkaExporterSpecFluentImpl that = (KafkaExporterSpecFluentImpl) o;
if (image != null ? !image.equals(that.image) :that.image != null) return false;
if (groupRegex != null ? !groupRegex.equals(that.groupRegex) :that.groupRegex != null) return false;
if (topicRegex != null ? !topicRegex.equals(that.topicRegex) :that.topicRegex != null) return false;
if (resources != null ? !resources.equals(that.resources) :that.resources != null) return false;
if (livenessProbe != null ? !livenessProbe.equals(that.livenessProbe) :that.livenessProbe != null) return false;
if (readinessProbe != null ? !readinessProbe.equals(that.readinessProbe) :that.readinessProbe != null) return false;
if (logging != null ? !logging.equals(that.logging) :that.logging != null) return false;
if (enableSaramaLogging != that.enableSaramaLogging) return false;
if (template != null ? !template.equals(that.template) :that.template != null) return false;
return true;
}
public class LivenessProbeNestedImpl extends ProbeFluentImpl> implements KafkaExporterSpecFluent.LivenessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
LivenessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
LivenessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) KafkaExporterSpecFluentImpl.this.withLivenessProbe(builder.build());
}
public N endLivenessProbe(){
return and();
}
}
public class ReadinessProbeNestedImpl extends ProbeFluentImpl> implements KafkaExporterSpecFluent.ReadinessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
ReadinessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
ReadinessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) KafkaExporterSpecFluentImpl.this.withReadinessProbe(builder.build());
}
public N endReadinessProbe(){
return and();
}
}
public class TemplateNestedImpl extends KafkaExporterTemplateFluentImpl> implements KafkaExporterSpecFluent.TemplateNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaExporterTemplateBuilder builder;
TemplateNestedImpl(KafkaExporterTemplate item){
this.builder = new KafkaExporterTemplateBuilder(this, item);
}
TemplateNestedImpl(){
this.builder = new KafkaExporterTemplateBuilder(this);
}
public N and(){
return (N) KafkaExporterSpecFluentImpl.this.withTemplate(builder.build());
}
public N endTemplate(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy