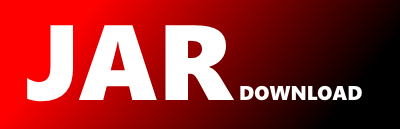
elements.index.cjs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mutation-testing-elements Show documentation
Show all versions of mutation-testing-elements Show documentation
A suite of web components for a mutation testing report.
"use strict";var Ki=Object.defineProperty;var qi=(r,e,t)=>e in r?Ki(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t;var x=(r,e,t)=>(qi(r,typeof e!="symbol"?e+"":e,t),t),Kt=(r,e,t)=>{if(!e.has(r))throw TypeError("Cannot "+t)};var I=(r,e,t)=>(Kt(r,e,"read from private field"),t?t.call(r):e.get(r)),ge=(r,e,t)=>{if(e.has(r))throw TypeError("Cannot add the same private member more than once");e instanceof WeakSet?e.add(r):e.set(r,t)},qt=(r,e,t,n)=>(Kt(r,e,"write to private field"),n?n.call(r,t):e.set(r,t),t);var Vt=(r,e,t)=>(Kt(r,e,"access private method"),t);Object.defineProperty(exports,Symbol.toStringTag,{value:"Module"});/**
* @license
* Copyright 2019 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const vt=globalThis,vr=vt.ShadowRoot&&(vt.ShadyCSS===void 0||vt.ShadyCSS.nativeShadow)&&"adoptedStyleSheets"in Document.prototype&&"replace"in CSSStyleSheet.prototype,xn=Symbol(),Lr=new WeakMap;let Vi=class{constructor(e,t,n){if(this._$cssResult$=!0,n!==xn)throw Error("CSSResult is not constructable. Use `unsafeCSS` or `css` instead.");this.cssText=e,this.t=t}get styleSheet(){let e=this.o;const t=this.t;if(vr&&e===void 0){const n=t!==void 0&&t.length===1;n&&(e=Lr.get(t)),e===void 0&&((this.o=e=new CSSStyleSheet).replaceSync(this.cssText),n&&Lr.set(t,e))}return e}toString(){return this.cssText}};const K=r=>new Vi(typeof r=="string"?r:r+"",void 0,xn),Wi=(r,e)=>{if(vr)r.adoptedStyleSheets=e.map(t=>t instanceof CSSStyleSheet?t:t.styleSheet);else for(const t of e){const n=document.createElement("style"),i=vt.litNonce;i!==void 0&&n.setAttribute("nonce",i),n.textContent=t.cssText,r.appendChild(n)}},Ur=vr?r=>r:r=>r instanceof CSSStyleSheet?(e=>{let t="";for(const n of e.cssRules)t+=n.cssText;return K(t)})(r):r;/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const{is:Zi,defineProperty:Gi,getOwnPropertyDescriptor:Yi,getOwnPropertyNames:Ji,getOwnPropertySymbols:Qi,getPrototypeOf:Xi}=Object,ce=globalThis,Hr=ce.trustedTypes,es=Hr?Hr.emptyScript:"",Wt=ce.reactiveElementPolyfillSupport,Ze=(r,e)=>r,$t={toAttribute(r,e){switch(e){case Boolean:r=r?es:null;break;case Object:case Array:r=r==null?r:JSON.stringify(r)}return r},fromAttribute(r,e){let t=r;switch(e){case Boolean:t=r!==null;break;case Number:t=r===null?null:Number(r);break;case Object:case Array:try{t=JSON.parse(r)}catch{t=null}}return t}},yr=(r,e)=>!Zi(r,e),Kr={attribute:!0,type:String,converter:$t,reflect:!1,hasChanged:yr};Symbol.metadata??(Symbol.metadata=Symbol("metadata")),ce.litPropertyMetadata??(ce.litPropertyMetadata=new WeakMap);let Oe=class extends HTMLElement{static addInitializer(e){this._$Ei(),(this.l??(this.l=[])).push(e)}static get observedAttributes(){return this.finalize(),this._$Eh&&[...this._$Eh.keys()]}static createProperty(e,t=Kr){if(t.state&&(t.attribute=!1),this._$Ei(),this.elementProperties.set(e,t),!t.noAccessor){const n=Symbol(),i=this.getPropertyDescriptor(e,n,t);i!==void 0&&Gi(this.prototype,e,i)}}static getPropertyDescriptor(e,t,n){const{get:i,set:s}=Yi(this.prototype,e)??{get(){return this[t]},set(a){this[t]=a}};return{get(){return i==null?void 0:i.call(this)},set(a){const o=i==null?void 0:i.call(this);s.call(this,a),this.requestUpdate(e,o,n)},configurable:!0,enumerable:!0}}static getPropertyOptions(e){return this.elementProperties.get(e)??Kr}static _$Ei(){if(this.hasOwnProperty(Ze("elementProperties")))return;const e=Xi(this);e.finalize(),e.l!==void 0&&(this.l=[...e.l]),this.elementProperties=new Map(e.elementProperties)}static finalize(){if(this.hasOwnProperty(Ze("finalized")))return;if(this.finalized=!0,this._$Ei(),this.hasOwnProperty(Ze("properties"))){const t=this.properties,n=[...Ji(t),...Qi(t)];for(const i of n)this.createProperty(i,t[i])}const e=this[Symbol.metadata];if(e!==null){const t=litPropertyMetadata.get(e);if(t!==void 0)for(const[n,i]of t)this.elementProperties.set(n,i)}this._$Eh=new Map;for(const[t,n]of this.elementProperties){const i=this._$Eu(t,n);i!==void 0&&this._$Eh.set(i,t)}this.elementStyles=this.finalizeStyles(this.styles)}static finalizeStyles(e){const t=[];if(Array.isArray(e)){const n=new Set(e.flat(1/0).reverse());for(const i of n)t.unshift(Ur(i))}else e!==void 0&&t.push(Ur(e));return t}static _$Eu(e,t){const n=t.attribute;return n===!1?void 0:typeof n=="string"?n:typeof e=="string"?e.toLowerCase():void 0}constructor(){super(),this._$Ep=void 0,this.isUpdatePending=!1,this.hasUpdated=!1,this._$Em=null,this._$Ev()}_$Ev(){var e;this._$Eg=new Promise(t=>this.enableUpdating=t),this._$AL=new Map,this._$E_(),this.requestUpdate(),(e=this.constructor.l)==null||e.forEach(t=>t(this))}addController(e){var t;(this._$ES??(this._$ES=[])).push(e),this.renderRoot!==void 0&&this.isConnected&&((t=e.hostConnected)==null||t.call(e))}removeController(e){var t;(t=this._$ES)==null||t.splice(this._$ES.indexOf(e)>>>0,1)}_$E_(){const e=new Map,t=this.constructor.elementProperties;for(const n of t.keys())this.hasOwnProperty(n)&&(e.set(n,this[n]),delete this[n]);e.size>0&&(this._$Ep=e)}createRenderRoot(){const e=this.shadowRoot??this.attachShadow(this.constructor.shadowRootOptions);return Wi(e,this.constructor.elementStyles),e}connectedCallback(){var e;this.renderRoot??(this.renderRoot=this.createRenderRoot()),this.enableUpdating(!0),(e=this._$ES)==null||e.forEach(t=>{var n;return(n=t.hostConnected)==null?void 0:n.call(t)})}enableUpdating(e){}disconnectedCallback(){var e;(e=this._$ES)==null||e.forEach(t=>{var n;return(n=t.hostDisconnected)==null?void 0:n.call(t)})}attributeChangedCallback(e,t,n){this._$AK(e,n)}_$EO(e,t){var s;const n=this.constructor.elementProperties.get(e),i=this.constructor._$Eu(e,n);if(i!==void 0&&n.reflect===!0){const a=(((s=n.converter)==null?void 0:s.toAttribute)!==void 0?n.converter:$t).toAttribute(t,n.type);this._$Em=e,a==null?this.removeAttribute(i):this.setAttribute(i,a),this._$Em=null}}_$AK(e,t){var s;const n=this.constructor,i=n._$Eh.get(e);if(i!==void 0&&this._$Em!==i){const a=n.getPropertyOptions(i),o=typeof a.converter=="function"?{fromAttribute:a.converter}:((s=a.converter)==null?void 0:s.fromAttribute)!==void 0?a.converter:$t;this._$Em=i,this[i]=o.fromAttribute(t,a.type),this._$Em=null}}requestUpdate(e,t,n,i=!1,s){if(e!==void 0){if(n??(n=this.constructor.getPropertyOptions(e)),!(n.hasChanged??yr)(i?s:this[e],t))return;this.C(e,t,n)}this.isUpdatePending===!1&&(this._$Eg=this._$EP())}C(e,t,n){this._$AL.has(e)||this._$AL.set(e,t),n.reflect===!0&&this._$Em!==e&&(this._$Ej??(this._$Ej=new Set)).add(e)}async _$EP(){this.isUpdatePending=!0;try{await this._$Eg}catch(t){Promise.reject(t)}const e=this.scheduleUpdate();return e!=null&&await e,!this.isUpdatePending}scheduleUpdate(){return this.performUpdate()}performUpdate(){var n;if(!this.isUpdatePending)return;if(!this.hasUpdated){if(this._$Ep){for(const[s,a]of this._$Ep)this[s]=a;this._$Ep=void 0}const i=this.constructor.elementProperties;if(i.size>0)for(const[s,a]of i)a.wrapped!==!0||this._$AL.has(s)||this[s]===void 0||this.C(s,this[s],a)}let e=!1;const t=this._$AL;try{e=this.shouldUpdate(t),e?(this.willUpdate(t),(n=this._$ES)==null||n.forEach(i=>{var s;return(s=i.hostUpdate)==null?void 0:s.call(i)}),this.update(t)):this._$ET()}catch(i){throw e=!1,this._$ET(),i}e&&this._$AE(t)}willUpdate(e){}_$AE(e){var t;(t=this._$ES)==null||t.forEach(n=>{var i;return(i=n.hostUpdated)==null?void 0:i.call(n)}),this.hasUpdated||(this.hasUpdated=!0,this.firstUpdated(e)),this.updated(e)}_$ET(){this._$AL=new Map,this.isUpdatePending=!1}get updateComplete(){return this.getUpdateComplete()}getUpdateComplete(){return this._$Eg}shouldUpdate(e){return!0}update(e){this._$Ej&&(this._$Ej=this._$Ej.forEach(t=>this._$EO(t,this[t]))),this._$ET()}updated(e){}firstUpdated(e){}};Oe.elementStyles=[],Oe.shadowRootOptions={mode:"open"},Oe[Ze("elementProperties")]=new Map,Oe[Ze("finalized")]=new Map,Wt==null||Wt({ReactiveElement:Oe}),(ce.reactiveElementVersions??(ce.reactiveElementVersions=[])).push("2.0.1");/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const Ge=globalThis,kt=Ge.trustedTypes,qr=kt?kt.createPolicy("lit-html",{createHTML:r=>r}):void 0,wr="$lit$",ee=`lit$${(Math.random()+"").slice(9)}$`,xr="?"+ee,ts=`<${xr}>`,_e=document,Qe=()=>_e.createComment(""),Xe=r=>r===null||typeof r!="object"&&typeof r!="function",$n=Array.isArray,kn=r=>$n(r)||typeof(r==null?void 0:r[Symbol.iterator])=="function",Zt=`[
\f\r]`,qe=/<(?:(!--|\/[^a-zA-Z])|(\/?[a-zA-Z][^>\s]*)|(\/?$))/g,Vr=/-->/g,Wr=/>/g,me=RegExp(`>|${Zt}(?:([^\\s"'>=/]+)(${Zt}*=${Zt}*(?:[^
\f\r"'\`<>=]|("|')|))|$)`,"g"),Zr=/'/g,Gr=/"/g,_n=/^(?:script|style|textarea|title)$/i,An=r=>(e,...t)=>({_$litType$:r,strings:e,values:t}),v=An(1),N=An(2),re=Symbol.for("lit-noChange"),A=Symbol.for("lit-nothing"),Yr=new WeakMap,ye=_e.createTreeWalker(_e,129);function Sn(r,e){if(!Array.isArray(r)||!r.hasOwnProperty("raw"))throw Error("invalid template strings array");return qr!==void 0?qr.createHTML(e):e}const Cn=(r,e)=>{const t=r.length-1,n=[];let i,s=e===2?"":"")),n]};class et{constructor({strings:e,_$litType$:t},n){let i;this.parts=[];let s=0,a=0;const o=e.length-1,l=this.parts,[c,g]=Cn(e,t);if(this.el=et.createElement(c,n),ye.currentNode=this.el.content,t===2){const d=this.el.content.firstChild;d.replaceWith(...d.childNodes)}for(;(i=ye.nextNode())!==null&&l.length0){i.textContent=kt?kt.emptyScript:"";for(let y=0;y2||n[0]!==""||n[1]!==""?(this._$AH=Array(n.length-1).fill(new String),this.strings=n):this._$AH=A}_$AI(e,t=this,n,i){const s=this.strings;let a=!1;if(s===void 0)e=Ae(this,e,t,0),a=!Xe(e)||e!==this._$AH&&e!==re,a&&(this._$AH=e);else{const o=e;let l,c;for(e=s[0],l=0;l{const n=(t==null?void 0:t.renderBefore)??e;let i=n._$litPart$;if(i===void 0){const s=(t==null?void 0:t.renderBefore)??null;n._$litPart$=i=new De(e.insertBefore(Qe(),s),s,void 0,t??{})}return i._$AI(r),i};/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/let W=class extends Oe{constructor(){super(...arguments),this.renderOptions={host:this},this._$Do=void 0}createRenderRoot(){var t;const e=super.createRenderRoot();return(t=this.renderOptions).renderBefore??(t.renderBefore=e.firstChild),e}update(e){const t=this.render();this.hasUpdated||(this.renderOptions.isConnected=this.isConnected),super.update(e),this._$Do=ns(t,this.renderRoot,this.renderOptions)}connectedCallback(){var e;super.connectedCallback(),(e=this._$Do)==null||e.setConnected(!0)}disconnectedCallback(){var e;super.disconnectedCallback(),(e=this._$Do)==null||e.setConnected(!1)}render(){return re}};var wn;W._$litElement$=!0,W.finalized=!0,(wn=globalThis.litElementHydrateSupport)==null||wn.call(globalThis,{LitElement:W});const Yt=globalThis.litElementPolyfillSupport;Yt==null||Yt({LitElement:W});(globalThis.litElementVersions??(globalThis.litElementVersions=[])).push("4.0.1");/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const D=r=>(e,t)=>{t!==void 0?t.addInitializer(()=>{customElements.define(r,e)}):customElements.define(r,e)};/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const is={attribute:!0,type:String,converter:$t,reflect:!1,hasChanged:yr},ss=(r=is,e,t)=>{const{kind:n,metadata:i}=t;let s=globalThis.litPropertyMetadata.get(i);if(s===void 0&&globalThis.litPropertyMetadata.set(i,s=new Map),s.set(t.name,r),n==="accessor"){const{name:a}=t;return{set(o){const l=e.get.call(this);e.set.call(this,o),this.requestUpdate(a,l,r)},init(o){return o!==void 0&&this.C(a,void 0,r),o}}}if(n==="setter"){const{name:a}=t;return function(o){const l=this[a];e.call(this,o),this.requestUpdate(a,l,r)}}throw Error("Unsupported decorator location: "+n)};function S(r){return(e,t)=>typeof t=="object"?ss(r,e,t):((n,i,s)=>{const a=i.hasOwnProperty(s);return i.constructor.createProperty(s,a?{...n,wrapped:!0}:n),a?Object.getOwnPropertyDescriptor(i,s):void 0})(r,e,t)}/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/function Z(r){return S({...r,state:!0,attribute:!1})}function as(r,e){return r.reduce((t,n)=>{const i=e(n);return Object.prototype.hasOwnProperty.call(t,i)||(t[i]=[]),t[i].push(n),t},{})}const os="/";function Jr(r,e,t){const n=Object.keys(r),i=ls(n),s=Object.create(null);return n.forEach(a=>{const o=Qr(a.startsWith(e)?a.substr(e.length):a);s[Qr(a.substr(i.length))]=t(r[a],o)}),s}function Qr(r){return r.split(/\/|\\/).filter(Boolean).join("/")}function ls(r){const e=r.map(n=>n.split(/\/|\\/).slice(0,-1));if(r.length)return e.reduce(t).join(os);return"";function t(n,i){for(let s=0;sn.file?`1${n.name}`:`0${n.name}`;return t(r).localeCompare(t(e))}var Q;(function(r){r[r.maxAsciiCharacter=127]="maxAsciiCharacter",r[r.lineFeed=10]="lineFeed",r[r.carriageReturn=13]="carriageReturn",r[r.lineSeparator=8232]="lineSeparator",r[r.paragraphSeparator=8233]="paragraphSeparator"})(Q||(Q={}));function us(r){return r===Q.lineFeed||r===Q.carriageReturn||r===Q.lineSeparator||r===Q.paragraphSeparator}function ds(r){const e=[];let t=0,n=0;function i(s){e.push(n),n=s}for(i(0);tQ.maxAsciiCharacter&&us(s)&&i(t);break}}return e.push(n),e}function Jt(r){if(r===void 0)throw new Error("mutant.sourceFile was not defined")}var we,xe;class ps{constructor(e){x(this,"coveredBy");x(this,"description");x(this,"duration");x(this,"id");x(this,"killedBy");x(this,"location");x(this,"mutatorName");x(this,"replacement");x(this,"static");x(this,"status");x(this,"statusReason");x(this,"testsCompleted");ge(this,we,new Map);ge(this,xe,new Map);this.coveredBy=e.coveredBy,this.description=e.description,this.duration=e.duration,this.id=e.id,this.killedBy=e.killedBy,this.location=e.location,this.mutatorName=e.mutatorName,this.replacement=e.replacement,this.static=e.static,this.status=e.status,this.statusReason=e.statusReason,this.testsCompleted=e.testsCompleted}get coveredByTests(){if(I(this,we).size)return Array.from(I(this,we).values())}set coveredByTests(e){qt(this,we,new Map(e.map(t=>[t.id,t])))}get killedByTests(){if(I(this,xe).size)return Array.from(I(this,xe).values())}set killedByTests(e){qt(this,xe,new Map(e.map(t=>[t.id,t])))}addCoveredBy(e){I(this,we).set(e.id,e)}addKilledBy(e){I(this,xe).set(e.id,e)}getMutatedLines(){return Jt(this.sourceFile),this.sourceFile.getMutationLines(this)}getOriginalLines(){return Jt(this.sourceFile),this.sourceFile.getLines(this.location)}get fileName(){return Jt(this.sourceFile),this.sourceFile.name}update(){var e,t;(t=(e=this.sourceFile)==null?void 0:e.result)!=null&&t.file&&this.sourceFile.result.updateAllMetrics()}}we=new WeakMap,xe=new WeakMap;function Xr(r){if(r===void 0)throw new Error("sourceFile.source is undefined")}class zn{constructor(){x(this,"lineMap")}getLineMap(){return Xr(this.source),this.lineMap??(this.lineMap=ds(this.source))}getLines(e){Xr(this.source);const t=this.getLineMap();return this.source.substring(t[e.start.line],t[(e.end??e.start).line+1])}}class hs extends zn{constructor(t,n){super();x(this,"name");x(this,"language");x(this,"source");x(this,"mutants");x(this,"result");this.name=n,this.language=t.language,this.source=t.source,this.mutants=t.mutants.map(i=>{const s=new ps(i);return s.sourceFile=this,s})}getMutationLines(t){const n=this.getLineMap(),i=n[t.location.start.line],s=n[t.location.end.line],a=n[t.location.end.line+1];return`${this.source.substr(i,t.location.start.column-1)}${t.replacement??t.description??t.mutatorName}${this.source.substring(s+t.location.end.column-1,a)}`}}var ot,ar;class On{constructor(e,t,n,i){ge(this,ot);x(this,"parent");x(this,"name");x(this,"file");x(this,"childResults");x(this,"metrics");this.name=e,this.childResults=t,this.metrics=n,this.file=i}updateParent(e){this.parent=e,this.childResults.forEach(t=>t.updateParent(this))}updateAllMetrics(){if(this.parent!==void 0){this.parent.updateAllMetrics();return}this.updateMetrics()}updateMetrics(){if(this.file===void 0){this.childResults.forEach(t=>{t.updateMetrics()});const e=Vt(this,ot,ar).call(this,this.childResults);if(e.length===0)return;e[0].tests?this.metrics=or(e):this.metrics=_t(e);return}this.file.tests?this.metrics=or([this.file]):this.metrics=_t([this.file])}}ot=new WeakSet,ar=function(e){const t=[];return e.length===0||e.forEach(n=>{if(n.file){t.push(n.file);return}t.push(...Vt(this,ot,ar).call(this,n.childResults))}),t};function en(r){if(r===void 0)throw new Error("test.sourceFile was not defined")}function fs(r){if(r===void 0)throw new Error("test.location was not defined")}var j;(function(r){r.Killing="Killing",r.Covering="Covering",r.NotCovering="NotCovering"})(j||(j={}));var $e,ke;class gs{constructor(e){x(this,"id");x(this,"name");x(this,"location");ge(this,$e,new Map);ge(this,ke,new Map);Object.entries(e).forEach(([t,n])=>{this[t]=n})}get killedMutants(){if(I(this,$e).size)return Array.from(I(this,$e).values())}get coveredMutants(){if(I(this,ke).size)return Array.from(I(this,ke).values())}addCovered(e){I(this,ke).set(e.id,e)}addKilled(e){I(this,$e).set(e.id,e)}getLines(){return en(this.sourceFile),fs(this.location),this.sourceFile.getLines(this.location)}get fileName(){return en(this.sourceFile),this.sourceFile.name}get status(){return I(this,$e).size?j.Killing:I(this,ke).size?j.Covering:j.NotCovering}update(){var e,t;(t=(e=this.sourceFile)==null?void 0:e.result)!=null&&t.file&&this.sourceFile.result.updateAllMetrics()}}$e=new WeakMap,ke=new WeakMap;class ms extends zn{constructor(t,n){super();x(this,"name");x(this,"tests");x(this,"source");x(this,"result");this.name=n,this.source=t.source,this.tests=t.tests.map(i=>{const s=new gs(i);return s.sourceFile=this,s})}}const tn=NaN,rn="All files",bs="All tests";function vs(r){const{files:e,testFiles:t,projectRoot:n=""}=r,i=Jr(e,n,(s,a)=>new hs(s,a));if(t&&Object.keys(t).length){const s=Jr(t,n,(a,o)=>new ms(a,o));return ws(Object.values(i).flatMap(a=>a.mutants),Object.values(s).flatMap(a=>a.tests)),{systemUnderTestMetrics:Qt(rn,i,_t),testMetrics:Qt(bs,s,or)}}return{systemUnderTestMetrics:Qt(rn,i,_t),testMetrics:void 0}}function Qt(r,e,t){const n=Object.keys(e);return n.length===1&&n[0]===""?jn(r,e[n[0]],t):Rn(r,e,t)}function Rn(r,e,t){const n=t(Object.values(e)),i=ys(e,t);return new On(r,i,n)}function jn(r,e,t){return new On(r,[],t([e]),e)}function ys(r,e){const t=as(Object.entries(r),n=>n[0].split("/")[0]);return Object.keys(t).map(n=>{if(t[n].length>1||t[n][0][0]!==n){const i={};return t[n].forEach(s=>i[s[0].substr(n.length+1)]=s[1]),Rn(n,i,e)}else{const[i,s]=t[n][0];return jn(i,s,e)}}).sort(cs)}function ws(r,e){const t=new Map(e.map(n=>[n.id,n]));for(const n of r){const i=n.coveredBy??[];for(const a of i){const o=t.get(a);o&&(n.addCoveredBy(o),o.addCovered(n))}const s=n.killedBy??[];for(const a of s){const o=t.get(a);o&&(n.addKilledBy(o),o.addKilled(n))}}}function or(r){const e=r.flatMap(n=>n.tests),t=n=>e.filter(i=>i.status===n).length;return{total:e.length,killing:t(j.Killing),covering:t(j.Covering),notCovering:t(j.NotCovering)}}function _t(r){const e=r.flatMap(F=>F.mutants),t=F=>e.filter(z=>z.status===F).length,n=t("Pending"),i=t("Killed"),s=t("Timeout"),a=t("Survived"),o=t("NoCoverage"),l=t("RuntimeError"),c=t("CompileError"),g=t("Ignored"),d=s+i,m=a+o,y=d+a,$=m+d,k=l+c;return{pending:n,killed:i,timeout:s,survived:a,noCoverage:o,runtimeErrors:l,compileErrors:c,ignored:g,totalDetected:d,totalUndetected:m,totalCovered:y,totalValid:$,totalInvalid:k,mutationScore:$>0?d/$*100:tn,totalMutants:$+k+g+n,mutationScoreBasedOnCoveredCode:$>0?d/y*100||0:tn}}const xs=`/*! tailwindcss v3.3.5 | MIT License | https://tailwindcss.com*/*,:after,:before{border-color:rgb(var(--mut-gray-200,228 228 231)/1);border-style:solid;border-width:0;box-sizing:border-box}:after,:before{--tw-content:""}html{-webkit-text-size-adjust:100%;font-feature-settings:normal;font-family:ui-sans-serif,system-ui,-apple-system,BlinkMacSystemFont,Segoe UI,Roboto,Helvetica Neue,Arial,Noto Sans,sans-serif,Apple Color Emoji,Segoe UI Emoji,Segoe UI Symbol,Noto Color Emoji;font-variation-settings:normal;line-height:1.5;-moz-tab-size:4;tab-size:4}body{line-height:inherit;margin:0}hr{border-top-width:1px;color:inherit;height:0}abbr:where([title]){-webkit-text-decoration:underline dotted;text-decoration:underline dotted}h1,h2,h3,h4,h5,h6{font-size:inherit;font-weight:inherit}a{color:inherit;text-decoration:inherit}b,strong{font-weight:bolder}code,kbd,pre,samp{font-family:ui-monospace,SFMono-Regular,Menlo,Monaco,Consolas,Liberation Mono,Courier New,monospace;font-size:1em}small{font-size:80%}sub,sup{font-size:75%;line-height:0;position:relative;vertical-align:baseline}sub{bottom:-.25em}sup{top:-.5em}table{border-collapse:collapse;border-color:inherit;text-indent:0}button,input,optgroup,select,textarea{font-feature-settings:inherit;color:inherit;font-family:inherit;font-size:100%;font-variation-settings:inherit;font-weight:inherit;line-height:inherit;margin:0;padding:0}button,select{text-transform:none}[type=button],[type=reset],[type=submit],button{-webkit-appearance:button;background-color:transparent;background-image:none}:-moz-focusring{outline:auto}:-moz-ui-invalid{box-shadow:none}progress{vertical-align:baseline}::-webkit-inner-spin-button,::-webkit-outer-spin-button{height:auto}[type=search]{-webkit-appearance:textfield;outline-offset:-2px}::-webkit-search-decoration{-webkit-appearance:none}::-webkit-file-upload-button{-webkit-appearance:button;font:inherit}summary{display:list-item}blockquote,dd,dl,figure,h1,h2,h3,h4,h5,h6,hr,p,pre{margin:0}fieldset{margin:0}fieldset,legend{padding:0}menu,ol,ul{list-style:none;margin:0;padding:0}dialog{padding:0}textarea{resize:vertical}input::placeholder,textarea::placeholder{color:rgb(var(--mut-gray-400,161 161 170)/1);opacity:1}[role=button],button{cursor:pointer}:disabled{cursor:default}audio,canvas,embed,iframe,img,object,svg,video{display:block;vertical-align:middle}img,video{height:auto;max-width:100%}[hidden]{display:none}[multiple],[type=date],[type=datetime-local],[type=email],[type=month],[type=number],[type=password],[type=search],[type=tel],[type=text],[type=time],[type=url],[type=week],input:where(:not([type])),select,textarea{--tw-shadow:0 0 #0000;-webkit-appearance:none;-moz-appearance:none;appearance:none;background-color:#fff;border-color:rgb(var(--mut-gray-500,113 113 122)/var(--tw-border-opacity,1));border-radius:0;border-width:1px;font-size:1rem;line-height:1.5rem;padding:.5rem .75rem}[multiple]:focus,[type=date]:focus,[type=datetime-local]:focus,[type=email]:focus,[type=month]:focus,[type=number]:focus,[type=password]:focus,[type=search]:focus,[type=tel]:focus,[type=text]:focus,[type=time]:focus,[type=url]:focus,[type=week]:focus,input:where(:not([type])):focus,select:focus,textarea:focus{--tw-ring-inset:var(--tw-empty, );--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:#2563eb;--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(1px + var(--tw-ring-offset-width)) var(--tw-ring-color);border-color:#2563eb;box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow);outline:2px solid transparent;outline-offset:2px}input::placeholder,textarea::placeholder{color:rgb(var(--mut-gray-500,113 113 122)/var(--tw-text-opacity,1));opacity:1}::-webkit-datetime-edit-fields-wrapper{padding:0}::-webkit-date-and-time-value{min-height:1.5em;text-align:inherit}::-webkit-datetime-edit{display:inline-flex}::-webkit-datetime-edit,::-webkit-datetime-edit-day-field,::-webkit-datetime-edit-hour-field,::-webkit-datetime-edit-meridiem-field,::-webkit-datetime-edit-millisecond-field,::-webkit-datetime-edit-minute-field,::-webkit-datetime-edit-month-field,::-webkit-datetime-edit-second-field,::-webkit-datetime-edit-year-field{padding-bottom:0;padding-top:0}select{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='none' viewBox='0 0 20 20'%3E%3Cpath stroke='rgb(var(--mut-gray-500, 113 113 122) / var(--tw-stroke-opacity, 1))' stroke-linecap='round' stroke-linejoin='round' stroke-width='1.5' d='m6 8 4 4 4-4'/%3E%3C/svg%3E");background-position:right .5rem center;background-repeat:no-repeat;background-size:1.5em 1.5em;padding-right:2.5rem;-webkit-print-color-adjust:exact;print-color-adjust:exact}[multiple],[size]:where(select:not([size="1"])){background-image:none;background-position:0 0;background-repeat:unset;background-size:initial;padding-right:.75rem;-webkit-print-color-adjust:unset;print-color-adjust:unset}[type=checkbox],[type=radio]{--tw-shadow:0 0 #0000;-webkit-appearance:none;-moz-appearance:none;appearance:none;background-color:#fff;background-origin:border-box;border-color:rgb(var(--mut-gray-500,113 113 122)/var(--tw-border-opacity,1));border-width:1px;color:#2563eb;display:inline-block;flex-shrink:0;height:1rem;padding:0;-webkit-print-color-adjust:exact;print-color-adjust:exact;-webkit-user-select:none;user-select:none;vertical-align:middle;width:1rem}[type=checkbox]{border-radius:0}[type=radio]{border-radius:100%}[type=checkbox]:focus,[type=radio]:focus{--tw-ring-inset:var(--tw-empty, );--tw-ring-offset-width:2px;--tw-ring-offset-color:#fff;--tw-ring-color:#2563eb;--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(2px + var(--tw-ring-offset-width)) var(--tw-ring-color);box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow);outline:2px solid transparent;outline-offset:2px}[type=checkbox]:checked,[type=radio]:checked{background-color:currentColor;background-position:50%;background-repeat:no-repeat;background-size:100% 100%;border-color:transparent}[type=checkbox]:checked{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='%23fff' viewBox='0 0 16 16'%3E%3Cpath d='M12.207 4.793a1 1 0 0 1 0 1.414l-5 5a1 1 0 0 1-1.414 0l-2-2a1 1 0 0 1 1.414-1.414L6.5 9.086l4.293-4.293a1 1 0 0 1 1.414 0z'/%3E%3C/svg%3E")}@media (forced-colors:active){[type=checkbox]:checked{-webkit-appearance:auto;-moz-appearance:auto;appearance:auto}}[type=radio]:checked{background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='%23fff' viewBox='0 0 16 16'%3E%3Ccircle cx='8' cy='8' r='3'/%3E%3C/svg%3E")}@media (forced-colors:active){[type=radio]:checked{-webkit-appearance:auto;-moz-appearance:auto;appearance:auto}}[type=checkbox]:checked:focus,[type=checkbox]:checked:hover,[type=radio]:checked:focus,[type=radio]:checked:hover{background-color:currentColor;border-color:transparent}[type=checkbox]:indeterminate{background-color:currentColor;background-image:url("data:image/svg+xml;charset=utf-8,%3Csvg xmlns='http://www.w3.org/2000/svg' fill='none' viewBox='0 0 16 16'%3E%3Cpath stroke='%23fff' stroke-linecap='round' stroke-linejoin='round' stroke-width='2' d='M4 8h8'/%3E%3C/svg%3E");background-position:50%;background-repeat:no-repeat;background-size:100% 100%;border-color:transparent}@media (forced-colors:active){[type=checkbox]:indeterminate{-webkit-appearance:auto;-moz-appearance:auto;appearance:auto}}[type=checkbox]:indeterminate:focus,[type=checkbox]:indeterminate:hover{background-color:currentColor;border-color:transparent}[type=file]{background:unset;border-color:inherit;border-radius:0;border-width:0;font-size:unset;line-height:inherit;padding:0}[type=file]:focus{outline:1px solid ButtonText;outline:1px auto -webkit-focus-ring-color}*,:after,:before{--tw-border-spacing-x:0;--tw-border-spacing-y:0;--tw-translate-x:0;--tw-translate-y:0;--tw-rotate:0;--tw-skew-x:0;--tw-skew-y:0;--tw-scale-x:1;--tw-scale-y:1;--tw-pan-x: ;--tw-pan-y: ;--tw-pinch-zoom: ;--tw-scroll-snap-strictness:proximity;--tw-gradient-from-position: ;--tw-gradient-via-position: ;--tw-gradient-to-position: ;--tw-ordinal: ;--tw-slashed-zero: ;--tw-numeric-figure: ;--tw-numeric-spacing: ;--tw-numeric-fraction: ;--tw-ring-inset: ;--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:rgba(59,130,246,.5);--tw-ring-offset-shadow:0 0 #0000;--tw-ring-shadow:0 0 #0000;--tw-shadow:0 0 #0000;--tw-shadow-colored:0 0 #0000;--tw-blur: ;--tw-brightness: ;--tw-contrast: ;--tw-grayscale: ;--tw-hue-rotate: ;--tw-invert: ;--tw-saturate: ;--tw-sepia: ;--tw-drop-shadow: ;--tw-backdrop-blur: ;--tw-backdrop-brightness: ;--tw-backdrop-contrast: ;--tw-backdrop-grayscale: ;--tw-backdrop-hue-rotate: ;--tw-backdrop-invert: ;--tw-backdrop-opacity: ;--tw-backdrop-saturate: ;--tw-backdrop-sepia: }::backdrop{--tw-border-spacing-x:0;--tw-border-spacing-y:0;--tw-translate-x:0;--tw-translate-y:0;--tw-rotate:0;--tw-skew-x:0;--tw-skew-y:0;--tw-scale-x:1;--tw-scale-y:1;--tw-pan-x: ;--tw-pan-y: ;--tw-pinch-zoom: ;--tw-scroll-snap-strictness:proximity;--tw-gradient-from-position: ;--tw-gradient-via-position: ;--tw-gradient-to-position: ;--tw-ordinal: ;--tw-slashed-zero: ;--tw-numeric-figure: ;--tw-numeric-spacing: ;--tw-numeric-fraction: ;--tw-ring-inset: ;--tw-ring-offset-width:0px;--tw-ring-offset-color:#fff;--tw-ring-color:rgba(59,130,246,.5);--tw-ring-offset-shadow:0 0 #0000;--tw-ring-shadow:0 0 #0000;--tw-shadow:0 0 #0000;--tw-shadow-colored:0 0 #0000;--tw-blur: ;--tw-brightness: ;--tw-contrast: ;--tw-grayscale: ;--tw-hue-rotate: ;--tw-invert: ;--tw-saturate: ;--tw-sepia: ;--tw-drop-shadow: ;--tw-backdrop-blur: ;--tw-backdrop-brightness: ;--tw-backdrop-contrast: ;--tw-backdrop-grayscale: ;--tw-backdrop-hue-rotate: ;--tw-backdrop-invert: ;--tw-backdrop-opacity: ;--tw-backdrop-saturate: ;--tw-backdrop-sepia: }.container{margin-left:auto;margin-right:auto;width:100%}@media (min-width:640px){.container{max-width:640px}}@media (min-width:768px){.container{max-width:768px}}@media (min-width:1024px){.container{max-width:1024px}}@media (min-width:1280px){.container{max-width:1280px}}@media (min-width:1536px){.container{max-width:1536px}}@media (min-width:2000px){.container{max-width:2000px}}.sr-only{clip:rect(0,0,0,0);border-width:0;height:1px;margin:-1px;overflow:hidden;padding:0;position:absolute;white-space:nowrap;width:1px}.pointer-events-none{pointer-events:none}.visible{visibility:visible}.static{position:static}.fixed{position:fixed}.absolute{position:absolute}.relative{position:relative}.sticky{position:sticky}.bottom-0{bottom:0}.left-0{left:0}.top-0{top:0}.top-offset{top:var(--top-offset,0)}.z-10{z-index:10}.z-20{z-index:20}.float-right{float:right}.mx-1{margin-left:.25rem;margin-right:.25rem}.mx-2{margin-left:.5rem;margin-right:.5rem}.mx-6{margin-left:1.5rem;margin-right:1.5rem}.my-3{margin-bottom:.75rem;margin-top:.75rem}.my-4{margin-bottom:1rem;margin-top:1rem}.-mb-px{margin-bottom:-1px}.mb-6{margin-bottom:1.5rem}.ml-1{margin-left:.25rem}.ml-2{margin-left:.5rem}.ml-4{margin-left:1rem}.mr-12{margin-right:3rem}.mr-2{margin-right:.5rem}.mr-3{margin-right:.75rem}.mr-4{margin-right:1rem}.mr-6{margin-right:1.5rem}.mr-auto{margin-right:auto}.ms-3{margin-inline-start:.75rem}.mt-2{margin-top:.5rem}.block{display:block}.inline-block{display:inline-block}.inline{display:inline}.flex{display:flex}.inline-flex{display:inline-flex}.table{display:table}.h-2{height:.5rem}.h-3{height:.75rem}.h-4{height:1rem}.h-5{height:1.25rem}.h-8{height:2rem}.w-12{width:3rem}.w-24{width:6rem}.w-4{width:1rem}.w-5{width:1.25rem}.w-full{width:100%}.min-w-\\[24px\\]{min-width:24px}.max-w-6xl{max-width:72rem}.table-auto{table-layout:auto}.rotate-180{--tw-rotate:180deg;transform:translate(var(--tw-translate-x),var(--tw-translate-y)) rotate(var(--tw-rotate)) skew(var(--tw-skew-x)) skewY(var(--tw-skew-y)) scaleX(var(--tw-scale-x)) scaleY(var(--tw-scale-y))}.cursor-help{cursor:help}.cursor-pointer{cursor:pointer}.flex-row{flex-direction:row}.flex-col{flex-direction:column}.flex-wrap{flex-wrap:wrap}.items-center{align-items:center}.justify-start{justify-content:flex-start}.justify-center{justify-content:center}.justify-around{justify-content:space-around}.gap-4{gap:1rem}.space-y-4>:not([hidden])~:not([hidden]){--tw-space-y-reverse:0;margin-bottom:calc(1rem*var(--tw-space-y-reverse));margin-top:calc(1rem*(1 - var(--tw-space-y-reverse)))}.divide-y>:not([hidden])~:not([hidden]){--tw-divide-y-reverse:0;border-bottom-width:calc(1px*var(--tw-divide-y-reverse));border-top-width:calc(1px*(1 - var(--tw-divide-y-reverse)))}.divide-gray-200>:not([hidden])~:not([hidden]){--tw-divide-opacity:1;border-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-divide-opacity))}.overflow-hidden{overflow:hidden}.overflow-x-auto{overflow-x:auto}.rounded{border-radius:.25rem}.rounded-full{border-radius:9999px}.rounded-lg{border-radius:.5rem}.rounded-md{border-radius:.375rem}.rounded-t-3xl{border-top-left-radius:1.5rem;border-top-right-radius:1.5rem}.rounded-t-lg{border-top-left-radius:.5rem;border-top-right-radius:.5rem}.border{border-width:1px}.border-b{border-bottom-width:1px}.border-b-2{border-bottom-width:2px}.border-none{border-style:none}.border-gray-200{--tw-border-opacity:1;border-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-border-opacity))}.border-gray-300{--tw-border-opacity:1;border-color:rgb(var(--mut-gray-300,212 212 216)/var(--tw-border-opacity))}.border-primary-600{--tw-border-opacity:1;border-color:rgb(var(--mut-primary-600,2 132 199)/var(--tw-border-opacity))}.border-transparent{border-color:transparent}.bg-blue-600{--tw-bg-opacity:1;background-color:rgb(37 99 235/var(--tw-bg-opacity))}.bg-gray-100{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-100,244 244 245)/var(--tw-bg-opacity))}.bg-gray-200{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-bg-opacity))}.bg-gray-200\\/60{background-color:rgb(var(--mut-gray-200,228 228 231)/.6)}.bg-gray-300{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-300,212 212 216)/var(--tw-bg-opacity))}.bg-green-100{--tw-bg-opacity:1;background-color:rgb(220 252 231/var(--tw-bg-opacity))}.bg-green-600{--tw-bg-opacity:1;background-color:rgb(22 163 74/var(--tw-bg-opacity))}.bg-orange-100{--tw-bg-opacity:1;background-color:rgb(255 237 213/var(--tw-bg-opacity))}.bg-primary-100{--tw-bg-opacity:1;background-color:rgb(var(--mut-primary-100,224 242 254)/var(--tw-bg-opacity))}.bg-red-100{--tw-bg-opacity:1;background-color:rgb(254 226 226/var(--tw-bg-opacity))}.bg-red-600{--tw-bg-opacity:1;background-color:rgb(220 38 38/var(--tw-bg-opacity))}.bg-white{--tw-bg-opacity:1;background-color:rgb(var(--mut-white,255 255 255)/var(--tw-bg-opacity))}.bg-yellow-100{--tw-bg-opacity:1;background-color:rgb(254 249 195/var(--tw-bg-opacity))}.bg-yellow-400{--tw-bg-opacity:1;background-color:rgb(250 204 21/var(--tw-bg-opacity))}.bg-yellow-600{--tw-bg-opacity:1;background-color:rgb(202 138 4/var(--tw-bg-opacity))}.p-1{padding:.25rem}.p-3{padding:.75rem}.p-4{padding:1rem}.px-2{padding-left:.5rem;padding-right:.5rem}.px-2\\.5{padding-left:.625rem;padding-right:.625rem}.px-4{padding-left:1rem;padding-right:1rem}.py-0{padding-bottom:0;padding-top:0}.py-0\\.5{padding-bottom:.125rem;padding-top:.125rem}.py-2{padding-bottom:.5rem;padding-top:.5rem}.py-3{padding-bottom:.75rem;padding-top:.75rem}.py-4{padding-bottom:1rem;padding-top:1rem}.py-6{padding-bottom:1.5rem;padding-top:1.5rem}.pb-4{padding-bottom:1rem}.pl-1{padding-left:.25rem}.pr-2{padding-right:.5rem}.pt-6{padding-top:1.5rem}.text-left{text-align:left}.text-center{text-align:center}.align-middle{vertical-align:middle}.font-sans{font-family:ui-sans-serif,system-ui,-apple-system,BlinkMacSystemFont,Segoe UI,Roboto,Helvetica Neue,Arial,Noto Sans,sans-serif,Apple Color Emoji,Segoe UI Emoji,Segoe UI Symbol,Noto Color Emoji}.text-5xl{font-size:3rem;line-height:1}.text-lg{font-size:1.125rem;line-height:1.75rem}.text-sm{font-size:.875rem;line-height:1.25rem}.font-bold{font-weight:700}.font-light{font-weight:300}.font-medium{font-weight:500}.font-semibold{font-weight:600}.tracking-tight{letter-spacing:-.025em}.text-gray-200{--tw-text-opacity:1;color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-text-opacity))}.text-gray-600{--tw-text-opacity:1;color:rgb(var(--mut-gray-600,82 82 91)/var(--tw-text-opacity))}.text-gray-700{--tw-text-opacity:1;color:rgb(var(--mut-gray-700,63 63 70)/var(--tw-text-opacity))}.text-gray-800{--tw-text-opacity:1;color:rgb(var(--mut-gray-800,39 39 42)/var(--tw-text-opacity))}.text-green-700{--tw-text-opacity:1;color:rgb(21 128 61/var(--tw-text-opacity))}.text-green-800{--tw-text-opacity:1;color:rgb(22 101 52/var(--tw-text-opacity))}.text-orange-800{--tw-text-opacity:1;color:rgb(154 52 18/var(--tw-text-opacity))}.text-primary-800{--tw-text-opacity:1;color:rgb(var(--mut-primary-800,7 89 133)/var(--tw-text-opacity))}.text-primary-on{--tw-text-opacity:1;color:rgb(var(--mut-primary-on,3 105 161)/var(--tw-text-opacity))}.text-red-700{--tw-text-opacity:1;color:rgb(185 28 28/var(--tw-text-opacity))}.text-red-800{--tw-text-opacity:1;color:rgb(153 27 27/var(--tw-text-opacity))}.text-yellow-600{--tw-text-opacity:1;color:rgb(202 138 4/var(--tw-text-opacity))}.text-yellow-800{--tw-text-opacity:1;color:rgb(133 77 14/var(--tw-text-opacity))}.underline{text-decoration-line:underline}.decoration-dotted{text-decoration-style:dotted}.opacity-0{opacity:0}.shadow{--tw-shadow:0 1px 3px 0 rgba(0,0,0,.1),0 1px 2px -1px rgba(0,0,0,.1);--tw-shadow-colored:0 1px 3px 0 var(--tw-shadow-color),0 1px 2px -1px var(--tw-shadow-color)}.shadow,.shadow-xl{box-shadow:var(--tw-ring-offset-shadow,0 0 #0000),var(--tw-ring-shadow,0 0 #0000),var(--tw-shadow)}.shadow-xl{--tw-shadow:0 20px 25px -5px rgba(0,0,0,.1),0 8px 10px -6px rgba(0,0,0,.1);--tw-shadow-colored:0 20px 25px -5px var(--tw-shadow-color),0 8px 10px -6px var(--tw-shadow-color)}.\\!ring-offset-gray-200{--tw-ring-offset-color:rgb(var(--mut-gray-200,228 228 231)/1)!important}.filter{filter:var(--tw-blur) var(--tw-brightness) var(--tw-contrast) var(--tw-grayscale) var(--tw-hue-rotate) var(--tw-invert) var(--tw-saturate) var(--tw-sepia) var(--tw-drop-shadow)}.backdrop-blur-lg{--tw-backdrop-blur:blur(16px);-webkit-backdrop-filter:var(--tw-backdrop-blur) var(--tw-backdrop-brightness) var(--tw-backdrop-contrast) var(--tw-backdrop-grayscale) var(--tw-backdrop-hue-rotate) var(--tw-backdrop-invert) var(--tw-backdrop-opacity) var(--tw-backdrop-saturate) var(--tw-backdrop-sepia);backdrop-filter:var(--tw-backdrop-blur) var(--tw-backdrop-brightness) var(--tw-backdrop-contrast) var(--tw-backdrop-grayscale) var(--tw-backdrop-hue-rotate) var(--tw-backdrop-invert) var(--tw-backdrop-opacity) var(--tw-backdrop-saturate) var(--tw-backdrop-sepia)}.transition-all{transition-duration:.15s;transition-property:all;transition-timing-function:cubic-bezier(.4,0,.2,1)}.transition-colors{transition-duration:.15s;transition-property:color,background-color,border-color,text-decoration-color,fill,stroke;transition-timing-function:cubic-bezier(.4,0,.2,1)}.after\\:text-gray-800:after{--tw-text-opacity:1;color:rgb(var(--mut-gray-800,39 39 42)/var(--tw-text-opacity));content:var(--tw-content)}.after\\:content-\\[\\'\\/\\'\\]:after{--tw-content:"/";content:var(--tw-content)}.even\\:bg-gray-100:nth-child(2n),.odd\\:bg-gray-100:nth-child(odd){--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-100,244 244 245)/var(--tw-bg-opacity))}.hover\\:cursor-pointer:hover{cursor:pointer}.hover\\:border-gray-300:hover{--tw-border-opacity:1;border-color:rgb(var(--mut-gray-300,212 212 216)/var(--tw-border-opacity))}.hover\\:bg-gray-100:hover{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-100,244 244 245)/var(--tw-bg-opacity))}.hover\\:bg-gray-200:hover{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-bg-opacity))}.hover\\:text-gray-700:hover{--tw-text-opacity:1;color:rgb(var(--mut-gray-700,63 63 70)/var(--tw-text-opacity))}.hover\\:text-gray-900:hover{--tw-text-opacity:1;color:rgb(var(--mut-gray-900,24 24 27)/var(--tw-text-opacity))}.hover\\:text-primary-on:hover{--tw-text-opacity:1;color:rgb(var(--mut-primary-on,3 105 161)/var(--tw-text-opacity))}.hover\\:underline:hover{text-decoration-line:underline}.focus\\:outline-none:focus{outline:2px solid transparent;outline-offset:2px}.focus\\:ring-2:focus{--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(2px + var(--tw-ring-offset-width)) var(--tw-ring-color);box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow,0 0 #0000)}.focus\\:ring-primary-500:focus{--tw-ring-opacity:1;--tw-ring-color:rgb(var(--mut-primary-500,14 165 233)/var(--tw-ring-opacity))}.active\\:bg-gray-200:active,.group:hover .group-hover\\:bg-gray-200{--tw-bg-opacity:1;background-color:rgb(var(--mut-gray-200,228 228 231)/var(--tw-bg-opacity))}.aria-selected\\:border-b-\\[3px\\][aria-selected=true]{border-bottom-width:3px}.aria-selected\\:border-primary-700[aria-selected=true]{--tw-border-opacity:1;border-color:rgb(var(--mut-primary-700,3 105 161)/var(--tw-border-opacity))}.aria-selected\\:text-primary-on[aria-selected=true]{--tw-text-opacity:1;color:rgb(var(--mut-primary-on,3 105 161)/var(--tw-text-opacity))}@media (prefers-reduced-motion:no-preference){.motion-safe\\:transition-\\[height\\,max-width\\]{transition-duration:.15s;transition-property:height,max-width;transition-timing-function:cubic-bezier(.4,0,.2,1)}.motion-safe\\:transition-max-width{transition-duration:.15s;transition-property:max-width;transition-timing-function:cubic-bezier(.4,0,.2,1)}.motion-safe\\:duration-200{transition-duration:.2s}}@media (min-width:768px){.md\\:ml-2{margin-left:.5rem}.md\\:after\\:pl-1:after{content:var(--tw-content);padding-left:.25rem}}@media (min-width:1536px){.\\32xl\\:w-28{width:7rem}}`,$s="code[class*=language-],pre[class*=language-]{color:var(--prism-maintext);direction:ltr;font-family:Consolas,Monaco,Andale Mono,Ubuntu Mono,monospace;font-size:1em;-webkit-hyphens:none;hyphens:none;line-height:1.5;-moz-tab-size:4;tab-size:4;text-align:left;white-space:pre;word-break:normal;word-spacing:normal}pre>code[class*=language-]{font-size:1em}pre[class*=language-]{border:1px solid var(--prism-border);border-radius:.25rem;margin:.5em 0;overflow:auto;padding:1em}:not(pre)>code[class*=language-],pre[class*=language-]{background:var(--prism-background)}.token.cdata,.token.comment,.token.doctype,.token.italic,.token.prolog{font-style:italic}.token.bold,.token.function,.token.important{font-weight:700}.token.namespace{opacity:.7}.token.atrule{color:var(--prism-atrule)}.token.attr{color:var(--prism-attr)}.token.attr-name{color:var(--prism-attr-name)}.token.boolean{color:var(--prism-boolean)}.token.builtin{color:var(--prism-builtin)}.token.cdata{color:var(--prism-cdata)}.token.changed{color:var(--prism-changed)}.token.char{color:var(--prism-char)}.token.comment{color:var(--prism-comment)}.token.constant{color:var(--prism-constant)}.token.deleted{color:var(--prism-deleted)}.token.doctype{color:var(--prism-doctype)}.token.entity{color:var(--prism-entity);cursor:help}.token.function{color:var(--prism-function)}.token.function-variable{color:var(--prism-function-variable,var(--prism-function))}.token.inserted{color:var(--prism-inserted)}.token.keyword{color:var(--prism-keyword)}.token.number{color:var(--prism-number)}.token.operator{color:var(--prism-operator)}.token.prolog{color:var(--prism-prolog)}.token.property{color:var(--prism-property)}.token.punctuation{color:var(--prism-punctuation)}.token.regex{color:var(--prism-regex)}.token.selector{color:var(--prism-selector)}.token.string{color:var(--prism-string)}.token.symbol{color:var(--prism-symbol)}.token.tag{color:var(--prism-tag)}.token.url{color:var(--prism-url)}.token.variable{color:var(--prism-variable)}.token.placeholder{color:var(--prism-placeholder)}.token.statement{color:var(--prism-statement)}.token.attr-value{color:var(--prism-attr-value)}.token.control{color:var(--prism-control)}.token.directive{color:var(--prism-directive)}.token.unit{color:var(--prism-unit)}.token.important{color:var(--prism-important)}.token.class-name{color:var(--prism-class-name)}pre[class*=language-].line-numbers{counter-reset:linenumber;padding-left:3.8em;position:relative}pre[class*=language-].line-numbers>code{position:relative;white-space:inherit}.line-numbers .line-numbers-rows{border-right:1px solid #999;font-size:100%;left:-3.8em;letter-spacing:-1px;pointer-events:none;position:absolute;top:0;-webkit-user-select:none;user-select:none;width:3em}.line-numbers-rows>span{counter-increment:linenumber;display:block}.line-numbers-rows>span:before{color:#999;content:counter(linenumber);display:block;padding-right:.8em;text-align:right}",ks="";var nn=typeof globalThis<"u"?globalThis:typeof window<"u"?window:typeof global<"u"?global:typeof self<"u"?self:{},In={exports:{}};(function(r){var e=typeof window<"u"?window:typeof WorkerGlobalScope<"u"&&self instanceof WorkerGlobalScope?self:{};/**
* Prism: Lightweight, robust, elegant syntax highlighting
*
* @license MIT
* @author Lea Verou
* @namespace
* @public
*/var t=function(n){var i=/(?:^|\s)lang(?:uage)?-([\w-]+)(?=\s|$)/i,s=0,a={},o={manual:n.Prism&&n.Prism.manual,disableWorkerMessageHandler:n.Prism&&n.Prism.disableWorkerMessageHandler,util:{encode:function h(p){return p instanceof l?new l(p.type,h(p.content),p.alias):Array.isArray(p)?p.map(h):p.replace(/&/g,"&").replace(/"u")return null;if("currentScript"in document&&1<2)return document.currentScript;try{throw new Error}catch(u){var h=(/at [^(\r\n]*\((.*):[^:]+:[^:]+\)$/i.exec(u.stack)||[])[1];if(h){var p=document.getElementsByTagName("script");for(var b in p)if(p[b].src==h)return p[b]}return null}},isActive:function(h,p,b){for(var u="no-"+p;h;){var f=h.classList;if(f.contains(p))return!0;if(f.contains(u))return!1;h=h.parentElement}return!!b}},languages:{plain:a,plaintext:a,text:a,txt:a,extend:function(h,p){var b=o.util.clone(o.languages[h]);for(var u in p)b[u]=p[u];return b},insertBefore:function(h,p,b,u){u=u||o.languages;var f=u[h],w={};for(var C in f)if(f.hasOwnProperty(C)){if(C==p)for(var _ in b)b.hasOwnProperty(_)&&(w[_]=b[_]);b.hasOwnProperty(C)||(w[C]=f[C])}var P=u[h];return u[h]=w,o.languages.DFS(o.languages,function(O,G){G===P&&O!=h&&(this[O]=w)}),w},DFS:function h(p,b,u,f){f=f||{};var w=o.util.objId;for(var C in p)if(p.hasOwnProperty(C)){b.call(p,C,p[C],u||C);var _=p[C],P=o.util.type(_);P==="Object"&&!f[w(_)]?(f[w(_)]=!0,h(_,b,null,f)):P==="Array"&&!f[w(_)]&&(f[w(_)]=!0,h(_,b,C,f))}}},plugins:{},highlightAll:function(h,p){o.highlightAllUnder(document,h,p)},highlightAllUnder:function(h,p,b){var u={callback:b,container:h,selector:'code[class*="language-"], [class*="language-"] code, code[class*="lang-"], [class*="lang-"] code'};o.hooks.run("before-highlightall",u),u.elements=Array.prototype.slice.apply(u.container.querySelectorAll(u.selector)),o.hooks.run("before-all-elements-highlight",u);for(var f=0,w;w=u.elements[f++];)o.highlightElement(w,p===!0,u.callback)},highlightElement:function(h,p,b){var u=o.util.getLanguage(h),f=o.languages[u];o.util.setLanguage(h,u);var w=h.parentElement;w&&w.nodeName.toLowerCase()==="pre"&&o.util.setLanguage(w,u);var C=h.textContent,_={element:h,language:u,grammar:f,code:C};function P(G){_.highlightedCode=G,o.hooks.run("before-insert",_),_.element.innerHTML=_.highlightedCode,o.hooks.run("after-highlight",_),o.hooks.run("complete",_),b&&b.call(_.element)}if(o.hooks.run("before-sanity-check",_),w=_.element.parentElement,w&&w.nodeName.toLowerCase()==="pre"&&!w.hasAttribute("tabindex")&&w.setAttribute("tabindex","0"),!_.code){o.hooks.run("complete",_),b&&b.call(_.element);return}if(o.hooks.run("before-highlight",_),!_.grammar){P(o.util.encode(_.code));return}if(p&&n.Worker){var O=new Worker(o.filename);O.onmessage=function(G){P(G.data)},O.postMessage(JSON.stringify({language:_.language,code:_.code,immediateClose:!0}))}else P(o.highlight(_.code,_.grammar,_.language))},highlight:function(h,p,b){var u={code:h,grammar:p,language:b};if(o.hooks.run("before-tokenize",u),!u.grammar)throw new Error('The language "'+u.language+'" has no grammar.');return u.tokens=o.tokenize(u.code,u.grammar),o.hooks.run("after-tokenize",u),l.stringify(o.util.encode(u.tokens),u.language)},tokenize:function(h,p){var b=p.rest;if(b){for(var u in b)p[u]=b[u];delete p.rest}var f=new d;return m(f,f.head,h),g(h,f,p,f.head,0),$(f)},hooks:{all:{},add:function(h,p){var b=o.hooks.all;b[h]=b[h]||[],b[h].push(p)},run:function(h,p){var b=o.hooks.all[h];if(!(!b||!b.length))for(var u=0,f;f=b[u++];)f(p)}},Token:l};n.Prism=o;function l(h,p,b,u){this.type=h,this.content=p,this.alias=b,this.length=(u||"").length|0}l.stringify=function h(p,b){if(typeof p=="string")return p;if(Array.isArray(p)){var u="";return p.forEach(function(P){u+=h(P,b)}),u}var f={type:p.type,content:h(p.content,b),tag:"span",classes:["token",p.type],attributes:{},language:b},w=p.alias;w&&(Array.isArray(w)?Array.prototype.push.apply(f.classes,w):f.classes.push(w)),o.hooks.run("wrap",f);var C="";for(var _ in f.attributes)C+=" "+_+'="'+(f.attributes[_]||"").replace(/"/g,""")+'"';return"<"+f.tag+' class="'+f.classes.join(" ")+'"'+C+">"+f.content+""+f.tag+">"};function c(h,p,b,u){h.lastIndex=p;var f=h.exec(b);if(f&&u&&f[1]){var w=f[1].length;f.index+=w,f[0]=f[0].slice(w)}return f}function g(h,p,b,u,f,w){for(var C in b)if(!(!b.hasOwnProperty(C)||!b[C])){var _=b[C];_=Array.isArray(_)?_:[_];for(var P=0;P<_.length;++P){if(w&&w.cause==C+","+P)return;var O=_[P],G=O.inside,ze=!!O.lookbehind,Ue=!!O.greedy,pt=O.alias;if(Ue&&!O.pattern.global){var ht=O.pattern.toString().match(/[imsuy]*$/)[0];O.pattern=RegExp(O.pattern.source,ht+"g")}for(var He=O.pattern||O,R=u.next,T=f;R!==p.tail&&!(w&&T>=w.reach);T+=R.value.length,R=R.next){var B=R.value;if(p.length>h.length)return;if(!(B instanceof l)){var Y=1,H;if(Ue){if(H=c(He,T,h,ze),!H||H.index>=h.length)break;var ft=H.index,Ui=H.index+H[0].length,oe=T;for(oe+=R.value.length;ft>=oe;)R=R.next,oe+=R.value.length;if(oe-=R.value.length,T=oe,R.value instanceof l)continue;for(var Ke=R;Ke!==p.tail&&(oew.reach&&(w.reach=Ut);var mt=R.prev;Lt&&(mt=m(p,mt,Lt),T+=Lt.length),y(p,mt,Y);var Hi=new l(C,G?o.tokenize(gt,G):gt,pt,gt);if(R=m(p,mt,Hi),Nr&&m(p,R,Nr),Y>1){var Ht={cause:C+","+P,reach:Ut};g(h,p,b,R.prev,T,Ht),w&&Ht.reach>w.reach&&(w.reach=Ht.reach)}}}}}}function d(){var h={value:null,prev:null,next:null},p={value:null,prev:h,next:null};h.next=p,this.head=h,this.tail=p,this.length=0}function m(h,p,b){var u=p.next,f={value:b,prev:p,next:u};return p.next=f,u.prev=f,h.length++,f}function y(h,p,b){for(var u=p.next,f=0;f"u"||typeof document>"u")return;var r="line-numbers",e=/\n(?!$)/g,t=Prism.plugins.lineNumbers={getLine:function(a,o){if(!(a.tagName!=="PRE"||!a.classList.contains(r))){var l=a.querySelector(".line-numbers-rows");if(l){var c=parseInt(a.getAttribute("data-start"),10)||1,g=c+(l.children.length-1);og&&(o=g);var d=o-c;return l.children[d]}}},resize:function(a){n([a])},assumeViewportIndependence:!0};function n(a){if(a=a.filter(function(l){var c=i(l),g=c["white-space"];return g==="pre-wrap"||g==="pre-line"}),a.length!=0){var o=a.map(function(l){var c=l.querySelector("code"),g=l.querySelector(".line-numbers-rows");if(!(!c||!g)){var d=l.querySelector(".line-numbers-sizer"),m=c.textContent.split(e);d||(d=document.createElement("span"),d.className="line-numbers-sizer",c.appendChild(d)),d.innerHTML="0",d.style.display="block";var y=d.getBoundingClientRect().height;return d.innerHTML="",{element:l,lines:m,lineHeights:[],oneLinerHeight:y,sizer:d}}}).filter(Boolean);o.forEach(function(l){var c=l.sizer,g=l.lines,d=l.lineHeights,m=l.oneLinerHeight;d[g.length-1]=void 0,g.forEach(function(y,$){if(y&&y.length>1){var k=c.appendChild(document.createElement("span"));k.style.display="block",k.textContent=y}else d[$]=m})}),o.forEach(function(l){for(var c=l.sizer,g=l.lineHeights,d=0,m=0;m");d=document.createElement("span"),d.setAttribute("aria-hidden","true"),d.className="line-numbers-rows",d.innerHTML=m,l.hasAttribute("data-start")&&(l.style.counterReset="linenumber "+(parseInt(l.getAttribute("data-start"),10)-1)),a.element.appendChild(d),n([l]),Prism.hooks.run("line-numbers",a)}}}),Prism.hooks.add("line-numbers",function(a){a.plugins=a.plugins||{},a.plugins.lineNumbers=!0})})();Prism.languages.clike={comment:[{pattern:/(^|[^\\])\/\*[\s\S]*?(?:\*\/|$)/,lookbehind:!0,greedy:!0},{pattern:/(^|[^\\:])\/\/.*/,lookbehind:!0,greedy:!0}],string:{pattern:/(["'])(?:\\(?:\r\n|[\s\S])|(?!\1)[^\\\r\n])*\1/,greedy:!0},"class-name":{pattern:/(\b(?:class|extends|implements|instanceof|interface|new|trait)\s+|\bcatch\s+\()[\w.\\]+/i,lookbehind:!0,inside:{punctuation:/[.\\]/}},keyword:/\b(?:break|catch|continue|do|else|finally|for|function|if|in|instanceof|new|null|return|throw|try|while)\b/,boolean:/\b(?:false|true)\b/,function:/\b\w+(?=\()/,number:/\b0x[\da-f]+\b|(?:\b\d+(?:\.\d*)?|\B\.\d+)(?:e[+-]?\d+)?/i,operator:/[<>]=?|[!=]=?=?|--?|\+\+?|&&?|\|\|?|[?*/~^%]/,punctuation:/[{}[\];(),.:]/};Prism.languages.javascript=Prism.languages.extend("clike",{"class-name":[Prism.languages.clike["class-name"],{pattern:/(^|[^$\w\xA0-\uFFFF])(?!\s)[_$A-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*(?=\.(?:constructor|prototype))/,lookbehind:!0}],keyword:[{pattern:/((?:^|\})\s*)catch\b/,lookbehind:!0},{pattern:/(^|[^.]|\.\.\.\s*)\b(?:as|assert(?=\s*\{)|async(?=\s*(?:function\b|\(|[$\w\xA0-\uFFFF]|$))|await|break|case|class|const|continue|debugger|default|delete|do|else|enum|export|extends|finally(?=\s*(?:\{|$))|for|from(?=\s*(?:['"]|$))|function|(?:get|set)(?=\s*(?:[#\[$\w\xA0-\uFFFF]|$))|if|implements|import|in|instanceof|interface|let|new|null|of|package|private|protected|public|return|static|super|switch|this|throw|try|typeof|undefined|var|void|while|with|yield)\b/,lookbehind:!0}],function:/#?(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*(?=\s*(?:\.\s*(?:apply|bind|call)\s*)?\()/,number:{pattern:RegExp(/(^|[^\w$])/.source+"(?:"+(/NaN|Infinity/.source+"|"+/0[bB][01]+(?:_[01]+)*n?/.source+"|"+/0[oO][0-7]+(?:_[0-7]+)*n?/.source+"|"+/0[xX][\dA-Fa-f]+(?:_[\dA-Fa-f]+)*n?/.source+"|"+/\d+(?:_\d+)*n/.source+"|"+/(?:\d+(?:_\d+)*(?:\.(?:\d+(?:_\d+)*)?)?|\.\d+(?:_\d+)*)(?:[Ee][+-]?\d+(?:_\d+)*)?/.source)+")"+/(?![\w$])/.source),lookbehind:!0},operator:/--|\+\+|\*\*=?|=>|&&=?|\|\|=?|[!=]==|<<=?|>>>?=?|[-+*/%&|^!=<>]=?|\.{3}|\?\?=?|\?\.?|[~:]/});Prism.languages.javascript["class-name"][0].pattern=/(\b(?:class|extends|implements|instanceof|interface|new)\s+)[\w.\\]+/;Prism.languages.insertBefore("javascript","keyword",{regex:{pattern:RegExp(/((?:^|[^$\w\xA0-\uFFFF."'\])\s]|\b(?:return|yield))\s*)/.source+/\//.source+"(?:"+/(?:\[(?:[^\]\\\r\n]|\\.)*\]|\\.|[^/\\\[\r\n])+\/[dgimyus]{0,7}/.source+"|"+/(?:\[(?:[^[\]\\\r\n]|\\.|\[(?:[^[\]\\\r\n]|\\.|\[(?:[^[\]\\\r\n]|\\.)*\])*\])*\]|\\.|[^/\\\[\r\n])+\/[dgimyus]{0,7}v[dgimyus]{0,7}/.source+")"+/(?=(?:\s|\/\*(?:[^*]|\*(?!\/))*\*\/)*(?:$|[\r\n,.;:})\]]|\/\/))/.source),lookbehind:!0,greedy:!0,inside:{"regex-source":{pattern:/^(\/)[\s\S]+(?=\/[a-z]*$)/,lookbehind:!0,alias:"language-regex",inside:Prism.languages.regex},"regex-delimiter":/^\/|\/$/,"regex-flags":/^[a-z]+$/}},"function-variable":{pattern:/#?(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*(?=\s*[=:]\s*(?:async\s*)?(?:\bfunction\b|(?:\((?:[^()]|\([^()]*\))*\)|(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*)\s*=>))/,alias:"function"},parameter:[{pattern:/(function(?:\s+(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*)?\s*\(\s*)(?!\s)(?:[^()\s]|\s+(?![\s)])|\([^()]*\))+(?=\s*\))/,lookbehind:!0,inside:Prism.languages.javascript},{pattern:/(^|[^$\w\xA0-\uFFFF])(?!\s)[_$a-z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*(?=\s*=>)/i,lookbehind:!0,inside:Prism.languages.javascript},{pattern:/(\(\s*)(?!\s)(?:[^()\s]|\s+(?![\s)])|\([^()]*\))+(?=\s*\)\s*=>)/,lookbehind:!0,inside:Prism.languages.javascript},{pattern:/((?:\b|\s|^)(?!(?:as|async|await|break|case|catch|class|const|continue|debugger|default|delete|do|else|enum|export|extends|finally|for|from|function|get|if|implements|import|in|instanceof|interface|let|new|null|of|package|private|protected|public|return|set|static|super|switch|this|throw|try|typeof|undefined|var|void|while|with|yield)(?![$\w\xA0-\uFFFF]))(?:(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*\s*)\(\s*|\]\s*\(\s*)(?!\s)(?:[^()\s]|\s+(?![\s)])|\([^()]*\))+(?=\s*\)\s*\{)/,lookbehind:!0,inside:Prism.languages.javascript}],constant:/\b[A-Z](?:[A-Z_]|\dx?)*\b/});Prism.languages.insertBefore("javascript","string",{hashbang:{pattern:/^#!.*/,greedy:!0,alias:"comment"},"template-string":{pattern:/`(?:\\[\s\S]|\$\{(?:[^{}]|\{(?:[^{}]|\{[^}]*\})*\})+\}|(?!\$\{)[^\\`])*`/,greedy:!0,inside:{"template-punctuation":{pattern:/^`|`$/,alias:"string"},interpolation:{pattern:/((?:^|[^\\])(?:\\{2})*)\$\{(?:[^{}]|\{(?:[^{}]|\{[^}]*\})*\})+\}/,lookbehind:!0,inside:{"interpolation-punctuation":{pattern:/^\$\{|\}$/,alias:"punctuation"},rest:Prism.languages.javascript}},string:/[\s\S]+/}},"string-property":{pattern:/((?:^|[,{])[ \t]*)(["'])(?:\\(?:\r\n|[\s\S])|(?!\2)[^\\\r\n])*\2(?=\s*:)/m,lookbehind:!0,greedy:!0,alias:"property"}});Prism.languages.insertBefore("javascript","operator",{"literal-property":{pattern:/((?:^|[,{])[ \t]*)(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*(?=\s*:)/m,lookbehind:!0,alias:"property"}});Prism.languages.markup&&(Prism.languages.markup.tag.addInlined("script","javascript"),Prism.languages.markup.tag.addAttribute(/on(?:abort|blur|change|click|composition(?:end|start|update)|dblclick|error|focus(?:in|out)?|key(?:down|up)|load|mouse(?:down|enter|leave|move|out|over|up)|reset|resize|scroll|select|slotchange|submit|unload|wheel)/.source,"javascript"));Prism.languages.js=Prism.languages.javascript;(function(r){r.languages.typescript=r.languages.extend("javascript",{"class-name":{pattern:/(\b(?:class|extends|implements|instanceof|interface|new|type)\s+)(?!keyof\b)(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*(?:\s*<(?:[^<>]|<(?:[^<>]|<[^<>]*>)*>)*>)?/,lookbehind:!0,greedy:!0,inside:null},builtin:/\b(?:Array|Function|Promise|any|boolean|console|never|number|string|symbol|unknown)\b/}),r.languages.typescript.keyword.push(/\b(?:abstract|declare|is|keyof|readonly|require)\b/,/\b(?:asserts|infer|interface|module|namespace|type)\b(?=\s*(?:[{_$a-zA-Z\xA0-\uFFFF]|$))/,/\btype\b(?=\s*(?:[\{*]|$))/),delete r.languages.typescript.parameter,delete r.languages.typescript["literal-property"];var e=r.languages.extend("typescript",{});delete e["class-name"],r.languages.typescript["class-name"].inside=e,r.languages.insertBefore("typescript","function",{decorator:{pattern:/@[$\w\xA0-\uFFFF]+/,inside:{at:{pattern:/^@/,alias:"operator"},function:/^[\s\S]+/}},"generic-function":{pattern:/#?(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*\s*<(?:[^<>]|<(?:[^<>]|<[^<>]*>)*>)*>(?=\s*\()/,greedy:!0,inside:{function:/^#?(?!\s)[_$a-zA-Z\xA0-\uFFFF](?:(?!\s)[$\w\xA0-\uFFFF])*/,generic:{pattern:/<[\s\S]+/,alias:"class-name",inside:e}}}}),r.languages.ts=r.languages.typescript})(Prism);(function(r){function e(T,B){return T.replace(/<<(\d+)>>/g,function(Y,H){return"(?:"+B[+H]+")"})}function t(T,B,Y){return RegExp(e(T,B),Y||"")}function n(T,B){for(var Y=0;Y>/g,function(){return"(?:"+T+")"});return T.replace(/<>/g,"[^\\s\\S]")}var i={type:"bool byte char decimal double dynamic float int long object sbyte short string uint ulong ushort var void",typeDeclaration:"class enum interface record struct",contextual:"add alias and ascending async await by descending from(?=\\s*(?:\\w|$)) get global group into init(?=\\s*;) join let nameof not notnull on or orderby partial remove select set unmanaged value when where with(?=\\s*{)",other:"abstract as base break case catch checked const continue default delegate do else event explicit extern finally fixed for foreach goto if implicit in internal is lock namespace new null operator out override params private protected public readonly ref return sealed sizeof stackalloc static switch this throw try typeof unchecked unsafe using virtual volatile while yield"};function s(T){return"\\b(?:"+T.trim().replace(/ /g,"|")+")\\b"}var a=s(i.typeDeclaration),o=RegExp(s(i.type+" "+i.typeDeclaration+" "+i.contextual+" "+i.other)),l=s(i.typeDeclaration+" "+i.contextual+" "+i.other),c=s(i.type+" "+i.typeDeclaration+" "+i.other),g=n(/<(?:[^<>;=+\-*/%&|^]|<>)*>/.source,2),d=n(/\((?:[^()]|<>)*\)/.source,2),m=/@?\b[A-Za-z_]\w*\b/.source,y=e(/<<0>>(?:\s*<<1>>)?/.source,[m,g]),$=e(/(?!<<0>>)<<1>>(?:\s*\.\s*<<1>>)*/.source,[l,y]),k=/\[\s*(?:,\s*)*\]/.source,F=e(/<<0>>(?:\s*(?:\?\s*)?<<1>>)*(?:\s*\?)?/.source,[$,k]),z=e(/[^,()<>[\];=+\-*/%&|^]|<<0>>|<<1>>|<<2>>/.source,[g,d,k]),h=e(/\(<<0>>+(?:,<<0>>+)+\)/.source,[z]),p=e(/(?:<<0>>|<<1>>)(?:\s*(?:\?\s*)?<<2>>)*(?:\s*\?)?/.source,[h,$,k]),b={keyword:o,punctuation:/[<>()?,.:[\]]/},u=/'(?:[^\r\n'\\]|\\.|\\[Uux][\da-fA-F]{1,8})'/.source,f=/"(?:\\.|[^\\"\r\n])*"/.source,w=/@"(?:""|\\[\s\S]|[^\\"])*"(?!")/.source;r.languages.csharp=r.languages.extend("clike",{string:[{pattern:t(/(^|[^$\\])<<0>>/.source,[w]),lookbehind:!0,greedy:!0},{pattern:t(/(^|[^@$\\])<<0>>/.source,[f]),lookbehind:!0,greedy:!0}],"class-name":[{pattern:t(/(\busing\s+static\s+)<<0>>(?=\s*;)/.source,[$]),lookbehind:!0,inside:b},{pattern:t(/(\busing\s+<<0>>\s*=\s*)<<1>>(?=\s*;)/.source,[m,p]),lookbehind:!0,inside:b},{pattern:t(/(\busing\s+)<<0>>(?=\s*=)/.source,[m]),lookbehind:!0},{pattern:t(/(\b<<0>>\s+)<<1>>/.source,[a,y]),lookbehind:!0,inside:b},{pattern:t(/(\bcatch\s*\(\s*)<<0>>/.source,[$]),lookbehind:!0,inside:b},{pattern:t(/(\bwhere\s+)<<0>>/.source,[m]),lookbehind:!0},{pattern:t(/(\b(?:is(?:\s+not)?|as)\s+)<<0>>/.source,[F]),lookbehind:!0,inside:b},{pattern:t(/\b<<0>>(?=\s+(?!<<1>>|with\s*\{)<<2>>(?:\s*[=,;:{)\]]|\s+(?:in|when)\b))/.source,[p,c,m]),inside:b}],keyword:o,number:/(?:\b0(?:x[\da-f_]*[\da-f]|b[01_]*[01])|(?:\B\.\d+(?:_+\d+)*|\b\d+(?:_+\d+)*(?:\.\d+(?:_+\d+)*)?)(?:e[-+]?\d+(?:_+\d+)*)?)(?:[dflmu]|lu|ul)?\b/i,operator:/>>=?|<<=?|[-=]>|([-+&|])\1|~|\?\?=?|[-+*/%&|^!=<>]=?/,punctuation:/\?\.?|::|[{}[\];(),.:]/}),r.languages.insertBefore("csharp","number",{range:{pattern:/\.\./,alias:"operator"}}),r.languages.insertBefore("csharp","punctuation",{"named-parameter":{pattern:t(/([(,]\s*)<<0>>(?=\s*:)/.source,[m]),lookbehind:!0,alias:"punctuation"}}),r.languages.insertBefore("csharp","class-name",{namespace:{pattern:t(/(\b(?:namespace|using)\s+)<<0>>(?:\s*\.\s*<<0>>)*(?=\s*[;{])/.source,[m]),lookbehind:!0,inside:{punctuation:/\./}},"type-expression":{pattern:t(/(\b(?:default|sizeof|typeof)\s*\(\s*(?!\s))(?:[^()\s]|\s(?!\s)|<<0>>)*(?=\s*\))/.source,[d]),lookbehind:!0,alias:"class-name",inside:b},"return-type":{pattern:t(/<<0>>(?=\s+(?:<<1>>\s*(?:=>|[({]|\.\s*this\s*\[)|this\s*\[))/.source,[p,$]),inside:b,alias:"class-name"},"constructor-invocation":{pattern:t(/(\bnew\s+)<<0>>(?=\s*[[({])/.source,[p]),lookbehind:!0,inside:b,alias:"class-name"},"generic-method":{pattern:t(/<<0>>\s*<<1>>(?=\s*\()/.source,[m,g]),inside:{function:t(/^<<0>>/.source,[m]),generic:{pattern:RegExp(g),alias:"class-name",inside:b}}},"type-list":{pattern:t(/\b((?:<<0>>\s+<<1>>|record\s+<<1>>\s*<<5>>|where\s+<<2>>)\s*:\s*)(?:<<3>>|<<4>>|<<1>>\s*<<5>>|<<6>>)(?:\s*,\s*(?:<<3>>|<<4>>|<<6>>))*(?=\s*(?:where|[{;]|=>|$))/.source,[a,y,m,p,o.source,d,/\bnew\s*\(\s*\)/.source]),lookbehind:!0,inside:{"record-arguments":{pattern:t(/(^(?!new\s*\()<<0>>\s*)<<1>>/.source,[y,d]),lookbehind:!0,greedy:!0,inside:r.languages.csharp},keyword:o,"class-name":{pattern:RegExp(p),greedy:!0,inside:b},punctuation:/[,()]/}},preprocessor:{pattern:/(^[\t ]*)#.*/m,lookbehind:!0,alias:"property",inside:{directive:{pattern:/(#)\b(?:define|elif|else|endif|endregion|error|if|line|nullable|pragma|region|undef|warning)\b/,lookbehind:!0,alias:"keyword"}}}});var C=f+"|"+u,_=e(/\/(?![*/])|\/\/[^\r\n]*[\r\n]|\/\*(?:[^*]|\*(?!\/))*\*\/|<<0>>/.source,[C]),P=n(e(/[^"'/()]|<<0>>|\(<>*\)/.source,[_]),2),O=/\b(?:assembly|event|field|method|module|param|property|return|type)\b/.source,G=e(/<<0>>(?:\s*\(<<1>>*\))?/.source,[$,P]);r.languages.insertBefore("csharp","class-name",{attribute:{pattern:t(/((?:^|[^\s\w>)?])\s*\[\s*)(?:<<0>>\s*:\s*)?<<1>>(?:\s*,\s*<<1>>)*(?=\s*\])/.source,[O,G]),lookbehind:!0,greedy:!0,inside:{target:{pattern:t(/^<<0>>(?=\s*:)/.source,[O]),alias:"keyword"},"attribute-arguments":{pattern:t(/\(<<0>>*\)/.source,[P]),inside:r.languages.csharp},"class-name":{pattern:RegExp($),inside:{punctuation:/\./}},punctuation:/[:,]/}}});var ze=/:[^}\r\n]+/.source,Ue=n(e(/[^"'/()]|<<0>>|\(<>*\)/.source,[_]),2),pt=e(/\{(?!\{)(?:(?![}:])<<0>>)*<<1>>?\}/.source,[Ue,ze]),ht=n(e(/[^"'/()]|\/(?!\*)|\/\*(?:[^*]|\*(?!\/))*\*\/|<<0>>|\(<>*\)/.source,[C]),2),He=e(/\{(?!\{)(?:(?![}:])<<0>>)*<<1>>?\}/.source,[ht,ze]);function R(T,B){return{interpolation:{pattern:t(/((?:^|[^{])(?:\{\{)*)<<0>>/.source,[T]),lookbehind:!0,inside:{"format-string":{pattern:t(/(^\{(?:(?![}:])<<0>>)*)<<1>>(?=\}$)/.source,[B,ze]),lookbehind:!0,inside:{punctuation:/^:/}},punctuation:/^\{|\}$/,expression:{pattern:/[\s\S]+/,alias:"language-csharp",inside:r.languages.csharp}}},string:/[\s\S]+/}}r.languages.insertBefore("csharp","string",{"interpolation-string":[{pattern:t(/(^|[^\\])(?:\$@|@\$)"(?:""|\\[\s\S]|\{\{|<<0>>|[^\\{"])*"/.source,[pt]),lookbehind:!0,greedy:!0,inside:R(pt,Ue)},{pattern:t(/(^|[^@\\])\$"(?:\\.|\{\{|<<0>>|[^\\"{])*"/.source,[He]),lookbehind:!0,greedy:!0,inside:R(He,ht)}],char:{pattern:RegExp(u),greedy:!0}}),r.languages.dotnet=r.languages.cs=r.languages.csharp})(Prism);(function(r){var e=/\b(?:abstract|assert|boolean|break|byte|case|catch|char|class|const|continue|default|do|double|else|enum|exports|extends|final|finally|float|for|goto|if|implements|import|instanceof|int|interface|long|module|native|new|non-sealed|null|open|opens|package|permits|private|protected|provides|public|record(?!\s*[(){}[\]<>=%~.:,;?+\-*/&|^])|requires|return|sealed|short|static|strictfp|super|switch|synchronized|this|throw|throws|to|transient|transitive|try|uses|var|void|volatile|while|with|yield)\b/,t=/(?:[a-z]\w*\s*\.\s*)*(?:[A-Z]\w*\s*\.\s*)*/.source,n={pattern:RegExp(/(^|[^\w.])/.source+t+/[A-Z](?:[\d_A-Z]*[a-z]\w*)?\b/.source),lookbehind:!0,inside:{namespace:{pattern:/^[a-z]\w*(?:\s*\.\s*[a-z]\w*)*(?:\s*\.)?/,inside:{punctuation:/\./}},punctuation:/\./}};r.languages.java=r.languages.extend("clike",{string:{pattern:/(^|[^\\])"(?:\\.|[^"\\\r\n])*"/,lookbehind:!0,greedy:!0},"class-name":[n,{pattern:RegExp(/(^|[^\w.])/.source+t+/[A-Z]\w*(?=\s+\w+\s*[;,=()]|\s*(?:\[[\s,]*\]\s*)?::\s*new\b)/.source),lookbehind:!0,inside:n.inside},{pattern:RegExp(/(\b(?:class|enum|extends|implements|instanceof|interface|new|record|throws)\s+)/.source+t+/[A-Z]\w*\b/.source),lookbehind:!0,inside:n.inside}],keyword:e,function:[r.languages.clike.function,{pattern:/(::\s*)[a-z_]\w*/,lookbehind:!0}],number:/\b0b[01][01_]*L?\b|\b0x(?:\.[\da-f_p+-]+|[\da-f_]+(?:\.[\da-f_p+-]+)?)\b|(?:\b\d[\d_]*(?:\.[\d_]*)?|\B\.\d[\d_]*)(?:e[+-]?\d[\d_]*)?[dfl]?/i,operator:{pattern:/(^|[^.])(?:<<=?|>>>?=?|->|--|\+\+|&&|\|\||::|[?:~]|[-+*/%&|^!=<>]=?)/m,lookbehind:!0},constant:/\b[A-Z][A-Z_\d]+\b/}),r.languages.insertBefore("java","string",{"triple-quoted-string":{pattern:/"""[ \t]*[\r\n](?:(?:"|"")?(?:\\.|[^"\\]))*"""/,greedy:!0,alias:"string"},char:{pattern:/'(?:\\.|[^'\\\r\n]){1,6}'/,greedy:!0}}),r.languages.insertBefore("java","class-name",{annotation:{pattern:/(^|[^.])@\w+(?:\s*\.\s*\w+)*/,lookbehind:!0,alias:"punctuation"},generics:{pattern:/<(?:[\w\s,.?]|&(?!&)|<(?:[\w\s,.?]|&(?!&)|<(?:[\w\s,.?]|&(?!&)|<(?:[\w\s,.?]|&(?!&))*>)*>)*>)*>/,inside:{"class-name":n,keyword:e,punctuation:/[<>(),.:]/,operator:/[?&|]/}},import:[{pattern:RegExp(/(\bimport\s+)/.source+t+/(?:[A-Z]\w*|\*)(?=\s*;)/.source),lookbehind:!0,inside:{namespace:n.inside.namespace,punctuation:/\./,operator:/\*/,"class-name":/\w+/}},{pattern:RegExp(/(\bimport\s+static\s+)/.source+t+/(?:\w+|\*)(?=\s*;)/.source),lookbehind:!0,alias:"static",inside:{namespace:n.inside.namespace,static:/\b\w+$/,punctuation:/\./,operator:/\*/,"class-name":/\w+/}}],namespace:{pattern:RegExp(/(\b(?:exports|import(?:\s+static)?|module|open|opens|package|provides|requires|to|transitive|uses|with)\s+)(?!)[a-z]\w*(?:\.[a-z]\w*)*\.?/.source.replace(//g,function(){return e.source})),lookbehind:!0,inside:{punctuation:/\./}}})})(Prism);Prism.languages.scala=Prism.languages.extend("java",{"triple-quoted-string":{pattern:/"""[\s\S]*?"""/,greedy:!0,alias:"string"},string:{pattern:/("|')(?:\\.|(?!\1)[^\\\r\n])*\1/,greedy:!0},keyword:/<-|=>|\b(?:abstract|case|catch|class|def|derives|do|else|enum|extends|extension|final|finally|for|forSome|given|if|implicit|import|infix|inline|lazy|match|new|null|object|opaque|open|override|package|private|protected|return|sealed|self|super|this|throw|trait|transparent|try|type|using|val|var|while|with|yield)\b/,number:/\b0x(?:[\da-f]*\.)?[\da-f]+|(?:\b\d+(?:\.\d*)?|\B\.\d+)(?:e\d+)?[dfl]?/i,builtin:/\b(?:Any|AnyRef|AnyVal|Boolean|Byte|Char|Double|Float|Int|Long|Nothing|Short|String|Unit)\b/,symbol:/'[^\d\s\\]\w*/});Prism.languages.insertBefore("scala","triple-quoted-string",{"string-interpolation":{pattern:/\b[a-z]\w*(?:"""(?:[^$]|\$(?:[^{]|\{(?:[^{}]|\{[^{}]*\})*\}))*?"""|"(?:[^$"\r\n]|\$(?:[^{]|\{(?:[^{}]|\{[^{}]*\})*\}))*")/i,greedy:!0,inside:{id:{pattern:/^\w+/,greedy:!0,alias:"function"},escape:{pattern:/\\\$"|\$[$"]/,greedy:!0,alias:"symbol"},interpolation:{pattern:/\$(?:\w+|\{(?:[^{}]|\{[^{}]*\})*\})/,greedy:!0,inside:{punctuation:/^\$\{?|\}$/,expression:{pattern:/[\s\S]+/,inside:Prism.languages.scala}}},string:/[\s\S]+/}}});delete Prism.languages.scala["class-name"];delete Prism.languages.scala.function;delete Prism.languages.scala.constant;(function(r){var e=/(?:\r?\n|\r)[ \t]*\|.+\|(?:(?!\|).)*/.source;r.languages.gherkin={pystring:{pattern:/("""|''')[\s\S]+?\1/,alias:"string"},comment:{pattern:/(^[ \t]*)#.*/m,lookbehind:!0},tag:{pattern:/(^[ \t]*)@\S*/m,lookbehind:!0},feature:{pattern:/((?:^|\r?\n|\r)[ \t]*)(?:Ability|Ahoy matey!|Arwedd|Aspekt|Besigheid Behoefte|Business Need|Caracteristica|Característica|Egenskab|Egenskap|Eiginleiki|Feature|Fīča|Fitur|Fonctionnalité|Fonksyonalite|Funcionalidade|Funcionalitat|Functionalitate|Funcţionalitate|Funcționalitate|Functionaliteit|Fungsi|Funkcia|Funkcija|Funkcionalitāte|Funkcionalnost|Funkcja|Funksie|Funktionalität|Funktionalitéit|Funzionalità|Hwaet|Hwæt|Jellemző|Karakteristik|Lastnost|Mak|Mogucnost|laH|Mogućnost|Moznosti|Možnosti|OH HAI|Omadus|Ominaisuus|Osobina|Özellik|Potrzeba biznesowa|perbogh|poQbogh malja'|Požadavek|Požiadavka|Pretty much|Qap|Qu'meH 'ut|Savybė|Tính năng|Trajto|Vermoë|Vlastnosť|Właściwość|Značilnost|Δυνατότητα|Λειτουργία|Могућност|Мөмкинлек|Особина|Свойство|Үзенчәлеклелек|Функционал|Функционалност|Функция|Функціонал|תכונה|خاصية|خصوصیت|صلاحیت|کاروبار کی ضرورت|وِیژگی|रूप लेख|ਖਾਸੀਅਤ|ਨਕਸ਼ ਨੁਹਾਰ|ਮੁਹਾਂਦਰਾ|గుణము|ಹೆಚ್ಚಳ|ความต้องการทางธุรกิจ|ความสามารถ|โครงหลัก|기능|フィーチャ|功能|機能):(?:[^:\r\n]+(?:\r?\n|\r|$))*/,lookbehind:!0,inside:{important:{pattern:/(:)[^\r\n]+/,lookbehind:!0},keyword:/[^:\r\n]+:/}},scenario:{pattern:/(^[ \t]*)(?:Abstract Scenario|Abstrakt Scenario|Achtergrond|Aer|Ær|Agtergrond|All y'all|Antecedentes|Antecedents|Atburðarás|Atburðarásir|Awww, look mate|B4|Background|Baggrund|Bakgrund|Bakgrunn|Bakgrunnur|Beispiele|Beispiller|Bối cảnh|Cefndir|Cenario|Cenário|Cenario de Fundo|Cenário de Fundo|Cenarios|Cenários|Contesto|Context|Contexte|Contexto|Conto|Contoh|Contone|Dæmi|Dasar|Dead men tell no tales|Delineacao do Cenario|Delineação do Cenário|Dis is what went down|Dữ liệu|Dyagram Senaryo|Dyagram senaryo|Egzanp|Ejemplos|Eksempler|Ekzemploj|Enghreifftiau|Esbozo do escenario|Escenari|Escenario|Esempi|Esquema de l'escenari|Esquema del escenario|Esquema do Cenario|Esquema do Cenário|EXAMPLZ|Examples|Exempel|Exemple|Exemples|Exemplos|First off|Fono|Forgatókönyv|Forgatókönyv vázlat|Fundo|Geçmiş|Grundlage|Hannergrond|ghantoH|Háttér|Heave to|Istorik|Juhtumid|Keadaan|Khung kịch bản|Khung tình huống|Kịch bản|Koncept|Konsep skenario|Kontèks|Kontekst|Kontekstas|Konteksts|Kontext|Konturo de la scenaro|Latar Belakang|lut chovnatlh|lut|lutmey|Lýsing Atburðarásar|Lýsing Dæma|MISHUN SRSLY|MISHUN|Menggariskan Senario|mo'|Náčrt Scenára|Náčrt Scénáře|Náčrt Scenáru|Oris scenarija|Örnekler|Osnova|Osnova Scenára|Osnova scénáře|Osnutek|Ozadje|Paraugs|Pavyzdžiai|Példák|Piemēri|Plan du scénario|Plan du Scénario|Plan Senaryo|Plan senaryo|Plang vum Szenario|Pozadí|Pozadie|Pozadina|Príklady|Příklady|Primer|Primeri|Primjeri|Przykłady|Raamstsenaarium|Reckon it's like|Rerefons|Scenár|Scénář|Scenarie|Scenarij|Scenarijai|Scenarijaus šablonas|Scenariji|Scenārijs|Scenārijs pēc parauga|Scenarijus|Scenario|Scénario|Scenario Amlinellol|Scenario Outline|Scenario Template|Scenariomal|Scenariomall|Scenarios|Scenariu|Scenariusz|Scenaro|Schema dello scenario|Se ðe|Se the|Se þe|Senario|Senaryo Deskripsyon|Senaryo deskripsyon|Senaryo|Senaryo taslağı|Shiver me timbers|Situācija|Situai|Situasie Uiteensetting|Situasie|Skenario konsep|Skenario|Skica|Structura scenariu|Structură scenariu|Struktura scenarija|Stsenaarium|Swa hwaer swa|Swa|Swa hwær swa|Szablon scenariusza|Szenario|Szenariogrundriss|Tapaukset|Tapaus|Tapausaihio|Taust|Tausta|Template Keadaan|Template Senario|Template Situai|The thing of it is|Tình huống|Variantai|Voorbeelde|Voorbeelden|Wharrimean is|Yo-ho-ho|You'll wanna|Założenia|Παραδείγματα|Περιγραφή Σεναρίου|Σενάρια|Σενάριο|Υπόβαθρο|Кереш|Контекст|Концепт|Мисаллар|Мисоллар|Основа|Передумова|Позадина|Предистория|Предыстория|Приклади|Пример|Примери|Примеры|Рамка на сценарий|Скица|Структура сценарија|Структура сценария|Структура сценарію|Сценарий|Сценарий структураси|Сценарийның төзелеше|Сценарији|Сценарио|Сценарій|Тарих|Үрнәкләр|דוגמאות|רקע|תבנית תרחיש|תרחיש|الخلفية|الگوی سناریو|امثلة|پس منظر|زمینه|سناریو|سيناريو|سيناريو مخطط|مثالیں|منظر نامے کا خاکہ|منظرنامہ|نمونه ها|उदाहरण|परिदृश्य|परिदृश्य रूपरेखा|पृष्ठभूमि|ਉਦਾਹਰਨਾਂ|ਪਟਕਥਾ|ਪਟਕਥਾ ਢਾਂਚਾ|ਪਟਕਥਾ ਰੂਪ ਰੇਖਾ|ਪਿਛੋਕੜ|ఉదాహరణలు|కథనం|నేపథ్యం|సన్నివేశం|ಉದಾಹರಣೆಗಳು|ಕಥಾಸಾರಾಂಶ|ವಿವರಣೆ|ಹಿನ್ನೆಲೆ|โครงสร้างของเหตุการณ์|ชุดของตัวอย่าง|ชุดของเหตุการณ์|แนวคิด|สรุปเหตุการณ์|เหตุการณ์|배경|시나리오|시나리오 개요|예|サンプル|シナリオ|シナリオアウトライン|シナリオテンプレ|シナリオテンプレート|テンプレ|例|例子|剧本|剧本大纲|劇本|劇本大綱|场景|场景大纲|場景|場景大綱|背景):[^:\r\n]*/m,lookbehind:!0,inside:{important:{pattern:/(:)[^\r\n]*/,lookbehind:!0},keyword:/[^:\r\n]+:/}},"table-body":{pattern:RegExp("("+e+")(?:"+e+")+"),lookbehind:!0,inside:{outline:{pattern:/<[^>]+>/,alias:"variable"},td:{pattern:/\s*[^\s|][^|]*/,alias:"string"},punctuation:/\|/}},"table-head":{pattern:RegExp(e),inside:{th:{pattern:/\s*[^\s|][^|]*/,alias:"variable"},punctuation:/\|/}},atrule:{pattern:/(^[ \t]+)(?:'a|'ach|'ej|7|a|A také|A taktiež|A tiež|A zároveň|Aber|Ac|Adott|Akkor|Ak|Aleshores|Ale|Ali|Allora|Alors|Als|Ama|Amennyiben|Amikor|Ampak|an|AN|Ananging|And y'all|And|Angenommen|Anrhegedig a|An|Apabila|Atès|Atesa|Atunci|Avast!|Aye|A|awer|Bagi|Banjur|Bet|Biết|Blimey!|Buh|But at the end of the day I reckon|But y'all|But|BUT|Cal|Când|Cand|Cando|Ce|Cuando|Če|Ða ðe|Ða|Dadas|Dada|Dados|Dado|DaH ghu' bejlu'|dann|Dann|Dano|Dan|Dar|Dat fiind|Data|Date fiind|Date|Dati fiind|Dati|Daţi fiind|Dați fiind|DEN|Dato|De|Den youse gotta|Dengan|Diberi|Diyelim ki|Donada|Donat|Donitaĵo|Do|Dun|Duota|Ðurh|Eeldades|Ef|Eğer ki|Entao|Então|Entón|E|En|Entonces|Epi|És|Etant donnée|Etant donné|Et|Étant données|Étant donnée|Étant donné|Etant données|Etant donnés|Étant donnés|Fakat|Gangway!|Gdy|Gegeben seien|Gegeben sei|Gegeven|Gegewe|ghu' noblu'|Gitt|Given y'all|Given|Givet|Givun|Ha|Cho|I CAN HAZ|In|Ir|It's just unbelievable|I|Ja|Jeśli|Jeżeli|Kad|Kada|Kadar|Kai|Kaj|Když|Keď|Kemudian|Ketika|Khi|Kiedy|Ko|Kuid|Kui|Kun|Lan|latlh|Le sa a|Let go and haul|Le|Lè sa a|Lè|Logo|Lorsqu'<|Lorsque|mä|Maar|Mais|Mając|Ma|Majd|Maka|Manawa|Mas|Men|Menawa|Mutta|Nalika|Nalikaning|Nanging|Når|När|Nato|Nhưng|Niin|Njuk|O zaman|Och|Og|Oletetaan|Ond|Onda|Oraz|Pak|Pero|Però|Podano|Pokiaľ|Pokud|Potem|Potom|Privzeto|Pryd|Quan|Quand|Quando|qaSDI'|Så|Sed|Se|Siis|Sipoze ke|Sipoze Ke|Sipoze|Si|Şi|Și|Soit|Stel|Tada|Tad|Takrat|Tak|Tapi|Ter|Tetapi|Tha the|Tha|Then y'all|Then|Thì|Thurh|Toda|Too right|Un|Und|ugeholl|Và|vaj|Vendar|Ve|wann|Wanneer|WEN|Wenn|When y'all|When|Wtedy|Wun|Y'know|Yeah nah|Yna|Youse know like when|Youse know when youse got|Y|Za predpokladu|Za předpokladu|Zadan|Zadani|Zadano|Zadate|Zadato|Zakładając|Zaradi|Zatati|Þa þe|Þa|Þá|Þegar|Þurh|Αλλά|Δεδομένου|Και|Όταν|Τότε|А також|Агар|Але|Али|Аммо|А|Әгәр|Әйтик|Әмма|Бирок|Ва|Вә|Дадено|Дано|Допустим|Если|Задате|Задати|Задато|И|І|К тому же|Када|Кад|Когато|Когда|Коли|Ләкин|Лекин|Нәтиҗәдә|Нехай|Но|Онда|Припустимо, що|Припустимо|Пусть|Также|Та|Тогда|Тоді|То|Унда|Һәм|Якщо|אבל|אזי|אז|בהינתן|וגם|כאשר|آنگاه|اذاً|اگر|اما|اور|با فرض|بالفرض|بفرض|پھر|تب|ثم|جب|عندما|فرض کیا|لكن|لیکن|متى|هنگامی|و|अगर|और|कदा|किन्तु|चूंकि|जब|तथा|तदा|तब|परन्तु|पर|यदि|ਅਤੇ|ਜਦੋਂ|ਜਿਵੇਂ ਕਿ|ਜੇਕਰ|ਤਦ|ਪਰ|అప్పుడు|ఈ పరిస్థితిలో|కాని|చెప్పబడినది|మరియు|ಆದರೆ|ನಂತರ|ನೀಡಿದ|ಮತ್ತು|ಸ್ಥಿತಿಯನ್ನು|กำหนดให้|ดังนั้น|แต่|เมื่อ|และ|그러면<|그리고<|단<|만약<|만일<|먼저<|조건<|하지만<|かつ<|しかし<|ただし<|ならば<|もし<|並且<|但し<|但是<|假如<|假定<|假設<|假设<|前提<|同时<|同時<|并且<|当<|當<|而且<|那么<|那麼<)(?=[ \t])/m,lookbehind:!0},string:{pattern:/"(?:\\.|[^"\\\r\n])*"|'(?:\\.|[^'\\\r\n])*'/,inside:{outline:{pattern:/<[^>]+>/,alias:"variable"}}},outline:{pattern:/<[^>]+>/,alias:"variable"}}})(Prism);Prism.languages.markup={comment:{pattern://,greedy:!0},prolog:{pattern:/<\?[\s\S]+?\?>/,greedy:!0},doctype:{pattern:/"'[\]]|"[^"]*"|'[^']*')+(?:\[(?:[^<"'\]]|"[^"]*"|'[^']*'|<(?!!--)|)*\]\s*)?>/i,greedy:!0,inside:{"internal-subset":{pattern:/(^[^\[]*\[)[\s\S]+(?=\]>$)/,lookbehind:!0,greedy:!0,inside:null},string:{pattern:/"[^"]*"|'[^']*'/,greedy:!0},punctuation:/^$|[[\]]/,"doctype-tag":/^DOCTYPE/i,name:/[^\s<>'"]+/}},cdata:{pattern://i,greedy:!0},tag:{pattern:/<\/?(?!\d)[^\s>\/=$<%]+(?:\s(?:\s*[^\s>\/=]+(?:\s*=\s*(?:"[^"]*"|'[^']*'|[^\s'">=]+(?=[\s>]))|(?=[\s/>])))+)?\s*\/?>/,greedy:!0,inside:{tag:{pattern:/^<\/?[^\s>\/]+/,inside:{punctuation:/^<\/?/,namespace:/^[^\s>\/:]+:/}},"special-attr":[],"attr-value":{pattern:/=\s*(?:"[^"]*"|'[^']*'|[^\s'">=]+)/,inside:{punctuation:[{pattern:/^=/,alias:"attr-equals"},{pattern:/^(\s*)["']|["']$/,lookbehind:!0}]}},punctuation:/\/?>/,"attr-name":{pattern:/[^\s>\/]+/,inside:{namespace:/^[^\s>\/:]+:/}}}},entity:[{pattern:/&[\da-z]{1,8};/i,alias:"named-entity"},/?[\da-f]{1,8};/i]};Prism.languages.markup.tag.inside["attr-value"].inside.entity=Prism.languages.markup.entity;Prism.languages.markup.doctype.inside["internal-subset"].inside=Prism.languages.markup;Prism.hooks.add("wrap",function(r){r.type==="entity"&&(r.attributes.title=r.content.replace(/&/,"&"))});Object.defineProperty(Prism.languages.markup.tag,"addInlined",{value:function(e,t){var n={};n["language-"+t]={pattern:/(^$)/i,lookbehind:!0,inside:Prism.languages[t]},n.cdata=/^$/i;var i={"included-cdata":{pattern://i,inside:n}};i["language-"+t]={pattern:/[\s\S]+/,inside:Prism.languages[t]};var s={};s[e]={pattern:RegExp(/(<__[^>]*>)(?:))*\]\]>|(?!)/.source.replace(/__/g,function(){return e}),"i"),lookbehind:!0,greedy:!0,inside:i},Prism.languages.insertBefore("markup","cdata",s)}});Object.defineProperty(Prism.languages.markup.tag,"addAttribute",{value:function(r,e){Prism.languages.markup.tag.inside["special-attr"].push({pattern:RegExp(/(^|["'\s])/.source+"(?:"+r+")"+/\s*=\s*(?:"[^"]*"|'[^']*'|[^\s'">=]+(?=[\s>]))/.source,"i"),lookbehind:!0,inside:{"attr-name":/^[^\s=]+/,"attr-value":{pattern:/=[\s\S]+/,inside:{value:{pattern:/(^=\s*(["']|(?!["'])))\S[\s\S]*(?=\2$)/,lookbehind:!0,alias:[e,"language-"+e],inside:Prism.languages[e]},punctuation:[{pattern:/^=/,alias:"attr-equals"},/"|'/]}}}})}});Prism.languages.html=Prism.languages.markup;Prism.languages.mathml=Prism.languages.markup;Prism.languages.svg=Prism.languages.markup;Prism.languages.xml=Prism.languages.extend("markup",{});Prism.languages.ssml=Prism.languages.xml;Prism.languages.atom=Prism.languages.xml;Prism.languages.rss=Prism.languages.xml;(function(r){function e(t,n){return"___"+t.toUpperCase()+n+"___"}Object.defineProperties(r.languages["markup-templating"]={},{buildPlaceholders:{value:function(t,n,i,s){if(t.language===n){var a=t.tokenStack=[];t.code=t.code.replace(i,function(o){if(typeof s=="function"&&!s(o))return o;for(var l=a.length,c;t.code.indexOf(c=e(n,l))!==-1;)++l;return a[l]=o,c}),t.grammar=r.languages.markup}}},tokenizePlaceholders:{value:function(t,n){if(t.language!==n||!t.tokenStack)return;t.grammar=r.languages[n];var i=0,s=Object.keys(t.tokenStack);function a(o){for(var l=0;l=s.length);l++){var c=o[l];if(typeof c=="string"||c.content&&typeof c.content=="string"){var g=s[i],d=t.tokenStack[g],m=typeof c=="string"?c:c.content,y=e(n,g),$=m.indexOf(y);if($>-1){++i;var k=m.substring(0,$),F=new r.Token(n,r.tokenize(d,t.grammar),"language-"+n,d),z=m.substring($+y.length),h=[];k&&h.push.apply(h,a([k])),h.push(F),z&&h.push.apply(h,a([z])),typeof c=="string"?o.splice.apply(o,[l,1].concat(h)):c.content=h}}else c.content&&a(c.content)}return o}a(t.tokens)}}})})(Prism);(function(r){var e=/\/\*[\s\S]*?\*\/|\/\/.*|#(?!\[).*/,t=[{pattern:/\b(?:false|true)\b/i,alias:"boolean"},{pattern:/(::\s*)\b[a-z_]\w*\b(?!\s*\()/i,greedy:!0,lookbehind:!0},{pattern:/(\b(?:case|const)\s+)\b[a-z_]\w*(?=\s*[;=])/i,greedy:!0,lookbehind:!0},/\b(?:null)\b/i,/\b[A-Z_][A-Z0-9_]*\b(?!\s*\()/],n=/\b0b[01]+(?:_[01]+)*\b|\b0o[0-7]+(?:_[0-7]+)*\b|\b0x[\da-f]+(?:_[\da-f]+)*\b|(?:\b\d+(?:_\d+)*\.?(?:\d+(?:_\d+)*)?|\B\.\d+)(?:e[+-]?\d+)?/i,i=/=>|\?\?=?|\.{3}|\??->|[!=]=?=?|::|\*\*=?|--|\+\+|&&|\|\||<<|>>|[?~]|[/^|%*&<>.+-]=?/,s=/[{}\[\](),:;]/;r.languages.php={delimiter:{pattern:/\?>$|^<\?(?:php(?=\s)|=)?/i,alias:"important"},comment:e,variable:/\$+(?:\w+\b|(?=\{))/,package:{pattern:/(namespace\s+|use\s+(?:function\s+)?)(?:\\?\b[a-z_]\w*)+\b(?!\\)/i,lookbehind:!0,inside:{punctuation:/\\/}},"class-name-definition":{pattern:/(\b(?:class|enum|interface|trait)\s+)\b[a-z_]\w*(?!\\)\b/i,lookbehind:!0,alias:"class-name"},"function-definition":{pattern:/(\bfunction\s+)[a-z_]\w*(?=\s*\()/i,lookbehind:!0,alias:"function"},keyword:[{pattern:/(\(\s*)\b(?:array|bool|boolean|float|int|integer|object|string)\b(?=\s*\))/i,alias:"type-casting",greedy:!0,lookbehind:!0},{pattern:/([(,?]\s*)\b(?:array(?!\s*\()|bool|callable|(?:false|null)(?=\s*\|)|float|int|iterable|mixed|object|self|static|string)\b(?=\s*\$)/i,alias:"type-hint",greedy:!0,lookbehind:!0},{pattern:/(\)\s*:\s*(?:\?\s*)?)\b(?:array(?!\s*\()|bool|callable|(?:false|null)(?=\s*\|)|float|int|iterable|mixed|never|object|self|static|string|void)\b/i,alias:"return-type",greedy:!0,lookbehind:!0},{pattern:/\b(?:array(?!\s*\()|bool|float|int|iterable|mixed|object|string|void)\b/i,alias:"type-declaration",greedy:!0},{pattern:/(\|\s*)(?:false|null)\b|\b(?:false|null)(?=\s*\|)/i,alias:"type-declaration",greedy:!0,lookbehind:!0},{pattern:/\b(?:parent|self|static)(?=\s*::)/i,alias:"static-context",greedy:!0},{pattern:/(\byield\s+)from\b/i,lookbehind:!0},/\bclass\b/i,{pattern:/((?:^|[^\s>:]|(?:^|[^-])>|(?:^|[^:]):)\s*)\b(?:abstract|and|array|as|break|callable|case|catch|clone|const|continue|declare|default|die|do|echo|else|elseif|empty|enddeclare|endfor|endforeach|endif|endswitch|endwhile|enum|eval|exit|extends|final|finally|fn|for|foreach|function|global|goto|if|implements|include|include_once|instanceof|insteadof|interface|isset|list|match|namespace|never|new|or|parent|print|private|protected|public|readonly|require|require_once|return|self|static|switch|throw|trait|try|unset|use|var|while|xor|yield|__halt_compiler)\b/i,lookbehind:!0}],"argument-name":{pattern:/([(,]\s*)\b[a-z_]\w*(?=\s*:(?!:))/i,lookbehind:!0},"class-name":[{pattern:/(\b(?:extends|implements|instanceof|new(?!\s+self|\s+static))\s+|\bcatch\s*\()\b[a-z_]\w*(?!\\)\b/i,greedy:!0,lookbehind:!0},{pattern:/(\|\s*)\b[a-z_]\w*(?!\\)\b/i,greedy:!0,lookbehind:!0},{pattern:/\b[a-z_]\w*(?!\\)\b(?=\s*\|)/i,greedy:!0},{pattern:/(\|\s*)(?:\\?\b[a-z_]\w*)+\b/i,alias:"class-name-fully-qualified",greedy:!0,lookbehind:!0,inside:{punctuation:/\\/}},{pattern:/(?:\\?\b[a-z_]\w*)+\b(?=\s*\|)/i,alias:"class-name-fully-qualified",greedy:!0,inside:{punctuation:/\\/}},{pattern:/(\b(?:extends|implements|instanceof|new(?!\s+self\b|\s+static\b))\s+|\bcatch\s*\()(?:\\?\b[a-z_]\w*)+\b(?!\\)/i,alias:"class-name-fully-qualified",greedy:!0,lookbehind:!0,inside:{punctuation:/\\/}},{pattern:/\b[a-z_]\w*(?=\s*\$)/i,alias:"type-declaration",greedy:!0},{pattern:/(?:\\?\b[a-z_]\w*)+(?=\s*\$)/i,alias:["class-name-fully-qualified","type-declaration"],greedy:!0,inside:{punctuation:/\\/}},{pattern:/\b[a-z_]\w*(?=\s*::)/i,alias:"static-context",greedy:!0},{pattern:/(?:\\?\b[a-z_]\w*)+(?=\s*::)/i,alias:["class-name-fully-qualified","static-context"],greedy:!0,inside:{punctuation:/\\/}},{pattern:/([(,?]\s*)[a-z_]\w*(?=\s*\$)/i,alias:"type-hint",greedy:!0,lookbehind:!0},{pattern:/([(,?]\s*)(?:\\?\b[a-z_]\w*)+(?=\s*\$)/i,alias:["class-name-fully-qualified","type-hint"],greedy:!0,lookbehind:!0,inside:{punctuation:/\\/}},{pattern:/(\)\s*:\s*(?:\?\s*)?)\b[a-z_]\w*(?!\\)\b/i,alias:"return-type",greedy:!0,lookbehind:!0},{pattern:/(\)\s*:\s*(?:\?\s*)?)(?:\\?\b[a-z_]\w*)+\b(?!\\)/i,alias:["class-name-fully-qualified","return-type"],greedy:!0,lookbehind:!0,inside:{punctuation:/\\/}}],constant:t,function:{pattern:/(^|[^\\\w])\\?[a-z_](?:[\w\\]*\w)?(?=\s*\()/i,lookbehind:!0,inside:{punctuation:/\\/}},property:{pattern:/(->\s*)\w+/,lookbehind:!0},number:n,operator:i,punctuation:s};var a={pattern:/\{\$(?:\{(?:\{[^{}]+\}|[^{}]+)\}|[^{}])+\}|(^|[^\\{])\$+(?:\w+(?:\[[^\r\n\[\]]+\]|->\w+)?)/,lookbehind:!0,inside:r.languages.php},o=[{pattern:/<<<'([^']+)'[\r\n](?:.*[\r\n])*?\1;/,alias:"nowdoc-string",greedy:!0,inside:{delimiter:{pattern:/^<<<'[^']+'|[a-z_]\w*;$/i,alias:"symbol",inside:{punctuation:/^<<<'?|[';]$/}}}},{pattern:/<<<(?:"([^"]+)"[\r\n](?:.*[\r\n])*?\1;|([a-z_]\w*)[\r\n](?:.*[\r\n])*?\2;)/i,alias:"heredoc-string",greedy:!0,inside:{delimiter:{pattern:/^<<<(?:"[^"]+"|[a-z_]\w*)|[a-z_]\w*;$/i,alias:"symbol",inside:{punctuation:/^<<<"?|[";]$/}},interpolation:a}},{pattern:/`(?:\\[\s\S]|[^\\`])*`/,alias:"backtick-quoted-string",greedy:!0},{pattern:/'(?:\\[\s\S]|[^\\'])*'/,alias:"single-quoted-string",greedy:!0},{pattern:/"(?:\\[\s\S]|[^\\"])*"/,alias:"double-quoted-string",greedy:!0,inside:{interpolation:a}}];r.languages.insertBefore("php","variable",{string:o,attribute:{pattern:/#\[(?:[^"'\/#]|\/(?![*/])|\/\/.*$|#(?!\[).*$|\/\*(?:[^*]|\*(?!\/))*\*\/|"(?:\\[\s\S]|[^\\"])*"|'(?:\\[\s\S]|[^\\'])*')+\](?=\s*[a-z$#])/im,greedy:!0,inside:{"attribute-content":{pattern:/^(#\[)[\s\S]+(?=\]$)/,lookbehind:!0,inside:{comment:e,string:o,"attribute-class-name":[{pattern:/([^:]|^)\b[a-z_]\w*(?!\\)\b/i,alias:"class-name",greedy:!0,lookbehind:!0},{pattern:/([^:]|^)(?:\\?\b[a-z_]\w*)+/i,alias:["class-name","class-name-fully-qualified"],greedy:!0,lookbehind:!0,inside:{punctuation:/\\/}}],constant:t,number:n,operator:i,punctuation:s}},delimiter:{pattern:/^#\[|\]$/,alias:"punctuation"}}}}),r.hooks.add("before-tokenize",function(l){if(/<\?/.test(l.code)){var c=/<\?(?:[^"'/#]|\/(?![*/])|("|')(?:\\[\s\S]|(?!\1)[^\\])*\1|(?:\/\/|#(?!\[))(?:[^?\n\r]|\?(?!>))*(?=$|\?>|[\r\n])|#\[|\/\*(?:[^*]|\*(?!\/))*(?:\*\/|$))*?(?:\?>|$)/g;r.languages["markup-templating"].buildPlaceholders(l,"php",c)}}),r.hooks.add("after-tokenize",function(l){r.languages["markup-templating"].tokenizePlaceholders(l,"php")})})(Prism);const an="(if|else if|await|then|catch|each|html|debug)";Prism.languages.svelte=Prism.languages.extend("markup",{each:{pattern:new RegExp("{[#/]each(?:(?:\\{(?:(?:\\{(?:[^{}])*\\})|(?:[^{}]))*\\})|(?:[^{}]))*}"),inside:{"language-javascript":[{pattern:/(as[\s\S]*)\([\s\S]*\)(?=\s*\})/,lookbehind:!0,inside:Prism.languages.javascript},{pattern:/(as[\s]*)[\s\S]*(?=\s*)/,lookbehind:!0,inside:Prism.languages.javascript},{pattern:/(#each[\s]*)[\s\S]*(?=as)/,lookbehind:!0,inside:Prism.languages.javascript}],keyword:/[#/]each|as/,punctuation:/{|}/}},block:{pattern:new RegExp("{[#:/@]/s"+an+"(?:(?:\\{(?:(?:\\{(?:[^{}])*\\})|(?:[^{}]))*\\})|(?:[^{}]))*}"),inside:{punctuation:/^{|}$/,keyword:[new RegExp("[#:/@]"+an+"( )*"),/as/,/then/],"language-javascript":{pattern:/[\s\S]*/,inside:Prism.languages.javascript}}},tag:{pattern:/<\/?(?!\d)[^\s>\/=$<%]+(?:\s(?:\s*[^\s>\/=]+(?:\s*=\s*(?:(?:"[^"]*"|'[^']*'|[^\s'">=]+(?=[\s>]))|(?:"[^"]*"|'[^']*'|{[\s\S]+?}(?=[\s/>])))|(?=[\s/>])))+)?\s*\/?>/i,greedy:!0,inside:{tag:{pattern:/^<\/?[^\s>\/]+/i,inside:{punctuation:/^<\/?/,namespace:/^[^\s>\/:]+:/}},"language-javascript":{pattern:/\{(?:(?:\{(?:(?:\{(?:[^{}])*\})|(?:[^{}]))*\})|(?:[^{}]))*\}/,inside:Prism.languages.javascript},"attr-value":{pattern:/=\s*(?:"[^"]*"|'[^']*'|[^\s'">=]+)/i,inside:{punctuation:[/^=/,{pattern:/^(\s*)["']|["']$/,lookbehind:!0}],"language-javascript":{pattern:/{[\s\S]+}/,inside:Prism.languages.javascript}}},punctuation:/\/?>/,"attr-name":{pattern:/[^\s>\/]+/,inside:{namespace:/^[^\s>\/:]+:/}}}},"language-javascript":{pattern:/\{(?:(?:\{(?:(?:\{(?:[^{}])*\})|(?:[^{}]))*\})|(?:[^{}]))*\}/,lookbehind:!0,inside:Prism.languages.javascript}});Prism.languages.svelte.tag.inside["attr-value"].inside.entity=Prism.languages.svelte.entity;Prism.hooks.add("wrap",r=>{r.type==="entity"&&(r.attributes.title=r.content.replace(/&/,"&"))});Object.defineProperty(Prism.languages.svelte.tag,"addInlined",{value:function(e,t){const n={};n["language-"+t]={pattern:/(^$)/i,lookbehind:!0,inside:Prism.languages[t]},n.cdata=/^$/i;const i={"included-cdata":{pattern://i,inside:n}};i["language-"+t]={pattern:/[\s\S]+/,inside:Prism.languages[t]};const s={};s[e]={pattern:RegExp(/(<__[\s\S]*?>)(?:\s*|[\s\S])*?(?=<\/__>)/.source.replace(/__/g,e),"i"),lookbehind:!0,greedy:!0,inside:i},Prism.languages.insertBefore("svelte","cdata",s)}});Prism.languages.svelte.tag.addInlined("style","css");Prism.languages.svelte.tag.addInlined("script","javascript");(function(){typeof Prism>"u"||typeof document>"u"||!document.createRange||(Prism.plugins.KeepMarkup=!0,Prism.hooks.add("before-highlight",function(r){if(!r.element.children.length||!Prism.util.isActive(r.element,"keep-markup",!0))return;var e=Prism.util.isActive(r.element,"drop-tokens",!1);function t(o){return!(e&&o.nodeName.toLowerCase()==="span"&&o.classList.contains("token"))}var n=0,i=[];function s(o){if(!t(o)){a(o);return}var l={element:o,posOpen:n};i.push(l),a(o),l.posClose=n}function a(o){for(var l=0,c=o.childNodes.length;ln.node.posOpen&&(n.nodeStart=a,n.nodeStartPos=n.node.posOpen-n.pos),n.nodeStart&&n.pos+a.data.length>=n.node.posClose&&(n.nodeEnd=a,n.nodeEndPos=n.node.posClose-n.pos),n.pos+=a.data.length);if(n.nodeStart&&n.nodeEnd){var o=document.createRange();return o.setStart(n.nodeStart,n.nodeStartPos),o.setEnd(n.nodeEnd,n.nodeEndPos),n.node.element.innerHTML="",n.node.element.appendChild(o.extractContents()),o.insertNode(n.node.element),o.detach(),!1}}return!0};r.keepMarkup.forEach(function(t){e(r.element,{node:t,pos:0})}),r.highlightedCode=r.element.innerHTML}}))})();const U=K(xs),Dn=K($s),_s=K(ks);var lr=function(r,e){return lr=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(t,n){t.__proto__=n}||function(t,n){for(var i in n)Object.prototype.hasOwnProperty.call(n,i)&&(t[i]=n[i])},lr(r,e)};function he(r,e){if(typeof e!="function"&&e!==null)throw new TypeError("Class extends value "+String(e)+" is not a constructor or null");lr(r,e);function t(){this.constructor=r}r.prototype=e===null?Object.create(e):(t.prototype=e.prototype,new t)}function As(r,e,t,n){function i(s){return s instanceof t?s:new t(function(a){a(s)})}return new(t||(t=Promise))(function(s,a){function o(g){try{c(n.next(g))}catch(d){a(d)}}function l(g){try{c(n.throw(g))}catch(d){a(d)}}function c(g){g.done?s(g.value):i(g.value).then(o,l)}c((n=n.apply(r,e||[])).next())})}function Bn(r,e){var t={label:0,sent:function(){if(s[0]&1)throw s[1];return s[1]},trys:[],ops:[]},n,i,s,a;return a={next:o(0),throw:o(1),return:o(2)},typeof Symbol=="function"&&(a[Symbol.iterator]=function(){return this}),a;function o(c){return function(g){return l([c,g])}}function l(c){if(n)throw new TypeError("Generator is already executing.");for(;a&&(a=0,c[0]&&(t=0)),t;)try{if(n=1,i&&(s=c[0]&2?i.return:c[0]?i.throw||((s=i.return)&&s.call(i),0):i.next)&&!(s=s.call(i,c[1])).done)return s;switch(i=0,s&&(c=[c[0]&2,s.value]),c[0]){case 0:case 1:s=c;break;case 4:return t.label++,{value:c[1],done:!1};case 5:t.label++,i=c[1],c=[0];continue;case 7:c=t.ops.pop(),t.trys.pop();continue;default:if(s=t.trys,!(s=s.length>0&&s[s.length-1])&&(c[0]===6||c[0]===2)){t=0;continue}if(c[0]===3&&(!s||c[1]>s[0]&&c[1]=r.length&&(r=void 0),{value:r&&r[n++],done:!r}}};throw new TypeError(e?"Object is not iterable.":"Symbol.iterator is not defined.")}function de(r,e){var t=typeof Symbol=="function"&&r[Symbol.iterator];if(!t)return r;var n=t.call(r),i,s=[],a;try{for(;(e===void 0||e-- >0)&&!(i=n.next()).done;)s.push(i.value)}catch(o){a={error:o}}finally{try{i&&!i.done&&(t=n.return)&&t.call(n)}finally{if(a)throw a.error}}return s}function Se(r,e,t){if(t||arguments.length===2)for(var n=0,i=e.length,s;n1||o(m,y)})})}function o(m,y){try{l(n[m](y))}catch($){d(s[0][3],$)}}function l(m){m.value instanceof Re?Promise.resolve(m.value.v).then(c,g):d(s[0][2],m)}function c(m){o("next",m)}function g(m){o("throw",m)}function d(m,y){m(y),s.shift(),s.length&&o(s[0][0],s[0][1])}}function Cs(r){if(!Symbol.asyncIterator)throw new TypeError("Symbol.asyncIterator is not defined.");var e=r[Symbol.asyncIterator],t;return e?e.call(r):(r=typeof je=="function"?je(r):r[Symbol.iterator](),t={},n("next"),n("throw"),n("return"),t[Symbol.asyncIterator]=function(){return this},t);function n(s){t[s]=r[s]&&function(a){return new Promise(function(o,l){a=r[s](a),i(o,l,a.done,a.value)})}}function i(s,a,o,l){Promise.resolve(l).then(function(c){s({value:c,done:o})},a)}}function E(r){return typeof r=="function"}function Nn(r){var e=function(n){Error.call(n),n.stack=new Error().stack},t=r(e);return t.prototype=Object.create(Error.prototype),t.prototype.constructor=t,t}var Xt=Nn(function(r){return function(t){r(this),this.message=t?t.length+` errors occurred during unsubscription:
`+t.map(function(n,i){return i+1+") "+n.toString()}).join(`
`):"",this.name="UnsubscriptionError",this.errors=t}});function At(r,e){if(r){var t=r.indexOf(e);0<=t&&r.splice(t,1)}}var Be=function(){function r(e){this.initialTeardown=e,this.closed=!1,this._parentage=null,this._finalizers=null}return r.prototype.unsubscribe=function(){var e,t,n,i,s;if(!this.closed){this.closed=!0;var a=this._parentage;if(a)if(this._parentage=null,Array.isArray(a))try{for(var o=je(a),l=o.next();!l.done;l=o.next()){var c=l.value;c.remove(this)}}catch(k){e={error:k}}finally{try{l&&!l.done&&(t=o.return)&&t.call(o)}finally{if(e)throw e.error}}else a.remove(this);var g=this.initialTeardown;if(E(g))try{g()}catch(k){s=k instanceof Xt?k.errors:[k]}var d=this._finalizers;if(d){this._finalizers=null;try{for(var m=je(d),y=m.next();!y.done;y=m.next()){var $=y.value;try{on($)}catch(k){s=s??[],k instanceof Xt?s=Se(Se([],de(s)),de(k.errors)):s.push(k)}}}catch(k){n={error:k}}finally{try{y&&!y.done&&(i=m.return)&&i.call(m)}finally{if(n)throw n.error}}}if(s)throw new Xt(s)}},r.prototype.add=function(e){var t;if(e&&e!==this)if(this.closed)on(e);else{if(e instanceof r){if(e.closed||e._hasParent(this))return;e._addParent(this)}(this._finalizers=(t=this._finalizers)!==null&&t!==void 0?t:[]).push(e)}},r.prototype._hasParent=function(e){var t=this._parentage;return t===e||Array.isArray(t)&&t.includes(e)},r.prototype._addParent=function(e){var t=this._parentage;this._parentage=Array.isArray(t)?(t.push(e),t):t?[t,e]:e},r.prototype._removeParent=function(e){var t=this._parentage;t===e?this._parentage=null:Array.isArray(t)&&At(t,e)},r.prototype.remove=function(e){var t=this._finalizers;t&&At(t,e),e instanceof r&&e._removeParent(this)},r.EMPTY=function(){var e=new r;return e.closed=!0,e}(),r}(),Ln=Be.EMPTY;function Un(r){return r instanceof Be||r&&"closed"in r&&E(r.remove)&&E(r.add)&&E(r.unsubscribe)}function on(r){E(r)?r():r.unsubscribe()}var Hn={onUnhandledError:null,onStoppedNotification:null,Promise:void 0,useDeprecatedSynchronousErrorHandling:!1,useDeprecatedNextContext:!1},cr={setTimeout:function(r,e){for(var t=[],n=2;n0},enumerable:!1,configurable:!0}),e.prototype._trySubscribe=function(t){return this._throwIfClosed(),r.prototype._trySubscribe.call(this,t)},e.prototype._subscribe=function(t){return this._throwIfClosed(),this._checkFinalizedStatuses(t),this._innerSubscribe(t)},e.prototype._innerSubscribe=function(t){var n=this,i=this,s=i.hasError,a=i.isStopped,o=i.observers;return s||a?Ln:(this.currentObservers=null,o.push(t),new Be(function(){n.currentObservers=null,At(o,t)}))},e.prototype._checkFinalizedStatuses=function(t){var n=this,i=n.hasError,s=n.thrownError,a=n.isStopped;i?t.error(s):a&&t.complete()},e.prototype.asObservable=function(){var t=new L;return t.source=this,t},e.create=function(t,n){return new cn(t,n)},e}(L),cn=function(r){he(e,r);function e(t,n){var i=r.call(this)||this;return i.destination=t,i.source=n,i}return e.prototype.next=function(t){var n,i;(i=(n=this.destination)===null||n===void 0?void 0:n.next)===null||i===void 0||i.call(n,t)},e.prototype.error=function(t){var n,i;(i=(n=this.destination)===null||n===void 0?void 0:n.error)===null||i===void 0||i.call(n,t)},e.prototype.complete=function(){var t,n;(n=(t=this.destination)===null||t===void 0?void 0:t.complete)===null||n===void 0||n.call(t)},e.prototype._subscribe=function(t){var n,i;return(i=(n=this.source)===null||n===void 0?void 0:n.subscribe(t))!==null&&i!==void 0?i:Ln},e}(qn),Vn={now:function(){return(Vn.delegate||Date).now()},delegate:void 0},Ds=function(r){he(e,r);function e(t,n){return r.call(this)||this}return e.prototype.schedule=function(t,n){return this},e}(Be),St={setInterval:function(r,e){for(var t=[],n=2;nr.preventDefault()))).pipe(Cr(()=>window.location.hash.substr(1).split("/").filter(Boolean).map(decodeURIComponent)));var J=(r=>(r.mutant="mutant",r.test="test",r))(J||{});const ka=`:host(:not([theme=dark])){--prism-maintext:#393a34;--prism-background:#f6f8fa;--prism-border:#ddd;--prism-cdata:#998;--prism-comment:var(--prism-cdata);--prism-doctype:var(--prism-cdata);--prism-prolog:var(--prism-cdata);--prism-attr-value:#e3116c;--prism-string:var(--prism-attr-value);--prism-boolean:#36acaa;--prism-entity:var(--prism-boolean);--prism-url:var(--prism-boolean);--prism-constant:var(--prism-boolean);--prism-inserted:var(--prism-boolean);--prism-number:var(--prism-boolean);--prism-property:var(--prism-boolean);--prism-regex:var(--prism-boolean);--prism-symbol:var(--prism-boolean);--prism-variable:var(--prism-boolean);--prism-atrule:#00a4db;--prism-attr-name:var(--prism-atrule);--prism-attr:var(--prism-atrule);--prism-operator:var(--prism-maintext);--prism-punctuation:var(--prism-maintext);--prism-deleted:#9a050f;--prism-function:var(--prism-deleted);--prism-function-variable:#6f42c1;--prism-selector:#00009f;--prism-tag:var(--prism-selector);--prism-keyword:var(--prism-selector)}:host([theme=dark]){--prism-maintext:#d3d0c8;--prism-background:#1d1f21;--prism-border:rgb(var(--mut-gray-200));--prism-cdata:#7c7c7c;--prism-comment:var(--prism-cdata);--prism-doctype:var(--prism-cdata);--prism-prolog:var(--prism-cdata);--prism-punctuation:#c5c8c6;--prism-tag:#96cbfe;--prism-property:var(--prism-tag);--prism-keyword:var(--prism-tag);--prism-class-name:#ffffb6;--prism-boolean:#9c9;--prism-constant:var(--prism-boolean);--prism-symbol:#f92672;--prism-deleted:var(--prism-symbol);--prism-number:#ff73fd;--prism-inserted:#a8ff60;--prism-selector:var(--prism-inserted);--prism-attr-name:var(--prism-inserted);--prism-string:var(--prism-inserted);--prism-char:var(--prism-inserted);--prism-builtin:var(--prism-inserted);--prism-variable:#c6c5fe;--prism-operator:#ededed;--prism-entity:#ffffb6;--prism-url:#96cbfe;--prism-attr-value:#f9ee98;--prism-atrule:var(--prism-attr-value);--prism-function:#dad085;--prism-regex:#e9c062;--prism-important:#fd971f}:host(:not([theme=dark])){--mut-file-ts-color:#498ba7;--mut-file-ts-test-color:#b7b73b;--mut-file-scala-color:#b8383d;--mut-file-java-color:#b8383d;--mut-file-js-color:#b7b73b;--mut-file-js-test-color:#cc6d2e;--mut-file-php-color:#9068b0;--mut-file-html-color:#498ba7;--mut-file-csharp-color:#498ba7;--mut-file-vue-color:#7fae42;--mut-file-gherkin-color:#00a818}:host([theme=dark]){--mut-file-ts-color:#519aba;--mut-file-ts-test-color:#cbcb41;--mut-file-scala-color:#cc3e44;--mut-file-java-color:#cc3e44;--mut-file-js-color:#cbcb41;--mut-file-js-test-color:#e37933;--mut-file-php-color:#a074c4;--mut-file-html-color:#519aba;--mut-file-csharp-color:#519aba;--mut-file-vue-color:#8dc149;--mut-file-gherkin-color:#10b828}:host(:not([theme=dark])){--mut-gray-bg:rgb(var(--mut-white,255 255 255)/1);--mut-octicon-icon-color:#498ba7;--mut-line-number:#6e7781;--mut-diff-add-bg:#e6ffec;--mut-diff-add-bg-line-number:#ccffd8;--mut-diff-add-line-number:#24292f;--mut-diff-del-bg:#ffebe9;--mut-diff-del-bg-line-number:#ffd7d5;--mut-diff-del-line-number:var(--mut-diff-add-line-number);--mut-badge-info-bg:#54c6ec;--mut-badge-info:#212529;--mut-code-lense:#919191;--mut-squiggly-Survived:url("data:image/svg+xml;charset=UTF8,");--mut-squiggly-NoCoverage:url("data:image/svg+xml;charset=UTF8,");--mut-body-bg:#fff}:host([theme=dark]){--mut-gray-50:24 24 27;--mut-gray-100:39 39 42;--mut-gray-200:63 63 70;--mut-gray-300:82 82 91;--mut-gray-400:113 113 122;--mut-gray-500:161 161 170;--mut-gray-600:212 212 216;--mut-gray-700:228 228 231;--mut-gray-800:244 244 245;--mut-gray-900:250 250 250;--mut-primary-100:7 89 133;--mut-primary-800:224 242 254;--mut-primary-900:240 249 255;--mut-primary-on:14 165 233;--mut-body-bg:#18181b;--mut-white:var(--mut-gray-50);--mut-octicon-icon-color:#519aba;--mut-line-number:#484f58;--mut-diff-add-bg:rgba(46,160,67,.15);--mut-diff-add-bg-line-number:rgba(63,185,80,.3);--mut-diff-add-line-number:#c9d1d9;--mut-diff-del-bg:rgba(248,81,73,.15);--mut-diff-del-bg-line-number:rgba(248,81,73,.3);--mut-diff-del-line-number:#c9d1d9;--mut-badge-info-bg:#17a3b8;--mut-badge-info:#fff;--mut-code-lense:#999;--mut-squiggly-Survived:url("data:image/svg+xml;charset=UTF8,");--mut-squiggly-NoCoverage:url("data:image/svg+xml;charset=UTF8,");color-scheme:dark}`;function te(r,e,t){return new CustomEvent(r,{detail:e,...t})}const Ct=(r,e)=>v`${e} `,ve=(r,e)=>v`${r}
`,ci=r=>v`${r}`,M=(r,e)=>v`${r}`,_a=":host([mode=closed]){height:0}:host([mode=half]){height:120px}:host([mode=open]){height:50%}:host([mode=open]) .scrollable{height:100%;overflow-x:hidden;overflow-y:auto}";var ui=Object.defineProperty,Aa=Object.getOwnPropertyDescriptor,Sa=(r,e,t)=>e in r?ui(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,zt=(r,e,t,n)=>{for(var i=n>1?void 0:n?Aa(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&ui(e,t,i),i},Ca=(r,e,t)=>(Sa(r,typeof e!="symbol"?e+"":e,t),t);const Ea=120;let Ie=class extends W{constructor(){super();x(this,"toggleReadMore",e=>{this.mode==="open"?this.mode="half":this.mode="open",e.preventDefault(),e.stopImmediatePropagation()});this.mode="closed",this.hasDetail=!1}get toggleMoreLabel(){switch(this.mode){case"half":return v`${M("🔼","up arrow")} More`;case"open":return v`${M("🔽","down arrow")} Less`;case"closed":return A}}render(){return v``}};Ca(Ie,"styles",[K(_a),U]);zt([S({reflect:!0})],Ie.prototype,"mode",2);zt([S({reflect:!0,type:Boolean})],Ie.prototype,"hasDetail",2);zt([S()],Ie.prototype,"toggleMoreLabel",1);Ie=zt([D("mte-drawer")],Ie);function rt(r,e){return r?typeof e=="function"?e():e:A}function Ye(r,e){return r==null?A:e(r)}function Ma(r){switch(r){case"Killed":return"success";case"NoCoverage":return"caution";case"Survived":return"danger";case"Timeout":return"warning";case"Ignored":case"RuntimeError":case"Pending":case"CompileError":return"secondary"}}function Ta(r){switch(r){case j.Killing:return"success";case j.Covering:return"warning";case j.NotCovering:return"caution"}}function pr(r){switch(r){case j.Killing:return M("✅",r);case j.Covering:return M("☂",r);case j.NotCovering:return M("🌧",r)}}function di(r){switch(r){case"Killed":return M("✅",r);case"NoCoverage":return M("🙈",r);case"Ignored":return M("🤥",r);case"Survived":return M("👽",r);case"Timeout":return M("⏰",r);case"Pending":return M("⌛",r);case"RuntimeError":case"CompileError":return M("💥",r)}}function tr(r){return r.replace(/&/g,"&").replace(//g,">").replace(/"/g,""").replace(/'/g,"'")}function Et(...r){const e=new URL(window.location.href);return new URL(`#${r.filter(Boolean).join("/")}`,e).href}function pi(r){return r.length>1?"s":""}function hi({fileName:r,location:e}){return r?`${r}${e?`:${e.start.line}:${e.start.column}`:""}`:""}function fi(r){r&&!Pa(r)&&r.scrollIntoView({block:"center",behavior:"smooth"})}function Pa(r){const{top:e,bottom:t}=r.getBoundingClientRect();return e>=0&&t<=(window.innerHeight||document.documentElement.clientHeight)-Ea}function pn(){const r="test";try{return localStorage.setItem(r,r),localStorage.removeItem(r),!0}catch{return!1}}const gi=new qn;var lt;class ae extends W{constructor(){super(...arguments);ge(this,lt,new Be)}shouldReactivate(){return!0}reactivate(){this.requestUpdate()}connectedCallback(){super.connectedCallback(),I(this,lt).add(gi.subscribe(()=>this.shouldReactivate()&&this.reactivate()))}disconnectedCallback(){super.disconnectedCallback(),I(this,lt).unsubscribe()}}lt=new WeakMap;var mi=Object.defineProperty,Fa=Object.getOwnPropertyDescriptor,za=(r,e,t)=>e in r?mi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,q=(r,e,t,n)=>{for(var i=n>1?void 0:n?Fa(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&mi(e,t,i),i},Oa=(r,e,t)=>(za(r,typeof e!="symbol"?e+"":e,t),t);const Ra=100;exports.MutationTestReportAppComponent=class extends ae{constructor(){super();x(this,"mutants",new Map);x(this,"tests",new Map);x(this,"themeSwitch",t=>{this.theme=t.detail,pn()&&localStorage.setItem("mutation-testing-elements-theme",this.theme)});x(this,"subscriptions",[]);x(this,"source");x(this,"sseSubscriptions",new Set);x(this,"theMutant");x(this,"theTest");this.context={view:J.mutant,path:[]},this.path=[]}get themeBackgroundColor(){return getComputedStyle(this).getPropertyValue("--mut-body-bg")}get title(){return this.context.result?this.titlePostfix?`${this.context.result.name} - ${this.titlePostfix}`:this.context.result.name:""}firstUpdated(){(this.path.length===0||this.path[0]!==J.mutant&&this.path[0]!==J.test)&&window.location.replace(Et(`${J.mutant}`))}async loadData(){if(this.src)try{const t=await fetch(this.src);this.report=await t.json()}catch(t){const n=String(t);this.errorMessage=n}}async willUpdate(t){this.report&&(this.theme||(this.theme=this.getTheme()),t.has("report")&&this.updateModel(this.report),(t.has("path")||t.has("report"))&&(this.updateContext(),this.updateTitle())),t.has("src")&&await this.loadData()}updated(t){t.has("theme")&&this.theme&&this.dispatchEvent(te("theme-changed",{theme:this.theme,themeBackgroundColor:this.themeBackgroundColor}))}getTheme(){var n;const t=pn()&&localStorage.getItem("mutation-testing-elements-theme");return t||(window.matchMedia&&((n=window.matchMedia("(prefers-color-scheme: dark)"))!=null&&n.matches)?"dark":"light")}updateModel(t){var i,s,a;this.rootModel=vs(t),n((o,l)=>{o.result=l,o.mutants.forEach(c=>this.mutants.set(c.id,c))})((i=this.rootModel)==null?void 0:i.systemUnderTestMetrics),n((o,l)=>{o.result=l,o.tests.forEach(c=>this.tests.set(c.id,c))})((s=this.rootModel)==null?void 0:s.testMetrics),this.rootModel.systemUnderTestMetrics.updateParent(),(a=this.rootModel.testMetrics)==null||a.updateParent();function n(o){return function l(c){c!=null&&c.file&&o(c.file,c),c==null||c.childResults.forEach(g=>{l(g)})}}}updateContext(){if(this.rootModel){const t=(i,s)=>s.reduce((a,o)=>a==null?void 0:a.childResults.find(l=>l.name===o),i),n=this.path.slice(1);this.path[0]===J.test&&this.rootModel.testMetrics?this.context={view:J.test,path:n,result:t(this.rootModel.testMetrics,this.path.slice(1))}:this.context={view:J.mutant,path:n,result:t(this.rootModel.systemUnderTestMetrics,this.path.slice(1))}}}updateTitle(){document.title=this.title}connectedCallback(){super.connectedCallback(),this.subscriptions.push($a.subscribe(t=>this.path=t)),this.initializeSse()}initializeSse(){if(!this.sse)return;this.source=new EventSource(this.sse);const t=tt(this.source,"mutant-tested").subscribe(i=>{const s=JSON.parse(i.data);if(!this.report)return;const a=this.mutants.get(s.id);if(a!==void 0){this.theMutant=a;for(const[o,l]of Object.entries(s))this.theMutant[o]=l;s.killedBy&&s.killedBy.forEach(o=>{const l=this.tests.get(o);l!==void 0&&(this.theTest=l,l.addKilled(this.theMutant),this.theMutant.addKilledBy(l))}),s.coveredBy&&s.coveredBy.forEach(o=>{const l=this.tests.get(o);l!==void 0&&(this.theTest=l,l.addCovered(this.theMutant),this.theMutant.addCoveredBy(l))})}}),n=tt(this.source,"mutant-tested").pipe(wa(Ra)).subscribe(()=>{this.applyChanges()});this.sseSubscriptions.add(t),this.sseSubscriptions.add(n),this.source.addEventListener("finished",()=>{var i;(i=this.source)==null||i.close(),this.applyChanges(),this.sseSubscriptions.forEach(s=>s.unsubscribe())})}applyChanges(){var t,n;(t=this.theMutant)==null||t.update(),(n=this.theTest)==null||n.update(),gi.next()}disconnectedCallback(){super.disconnectedCallback(),this.subscriptions.forEach(t=>t.unsubscribe())}renderTitle(){return this.context.result?v`
${this.context.result.name}${this.titlePostfix?v`${this.titlePostfix}`:A}
`:A}render(){var t,n,i,s,a;return this.context.result??this.errorMessage?v`
${this.renderErrorMessage()}
${this.renderTitle()} ${this.renderTabs()}
${this.context.view==="mutant"&&this.context.result?v` `:A}
${this.context.view==="test"&&this.context.result?v` `:A}
`:v``}renderErrorMessage(){return this.errorMessage?v`${this.errorMessage}`:A}renderTabs(){var t;if((t=this.rootModel)!=null&&t.testMetrics){const n=this.context.view==="mutant",i=this.context.view==="test";return v`
`}else return A}};Oa(exports.MutationTestReportAppComponent,"styles",[_s,K(ka),U]);q([S({attribute:!1})],exports.MutationTestReportAppComponent.prototype,"report",2);q([S({attribute:!1})],exports.MutationTestReportAppComponent.prototype,"rootModel",2);q([S()],exports.MutationTestReportAppComponent.prototype,"src",2);q([S()],exports.MutationTestReportAppComponent.prototype,"sse",2);q([S({attribute:!1})],exports.MutationTestReportAppComponent.prototype,"errorMessage",2);q([S({attribute:!1})],exports.MutationTestReportAppComponent.prototype,"context",2);q([S()],exports.MutationTestReportAppComponent.prototype,"path",2);q([S({attribute:"title-postfix"})],exports.MutationTestReportAppComponent.prototype,"titlePostfix",2);q([S({reflect:!0})],exports.MutationTestReportAppComponent.prototype,"theme",2);q([S({attribute:!1})],exports.MutationTestReportAppComponent.prototype,"themeBackgroundColor",1);q([S()],exports.MutationTestReportAppComponent.prototype,"title",1);exports.MutationTestReportAppComponent=q([D("mutation-test-report-app")],exports.MutationTestReportAppComponent);/**
* @license
* Copyright 2020 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const{D:ja}=rs,Ia=r=>r.strings===void 0,hn=()=>document.createComment(""),Ve=(r,e,t)=>{var s;const n=r._$AA.parentNode,i=e===void 0?r._$AB:e._$AA;if(t===void 0){const a=n.insertBefore(hn(),i),o=n.insertBefore(hn(),i);t=new ja(a,o,r,r.options)}else{const a=t._$AB.nextSibling,o=t._$AM,l=o!==r;if(l){let c;(s=t._$AQ)==null||s.call(t,r),t._$AM=r,t._$AP!==void 0&&(c=r._$AU)!==o._$AU&&t._$AP(c)}if(a!==i||l){let c=t._$AA;for(;c!==a;){const g=c.nextSibling;n.insertBefore(c,i),c=g}}}return t},be=(r,e,t=r)=>(r._$AI(e,t),r),Da={},Ba=(r,e=Da)=>r._$AH=e,Na=r=>r._$AH,rr=r=>{var n;(n=r._$AP)==null||n.call(r,!1,!0);let e=r._$AA;const t=r._$AB.nextSibling;for(;e!==t;){const i=e.nextSibling;e.remove(),e=i}};/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const Ot={ATTRIBUTE:1,CHILD:2,PROPERTY:3,BOOLEAN_ATTRIBUTE:4,EVENT:5,ELEMENT:6},Rt=r=>(...e)=>({_$litDirective$:r,values:e});class jt{constructor(e){}get _$AU(){return this._$AM._$AU}_$AT(e,t,n){this._$Ct=e,this._$AM=t,this._$Ci=n}_$AS(e,t){return this.update(e,t)}update(e,t){return this.render(...t)}}/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const Je=(r,e)=>{var n;const t=r._$AN;if(t===void 0)return!1;for(const i of t)(n=i._$AO)==null||n.call(i,e,!1),Je(i,e);return!0},Mt=r=>{let e,t;do{if((e=r._$AM)===void 0)break;t=e._$AN,t.delete(r),r=e}while((t==null?void 0:t.size)===0)},bi=r=>{for(let e;e=r._$AM;r=e){let t=e._$AN;if(t===void 0)e._$AN=t=new Set;else if(t.has(r))break;t.add(r),Ha(e)}};function La(r){this._$AN!==void 0?(Mt(this),this._$AM=r,bi(this)):this._$AM=r}function Ua(r,e=!1,t=0){const n=this._$AH,i=this._$AN;if(i!==void 0&&i.size!==0)if(e)if(Array.isArray(n))for(let s=t;s{r.type==Ot.CHILD&&(r._$AP??(r._$AP=Ua),r._$AQ??(r._$AQ=La))};class Ka extends jt{constructor(){super(...arguments),this._$AN=void 0}_$AT(e,t,n){super._$AT(e,t,n),bi(this),this.isConnected=e._$AU}_$AO(e,t=!0){var n,i;e!==this.isConnected&&(this.isConnected=e,e?(n=this.reconnected)==null||n.call(this):(i=this.disconnected)==null||i.call(this)),t&&(Je(this,e),Mt(this))}setValue(e){if(Ia(this._$Ct))this._$Ct._$AI(e,this);else{const t=[...this._$Ct._$AH];t[this._$Ci]=e,this._$Ct._$AI(t,this,0)}}disconnected(){}reconnected(){}}/**
* @license
* Copyright 2020 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const qa=()=>new Va;class Va{}const nr=new WeakMap,Wa=Rt(class extends Ka{render(r){return A}update(r,[e]){var n;const t=e!==this.G;return t&&this.G!==void 0&&this.ot(void 0),(t||this.rt!==this.lt)&&(this.G=e,this.ct=(n=r.options)==null?void 0:n.host,this.ot(this.lt=r.element)),A}ot(r){if(typeof this.G=="function"){const e=this.ct??globalThis;let t=nr.get(e);t===void 0&&(t=new WeakMap,nr.set(e,t)),t.get(this.G)!==void 0&&this.G.call(this.ct,void 0),t.set(this.G,r),r!==void 0&&this.G.call(this.ct,r)}else this.G.value=r}get rt(){var r,e;return typeof this.G=="function"?(r=nr.get(this.ct??globalThis))==null?void 0:r.get(this.G):(e=this.G)==null?void 0:e.value}disconnected(){this.rt===this.lt&&this.ot(void 0)}reconnected(){this.ot(this.lt)}});var V=(r=>(r.csharp="cs",r.java="java",r.javascript="javascript",r.html="html",r.php="php",r.scala="scala",r.typescript="typescript",r.vue="vue",r.gherkin="gherkin",r.svelte="svelte",r))(V||{});function Za(r){return r.substr(r.lastIndexOf(".")+1).toLocaleLowerCase()}function Mr(r){switch(Za(r)){case"cs":return"cs";case"html":return"html";case"java":return"java";case"js":case"cjs":case"mjs":return"javascript";case"ts":case"tsx":case"cts":case"mts":return"typescript";case"scala":return"scala";case"php":return"php";case"vue":return"vue";case"feature":return"gherkin";case"svelte":return"svelte";default:return}}function hr(r,e){const t=Mr(e)??"plain";let n=t;return t==="vue"&&(n="html"),sn.highlight(r,sn.languages[n],n)}function fr(r,e){let t=[];const n=[],i={column:0,line:1,offset:-1},s=[];let a=!1,o=0;for(;ol(d(u)))}function g(){s.forEach(u=>l(d({...u,isClosing:!0})))}function d({attributes:u,elementName:f,isClosing:w}){return w?`${f}>`:`<${f}${Object.entries(u??{}).reduce((C,[_,P])=>P===void 0?`${C} ${_}`:`${C} ${_}="${P}"`,"")}>`}function m(){g(),n.push(t.join("")),t=[]}function y(u){if(i.column++,i.offset++,e)for(const f of e(i))f.isClosing?F(f):(l(d(f)),s.push(f));l(u)}function $(){o++;const u=r[o]==="/"?!0:void 0;u&&o++;const f=o;for(;!wt(r[o])&&r[o]!==">";)o++;const w=r.substring(f,o),C=z();return{elementName:w,attributes:C,isClosing:u}}function k(u){s.push(u),l(d(u))}function F(u){let f;for(f=s.length-1;f>=0;f--){const w=s[f];if(u.elementName===w.elementName&&w.id===u.id){l(d(u)),s.splice(f,1);for(let C=f;C")return u;if(!wt(f)){const{name:w,value:C}=h();u[w]=C}o++}throw new Error(`Missing closing tag near ${r.substr(o-10)}`)}function h(){const u=o;for(;r[o]!=="=";)o++;const f=r.substring(u,o);o++;const w=p();return{name:f,value:w}}function p(){r[o]==='"'&&o++;const u=o;for(;r[o]!=='"';)o++;return r.substring(u,o)}function b(){const u=o;for(;r[o]!==";";)o++;return r.substring(u,o+1)}}function wt(r){return r===`
`||r===" "||r===" "}function Ga(r,e){let t=0,n=e.length-1;for(;r[t]===e[t]&&tt;)n--;n===t&&(wt(e[t-1])||t--),n++;const s=e.substring(t,n);return["true","false"].forEach(a=>{s===a.substr(0,a.length-1)&&a.endsWith(e[n])&&n++,s===a.substr(1,a.length)&&a.startsWith(e[t-1])&&t--}),[t,n]}function xt(r,e){return r.line>e.line||r.line===e.line&&r.column>=e.column}const Ya='#report-code-block{background:var(--prism-background);border:1px solid var(--prism-border);overflow-x:auto;overflow-y:visible}.line-numbers{counter-reset:mte-line-number}.line .line-number{color:var(--mut-line-number);counter-increment:mte-line-number;padding:0 10px 0 15px;text-align:right}.line .line-number:before{content:counter(mte-line-number)}.line-marker:before{content:" ";padding:0 5px}.mte-selected-Pending .mutant.Pending{border-bottom:2px solid;border-color:#a3a3a3;cursor:pointer}svg.mutant-dot.Pending{fill:#a3a3a3}.mte-selected-Killed .mutant.Killed{border-bottom:2px solid;border-color:#16a34a;cursor:pointer}svg.mutant-dot.Killed{fill:#16a34a}svg.mutant-dot.NoCoverage{fill:#f97316}svg.mutant-dot.Survived{fill:#ef4444}.mte-selected-Timeout .mutant.Timeout{border-bottom:2px solid;border-color:#fbbf24;cursor:pointer}svg.mutant-dot.Timeout{fill:#fbbf24}.mte-selected-CompileError .mutant.CompileError{border-bottom:2px solid;border-color:#a3a3a3;cursor:pointer}svg.mutant-dot.CompileError{fill:#a3a3a3}.mte-selected-RuntimeError .mutant.RuntimeError{border-bottom:2px solid;border-color:#a3a3a3;cursor:pointer}svg.mutant-dot.RuntimeError{fill:#a3a3a3}.mte-selected-Ignored .mutant.Ignored{border-bottom:2px solid;border-color:#a3a3a3;cursor:pointer}svg.mutant-dot.Ignored{fill:#a3a3a3}svg.mutant-dot.selected{fill:#38bdf8}.mte-selected-Survived .mutant.Survived{border-bottom-style:solid;border-image-outset:6px;border-image-repeat:repeat;border-image-slice:0 0 4 0;border-image-source:var(--mut-squiggly-Survived);border-image-width:4px;cursor:pointer}.mte-selected-Survived .mutant.Survived .mutant.NoCoverage,.mte-selected-Survived .mutant.Survived .mutant.Survived{border-bottom-style:none;border-image:none}.mte-selected-NoCoverage .mutant.NoCoverage{border-bottom-style:solid;border-image-outset:6px;border-image-repeat:repeat;border-image-slice:0 0 4 0;border-image-source:var(--mut-squiggly-NoCoverage);border-image-width:4px;cursor:pointer}.mte-selected-NoCoverage .mutant.NoCoverage .mutant.NoCoverage,.mte-selected-NoCoverage .mutant.NoCoverage .mutant.Survived{border-bottom-style:none;border-image:none}.mutant-dot{cursor:pointer}svg.mutant-dot{margin:2px}.diff-old{background-color:var(--mut-diff-del-bg)}.diff-focus{background-color:var(--mut-diff-add-bg-line-number)}.diff-old .line-number{background-color:var(--mut-diff-del-bg-line-number);color:var(--mut-diff-del-line-number)}.diff-old .line-marker:before{content:"-"}.diff-new{background-color:var(--mut-diff-add-bg)}.diff-new .empty-line-number{background-color:var(--mut-diff-add-bg-line-number);color:var(--mut-diff-add-line-number)}.diff-new .line-marker:before{content:"+"}';/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/let gr=class extends jt{constructor(e){if(super(e),this.et=A,e.type!==Ot.CHILD)throw Error(this.constructor.directiveName+"() can only be used in child bindings")}render(e){if(e===A||e==null)return this.vt=void 0,this.et=e;if(e===re)return e;if(typeof e!="string")throw Error(this.constructor.directiveName+"() called with a non-string value");if(e===this.et)return this.vt;this.et=e;const t=[e];return t.raw=t,this.vt={_$litType$:this.constructor.resultType,strings:t,values:[]}}};gr.directiveName="unsafeHTML",gr.resultType=1;const Ja=Rt(gr);function vi(r,e){return r===A&&e===A?A:v`${r}${e}`}function yi(r,e){return v`${Ja(r)}${e} `}var wi=Object.defineProperty,Qa=Object.getOwnPropertyDescriptor,Xa=(r,e,t)=>e in r?wi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Te=(r,e,t,n)=>{for(var i=n>1?void 0:n?Qa(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&wi(e,t,i),i},eo=(r,e,t)=>(Xa(r,typeof e!="symbol"?e+"":e,t),t);const ir="diff-old",fn="diff-new";let ne=class extends ae{constructor(){super();x(this,"codeRef",qa());x(this,"filtersChanged",e=>{this.selectedMutantStates=e.detail.concat(["Pending"])});x(this,"codeClicked",e=>{if(e.stopPropagation(),e.target instanceof Element){let t=e.target;const n=[];for(;t instanceof Element;t=t.parentElement){const s=t.getAttribute("mutant-id"),a=this.mutants.find(({id:o})=>o.toString()===s);a&&n.push(a)}const i=(this.selectedMutant?n.indexOf(this.selectedMutant):-1)+1;n[i]?(this.toggleMutant(n[i]),mn()):this.selectedMutant&&(this.toggleMutant(this.selectedMutant),mn())}});x(this,"nextMutant",()=>{const e=this.selectedMutant?(this.mutants.indexOf(this.selectedMutant)+1)%this.mutants.length:0;this.mutants[e]&&this.toggleMutant(this.mutants[e])});x(this,"previousMutant",()=>{const e=this.selectedMutant?(this.mutants.indexOf(this.selectedMutant)+this.mutants.length-1)%this.mutants.length:this.mutants.length-1;this.mutants[e]&&this.toggleMutant(this.mutants[e])});this.filters=[],this.selectedMutantStates=[],this.lines=[],this.mutants=[]}render(){const e=new Map;for(const n of this.mutants){let i=e.get(n.location.start.line);i||(i=[],e.set(n.location.start.line,i)),i.push(n)}const t=n=>this.renderMutantDots([...e.entries()].filter(([i])=>i>n).flatMap(([,i])=>i));return v`
${this.lines.map((n,i)=>{const s=i+1,a=this.renderMutantDots(e.get(s)),o=this.lines.length===s?t(s):A;return yi(n,vi(a,o))})}
`}renderMutantDots(e){return e!=null&&e.length?e.map(t=>{var n;return N``}):A}toggleMutant(e){if(this.removeCurrentDiff(),this.selectedMutant===e){this.selectedMutant=void 0,this.dispatchEvent(te("mutant-selected",{selected:!1,mutant:e}));return}this.selectedMutant=e;const t=this.codeRef.value.querySelectorAll("tr.line");for(let s=e.location.start.line-1;sn.classList.remove(ir)),this.codeRef.value.querySelectorAll(`.${fn}`).forEach(n=>n.remove())}reactivate(){super.reactivate(),this.updateFileRepresentation()}update(e){e.has("model")&&this.model&&this.updateFileRepresentation(),(e.has("model")&&this.model||e.has("selectedMutantStates"))&&(this.mutants=this.model.mutants.filter(t=>this.selectedMutantStates.includes(t.status)).sort((t,n)=>xt(t.location.start,n.location.start)?1:-1),this.selectedMutant&&!this.mutants.includes(this.selectedMutant)&&e.has("selectedMutantStates")&&this.selectedMutantsHaveChanged(e.get("selectedMutantStates")??[])&&this.toggleMutant(this.selectedMutant)),super.update(e)}updateFileRepresentation(){this.filters=["Killed","Survived","NoCoverage","Ignored","Timeout","CompileError","RuntimeError"].filter(i=>this.model.mutants.some(s=>s.status===i)).map(i=>({enabled:[...this.selectedMutantStates,"Survived","NoCoverage","Timeout"].includes(i),count:this.model.mutants.filter(s=>s.status===i).length,status:i,label:v`${di(i)} ${i}`,context:Ma(i)}));const e=hr(this.model.source,this.model.name),t=new Set,n=new Set(this.model.mutants);this.lines=fr(e,function*(i){for(const s of t)xt(i,s.location.end)&&(t.delete(s),yield{elementName:"span",id:s.id,isClosing:!0});for(const s of n)xt(i,s.location.start)&&(t.add(s),n.delete(s),yield{elementName:"span",id:s.id,attributes:{class:tr(`mutant border-none ${s.status}`),title:tr(gn(s)),"mutant-id":tr(s.id.toString())}})})}selectedMutantsHaveChanged(e){return e.length!==this.selectedMutantStates.length?!0:!e.every((t,n)=>this.selectedMutantStates[n]===t)}highlightedReplacementRows(e){const t=e.getMutatedLines().trimEnd(),n=e.getOriginalLines().trimEnd(),[i,s]=Ga(n,t),a=fr(hr(t,this.model.name),function*({offset:c}){c===i?yield{elementName:"span",id:"diff-focus",attributes:{class:"diff-focus"}}:c===s&&(yield{elementName:"span",id:"diff-focus",isClosing:!0})}),o=``,l=" ";return a.map(c=>`${o}${c}${l}`).join("")}};eo(ne,"styles",[Dn,U,K(Ya)]);Te([Z()],ne.prototype,"filters",2);Te([S()],ne.prototype,"model",2);Te([Z()],ne.prototype,"selectedMutantStates",2);Te([Z()],ne.prototype,"selectedMutant",2);Te([Z()],ne.prototype,"lines",2);Te([Z()],ne.prototype,"mutants",2);ne=Te([D("mte-file")],ne);function gn(r){return`${r.mutatorName} ${r.status}`}function mn(){var r;(r=window.getSelection())==null||r.removeAllRanges()}/**
* @license
* Copyright 2017 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const bn=(r,e,t)=>{const n=new Map;for(let i=e;i<=t;i++)n.set(r[i],i);return n},It=Rt(class extends jt{constructor(r){if(super(r),r.type!==Ot.CHILD)throw Error("repeat() can only be used in text expressions")}ht(r,e,t){let n;t===void 0?t=e:e!==void 0&&(n=e);const i=[],s=[];let a=0;for(const o of r)i[a]=n?n(o,a):a,s[a]=t(o,a),a++;return{values:s,keys:i}}render(r,e,t){return this.ht(r,e,t).values}update(r,[e,t,n]){const i=Na(r),{values:s,keys:a}=this.ht(e,t,n);if(!Array.isArray(i))return this.dt=a,s;const o=this.dt??(this.dt=[]),l=[];let c,g,d=0,m=i.length-1,y=0,$=s.length-1;for(;d<=m&&y<=$;)if(i[d]===null)d++;else if(i[m]===null)m--;else if(o[d]===a[y])l[y]=be(i[d],s[y]),d++,y++;else if(o[m]===a[$])l[$]=be(i[m],s[$]),m--,$--;else if(o[d]===a[$])l[$]=be(i[d],s[$]),Ve(r,l[$+1],i[d]),d++,$--;else if(o[m]===a[y])l[y]=be(i[m],s[y]),Ve(r,i[d],i[m]),m--,y++;else if(c===void 0&&(c=bn(a,y,$),g=bn(o,d,m)),c.has(o[d]))if(c.has(o[m])){const k=g.get(a[y]),F=k!==void 0?i[k]:null;if(F===null){const z=Ve(r,i[d]);be(z,s[y]),l[y]=z}else l[y]=be(F,s[y]),Ve(r,i[d],F),i[k]=null;y++}else rr(i[m]),m--;else rr(i[d]),d++;for(;y<=$;){const k=Ve(r,l[$+1]);be(k,s[y]),l[y++]=k}for(;d<=m;){const k=i[d++];k!==null&&rr(k)}return this.dt=a,Ba(r,l),re}});var xi=Object.defineProperty,to=Object.getOwnPropertyDescriptor,ro=(r,e,t)=>e in r?xi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Tr=(r,e,t,n)=>{for(var i=n>1?void 0:n?to(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&xi(e,t,i),i},no=(r,e,t)=>(ro(r,typeof e!="symbol"?e+"":e,t),t);let nt=class extends W{get rootName(){switch(this.view){case J.mutant:return"All files";case J.test:return"All tests"}}render(){return v` `}renderBreadcrumbItems(){if(this.path){const r=this.path;return It(r,e=>e,(e,t)=>t===r.length-1?this.renderActiveItem(e):this.renderLink(e,r.slice(0,t+1)))}}renderActiveItem(r){return v`
${r}
`}renderLink(r,e){return v`
${r}
`}};no(nt,"styles",[U]);Tr([S()],nt.prototype,"path",2);Tr([S()],nt.prototype,"view",2);nt=Tr([D("mte-breadcrumb")],nt);const io=".step-button{--tw-bg-opacity:1;--tw-text-opacity:1;align-items:center;background-color:rgb(var(--mut-primary-600,2 132 199)/var(--tw-bg-opacity));border-radius:.375rem;color:rgb(var(--mut-white,255 255 255)/var(--tw-text-opacity));display:inline-flex;margin-right:.5rem;padding:.25rem;text-align:center}.step-button:hover{--tw-bg-opacity:1;background-color:rgb(var(--mut-primary-700,3 105 161)/var(--tw-bg-opacity))}.step-button:focus{--tw-ring-offset-shadow:var(--tw-ring-inset) 0 0 0 var(--tw-ring-offset-width) var(--tw-ring-offset-color);--tw-ring-shadow:var(--tw-ring-inset) 0 0 0 calc(2px + var(--tw-ring-offset-width)) var(--tw-ring-color);--tw-ring-opacity:1;--tw-ring-color:rgb(var(--mut-primary-500,14 165 233)/var(--tw-ring-opacity));box-shadow:var(--tw-ring-offset-shadow),var(--tw-ring-shadow),var(--tw-shadow,0 0 #0000);outline:2px solid transparent;outline-offset:2px}";var $i=Object.defineProperty,so=Object.getOwnPropertyDescriptor,ao=(r,e,t)=>e in r?$i(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,ki=(r,e,t,n)=>{for(var i=n>1?void 0:n?so(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&$i(e,t,i),i},oo=(r,e,t)=>(ao(r,typeof e!="symbol"?e+"":e,t),t);let Tt=class extends ae{constructor(){super(...arguments);x(this,"next",e=>{e.stopPropagation(),this.dispatchEvent(te("next",void 0,{bubbles:!0,composed:!0}))});x(this,"previous",e=>{e.stopPropagation(),this.dispatchEvent(te("previous",void 0,{bubbles:!0,composed:!0}))})}updated(e){e.has("filters")&&this.dispatchFiltersChangedEvent()}checkboxChanged(e,t){e.enabled=t,this.dispatchFiltersChangedEvent()}dispatchFiltersChangedEvent(){this.dispatchEvent(te("filters-changed",this.filters.filter(({enabled:e})=>e).map(({status:e})=>e)))}render(){var e;return v`
${rt((e=this.filters)==null?void 0:e.length,It(this.filters,t=>t.status,t=>v`
this.checkboxChanged(t,n.target.checked)}"
class="h-5 w-5 rounded border-gray-300 bg-gray-100 text-primary-on !ring-offset-gray-200 focus:outline-none focus:ring-2 focus:ring-primary-500"
/>
`))}
`}bgForContext(e){switch(e){case"success":return"bg-green-100 text-green-800";case"warning":return"bg-yellow-100 text-yellow-800";case"danger":return"bg-red-100 text-red-800";case"caution":return"bg-orange-100 text-orange-800";default:return"bg-gray-100 text-gray-800"}}};oo(Tt,"styles",[U,K(io)]);ki([S({type:Array})],Tt.prototype,"filters",2);Tt=ki([D("mte-state-filter")],Tt);const lo='#darkTheme{position:absolute;right:100vw}#darkTheme+label{--i:0;--j:calc(1 - var(--i));grid-gap:.15em .06em;background:hsl(199,98%,calc(var(--j)*48%));border-radius:.75em;color:transparent;cursor:pointer;display:grid;height:1.5em;overflow:hidden;padding:.15em;transition:.3s;-webkit-user-select:none;user-select:none}#darkTheme+label:after,#darkTheme+label:before{content:"";height:1.2em;transition:inherit;width:1.2em}#darkTheme+label:before{--poly:polygon(44.133707561% 12.9616872277%,50% 0%,55.866292439% 12.9616872277%,59.7057141913% 13.7777815142%,63.4387981079% 14.9907340064%,67.0246437402% 16.5872553429%,79.3892626146% 9.5491502813%,76.5165042945% 23.4834957055%,79.1429735546% 26.4004853356%,81.450146298% 29.5760361869%,83.4127446571% 32.9753562598%,97.5528258148% 34.5491502813%,87.0383127723% 44.133707561%,87.4486075533% 48.0374016409%,87.4486075533% 51.9625983591%,87.0383127723% 55.866292439%,97.5528258148% 65.4508497187%,83.4127446571% 67.0246437402%,81.450146298% 70.4239638131%,79.1429735546% 73.5995146644%,76.5165042945% 76.5165042945%,79.3892626146% 90.4508497187%,67.0246437402% 83.4127446571%,63.4387981079% 85.0092659936%,59.7057141913% 86.2222184858%,55.866292439% 87.0383127723%,50% 100%,44.133707561% 87.0383127723%,40.2942858087% 86.2222184858%,36.561201892% 85.0092659936%,32.9753562598% 83.4127446571%,20.6107373854% 90.4508497187%,23.4834957055% 76.5165042945%,20.8570264454% 73.5995146644%,18.5498537021% 70.4239638131%,16.587255343% 67.0246437402%,2.4471741856% 65.4508497188%,12.9616872286% 55.8662924391%,12.5513924487% 51.9625983594%,12.5513924508% 48.0374016414%,12.961687236% 44.1337075622%,2.4471742159% 34.5491502859%,16.587255404% 32.9753562694%,18.5498538164% 29.5760362054%,20.8570266557% 26.4004853707%,23.4834960862% 23.4834957706%,20.6107385856% 9.5491504949%,32.97535832% 16.5872557238%,36.5612054098% 14.9907346728%,40.2942917387% 13.7777826649%);background:#ff0;-webkit-clip-path:var(--poly);clip-path:var(--poly);transform:translate(calc(var(--i)*(100% + .06em))) scale(calc(1 - var(--i)*.8));transform-origin:20% 20%}#darkTheme+label:after{background:radial-gradient(circle at 19% 19%,transparent 41%,#fff 43%);border-radius:50%;grid-column:2;transform:translatey(calc(var(--i)*(-130% - .15em)))}#darkTheme:checked+label{--i:1}.check-box-container{width:2.9em}';var _i=Object.defineProperty,co=Object.getOwnPropertyDescriptor,uo=(r,e,t)=>e in r?_i(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Ai=(r,e,t,n)=>{for(var i=n>1?void 0:n?co(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&_i(e,t,i),i},po=(r,e,t)=>(uo(r,typeof e!="symbol"?e+"":e,t),t);let Pt=class extends W{constructor(){super(...arguments);x(this,"dispatchThemeChangedEvent",t=>{const n=t.target.checked;this.dispatchEvent(te("theme-switch",n?"dark":"light"))})}render(){return v`
t.stopPropagation()}">
`}};po(Pt,"styles",[U,K(lo)]);Ai([S()],Pt.prototype,"theme",2);Pt=Ai([D("mte-theme-switch")],Pt);const Si=({hasDetail:r,mode:e},t)=>v`
${t}
`;var Ci=Object.defineProperty,ho=Object.getOwnPropertyDescriptor,fo=(r,e,t)=>e in r?Ci(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Pr=(r,e,t,n)=>{for(var i=n>1?void 0:n?ho(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Ci(e,t,i),i},go=(r,e,t)=>(fo(r,typeof e!="symbol"?e+"":e,t),t);const vn=r=>`${r.name}${r.sourceFile&&r.location?` (${hi(r)})`:""}`;let it=class extends ae{constructor(){super(),this.mode="closed"}render(){var r,e,t,n;return Si({hasDetail:!!((e=(r=this.mutant)==null?void 0:r.killedByTests)!=null&&e.length||(n=(t=this.mutant)==null?void 0:t.coveredByTests)!=null&&n.length),mode:this.mode},Ye(this.mutant,i=>v`
${di(i.status)} ${i.mutatorName} ${i.status}
(${i.location.start.line}:${i.location.start.column})
${this.renderSummary()}
${this.renderDetail()}
`))}renderSummary(){var r,e,t,n,i,s,a,o;return ci(v`${(e=(r=this.mutant)==null?void 0:r.killedByTests)!=null&&e[0]?ve(v`${M("🎯","killed")} Killed by:
${(t=this.mutant.killedByTests)==null?void 0:t[0].name}${this.mutant.killedByTests.length>1?`(and ${this.mutant.killedByTests.length-1} more)`:""}`):A}
${rt((n=this.mutant)==null?void 0:n.static,ve(v`${M("🗿","static")} Static mutant`))}
${Ye((i=this.mutant)==null?void 0:i.coveredByTests,l=>{var c;return ve(v`${M("☂️","umbrella")} Covered by ${l.length}
test${pi(l)}${((c=this.mutant)==null?void 0:c.status)==="Survived"?" (yet still survived)":""}`)})}
${rt((a=(s=this.mutant)==null?void 0:s.statusReason)==null?void 0:a.trim(),ve(v`${M("🕵️","spy")} ${this.mutant.statusReason}`,`Reason for the ${this.mutant.status} status`))}
${Ye((o=this.mutant)==null?void 0:o.description,l=>ve(v`${M("📖","book")} ${l}`))}`)}renderDetail(){var r,e,t,n;return v`
${(e=(r=this.mutant)==null?void 0:r.killedByTests)==null?void 0:e.map(i=>Ct("This mutant was killed by this test",v`${M("🎯","killed")} ${vn(i)}`))}
${(n=(t=this.mutant)==null?void 0:t.coveredByTests)==null?void 0:n.filter(i=>{var s,a;return!((a=(s=this.mutant)==null?void 0:s.killedByTests)!=null&&a.includes(i))}).map(i=>Ct("This mutant was covered by this test",v`${M("☂️","umbrella")} ${vn(i)}`))}
`}};go(it,"styles",[U]);Pr([S()],it.prototype,"mutant",2);Pr([S({reflect:!0})],it.prototype,"mode",2);it=Pr([D("mte-drawer-mutant")],it);const mo="main{padding-bottom:120px}";var Ei=Object.defineProperty,bo=Object.getOwnPropertyDescriptor,vo=(r,e,t)=>e in r?Ei(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Le=(r,e,t,n)=>{for(var i=n>1?void 0:n?bo(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Ei(e,t,i),i},yo=(r,e,t)=>(vo(r,typeof e!="symbol"?e+"":e,t),t);let pe=class extends ae{constructor(){super();x(this,"handleClick",()=>{this.drawerMode="closed"});x(this,"handleMutantSelected",e=>{this.selectedMutant=e.detail.mutant,this.drawerMode=e.detail.selected?"half":"closed"});this.drawerMode="closed"}updated(e){e.has("result")&&!this.result.file&&(this.drawerMode="closed")}render(){return v`
${this.result.file?v` `:A}
`}};yo(pe,"styles",[K(mo),U]);Le([S()],pe.prototype,"drawerMode",2);Le([S()],pe.prototype,"selectedMutant",2);Le([S()],pe.prototype,"result",2);Le([S({attribute:!1,reflect:!1})],pe.prototype,"thresholds",2);Le([S({attribute:!1,reflect:!1})],pe.prototype,"path",2);pe=Le([D("mte-mutant-view")],pe);const wo=[{key:"mutationScore",label:"Mutation score",tooltip:"The percentage of mutants that were detected. The higher, the better!",category:"percentage"},{key:"killed",label:"Killed",tooltip:"At least one test failed while these mutants were active. This is what you want!",category:"number"},{key:"survived",label:"Survived",tooltip:"All tests passed while these mutants were active. You're missing a test for them.",category:"number"},{key:"timeout",label:"Timeout",tooltip:"Running the tests while these mutants were active resulted in a timeout. For example, an infinite loop.",category:"number"},{key:"noCoverage",label:"No coverage",tooltip:"These mutants aren't covered by one of your tests and survived as a result.",category:"number"},{key:"ignored",label:"Ignored",tooltip:"These mutants weren't tested because they are ignored. Either by user action, or for another reason.",category:"number"},{key:"runtimeErrors",label:"Runtime errors",tooltip:"Running tests when these mutants are active resulted in an error (rather than a failed test). For example: an out of memory error.",category:"number"},{key:"compileErrors",label:"Compile errors",tooltip:"Mutants that caused a compile error.",category:"number"},{key:"totalDetected",label:"Detected",tooltip:"The number of mutants detected by your tests (killed + timeout).",category:"number",width:"large",isBold:!0},{key:"totalUndetected",label:"Undetected",tooltip:"The number of mutants that are not detected by your tests (survived + no coverage).",category:"number",width:"large",isBold:!0},{key:"totalMutants",label:"Total",tooltip:"All mutants (except runtimeErrors + compileErrors)",category:"number",width:"large",isBold:!0}],xo="main{padding-bottom:120px}";var Mi=Object.defineProperty,$o=Object.getOwnPropertyDescriptor,ko=(r,e,t)=>e in r?Mi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,ut=(r,e,t,n)=>{for(var i=n>1?void 0:n?$o(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Mi(e,t,i),i},_o=(r,e,t)=>(ko(r,typeof e!="symbol"?e+"":e,t),t);let Ee=class extends ae{constructor(){super();x(this,"handleClick",()=>{this.drawerMode="closed"});x(this,"handleTestSelected",e=>{this.selectedTest=e.detail.test,this.drawerMode=e.detail.selected?"half":"closed"});this.drawerMode="closed"}updated(e){e.has("result")&&!this.result.file&&(this.drawerMode="closed")}render(){return v`
${this.result.file?v` `:A}
`}};_o(Ee,"styles",[K(xo),U]);ut([S()],Ee.prototype,"drawerMode",2);ut([S()],Ee.prototype,"result",2);ut([S({attribute:!1,reflect:!1})],Ee.prototype,"path",2);ut([S()],Ee.prototype,"selectedTest",2);Ee=ut([D("mte-test-view")],Ee);const Ao=[{key:"killing",label:"Killing",tooltip:"These tests killed at least one mutant",width:"normal",category:"number"},{key:"covering",label:"Covering",tooltip:"These tests are covering at least one mutant, but not killing any of them.",width:"normal",category:"number"},{key:"notCovering",label:"Not Covering",tooltip:"These tests were not covering a mutant (and thus not killing any of them).",width:"normal",category:"number"},{key:"total",label:"Total tests",width:"large",category:"number",isBold:!0}];/**
* @license
* Copyright 2018 Google LLC
* SPDX-License-Identifier: BSD-3-Clause
*/const Ti=Rt(class extends jt{constructor(r){var e;if(super(r),r.type!==Ot.ATTRIBUTE||r.name!=="class"||((e=r.strings)==null?void 0:e.length)>2)throw Error("`classMap()` can only be used in the `class` attribute and must be the only part in the attribute.")}render(r){return" "+Object.keys(r).filter(e=>r[e]).join(" ")+" "}update(r,[e]){var n,i;if(this.it===void 0){this.it=new Set,r.strings!==void 0&&(this.st=new Set(r.strings.join(" ").split(/\s/).filter(s=>s!=="")));for(const s in e)e[s]&&!((n=this.st)!=null&&n.has(s))&&this.it.add(s);return this.render(e)}const t=r.element.classList;for(const s of this.it)s in e||(t.remove(s),this.it.delete(s));for(const s in e){const a=!!e[s];a===this.it.has(s)||(i=this.st)!=null&&i.has(s)||(a?(t.add(s),this.it.add(s)):(t.remove(s),this.it.delete(s)))}return re}});var Pi=Object.defineProperty,So=Object.getOwnPropertyDescriptor,Co=(r,e,t)=>e in r?Pi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,dt=(r,e,t,n)=>{for(var i=n>1?void 0:n?So(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Pi(e,t,i),i},Eo=(r,e,t)=>(Co(r,typeof e!="symbol"?e+"":e,t),t);let Me=class extends ae{constructor(){super();x(this,"hasMultipleColspan",!1);this.currentPath=[],this.thresholds={high:80,low:60}}willUpdate(e){e.has("columns")&&(this.hasMultipleColspan=this.columns.some(t=>t.category==="percentage"))}render(){return v`${this.model?v`
${this.renderTableHeadRow()}${this.renderTableBody(this.model)}
`:A}`}renderTableHeadRow(){return v`
File / Directory${M("ℹ","info icon")}
${It(this.columns,e=>e.key,e=>this.renderTableHead(e))}
`}renderTableHead(e){const t=`tooltip-${e.key.toString()}`,n=e.tooltip?v`${e.label} `:v`${e.label}`;return e.category==="percentage"?v` ${n} `:v`
${n}
`}renderTableBody(e){const t=()=>e.file?A:e.childResults.map(n=>{const i=[n.name];for(;!n.file&&n.childResults.length===1;)n=n.childResults[0],i.push(n.name);return this.renderRow(i.join("/"),n,...this.currentPath,...i)});return v`${this.renderRow(e.name,e)} ${t()}`}renderRow(e,t,...n){return v`
${this.columns.map(i=>this.renderCell(i,t.metrics))}
`}renderCell(e,t){const n=t[e.key],i=this.hasMultipleColspan?"odd:bg-gray-100":"even:bg-gray-100";if(e.category==="percentage"){const s=!isNaN(n),a=this.determineBgColoringClass(n),o=this.determineTextColoringClass(n),l=n.toFixed(2),c=`width: ${n}%`;return v`
${s?v`
`:v` N/A `}
${s?v`${l}`:A} `}return v`${n} `}determineBgColoringClass(e){return!isNaN(e)&&this.thresholds?ee in r?Fi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Pe=(r,e,t,n)=>{for(var i=n>1?void 0:n?To(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Fi(e,t,i),i},Fo=(r,e,t)=>(Po(r,typeof e!="symbol"?e+"":e,t),t);let ie=class extends ae{constructor(){super();x(this,"filtersChanged",e=>{this.enabledStates=e.detail,this.selectedTest&&!this.enabledStates.includes(this.selectedTest.status)&&this.toggleTest(this.selectedTest)});x(this,"nextTest",()=>{const e=this.selectedTest?(this.tests.findIndex(({id:t})=>t===this.selectedTest.id)+1)%this.tests.length:0;this.selectTest(this.tests[e])});x(this,"previousTest",()=>{const e=this.selectedTest?(this.tests.findIndex(({id:t})=>t===this.selectedTest.id)+this.tests.length-1)%this.tests.length:this.tests.length-1;this.selectTest(this.tests[e])});this.filters=[],this.lines=[],this.enabledStates=[],this.tests=[]}toggleTest(e){this.selectedTest===e?(this.selectedTest=void 0,this.dispatchEvent(te("test-selected",{selected:!1,test:e}))):(this.selectedTest=e,this.dispatchEvent(te("test-selected",{selected:!0,test:e})),fi(this.shadowRoot.querySelector(`[test-id="${e.id}"]`)))}selectTest(e){e&&this.toggleTest(e)}render(){return v`
${this.renderTestList()} ${this.renderCode()}
`}renderTestList(){const e=this.tests.filter(t=>!t.location);return e.length?v`
${It(e,t=>t.id,t=>v`-
`)}
`:A}renderCode(){var e;if((e=this.model)!=null&&e.source){const t=new Map;for(const i of this.tests)if(i.location){let s=t.get(i.location.start.line);s||(s=[],t.set(i.location.start.line,s)),s.push(i)}const n=i=>this.renderTestDots([...t.entries()].filter(([s])=>s>i).flatMap(([,s])=>s));return v`
${this.lines.map((i,s)=>{const a=s+1,o=this.renderTestDots(t.get(a)),l=this.lines.length===a?n(a):A;return yi(i,vi(o,l))})}
`}return A}renderTestDots(e){return e!=null&&e.length?e.map(t=>N``):A}reactivate(){super.reactivate(),this.updateFileRepresentation()}willUpdate(e){e.has("model")&&this.updateFileRepresentation(),(e.has("model")||e.has("enabledStates"))&&this.model&&(this.tests=this.model.tests.filter(t=>this.enabledStates.includes(t.status)).sort((t,n)=>t.location&&n.location?xt(t.location.start,n.location.start)?1:-1:this.model.tests.indexOf(t)-this.model.tests.indexOf(n))),super.update(e)}updateFileRepresentation(){if(!this.model)return;const e=this.model;this.filters=[j.Killing,j.Covering,j.NotCovering].filter(t=>e.tests.some(n=>n.status===t)).map(t=>({enabled:!0,count:e.tests.filter(n=>n.status===t).length,status:t,label:v`${pr(t)} ${t}`,context:Ta(t)})),this.model.source&&(this.lines=fr(hr(this.model.source,this.model.name)))}};Fo(ie,"styles",[Dn,U,K(Mo)]);Pe([S()],ie.prototype,"model",2);Pe([Z()],ie.prototype,"filters",2);Pe([Z()],ie.prototype,"lines",2);Pe([Z()],ie.prototype,"enabledStates",2);Pe([Z()],ie.prototype,"selectedTest",2);Pe([Z()],ie.prototype,"tests",2);ie=Pe([D("mte-test-file")],ie);function zo(r){return`${r.name} (${r.status})`}var zi=Object.defineProperty,Oo=Object.getOwnPropertyDescriptor,Ro=(r,e,t)=>e in r?zi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Fr=(r,e,t,n)=>{for(var i=n>1?void 0:n?Oo(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&zi(e,t,i),i},jo=(r,e,t)=>(Ro(r,typeof e!="symbol"?e+"":e,t),t);const sr=r=>v`${r.getMutatedLines()}
(${hi(r)})`;let st=class extends ae{constructor(){super(),this.mode="closed"}render(){var r,e,t,n;return Si({hasDetail:!!((e=(r=this.test)==null?void 0:r.killedMutants)!=null&&e.length||(n=(t=this.test)==null?void 0:t.coveredMutants)!=null&&n.length),mode:this.mode},Ye(this.test,i=>v`
${pr(i.status)} ${i.name} [${i.status}]
${i.location?v`(${i.location.start.line}:${i.location.start.column})`:A}
${this.renderSummary()}
${this.renderDetail()}
`))}renderSummary(){var r,e,t,n;return ci(v`${(e=(r=this.test)==null?void 0:r.killedMutants)!=null&&e[0]?ve(v`${M("🎯","killed")} Killed:
${sr((t=this.test.killedMutants)==null?void 0:t[0])}${this.test.killedMutants.length>1?v` (and ${this.test.killedMutants.length-1} more)`:""}`):A}
${Ye((n=this.test)==null?void 0:n.coveredMutants,i=>{var s;return ve(v`${M("☂️","umbrella")} Covered ${i.length}
mutant${pi(i)}${((s=this.test)==null?void 0:s.status)===j.Covering?" (yet didn't kill any of them)":""}`)})}`)}renderDetail(){var r,e,t,n;return v`
${(e=(r=this.test)==null?void 0:r.killedMutants)==null?void 0:e.map(i=>Ct("This test killed this mutant",v`${M("🎯","killed")} ${sr(i)}`))}
${(n=(t=this.test)==null?void 0:t.coveredMutants)==null?void 0:n.filter(i=>{var s,a;return!((a=(s=this.test)==null?void 0:s.killedMutants)!=null&&a.includes(i))}).map(i=>Ct("This test covered this mutant",v`${M("☂️","umbrella")} ${sr(i)}`))}
`}};jo(st,"styles",[U]);Fr([S()],st.prototype,"test",2);Fr([S({reflect:!0})],st.prototype,"mode",2);st=Fr([D("mte-drawer-test")],st);const Io="svg.cs{fill:var(--mut-file-csharp-color)}svg.html{fill:var(--mut-file-html-color)}svg.java{fill:var(--mut-file-java-color)}svg.javascript{fill:var(--mut-file-js-color)}svg.scala{fill:var(--mut-file-scala-color)}svg.typescript{fill:var(--mut-file-ts-color)}svg.php{fill:var(--mut-file-php-color)}svg.vue{fill:var(--mut-file-vue-color)}svg.octicon{fill:var(--mut-octicon-icon-color)}svg.javascript.test,svg.typescript.test{fill:var(--mut-file-js-test-color)}svg.gherkin{fill:var(--mut-file-gherkin-color)}svg{vertical-align:middle;width:20px}";var Oi=Object.defineProperty,Do=Object.getOwnPropertyDescriptor,Bo=(r,e,t)=>e in r?Oi(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,zr=(r,e,t,n)=>{for(var i=n>1?void 0:n?Do(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Oi(e,t,i),i},No=(r,e,t)=>(Bo(r,typeof e!="symbol"?e+"":e,t),t);let at=class extends W{get language(){return Mr(this.fileName)}get isTestFile(){const r=this.fileName.substr(0,this.fileName.lastIndexOf(".")).toLowerCase();return r.endsWith("spec")||r.endsWith("test")}get cssClass(){var r;return Ti({[((r=this.language)==null?void 0:r.toString())??"unknown"]:this.isFile,test:this.isTestFile})}render(){if(!this.isFile)return N``;if(!this.language)return N``;switch(this.language){case V.csharp:return N``;case V.html:return N``;case V.java:return N``;case V.javascript:return N``;case V.typescript:return N``;case V.scala:return N``;case V.php:return N``;case V.vue:return N``;case V.gherkin:return N``;case V.svelte:return N``}}};No(at,"styles",[K(Io)]);zr([S({attribute:"file-name"})],at.prototype,"fileName",2);zr([S({attribute:"file",type:Boolean})],at.prototype,"isFile",2);at=zr([D("mte-file-icon")],at);var Ri=Object.defineProperty,Lo=Object.getOwnPropertyDescriptor,Uo=(r,e,t)=>e in r?Ri(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,ji=(r,e,t,n)=>{for(var i=n>1?void 0:n?Lo(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Ri(e,t,i),i},Ho=(r,e,t)=>(Uo(r,typeof e!="symbol"?e+"":e,t),t);let Ft=class extends W{render(){return v` `}};Ho(Ft,"styles",[U]);ji([S({attribute:!0})],Ft.prototype,"title",2);Ft=ji([D("mte-tooltip")],Ft);var Ii=Object.defineProperty,Ko=Object.getOwnPropertyDescriptor,qo=(r,e,t)=>e in r?Ii(r,e,{enumerable:!0,configurable:!0,writable:!0,value:t}):r[e]=t,Fe=(r,e,t,n)=>{for(var i=n>1?void 0:n?Ko(e,t):e,s=r.length-1,a;s>=0;s--)(a=r[s])&&(i=(n?a(e,t,i):a(i))||i);return n&&i&&Ii(e,t,i),i},Vo=(r,e,t)=>(qo(r,typeof e!="symbol"?e+"":e,t),t),Or=(r,e,t)=>{if(!e.has(r))throw TypeError("Cannot "+t)},yn=(r,e,t)=>(Or(r,e,"read from private field"),t?t.call(r):e.get(r)),le=(r,e,t)=>{if(e.has(r))throw TypeError("Cannot add the same private member more than once");e instanceof WeakSet?e.add(r):e.set(r,t)},Wo=(r,e,t,n)=>(Or(r,e,"write to private field"),n?n.call(r,t):e.set(r,t),t),X=(r,e,t)=>(Or(r,e,"access private method"),t),We,mr,Di,Rr,Bi,br,Ni,jr,Li,Dt,Ir,Bt,Dr,Nt,Br;let se=class extends W{constructor(){super(),le(this,mr),le(this,Rr),le(this,br),le(this,jr),le(this,Dt),le(this,Bt),le(this,Nt),le(this,We,void 0),this.detected=0,this.noCoverage=0,this.pending=0,this.survived=0,this.total=0,this.shouldBeSmall=!1}connectedCallback(){super.connectedCallback(),Wo(this,We,new window.IntersectionObserver(([r])=>{r.isIntersecting?this.shouldBeSmall=!1:this.shouldBeSmall=!0,this.requestUpdate()})),yn(this,We).observe(this)}disconnectedCallback(){var r;super.disconnectedCallback(),(r=yn(this,We))==null||r.disconnect()}render(){return v`
${X(this,mr,Di).call(this)}
${X(this,br,Ni).call(this)}
`}};We=new WeakMap;mr=new WeakSet;Di=function(){return v`
${X(this,Dt,Ir).call(this).map(r=>X(this,Rr,Bi).call(this,r))}
`};Rr=new WeakSet;Bi=function(r){return v``};br=new WeakSet;Ni=function(){return v`${X(this,Dt,Ir).call(this).map(r=>X(this,jr,Li).call(this,r))}`};jr=new WeakSet;Li=function(r){return v`
${r.amount}
`};Dt=new WeakSet;Ir=function(){return[{type:"detected",amount:this.detected,tooltip:`killed + timeout (${this.detected})`},{type:"survived",amount:this.survived,tooltip:`survived (${this.survived})`},{type:"no coverage",amount:this.noCoverage,tooltip:`no coverage (${this.noCoverage})`},{type:"pending",amount:this.pending,tooltip:"pending"}]};Bt=new WeakSet;Dr=function(r){switch(r){case"detected":return"bg-green-600";case"survived":return"bg-red-600";case"no coverage":return"bg-yellow-600";default:return"bg-gray-200"}};Nt=new WeakSet;Br=function(r){return this.total!==0?100*r/this.total:0};Vo(se,"styles",[U]);Fe([S({attribute:!1})],se.prototype,"detected",2);Fe([S({attribute:!1})],se.prototype,"noCoverage",2);Fe([S({attribute:!1})],se.prototype,"pending",2);Fe([S({attribute:!1})],se.prototype,"survived",2);Fe([S({attribute:!1})],se.prototype,"total",2);Fe([Z()],se.prototype,"shouldBeSmall",2);se=Fe([D("mte-result-status-bar")],se);
© 2015 - 2024 Weber Informatics LLC | Privacy Policy