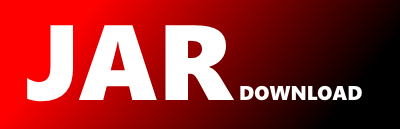
io.substrait.proto.RelCommon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Create a well-defined, cross-language specification for data compute operations
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: substrait/algebra.proto
// Protobuf Java Version: 3.25.5
package io.substrait.proto;
/**
*
* Common fields for all relational operators
*
*
* Protobuf type {@code substrait.RelCommon}
*/
public final class RelCommon extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:substrait.RelCommon)
RelCommonOrBuilder {
private static final long serialVersionUID = 0L;
// Use RelCommon.newBuilder() to construct.
private RelCommon(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RelCommon() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RelCommon();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.class, io.substrait.proto.RelCommon.Builder.class);
}
public interface DirectOrBuilder extends
// @@protoc_insertion_point(interface_extends:substrait.RelCommon.Direct)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* Direct indicates no change on presence and ordering of fields in the output
*
*
* Protobuf type {@code substrait.RelCommon.Direct}
*/
public static final class Direct extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:substrait.RelCommon.Direct)
DirectOrBuilder {
private static final long serialVersionUID = 0L;
// Use Direct.newBuilder() to construct.
private Direct(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Direct() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Direct();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Direct_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Direct_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Direct.class, io.substrait.proto.RelCommon.Direct.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.substrait.proto.RelCommon.Direct)) {
return super.equals(obj);
}
io.substrait.proto.RelCommon.Direct other = (io.substrait.proto.RelCommon.Direct) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Direct parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Direct parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Direct parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.substrait.proto.RelCommon.Direct prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Direct indicates no change on presence and ordering of fields in the output
*
*
* Protobuf type {@code substrait.RelCommon.Direct}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:substrait.RelCommon.Direct)
io.substrait.proto.RelCommon.DirectOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Direct_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Direct_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Direct.class, io.substrait.proto.RelCommon.Direct.Builder.class);
}
// Construct using io.substrait.proto.RelCommon.Direct.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Direct_descriptor;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Direct getDefaultInstanceForType() {
return io.substrait.proto.RelCommon.Direct.getDefaultInstance();
}
@java.lang.Override
public io.substrait.proto.RelCommon.Direct build() {
io.substrait.proto.RelCommon.Direct result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Direct buildPartial() {
io.substrait.proto.RelCommon.Direct result = new io.substrait.proto.RelCommon.Direct(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.substrait.proto.RelCommon.Direct) {
return mergeFrom((io.substrait.proto.RelCommon.Direct)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.substrait.proto.RelCommon.Direct other) {
if (other == io.substrait.proto.RelCommon.Direct.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:substrait.RelCommon.Direct)
}
// @@protoc_insertion_point(class_scope:substrait.RelCommon.Direct)
private static final io.substrait.proto.RelCommon.Direct DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.substrait.proto.RelCommon.Direct();
}
public static io.substrait.proto.RelCommon.Direct getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Direct parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Direct getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EmitOrBuilder extends
// @@protoc_insertion_point(interface_extends:substrait.RelCommon.Emit)
com.google.protobuf.MessageOrBuilder {
/**
* repeated int32 output_mapping = 1;
* @return A list containing the outputMapping.
*/
java.util.List getOutputMappingList();
/**
* repeated int32 output_mapping = 1;
* @return The count of outputMapping.
*/
int getOutputMappingCount();
/**
* repeated int32 output_mapping = 1;
* @param index The index of the element to return.
* @return The outputMapping at the given index.
*/
int getOutputMapping(int index);
}
/**
*
* Remap which fields are output and in which order
*
*
* Protobuf type {@code substrait.RelCommon.Emit}
*/
public static final class Emit extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:substrait.RelCommon.Emit)
EmitOrBuilder {
private static final long serialVersionUID = 0L;
// Use Emit.newBuilder() to construct.
private Emit(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Emit() {
outputMapping_ = emptyIntList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Emit();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Emit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Emit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Emit.class, io.substrait.proto.RelCommon.Emit.Builder.class);
}
public static final int OUTPUT_MAPPING_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.IntList outputMapping_ =
emptyIntList();
/**
* repeated int32 output_mapping = 1;
* @return A list containing the outputMapping.
*/
@java.lang.Override
public java.util.List
getOutputMappingList() {
return outputMapping_;
}
/**
* repeated int32 output_mapping = 1;
* @return The count of outputMapping.
*/
public int getOutputMappingCount() {
return outputMapping_.size();
}
/**
* repeated int32 output_mapping = 1;
* @param index The index of the element to return.
* @return The outputMapping at the given index.
*/
public int getOutputMapping(int index) {
return outputMapping_.getInt(index);
}
private int outputMappingMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getOutputMappingList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(outputMappingMemoizedSerializedSize);
}
for (int i = 0; i < outputMapping_.size(); i++) {
output.writeInt32NoTag(outputMapping_.getInt(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < outputMapping_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(outputMapping_.getInt(i));
}
size += dataSize;
if (!getOutputMappingList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
outputMappingMemoizedSerializedSize = dataSize;
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.substrait.proto.RelCommon.Emit)) {
return super.equals(obj);
}
io.substrait.proto.RelCommon.Emit other = (io.substrait.proto.RelCommon.Emit) obj;
if (!getOutputMappingList()
.equals(other.getOutputMappingList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getOutputMappingCount() > 0) {
hash = (37 * hash) + OUTPUT_MAPPING_FIELD_NUMBER;
hash = (53 * hash) + getOutputMappingList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Emit parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Emit parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Emit parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.substrait.proto.RelCommon.Emit prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Remap which fields are output and in which order
*
*
* Protobuf type {@code substrait.RelCommon.Emit}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:substrait.RelCommon.Emit)
io.substrait.proto.RelCommon.EmitOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Emit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Emit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Emit.class, io.substrait.proto.RelCommon.Emit.Builder.class);
}
// Construct using io.substrait.proto.RelCommon.Emit.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
outputMapping_ = emptyIntList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Emit_descriptor;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Emit getDefaultInstanceForType() {
return io.substrait.proto.RelCommon.Emit.getDefaultInstance();
}
@java.lang.Override
public io.substrait.proto.RelCommon.Emit build() {
io.substrait.proto.RelCommon.Emit result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Emit buildPartial() {
io.substrait.proto.RelCommon.Emit result = new io.substrait.proto.RelCommon.Emit(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(io.substrait.proto.RelCommon.Emit result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
outputMapping_.makeImmutable();
result.outputMapping_ = outputMapping_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.substrait.proto.RelCommon.Emit) {
return mergeFrom((io.substrait.proto.RelCommon.Emit)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.substrait.proto.RelCommon.Emit other) {
if (other == io.substrait.proto.RelCommon.Emit.getDefaultInstance()) return this;
if (!other.outputMapping_.isEmpty()) {
if (outputMapping_.isEmpty()) {
outputMapping_ = other.outputMapping_;
outputMapping_.makeImmutable();
bitField0_ |= 0x00000001;
} else {
ensureOutputMappingIsMutable();
outputMapping_.addAll(other.outputMapping_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int v = input.readInt32();
ensureOutputMappingIsMutable();
outputMapping_.addInt(v);
break;
} // case 8
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureOutputMappingIsMutable();
while (input.getBytesUntilLimit() > 0) {
outputMapping_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.IntList outputMapping_ = emptyIntList();
private void ensureOutputMappingIsMutable() {
if (!outputMapping_.isModifiable()) {
outputMapping_ = makeMutableCopy(outputMapping_);
}
bitField0_ |= 0x00000001;
}
/**
* repeated int32 output_mapping = 1;
* @return A list containing the outputMapping.
*/
public java.util.List
getOutputMappingList() {
outputMapping_.makeImmutable();
return outputMapping_;
}
/**
* repeated int32 output_mapping = 1;
* @return The count of outputMapping.
*/
public int getOutputMappingCount() {
return outputMapping_.size();
}
/**
* repeated int32 output_mapping = 1;
* @param index The index of the element to return.
* @return The outputMapping at the given index.
*/
public int getOutputMapping(int index) {
return outputMapping_.getInt(index);
}
/**
* repeated int32 output_mapping = 1;
* @param index The index to set the value at.
* @param value The outputMapping to set.
* @return This builder for chaining.
*/
public Builder setOutputMapping(
int index, int value) {
ensureOutputMappingIsMutable();
outputMapping_.setInt(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated int32 output_mapping = 1;
* @param value The outputMapping to add.
* @return This builder for chaining.
*/
public Builder addOutputMapping(int value) {
ensureOutputMappingIsMutable();
outputMapping_.addInt(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated int32 output_mapping = 1;
* @param values The outputMapping to add.
* @return This builder for chaining.
*/
public Builder addAllOutputMapping(
java.lang.Iterable extends java.lang.Integer> values) {
ensureOutputMappingIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputMapping_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* repeated int32 output_mapping = 1;
* @return This builder for chaining.
*/
public Builder clearOutputMapping() {
outputMapping_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:substrait.RelCommon.Emit)
}
// @@protoc_insertion_point(class_scope:substrait.RelCommon.Emit)
private static final io.substrait.proto.RelCommon.Emit DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.substrait.proto.RelCommon.Emit();
}
public static io.substrait.proto.RelCommon.Emit getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Emit parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Emit getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HintOrBuilder extends
// @@protoc_insertion_point(interface_extends:substrait.RelCommon.Hint)
com.google.protobuf.MessageOrBuilder {
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
* @return Whether the stats field is set.
*/
boolean hasStats();
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
* @return The stats.
*/
io.substrait.proto.RelCommon.Hint.Stats getStats();
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
io.substrait.proto.RelCommon.Hint.StatsOrBuilder getStatsOrBuilder();
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
* @return Whether the constraint field is set.
*/
boolean hasConstraint();
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
* @return The constraint.
*/
io.substrait.proto.RelCommon.Hint.RuntimeConstraint getConstraint();
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
io.substrait.proto.RelCommon.Hint.RuntimeConstraintOrBuilder getConstraintOrBuilder();
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @return The alias.
*/
java.lang.String getAlias();
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @return The bytes for alias.
*/
com.google.protobuf.ByteString
getAliasBytes();
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @return A list containing the outputNames.
*/
java.util.List
getOutputNamesList();
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @return The count of outputNames.
*/
int getOutputNamesCount();
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param index The index of the element to return.
* @return The outputNames at the given index.
*/
java.lang.String getOutputNames(int index);
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param index The index of the value to return.
* @return The bytes of the outputNames at the given index.
*/
com.google.protobuf.ByteString
getOutputNamesBytes(int index);
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
boolean hasAdvancedExtension();
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
io.substrait.proto.AdvancedExtension getAdvancedExtension();
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder();
}
/**
*
* Changes to the operation that can influence efficiency/performance but
* should not impact correctness.
*
*
* Protobuf type {@code substrait.RelCommon.Hint}
*/
public static final class Hint extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:substrait.RelCommon.Hint)
HintOrBuilder {
private static final long serialVersionUID = 0L;
// Use Hint.newBuilder() to construct.
private Hint(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Hint() {
alias_ = "";
outputNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Hint();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Hint.class, io.substrait.proto.RelCommon.Hint.Builder.class);
}
public interface StatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:substrait.RelCommon.Hint.Stats)
com.google.protobuf.MessageOrBuilder {
/**
* double row_count = 1;
* @return The rowCount.
*/
double getRowCount();
/**
* double record_size = 2;
* @return The recordSize.
*/
double getRecordSize();
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
boolean hasAdvancedExtension();
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
io.substrait.proto.AdvancedExtension getAdvancedExtension();
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder();
}
/**
*
* The statistics related to a hint (physical properties of records)
*
*
* Protobuf type {@code substrait.RelCommon.Hint.Stats}
*/
public static final class Stats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:substrait.RelCommon.Hint.Stats)
StatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use Stats.newBuilder() to construct.
private Stats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Stats() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Stats();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_Stats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_Stats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Hint.Stats.class, io.substrait.proto.RelCommon.Hint.Stats.Builder.class);
}
private int bitField0_;
public static final int ROW_COUNT_FIELD_NUMBER = 1;
private double rowCount_ = 0D;
/**
* double row_count = 1;
* @return The rowCount.
*/
@java.lang.Override
public double getRowCount() {
return rowCount_;
}
public static final int RECORD_SIZE_FIELD_NUMBER = 2;
private double recordSize_ = 0D;
/**
* double record_size = 2;
* @return The recordSize.
*/
@java.lang.Override
public double getRecordSize() {
return recordSize_;
}
public static final int ADVANCED_EXTENSION_FIELD_NUMBER = 10;
private io.substrait.proto.AdvancedExtension advancedExtension_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
@java.lang.Override
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (java.lang.Double.doubleToRawLongBits(rowCount_) != 0) {
output.writeDouble(1, rowCount_);
}
if (java.lang.Double.doubleToRawLongBits(recordSize_) != 0) {
output.writeDouble(2, recordSize_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(10, getAdvancedExtension());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (java.lang.Double.doubleToRawLongBits(rowCount_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1, rowCount_);
}
if (java.lang.Double.doubleToRawLongBits(recordSize_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, recordSize_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getAdvancedExtension());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.substrait.proto.RelCommon.Hint.Stats)) {
return super.equals(obj);
}
io.substrait.proto.RelCommon.Hint.Stats other = (io.substrait.proto.RelCommon.Hint.Stats) obj;
if (java.lang.Double.doubleToLongBits(getRowCount())
!= java.lang.Double.doubleToLongBits(
other.getRowCount())) return false;
if (java.lang.Double.doubleToLongBits(getRecordSize())
!= java.lang.Double.doubleToLongBits(
other.getRecordSize())) return false;
if (hasAdvancedExtension() != other.hasAdvancedExtension()) return false;
if (hasAdvancedExtension()) {
if (!getAdvancedExtension()
.equals(other.getAdvancedExtension())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ROW_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getRowCount()));
hash = (37 * hash) + RECORD_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getRecordSize()));
if (hasAdvancedExtension()) {
hash = (37 * hash) + ADVANCED_EXTENSION_FIELD_NUMBER;
hash = (53 * hash) + getAdvancedExtension().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint.Stats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.substrait.proto.RelCommon.Hint.Stats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The statistics related to a hint (physical properties of records)
*
*
* Protobuf type {@code substrait.RelCommon.Hint.Stats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:substrait.RelCommon.Hint.Stats)
io.substrait.proto.RelCommon.Hint.StatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_Stats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_Stats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Hint.Stats.class, io.substrait.proto.RelCommon.Hint.Stats.Builder.class);
}
// Construct using io.substrait.proto.RelCommon.Hint.Stats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdvancedExtensionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
rowCount_ = 0D;
recordSize_ = 0D;
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_Stats_descriptor;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.Stats getDefaultInstanceForType() {
return io.substrait.proto.RelCommon.Hint.Stats.getDefaultInstance();
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.Stats build() {
io.substrait.proto.RelCommon.Hint.Stats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.Stats buildPartial() {
io.substrait.proto.RelCommon.Hint.Stats result = new io.substrait.proto.RelCommon.Hint.Stats(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(io.substrait.proto.RelCommon.Hint.Stats result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.rowCount_ = rowCount_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.recordSize_ = recordSize_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.advancedExtension_ = advancedExtensionBuilder_ == null
? advancedExtension_
: advancedExtensionBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.substrait.proto.RelCommon.Hint.Stats) {
return mergeFrom((io.substrait.proto.RelCommon.Hint.Stats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.substrait.proto.RelCommon.Hint.Stats other) {
if (other == io.substrait.proto.RelCommon.Hint.Stats.getDefaultInstance()) return this;
if (other.getRowCount() != 0D) {
setRowCount(other.getRowCount());
}
if (other.getRecordSize() != 0D) {
setRecordSize(other.getRecordSize());
}
if (other.hasAdvancedExtension()) {
mergeAdvancedExtension(other.getAdvancedExtension());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 9: {
rowCount_ = input.readDouble();
bitField0_ |= 0x00000001;
break;
} // case 9
case 17: {
recordSize_ = input.readDouble();
bitField0_ |= 0x00000002;
break;
} // case 17
case 82: {
input.readMessage(
getAdvancedExtensionFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 82
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private double rowCount_ ;
/**
* double row_count = 1;
* @return The rowCount.
*/
@java.lang.Override
public double getRowCount() {
return rowCount_;
}
/**
* double row_count = 1;
* @param value The rowCount to set.
* @return This builder for chaining.
*/
public Builder setRowCount(double value) {
rowCount_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* double row_count = 1;
* @return This builder for chaining.
*/
public Builder clearRowCount() {
bitField0_ = (bitField0_ & ~0x00000001);
rowCount_ = 0D;
onChanged();
return this;
}
private double recordSize_ ;
/**
* double record_size = 2;
* @return The recordSize.
*/
@java.lang.Override
public double getRecordSize() {
return recordSize_;
}
/**
* double record_size = 2;
* @param value The recordSize to set.
* @return This builder for chaining.
*/
public Builder setRecordSize(double value) {
recordSize_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* double record_size = 2;
* @return This builder for chaining.
*/
public Builder clearRecordSize() {
bitField0_ = (bitField0_ & ~0x00000002);
recordSize_ = 0D;
onChanged();
return this;
}
private io.substrait.proto.AdvancedExtension advancedExtension_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder> advancedExtensionBuilder_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
if (advancedExtensionBuilder_ == null) {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
} else {
return advancedExtensionBuilder_.getMessage();
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder setAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
advancedExtension_ = value;
} else {
advancedExtensionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder setAdvancedExtension(
io.substrait.proto.AdvancedExtension.Builder builderForValue) {
if (advancedExtensionBuilder_ == null) {
advancedExtension_ = builderForValue.build();
} else {
advancedExtensionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder mergeAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
advancedExtension_ != null &&
advancedExtension_ != io.substrait.proto.AdvancedExtension.getDefaultInstance()) {
getAdvancedExtensionBuilder().mergeFrom(value);
} else {
advancedExtension_ = value;
}
} else {
advancedExtensionBuilder_.mergeFrom(value);
}
if (advancedExtension_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder clearAdvancedExtension() {
bitField0_ = (bitField0_ & ~0x00000004);
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public io.substrait.proto.AdvancedExtension.Builder getAdvancedExtensionBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getAdvancedExtensionFieldBuilder().getBuilder();
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
if (advancedExtensionBuilder_ != null) {
return advancedExtensionBuilder_.getMessageOrBuilder();
} else {
return advancedExtension_ == null ?
io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>
getAdvancedExtensionFieldBuilder() {
if (advancedExtensionBuilder_ == null) {
advancedExtensionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>(
getAdvancedExtension(),
getParentForChildren(),
isClean());
advancedExtension_ = null;
}
return advancedExtensionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:substrait.RelCommon.Hint.Stats)
}
// @@protoc_insertion_point(class_scope:substrait.RelCommon.Hint.Stats)
private static final io.substrait.proto.RelCommon.Hint.Stats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.substrait.proto.RelCommon.Hint.Stats();
}
public static io.substrait.proto.RelCommon.Hint.Stats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Stats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.Stats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RuntimeConstraintOrBuilder extends
// @@protoc_insertion_point(interface_extends:substrait.RelCommon.Hint.RuntimeConstraint)
com.google.protobuf.MessageOrBuilder {
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
boolean hasAdvancedExtension();
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
io.substrait.proto.AdvancedExtension getAdvancedExtension();
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder();
}
/**
*
* TODO: nodes, cpu threads/%, memory, iops, etc.
*
*
* Protobuf type {@code substrait.RelCommon.Hint.RuntimeConstraint}
*/
public static final class RuntimeConstraint extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:substrait.RelCommon.Hint.RuntimeConstraint)
RuntimeConstraintOrBuilder {
private static final long serialVersionUID = 0L;
// Use RuntimeConstraint.newBuilder() to construct.
private RuntimeConstraint(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RuntimeConstraint() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RuntimeConstraint();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_RuntimeConstraint_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_RuntimeConstraint_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Hint.RuntimeConstraint.class, io.substrait.proto.RelCommon.Hint.RuntimeConstraint.Builder.class);
}
private int bitField0_;
public static final int ADVANCED_EXTENSION_FIELD_NUMBER = 10;
private io.substrait.proto.AdvancedExtension advancedExtension_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
@java.lang.Override
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(10, getAdvancedExtension());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getAdvancedExtension());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.substrait.proto.RelCommon.Hint.RuntimeConstraint)) {
return super.equals(obj);
}
io.substrait.proto.RelCommon.Hint.RuntimeConstraint other = (io.substrait.proto.RelCommon.Hint.RuntimeConstraint) obj;
if (hasAdvancedExtension() != other.hasAdvancedExtension()) return false;
if (hasAdvancedExtension()) {
if (!getAdvancedExtension()
.equals(other.getAdvancedExtension())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAdvancedExtension()) {
hash = (37 * hash) + ADVANCED_EXTENSION_FIELD_NUMBER;
hash = (53 * hash) + getAdvancedExtension().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.substrait.proto.RelCommon.Hint.RuntimeConstraint prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TODO: nodes, cpu threads/%, memory, iops, etc.
*
*
* Protobuf type {@code substrait.RelCommon.Hint.RuntimeConstraint}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:substrait.RelCommon.Hint.RuntimeConstraint)
io.substrait.proto.RelCommon.Hint.RuntimeConstraintOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_RuntimeConstraint_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_RuntimeConstraint_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Hint.RuntimeConstraint.class, io.substrait.proto.RelCommon.Hint.RuntimeConstraint.Builder.class);
}
// Construct using io.substrait.proto.RelCommon.Hint.RuntimeConstraint.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAdvancedExtensionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_RuntimeConstraint_descriptor;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.RuntimeConstraint getDefaultInstanceForType() {
return io.substrait.proto.RelCommon.Hint.RuntimeConstraint.getDefaultInstance();
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.RuntimeConstraint build() {
io.substrait.proto.RelCommon.Hint.RuntimeConstraint result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.RuntimeConstraint buildPartial() {
io.substrait.proto.RelCommon.Hint.RuntimeConstraint result = new io.substrait.proto.RelCommon.Hint.RuntimeConstraint(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(io.substrait.proto.RelCommon.Hint.RuntimeConstraint result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.advancedExtension_ = advancedExtensionBuilder_ == null
? advancedExtension_
: advancedExtensionBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.substrait.proto.RelCommon.Hint.RuntimeConstraint) {
return mergeFrom((io.substrait.proto.RelCommon.Hint.RuntimeConstraint)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.substrait.proto.RelCommon.Hint.RuntimeConstraint other) {
if (other == io.substrait.proto.RelCommon.Hint.RuntimeConstraint.getDefaultInstance()) return this;
if (other.hasAdvancedExtension()) {
mergeAdvancedExtension(other.getAdvancedExtension());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 82: {
input.readMessage(
getAdvancedExtensionFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 82
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private io.substrait.proto.AdvancedExtension advancedExtension_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder> advancedExtensionBuilder_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
if (advancedExtensionBuilder_ == null) {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
} else {
return advancedExtensionBuilder_.getMessage();
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder setAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
advancedExtension_ = value;
} else {
advancedExtensionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder setAdvancedExtension(
io.substrait.proto.AdvancedExtension.Builder builderForValue) {
if (advancedExtensionBuilder_ == null) {
advancedExtension_ = builderForValue.build();
} else {
advancedExtensionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder mergeAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
advancedExtension_ != null &&
advancedExtension_ != io.substrait.proto.AdvancedExtension.getDefaultInstance()) {
getAdvancedExtensionBuilder().mergeFrom(value);
} else {
advancedExtension_ = value;
}
} else {
advancedExtensionBuilder_.mergeFrom(value);
}
if (advancedExtension_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder clearAdvancedExtension() {
bitField0_ = (bitField0_ & ~0x00000001);
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public io.substrait.proto.AdvancedExtension.Builder getAdvancedExtensionBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getAdvancedExtensionFieldBuilder().getBuilder();
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
if (advancedExtensionBuilder_ != null) {
return advancedExtensionBuilder_.getMessageOrBuilder();
} else {
return advancedExtension_ == null ?
io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>
getAdvancedExtensionFieldBuilder() {
if (advancedExtensionBuilder_ == null) {
advancedExtensionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>(
getAdvancedExtension(),
getParentForChildren(),
isClean());
advancedExtension_ = null;
}
return advancedExtensionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:substrait.RelCommon.Hint.RuntimeConstraint)
}
// @@protoc_insertion_point(class_scope:substrait.RelCommon.Hint.RuntimeConstraint)
private static final io.substrait.proto.RelCommon.Hint.RuntimeConstraint DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.substrait.proto.RelCommon.Hint.RuntimeConstraint();
}
public static io.substrait.proto.RelCommon.Hint.RuntimeConstraint getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RuntimeConstraint parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.RuntimeConstraint getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int STATS_FIELD_NUMBER = 1;
private io.substrait.proto.RelCommon.Hint.Stats stats_;
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
* @return Whether the stats field is set.
*/
@java.lang.Override
public boolean hasStats() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
* @return The stats.
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.Stats getStats() {
return stats_ == null ? io.substrait.proto.RelCommon.Hint.Stats.getDefaultInstance() : stats_;
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.StatsOrBuilder getStatsOrBuilder() {
return stats_ == null ? io.substrait.proto.RelCommon.Hint.Stats.getDefaultInstance() : stats_;
}
public static final int CONSTRAINT_FIELD_NUMBER = 2;
private io.substrait.proto.RelCommon.Hint.RuntimeConstraint constraint_;
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
* @return Whether the constraint field is set.
*/
@java.lang.Override
public boolean hasConstraint() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
* @return The constraint.
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.RuntimeConstraint getConstraint() {
return constraint_ == null ? io.substrait.proto.RelCommon.Hint.RuntimeConstraint.getDefaultInstance() : constraint_;
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Hint.RuntimeConstraintOrBuilder getConstraintOrBuilder() {
return constraint_ == null ? io.substrait.proto.RelCommon.Hint.RuntimeConstraint.getDefaultInstance() : constraint_;
}
public static final int ALIAS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object alias_ = "";
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @return The alias.
*/
@java.lang.Override
public java.lang.String getAlias() {
java.lang.Object ref = alias_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alias_ = s;
return s;
}
}
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @return The bytes for alias.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAliasBytes() {
java.lang.Object ref = alias_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alias_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OUTPUT_NAMES_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList outputNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @return A list containing the outputNames.
*/
public com.google.protobuf.ProtocolStringList
getOutputNamesList() {
return outputNames_;
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @return The count of outputNames.
*/
public int getOutputNamesCount() {
return outputNames_.size();
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param index The index of the element to return.
* @return The outputNames at the given index.
*/
public java.lang.String getOutputNames(int index) {
return outputNames_.get(index);
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param index The index of the value to return.
* @return The bytes of the outputNames at the given index.
*/
public com.google.protobuf.ByteString
getOutputNamesBytes(int index) {
return outputNames_.getByteString(index);
}
public static final int ADVANCED_EXTENSION_FIELD_NUMBER = 10;
private io.substrait.proto.AdvancedExtension advancedExtension_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
@java.lang.Override
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getStats());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getConstraint());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(alias_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, alias_);
}
for (int i = 0; i < outputNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, outputNames_.getRaw(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(10, getAdvancedExtension());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStats());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getConstraint());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(alias_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, alias_);
}
{
int dataSize = 0;
for (int i = 0; i < outputNames_.size(); i++) {
dataSize += computeStringSizeNoTag(outputNames_.getRaw(i));
}
size += dataSize;
size += 1 * getOutputNamesList().size();
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getAdvancedExtension());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.substrait.proto.RelCommon.Hint)) {
return super.equals(obj);
}
io.substrait.proto.RelCommon.Hint other = (io.substrait.proto.RelCommon.Hint) obj;
if (hasStats() != other.hasStats()) return false;
if (hasStats()) {
if (!getStats()
.equals(other.getStats())) return false;
}
if (hasConstraint() != other.hasConstraint()) return false;
if (hasConstraint()) {
if (!getConstraint()
.equals(other.getConstraint())) return false;
}
if (!getAlias()
.equals(other.getAlias())) return false;
if (!getOutputNamesList()
.equals(other.getOutputNamesList())) return false;
if (hasAdvancedExtension() != other.hasAdvancedExtension()) return false;
if (hasAdvancedExtension()) {
if (!getAdvancedExtension()
.equals(other.getAdvancedExtension())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStats()) {
hash = (37 * hash) + STATS_FIELD_NUMBER;
hash = (53 * hash) + getStats().hashCode();
}
if (hasConstraint()) {
hash = (37 * hash) + CONSTRAINT_FIELD_NUMBER;
hash = (53 * hash) + getConstraint().hashCode();
}
hash = (37 * hash) + ALIAS_FIELD_NUMBER;
hash = (53 * hash) + getAlias().hashCode();
if (getOutputNamesCount() > 0) {
hash = (37 * hash) + OUTPUT_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getOutputNamesList().hashCode();
}
if (hasAdvancedExtension()) {
hash = (37 * hash) + ADVANCED_EXTENSION_FIELD_NUMBER;
hash = (53 * hash) + getAdvancedExtension().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon.Hint parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.substrait.proto.RelCommon.Hint prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Changes to the operation that can influence efficiency/performance but
* should not impact correctness.
*
*
* Protobuf type {@code substrait.RelCommon.Hint}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:substrait.RelCommon.Hint)
io.substrait.proto.RelCommon.HintOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.Hint.class, io.substrait.proto.RelCommon.Hint.Builder.class);
}
// Construct using io.substrait.proto.RelCommon.Hint.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStatsFieldBuilder();
getConstraintFieldBuilder();
getAdvancedExtensionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
stats_ = null;
if (statsBuilder_ != null) {
statsBuilder_.dispose();
statsBuilder_ = null;
}
constraint_ = null;
if (constraintBuilder_ != null) {
constraintBuilder_.dispose();
constraintBuilder_ = null;
}
alias_ = "";
outputNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_Hint_descriptor;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint getDefaultInstanceForType() {
return io.substrait.proto.RelCommon.Hint.getDefaultInstance();
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint build() {
io.substrait.proto.RelCommon.Hint result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint buildPartial() {
io.substrait.proto.RelCommon.Hint result = new io.substrait.proto.RelCommon.Hint(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(io.substrait.proto.RelCommon.Hint result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.stats_ = statsBuilder_ == null
? stats_
: statsBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.constraint_ = constraintBuilder_ == null
? constraint_
: constraintBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.alias_ = alias_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
outputNames_.makeImmutable();
result.outputNames_ = outputNames_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.advancedExtension_ = advancedExtensionBuilder_ == null
? advancedExtension_
: advancedExtensionBuilder_.build();
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.substrait.proto.RelCommon.Hint) {
return mergeFrom((io.substrait.proto.RelCommon.Hint)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.substrait.proto.RelCommon.Hint other) {
if (other == io.substrait.proto.RelCommon.Hint.getDefaultInstance()) return this;
if (other.hasStats()) {
mergeStats(other.getStats());
}
if (other.hasConstraint()) {
mergeConstraint(other.getConstraint());
}
if (!other.getAlias().isEmpty()) {
alias_ = other.alias_;
bitField0_ |= 0x00000004;
onChanged();
}
if (!other.outputNames_.isEmpty()) {
if (outputNames_.isEmpty()) {
outputNames_ = other.outputNames_;
bitField0_ |= 0x00000008;
} else {
ensureOutputNamesIsMutable();
outputNames_.addAll(other.outputNames_);
}
onChanged();
}
if (other.hasAdvancedExtension()) {
mergeAdvancedExtension(other.getAdvancedExtension());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getStatsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getConstraintFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
alias_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
java.lang.String s = input.readStringRequireUtf8();
ensureOutputNamesIsMutable();
outputNames_.add(s);
break;
} // case 34
case 82: {
input.readMessage(
getAdvancedExtensionFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 82
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private io.substrait.proto.RelCommon.Hint.Stats stats_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint.Stats, io.substrait.proto.RelCommon.Hint.Stats.Builder, io.substrait.proto.RelCommon.Hint.StatsOrBuilder> statsBuilder_;
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
* @return Whether the stats field is set.
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
* @return The stats.
*/
public io.substrait.proto.RelCommon.Hint.Stats getStats() {
if (statsBuilder_ == null) {
return stats_ == null ? io.substrait.proto.RelCommon.Hint.Stats.getDefaultInstance() : stats_;
} else {
return statsBuilder_.getMessage();
}
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
public Builder setStats(io.substrait.proto.RelCommon.Hint.Stats value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stats_ = value;
} else {
statsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
public Builder setStats(
io.substrait.proto.RelCommon.Hint.Stats.Builder builderForValue) {
if (statsBuilder_ == null) {
stats_ = builderForValue.build();
} else {
statsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
public Builder mergeStats(io.substrait.proto.RelCommon.Hint.Stats value) {
if (statsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
stats_ != null &&
stats_ != io.substrait.proto.RelCommon.Hint.Stats.getDefaultInstance()) {
getStatsBuilder().mergeFrom(value);
} else {
stats_ = value;
}
} else {
statsBuilder_.mergeFrom(value);
}
if (stats_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
public Builder clearStats() {
bitField0_ = (bitField0_ & ~0x00000001);
stats_ = null;
if (statsBuilder_ != null) {
statsBuilder_.dispose();
statsBuilder_ = null;
}
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
public io.substrait.proto.RelCommon.Hint.Stats.Builder getStatsBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getStatsFieldBuilder().getBuilder();
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
public io.substrait.proto.RelCommon.Hint.StatsOrBuilder getStatsOrBuilder() {
if (statsBuilder_ != null) {
return statsBuilder_.getMessageOrBuilder();
} else {
return stats_ == null ?
io.substrait.proto.RelCommon.Hint.Stats.getDefaultInstance() : stats_;
}
}
/**
* .substrait.RelCommon.Hint.Stats stats = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint.Stats, io.substrait.proto.RelCommon.Hint.Stats.Builder, io.substrait.proto.RelCommon.Hint.StatsOrBuilder>
getStatsFieldBuilder() {
if (statsBuilder_ == null) {
statsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint.Stats, io.substrait.proto.RelCommon.Hint.Stats.Builder, io.substrait.proto.RelCommon.Hint.StatsOrBuilder>(
getStats(),
getParentForChildren(),
isClean());
stats_ = null;
}
return statsBuilder_;
}
private io.substrait.proto.RelCommon.Hint.RuntimeConstraint constraint_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint.RuntimeConstraint, io.substrait.proto.RelCommon.Hint.RuntimeConstraint.Builder, io.substrait.proto.RelCommon.Hint.RuntimeConstraintOrBuilder> constraintBuilder_;
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
* @return Whether the constraint field is set.
*/
public boolean hasConstraint() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
* @return The constraint.
*/
public io.substrait.proto.RelCommon.Hint.RuntimeConstraint getConstraint() {
if (constraintBuilder_ == null) {
return constraint_ == null ? io.substrait.proto.RelCommon.Hint.RuntimeConstraint.getDefaultInstance() : constraint_;
} else {
return constraintBuilder_.getMessage();
}
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
public Builder setConstraint(io.substrait.proto.RelCommon.Hint.RuntimeConstraint value) {
if (constraintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
constraint_ = value;
} else {
constraintBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
public Builder setConstraint(
io.substrait.proto.RelCommon.Hint.RuntimeConstraint.Builder builderForValue) {
if (constraintBuilder_ == null) {
constraint_ = builderForValue.build();
} else {
constraintBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
public Builder mergeConstraint(io.substrait.proto.RelCommon.Hint.RuntimeConstraint value) {
if (constraintBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
constraint_ != null &&
constraint_ != io.substrait.proto.RelCommon.Hint.RuntimeConstraint.getDefaultInstance()) {
getConstraintBuilder().mergeFrom(value);
} else {
constraint_ = value;
}
} else {
constraintBuilder_.mergeFrom(value);
}
if (constraint_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
public Builder clearConstraint() {
bitField0_ = (bitField0_ & ~0x00000002);
constraint_ = null;
if (constraintBuilder_ != null) {
constraintBuilder_.dispose();
constraintBuilder_ = null;
}
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
public io.substrait.proto.RelCommon.Hint.RuntimeConstraint.Builder getConstraintBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getConstraintFieldBuilder().getBuilder();
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
public io.substrait.proto.RelCommon.Hint.RuntimeConstraintOrBuilder getConstraintOrBuilder() {
if (constraintBuilder_ != null) {
return constraintBuilder_.getMessageOrBuilder();
} else {
return constraint_ == null ?
io.substrait.proto.RelCommon.Hint.RuntimeConstraint.getDefaultInstance() : constraint_;
}
}
/**
* .substrait.RelCommon.Hint.RuntimeConstraint constraint = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint.RuntimeConstraint, io.substrait.proto.RelCommon.Hint.RuntimeConstraint.Builder, io.substrait.proto.RelCommon.Hint.RuntimeConstraintOrBuilder>
getConstraintFieldBuilder() {
if (constraintBuilder_ == null) {
constraintBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint.RuntimeConstraint, io.substrait.proto.RelCommon.Hint.RuntimeConstraint.Builder, io.substrait.proto.RelCommon.Hint.RuntimeConstraintOrBuilder>(
getConstraint(),
getParentForChildren(),
isClean());
constraint_ = null;
}
return constraintBuilder_;
}
private java.lang.Object alias_ = "";
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @return The alias.
*/
public java.lang.String getAlias() {
java.lang.Object ref = alias_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
alias_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @return The bytes for alias.
*/
public com.google.protobuf.ByteString
getAliasBytes() {
java.lang.Object ref = alias_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
alias_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @param value The alias to set.
* @return This builder for chaining.
*/
public Builder setAlias(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
alias_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @return This builder for chaining.
*/
public Builder clearAlias() {
alias_ = getDefaultInstance().getAlias();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Name (alias) for this relation. Can be used for e.g. qualifying the relation (see e.g.
* Spark's SubqueryAlias), or debugging.
*
*
* string alias = 3;
* @param value The bytes for alias to set.
* @return This builder for chaining.
*/
public Builder setAliasBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
alias_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList outputNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureOutputNamesIsMutable() {
if (!outputNames_.isModifiable()) {
outputNames_ = new com.google.protobuf.LazyStringArrayList(outputNames_);
}
bitField0_ |= 0x00000008;
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @return A list containing the outputNames.
*/
public com.google.protobuf.ProtocolStringList
getOutputNamesList() {
outputNames_.makeImmutable();
return outputNames_;
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @return The count of outputNames.
*/
public int getOutputNamesCount() {
return outputNames_.size();
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param index The index of the element to return.
* @return The outputNames at the given index.
*/
public java.lang.String getOutputNames(int index) {
return outputNames_.get(index);
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param index The index of the value to return.
* @return The bytes of the outputNames at the given index.
*/
public com.google.protobuf.ByteString
getOutputNamesBytes(int index) {
return outputNames_.getByteString(index);
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param index The index to set the value at.
* @param value The outputNames to set.
* @return This builder for chaining.
*/
public Builder setOutputNames(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureOutputNamesIsMutable();
outputNames_.set(index, value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param value The outputNames to add.
* @return This builder for chaining.
*/
public Builder addOutputNames(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureOutputNamesIsMutable();
outputNames_.add(value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param values The outputNames to add.
* @return This builder for chaining.
*/
public Builder addAllOutputNames(
java.lang.Iterable values) {
ensureOutputNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputNames_);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @return This builder for chaining.
*/
public Builder clearOutputNames() {
outputNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);;
onChanged();
return this;
}
/**
*
* Assigns alternative output field names for any relation. Equivalent to the names field
* in RelRoot but applies to the output of the relation this RelCommon is attached to.
*
*
* repeated string output_names = 4;
* @param value The bytes of the outputNames to add.
* @return This builder for chaining.
*/
public Builder addOutputNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureOutputNamesIsMutable();
outputNames_.add(value);
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private io.substrait.proto.AdvancedExtension advancedExtension_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder> advancedExtensionBuilder_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return Whether the advancedExtension field is set.
*/
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
* @return The advancedExtension.
*/
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
if (advancedExtensionBuilder_ == null) {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
} else {
return advancedExtensionBuilder_.getMessage();
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder setAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
advancedExtension_ = value;
} else {
advancedExtensionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder setAdvancedExtension(
io.substrait.proto.AdvancedExtension.Builder builderForValue) {
if (advancedExtensionBuilder_ == null) {
advancedExtension_ = builderForValue.build();
} else {
advancedExtensionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder mergeAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
advancedExtension_ != null &&
advancedExtension_ != io.substrait.proto.AdvancedExtension.getDefaultInstance()) {
getAdvancedExtensionBuilder().mergeFrom(value);
} else {
advancedExtension_ = value;
}
} else {
advancedExtensionBuilder_.mergeFrom(value);
}
if (advancedExtension_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public Builder clearAdvancedExtension() {
bitField0_ = (bitField0_ & ~0x00000010);
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public io.substrait.proto.AdvancedExtension.Builder getAdvancedExtensionBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getAdvancedExtensionFieldBuilder().getBuilder();
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
if (advancedExtensionBuilder_ != null) {
return advancedExtensionBuilder_.getMessageOrBuilder();
} else {
return advancedExtension_ == null ?
io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>
getAdvancedExtensionFieldBuilder() {
if (advancedExtensionBuilder_ == null) {
advancedExtensionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>(
getAdvancedExtension(),
getParentForChildren(),
isClean());
advancedExtension_ = null;
}
return advancedExtensionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:substrait.RelCommon.Hint)
}
// @@protoc_insertion_point(class_scope:substrait.RelCommon.Hint)
private static final io.substrait.proto.RelCommon.Hint DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.substrait.proto.RelCommon.Hint();
}
public static io.substrait.proto.RelCommon.Hint getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Hint parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.substrait.proto.RelCommon.Hint getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int emitKindCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object emitKind_;
public enum EmitKindCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
DIRECT(1),
EMIT(2),
EMITKIND_NOT_SET(0);
private final int value;
private EmitKindCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EmitKindCase valueOf(int value) {
return forNumber(value);
}
public static EmitKindCase forNumber(int value) {
switch (value) {
case 1: return DIRECT;
case 2: return EMIT;
case 0: return EMITKIND_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public EmitKindCase
getEmitKindCase() {
return EmitKindCase.forNumber(
emitKindCase_);
}
public static final int DIRECT_FIELD_NUMBER = 1;
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
* @return Whether the direct field is set.
*/
@java.lang.Override
public boolean hasDirect() {
return emitKindCase_ == 1;
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
* @return The direct.
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Direct getDirect() {
if (emitKindCase_ == 1) {
return (io.substrait.proto.RelCommon.Direct) emitKind_;
}
return io.substrait.proto.RelCommon.Direct.getDefaultInstance();
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
@java.lang.Override
public io.substrait.proto.RelCommon.DirectOrBuilder getDirectOrBuilder() {
if (emitKindCase_ == 1) {
return (io.substrait.proto.RelCommon.Direct) emitKind_;
}
return io.substrait.proto.RelCommon.Direct.getDefaultInstance();
}
public static final int EMIT_FIELD_NUMBER = 2;
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
* @return Whether the emit field is set.
*/
@java.lang.Override
public boolean hasEmit() {
return emitKindCase_ == 2;
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
* @return The emit.
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Emit getEmit() {
if (emitKindCase_ == 2) {
return (io.substrait.proto.RelCommon.Emit) emitKind_;
}
return io.substrait.proto.RelCommon.Emit.getDefaultInstance();
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
@java.lang.Override
public io.substrait.proto.RelCommon.EmitOrBuilder getEmitOrBuilder() {
if (emitKindCase_ == 2) {
return (io.substrait.proto.RelCommon.Emit) emitKind_;
}
return io.substrait.proto.RelCommon.Emit.getDefaultInstance();
}
public static final int HINT_FIELD_NUMBER = 3;
private io.substrait.proto.RelCommon.Hint hint_;
/**
* .substrait.RelCommon.Hint hint = 3;
* @return Whether the hint field is set.
*/
@java.lang.Override
public boolean hasHint() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .substrait.RelCommon.Hint hint = 3;
* @return The hint.
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Hint getHint() {
return hint_ == null ? io.substrait.proto.RelCommon.Hint.getDefaultInstance() : hint_;
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
@java.lang.Override
public io.substrait.proto.RelCommon.HintOrBuilder getHintOrBuilder() {
return hint_ == null ? io.substrait.proto.RelCommon.Hint.getDefaultInstance() : hint_;
}
public static final int ADVANCED_EXTENSION_FIELD_NUMBER = 4;
private io.substrait.proto.AdvancedExtension advancedExtension_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
* @return Whether the advancedExtension field is set.
*/
@java.lang.Override
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
* @return The advancedExtension.
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
@java.lang.Override
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (emitKindCase_ == 1) {
output.writeMessage(1, (io.substrait.proto.RelCommon.Direct) emitKind_);
}
if (emitKindCase_ == 2) {
output.writeMessage(2, (io.substrait.proto.RelCommon.Emit) emitKind_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(3, getHint());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(4, getAdvancedExtension());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (emitKindCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (io.substrait.proto.RelCommon.Direct) emitKind_);
}
if (emitKindCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (io.substrait.proto.RelCommon.Emit) emitKind_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHint());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getAdvancedExtension());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.substrait.proto.RelCommon)) {
return super.equals(obj);
}
io.substrait.proto.RelCommon other = (io.substrait.proto.RelCommon) obj;
if (hasHint() != other.hasHint()) return false;
if (hasHint()) {
if (!getHint()
.equals(other.getHint())) return false;
}
if (hasAdvancedExtension() != other.hasAdvancedExtension()) return false;
if (hasAdvancedExtension()) {
if (!getAdvancedExtension()
.equals(other.getAdvancedExtension())) return false;
}
if (!getEmitKindCase().equals(other.getEmitKindCase())) return false;
switch (emitKindCase_) {
case 1:
if (!getDirect()
.equals(other.getDirect())) return false;
break;
case 2:
if (!getEmit()
.equals(other.getEmit())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHint()) {
hash = (37 * hash) + HINT_FIELD_NUMBER;
hash = (53 * hash) + getHint().hashCode();
}
if (hasAdvancedExtension()) {
hash = (37 * hash) + ADVANCED_EXTENSION_FIELD_NUMBER;
hash = (53 * hash) + getAdvancedExtension().hashCode();
}
switch (emitKindCase_) {
case 1:
hash = (37 * hash) + DIRECT_FIELD_NUMBER;
hash = (53 * hash) + getDirect().hashCode();
break;
case 2:
hash = (37 * hash) + EMIT_FIELD_NUMBER;
hash = (53 * hash) + getEmit().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.substrait.proto.RelCommon parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.substrait.proto.RelCommon parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.substrait.proto.RelCommon parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.substrait.proto.RelCommon parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.substrait.proto.RelCommon parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.substrait.proto.RelCommon prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Common fields for all relational operators
*
*
* Protobuf type {@code substrait.RelCommon}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:substrait.RelCommon)
io.substrait.proto.RelCommonOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.substrait.proto.RelCommon.class, io.substrait.proto.RelCommon.Builder.class);
}
// Construct using io.substrait.proto.RelCommon.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getHintFieldBuilder();
getAdvancedExtensionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (directBuilder_ != null) {
directBuilder_.clear();
}
if (emitBuilder_ != null) {
emitBuilder_.clear();
}
hint_ = null;
if (hintBuilder_ != null) {
hintBuilder_.dispose();
hintBuilder_ = null;
}
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
emitKindCase_ = 0;
emitKind_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.substrait.proto.Algebra.internal_static_substrait_RelCommon_descriptor;
}
@java.lang.Override
public io.substrait.proto.RelCommon getDefaultInstanceForType() {
return io.substrait.proto.RelCommon.getDefaultInstance();
}
@java.lang.Override
public io.substrait.proto.RelCommon build() {
io.substrait.proto.RelCommon result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.substrait.proto.RelCommon buildPartial() {
io.substrait.proto.RelCommon result = new io.substrait.proto.RelCommon(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(io.substrait.proto.RelCommon result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.hint_ = hintBuilder_ == null
? hint_
: hintBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.advancedExtension_ = advancedExtensionBuilder_ == null
? advancedExtension_
: advancedExtensionBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(io.substrait.proto.RelCommon result) {
result.emitKindCase_ = emitKindCase_;
result.emitKind_ = this.emitKind_;
if (emitKindCase_ == 1 &&
directBuilder_ != null) {
result.emitKind_ = directBuilder_.build();
}
if (emitKindCase_ == 2 &&
emitBuilder_ != null) {
result.emitKind_ = emitBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.substrait.proto.RelCommon) {
return mergeFrom((io.substrait.proto.RelCommon)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.substrait.proto.RelCommon other) {
if (other == io.substrait.proto.RelCommon.getDefaultInstance()) return this;
if (other.hasHint()) {
mergeHint(other.getHint());
}
if (other.hasAdvancedExtension()) {
mergeAdvancedExtension(other.getAdvancedExtension());
}
switch (other.getEmitKindCase()) {
case DIRECT: {
mergeDirect(other.getDirect());
break;
}
case EMIT: {
mergeEmit(other.getEmit());
break;
}
case EMITKIND_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getDirectFieldBuilder().getBuilder(),
extensionRegistry);
emitKindCase_ = 1;
break;
} // case 10
case 18: {
input.readMessage(
getEmitFieldBuilder().getBuilder(),
extensionRegistry);
emitKindCase_ = 2;
break;
} // case 18
case 26: {
input.readMessage(
getHintFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getAdvancedExtensionFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int emitKindCase_ = 0;
private java.lang.Object emitKind_;
public EmitKindCase
getEmitKindCase() {
return EmitKindCase.forNumber(
emitKindCase_);
}
public Builder clearEmitKind() {
emitKindCase_ = 0;
emitKind_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Direct, io.substrait.proto.RelCommon.Direct.Builder, io.substrait.proto.RelCommon.DirectOrBuilder> directBuilder_;
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
* @return Whether the direct field is set.
*/
@java.lang.Override
public boolean hasDirect() {
return emitKindCase_ == 1;
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
* @return The direct.
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Direct getDirect() {
if (directBuilder_ == null) {
if (emitKindCase_ == 1) {
return (io.substrait.proto.RelCommon.Direct) emitKind_;
}
return io.substrait.proto.RelCommon.Direct.getDefaultInstance();
} else {
if (emitKindCase_ == 1) {
return directBuilder_.getMessage();
}
return io.substrait.proto.RelCommon.Direct.getDefaultInstance();
}
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
public Builder setDirect(io.substrait.proto.RelCommon.Direct value) {
if (directBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
emitKind_ = value;
onChanged();
} else {
directBuilder_.setMessage(value);
}
emitKindCase_ = 1;
return this;
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
public Builder setDirect(
io.substrait.proto.RelCommon.Direct.Builder builderForValue) {
if (directBuilder_ == null) {
emitKind_ = builderForValue.build();
onChanged();
} else {
directBuilder_.setMessage(builderForValue.build());
}
emitKindCase_ = 1;
return this;
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
public Builder mergeDirect(io.substrait.proto.RelCommon.Direct value) {
if (directBuilder_ == null) {
if (emitKindCase_ == 1 &&
emitKind_ != io.substrait.proto.RelCommon.Direct.getDefaultInstance()) {
emitKind_ = io.substrait.proto.RelCommon.Direct.newBuilder((io.substrait.proto.RelCommon.Direct) emitKind_)
.mergeFrom(value).buildPartial();
} else {
emitKind_ = value;
}
onChanged();
} else {
if (emitKindCase_ == 1) {
directBuilder_.mergeFrom(value);
} else {
directBuilder_.setMessage(value);
}
}
emitKindCase_ = 1;
return this;
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
public Builder clearDirect() {
if (directBuilder_ == null) {
if (emitKindCase_ == 1) {
emitKindCase_ = 0;
emitKind_ = null;
onChanged();
}
} else {
if (emitKindCase_ == 1) {
emitKindCase_ = 0;
emitKind_ = null;
}
directBuilder_.clear();
}
return this;
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
public io.substrait.proto.RelCommon.Direct.Builder getDirectBuilder() {
return getDirectFieldBuilder().getBuilder();
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
@java.lang.Override
public io.substrait.proto.RelCommon.DirectOrBuilder getDirectOrBuilder() {
if ((emitKindCase_ == 1) && (directBuilder_ != null)) {
return directBuilder_.getMessageOrBuilder();
} else {
if (emitKindCase_ == 1) {
return (io.substrait.proto.RelCommon.Direct) emitKind_;
}
return io.substrait.proto.RelCommon.Direct.getDefaultInstance();
}
}
/**
*
* The underlying relation is output as is (no reordering or projection of columns)
*
*
* .substrait.RelCommon.Direct direct = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Direct, io.substrait.proto.RelCommon.Direct.Builder, io.substrait.proto.RelCommon.DirectOrBuilder>
getDirectFieldBuilder() {
if (directBuilder_ == null) {
if (!(emitKindCase_ == 1)) {
emitKind_ = io.substrait.proto.RelCommon.Direct.getDefaultInstance();
}
directBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Direct, io.substrait.proto.RelCommon.Direct.Builder, io.substrait.proto.RelCommon.DirectOrBuilder>(
(io.substrait.proto.RelCommon.Direct) emitKind_,
getParentForChildren(),
isClean());
emitKind_ = null;
}
emitKindCase_ = 1;
onChanged();
return directBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Emit, io.substrait.proto.RelCommon.Emit.Builder, io.substrait.proto.RelCommon.EmitOrBuilder> emitBuilder_;
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
* @return Whether the emit field is set.
*/
@java.lang.Override
public boolean hasEmit() {
return emitKindCase_ == 2;
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
* @return The emit.
*/
@java.lang.Override
public io.substrait.proto.RelCommon.Emit getEmit() {
if (emitBuilder_ == null) {
if (emitKindCase_ == 2) {
return (io.substrait.proto.RelCommon.Emit) emitKind_;
}
return io.substrait.proto.RelCommon.Emit.getDefaultInstance();
} else {
if (emitKindCase_ == 2) {
return emitBuilder_.getMessage();
}
return io.substrait.proto.RelCommon.Emit.getDefaultInstance();
}
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
public Builder setEmit(io.substrait.proto.RelCommon.Emit value) {
if (emitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
emitKind_ = value;
onChanged();
} else {
emitBuilder_.setMessage(value);
}
emitKindCase_ = 2;
return this;
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
public Builder setEmit(
io.substrait.proto.RelCommon.Emit.Builder builderForValue) {
if (emitBuilder_ == null) {
emitKind_ = builderForValue.build();
onChanged();
} else {
emitBuilder_.setMessage(builderForValue.build());
}
emitKindCase_ = 2;
return this;
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
public Builder mergeEmit(io.substrait.proto.RelCommon.Emit value) {
if (emitBuilder_ == null) {
if (emitKindCase_ == 2 &&
emitKind_ != io.substrait.proto.RelCommon.Emit.getDefaultInstance()) {
emitKind_ = io.substrait.proto.RelCommon.Emit.newBuilder((io.substrait.proto.RelCommon.Emit) emitKind_)
.mergeFrom(value).buildPartial();
} else {
emitKind_ = value;
}
onChanged();
} else {
if (emitKindCase_ == 2) {
emitBuilder_.mergeFrom(value);
} else {
emitBuilder_.setMessage(value);
}
}
emitKindCase_ = 2;
return this;
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
public Builder clearEmit() {
if (emitBuilder_ == null) {
if (emitKindCase_ == 2) {
emitKindCase_ = 0;
emitKind_ = null;
onChanged();
}
} else {
if (emitKindCase_ == 2) {
emitKindCase_ = 0;
emitKind_ = null;
}
emitBuilder_.clear();
}
return this;
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
public io.substrait.proto.RelCommon.Emit.Builder getEmitBuilder() {
return getEmitFieldBuilder().getBuilder();
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
@java.lang.Override
public io.substrait.proto.RelCommon.EmitOrBuilder getEmitOrBuilder() {
if ((emitKindCase_ == 2) && (emitBuilder_ != null)) {
return emitBuilder_.getMessageOrBuilder();
} else {
if (emitKindCase_ == 2) {
return (io.substrait.proto.RelCommon.Emit) emitKind_;
}
return io.substrait.proto.RelCommon.Emit.getDefaultInstance();
}
}
/**
*
* Allows to control for order and inclusion of fields
*
*
* .substrait.RelCommon.Emit emit = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Emit, io.substrait.proto.RelCommon.Emit.Builder, io.substrait.proto.RelCommon.EmitOrBuilder>
getEmitFieldBuilder() {
if (emitBuilder_ == null) {
if (!(emitKindCase_ == 2)) {
emitKind_ = io.substrait.proto.RelCommon.Emit.getDefaultInstance();
}
emitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Emit, io.substrait.proto.RelCommon.Emit.Builder, io.substrait.proto.RelCommon.EmitOrBuilder>(
(io.substrait.proto.RelCommon.Emit) emitKind_,
getParentForChildren(),
isClean());
emitKind_ = null;
}
emitKindCase_ = 2;
onChanged();
return emitBuilder_;
}
private io.substrait.proto.RelCommon.Hint hint_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint, io.substrait.proto.RelCommon.Hint.Builder, io.substrait.proto.RelCommon.HintOrBuilder> hintBuilder_;
/**
* .substrait.RelCommon.Hint hint = 3;
* @return Whether the hint field is set.
*/
public boolean hasHint() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .substrait.RelCommon.Hint hint = 3;
* @return The hint.
*/
public io.substrait.proto.RelCommon.Hint getHint() {
if (hintBuilder_ == null) {
return hint_ == null ? io.substrait.proto.RelCommon.Hint.getDefaultInstance() : hint_;
} else {
return hintBuilder_.getMessage();
}
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
public Builder setHint(io.substrait.proto.RelCommon.Hint value) {
if (hintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
hint_ = value;
} else {
hintBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
public Builder setHint(
io.substrait.proto.RelCommon.Hint.Builder builderForValue) {
if (hintBuilder_ == null) {
hint_ = builderForValue.build();
} else {
hintBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
public Builder mergeHint(io.substrait.proto.RelCommon.Hint value) {
if (hintBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
hint_ != null &&
hint_ != io.substrait.proto.RelCommon.Hint.getDefaultInstance()) {
getHintBuilder().mergeFrom(value);
} else {
hint_ = value;
}
} else {
hintBuilder_.mergeFrom(value);
}
if (hint_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
public Builder clearHint() {
bitField0_ = (bitField0_ & ~0x00000004);
hint_ = null;
if (hintBuilder_ != null) {
hintBuilder_.dispose();
hintBuilder_ = null;
}
onChanged();
return this;
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
public io.substrait.proto.RelCommon.Hint.Builder getHintBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getHintFieldBuilder().getBuilder();
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
public io.substrait.proto.RelCommon.HintOrBuilder getHintOrBuilder() {
if (hintBuilder_ != null) {
return hintBuilder_.getMessageOrBuilder();
} else {
return hint_ == null ?
io.substrait.proto.RelCommon.Hint.getDefaultInstance() : hint_;
}
}
/**
* .substrait.RelCommon.Hint hint = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint, io.substrait.proto.RelCommon.Hint.Builder, io.substrait.proto.RelCommon.HintOrBuilder>
getHintFieldBuilder() {
if (hintBuilder_ == null) {
hintBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.RelCommon.Hint, io.substrait.proto.RelCommon.Hint.Builder, io.substrait.proto.RelCommon.HintOrBuilder>(
getHint(),
getParentForChildren(),
isClean());
hint_ = null;
}
return hintBuilder_;
}
private io.substrait.proto.AdvancedExtension advancedExtension_;
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder> advancedExtensionBuilder_;
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
* @return Whether the advancedExtension field is set.
*/
public boolean hasAdvancedExtension() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
* @return The advancedExtension.
*/
public io.substrait.proto.AdvancedExtension getAdvancedExtension() {
if (advancedExtensionBuilder_ == null) {
return advancedExtension_ == null ? io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
} else {
return advancedExtensionBuilder_.getMessage();
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
public Builder setAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
advancedExtension_ = value;
} else {
advancedExtensionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
public Builder setAdvancedExtension(
io.substrait.proto.AdvancedExtension.Builder builderForValue) {
if (advancedExtensionBuilder_ == null) {
advancedExtension_ = builderForValue.build();
} else {
advancedExtensionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
public Builder mergeAdvancedExtension(io.substrait.proto.AdvancedExtension value) {
if (advancedExtensionBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
advancedExtension_ != null &&
advancedExtension_ != io.substrait.proto.AdvancedExtension.getDefaultInstance()) {
getAdvancedExtensionBuilder().mergeFrom(value);
} else {
advancedExtension_ = value;
}
} else {
advancedExtensionBuilder_.mergeFrom(value);
}
if (advancedExtension_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
public Builder clearAdvancedExtension() {
bitField0_ = (bitField0_ & ~0x00000008);
advancedExtension_ = null;
if (advancedExtensionBuilder_ != null) {
advancedExtensionBuilder_.dispose();
advancedExtensionBuilder_ = null;
}
onChanged();
return this;
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
public io.substrait.proto.AdvancedExtension.Builder getAdvancedExtensionBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getAdvancedExtensionFieldBuilder().getBuilder();
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
public io.substrait.proto.AdvancedExtensionOrBuilder getAdvancedExtensionOrBuilder() {
if (advancedExtensionBuilder_ != null) {
return advancedExtensionBuilder_.getMessageOrBuilder();
} else {
return advancedExtension_ == null ?
io.substrait.proto.AdvancedExtension.getDefaultInstance() : advancedExtension_;
}
}
/**
* .substrait.extensions.AdvancedExtension advanced_extension = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>
getAdvancedExtensionFieldBuilder() {
if (advancedExtensionBuilder_ == null) {
advancedExtensionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.substrait.proto.AdvancedExtension, io.substrait.proto.AdvancedExtension.Builder, io.substrait.proto.AdvancedExtensionOrBuilder>(
getAdvancedExtension(),
getParentForChildren(),
isClean());
advancedExtension_ = null;
}
return advancedExtensionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:substrait.RelCommon)
}
// @@protoc_insertion_point(class_scope:substrait.RelCommon)
private static final io.substrait.proto.RelCommon DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.substrait.proto.RelCommon();
}
public static io.substrait.proto.RelCommon getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RelCommon parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.substrait.proto.RelCommon getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy