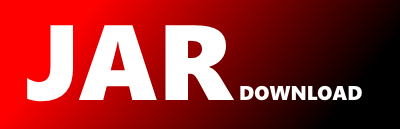
io.strimzi.api.kafka.model.CruiseControlSpecFluent Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.String;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import java.util.LinkedHashMap;
import io.strimzi.api.kafka.model.balancing.BrokerCapacityFluent;
import java.lang.Deprecated;
import java.lang.Boolean;
import io.strimzi.api.kafka.model.balancing.BrokerCapacityBuilder;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.strimzi.api.kafka.model.template.CruiseControlTemplateBuilder;
import io.strimzi.api.kafka.model.template.CruiseControlTemplate;
import java.lang.StringBuffer;
import io.strimzi.api.kafka.model.balancing.BrokerCapacity;
import java.lang.Object;
import io.strimzi.api.kafka.model.template.CruiseControlTemplateFluent;
import java.util.Map;
public interface CruiseControlSpecFluent> extends Fluent{
public String getImage();
public A withImage(String image);
public Boolean hasImage();
public A withNewImage(String arg1);
public A withNewImage(StringBuilder arg1);
public A withNewImage(StringBuffer arg1);
/**
* This method has been deprecated, please use method buildTlsSidecar instead.
* @return The buildable object.
*/
@Deprecated public TlsSidecar getTlsSidecar();
public TlsSidecar buildTlsSidecar();
public A withTlsSidecar(TlsSidecar tlsSidecar);
public Boolean hasTlsSidecar();
public CruiseControlSpecFluent.TlsSidecarNested withNewTlsSidecar();
public CruiseControlSpecFluent.TlsSidecarNested withNewTlsSidecarLike(TlsSidecar item);
public CruiseControlSpecFluent.TlsSidecarNested editTlsSidecar();
public CruiseControlSpecFluent.TlsSidecarNested editOrNewTlsSidecar();
public CruiseControlSpecFluent.TlsSidecarNested editOrNewTlsSidecarLike(TlsSidecar item);
public ResourceRequirements getResources();
public A withResources(ResourceRequirements resources);
public Boolean hasResources();
/**
* This method has been deprecated, please use method buildLivenessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getLivenessProbe();
public Probe buildLivenessProbe();
public A withLivenessProbe(Probe livenessProbe);
public Boolean hasLivenessProbe();
public A withNewLivenessProbe(int initialDelaySeconds,int timeoutSeconds);
public CruiseControlSpecFluent.LivenessProbeNested withNewLivenessProbe();
public CruiseControlSpecFluent.LivenessProbeNested withNewLivenessProbeLike(Probe item);
public CruiseControlSpecFluent.LivenessProbeNested editLivenessProbe();
public CruiseControlSpecFluent.LivenessProbeNested editOrNewLivenessProbe();
public CruiseControlSpecFluent.LivenessProbeNested editOrNewLivenessProbeLike(Probe item);
/**
* This method has been deprecated, please use method buildReadinessProbe instead.
* @return The buildable object.
*/
@Deprecated public Probe getReadinessProbe();
public Probe buildReadinessProbe();
public A withReadinessProbe(Probe readinessProbe);
public Boolean hasReadinessProbe();
public A withNewReadinessProbe(int initialDelaySeconds,int timeoutSeconds);
public CruiseControlSpecFluent.ReadinessProbeNested withNewReadinessProbe();
public CruiseControlSpecFluent.ReadinessProbeNested withNewReadinessProbeLike(Probe item);
public CruiseControlSpecFluent.ReadinessProbeNested editReadinessProbe();
public CruiseControlSpecFluent.ReadinessProbeNested editOrNewReadinessProbe();
public CruiseControlSpecFluent.ReadinessProbeNested editOrNewReadinessProbeLike(Probe item);
/**
* This method has been deprecated, please use method buildJvmOptions instead.
* @return The buildable object.
*/
@Deprecated public JvmOptions getJvmOptions();
public JvmOptions buildJvmOptions();
public A withJvmOptions(JvmOptions jvmOptions);
public Boolean hasJvmOptions();
public CruiseControlSpecFluent.JvmOptionsNested withNewJvmOptions();
public CruiseControlSpecFluent.JvmOptionsNested withNewJvmOptionsLike(JvmOptions item);
public CruiseControlSpecFluent.JvmOptionsNested editJvmOptions();
public CruiseControlSpecFluent.JvmOptionsNested editOrNewJvmOptions();
public CruiseControlSpecFluent.JvmOptionsNested editOrNewJvmOptionsLike(JvmOptions item);
/**
* This method has been deprecated, please use method buildLogging instead.
* @return The buildable object.
*/
@Deprecated public Logging getLogging();
public Logging buildLogging();
public A withLogging(Logging logging);
public Boolean hasLogging();
public A withExternalLogging(ExternalLogging externalLogging);
public CruiseControlSpecFluent.ExternalLoggingNested withNewExternalLogging();
public CruiseControlSpecFluent.ExternalLoggingNested withNewExternalLoggingLike(ExternalLogging item);
public A withInlineLogging(InlineLogging inlineLogging);
public CruiseControlSpecFluent.InlineLoggingNested withNewInlineLogging();
public CruiseControlSpecFluent.InlineLoggingNested withNewInlineLoggingLike(InlineLogging item);
/**
* This method has been deprecated, please use method buildTemplate instead.
* @return The buildable object.
*/
@Deprecated public CruiseControlTemplate getTemplate();
public CruiseControlTemplate buildTemplate();
public A withTemplate(CruiseControlTemplate template);
public Boolean hasTemplate();
public CruiseControlSpecFluent.TemplateNested withNewTemplate();
public CruiseControlSpecFluent.TemplateNested withNewTemplateLike(CruiseControlTemplate item);
public CruiseControlSpecFluent.TemplateNested editTemplate();
public CruiseControlSpecFluent.TemplateNested editOrNewTemplate();
public CruiseControlSpecFluent.TemplateNested editOrNewTemplateLike(CruiseControlTemplate item);
/**
* This method has been deprecated, please use method buildBrokerCapacity instead.
* @return The buildable object.
*/
@Deprecated public BrokerCapacity getBrokerCapacity();
public BrokerCapacity buildBrokerCapacity();
public A withBrokerCapacity(BrokerCapacity brokerCapacity);
public Boolean hasBrokerCapacity();
public CruiseControlSpecFluent.BrokerCapacityNested withNewBrokerCapacity();
public CruiseControlSpecFluent.BrokerCapacityNested withNewBrokerCapacityLike(BrokerCapacity item);
public CruiseControlSpecFluent.BrokerCapacityNested editBrokerCapacity();
public CruiseControlSpecFluent.BrokerCapacityNested editOrNewBrokerCapacity();
public CruiseControlSpecFluent.BrokerCapacityNested editOrNewBrokerCapacityLike(BrokerCapacity item);
public A addToConfig(String key,Object value);
public A addToConfig(Map map);
public A removeFromConfig(String key);
public A removeFromConfig(Map map);
public Map getConfig();
public A withConfig(Map config);
public Boolean hasConfig();
public A addToMetrics(String key,Object value);
public A addToMetrics(Map map);
public A removeFromMetrics(String key);
public A removeFromMetrics(Map map);
public Map getMetrics();
public A withMetrics(Map metrics);
public Boolean hasMetrics();
public interface TlsSidecarNested extends io.fabric8.kubernetes.api.builder.Nested,TlsSidecarFluent>{
public N and(); public N endTlsSidecar();
}
public interface LivenessProbeNested extends io.fabric8.kubernetes.api.builder.Nested,ProbeFluent>{
public N and(); public N endLivenessProbe();
}
public interface ReadinessProbeNested extends io.fabric8.kubernetes.api.builder.Nested,ProbeFluent>{
public N and(); public N endReadinessProbe();
}
public interface JvmOptionsNested extends io.fabric8.kubernetes.api.builder.Nested,JvmOptionsFluent>{
public N and(); public N endJvmOptions();
}
public interface ExternalLoggingNested extends io.fabric8.kubernetes.api.builder.Nested,ExternalLoggingFluent>{
public N and(); public N endExternalLogging();
}
public interface InlineLoggingNested extends io.fabric8.kubernetes.api.builder.Nested,InlineLoggingFluent>{
public N and(); public N endInlineLogging();
}
public interface TemplateNested extends io.fabric8.kubernetes.api.builder.Nested,CruiseControlTemplateFluent>{
public N and(); public N endTemplate();
}
public interface BrokerCapacityNested extends io.fabric8.kubernetes.api.builder.Nested,BrokerCapacityFluent>{
public N and(); public N endBrokerCapacity();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy