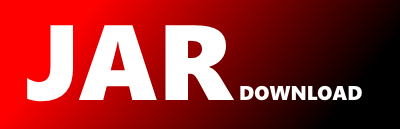
io.strimzi.api.kafka.model.KafkaAuthorizationOpaFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import java.lang.StringBuilder;
import java.util.ArrayList;
import java.lang.String;
import io.fabric8.kubernetes.api.builder.Predicate;
import java.lang.StringBuffer;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.Collection;
import java.lang.Object;
import java.util.List;
import java.lang.Boolean;
public class KafkaAuthorizationOpaFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements KafkaAuthorizationOpaFluent{
private java.util.List superUsers;
private String url;
private boolean allowOnError;
private int initialCacheCapacity;
private int maximumCacheSize;
private long expireAfterMs;
public KafkaAuthorizationOpaFluentImpl(){
}
public KafkaAuthorizationOpaFluentImpl(KafkaAuthorizationOpa instance){
this.withSuperUsers(instance.getSuperUsers());
this.withUrl(instance.getUrl());
this.withAllowOnError(instance.isAllowOnError());
this.withInitialCacheCapacity(instance.getInitialCacheCapacity());
this.withMaximumCacheSize(instance.getMaximumCacheSize());
this.withExpireAfterMs(instance.getExpireAfterMs());
}
public A addToSuperUsers(int index,String item){
if (this.superUsers == null) {this.superUsers = new ArrayList();}
this.superUsers.add(index, item);
return (A)this;
}
public A setToSuperUsers(int index,String item){
if (this.superUsers == null) {this.superUsers = new ArrayList();}
this.superUsers.set(index, item); return (A)this;
}
public A addToSuperUsers(String... items){
if (this.superUsers == null) {this.superUsers = new ArrayList();}
for (String item : items) {this.superUsers.add(item);} return (A)this;
}
public A addAllToSuperUsers(Collection items){
if (this.superUsers == null) {this.superUsers = new ArrayList();}
for (String item : items) {this.superUsers.add(item);} return (A)this;
}
public A removeFromSuperUsers(String... items){
for (String item : items) {if (this.superUsers!= null){ this.superUsers.remove(item);}} return (A)this;
}
public A removeAllFromSuperUsers(Collection items){
for (String item : items) {if (this.superUsers!= null){ this.superUsers.remove(item);}} return (A)this;
}
public java.util.List getSuperUsers(){
return this.superUsers;
}
public String getSuperUser(int index){
return this.superUsers.get(index);
}
public String getFirstSuperUser(){
return this.superUsers.get(0);
}
public String getLastSuperUser(){
return this.superUsers.get(superUsers.size() - 1);
}
public String getMatchingSuperUser(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (String item: superUsers) { if(predicate.apply(item)){ return item;} } return null;
}
public Boolean hasMatchingSuperUser(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (String item: superUsers) { if(predicate.apply(item)){ return true;} } return false;
}
public A withSuperUsers(java.util.List superUsers){
if (this.superUsers != null) { _visitables.get("superUsers").removeAll(this.superUsers);}
if (superUsers != null) {this.superUsers = new ArrayList(); for (String item : superUsers){this.addToSuperUsers(item);}} else { this.superUsers = null;} return (A) this;
}
public A withSuperUsers(String... superUsers){
if (this.superUsers != null) {this.superUsers.clear();}
if (superUsers != null) {for (String item :superUsers){ this.addToSuperUsers(item);}} return (A) this;
}
public Boolean hasSuperUsers(){
return superUsers != null && !superUsers.isEmpty();
}
public A addNewSuperUser(String arg1){
return (A)addToSuperUsers(new String(arg1));
}
public A addNewSuperUser(StringBuilder arg1){
return (A)addToSuperUsers(new String(arg1));
}
public A addNewSuperUser(StringBuffer arg1){
return (A)addToSuperUsers(new String(arg1));
}
public String getUrl(){
return this.url;
}
public A withUrl(String url){
this.url=url; return (A) this;
}
public Boolean hasUrl(){
return this.url != null;
}
public A withNewUrl(String arg1){
return (A)withUrl(new String(arg1));
}
public A withNewUrl(StringBuilder arg1){
return (A)withUrl(new String(arg1));
}
public A withNewUrl(StringBuffer arg1){
return (A)withUrl(new String(arg1));
}
public boolean isAllowOnError(){
return this.allowOnError;
}
public A withAllowOnError(boolean allowOnError){
this.allowOnError=allowOnError; return (A) this;
}
public Boolean hasAllowOnError(){
return true;
}
public int getInitialCacheCapacity(){
return this.initialCacheCapacity;
}
public A withInitialCacheCapacity(int initialCacheCapacity){
this.initialCacheCapacity=initialCacheCapacity; return (A) this;
}
public Boolean hasInitialCacheCapacity(){
return true;
}
public int getMaximumCacheSize(){
return this.maximumCacheSize;
}
public A withMaximumCacheSize(int maximumCacheSize){
this.maximumCacheSize=maximumCacheSize; return (A) this;
}
public Boolean hasMaximumCacheSize(){
return true;
}
public long getExpireAfterMs(){
return this.expireAfterMs;
}
public A withExpireAfterMs(long expireAfterMs){
this.expireAfterMs=expireAfterMs; return (A) this;
}
public Boolean hasExpireAfterMs(){
return true;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
KafkaAuthorizationOpaFluentImpl that = (KafkaAuthorizationOpaFluentImpl) o;
if (superUsers != null ? !superUsers.equals(that.superUsers) :that.superUsers != null) return false;
if (url != null ? !url.equals(that.url) :that.url != null) return false;
if (allowOnError != that.allowOnError) return false;
if (initialCacheCapacity != that.initialCacheCapacity) return false;
if (maximumCacheSize != that.maximumCacheSize) return false;
if (expireAfterMs != that.expireAfterMs) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy