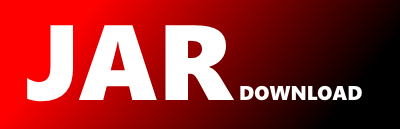
io.strimzi.api.kafka.model.KafkaMirrorMaker2ClusterSpecFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationOAuthBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationScramSha512FluentImpl;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.String;
import java.util.LinkedHashMap;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationScramSha512Builder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationOAuth;
import java.lang.Deprecated;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationTlsFluentImpl;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationPlainBuilder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationTlsBuilder;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationPlain;
import java.lang.Boolean;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationPlainFluentImpl;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationScramSha512;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationTls;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthenticationOAuthFluentImpl;
import io.strimzi.api.kafka.model.authentication.KafkaClientAuthentication;
import java.lang.StringBuffer;
import java.lang.Object;
import java.util.Map;
public class KafkaMirrorMaker2ClusterSpecFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements KafkaMirrorMaker2ClusterSpecFluent{
private String alias;
private String bootstrapServers;
private Map config;
private KafkaMirrorMaker2TlsBuilder tls;
private VisitableBuilder extends KafkaClientAuthentication,?> authentication;
public KafkaMirrorMaker2ClusterSpecFluentImpl(){
}
public KafkaMirrorMaker2ClusterSpecFluentImpl(KafkaMirrorMaker2ClusterSpec instance){
this.withAlias(instance.getAlias());
this.withBootstrapServers(instance.getBootstrapServers());
this.withConfig(instance.getConfig());
this.withTls(instance.getTls());
this.withAuthentication(instance.getAuthentication());
}
public String getAlias(){
return this.alias;
}
public A withAlias(String alias){
this.alias=alias; return (A) this;
}
public Boolean hasAlias(){
return this.alias != null;
}
public A withNewAlias(String arg1){
return (A)withAlias(new String(arg1));
}
public A withNewAlias(StringBuilder arg1){
return (A)withAlias(new String(arg1));
}
public A withNewAlias(StringBuffer arg1){
return (A)withAlias(new String(arg1));
}
public String getBootstrapServers(){
return this.bootstrapServers;
}
public A withBootstrapServers(String bootstrapServers){
this.bootstrapServers=bootstrapServers; return (A) this;
}
public Boolean hasBootstrapServers(){
return this.bootstrapServers != null;
}
public A withNewBootstrapServers(String arg1){
return (A)withBootstrapServers(new String(arg1));
}
public A withNewBootstrapServers(StringBuilder arg1){
return (A)withBootstrapServers(new String(arg1));
}
public A withNewBootstrapServers(StringBuffer arg1){
return (A)withBootstrapServers(new String(arg1));
}
public A addToConfig(String key,Object value){
if(this.config == null && key != null && value != null) { this.config = new LinkedHashMap(); }
if(key != null && value != null) {this.config.put(key, value);} return (A)this;
}
public A addToConfig(Map map){
if(this.config == null && map != null) { this.config = new LinkedHashMap(); }
if(map != null) { this.config.putAll(map);} return (A)this;
}
public A removeFromConfig(String key){
if(this.config == null) { return (A) this; }
if(key != null && this.config != null) {this.config.remove(key);} return (A)this;
}
public A removeFromConfig(Map map){
if(this.config == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.config != null){this.config.remove(key);}}} return (A)this;
}
public Map getConfig(){
return this.config;
}
public A withConfig(Map config){
if (config == null) { this.config = null;} else {this.config = new LinkedHashMap(config);} return (A) this;
}
public Boolean hasConfig(){
return this.config != null;
}
/**
* This method has been deprecated, please use method buildTls instead.
* @return The buildable object.
*/
@Deprecated public KafkaMirrorMaker2Tls getTls(){
return this.tls!=null?this.tls.build():null;
}
public KafkaMirrorMaker2Tls buildTls(){
return this.tls!=null?this.tls.build():null;
}
public A withTls(KafkaMirrorMaker2Tls tls){
_visitables.get("tls").remove(this.tls);
if (tls!=null){ this.tls= new KafkaMirrorMaker2TlsBuilder(tls); _visitables.get("tls").add(this.tls);} return (A) this;
}
public Boolean hasTls(){
return this.tls != null;
}
public KafkaMirrorMaker2ClusterSpecFluent.TlsNested withNewTls(){
return new TlsNestedImpl();
}
public KafkaMirrorMaker2ClusterSpecFluent.TlsNested withNewTlsLike(KafkaMirrorMaker2Tls item){
return new TlsNestedImpl(item);
}
public KafkaMirrorMaker2ClusterSpecFluent.TlsNested editTls(){
return withNewTlsLike(getTls());
}
public KafkaMirrorMaker2ClusterSpecFluent.TlsNested editOrNewTls(){
return withNewTlsLike(getTls() != null ? getTls(): new KafkaMirrorMaker2TlsBuilder().build());
}
public KafkaMirrorMaker2ClusterSpecFluent.TlsNested editOrNewTlsLike(KafkaMirrorMaker2Tls item){
return withNewTlsLike(getTls() != null ? getTls(): item);
}
/**
* This method has been deprecated, please use method buildAuthentication instead.
* @return The buildable object.
*/
@Deprecated public KafkaClientAuthentication getAuthentication(){
return this.authentication!=null?this.authentication.build():null;
}
public KafkaClientAuthentication buildAuthentication(){
return this.authentication!=null?this.authentication.build():null;
}
public A withAuthentication(KafkaClientAuthentication authentication){
if (authentication instanceof KafkaClientAuthenticationScramSha512){ this.authentication= new KafkaClientAuthenticationScramSha512Builder((KafkaClientAuthenticationScramSha512)authentication); _visitables.get("authentication").add(this.authentication);}
if (authentication instanceof KafkaClientAuthenticationPlain){ this.authentication= new KafkaClientAuthenticationPlainBuilder((KafkaClientAuthenticationPlain)authentication); _visitables.get("authentication").add(this.authentication);}
if (authentication instanceof KafkaClientAuthenticationOAuth){ this.authentication= new KafkaClientAuthenticationOAuthBuilder((KafkaClientAuthenticationOAuth)authentication); _visitables.get("authentication").add(this.authentication);}
if (authentication instanceof KafkaClientAuthenticationTls){ this.authentication= new KafkaClientAuthenticationTlsBuilder((KafkaClientAuthenticationTls)authentication); _visitables.get("authentication").add(this.authentication);}
return (A) this;
}
public Boolean hasAuthentication(){
return this.authentication != null;
}
public A withKafkaClientAuthenticationScramSha512(KafkaClientAuthenticationScramSha512 kafkaClientAuthenticationScramSha512){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationScramSha512!=null){ this.authentication= new KafkaClientAuthenticationScramSha512Builder(kafkaClientAuthenticationScramSha512); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationScramSha512Nested withNewKafkaClientAuthenticationScramSha512(){
return new KafkaClientAuthenticationScramSha512NestedImpl();
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationScramSha512Nested withNewKafkaClientAuthenticationScramSha512Like(KafkaClientAuthenticationScramSha512 item){
return new KafkaClientAuthenticationScramSha512NestedImpl(item);
}
public A withKafkaClientAuthenticationPlain(KafkaClientAuthenticationPlain kafkaClientAuthenticationPlain){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationPlain!=null){ this.authentication= new KafkaClientAuthenticationPlainBuilder(kafkaClientAuthenticationPlain); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationPlainNested withNewKafkaClientAuthenticationPlain(){
return new KafkaClientAuthenticationPlainNestedImpl();
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationPlainNested withNewKafkaClientAuthenticationPlainLike(KafkaClientAuthenticationPlain item){
return new KafkaClientAuthenticationPlainNestedImpl(item);
}
public A withKafkaClientAuthenticationOAuth(KafkaClientAuthenticationOAuth kafkaClientAuthenticationOAuth){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationOAuth!=null){ this.authentication= new KafkaClientAuthenticationOAuthBuilder(kafkaClientAuthenticationOAuth); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationOAuthNested withNewKafkaClientAuthenticationOAuth(){
return new KafkaClientAuthenticationOAuthNestedImpl();
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationOAuthNested withNewKafkaClientAuthenticationOAuthLike(KafkaClientAuthenticationOAuth item){
return new KafkaClientAuthenticationOAuthNestedImpl(item);
}
public A withKafkaClientAuthenticationTls(KafkaClientAuthenticationTls kafkaClientAuthenticationTls){
_visitables.get("authentication").remove(this.authentication);
if (kafkaClientAuthenticationTls!=null){ this.authentication= new KafkaClientAuthenticationTlsBuilder(kafkaClientAuthenticationTls); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationTlsNested withNewKafkaClientAuthenticationTls(){
return new KafkaClientAuthenticationTlsNestedImpl();
}
public KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationTlsNested withNewKafkaClientAuthenticationTlsLike(KafkaClientAuthenticationTls item){
return new KafkaClientAuthenticationTlsNestedImpl(item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
KafkaMirrorMaker2ClusterSpecFluentImpl that = (KafkaMirrorMaker2ClusterSpecFluentImpl) o;
if (alias != null ? !alias.equals(that.alias) :that.alias != null) return false;
if (bootstrapServers != null ? !bootstrapServers.equals(that.bootstrapServers) :that.bootstrapServers != null) return false;
if (config != null ? !config.equals(that.config) :that.config != null) return false;
if (tls != null ? !tls.equals(that.tls) :that.tls != null) return false;
if (authentication != null ? !authentication.equals(that.authentication) :that.authentication != null) return false;
return true;
}
public class TlsNestedImpl extends KafkaMirrorMaker2TlsFluentImpl> implements KafkaMirrorMaker2ClusterSpecFluent.TlsNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaMirrorMaker2TlsBuilder builder;
TlsNestedImpl(KafkaMirrorMaker2Tls item){
this.builder = new KafkaMirrorMaker2TlsBuilder(this, item);
}
TlsNestedImpl(){
this.builder = new KafkaMirrorMaker2TlsBuilder(this);
}
public N and(){
return (N) KafkaMirrorMaker2ClusterSpecFluentImpl.this.withTls(builder.build());
}
public N endTls(){
return and();
}
}
public class KafkaClientAuthenticationScramSha512NestedImpl extends KafkaClientAuthenticationScramSha512FluentImpl> implements KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationScramSha512Nested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationScramSha512Builder builder;
KafkaClientAuthenticationScramSha512NestedImpl(KafkaClientAuthenticationScramSha512 item){
this.builder = new KafkaClientAuthenticationScramSha512Builder(this, item);
}
KafkaClientAuthenticationScramSha512NestedImpl(){
this.builder = new KafkaClientAuthenticationScramSha512Builder(this);
}
public N and(){
return (N) KafkaMirrorMaker2ClusterSpecFluentImpl.this.withKafkaClientAuthenticationScramSha512(builder.build());
}
public N endKafkaClientAuthenticationScramSha512(){
return and();
}
}
public class KafkaClientAuthenticationPlainNestedImpl extends KafkaClientAuthenticationPlainFluentImpl> implements KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationPlainNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationPlainBuilder builder;
KafkaClientAuthenticationPlainNestedImpl(KafkaClientAuthenticationPlain item){
this.builder = new KafkaClientAuthenticationPlainBuilder(this, item);
}
KafkaClientAuthenticationPlainNestedImpl(){
this.builder = new KafkaClientAuthenticationPlainBuilder(this);
}
public N and(){
return (N) KafkaMirrorMaker2ClusterSpecFluentImpl.this.withKafkaClientAuthenticationPlain(builder.build());
}
public N endKafkaClientAuthenticationPlain(){
return and();
}
}
public class KafkaClientAuthenticationOAuthNestedImpl extends KafkaClientAuthenticationOAuthFluentImpl> implements KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationOAuthNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationOAuthBuilder builder;
KafkaClientAuthenticationOAuthNestedImpl(KafkaClientAuthenticationOAuth item){
this.builder = new KafkaClientAuthenticationOAuthBuilder(this, item);
}
KafkaClientAuthenticationOAuthNestedImpl(){
this.builder = new KafkaClientAuthenticationOAuthBuilder(this);
}
public N and(){
return (N) KafkaMirrorMaker2ClusterSpecFluentImpl.this.withKafkaClientAuthenticationOAuth(builder.build());
}
public N endKafkaClientAuthenticationOAuth(){
return and();
}
}
public class KafkaClientAuthenticationTlsNestedImpl extends KafkaClientAuthenticationTlsFluentImpl> implements KafkaMirrorMaker2ClusterSpecFluent.KafkaClientAuthenticationTlsNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaClientAuthenticationTlsBuilder builder;
KafkaClientAuthenticationTlsNestedImpl(KafkaClientAuthenticationTls item){
this.builder = new KafkaClientAuthenticationTlsBuilder(this, item);
}
KafkaClientAuthenticationTlsNestedImpl(){
this.builder = new KafkaClientAuthenticationTlsBuilder(this);
}
public N and(){
return (N) KafkaMirrorMaker2ClusterSpecFluentImpl.this.withKafkaClientAuthenticationTls(builder.build());
}
public N endKafkaClientAuthenticationTls(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy