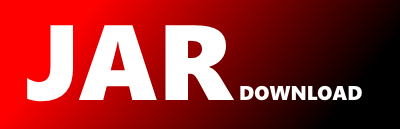
io.substrait.proto.AggregateFunctionOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Create a well-defined, cross-language specification for data compute operations
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: substrait/algebra.proto
// Protobuf Java Version: 3.25.5
package io.substrait.proto;
public interface AggregateFunctionOrBuilder extends
// @@protoc_insertion_point(interface_extends:substrait.AggregateFunction)
com.google.protobuf.MessageOrBuilder {
/**
*
* Points to a function_anchor defined in this plan, which must refer
* to an aggregate function in the associated YAML file. Required; 0 is
* considered to be a valid anchor/reference.
*
*
* uint32 function_reference = 1;
* @return The functionReference.
*/
int getFunctionReference();
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
java.util.List
getArgumentsList();
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
io.substrait.proto.FunctionArgument getArguments(int index);
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
int getArgumentsCount();
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
java.util.List extends io.substrait.proto.FunctionArgumentOrBuilder>
getArgumentsOrBuilderList();
/**
*
* The arguments to be bound to the function. This must have exactly the
* number of arguments specified in the function definition, and the
* argument types must also match exactly:
*
* - Value arguments must be bound using FunctionArgument.value, and
* the expression in that must yield a value of a type that a function
* overload is defined for.
* - Type arguments must be bound using FunctionArgument.type, and a
* function overload must be defined for that type.
* - Enum arguments must be bound using FunctionArgument.enum
* followed by Enum.specified, with a string that case-insensitively
* matches one of the allowed options.
* - Optional enum arguments must be bound using FunctionArgument.enum
* followed by either Enum.specified or Enum.unspecified. If specified,
* the string must case-insensitively match one of the allowed options.
*
*
* repeated .substrait.FunctionArgument arguments = 7;
*/
io.substrait.proto.FunctionArgumentOrBuilder getArgumentsOrBuilder(
int index);
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
java.util.List
getOptionsList();
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
io.substrait.proto.FunctionOption getOptions(int index);
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
int getOptionsCount();
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
java.util.List extends io.substrait.proto.FunctionOptionOrBuilder>
getOptionsOrBuilderList();
/**
*
* Options to specify behavior for corner cases, or leave behavior
* unspecified if the consumer does not need specific behavior in these
* cases.
*
*
* repeated .substrait.FunctionOption options = 8;
*/
io.substrait.proto.FunctionOptionOrBuilder getOptionsOrBuilder(
int index);
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
* @return Whether the outputType field is set.
*/
boolean hasOutputType();
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
* @return The outputType.
*/
io.substrait.proto.Type getOutputType();
/**
*
* Must be set to the return type of the function, exactly as derived
* using the declaration in the extension.
*
*
* .substrait.Type output_type = 5;
*/
io.substrait.proto.TypeOrBuilder getOutputTypeOrBuilder();
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @return The enum numeric value on the wire for phase.
*/
int getPhaseValue();
/**
*
* Describes which part of the aggregation to perform within the context of
* distributed algorithms. Required. Must be set to INITIAL_TO_RESULT for
* aggregate functions that are not decomposable.
*
*
* .substrait.AggregationPhase phase = 4;
* @return The phase.
*/
io.substrait.proto.AggregationPhase getPhase();
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
java.util.List
getSortsList();
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
io.substrait.proto.SortField getSorts(int index);
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
int getSortsCount();
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
java.util.List extends io.substrait.proto.SortFieldOrBuilder>
getSortsOrBuilderList();
/**
*
* If specified, the aggregated records are ordered according to this list
* before they are aggregated. The first sort field has the highest
* priority; only if a sort field determines two records to be equivalent is
* the next field queried. This field is optional.
*
*
* repeated .substrait.SortField sorts = 3;
*/
io.substrait.proto.SortFieldOrBuilder getSortsOrBuilder(
int index);
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @return The enum numeric value on the wire for invocation.
*/
int getInvocationValue();
/**
*
* Specifies whether equivalent records are merged before being aggregated.
* Optional, defaults to AGGREGATION_INVOCATION_ALL.
*
*
* .substrait.AggregateFunction.AggregationInvocation invocation = 6;
* @return The invocation.
*/
io.substrait.proto.AggregateFunction.AggregationInvocation getInvocation();
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated java.util.List
getArgsList();
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated io.substrait.proto.Expression getArgs(int index);
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated int getArgsCount();
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated java.util.List extends io.substrait.proto.ExpressionOrBuilder>
getArgsOrBuilderList();
/**
*
* deprecated; use arguments instead
*
*
* repeated .substrait.Expression args = 2 [deprecated = true];
*/
@java.lang.Deprecated io.substrait.proto.ExpressionOrBuilder getArgsOrBuilder(
int index);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy