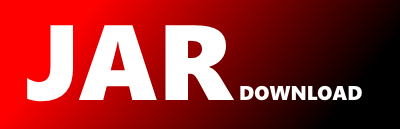
io.strimzi.api.kafka.model.TlsSidecarFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.Deprecated;
import java.lang.Object;
import java.lang.Boolean;
public class TlsSidecarFluentImpl> extends SidecarFluentImpl implements TlsSidecarFluent{
private TlsSidecarLogLevel logLevel;
private ProbeBuilder livenessProbe;
private ProbeBuilder readinessProbe;
public TlsSidecarFluentImpl(){
}
public TlsSidecarFluentImpl(TlsSidecar instance){
this.withLogLevel(instance.getLogLevel());
this.withLivenessProbe(instance.getLivenessProbe());
this.withReadinessProbe(instance.getReadinessProbe());
this.withImage(instance.getImage());
this.withResources(instance.getResources());
}
public TlsSidecarLogLevel getLogLevel(){
return this.logLevel;
}
public A withLogLevel(TlsSidecarLogLevel logLevel){
this.logLevel=logLevel; return (A) this;
}
public Boolean hasLogLevel(){
return this.logLevel != null;
}
/**
* This method has been deprecated, please use method buildLivenessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public Probe buildLivenessProbe(){
return this.livenessProbe!=null?this.livenessProbe.build():null;
}
public A withLivenessProbe(Probe livenessProbe){
_visitables.get("livenessProbe").remove(this.livenessProbe);
if (livenessProbe!=null){ this.livenessProbe= new ProbeBuilder(livenessProbe); _visitables.get("livenessProbe").add(this.livenessProbe);} return (A) this;
}
public Boolean hasLivenessProbe(){
return this.livenessProbe != null;
}
public A withNewLivenessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withLivenessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public TlsSidecarFluent.LivenessProbeNested withNewLivenessProbe(){
return new LivenessProbeNestedImpl();
}
public TlsSidecarFluent.LivenessProbeNested withNewLivenessProbeLike(Probe item){
return new LivenessProbeNestedImpl(item);
}
public TlsSidecarFluent.LivenessProbeNested editLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe());
}
public TlsSidecarFluent.LivenessProbeNested editOrNewLivenessProbe(){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): new ProbeBuilder().build());
}
public TlsSidecarFluent.LivenessProbeNested editOrNewLivenessProbeLike(Probe item){
return withNewLivenessProbeLike(getLivenessProbe() != null ? getLivenessProbe(): item);
}
/**
* This method has been deprecated, please use method buildReadinessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public Probe buildReadinessProbe(){
return this.readinessProbe!=null?this.readinessProbe.build():null;
}
public A withReadinessProbe(Probe readinessProbe){
_visitables.get("readinessProbe").remove(this.readinessProbe);
if (readinessProbe!=null){ this.readinessProbe= new ProbeBuilder(readinessProbe); _visitables.get("readinessProbe").add(this.readinessProbe);} return (A) this;
}
public Boolean hasReadinessProbe(){
return this.readinessProbe != null;
}
public A withNewReadinessProbe(int initialDelaySeconds,int timeoutSeconds){
return (A)withReadinessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public TlsSidecarFluent.ReadinessProbeNested withNewReadinessProbe(){
return new ReadinessProbeNestedImpl();
}
public TlsSidecarFluent.ReadinessProbeNested withNewReadinessProbeLike(Probe item){
return new ReadinessProbeNestedImpl(item);
}
public TlsSidecarFluent.ReadinessProbeNested editReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe());
}
public TlsSidecarFluent.ReadinessProbeNested editOrNewReadinessProbe(){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): new ProbeBuilder().build());
}
public TlsSidecarFluent.ReadinessProbeNested editOrNewReadinessProbeLike(Probe item){
return withNewReadinessProbeLike(getReadinessProbe() != null ? getReadinessProbe(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
TlsSidecarFluentImpl that = (TlsSidecarFluentImpl) o;
if (logLevel != null ? !logLevel.equals(that.logLevel) :that.logLevel != null) return false;
if (livenessProbe != null ? !livenessProbe.equals(that.livenessProbe) :that.livenessProbe != null) return false;
if (readinessProbe != null ? !readinessProbe.equals(that.readinessProbe) :that.readinessProbe != null) return false;
return true;
}
public class LivenessProbeNestedImpl extends ProbeFluentImpl> implements TlsSidecarFluent.LivenessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
LivenessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
LivenessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) TlsSidecarFluentImpl.this.withLivenessProbe(builder.build());
}
public N endLivenessProbe(){
return and();
}
}
public class ReadinessProbeNestedImpl extends ProbeFluentImpl> implements TlsSidecarFluent.ReadinessProbeNested,io.fabric8.kubernetes.api.builder.Nested{
private final ProbeBuilder builder;
ReadinessProbeNestedImpl(Probe item){
this.builder = new ProbeBuilder(this, item);
}
ReadinessProbeNestedImpl(){
this.builder = new ProbeBuilder(this);
}
public N and(){
return (N) TlsSidecarFluentImpl.this.withReadinessProbe(builder.build());
}
public N endReadinessProbe(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy