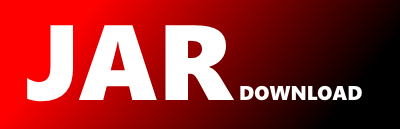
io.substrait.proto.AggregateFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Create a well-defined, cross-language specification for data compute operations
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: substrait/algebra.proto
// Protobuf Java Version: 3.25.5
package io.substrait.proto;
/**
*
* An aggregate function.
*
*
* Protobuf type {@code substrait.AggregateFunction}
*/
public final class AggregateFunction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:substrait.AggregateFunction)
AggregateFunctionOrBuilder {
private static final long serialVersionUID = 0L;
// Use AggregateFunction.newBuilder() to construct.
private AggregateFunction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AggregateFunction() {
arguments_ = java.util.Collections.emptyList();
options_ = java.util.Collections.emptyList();
phase_ = 0;
sorts_ = java.util.Collections.emptyList();
invocation_ = 0;
args_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AggregateFunction();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_AggregateFunction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_AggregateFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.AggregateFunction.class, io.substrait.proto.AggregateFunction.Builder.class);
}
/**
*
* Method in which equivalent records are merged before being aggregated.
*
*
* Protobuf enum {@code substrait.AggregateFunction.AggregationInvocation}
*/
public enum AggregationInvocation
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* This default value implies AGGREGATION_INVOCATION_ALL.
*
*
* AGGREGATION_INVOCATION_UNSPECIFIED = 0;
*/
AGGREGATION_INVOCATION_UNSPECIFIED(0),
/**
*
* Use all values in the aggregation calculation.
*
*
* AGGREGATION_INVOCATION_ALL = 1;
*/
AGGREGATION_INVOCATION_ALL(1),
/**
*
* Use only distinct values in the aggregation calculation.
*
*
* AGGREGATION_INVOCATION_DISTINCT = 2;
*/
AGGREGATION_INVOCATION_DISTINCT(2),
UNRECOGNIZED(-1),
;
/**
*
* This default value implies AGGREGATION_INVOCATION_ALL.
*
*
* AGGREGATION_INVOCATION_UNSPECIFIED = 0;
*/
public static final int AGGREGATION_INVOCATION_UNSPECIFIED_VALUE = 0;
/**
*
* Use all values in the aggregation calculation.
*
*
* AGGREGATION_INVOCATION_ALL = 1;
*/
public static final int AGGREGATION_INVOCATION_ALL_VALUE = 1;
/**
*
* Use only distinct values in the aggregation calculation.
*
*
* AGGREGATION_INVOCATION_DISTINCT = 2;
*/
public static final int AGGREGATION_INVOCATION_DISTINCT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AggregationInvocation valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static AggregationInvocation forNumber(int value) {
switch (value) {
case 0: return AGGREGATION_INVOCATION_UNSPECIFIED;
case 1: return AGGREGATION_INVOCATION_ALL;
case 2: return AGGREGATION_INVOCATION_DISTINCT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
AggregationInvocation> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AggregationInvocation findValueByNumber(int number) {
return AggregationInvocation.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.substrait.proto.AggregateFunction.getDescriptor().getEnumTypes().get(0);
}
private static final AggregationInvocation[] VALUES = values();
public static AggregationInvocation valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private AggregationInvocation(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:substrait.AggregateFunction.AggregationInvocation)
}
private int bitField0_;
public static final int FUNCTION_REFERENCE_FIELD_NUMBER = 1;
private int functionReference_ = 0;
/**
*
* Points to a function_anchor defined in this plan, which must refer
* to an aggregate function in the associated YAML file. Required; 0 is
* considered to be a valid anchor/reference.
*
*
* uint32 function_reference = 1;
* @return The functionReference.
*/
@java.lang.Override
public int getFunctionReference() {
return functionReference_;
}
public static final int ARGUMENTS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List arguments_;
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
@java.lang.Override
public java.util.List getArgumentsList() {
return arguments_;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
@java.lang.Override
public java.util.List extends io.substrait.proto.FunctionArgumentOrBuilder>
getArgumentsOrBuilderList() {
return arguments_;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
@java.lang.Override
public int getArgumentsCount() {
return arguments_.size();
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
@java.lang.Override
public io.substrait.proto.FunctionArgument getArguments(int index) {
return arguments_.get(index);
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
@java.lang.Override
public io.substrait.proto.FunctionArgumentOrBuilder getArgumentsOrBuilder(
int index) {
return arguments_.get(index);
}
public static final int OPTIONS_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private java.util.List options_;
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
@java.lang.Override
public java.util.List getOptionsList() {
return options_;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
@java.lang.Override
public java.util.List extends io.substrait.proto.FunctionOptionOrBuilder>
getOptionsOrBuilderList() {
return options_;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
@java.lang.Override
public int getOptionsCount() {
return options_.size();
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
@java.lang.Override
public io.substrait.proto.FunctionOption getOptions(int index) {
return options_.get(index);
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
@java.lang.Override
public io.substrait.proto.FunctionOptionOrBuilder getOptionsOrBuilder(
int index) {
return options_.get(index);
}
public static final int OUTPUT_TYPE_FIELD_NUMBER = 5;
private io.substrait.proto.Type outputType_;
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
* @return Whether the outputType field is set.
*/
@java.lang.Override
public boolean hasOutputType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
* @return The outputType.
*/
@java.lang.Override
public io.substrait.proto.Type getOutputType() {
return outputType_ == null ? io.substrait.proto.Type.getDefaultInstance() : outputType_;
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
@java.lang.Override
public io.substrait.proto.TypeOrBuilder getOutputTypeOrBuilder() {
return outputType_ == null ? io.substrait.proto.Type.getDefaultInstance() : outputType_;
}
public static final int PHASE_FIELD_NUMBER = 4;
private int phase_ = 0;
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @return The enum numeric value on the wire for phase.
*/
@java.lang.Override public int getPhaseValue() {
return phase_;
}
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @return The phase.
*/
@java.lang.Override public io.substrait.proto.AggregationPhase getPhase() {
io.substrait.proto.AggregationPhase result = io.substrait.proto.AggregationPhase.forNumber(phase_);
return result == null ? io.substrait.proto.AggregationPhase.UNRECOGNIZED : result;
}
public static final int SORTS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List sorts_;
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
@java.lang.Override
public java.util.List getSortsList() {
return sorts_;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
@java.lang.Override
public java.util.List extends io.substrait.proto.SortFieldOrBuilder>
getSortsOrBuilderList() {
return sorts_;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
@java.lang.Override
public int getSortsCount() {
return sorts_.size();
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
@java.lang.Override
public io.substrait.proto.SortField getSorts(int index) {
return sorts_.get(index);
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
@java.lang.Override
public io.substrait.proto.SortFieldOrBuilder getSortsOrBuilder(
int index) {
return sorts_.get(index);
}
public static final int INVOCATION_FIELD_NUMBER = 6;
private int invocation_ = 0;
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @return The enum numeric value on the wire for invocation.
*/
@java.lang.Override public int getInvocationValue() {
return invocation_;
}
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @return The invocation.
*/
@java.lang.Override public io.substrait.proto.AggregateFunction.AggregationInvocation getInvocation() {
io.substrait.proto.AggregateFunction.AggregationInvocation result = io.substrait.proto.AggregateFunction.AggregationInvocation.forNumber(invocation_);
return result == null ? io.substrait.proto.AggregateFunction.AggregationInvocation.UNRECOGNIZED : result;
}
public static final int ARGS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List args_;
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public java.util.List getArgsList() {
return args_;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public java.util.List extends io.substrait.proto.ExpressionOrBuilder>
getArgsOrBuilderList() {
return args_;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public int getArgsCount() {
return args_.size();
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public io.substrait.proto.Expression getArgs(int index) {
return args_.get(index);
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public io.substrait.proto.ExpressionOrBuilder getArgsOrBuilder(
int index) {
return args_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (functionReference_ != 0) {
output.writeUInt32(1, functionReference_);
}
for (int i = 0; i < args_.size(); i++) {
output.writeMessage(2, args_.get(i));
}
for (int i = 0; i < sorts_.size(); i++) {
output.writeMessage(3, sorts_.get(i));
}
if (phase_ != io.substrait.proto.AggregationPhase.AGGREGATION_PHASE_UNSPECIFIED.getNumber()) {
output.writeEnum(4, phase_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(5, getOutputType());
}
if (invocation_ != io.substrait.proto.AggregateFunction.AggregationInvocation.AGGREGATION_INVOCATION_UNSPECIFIED.getNumber()) {
output.writeEnum(6, invocation_);
}
for (int i = 0; i < arguments_.size(); i++) {
output.writeMessage(7, arguments_.get(i));
}
for (int i = 0; i < options_.size(); i++) {
output.writeMessage(8, options_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (functionReference_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, functionReference_);
}
for (int i = 0; i < args_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, args_.get(i));
}
for (int i = 0; i < sorts_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, sorts_.get(i));
}
if (phase_ != io.substrait.proto.AggregationPhase.AGGREGATION_PHASE_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, phase_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getOutputType());
}
if (invocation_ != io.substrait.proto.AggregateFunction.AggregationInvocation.AGGREGATION_INVOCATION_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, invocation_);
}
for (int i = 0; i < arguments_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, arguments_.get(i));
}
for (int i = 0; i < options_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, options_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.substrait.proto.AggregateFunction)) {
return super.equals(obj);
}
io.substrait.proto.AggregateFunction other = (io.substrait.proto.AggregateFunction) obj;
if (getFunctionReference()
!= other.getFunctionReference()) return false;
if (!getArgumentsList()
.equals(other.getArgumentsList())) return false;
if (!getOptionsList()
.equals(other.getOptionsList())) return false;
if (hasOutputType() != other.hasOutputType()) return false;
if (hasOutputType()) {
if (!getOutputType()
.equals(other.getOutputType())) return false;
}
if (phase_ != other.phase_) return false;
if (!getSortsList()
.equals(other.getSortsList())) return false;
if (invocation_ != other.invocation_) return false;
if (!getArgsList()
.equals(other.getArgsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FUNCTION_REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getFunctionReference();
if (getArgumentsCount() > 0) {
hash = (37 * hash) + ARGUMENTS_FIELD_NUMBER;
hash = (53 * hash) + getArgumentsList().hashCode();
}
if (getOptionsCount() > 0) {
hash = (37 * hash) + OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getOptionsList().hashCode();
}
if (hasOutputType()) {
hash = (37 * hash) + OUTPUT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getOutputType().hashCode();
}
hash = (37 * hash) + PHASE_FIELD_NUMBER;
hash = (53 * hash) + phase_;
if (getSortsCount() > 0) {
hash = (37 * hash) + SORTS_FIELD_NUMBER;
hash = (53 * hash) + getSortsList().hashCode();
}
hash = (37 * hash) + INVOCATION_FIELD_NUMBER;
hash = (53 * hash) + invocation_;
if (getArgsCount() > 0) {
hash = (37 * hash) + ARGS_FIELD_NUMBER;
hash = (53 * hash) + getArgsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.substrait.proto.AggregateFunction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.AggregateFunction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.AggregateFunction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.AggregateFunction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.AggregateFunction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.AggregateFunction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.AggregateFunction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.AggregateFunction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.AggregateFunction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.substrait.proto.AggregateFunction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.AggregateFunction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.AggregateFunction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.substrait.proto.AggregateFunction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An aggregate function.
*
*
* Protobuf type {@code substrait.AggregateFunction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:substrait.AggregateFunction)
io.substrait.proto.AggregateFunctionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_AggregateFunction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_AggregateFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.AggregateFunction.class, io.substrait.proto.AggregateFunction.Builder.class);
}
// Construct using io.substrait.proto.AggregateFunction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getArgumentsFieldBuilder();
getOptionsFieldBuilder();
getOutputTypeFieldBuilder();
getSortsFieldBuilder();
getArgsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
functionReference_ = 0;
if (argumentsBuilder_ == null) {
arguments_ = java.util.Collections.emptyList();
} else {
arguments_ = null;
argumentsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (optionsBuilder_ == null) {
options_ = java.util.Collections.emptyList();
} else {
options_ = null;
optionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
outputType_ = null;
if (outputTypeBuilder_ != null) {
outputTypeBuilder_.dispose();
outputTypeBuilder_ = null;
}
phase_ = 0;
if (sortsBuilder_ == null) {
sorts_ = java.util.Collections.emptyList();
} else {
sorts_ = null;
sortsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
invocation_ = 0;
if (argsBuilder_ == null) {
args_ = java.util.Collections.emptyList();
} else {
args_ = null;
argsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.substrait.proto.Algebra.internal_static_substrait_AggregateFunction_descriptor;
}
@java.lang.Override
public io.substrait.proto.AggregateFunction getDefaultInstanceForType() {
return io.substrait.proto.AggregateFunction.getDefaultInstance();
}
@java.lang.Override
public io.substrait.proto.AggregateFunction build() {
io.substrait.proto.AggregateFunction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.substrait.proto.AggregateFunction buildPartial() {
io.substrait.proto.AggregateFunction result = new io.substrait.proto.AggregateFunction(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(io.substrait.proto.AggregateFunction result) {
if (argumentsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
arguments_ = java.util.Collections.unmodifiableList(arguments_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.arguments_ = arguments_;
} else {
result.arguments_ = argumentsBuilder_.build();
}
if (optionsBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
options_ = java.util.Collections.unmodifiableList(options_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.options_ = options_;
} else {
result.options_ = optionsBuilder_.build();
}
if (sortsBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
sorts_ = java.util.Collections.unmodifiableList(sorts_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.sorts_ = sorts_;
} else {
result.sorts_ = sortsBuilder_.build();
}
if (argsBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
args_ = java.util.Collections.unmodifiableList(args_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.args_ = args_;
} else {
result.args_ = argsBuilder_.build();
}
}
private void buildPartial0(io.substrait.proto.AggregateFunction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.functionReference_ = functionReference_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.outputType_ = outputTypeBuilder_ == null
? outputType_
: outputTypeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.phase_ = phase_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.invocation_ = invocation_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.substrait.proto.AggregateFunction) {
return mergeFrom((io.substrait.proto.AggregateFunction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.substrait.proto.AggregateFunction other) {
if (other == io.substrait.proto.AggregateFunction.getDefaultInstance()) return this;
if (other.getFunctionReference() != 0) {
setFunctionReference(other.getFunctionReference());
}
if (argumentsBuilder_ == null) {
if (!other.arguments_.isEmpty()) {
if (arguments_.isEmpty()) {
arguments_ = other.arguments_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureArgumentsIsMutable();
arguments_.addAll(other.arguments_);
}
onChanged();
}
} else {
if (!other.arguments_.isEmpty()) {
if (argumentsBuilder_.isEmpty()) {
argumentsBuilder_.dispose();
argumentsBuilder_ = null;
arguments_ = other.arguments_;
bitField0_ = (bitField0_ & ~0x00000002);
argumentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getArgumentsFieldBuilder() : null;
} else {
argumentsBuilder_.addAllMessages(other.arguments_);
}
}
}
if (optionsBuilder_ == null) {
if (!other.options_.isEmpty()) {
if (options_.isEmpty()) {
options_ = other.options_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureOptionsIsMutable();
options_.addAll(other.options_);
}
onChanged();
}
} else {
if (!other.options_.isEmpty()) {
if (optionsBuilder_.isEmpty()) {
optionsBuilder_.dispose();
optionsBuilder_ = null;
options_ = other.options_;
bitField0_ = (bitField0_ & ~0x00000004);
optionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOptionsFieldBuilder() : null;
} else {
optionsBuilder_.addAllMessages(other.options_);
}
}
}
if (other.hasOutputType()) {
mergeOutputType(other.getOutputType());
}
if (other.phase_ != 0) {
setPhaseValue(other.getPhaseValue());
}
if (sortsBuilder_ == null) {
if (!other.sorts_.isEmpty()) {
if (sorts_.isEmpty()) {
sorts_ = other.sorts_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureSortsIsMutable();
sorts_.addAll(other.sorts_);
}
onChanged();
}
} else {
if (!other.sorts_.isEmpty()) {
if (sortsBuilder_.isEmpty()) {
sortsBuilder_.dispose();
sortsBuilder_ = null;
sorts_ = other.sorts_;
bitField0_ = (bitField0_ & ~0x00000020);
sortsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSortsFieldBuilder() : null;
} else {
sortsBuilder_.addAllMessages(other.sorts_);
}
}
}
if (other.invocation_ != 0) {
setInvocationValue(other.getInvocationValue());
}
if (argsBuilder_ == null) {
if (!other.args_.isEmpty()) {
if (args_.isEmpty()) {
args_ = other.args_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureArgsIsMutable();
args_.addAll(other.args_);
}
onChanged();
}
} else {
if (!other.args_.isEmpty()) {
if (argsBuilder_.isEmpty()) {
argsBuilder_.dispose();
argsBuilder_ = null;
args_ = other.args_;
bitField0_ = (bitField0_ & ~0x00000080);
argsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getArgsFieldBuilder() : null;
} else {
argsBuilder_.addAllMessages(other.args_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
functionReference_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
io.substrait.proto.Expression m =
input.readMessage(
io.substrait.proto.Expression.parser(),
extensionRegistry);
if (argsBuilder_ == null) {
ensureArgsIsMutable();
args_.add(m);
} else {
argsBuilder_.addMessage(m);
}
break;
} // case 18
case 26: {
io.substrait.proto.SortField m =
input.readMessage(
io.substrait.proto.SortField.parser(),
extensionRegistry);
if (sortsBuilder_ == null) {
ensureSortsIsMutable();
sorts_.add(m);
} else {
sortsBuilder_.addMessage(m);
}
break;
} // case 26
case 32: {
phase_ = input.readEnum();
bitField0_ |= 0x00000010;
break;
} // case 32
case 42: {
input.readMessage(
getOutputTypeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 42
case 48: {
invocation_ = input.readEnum();
bitField0_ |= 0x00000040;
break;
} // case 48
case 58: {
io.substrait.proto.FunctionArgument m =
input.readMessage(
io.substrait.proto.FunctionArgument.parser(),
extensionRegistry);
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.add(m);
} else {
argumentsBuilder_.addMessage(m);
}
break;
} // case 58
case 66: {
io.substrait.proto.FunctionOption m =
input.readMessage(
io.substrait.proto.FunctionOption.parser(),
extensionRegistry);
if (optionsBuilder_ == null) {
ensureOptionsIsMutable();
options_.add(m);
} else {
optionsBuilder_.addMessage(m);
}
break;
} // case 66
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int functionReference_ ;
/**
*
* Points to a function_anchor defined in this plan, which must refer
* to an aggregate function in the associated YAML file. Required; 0 is
* considered to be a valid anchor/reference.
*
*
* uint32 function_reference = 1;
* @return The functionReference.
*/
@java.lang.Override
public int getFunctionReference() {
return functionReference_;
}
/**
*
* Points to a function_anchor defined in this plan, which must refer
* to an aggregate function in the associated YAML file. Required; 0 is
* considered to be a valid anchor/reference.
*
*
* uint32 function_reference = 1;
* @param value The functionReference to set.
* @return This builder for chaining.
*/
public Builder setFunctionReference(int value) {
functionReference_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Points to a function_anchor defined in this plan, which must refer
* to an aggregate function in the associated YAML file. Required; 0 is
* considered to be a valid anchor/reference.
*
*
* uint32 function_reference = 1;
* @return This builder for chaining.
*/
public Builder clearFunctionReference() {
bitField0_ = (bitField0_ & ~0x00000001);
functionReference_ = 0;
onChanged();
return this;
}
private java.util.List arguments_ =
java.util.Collections.emptyList();
private void ensureArgumentsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
arguments_ = new java.util.ArrayList(arguments_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.FunctionArgument, io.substrait.proto.FunctionArgument.Builder, io.substrait.proto.FunctionArgumentOrBuilder> argumentsBuilder_;
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public java.util.List getArgumentsList() {
if (argumentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(arguments_);
} else {
return argumentsBuilder_.getMessageList();
}
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public int getArgumentsCount() {
if (argumentsBuilder_ == null) {
return arguments_.size();
} else {
return argumentsBuilder_.getCount();
}
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public io.substrait.proto.FunctionArgument getArguments(int index) {
if (argumentsBuilder_ == null) {
return arguments_.get(index);
} else {
return argumentsBuilder_.getMessage(index);
}
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder setArguments(
int index, io.substrait.proto.FunctionArgument value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.set(index, value);
onChanged();
} else {
argumentsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder setArguments(
int index, io.substrait.proto.FunctionArgument.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.set(index, builderForValue.build());
onChanged();
} else {
argumentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder addArguments(io.substrait.proto.FunctionArgument value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(value);
onChanged();
} else {
argumentsBuilder_.addMessage(value);
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder addArguments(
int index, io.substrait.proto.FunctionArgument value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(index, value);
onChanged();
} else {
argumentsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder addArguments(
io.substrait.proto.FunctionArgument.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.add(builderForValue.build());
onChanged();
} else {
argumentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder addArguments(
int index, io.substrait.proto.FunctionArgument.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.add(index, builderForValue.build());
onChanged();
} else {
argumentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder addAllArguments(
java.lang.Iterable extends io.substrait.proto.FunctionArgument> values) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, arguments_);
onChanged();
} else {
argumentsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder clearArguments() {
if (argumentsBuilder_ == null) {
arguments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
argumentsBuilder_.clear();
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public Builder removeArguments(int index) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.remove(index);
onChanged();
} else {
argumentsBuilder_.remove(index);
}
return this;
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public io.substrait.proto.FunctionArgument.Builder getArgumentsBuilder(
int index) {
return getArgumentsFieldBuilder().getBuilder(index);
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public io.substrait.proto.FunctionArgumentOrBuilder getArgumentsOrBuilder(
int index) {
if (argumentsBuilder_ == null) {
return arguments_.get(index); } else {
return argumentsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public java.util.List extends io.substrait.proto.FunctionArgumentOrBuilder>
getArgumentsOrBuilderList() {
if (argumentsBuilder_ != null) {
return argumentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(arguments_);
}
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public io.substrait.proto.FunctionArgument.Builder addArgumentsBuilder() {
return getArgumentsFieldBuilder().addBuilder(
io.substrait.proto.FunctionArgument.getDefaultInstance());
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public io.substrait.proto.FunctionArgument.Builder addArgumentsBuilder(
int index) {
return getArgumentsFieldBuilder().addBuilder(
index, io.substrait.proto.FunctionArgument.getDefaultInstance());
}
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
public java.util.List
getArgumentsBuilderList() {
return getArgumentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.FunctionArgument, io.substrait.proto.FunctionArgument.Builder, io.substrait.proto.FunctionArgumentOrBuilder>
getArgumentsFieldBuilder() {
if (argumentsBuilder_ == null) {
argumentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.FunctionArgument, io.substrait.proto.FunctionArgument.Builder, io.substrait.proto.FunctionArgumentOrBuilder>(
arguments_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
arguments_ = null;
}
return argumentsBuilder_;
}
private java.util.List options_ =
java.util.Collections.emptyList();
private void ensureOptionsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
options_ = new java.util.ArrayList(options_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.FunctionOption, io.substrait.proto.FunctionOption.Builder, io.substrait.proto.FunctionOptionOrBuilder> optionsBuilder_;
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public java.util.List getOptionsList() {
if (optionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(options_);
} else {
return optionsBuilder_.getMessageList();
}
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public int getOptionsCount() {
if (optionsBuilder_ == null) {
return options_.size();
} else {
return optionsBuilder_.getCount();
}
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public io.substrait.proto.FunctionOption getOptions(int index) {
if (optionsBuilder_ == null) {
return options_.get(index);
} else {
return optionsBuilder_.getMessage(index);
}
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder setOptions(
int index, io.substrait.proto.FunctionOption value) {
if (optionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionsIsMutable();
options_.set(index, value);
onChanged();
} else {
optionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder setOptions(
int index, io.substrait.proto.FunctionOption.Builder builderForValue) {
if (optionsBuilder_ == null) {
ensureOptionsIsMutable();
options_.set(index, builderForValue.build());
onChanged();
} else {
optionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder addOptions(io.substrait.proto.FunctionOption value) {
if (optionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionsIsMutable();
options_.add(value);
onChanged();
} else {
optionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder addOptions(
int index, io.substrait.proto.FunctionOption value) {
if (optionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionsIsMutable();
options_.add(index, value);
onChanged();
} else {
optionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder addOptions(
io.substrait.proto.FunctionOption.Builder builderForValue) {
if (optionsBuilder_ == null) {
ensureOptionsIsMutable();
options_.add(builderForValue.build());
onChanged();
} else {
optionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder addOptions(
int index, io.substrait.proto.FunctionOption.Builder builderForValue) {
if (optionsBuilder_ == null) {
ensureOptionsIsMutable();
options_.add(index, builderForValue.build());
onChanged();
} else {
optionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder addAllOptions(
java.lang.Iterable extends io.substrait.proto.FunctionOption> values) {
if (optionsBuilder_ == null) {
ensureOptionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, options_);
onChanged();
} else {
optionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder clearOptions() {
if (optionsBuilder_ == null) {
options_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
optionsBuilder_.clear();
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public Builder removeOptions(int index) {
if (optionsBuilder_ == null) {
ensureOptionsIsMutable();
options_.remove(index);
onChanged();
} else {
optionsBuilder_.remove(index);
}
return this;
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public io.substrait.proto.FunctionOption.Builder getOptionsBuilder(
int index) {
return getOptionsFieldBuilder().getBuilder(index);
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public io.substrait.proto.FunctionOptionOrBuilder getOptionsOrBuilder(
int index) {
if (optionsBuilder_ == null) {
return options_.get(index); } else {
return optionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public java.util.List extends io.substrait.proto.FunctionOptionOrBuilder>
getOptionsOrBuilderList() {
if (optionsBuilder_ != null) {
return optionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(options_);
}
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public io.substrait.proto.FunctionOption.Builder addOptionsBuilder() {
return getOptionsFieldBuilder().addBuilder(
io.substrait.proto.FunctionOption.getDefaultInstance());
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public io.substrait.proto.FunctionOption.Builder addOptionsBuilder(
int index) {
return getOptionsFieldBuilder().addBuilder(
index, io.substrait.proto.FunctionOption.getDefaultInstance());
}
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
public java.util.List
getOptionsBuilderList() {
return getOptionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.FunctionOption, io.substrait.proto.FunctionOption.Builder, io.substrait.proto.FunctionOptionOrBuilder>
getOptionsFieldBuilder() {
if (optionsBuilder_ == null) {
optionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.FunctionOption, io.substrait.proto.FunctionOption.Builder, io.substrait.proto.FunctionOptionOrBuilder>(
options_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
options_ = null;
}
return optionsBuilder_;
}
private io.substrait.proto.Type outputType_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.Type, io.substrait.proto.Type.Builder, io.substrait.proto.TypeOrBuilder> outputTypeBuilder_;
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
* @return Whether the outputType field is set.
*/
public boolean hasOutputType() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
* @return The outputType.
*/
public io.substrait.proto.Type getOutputType() {
if (outputTypeBuilder_ == null) {
return outputType_ == null ? io.substrait.proto.Type.getDefaultInstance() : outputType_;
} else {
return outputTypeBuilder_.getMessage();
}
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
public Builder setOutputType(io.substrait.proto.Type value) {
if (outputTypeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
outputType_ = value;
} else {
outputTypeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
public Builder setOutputType(
io.substrait.proto.Type.Builder builderForValue) {
if (outputTypeBuilder_ == null) {
outputType_ = builderForValue.build();
} else {
outputTypeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
public Builder mergeOutputType(io.substrait.proto.Type value) {
if (outputTypeBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
outputType_ != null &&
outputType_ != io.substrait.proto.Type.getDefaultInstance()) {
getOutputTypeBuilder().mergeFrom(value);
} else {
outputType_ = value;
}
} else {
outputTypeBuilder_.mergeFrom(value);
}
if (outputType_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
public Builder clearOutputType() {
bitField0_ = (bitField0_ & ~0x00000008);
outputType_ = null;
if (outputTypeBuilder_ != null) {
outputTypeBuilder_.dispose();
outputTypeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
public io.substrait.proto.Type.Builder getOutputTypeBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getOutputTypeFieldBuilder().getBuilder();
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
public io.substrait.proto.TypeOrBuilder getOutputTypeOrBuilder() {
if (outputTypeBuilder_ != null) {
return outputTypeBuilder_.getMessageOrBuilder();
} else {
return outputType_ == null ?
io.substrait.proto.Type.getDefaultInstance() : outputType_;
}
}
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.Type, io.substrait.proto.Type.Builder, io.substrait.proto.TypeOrBuilder>
getOutputTypeFieldBuilder() {
if (outputTypeBuilder_ == null) {
outputTypeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.Type, io.substrait.proto.Type.Builder, io.substrait.proto.TypeOrBuilder>(
getOutputType(),
getParentForChildren(),
isClean());
outputType_ = null;
}
return outputTypeBuilder_;
}
private int phase_ = 0;
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @return The enum numeric value on the wire for phase.
*/
@java.lang.Override public int getPhaseValue() {
return phase_;
}
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @param value The enum numeric value on the wire for phase to set.
* @return This builder for chaining.
*/
public Builder setPhaseValue(int value) {
phase_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @return The phase.
*/
@java.lang.Override
public io.substrait.proto.AggregationPhase getPhase() {
io.substrait.proto.AggregationPhase result = io.substrait.proto.AggregationPhase.forNumber(phase_);
return result == null ? io.substrait.proto.AggregationPhase.UNRECOGNIZED : result;
}
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @param value The phase to set.
* @return This builder for chaining.
*/
public Builder setPhase(io.substrait.proto.AggregationPhase value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
phase_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @return This builder for chaining.
*/
public Builder clearPhase() {
bitField0_ = (bitField0_ & ~0x00000010);
phase_ = 0;
onChanged();
return this;
}
private java.util.List sorts_ =
java.util.Collections.emptyList();
private void ensureSortsIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
sorts_ = new java.util.ArrayList(sorts_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.SortField, io.substrait.proto.SortField.Builder, io.substrait.proto.SortFieldOrBuilder> sortsBuilder_;
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public java.util.List getSortsList() {
if (sortsBuilder_ == null) {
return java.util.Collections.unmodifiableList(sorts_);
} else {
return sortsBuilder_.getMessageList();
}
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public int getSortsCount() {
if (sortsBuilder_ == null) {
return sorts_.size();
} else {
return sortsBuilder_.getCount();
}
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public io.substrait.proto.SortField getSorts(int index) {
if (sortsBuilder_ == null) {
return sorts_.get(index);
} else {
return sortsBuilder_.getMessage(index);
}
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder setSorts(
int index, io.substrait.proto.SortField value) {
if (sortsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSortsIsMutable();
sorts_.set(index, value);
onChanged();
} else {
sortsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder setSorts(
int index, io.substrait.proto.SortField.Builder builderForValue) {
if (sortsBuilder_ == null) {
ensureSortsIsMutable();
sorts_.set(index, builderForValue.build());
onChanged();
} else {
sortsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder addSorts(io.substrait.proto.SortField value) {
if (sortsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSortsIsMutable();
sorts_.add(value);
onChanged();
} else {
sortsBuilder_.addMessage(value);
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder addSorts(
int index, io.substrait.proto.SortField value) {
if (sortsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSortsIsMutable();
sorts_.add(index, value);
onChanged();
} else {
sortsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder addSorts(
io.substrait.proto.SortField.Builder builderForValue) {
if (sortsBuilder_ == null) {
ensureSortsIsMutable();
sorts_.add(builderForValue.build());
onChanged();
} else {
sortsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder addSorts(
int index, io.substrait.proto.SortField.Builder builderForValue) {
if (sortsBuilder_ == null) {
ensureSortsIsMutable();
sorts_.add(index, builderForValue.build());
onChanged();
} else {
sortsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder addAllSorts(
java.lang.Iterable extends io.substrait.proto.SortField> values) {
if (sortsBuilder_ == null) {
ensureSortsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sorts_);
onChanged();
} else {
sortsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder clearSorts() {
if (sortsBuilder_ == null) {
sorts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
sortsBuilder_.clear();
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public Builder removeSorts(int index) {
if (sortsBuilder_ == null) {
ensureSortsIsMutable();
sorts_.remove(index);
onChanged();
} else {
sortsBuilder_.remove(index);
}
return this;
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public io.substrait.proto.SortField.Builder getSortsBuilder(
int index) {
return getSortsFieldBuilder().getBuilder(index);
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public io.substrait.proto.SortFieldOrBuilder getSortsOrBuilder(
int index) {
if (sortsBuilder_ == null) {
return sorts_.get(index); } else {
return sortsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public java.util.List extends io.substrait.proto.SortFieldOrBuilder>
getSortsOrBuilderList() {
if (sortsBuilder_ != null) {
return sortsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sorts_);
}
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public io.substrait.proto.SortField.Builder addSortsBuilder() {
return getSortsFieldBuilder().addBuilder(
io.substrait.proto.SortField.getDefaultInstance());
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public io.substrait.proto.SortField.Builder addSortsBuilder(
int index) {
return getSortsFieldBuilder().addBuilder(
index, io.substrait.proto.SortField.getDefaultInstance());
}
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
public java.util.List
getSortsBuilderList() {
return getSortsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.SortField, io.substrait.proto.SortField.Builder, io.substrait.proto.SortFieldOrBuilder>
getSortsFieldBuilder() {
if (sortsBuilder_ == null) {
sortsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.SortField, io.substrait.proto.SortField.Builder, io.substrait.proto.SortFieldOrBuilder>(
sorts_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
sorts_ = null;
}
return sortsBuilder_;
}
private int invocation_ = 0;
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @return The enum numeric value on the wire for invocation.
*/
@java.lang.Override public int getInvocationValue() {
return invocation_;
}
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @param value The enum numeric value on the wire for invocation to set.
* @return This builder for chaining.
*/
public Builder setInvocationValue(int value) {
invocation_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @return The invocation.
*/
@java.lang.Override
public io.substrait.proto.AggregateFunction.AggregationInvocation getInvocation() {
io.substrait.proto.AggregateFunction.AggregationInvocation result = io.substrait.proto.AggregateFunction.AggregationInvocation.forNumber(invocation_);
return result == null ? io.substrait.proto.AggregateFunction.AggregationInvocation.UNRECOGNIZED : result;
}
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @param value The invocation to set.
* @return This builder for chaining.
*/
public Builder setInvocation(io.substrait.proto.AggregateFunction.AggregationInvocation value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
invocation_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @return This builder for chaining.
*/
public Builder clearInvocation() {
bitField0_ = (bitField0_ & ~0x00000040);
invocation_ = 0;
onChanged();
return this;
}
private java.util.List args_ =
java.util.Collections.emptyList();
private void ensureArgsIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
args_ = new java.util.ArrayList(args_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.Expression, io.substrait.proto.Expression.Builder, io.substrait.proto.ExpressionOrBuilder> argsBuilder_;
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List getArgsList() {
if (argsBuilder_ == null) {
return java.util.Collections.unmodifiableList(args_);
} else {
return argsBuilder_.getMessageList();
}
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public int getArgsCount() {
if (argsBuilder_ == null) {
return args_.size();
} else {
return argsBuilder_.getCount();
}
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public io.substrait.proto.Expression getArgs(int index) {
if (argsBuilder_ == null) {
return args_.get(index);
} else {
return argsBuilder_.getMessage(index);
}
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder setArgs(
int index, io.substrait.proto.Expression value) {
if (argsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgsIsMutable();
args_.set(index, value);
onChanged();
} else {
argsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder setArgs(
int index, io.substrait.proto.Expression.Builder builderForValue) {
if (argsBuilder_ == null) {
ensureArgsIsMutable();
args_.set(index, builderForValue.build());
onChanged();
} else {
argsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder addArgs(io.substrait.proto.Expression value) {
if (argsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgsIsMutable();
args_.add(value);
onChanged();
} else {
argsBuilder_.addMessage(value);
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder addArgs(
int index, io.substrait.proto.Expression value) {
if (argsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgsIsMutable();
args_.add(index, value);
onChanged();
} else {
argsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder addArgs(
io.substrait.proto.Expression.Builder builderForValue) {
if (argsBuilder_ == null) {
ensureArgsIsMutable();
args_.add(builderForValue.build());
onChanged();
} else {
argsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder addArgs(
int index, io.substrait.proto.Expression.Builder builderForValue) {
if (argsBuilder_ == null) {
ensureArgsIsMutable();
args_.add(index, builderForValue.build());
onChanged();
} else {
argsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder addAllArgs(
java.lang.Iterable extends io.substrait.proto.Expression> values) {
if (argsBuilder_ == null) {
ensureArgsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, args_);
onChanged();
} else {
argsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearArgs() {
if (argsBuilder_ == null) {
args_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
argsBuilder_.clear();
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public Builder removeArgs(int index) {
if (argsBuilder_ == null) {
ensureArgsIsMutable();
args_.remove(index);
onChanged();
} else {
argsBuilder_.remove(index);
}
return this;
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public io.substrait.proto.Expression.Builder getArgsBuilder(
int index) {
return getArgsFieldBuilder().getBuilder(index);
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public io.substrait.proto.ExpressionOrBuilder getArgsOrBuilder(
int index) {
if (argsBuilder_ == null) {
return args_.get(index); } else {
return argsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List extends io.substrait.proto.ExpressionOrBuilder>
getArgsOrBuilderList() {
if (argsBuilder_ != null) {
return argsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(args_);
}
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public io.substrait.proto.Expression.Builder addArgsBuilder() {
return getArgsFieldBuilder().addBuilder(
io.substrait.proto.Expression.getDefaultInstance());
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public io.substrait.proto.Expression.Builder addArgsBuilder(
int index) {
return getArgsFieldBuilder().addBuilder(
index, io.substrait.proto.Expression.getDefaultInstance());
}
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List
getArgsBuilderList() {
return getArgsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.Expression, io.substrait.proto.Expression.Builder, io.substrait.proto.ExpressionOrBuilder>
getArgsFieldBuilder() {
if (argsBuilder_ == null) {
argsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.substrait.proto.Expression, io.substrait.proto.Expression.Builder, io.substrait.proto.ExpressionOrBuilder>(
args_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
args_ = null;
}
return argsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:substrait.AggregateFunction)
}
// @@protoc_insertion_point(class_scope:substrait.AggregateFunction)
private static final io.substrait.proto.AggregateFunction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.substrait.proto.AggregateFunction();
}
public static io.substrait.proto.AggregateFunction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AggregateFunction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.substrait.proto.AggregateFunction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy