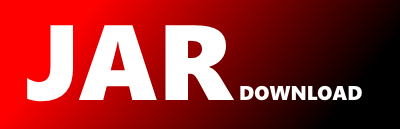
io.strimzi.api.kafka.model.jmxtrans.JmxTransOutputDefinitionTemplateFluent Maven / Gradle / Ivy
The newest version!
package io.strimzi.api.kafka.model.jmxtrans;
import java.lang.Integer;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.ArrayList;
import java.util.Collection;
import java.lang.Object;
import java.util.List;
import java.lang.String;
import java.util.function.Predicate;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class JmxTransOutputDefinitionTemplateFluent> extends BaseFluent{
public JmxTransOutputDefinitionTemplateFluent() {
}
public JmxTransOutputDefinitionTemplateFluent(JmxTransOutputDefinitionTemplate instance) {
this.copyInstance(instance);
}
private String outputType;
private String host;
private Integer port;
private Integer flushDelayInSeconds;
private String name;
private List typeNames;
protected void copyInstance(JmxTransOutputDefinitionTemplate instance) {
instance = (instance != null ? instance : new JmxTransOutputDefinitionTemplate());
if (instance != null) {
this.withOutputType(instance.getOutputType());
this.withHost(instance.getHost());
this.withPort(instance.getPort());
this.withFlushDelayInSeconds(instance.getFlushDelayInSeconds());
this.withName(instance.getName());
this.withTypeNames(instance.getTypeNames());
}
}
public String getOutputType() {
return this.outputType;
}
public A withOutputType(String outputType) {
this.outputType = outputType;
return (A) this;
}
public boolean hasOutputType() {
return this.outputType != null;
}
public String getHost() {
return this.host;
}
public A withHost(String host) {
this.host = host;
return (A) this;
}
public boolean hasHost() {
return this.host != null;
}
public Integer getPort() {
return this.port;
}
public A withPort(Integer port) {
this.port = port;
return (A) this;
}
public boolean hasPort() {
return this.port != null;
}
public Integer getFlushDelayInSeconds() {
return this.flushDelayInSeconds;
}
public A withFlushDelayInSeconds(Integer flushDelayInSeconds) {
this.flushDelayInSeconds = flushDelayInSeconds;
return (A) this;
}
public boolean hasFlushDelayInSeconds() {
return this.flushDelayInSeconds != null;
}
public String getName() {
return this.name;
}
public A withName(String name) {
this.name = name;
return (A) this;
}
public boolean hasName() {
return this.name != null;
}
public A addToTypeNames(int index,String item) {
if (this.typeNames == null) {this.typeNames = new ArrayList();}
this.typeNames.add(index, item);
return (A)this;
}
public A setToTypeNames(int index,String item) {
if (this.typeNames == null) {this.typeNames = new ArrayList();}
this.typeNames.set(index, item); return (A)this;
}
public A addToTypeNames(java.lang.String... items) {
if (this.typeNames == null) {this.typeNames = new ArrayList();}
for (String item : items) {this.typeNames.add(item);} return (A)this;
}
public A addAllToTypeNames(Collection items) {
if (this.typeNames == null) {this.typeNames = new ArrayList();}
for (String item : items) {this.typeNames.add(item);} return (A)this;
}
public A removeFromTypeNames(java.lang.String... items) {
if (this.typeNames == null) return (A)this;
for (String item : items) { this.typeNames.remove(item);} return (A)this;
}
public A removeAllFromTypeNames(Collection items) {
if (this.typeNames == null) return (A)this;
for (String item : items) { this.typeNames.remove(item);} return (A)this;
}
public List getTypeNames() {
return this.typeNames;
}
public String getTypeName(int index) {
return this.typeNames.get(index);
}
public String getFirstTypeName() {
return this.typeNames.get(0);
}
public String getLastTypeName() {
return this.typeNames.get(typeNames.size() - 1);
}
public String getMatchingTypeName(Predicate predicate) {
for (String item : typeNames) {
if (predicate.test(item)) {
return item;
}
}
return null;
}
public boolean hasMatchingTypeName(Predicate predicate) {
for (String item : typeNames) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withTypeNames(List typeNames) {
if (typeNames != null) {
this.typeNames = new ArrayList();
for (String item : typeNames) {
this.addToTypeNames(item);
}
} else {
this.typeNames = null;
}
return (A) this;
}
public A withTypeNames(java.lang.String... typeNames) {
if (this.typeNames != null) {
this.typeNames.clear();
_visitables.remove("typeNames");
}
if (typeNames != null) {
for (String item : typeNames) {
this.addToTypeNames(item);
}
}
return (A) this;
}
public boolean hasTypeNames() {
return this.typeNames != null && !this.typeNames.isEmpty();
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
JmxTransOutputDefinitionTemplateFluent that = (JmxTransOutputDefinitionTemplateFluent) o;
if (!java.util.Objects.equals(outputType, that.outputType)) return false;
if (!java.util.Objects.equals(host, that.host)) return false;
if (!java.util.Objects.equals(port, that.port)) return false;
if (!java.util.Objects.equals(flushDelayInSeconds, that.flushDelayInSeconds)) return false;
if (!java.util.Objects.equals(name, that.name)) return false;
if (!java.util.Objects.equals(typeNames, that.typeNames)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(outputType, host, port, flushDelayInSeconds, name, typeNames, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (outputType != null) { sb.append("outputType:"); sb.append(outputType + ","); }
if (host != null) { sb.append("host:"); sb.append(host + ","); }
if (port != null) { sb.append("port:"); sb.append(port + ","); }
if (flushDelayInSeconds != null) { sb.append("flushDelayInSeconds:"); sb.append(flushDelayInSeconds + ","); }
if (name != null) { sb.append("name:"); sb.append(name + ","); }
if (typeNames != null && !typeNames.isEmpty()) { sb.append("typeNames:"); sb.append(typeNames); }
sb.append("}");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy