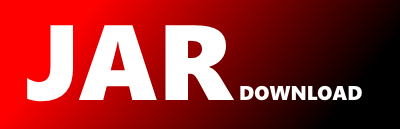
io.strimzi.api.kafka.model.KafkaUserSpecFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.strimzi.api.kafka.model.template.KafkaUserTemplate;
import io.fabric8.kubernetes.api.builder.Nested;
import io.strimzi.api.kafka.model.template.KafkaUserTemplateBuilder;
import java.lang.Deprecated;
import java.lang.Boolean;
import java.lang.Object;
import io.strimzi.api.kafka.model.template.KafkaUserTemplateFluentImpl;
public class KafkaUserSpecFluentImpl> extends SpecFluentImpl implements KafkaUserSpecFluent{
private VisitableBuilder extends KafkaUserAuthentication,?> authentication;
private VisitableBuilder extends KafkaUserAuthorization,?> authorization;
private KafkaUserQuotasBuilder quotas;
private KafkaUserTemplateBuilder template;
public KafkaUserSpecFluentImpl(){
}
public KafkaUserSpecFluentImpl(KafkaUserSpec instance){
this.withAuthentication(instance.getAuthentication());
this.withAuthorization(instance.getAuthorization());
this.withQuotas(instance.getQuotas());
this.withTemplate(instance.getTemplate());
}
/**
* This method has been deprecated, please use method buildAuthentication instead.
* @return The buildable object.
*/
@Deprecated public KafkaUserAuthentication getAuthentication(){
return this.authentication!=null?this.authentication.build():null;
}
public KafkaUserAuthentication buildAuthentication(){
return this.authentication!=null?this.authentication.build():null;
}
public A withAuthentication(KafkaUserAuthentication authentication){
if (authentication instanceof KafkaUserTlsClientAuthentication){ this.authentication= new KafkaUserTlsClientAuthenticationBuilder((KafkaUserTlsClientAuthentication)authentication); _visitables.get("authentication").add(this.authentication);}
if (authentication instanceof KafkaUserScramSha512ClientAuthentication){ this.authentication= new KafkaUserScramSha512ClientAuthenticationBuilder((KafkaUserScramSha512ClientAuthentication)authentication); _visitables.get("authentication").add(this.authentication);}
return (A) this;
}
public Boolean hasAuthentication(){
return this.authentication != null;
}
public A withKafkaUserTlsClientAuthentication(KafkaUserTlsClientAuthentication kafkaUserTlsClientAuthentication){
_visitables.get("authentication").remove(this.authentication);
if (kafkaUserTlsClientAuthentication!=null){ this.authentication= new KafkaUserTlsClientAuthenticationBuilder(kafkaUserTlsClientAuthentication); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaUserSpecFluent.KafkaUserTlsClientAuthenticationNested withNewKafkaUserTlsClientAuthentication(){
return new KafkaUserTlsClientAuthenticationNestedImpl();
}
public KafkaUserSpecFluent.KafkaUserTlsClientAuthenticationNested withNewKafkaUserTlsClientAuthenticationLike(KafkaUserTlsClientAuthentication item){
return new KafkaUserTlsClientAuthenticationNestedImpl(item);
}
public A withKafkaUserScramSha512ClientAuthentication(KafkaUserScramSha512ClientAuthentication kafkaUserScramSha512ClientAuthentication){
_visitables.get("authentication").remove(this.authentication);
if (kafkaUserScramSha512ClientAuthentication!=null){ this.authentication= new KafkaUserScramSha512ClientAuthenticationBuilder(kafkaUserScramSha512ClientAuthentication); _visitables.get("authentication").add(this.authentication);} return (A) this;
}
public KafkaUserSpecFluent.KafkaUserScramSha512ClientAuthenticationNested withNewKafkaUserScramSha512ClientAuthentication(){
return new KafkaUserScramSha512ClientAuthenticationNestedImpl();
}
public KafkaUserSpecFluent.KafkaUserScramSha512ClientAuthenticationNested withNewKafkaUserScramSha512ClientAuthenticationLike(KafkaUserScramSha512ClientAuthentication item){
return new KafkaUserScramSha512ClientAuthenticationNestedImpl(item);
}
/**
* This method has been deprecated, please use method buildAuthorization instead.
* @return The buildable object.
*/
@Deprecated public KafkaUserAuthorization getAuthorization(){
return this.authorization!=null?this.authorization.build():null;
}
public KafkaUserAuthorization buildAuthorization(){
return this.authorization!=null?this.authorization.build():null;
}
public A withAuthorization(KafkaUserAuthorization authorization){
if (authorization instanceof KafkaUserAuthorizationSimple){ this.authorization= new KafkaUserAuthorizationSimpleBuilder((KafkaUserAuthorizationSimple)authorization); _visitables.get("authorization").add(this.authorization);}
return (A) this;
}
public Boolean hasAuthorization(){
return this.authorization != null;
}
public A withKafkaUserAuthorizationSimple(KafkaUserAuthorizationSimple kafkaUserAuthorizationSimple){
_visitables.get("authorization").remove(this.authorization);
if (kafkaUserAuthorizationSimple!=null){ this.authorization= new KafkaUserAuthorizationSimpleBuilder(kafkaUserAuthorizationSimple); _visitables.get("authorization").add(this.authorization);} return (A) this;
}
public KafkaUserSpecFluent.KafkaUserAuthorizationSimpleNested withNewKafkaUserAuthorizationSimple(){
return new KafkaUserAuthorizationSimpleNestedImpl();
}
public KafkaUserSpecFluent.KafkaUserAuthorizationSimpleNested withNewKafkaUserAuthorizationSimpleLike(KafkaUserAuthorizationSimple item){
return new KafkaUserAuthorizationSimpleNestedImpl(item);
}
/**
* This method has been deprecated, please use method buildQuotas instead.
* @return The buildable object.
*/
@Deprecated public KafkaUserQuotas getQuotas(){
return this.quotas!=null?this.quotas.build():null;
}
public KafkaUserQuotas buildQuotas(){
return this.quotas!=null?this.quotas.build():null;
}
public A withQuotas(KafkaUserQuotas quotas){
_visitables.get("quotas").remove(this.quotas);
if (quotas!=null){ this.quotas= new KafkaUserQuotasBuilder(quotas); _visitables.get("quotas").add(this.quotas);} return (A) this;
}
public Boolean hasQuotas(){
return this.quotas != null;
}
public KafkaUserSpecFluent.QuotasNested withNewQuotas(){
return new QuotasNestedImpl();
}
public KafkaUserSpecFluent.QuotasNested withNewQuotasLike(KafkaUserQuotas item){
return new QuotasNestedImpl(item);
}
public KafkaUserSpecFluent.QuotasNested editQuotas(){
return withNewQuotasLike(getQuotas());
}
public KafkaUserSpecFluent.QuotasNested editOrNewQuotas(){
return withNewQuotasLike(getQuotas() != null ? getQuotas(): new KafkaUserQuotasBuilder().build());
}
public KafkaUserSpecFluent.QuotasNested editOrNewQuotasLike(KafkaUserQuotas item){
return withNewQuotasLike(getQuotas() != null ? getQuotas(): item);
}
/**
* This method has been deprecated, please use method buildTemplate instead.
* @return The buildable object.
*/
@Deprecated public KafkaUserTemplate getTemplate(){
return this.template!=null?this.template.build():null;
}
public KafkaUserTemplate buildTemplate(){
return this.template!=null?this.template.build():null;
}
public A withTemplate(KafkaUserTemplate template){
_visitables.get("template").remove(this.template);
if (template!=null){ this.template= new KafkaUserTemplateBuilder(template); _visitables.get("template").add(this.template);} return (A) this;
}
public Boolean hasTemplate(){
return this.template != null;
}
public KafkaUserSpecFluent.TemplateNested withNewTemplate(){
return new TemplateNestedImpl();
}
public KafkaUserSpecFluent.TemplateNested withNewTemplateLike(KafkaUserTemplate item){
return new TemplateNestedImpl(item);
}
public KafkaUserSpecFluent.TemplateNested editTemplate(){
return withNewTemplateLike(getTemplate());
}
public KafkaUserSpecFluent.TemplateNested editOrNewTemplate(){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): new KafkaUserTemplateBuilder().build());
}
public KafkaUserSpecFluent.TemplateNested editOrNewTemplateLike(KafkaUserTemplate item){
return withNewTemplateLike(getTemplate() != null ? getTemplate(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
KafkaUserSpecFluentImpl that = (KafkaUserSpecFluentImpl) o;
if (authentication != null ? !authentication.equals(that.authentication) :that.authentication != null) return false;
if (authorization != null ? !authorization.equals(that.authorization) :that.authorization != null) return false;
if (quotas != null ? !quotas.equals(that.quotas) :that.quotas != null) return false;
if (template != null ? !template.equals(that.template) :that.template != null) return false;
return true;
}
public class KafkaUserTlsClientAuthenticationNestedImpl extends KafkaUserTlsClientAuthenticationFluentImpl> implements KafkaUserSpecFluent.KafkaUserTlsClientAuthenticationNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaUserTlsClientAuthenticationBuilder builder;
KafkaUserTlsClientAuthenticationNestedImpl(KafkaUserTlsClientAuthentication item){
this.builder = new KafkaUserTlsClientAuthenticationBuilder(this, item);
}
KafkaUserTlsClientAuthenticationNestedImpl(){
this.builder = new KafkaUserTlsClientAuthenticationBuilder(this);
}
public N and(){
return (N) KafkaUserSpecFluentImpl.this.withKafkaUserTlsClientAuthentication(builder.build());
}
public N endKafkaUserTlsClientAuthentication(){
return and();
}
}
public class KafkaUserScramSha512ClientAuthenticationNestedImpl extends KafkaUserScramSha512ClientAuthenticationFluentImpl> implements KafkaUserSpecFluent.KafkaUserScramSha512ClientAuthenticationNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaUserScramSha512ClientAuthenticationBuilder builder;
KafkaUserScramSha512ClientAuthenticationNestedImpl(KafkaUserScramSha512ClientAuthentication item){
this.builder = new KafkaUserScramSha512ClientAuthenticationBuilder(this, item);
}
KafkaUserScramSha512ClientAuthenticationNestedImpl(){
this.builder = new KafkaUserScramSha512ClientAuthenticationBuilder(this);
}
public N and(){
return (N) KafkaUserSpecFluentImpl.this.withKafkaUserScramSha512ClientAuthentication(builder.build());
}
public N endKafkaUserScramSha512ClientAuthentication(){
return and();
}
}
public class KafkaUserAuthorizationSimpleNestedImpl extends KafkaUserAuthorizationSimpleFluentImpl> implements KafkaUserSpecFluent.KafkaUserAuthorizationSimpleNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaUserAuthorizationSimpleBuilder builder;
KafkaUserAuthorizationSimpleNestedImpl(KafkaUserAuthorizationSimple item){
this.builder = new KafkaUserAuthorizationSimpleBuilder(this, item);
}
KafkaUserAuthorizationSimpleNestedImpl(){
this.builder = new KafkaUserAuthorizationSimpleBuilder(this);
}
public N and(){
return (N) KafkaUserSpecFluentImpl.this.withKafkaUserAuthorizationSimple(builder.build());
}
public N endKafkaUserAuthorizationSimple(){
return and();
}
}
public class QuotasNestedImpl extends KafkaUserQuotasFluentImpl> implements KafkaUserSpecFluent.QuotasNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaUserQuotasBuilder builder;
QuotasNestedImpl(KafkaUserQuotas item){
this.builder = new KafkaUserQuotasBuilder(this, item);
}
QuotasNestedImpl(){
this.builder = new KafkaUserQuotasBuilder(this);
}
public N and(){
return (N) KafkaUserSpecFluentImpl.this.withQuotas(builder.build());
}
public N endQuotas(){
return and();
}
}
public class TemplateNestedImpl extends KafkaUserTemplateFluentImpl> implements KafkaUserSpecFluent.TemplateNested,io.fabric8.kubernetes.api.builder.Nested{
private final KafkaUserTemplateBuilder builder;
TemplateNestedImpl(KafkaUserTemplate item){
this.builder = new KafkaUserTemplateBuilder(this, item);
}
TemplateNestedImpl(){
this.builder = new KafkaUserTemplateBuilder(this);
}
public N and(){
return (N) KafkaUserSpecFluentImpl.this.withTemplate(builder.build());
}
public N endTemplate(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy