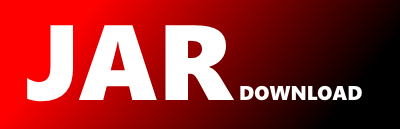
io.strimzi.api.kafka.model.template.KafkaClusterTemplateFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model.template;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Boolean;
import java.lang.Object;
import io.fabric8.kubernetes.api.builder.Nested;
import java.lang.Deprecated;
public class KafkaClusterTemplateFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements KafkaClusterTemplateFluent{
private StatefulSetTemplateBuilder statefulset;
private PodTemplateBuilder pod;
private ResourceTemplateBuilder bootstrapService;
private ResourceTemplateBuilder brokersService;
private ExternalServiceTemplateBuilder externalBootstrapService;
private ExternalServiceTemplateBuilder perPodService;
private ResourceTemplateBuilder externalBootstrapRoute;
private ResourceTemplateBuilder perPodRoute;
private ResourceTemplateBuilder externalBootstrapIngress;
private ResourceTemplateBuilder perPodIngress;
private ResourceTemplateBuilder persistentVolumeClaim;
private PodDisruptionBudgetTemplateBuilder podDisruptionBudget;
private ContainerTemplateBuilder kafkaContainer;
private ContainerTemplateBuilder tlsSidecarContainer;
private ContainerTemplateBuilder initContainer;
public KafkaClusterTemplateFluentImpl(){
}
public KafkaClusterTemplateFluentImpl(KafkaClusterTemplate instance){
this.withStatefulset(instance.getStatefulset());
this.withPod(instance.getPod());
this.withBootstrapService(instance.getBootstrapService());
this.withBrokersService(instance.getBrokersService());
this.withExternalBootstrapService(instance.getExternalBootstrapService());
this.withPerPodService(instance.getPerPodService());
this.withExternalBootstrapRoute(instance.getExternalBootstrapRoute());
this.withPerPodRoute(instance.getPerPodRoute());
this.withExternalBootstrapIngress(instance.getExternalBootstrapIngress());
this.withPerPodIngress(instance.getPerPodIngress());
this.withPersistentVolumeClaim(instance.getPersistentVolumeClaim());
this.withPodDisruptionBudget(instance.getPodDisruptionBudget());
this.withKafkaContainer(instance.getKafkaContainer());
this.withTlsSidecarContainer(instance.getTlsSidecarContainer());
this.withInitContainer(instance.getInitContainer());
}
/**
* This method has been deprecated, please use method buildStatefulset instead.
* @return The buildable object.
*/
@Deprecated public StatefulSetTemplate getStatefulset(){
return this.statefulset!=null?this.statefulset.build():null;
}
public StatefulSetTemplate buildStatefulset(){
return this.statefulset!=null?this.statefulset.build():null;
}
public A withStatefulset(StatefulSetTemplate statefulset){
_visitables.get("statefulset").remove(this.statefulset);
if (statefulset!=null){ this.statefulset= new StatefulSetTemplateBuilder(statefulset); _visitables.get("statefulset").add(this.statefulset);} return (A) this;
}
public Boolean hasStatefulset(){
return this.statefulset != null;
}
public KafkaClusterTemplateFluent.StatefulsetNested withNewStatefulset(){
return new StatefulsetNestedImpl();
}
public KafkaClusterTemplateFluent.StatefulsetNested withNewStatefulsetLike(StatefulSetTemplate item){
return new StatefulsetNestedImpl(item);
}
public KafkaClusterTemplateFluent.StatefulsetNested editStatefulset(){
return withNewStatefulsetLike(getStatefulset());
}
public KafkaClusterTemplateFluent.StatefulsetNested editOrNewStatefulset(){
return withNewStatefulsetLike(getStatefulset() != null ? getStatefulset(): new StatefulSetTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.StatefulsetNested editOrNewStatefulsetLike(StatefulSetTemplate item){
return withNewStatefulsetLike(getStatefulset() != null ? getStatefulset(): item);
}
/**
* This method has been deprecated, please use method buildPod instead.
* @return The buildable object.
*/
@Deprecated public PodTemplate getPod(){
return this.pod!=null?this.pod.build():null;
}
public PodTemplate buildPod(){
return this.pod!=null?this.pod.build():null;
}
public A withPod(PodTemplate pod){
_visitables.get("pod").remove(this.pod);
if (pod!=null){ this.pod= new PodTemplateBuilder(pod); _visitables.get("pod").add(this.pod);} return (A) this;
}
public Boolean hasPod(){
return this.pod != null;
}
public KafkaClusterTemplateFluent.PodNested withNewPod(){
return new PodNestedImpl();
}
public KafkaClusterTemplateFluent.PodNested withNewPodLike(PodTemplate item){
return new PodNestedImpl(item);
}
public KafkaClusterTemplateFluent.PodNested editPod(){
return withNewPodLike(getPod());
}
public KafkaClusterTemplateFluent.PodNested editOrNewPod(){
return withNewPodLike(getPod() != null ? getPod(): new PodTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.PodNested editOrNewPodLike(PodTemplate item){
return withNewPodLike(getPod() != null ? getPod(): item);
}
/**
* This method has been deprecated, please use method buildBootstrapService instead.
* @return The buildable object.
*/
@Deprecated public ResourceTemplate getBootstrapService(){
return this.bootstrapService!=null?this.bootstrapService.build():null;
}
public ResourceTemplate buildBootstrapService(){
return this.bootstrapService!=null?this.bootstrapService.build():null;
}
public A withBootstrapService(ResourceTemplate bootstrapService){
_visitables.get("bootstrapService").remove(this.bootstrapService);
if (bootstrapService!=null){ this.bootstrapService= new ResourceTemplateBuilder(bootstrapService); _visitables.get("bootstrapService").add(this.bootstrapService);} return (A) this;
}
public Boolean hasBootstrapService(){
return this.bootstrapService != null;
}
public KafkaClusterTemplateFluent.BootstrapServiceNested withNewBootstrapService(){
return new BootstrapServiceNestedImpl();
}
public KafkaClusterTemplateFluent.BootstrapServiceNested withNewBootstrapServiceLike(ResourceTemplate item){
return new BootstrapServiceNestedImpl(item);
}
public KafkaClusterTemplateFluent.BootstrapServiceNested editBootstrapService(){
return withNewBootstrapServiceLike(getBootstrapService());
}
public KafkaClusterTemplateFluent.BootstrapServiceNested editOrNewBootstrapService(){
return withNewBootstrapServiceLike(getBootstrapService() != null ? getBootstrapService(): new ResourceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.BootstrapServiceNested editOrNewBootstrapServiceLike(ResourceTemplate item){
return withNewBootstrapServiceLike(getBootstrapService() != null ? getBootstrapService(): item);
}
/**
* This method has been deprecated, please use method buildBrokersService instead.
* @return The buildable object.
*/
@Deprecated public ResourceTemplate getBrokersService(){
return this.brokersService!=null?this.brokersService.build():null;
}
public ResourceTemplate buildBrokersService(){
return this.brokersService!=null?this.brokersService.build():null;
}
public A withBrokersService(ResourceTemplate brokersService){
_visitables.get("brokersService").remove(this.brokersService);
if (brokersService!=null){ this.brokersService= new ResourceTemplateBuilder(brokersService); _visitables.get("brokersService").add(this.brokersService);} return (A) this;
}
public Boolean hasBrokersService(){
return this.brokersService != null;
}
public KafkaClusterTemplateFluent.BrokersServiceNested withNewBrokersService(){
return new BrokersServiceNestedImpl();
}
public KafkaClusterTemplateFluent.BrokersServiceNested withNewBrokersServiceLike(ResourceTemplate item){
return new BrokersServiceNestedImpl(item);
}
public KafkaClusterTemplateFluent.BrokersServiceNested editBrokersService(){
return withNewBrokersServiceLike(getBrokersService());
}
public KafkaClusterTemplateFluent.BrokersServiceNested editOrNewBrokersService(){
return withNewBrokersServiceLike(getBrokersService() != null ? getBrokersService(): new ResourceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.BrokersServiceNested editOrNewBrokersServiceLike(ResourceTemplate item){
return withNewBrokersServiceLike(getBrokersService() != null ? getBrokersService(): item);
}
/**
* This method has been deprecated, please use method buildExternalBootstrapService instead.
* @return The buildable object.
*/
@Deprecated public ExternalServiceTemplate getExternalBootstrapService(){
return this.externalBootstrapService!=null?this.externalBootstrapService.build():null;
}
public ExternalServiceTemplate buildExternalBootstrapService(){
return this.externalBootstrapService!=null?this.externalBootstrapService.build():null;
}
public A withExternalBootstrapService(ExternalServiceTemplate externalBootstrapService){
_visitables.get("externalBootstrapService").remove(this.externalBootstrapService);
if (externalBootstrapService!=null){ this.externalBootstrapService= new ExternalServiceTemplateBuilder(externalBootstrapService); _visitables.get("externalBootstrapService").add(this.externalBootstrapService);} return (A) this;
}
public Boolean hasExternalBootstrapService(){
return this.externalBootstrapService != null;
}
public KafkaClusterTemplateFluent.ExternalBootstrapServiceNested withNewExternalBootstrapService(){
return new ExternalBootstrapServiceNestedImpl();
}
public KafkaClusterTemplateFluent.ExternalBootstrapServiceNested withNewExternalBootstrapServiceLike(ExternalServiceTemplate item){
return new ExternalBootstrapServiceNestedImpl(item);
}
public KafkaClusterTemplateFluent.ExternalBootstrapServiceNested editExternalBootstrapService(){
return withNewExternalBootstrapServiceLike(getExternalBootstrapService());
}
public KafkaClusterTemplateFluent.ExternalBootstrapServiceNested editOrNewExternalBootstrapService(){
return withNewExternalBootstrapServiceLike(getExternalBootstrapService() != null ? getExternalBootstrapService(): new ExternalServiceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.ExternalBootstrapServiceNested editOrNewExternalBootstrapServiceLike(ExternalServiceTemplate item){
return withNewExternalBootstrapServiceLike(getExternalBootstrapService() != null ? getExternalBootstrapService(): item);
}
/**
* This method has been deprecated, please use method buildPerPodService instead.
* @return The buildable object.
*/
@Deprecated public ExternalServiceTemplate getPerPodService(){
return this.perPodService!=null?this.perPodService.build():null;
}
public ExternalServiceTemplate buildPerPodService(){
return this.perPodService!=null?this.perPodService.build():null;
}
public A withPerPodService(ExternalServiceTemplate perPodService){
_visitables.get("perPodService").remove(this.perPodService);
if (perPodService!=null){ this.perPodService= new ExternalServiceTemplateBuilder(perPodService); _visitables.get("perPodService").add(this.perPodService);} return (A) this;
}
public Boolean hasPerPodService(){
return this.perPodService != null;
}
public KafkaClusterTemplateFluent.PerPodServiceNested withNewPerPodService(){
return new PerPodServiceNestedImpl();
}
public KafkaClusterTemplateFluent.PerPodServiceNested withNewPerPodServiceLike(ExternalServiceTemplate item){
return new PerPodServiceNestedImpl(item);
}
public KafkaClusterTemplateFluent.PerPodServiceNested editPerPodService(){
return withNewPerPodServiceLike(getPerPodService());
}
public KafkaClusterTemplateFluent.PerPodServiceNested editOrNewPerPodService(){
return withNewPerPodServiceLike(getPerPodService() != null ? getPerPodService(): new ExternalServiceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.PerPodServiceNested editOrNewPerPodServiceLike(ExternalServiceTemplate item){
return withNewPerPodServiceLike(getPerPodService() != null ? getPerPodService(): item);
}
/**
* This method has been deprecated, please use method buildExternalBootstrapRoute instead.
* @return The buildable object.
*/
@Deprecated public ResourceTemplate getExternalBootstrapRoute(){
return this.externalBootstrapRoute!=null?this.externalBootstrapRoute.build():null;
}
public ResourceTemplate buildExternalBootstrapRoute(){
return this.externalBootstrapRoute!=null?this.externalBootstrapRoute.build():null;
}
public A withExternalBootstrapRoute(ResourceTemplate externalBootstrapRoute){
_visitables.get("externalBootstrapRoute").remove(this.externalBootstrapRoute);
if (externalBootstrapRoute!=null){ this.externalBootstrapRoute= new ResourceTemplateBuilder(externalBootstrapRoute); _visitables.get("externalBootstrapRoute").add(this.externalBootstrapRoute);} return (A) this;
}
public Boolean hasExternalBootstrapRoute(){
return this.externalBootstrapRoute != null;
}
public KafkaClusterTemplateFluent.ExternalBootstrapRouteNested withNewExternalBootstrapRoute(){
return new ExternalBootstrapRouteNestedImpl();
}
public KafkaClusterTemplateFluent.ExternalBootstrapRouteNested withNewExternalBootstrapRouteLike(ResourceTemplate item){
return new ExternalBootstrapRouteNestedImpl(item);
}
public KafkaClusterTemplateFluent.ExternalBootstrapRouteNested editExternalBootstrapRoute(){
return withNewExternalBootstrapRouteLike(getExternalBootstrapRoute());
}
public KafkaClusterTemplateFluent.ExternalBootstrapRouteNested editOrNewExternalBootstrapRoute(){
return withNewExternalBootstrapRouteLike(getExternalBootstrapRoute() != null ? getExternalBootstrapRoute(): new ResourceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.ExternalBootstrapRouteNested editOrNewExternalBootstrapRouteLike(ResourceTemplate item){
return withNewExternalBootstrapRouteLike(getExternalBootstrapRoute() != null ? getExternalBootstrapRoute(): item);
}
/**
* This method has been deprecated, please use method buildPerPodRoute instead.
* @return The buildable object.
*/
@Deprecated public ResourceTemplate getPerPodRoute(){
return this.perPodRoute!=null?this.perPodRoute.build():null;
}
public ResourceTemplate buildPerPodRoute(){
return this.perPodRoute!=null?this.perPodRoute.build():null;
}
public A withPerPodRoute(ResourceTemplate perPodRoute){
_visitables.get("perPodRoute").remove(this.perPodRoute);
if (perPodRoute!=null){ this.perPodRoute= new ResourceTemplateBuilder(perPodRoute); _visitables.get("perPodRoute").add(this.perPodRoute);} return (A) this;
}
public Boolean hasPerPodRoute(){
return this.perPodRoute != null;
}
public KafkaClusterTemplateFluent.PerPodRouteNested withNewPerPodRoute(){
return new PerPodRouteNestedImpl();
}
public KafkaClusterTemplateFluent.PerPodRouteNested withNewPerPodRouteLike(ResourceTemplate item){
return new PerPodRouteNestedImpl(item);
}
public KafkaClusterTemplateFluent.PerPodRouteNested editPerPodRoute(){
return withNewPerPodRouteLike(getPerPodRoute());
}
public KafkaClusterTemplateFluent.PerPodRouteNested editOrNewPerPodRoute(){
return withNewPerPodRouteLike(getPerPodRoute() != null ? getPerPodRoute(): new ResourceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.PerPodRouteNested editOrNewPerPodRouteLike(ResourceTemplate item){
return withNewPerPodRouteLike(getPerPodRoute() != null ? getPerPodRoute(): item);
}
/**
* This method has been deprecated, please use method buildExternalBootstrapIngress instead.
* @return The buildable object.
*/
@Deprecated public ResourceTemplate getExternalBootstrapIngress(){
return this.externalBootstrapIngress!=null?this.externalBootstrapIngress.build():null;
}
public ResourceTemplate buildExternalBootstrapIngress(){
return this.externalBootstrapIngress!=null?this.externalBootstrapIngress.build():null;
}
public A withExternalBootstrapIngress(ResourceTemplate externalBootstrapIngress){
_visitables.get("externalBootstrapIngress").remove(this.externalBootstrapIngress);
if (externalBootstrapIngress!=null){ this.externalBootstrapIngress= new ResourceTemplateBuilder(externalBootstrapIngress); _visitables.get("externalBootstrapIngress").add(this.externalBootstrapIngress);} return (A) this;
}
public Boolean hasExternalBootstrapIngress(){
return this.externalBootstrapIngress != null;
}
public KafkaClusterTemplateFluent.ExternalBootstrapIngressNested withNewExternalBootstrapIngress(){
return new ExternalBootstrapIngressNestedImpl();
}
public KafkaClusterTemplateFluent.ExternalBootstrapIngressNested withNewExternalBootstrapIngressLike(ResourceTemplate item){
return new ExternalBootstrapIngressNestedImpl(item);
}
public KafkaClusterTemplateFluent.ExternalBootstrapIngressNested editExternalBootstrapIngress(){
return withNewExternalBootstrapIngressLike(getExternalBootstrapIngress());
}
public KafkaClusterTemplateFluent.ExternalBootstrapIngressNested editOrNewExternalBootstrapIngress(){
return withNewExternalBootstrapIngressLike(getExternalBootstrapIngress() != null ? getExternalBootstrapIngress(): new ResourceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.ExternalBootstrapIngressNested editOrNewExternalBootstrapIngressLike(ResourceTemplate item){
return withNewExternalBootstrapIngressLike(getExternalBootstrapIngress() != null ? getExternalBootstrapIngress(): item);
}
/**
* This method has been deprecated, please use method buildPerPodIngress instead.
* @return The buildable object.
*/
@Deprecated public ResourceTemplate getPerPodIngress(){
return this.perPodIngress!=null?this.perPodIngress.build():null;
}
public ResourceTemplate buildPerPodIngress(){
return this.perPodIngress!=null?this.perPodIngress.build():null;
}
public A withPerPodIngress(ResourceTemplate perPodIngress){
_visitables.get("perPodIngress").remove(this.perPodIngress);
if (perPodIngress!=null){ this.perPodIngress= new ResourceTemplateBuilder(perPodIngress); _visitables.get("perPodIngress").add(this.perPodIngress);} return (A) this;
}
public Boolean hasPerPodIngress(){
return this.perPodIngress != null;
}
public KafkaClusterTemplateFluent.PerPodIngressNested withNewPerPodIngress(){
return new PerPodIngressNestedImpl();
}
public KafkaClusterTemplateFluent.PerPodIngressNested withNewPerPodIngressLike(ResourceTemplate item){
return new PerPodIngressNestedImpl(item);
}
public KafkaClusterTemplateFluent.PerPodIngressNested editPerPodIngress(){
return withNewPerPodIngressLike(getPerPodIngress());
}
public KafkaClusterTemplateFluent.PerPodIngressNested editOrNewPerPodIngress(){
return withNewPerPodIngressLike(getPerPodIngress() != null ? getPerPodIngress(): new ResourceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.PerPodIngressNested editOrNewPerPodIngressLike(ResourceTemplate item){
return withNewPerPodIngressLike(getPerPodIngress() != null ? getPerPodIngress(): item);
}
/**
* This method has been deprecated, please use method buildPersistentVolumeClaim instead.
* @return The buildable object.
*/
@Deprecated public ResourceTemplate getPersistentVolumeClaim(){
return this.persistentVolumeClaim!=null?this.persistentVolumeClaim.build():null;
}
public ResourceTemplate buildPersistentVolumeClaim(){
return this.persistentVolumeClaim!=null?this.persistentVolumeClaim.build():null;
}
public A withPersistentVolumeClaim(ResourceTemplate persistentVolumeClaim){
_visitables.get("persistentVolumeClaim").remove(this.persistentVolumeClaim);
if (persistentVolumeClaim!=null){ this.persistentVolumeClaim= new ResourceTemplateBuilder(persistentVolumeClaim); _visitables.get("persistentVolumeClaim").add(this.persistentVolumeClaim);} return (A) this;
}
public Boolean hasPersistentVolumeClaim(){
return this.persistentVolumeClaim != null;
}
public KafkaClusterTemplateFluent.PersistentVolumeClaimNested withNewPersistentVolumeClaim(){
return new PersistentVolumeClaimNestedImpl();
}
public KafkaClusterTemplateFluent.PersistentVolumeClaimNested withNewPersistentVolumeClaimLike(ResourceTemplate item){
return new PersistentVolumeClaimNestedImpl(item);
}
public KafkaClusterTemplateFluent.PersistentVolumeClaimNested editPersistentVolumeClaim(){
return withNewPersistentVolumeClaimLike(getPersistentVolumeClaim());
}
public KafkaClusterTemplateFluent.PersistentVolumeClaimNested editOrNewPersistentVolumeClaim(){
return withNewPersistentVolumeClaimLike(getPersistentVolumeClaim() != null ? getPersistentVolumeClaim(): new ResourceTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.PersistentVolumeClaimNested editOrNewPersistentVolumeClaimLike(ResourceTemplate item){
return withNewPersistentVolumeClaimLike(getPersistentVolumeClaim() != null ? getPersistentVolumeClaim(): item);
}
/**
* This method has been deprecated, please use method buildPodDisruptionBudget instead.
* @return The buildable object.
*/
@Deprecated public PodDisruptionBudgetTemplate getPodDisruptionBudget(){
return this.podDisruptionBudget!=null?this.podDisruptionBudget.build():null;
}
public PodDisruptionBudgetTemplate buildPodDisruptionBudget(){
return this.podDisruptionBudget!=null?this.podDisruptionBudget.build():null;
}
public A withPodDisruptionBudget(PodDisruptionBudgetTemplate podDisruptionBudget){
_visitables.get("podDisruptionBudget").remove(this.podDisruptionBudget);
if (podDisruptionBudget!=null){ this.podDisruptionBudget= new PodDisruptionBudgetTemplateBuilder(podDisruptionBudget); _visitables.get("podDisruptionBudget").add(this.podDisruptionBudget);} return (A) this;
}
public Boolean hasPodDisruptionBudget(){
return this.podDisruptionBudget != null;
}
public KafkaClusterTemplateFluent.PodDisruptionBudgetNested withNewPodDisruptionBudget(){
return new PodDisruptionBudgetNestedImpl();
}
public KafkaClusterTemplateFluent.PodDisruptionBudgetNested withNewPodDisruptionBudgetLike(PodDisruptionBudgetTemplate item){
return new PodDisruptionBudgetNestedImpl(item);
}
public KafkaClusterTemplateFluent.PodDisruptionBudgetNested editPodDisruptionBudget(){
return withNewPodDisruptionBudgetLike(getPodDisruptionBudget());
}
public KafkaClusterTemplateFluent.PodDisruptionBudgetNested editOrNewPodDisruptionBudget(){
return withNewPodDisruptionBudgetLike(getPodDisruptionBudget() != null ? getPodDisruptionBudget(): new PodDisruptionBudgetTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.PodDisruptionBudgetNested editOrNewPodDisruptionBudgetLike(PodDisruptionBudgetTemplate item){
return withNewPodDisruptionBudgetLike(getPodDisruptionBudget() != null ? getPodDisruptionBudget(): item);
}
/**
* This method has been deprecated, please use method buildKafkaContainer instead.
* @return The buildable object.
*/
@Deprecated public ContainerTemplate getKafkaContainer(){
return this.kafkaContainer!=null?this.kafkaContainer.build():null;
}
public ContainerTemplate buildKafkaContainer(){
return this.kafkaContainer!=null?this.kafkaContainer.build():null;
}
public A withKafkaContainer(ContainerTemplate kafkaContainer){
_visitables.get("kafkaContainer").remove(this.kafkaContainer);
if (kafkaContainer!=null){ this.kafkaContainer= new ContainerTemplateBuilder(kafkaContainer); _visitables.get("kafkaContainer").add(this.kafkaContainer);} return (A) this;
}
public Boolean hasKafkaContainer(){
return this.kafkaContainer != null;
}
public KafkaClusterTemplateFluent.KafkaContainerNested withNewKafkaContainer(){
return new KafkaContainerNestedImpl();
}
public KafkaClusterTemplateFluent.KafkaContainerNested withNewKafkaContainerLike(ContainerTemplate item){
return new KafkaContainerNestedImpl(item);
}
public KafkaClusterTemplateFluent.KafkaContainerNested editKafkaContainer(){
return withNewKafkaContainerLike(getKafkaContainer());
}
public KafkaClusterTemplateFluent.KafkaContainerNested editOrNewKafkaContainer(){
return withNewKafkaContainerLike(getKafkaContainer() != null ? getKafkaContainer(): new ContainerTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.KafkaContainerNested editOrNewKafkaContainerLike(ContainerTemplate item){
return withNewKafkaContainerLike(getKafkaContainer() != null ? getKafkaContainer(): item);
}
/**
* This method has been deprecated, please use method buildTlsSidecarContainer instead.
* @return The buildable object.
*/
@Deprecated public ContainerTemplate getTlsSidecarContainer(){
return this.tlsSidecarContainer!=null?this.tlsSidecarContainer.build():null;
}
public ContainerTemplate buildTlsSidecarContainer(){
return this.tlsSidecarContainer!=null?this.tlsSidecarContainer.build():null;
}
public A withTlsSidecarContainer(ContainerTemplate tlsSidecarContainer){
_visitables.get("tlsSidecarContainer").remove(this.tlsSidecarContainer);
if (tlsSidecarContainer!=null){ this.tlsSidecarContainer= new ContainerTemplateBuilder(tlsSidecarContainer); _visitables.get("tlsSidecarContainer").add(this.tlsSidecarContainer);} return (A) this;
}
public Boolean hasTlsSidecarContainer(){
return this.tlsSidecarContainer != null;
}
public KafkaClusterTemplateFluent.TlsSidecarContainerNested withNewTlsSidecarContainer(){
return new TlsSidecarContainerNestedImpl();
}
public KafkaClusterTemplateFluent.TlsSidecarContainerNested withNewTlsSidecarContainerLike(ContainerTemplate item){
return new TlsSidecarContainerNestedImpl(item);
}
public KafkaClusterTemplateFluent.TlsSidecarContainerNested editTlsSidecarContainer(){
return withNewTlsSidecarContainerLike(getTlsSidecarContainer());
}
public KafkaClusterTemplateFluent.TlsSidecarContainerNested editOrNewTlsSidecarContainer(){
return withNewTlsSidecarContainerLike(getTlsSidecarContainer() != null ? getTlsSidecarContainer(): new ContainerTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.TlsSidecarContainerNested editOrNewTlsSidecarContainerLike(ContainerTemplate item){
return withNewTlsSidecarContainerLike(getTlsSidecarContainer() != null ? getTlsSidecarContainer(): item);
}
/**
* This method has been deprecated, please use method buildInitContainer instead.
* @return The buildable object.
*/
@Deprecated public ContainerTemplate getInitContainer(){
return this.initContainer!=null?this.initContainer.build():null;
}
public ContainerTemplate buildInitContainer(){
return this.initContainer!=null?this.initContainer.build():null;
}
public A withInitContainer(ContainerTemplate initContainer){
_visitables.get("initContainer").remove(this.initContainer);
if (initContainer!=null){ this.initContainer= new ContainerTemplateBuilder(initContainer); _visitables.get("initContainer").add(this.initContainer);} return (A) this;
}
public Boolean hasInitContainer(){
return this.initContainer != null;
}
public KafkaClusterTemplateFluent.InitContainerNested withNewInitContainer(){
return new InitContainerNestedImpl();
}
public KafkaClusterTemplateFluent.InitContainerNested withNewInitContainerLike(ContainerTemplate item){
return new InitContainerNestedImpl(item);
}
public KafkaClusterTemplateFluent.InitContainerNested editInitContainer(){
return withNewInitContainerLike(getInitContainer());
}
public KafkaClusterTemplateFluent.InitContainerNested editOrNewInitContainer(){
return withNewInitContainerLike(getInitContainer() != null ? getInitContainer(): new ContainerTemplateBuilder().build());
}
public KafkaClusterTemplateFluent.InitContainerNested editOrNewInitContainerLike(ContainerTemplate item){
return withNewInitContainerLike(getInitContainer() != null ? getInitContainer(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
KafkaClusterTemplateFluentImpl that = (KafkaClusterTemplateFluentImpl) o;
if (statefulset != null ? !statefulset.equals(that.statefulset) :that.statefulset != null) return false;
if (pod != null ? !pod.equals(that.pod) :that.pod != null) return false;
if (bootstrapService != null ? !bootstrapService.equals(that.bootstrapService) :that.bootstrapService != null) return false;
if (brokersService != null ? !brokersService.equals(that.brokersService) :that.brokersService != null) return false;
if (externalBootstrapService != null ? !externalBootstrapService.equals(that.externalBootstrapService) :that.externalBootstrapService != null) return false;
if (perPodService != null ? !perPodService.equals(that.perPodService) :that.perPodService != null) return false;
if (externalBootstrapRoute != null ? !externalBootstrapRoute.equals(that.externalBootstrapRoute) :that.externalBootstrapRoute != null) return false;
if (perPodRoute != null ? !perPodRoute.equals(that.perPodRoute) :that.perPodRoute != null) return false;
if (externalBootstrapIngress != null ? !externalBootstrapIngress.equals(that.externalBootstrapIngress) :that.externalBootstrapIngress != null) return false;
if (perPodIngress != null ? !perPodIngress.equals(that.perPodIngress) :that.perPodIngress != null) return false;
if (persistentVolumeClaim != null ? !persistentVolumeClaim.equals(that.persistentVolumeClaim) :that.persistentVolumeClaim != null) return false;
if (podDisruptionBudget != null ? !podDisruptionBudget.equals(that.podDisruptionBudget) :that.podDisruptionBudget != null) return false;
if (kafkaContainer != null ? !kafkaContainer.equals(that.kafkaContainer) :that.kafkaContainer != null) return false;
if (tlsSidecarContainer != null ? !tlsSidecarContainer.equals(that.tlsSidecarContainer) :that.tlsSidecarContainer != null) return false;
if (initContainer != null ? !initContainer.equals(that.initContainer) :that.initContainer != null) return false;
return true;
}
public class StatefulsetNestedImpl extends StatefulSetTemplateFluentImpl> implements KafkaClusterTemplateFluent.StatefulsetNested,io.fabric8.kubernetes.api.builder.Nested{
private final StatefulSetTemplateBuilder builder;
StatefulsetNestedImpl(StatefulSetTemplate item){
this.builder = new StatefulSetTemplateBuilder(this, item);
}
StatefulsetNestedImpl(){
this.builder = new StatefulSetTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withStatefulset(builder.build());
}
public N endStatefulset(){
return and();
}
}
public class PodNestedImpl extends PodTemplateFluentImpl> implements KafkaClusterTemplateFluent.PodNested,io.fabric8.kubernetes.api.builder.Nested{
private final PodTemplateBuilder builder;
PodNestedImpl(PodTemplate item){
this.builder = new PodTemplateBuilder(this, item);
}
PodNestedImpl(){
this.builder = new PodTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withPod(builder.build());
}
public N endPod(){
return and();
}
}
public class BootstrapServiceNestedImpl extends ResourceTemplateFluentImpl> implements KafkaClusterTemplateFluent.BootstrapServiceNested,io.fabric8.kubernetes.api.builder.Nested{
private final ResourceTemplateBuilder builder;
BootstrapServiceNestedImpl(ResourceTemplate item){
this.builder = new ResourceTemplateBuilder(this, item);
}
BootstrapServiceNestedImpl(){
this.builder = new ResourceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withBootstrapService(builder.build());
}
public N endBootstrapService(){
return and();
}
}
public class BrokersServiceNestedImpl extends ResourceTemplateFluentImpl> implements KafkaClusterTemplateFluent.BrokersServiceNested,io.fabric8.kubernetes.api.builder.Nested{
private final ResourceTemplateBuilder builder;
BrokersServiceNestedImpl(ResourceTemplate item){
this.builder = new ResourceTemplateBuilder(this, item);
}
BrokersServiceNestedImpl(){
this.builder = new ResourceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withBrokersService(builder.build());
}
public N endBrokersService(){
return and();
}
}
public class ExternalBootstrapServiceNestedImpl extends ExternalServiceTemplateFluentImpl> implements KafkaClusterTemplateFluent.ExternalBootstrapServiceNested,io.fabric8.kubernetes.api.builder.Nested{
private final ExternalServiceTemplateBuilder builder;
ExternalBootstrapServiceNestedImpl(ExternalServiceTemplate item){
this.builder = new ExternalServiceTemplateBuilder(this, item);
}
ExternalBootstrapServiceNestedImpl(){
this.builder = new ExternalServiceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withExternalBootstrapService(builder.build());
}
public N endExternalBootstrapService(){
return and();
}
}
public class PerPodServiceNestedImpl extends ExternalServiceTemplateFluentImpl> implements KafkaClusterTemplateFluent.PerPodServiceNested,io.fabric8.kubernetes.api.builder.Nested{
private final ExternalServiceTemplateBuilder builder;
PerPodServiceNestedImpl(ExternalServiceTemplate item){
this.builder = new ExternalServiceTemplateBuilder(this, item);
}
PerPodServiceNestedImpl(){
this.builder = new ExternalServiceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withPerPodService(builder.build());
}
public N endPerPodService(){
return and();
}
}
public class ExternalBootstrapRouteNestedImpl extends ResourceTemplateFluentImpl> implements KafkaClusterTemplateFluent.ExternalBootstrapRouteNested,io.fabric8.kubernetes.api.builder.Nested{
private final ResourceTemplateBuilder builder;
ExternalBootstrapRouteNestedImpl(ResourceTemplate item){
this.builder = new ResourceTemplateBuilder(this, item);
}
ExternalBootstrapRouteNestedImpl(){
this.builder = new ResourceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withExternalBootstrapRoute(builder.build());
}
public N endExternalBootstrapRoute(){
return and();
}
}
public class PerPodRouteNestedImpl extends ResourceTemplateFluentImpl> implements KafkaClusterTemplateFluent.PerPodRouteNested,io.fabric8.kubernetes.api.builder.Nested{
private final ResourceTemplateBuilder builder;
PerPodRouteNestedImpl(ResourceTemplate item){
this.builder = new ResourceTemplateBuilder(this, item);
}
PerPodRouteNestedImpl(){
this.builder = new ResourceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withPerPodRoute(builder.build());
}
public N endPerPodRoute(){
return and();
}
}
public class ExternalBootstrapIngressNestedImpl extends ResourceTemplateFluentImpl> implements KafkaClusterTemplateFluent.ExternalBootstrapIngressNested,io.fabric8.kubernetes.api.builder.Nested{
private final ResourceTemplateBuilder builder;
ExternalBootstrapIngressNestedImpl(ResourceTemplate item){
this.builder = new ResourceTemplateBuilder(this, item);
}
ExternalBootstrapIngressNestedImpl(){
this.builder = new ResourceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withExternalBootstrapIngress(builder.build());
}
public N endExternalBootstrapIngress(){
return and();
}
}
public class PerPodIngressNestedImpl extends ResourceTemplateFluentImpl> implements KafkaClusterTemplateFluent.PerPodIngressNested,io.fabric8.kubernetes.api.builder.Nested{
private final ResourceTemplateBuilder builder;
PerPodIngressNestedImpl(ResourceTemplate item){
this.builder = new ResourceTemplateBuilder(this, item);
}
PerPodIngressNestedImpl(){
this.builder = new ResourceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withPerPodIngress(builder.build());
}
public N endPerPodIngress(){
return and();
}
}
public class PersistentVolumeClaimNestedImpl extends ResourceTemplateFluentImpl> implements KafkaClusterTemplateFluent.PersistentVolumeClaimNested,io.fabric8.kubernetes.api.builder.Nested{
private final ResourceTemplateBuilder builder;
PersistentVolumeClaimNestedImpl(ResourceTemplate item){
this.builder = new ResourceTemplateBuilder(this, item);
}
PersistentVolumeClaimNestedImpl(){
this.builder = new ResourceTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withPersistentVolumeClaim(builder.build());
}
public N endPersistentVolumeClaim(){
return and();
}
}
public class PodDisruptionBudgetNestedImpl extends PodDisruptionBudgetTemplateFluentImpl> implements KafkaClusterTemplateFluent.PodDisruptionBudgetNested,io.fabric8.kubernetes.api.builder.Nested{
private final PodDisruptionBudgetTemplateBuilder builder;
PodDisruptionBudgetNestedImpl(PodDisruptionBudgetTemplate item){
this.builder = new PodDisruptionBudgetTemplateBuilder(this, item);
}
PodDisruptionBudgetNestedImpl(){
this.builder = new PodDisruptionBudgetTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withPodDisruptionBudget(builder.build());
}
public N endPodDisruptionBudget(){
return and();
}
}
public class KafkaContainerNestedImpl extends ContainerTemplateFluentImpl> implements KafkaClusterTemplateFluent.KafkaContainerNested,io.fabric8.kubernetes.api.builder.Nested{
private final ContainerTemplateBuilder builder;
KafkaContainerNestedImpl(ContainerTemplate item){
this.builder = new ContainerTemplateBuilder(this, item);
}
KafkaContainerNestedImpl(){
this.builder = new ContainerTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withKafkaContainer(builder.build());
}
public N endKafkaContainer(){
return and();
}
}
public class TlsSidecarContainerNestedImpl extends ContainerTemplateFluentImpl> implements KafkaClusterTemplateFluent.TlsSidecarContainerNested,io.fabric8.kubernetes.api.builder.Nested{
private final ContainerTemplateBuilder builder;
TlsSidecarContainerNestedImpl(ContainerTemplate item){
this.builder = new ContainerTemplateBuilder(this, item);
}
TlsSidecarContainerNestedImpl(){
this.builder = new ContainerTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withTlsSidecarContainer(builder.build());
}
public N endTlsSidecarContainer(){
return and();
}
}
public class InitContainerNestedImpl extends ContainerTemplateFluentImpl> implements KafkaClusterTemplateFluent.InitContainerNested,io.fabric8.kubernetes.api.builder.Nested{
private final ContainerTemplateBuilder builder;
InitContainerNestedImpl(ContainerTemplate item){
this.builder = new ContainerTemplateBuilder(this, item);
}
InitContainerNestedImpl(){
this.builder = new ContainerTemplateBuilder(this);
}
public N and(){
return (N) KafkaClusterTemplateFluentImpl.this.withInitContainer(builder.build());
}
public N endInitContainer(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy