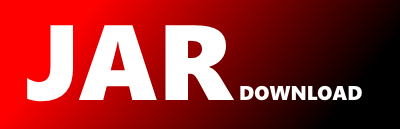
io.strimzi.api.kafka.model.zookeeper.ZookeeperClusterSpecFluent Maven / Gradle / Ivy
The newest version!
package io.strimzi.api.kafka.model.zookeeper;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.strimzi.api.kafka.model.common.metrics.JmxPrometheusExporterMetricsFluent;
import java.lang.String;
import io.strimzi.api.kafka.model.common.Probe;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import io.strimzi.api.kafka.model.kafka.PersistentClaimStorageFluent;
import java.util.LinkedHashMap;
import io.strimzi.api.kafka.model.kafka.PersistentClaimStorage;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.strimzi.api.kafka.model.common.Logging;
import io.strimzi.api.kafka.model.common.jmx.KafkaJmxOptionsFluent;
import io.strimzi.api.kafka.model.common.ProbeFluent;
import io.strimzi.api.kafka.model.common.jmx.KafkaJmxOptionsBuilder;
import io.strimzi.api.kafka.model.common.metrics.JmxPrometheusExporterMetricsBuilder;
import io.strimzi.api.kafka.model.kafka.SingleVolumeStorage;
import io.strimzi.api.kafka.model.common.JvmOptions;
import io.strimzi.api.kafka.model.common.InlineLoggingFluent;
import io.strimzi.api.kafka.model.kafka.EphemeralStorageBuilder;
import java.lang.Object;
import io.strimzi.api.kafka.model.kafka.EphemeralStorage;
import java.util.Map;
import io.strimzi.api.kafka.model.common.JvmOptionsFluent;
import io.strimzi.api.kafka.model.common.InlineLoggingBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import io.strimzi.api.kafka.model.common.JvmOptionsBuilder;
import io.strimzi.api.kafka.model.kafka.PersistentClaimStorageBuilder;
import io.strimzi.api.kafka.model.common.InlineLogging;
import io.strimzi.api.kafka.model.common.metrics.MetricsConfig;
import io.strimzi.api.kafka.model.common.ExternalLoggingBuilder;
import io.strimzi.api.kafka.model.common.ProbeBuilder;
import io.strimzi.api.kafka.model.common.metrics.JmxPrometheusExporterMetrics;
import io.strimzi.api.kafka.model.common.ExternalLoggingFluent;
import io.strimzi.api.kafka.model.kafka.EphemeralStorageFluent;
import io.strimzi.api.kafka.model.common.ExternalLogging;
import io.strimzi.api.kafka.model.common.jmx.KafkaJmxOptions;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class ZookeeperClusterSpecFluent> extends BaseFluent{
public ZookeeperClusterSpecFluent() {
}
public ZookeeperClusterSpecFluent(ZookeeperClusterSpec instance) {
this.copyInstance(instance);
}
private VisitableBuilder extends SingleVolumeStorage,?> storage;
private Map config;
private VisitableBuilder extends Logging,?> logging;
private int replicas;
private String image;
private ResourceRequirements resources;
private ProbeBuilder livenessProbe;
private ProbeBuilder readinessProbe;
private JvmOptionsBuilder jvmOptions;
private KafkaJmxOptionsBuilder jmxOptions;
private VisitableBuilder extends MetricsConfig,?> metricsConfig;
private ZookeeperClusterTemplateBuilder template;
protected void copyInstance(ZookeeperClusterSpec instance) {
instance = (instance != null ? instance : new ZookeeperClusterSpec());
if (instance != null) {
this.withStorage(instance.getStorage());
this.withConfig(instance.getConfig());
this.withLogging(instance.getLogging());
this.withReplicas(instance.getReplicas());
this.withImage(instance.getImage());
this.withResources(instance.getResources());
this.withLivenessProbe(instance.getLivenessProbe());
this.withReadinessProbe(instance.getReadinessProbe());
this.withJvmOptions(instance.getJvmOptions());
this.withJmxOptions(instance.getJmxOptions());
this.withMetricsConfig(instance.getMetricsConfig());
this.withTemplate(instance.getTemplate());
}
}
public SingleVolumeStorage buildStorage() {
return this.storage != null ? this.storage.build() : null;
}
public A withStorage(SingleVolumeStorage storage) {
if (storage == null) {
this.storage = null;
this._visitables.remove("storage");
return (A) this;
} else {
VisitableBuilder extends SingleVolumeStorage,?> builder = builder(storage);;
this._visitables.get("storage").clear();
this._visitables.get("storage").add(builder);
this.storage = builder;
return (A) this;
}
}
public boolean hasStorage() {
return this.storage != null;
}
public EphemeralStorageNested withNewEphemeralStorage() {
return new EphemeralStorageNested(null);
}
public EphemeralStorageNested withNewEphemeralStorageLike(EphemeralStorage item) {
return new EphemeralStorageNested(item);
}
public PersistentClaimStorageNested withNewPersistentClaimStorage() {
return new PersistentClaimStorageNested(null);
}
public PersistentClaimStorageNested withNewPersistentClaimStorageLike(PersistentClaimStorage item) {
return new PersistentClaimStorageNested(item);
}
public A addToConfig(String key,Object value) {
if(this.config == null && key != null && value != null) { this.config = new LinkedHashMap(); }
if(key != null && value != null) {this.config.put(key, value);} return (A)this;
}
public A addToConfig(Map map) {
if(this.config == null && map != null) { this.config = new LinkedHashMap(); }
if(map != null) { this.config.putAll(map);} return (A)this;
}
public A removeFromConfig(String key) {
if(this.config == null) { return (A) this; }
if(key != null && this.config != null) {this.config.remove(key);} return (A)this;
}
public A removeFromConfig(Map map) {
if(this.config == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.config != null){this.config.remove(key);}}} return (A)this;
}
public Map getConfig() {
return this.config;
}
public A withConfig(Map config) {
if (config == null) {
this.config = null;
} else {
this.config = new LinkedHashMap(config);
}
return (A) this;
}
public boolean hasConfig() {
return this.config != null;
}
public Logging buildLogging() {
return this.logging != null ? this.logging.build() : null;
}
public A withLogging(Logging logging) {
if (logging == null) {
this.logging = null;
this._visitables.remove("logging");
return (A) this;
} else {
VisitableBuilder extends Logging,?> builder = builder(logging);;
this._visitables.get("logging").clear();
this._visitables.get("logging").add(builder);
this.logging = builder;
return (A) this;
}
}
public boolean hasLogging() {
return this.logging != null;
}
public InlineLoggingNested withNewInlineLogging() {
return new InlineLoggingNested(null);
}
public InlineLoggingNested withNewInlineLoggingLike(InlineLogging item) {
return new InlineLoggingNested(item);
}
public ExternalLoggingNested withNewExternalLogging() {
return new ExternalLoggingNested(null);
}
public ExternalLoggingNested withNewExternalLoggingLike(ExternalLogging item) {
return new ExternalLoggingNested(item);
}
public int getReplicas() {
return this.replicas;
}
public A withReplicas(int replicas) {
this.replicas = replicas;
return (A) this;
}
public boolean hasReplicas() {
return true;
}
public String getImage() {
return this.image;
}
public A withImage(String image) {
this.image = image;
return (A) this;
}
public boolean hasImage() {
return this.image != null;
}
public ResourceRequirements getResources() {
return this.resources;
}
public A withResources(ResourceRequirements resources) {
this.resources = resources;
return (A) this;
}
public boolean hasResources() {
return this.resources != null;
}
public Probe buildLivenessProbe() {
return this.livenessProbe != null ? this.livenessProbe.build() : null;
}
public A withLivenessProbe(Probe livenessProbe) {
this._visitables.remove("livenessProbe");
if (livenessProbe != null) {
this.livenessProbe = new ProbeBuilder(livenessProbe);
this._visitables.get("livenessProbe").add(this.livenessProbe);
} else {
this.livenessProbe = null;
this._visitables.get("livenessProbe").remove(this.livenessProbe);
}
return (A) this;
}
public boolean hasLivenessProbe() {
return this.livenessProbe != null;
}
public A withNewLivenessProbe(int initialDelaySeconds,int timeoutSeconds) {
return (A)withLivenessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public LivenessProbeNested withNewLivenessProbe() {
return new LivenessProbeNested(null);
}
public LivenessProbeNested withNewLivenessProbeLike(Probe item) {
return new LivenessProbeNested(item);
}
public LivenessProbeNested editLivenessProbe() {
return withNewLivenessProbeLike(java.util.Optional.ofNullable(buildLivenessProbe()).orElse(null));
}
public LivenessProbeNested editOrNewLivenessProbe() {
return withNewLivenessProbeLike(java.util.Optional.ofNullable(buildLivenessProbe()).orElse(new ProbeBuilder().build()));
}
public LivenessProbeNested editOrNewLivenessProbeLike(Probe item) {
return withNewLivenessProbeLike(java.util.Optional.ofNullable(buildLivenessProbe()).orElse(item));
}
public Probe buildReadinessProbe() {
return this.readinessProbe != null ? this.readinessProbe.build() : null;
}
public A withReadinessProbe(Probe readinessProbe) {
this._visitables.remove("readinessProbe");
if (readinessProbe != null) {
this.readinessProbe = new ProbeBuilder(readinessProbe);
this._visitables.get("readinessProbe").add(this.readinessProbe);
} else {
this.readinessProbe = null;
this._visitables.get("readinessProbe").remove(this.readinessProbe);
}
return (A) this;
}
public boolean hasReadinessProbe() {
return this.readinessProbe != null;
}
public A withNewReadinessProbe(int initialDelaySeconds,int timeoutSeconds) {
return (A)withReadinessProbe(new Probe(initialDelaySeconds, timeoutSeconds));
}
public ReadinessProbeNested withNewReadinessProbe() {
return new ReadinessProbeNested(null);
}
public ReadinessProbeNested withNewReadinessProbeLike(Probe item) {
return new ReadinessProbeNested(item);
}
public ReadinessProbeNested editReadinessProbe() {
return withNewReadinessProbeLike(java.util.Optional.ofNullable(buildReadinessProbe()).orElse(null));
}
public ReadinessProbeNested editOrNewReadinessProbe() {
return withNewReadinessProbeLike(java.util.Optional.ofNullable(buildReadinessProbe()).orElse(new ProbeBuilder().build()));
}
public ReadinessProbeNested editOrNewReadinessProbeLike(Probe item) {
return withNewReadinessProbeLike(java.util.Optional.ofNullable(buildReadinessProbe()).orElse(item));
}
public JvmOptions buildJvmOptions() {
return this.jvmOptions != null ? this.jvmOptions.build() : null;
}
public A withJvmOptions(JvmOptions jvmOptions) {
this._visitables.remove("jvmOptions");
if (jvmOptions != null) {
this.jvmOptions = new JvmOptionsBuilder(jvmOptions);
this._visitables.get("jvmOptions").add(this.jvmOptions);
} else {
this.jvmOptions = null;
this._visitables.get("jvmOptions").remove(this.jvmOptions);
}
return (A) this;
}
public boolean hasJvmOptions() {
return this.jvmOptions != null;
}
public JvmOptionsNested withNewJvmOptions() {
return new JvmOptionsNested(null);
}
public JvmOptionsNested withNewJvmOptionsLike(JvmOptions item) {
return new JvmOptionsNested(item);
}
public JvmOptionsNested editJvmOptions() {
return withNewJvmOptionsLike(java.util.Optional.ofNullable(buildJvmOptions()).orElse(null));
}
public JvmOptionsNested editOrNewJvmOptions() {
return withNewJvmOptionsLike(java.util.Optional.ofNullable(buildJvmOptions()).orElse(new JvmOptionsBuilder().build()));
}
public JvmOptionsNested editOrNewJvmOptionsLike(JvmOptions item) {
return withNewJvmOptionsLike(java.util.Optional.ofNullable(buildJvmOptions()).orElse(item));
}
public KafkaJmxOptions buildJmxOptions() {
return this.jmxOptions != null ? this.jmxOptions.build() : null;
}
public A withJmxOptions(KafkaJmxOptions jmxOptions) {
this._visitables.remove("jmxOptions");
if (jmxOptions != null) {
this.jmxOptions = new KafkaJmxOptionsBuilder(jmxOptions);
this._visitables.get("jmxOptions").add(this.jmxOptions);
} else {
this.jmxOptions = null;
this._visitables.get("jmxOptions").remove(this.jmxOptions);
}
return (A) this;
}
public boolean hasJmxOptions() {
return this.jmxOptions != null;
}
public JmxOptionsNested withNewJmxOptions() {
return new JmxOptionsNested(null);
}
public JmxOptionsNested withNewJmxOptionsLike(KafkaJmxOptions item) {
return new JmxOptionsNested(item);
}
public JmxOptionsNested editJmxOptions() {
return withNewJmxOptionsLike(java.util.Optional.ofNullable(buildJmxOptions()).orElse(null));
}
public JmxOptionsNested editOrNewJmxOptions() {
return withNewJmxOptionsLike(java.util.Optional.ofNullable(buildJmxOptions()).orElse(new KafkaJmxOptionsBuilder().build()));
}
public JmxOptionsNested editOrNewJmxOptionsLike(KafkaJmxOptions item) {
return withNewJmxOptionsLike(java.util.Optional.ofNullable(buildJmxOptions()).orElse(item));
}
public MetricsConfig buildMetricsConfig() {
return this.metricsConfig != null ? this.metricsConfig.build() : null;
}
public A withMetricsConfig(MetricsConfig metricsConfig) {
if (metricsConfig == null) {
this.metricsConfig = null;
this._visitables.remove("metricsConfig");
return (A) this;
} else {
VisitableBuilder extends MetricsConfig,?> builder = builder(metricsConfig);;
this._visitables.get("metricsConfig").clear();
this._visitables.get("metricsConfig").add(builder);
this.metricsConfig = builder;
return (A) this;
}
}
public boolean hasMetricsConfig() {
return this.metricsConfig != null;
}
public JmxPrometheusExporterMetricsConfigNested withNewJmxPrometheusExporterMetricsConfig() {
return new JmxPrometheusExporterMetricsConfigNested(null);
}
public JmxPrometheusExporterMetricsConfigNested withNewJmxPrometheusExporterMetricsConfigLike(JmxPrometheusExporterMetrics item) {
return new JmxPrometheusExporterMetricsConfigNested(item);
}
public ZookeeperClusterTemplate buildTemplate() {
return this.template != null ? this.template.build() : null;
}
public A withTemplate(ZookeeperClusterTemplate template) {
this._visitables.remove("template");
if (template != null) {
this.template = new ZookeeperClusterTemplateBuilder(template);
this._visitables.get("template").add(this.template);
} else {
this.template = null;
this._visitables.get("template").remove(this.template);
}
return (A) this;
}
public boolean hasTemplate() {
return this.template != null;
}
public TemplateNested withNewTemplate() {
return new TemplateNested(null);
}
public TemplateNested withNewTemplateLike(ZookeeperClusterTemplate item) {
return new TemplateNested(item);
}
public TemplateNested editTemplate() {
return withNewTemplateLike(java.util.Optional.ofNullable(buildTemplate()).orElse(null));
}
public TemplateNested editOrNewTemplate() {
return withNewTemplateLike(java.util.Optional.ofNullable(buildTemplate()).orElse(new ZookeeperClusterTemplateBuilder().build()));
}
public TemplateNested editOrNewTemplateLike(ZookeeperClusterTemplate item) {
return withNewTemplateLike(java.util.Optional.ofNullable(buildTemplate()).orElse(item));
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ZookeeperClusterSpecFluent that = (ZookeeperClusterSpecFluent) o;
if (!java.util.Objects.equals(storage, that.storage)) return false;
if (!java.util.Objects.equals(config, that.config)) return false;
if (!java.util.Objects.equals(logging, that.logging)) return false;
if (replicas != that.replicas) return false;
if (!java.util.Objects.equals(image, that.image)) return false;
if (!java.util.Objects.equals(resources, that.resources)) return false;
if (!java.util.Objects.equals(livenessProbe, that.livenessProbe)) return false;
if (!java.util.Objects.equals(readinessProbe, that.readinessProbe)) return false;
if (!java.util.Objects.equals(jvmOptions, that.jvmOptions)) return false;
if (!java.util.Objects.equals(jmxOptions, that.jmxOptions)) return false;
if (!java.util.Objects.equals(metricsConfig, that.metricsConfig)) return false;
if (!java.util.Objects.equals(template, that.template)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(storage, config, logging, replicas, image, resources, livenessProbe, readinessProbe, jvmOptions, jmxOptions, metricsConfig, template, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (storage != null) { sb.append("storage:"); sb.append(storage + ","); }
if (config != null && !config.isEmpty()) { sb.append("config:"); sb.append(config + ","); }
if (logging != null) { sb.append("logging:"); sb.append(logging + ","); }
sb.append("replicas:"); sb.append(replicas + ",");
if (image != null) { sb.append("image:"); sb.append(image + ","); }
if (resources != null) { sb.append("resources:"); sb.append(resources + ","); }
if (livenessProbe != null) { sb.append("livenessProbe:"); sb.append(livenessProbe + ","); }
if (readinessProbe != null) { sb.append("readinessProbe:"); sb.append(readinessProbe + ","); }
if (jvmOptions != null) { sb.append("jvmOptions:"); sb.append(jvmOptions + ","); }
if (jmxOptions != null) { sb.append("jmxOptions:"); sb.append(jmxOptions + ","); }
if (metricsConfig != null) { sb.append("metricsConfig:"); sb.append(metricsConfig + ","); }
if (template != null) { sb.append("template:"); sb.append(template); }
sb.append("}");
return sb.toString();
}
protected static VisitableBuilder builder(Object item) {
switch (item.getClass().getName()) {
case "io.strimzi.api.kafka.model.kafka."+"EphemeralStorage": return (VisitableBuilder)new EphemeralStorageBuilder((EphemeralStorage) item);
case "io.strimzi.api.kafka.model.kafka."+"PersistentClaimStorage": return (VisitableBuilder)new PersistentClaimStorageBuilder((PersistentClaimStorage) item);
case "io.strimzi.api.kafka.model.common."+"InlineLogging": return (VisitableBuilder)new InlineLoggingBuilder((InlineLogging) item);
case "io.strimzi.api.kafka.model.common."+"ExternalLogging": return (VisitableBuilder)new ExternalLoggingBuilder((ExternalLogging) item);
case "io.strimzi.api.kafka.model.common.metrics."+"JmxPrometheusExporterMetrics": return (VisitableBuilder)new JmxPrometheusExporterMetricsBuilder((JmxPrometheusExporterMetrics) item);
}
return (VisitableBuilder)builderOf(item);
}
public class EphemeralStorageNested extends EphemeralStorageFluent> implements Nested{
EphemeralStorageNested(EphemeralStorage item) {
this.builder = new EphemeralStorageBuilder(this, item);
}
EphemeralStorageBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withStorage(builder.build());
}
public N endEphemeralStorage() {
return and();
}
}
public class PersistentClaimStorageNested extends PersistentClaimStorageFluent> implements Nested{
PersistentClaimStorageNested(PersistentClaimStorage item) {
this.builder = new PersistentClaimStorageBuilder(this, item);
}
PersistentClaimStorageBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withStorage(builder.build());
}
public N endPersistentClaimStorage() {
return and();
}
}
public class InlineLoggingNested extends InlineLoggingFluent> implements Nested{
InlineLoggingNested(InlineLogging item) {
this.builder = new InlineLoggingBuilder(this, item);
}
InlineLoggingBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withLogging(builder.build());
}
public N endInlineLogging() {
return and();
}
}
public class ExternalLoggingNested extends ExternalLoggingFluent> implements Nested{
ExternalLoggingNested(ExternalLogging item) {
this.builder = new ExternalLoggingBuilder(this, item);
}
ExternalLoggingBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withLogging(builder.build());
}
public N endExternalLogging() {
return and();
}
}
public class LivenessProbeNested extends ProbeFluent> implements Nested{
LivenessProbeNested(Probe item) {
this.builder = new ProbeBuilder(this, item);
}
ProbeBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withLivenessProbe(builder.build());
}
public N endLivenessProbe() {
return and();
}
}
public class ReadinessProbeNested extends ProbeFluent> implements Nested{
ReadinessProbeNested(Probe item) {
this.builder = new ProbeBuilder(this, item);
}
ProbeBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withReadinessProbe(builder.build());
}
public N endReadinessProbe() {
return and();
}
}
public class JvmOptionsNested extends JvmOptionsFluent> implements Nested{
JvmOptionsNested(JvmOptions item) {
this.builder = new JvmOptionsBuilder(this, item);
}
JvmOptionsBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withJvmOptions(builder.build());
}
public N endJvmOptions() {
return and();
}
}
public class JmxOptionsNested extends KafkaJmxOptionsFluent> implements Nested{
JmxOptionsNested(KafkaJmxOptions item) {
this.builder = new KafkaJmxOptionsBuilder(this, item);
}
KafkaJmxOptionsBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withJmxOptions(builder.build());
}
public N endJmxOptions() {
return and();
}
}
public class JmxPrometheusExporterMetricsConfigNested extends JmxPrometheusExporterMetricsFluent> implements Nested{
JmxPrometheusExporterMetricsConfigNested(JmxPrometheusExporterMetrics item) {
this.builder = new JmxPrometheusExporterMetricsBuilder(this, item);
}
JmxPrometheusExporterMetricsBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withMetricsConfig(builder.build());
}
public N endJmxPrometheusExporterMetricsConfig() {
return and();
}
}
public class TemplateNested extends ZookeeperClusterTemplateFluent> implements Nested{
TemplateNested(ZookeeperClusterTemplate item) {
this.builder = new ZookeeperClusterTemplateBuilder(this, item);
}
ZookeeperClusterTemplateBuilder builder;
public N and() {
return (N) ZookeeperClusterSpecFluent.this.withTemplate(builder.build());
}
public N endTemplate() {
return and();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy