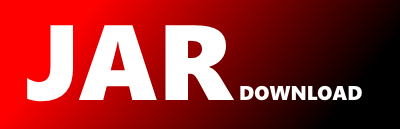
io.strimzi.api.kafka.model.template.PodTemplateFluentImpl Maven / Gradle / Ivy
package io.strimzi.api.kafka.model.template;
import java.lang.StringBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import io.fabric8.kubernetes.api.builder.Predicate;
import java.lang.Deprecated;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.List;
import java.lang.Boolean;
import io.fabric8.kubernetes.api.model.PodSecurityContext;
import io.fabric8.kubernetes.api.model.HostAlias;
import java.lang.StringBuffer;
import io.fabric8.kubernetes.api.model.LocalObjectReference;
import io.fabric8.kubernetes.api.model.Toleration;
import java.lang.Long;
import io.fabric8.kubernetes.api.model.Affinity;
import java.util.Collection;
import java.lang.Object;
public class PodTemplateFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements PodTemplateFluent{
private MetadataTemplateBuilder metadata;
private java.util.List imagePullSecrets;
private PodSecurityContext securityContext;
private int terminationGracePeriodSeconds;
private Affinity affinity;
private java.util.List tolerations;
private String priorityClassName;
private String schedulerName;
private java.util.List hostAliases;
public PodTemplateFluentImpl(){
}
public PodTemplateFluentImpl(PodTemplate instance){
this.withMetadata(instance.getMetadata());
this.withImagePullSecrets(instance.getImagePullSecrets());
this.withSecurityContext(instance.getSecurityContext());
this.withTerminationGracePeriodSeconds(instance.getTerminationGracePeriodSeconds());
this.withAffinity(instance.getAffinity());
this.withTolerations(instance.getTolerations());
this.withPriorityClassName(instance.getPriorityClassName());
this.withSchedulerName(instance.getSchedulerName());
this.withHostAliases(instance.getHostAliases());
}
/**
* This method has been deprecated, please use method buildMetadata instead.
* @return The buildable object.
*/
@Deprecated public MetadataTemplate getMetadata(){
return this.metadata!=null?this.metadata.build():null;
}
public MetadataTemplate buildMetadata(){
return this.metadata!=null?this.metadata.build():null;
}
public A withMetadata(MetadataTemplate metadata){
_visitables.get("metadata").remove(this.metadata);
if (metadata!=null){ this.metadata= new MetadataTemplateBuilder(metadata); _visitables.get("metadata").add(this.metadata);} return (A) this;
}
public Boolean hasMetadata(){
return this.metadata != null;
}
public PodTemplateFluent.MetadataNested withNewMetadata(){
return new MetadataNestedImpl();
}
public PodTemplateFluent.MetadataNested withNewMetadataLike(MetadataTemplate item){
return new MetadataNestedImpl(item);
}
public PodTemplateFluent.MetadataNested editMetadata(){
return withNewMetadataLike(getMetadata());
}
public PodTemplateFluent.MetadataNested editOrNewMetadata(){
return withNewMetadataLike(getMetadata() != null ? getMetadata(): new MetadataTemplateBuilder().build());
}
public PodTemplateFluent.MetadataNested editOrNewMetadataLike(MetadataTemplate item){
return withNewMetadataLike(getMetadata() != null ? getMetadata(): item);
}
public A addToImagePullSecrets(int index,LocalObjectReference item){
if (this.imagePullSecrets == null) {this.imagePullSecrets = new ArrayList();}
this.imagePullSecrets.add(index, item);
return (A)this;
}
public A setToImagePullSecrets(int index,LocalObjectReference item){
if (this.imagePullSecrets == null) {this.imagePullSecrets = new ArrayList();}
this.imagePullSecrets.set(index, item); return (A)this;
}
public A addToImagePullSecrets(LocalObjectReference... items){
if (this.imagePullSecrets == null) {this.imagePullSecrets = new ArrayList();}
for (LocalObjectReference item : items) {this.imagePullSecrets.add(item);} return (A)this;
}
public A addAllToImagePullSecrets(Collection items){
if (this.imagePullSecrets == null) {this.imagePullSecrets = new ArrayList();}
for (LocalObjectReference item : items) {this.imagePullSecrets.add(item);} return (A)this;
}
public A removeFromImagePullSecrets(LocalObjectReference... items){
for (LocalObjectReference item : items) {if (this.imagePullSecrets!= null){ this.imagePullSecrets.remove(item);}} return (A)this;
}
public A removeAllFromImagePullSecrets(Collection items){
for (LocalObjectReference item : items) {if (this.imagePullSecrets!= null){ this.imagePullSecrets.remove(item);}} return (A)this;
}
public java.util.List getImagePullSecrets(){
return this.imagePullSecrets;
}
public LocalObjectReference getImagePullSecret(int index){
return this.imagePullSecrets.get(index);
}
public LocalObjectReference getFirstImagePullSecret(){
return this.imagePullSecrets.get(0);
}
public LocalObjectReference getLastImagePullSecret(){
return this.imagePullSecrets.get(imagePullSecrets.size() - 1);
}
public LocalObjectReference getMatchingImagePullSecret(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (LocalObjectReference item: imagePullSecrets) { if(predicate.apply(item)){ return item;} } return null;
}
public Boolean hasMatchingImagePullSecret(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (LocalObjectReference item: imagePullSecrets) { if(predicate.apply(item)){ return true;} } return false;
}
public A withImagePullSecrets(java.util.List imagePullSecrets){
if (this.imagePullSecrets != null) { _visitables.get("imagePullSecrets").removeAll(this.imagePullSecrets);}
if (imagePullSecrets != null) {this.imagePullSecrets = new ArrayList(); for (LocalObjectReference item : imagePullSecrets){this.addToImagePullSecrets(item);}} else { this.imagePullSecrets = null;} return (A) this;
}
public A withImagePullSecrets(LocalObjectReference... imagePullSecrets){
if (this.imagePullSecrets != null) {this.imagePullSecrets.clear();}
if (imagePullSecrets != null) {for (LocalObjectReference item :imagePullSecrets){ this.addToImagePullSecrets(item);}} return (A) this;
}
public Boolean hasImagePullSecrets(){
return imagePullSecrets != null && !imagePullSecrets.isEmpty();
}
public A addNewImagePullSecret(String name){
return (A)addToImagePullSecrets(new LocalObjectReference(name));
}
public PodSecurityContext getSecurityContext(){
return this.securityContext;
}
public A withSecurityContext(PodSecurityContext securityContext){
this.securityContext=securityContext; return (A) this;
}
public Boolean hasSecurityContext(){
return this.securityContext != null;
}
public int getTerminationGracePeriodSeconds(){
return this.terminationGracePeriodSeconds;
}
public A withTerminationGracePeriodSeconds(int terminationGracePeriodSeconds){
this.terminationGracePeriodSeconds=terminationGracePeriodSeconds; return (A) this;
}
public Boolean hasTerminationGracePeriodSeconds(){
return true;
}
public Affinity getAffinity(){
return this.affinity;
}
public A withAffinity(Affinity affinity){
this.affinity=affinity; return (A) this;
}
public Boolean hasAffinity(){
return this.affinity != null;
}
public A addToTolerations(int index,Toleration item){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
this.tolerations.add(index, item);
return (A)this;
}
public A setToTolerations(int index,Toleration item){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
this.tolerations.set(index, item); return (A)this;
}
public A addToTolerations(Toleration... items){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
for (Toleration item : items) {this.tolerations.add(item);} return (A)this;
}
public A addAllToTolerations(Collection items){
if (this.tolerations == null) {this.tolerations = new ArrayList();}
for (Toleration item : items) {this.tolerations.add(item);} return (A)this;
}
public A removeFromTolerations(Toleration... items){
for (Toleration item : items) {if (this.tolerations!= null){ this.tolerations.remove(item);}} return (A)this;
}
public A removeAllFromTolerations(Collection items){
for (Toleration item : items) {if (this.tolerations!= null){ this.tolerations.remove(item);}} return (A)this;
}
public java.util.List getTolerations(){
return this.tolerations;
}
public Toleration getToleration(int index){
return this.tolerations.get(index);
}
public Toleration getFirstToleration(){
return this.tolerations.get(0);
}
public Toleration getLastToleration(){
return this.tolerations.get(tolerations.size() - 1);
}
public Toleration getMatchingToleration(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (Toleration item: tolerations) { if(predicate.apply(item)){ return item;} } return null;
}
public Boolean hasMatchingToleration(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (Toleration item: tolerations) { if(predicate.apply(item)){ return true;} } return false;
}
public A withTolerations(java.util.List tolerations){
if (this.tolerations != null) { _visitables.get("tolerations").removeAll(this.tolerations);}
if (tolerations != null) {this.tolerations = new ArrayList(); for (Toleration item : tolerations){this.addToTolerations(item);}} else { this.tolerations = null;} return (A) this;
}
public A withTolerations(Toleration... tolerations){
if (this.tolerations != null) {this.tolerations.clear();}
if (tolerations != null) {for (Toleration item :tolerations){ this.addToTolerations(item);}} return (A) this;
}
public Boolean hasTolerations(){
return tolerations != null && !tolerations.isEmpty();
}
public A addNewToleration(String effect,String key,String operator,Long tolerationSeconds,String value){
return (A)addToTolerations(new Toleration(effect, key, operator, tolerationSeconds, value));
}
public String getPriorityClassName(){
return this.priorityClassName;
}
public A withPriorityClassName(String priorityClassName){
this.priorityClassName=priorityClassName; return (A) this;
}
public Boolean hasPriorityClassName(){
return this.priorityClassName != null;
}
public A withNewPriorityClassName(String arg1){
return (A)withPriorityClassName(new String(arg1));
}
public A withNewPriorityClassName(StringBuilder arg1){
return (A)withPriorityClassName(new String(arg1));
}
public A withNewPriorityClassName(StringBuffer arg1){
return (A)withPriorityClassName(new String(arg1));
}
public String getSchedulerName(){
return this.schedulerName;
}
public A withSchedulerName(String schedulerName){
this.schedulerName=schedulerName; return (A) this;
}
public Boolean hasSchedulerName(){
return this.schedulerName != null;
}
public A withNewSchedulerName(String arg1){
return (A)withSchedulerName(new String(arg1));
}
public A withNewSchedulerName(StringBuilder arg1){
return (A)withSchedulerName(new String(arg1));
}
public A withNewSchedulerName(StringBuffer arg1){
return (A)withSchedulerName(new String(arg1));
}
public A addToHostAliases(int index,HostAlias item){
if (this.hostAliases == null) {this.hostAliases = new ArrayList();}
this.hostAliases.add(index, item);
return (A)this;
}
public A setToHostAliases(int index,HostAlias item){
if (this.hostAliases == null) {this.hostAliases = new ArrayList();}
this.hostAliases.set(index, item); return (A)this;
}
public A addToHostAliases(HostAlias... items){
if (this.hostAliases == null) {this.hostAliases = new ArrayList();}
for (HostAlias item : items) {this.hostAliases.add(item);} return (A)this;
}
public A addAllToHostAliases(Collection items){
if (this.hostAliases == null) {this.hostAliases = new ArrayList();}
for (HostAlias item : items) {this.hostAliases.add(item);} return (A)this;
}
public A removeFromHostAliases(HostAlias... items){
for (HostAlias item : items) {if (this.hostAliases!= null){ this.hostAliases.remove(item);}} return (A)this;
}
public A removeAllFromHostAliases(Collection items){
for (HostAlias item : items) {if (this.hostAliases!= null){ this.hostAliases.remove(item);}} return (A)this;
}
public java.util.List getHostAliases(){
return this.hostAliases;
}
public HostAlias getHostAlias(int index){
return this.hostAliases.get(index);
}
public HostAlias getFirstHostAlias(){
return this.hostAliases.get(0);
}
public HostAlias getLastHostAlias(){
return this.hostAliases.get(hostAliases.size() - 1);
}
public HostAlias getMatchingHostAlias(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (HostAlias item: hostAliases) { if(predicate.apply(item)){ return item;} } return null;
}
public Boolean hasMatchingHostAlias(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (HostAlias item: hostAliases) { if(predicate.apply(item)){ return true;} } return false;
}
public A withHostAliases(java.util.List hostAliases){
if (this.hostAliases != null) { _visitables.get("hostAliases").removeAll(this.hostAliases);}
if (hostAliases != null) {this.hostAliases = new ArrayList(); for (HostAlias item : hostAliases){this.addToHostAliases(item);}} else { this.hostAliases = null;} return (A) this;
}
public A withHostAliases(HostAlias... hostAliases){
if (this.hostAliases != null) {this.hostAliases.clear();}
if (hostAliases != null) {for (HostAlias item :hostAliases){ this.addToHostAliases(item);}} return (A) this;
}
public Boolean hasHostAliases(){
return hostAliases != null && !hostAliases.isEmpty();
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PodTemplateFluentImpl that = (PodTemplateFluentImpl) o;
if (metadata != null ? !metadata.equals(that.metadata) :that.metadata != null) return false;
if (imagePullSecrets != null ? !imagePullSecrets.equals(that.imagePullSecrets) :that.imagePullSecrets != null) return false;
if (securityContext != null ? !securityContext.equals(that.securityContext) :that.securityContext != null) return false;
if (terminationGracePeriodSeconds != that.terminationGracePeriodSeconds) return false;
if (affinity != null ? !affinity.equals(that.affinity) :that.affinity != null) return false;
if (tolerations != null ? !tolerations.equals(that.tolerations) :that.tolerations != null) return false;
if (priorityClassName != null ? !priorityClassName.equals(that.priorityClassName) :that.priorityClassName != null) return false;
if (schedulerName != null ? !schedulerName.equals(that.schedulerName) :that.schedulerName != null) return false;
if (hostAliases != null ? !hostAliases.equals(that.hostAliases) :that.hostAliases != null) return false;
return true;
}
public class MetadataNestedImpl extends MetadataTemplateFluentImpl> implements PodTemplateFluent.MetadataNested,io.fabric8.kubernetes.api.builder.Nested{
private final MetadataTemplateBuilder builder;
MetadataNestedImpl(MetadataTemplate item){
this.builder = new MetadataTemplateBuilder(this, item);
}
MetadataNestedImpl(){
this.builder = new MetadataTemplateBuilder(this);
}
public N and(){
return (N) PodTemplateFluentImpl.this.withMetadata(builder.build());
}
public N endMetadata(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy