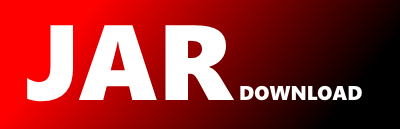
io.substrait.proto.AggregationPhase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Create a well-defined, cross-language specification for data compute operations
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: substrait/algebra.proto
// Protobuf Java Version: 3.25.5
package io.substrait.proto;
/**
*
* Describes which part of an aggregation or window function to perform within
* the context of distributed algorithms.
*
*
* Protobuf enum {@code substrait.AggregationPhase}
*/
public enum AggregationPhase
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Implies `INTERMEDIATE_TO_RESULT`.
*
*
* AGGREGATION_PHASE_UNSPECIFIED = 0;
*/
AGGREGATION_PHASE_UNSPECIFIED(0),
/**
*
* Specifies that the function should be run only up to the point of
* generating an intermediate value, to be further aggregated later using
* INTERMEDIATE_TO_INTERMEDIATE or INTERMEDIATE_TO_RESULT.
*
*
* AGGREGATION_PHASE_INITIAL_TO_INTERMEDIATE = 1;
*/
AGGREGATION_PHASE_INITIAL_TO_INTERMEDIATE(1),
/**
*
* Specifies that the inputs of the aggregate or window function are the
* intermediate values of the function, and that the output should also be
* an intermediate value, to be further aggregated later using
* INTERMEDIATE_TO_INTERMEDIATE or INTERMEDIATE_TO_RESULT.
*
*
* AGGREGATION_PHASE_INTERMEDIATE_TO_INTERMEDIATE = 2;
*/
AGGREGATION_PHASE_INTERMEDIATE_TO_INTERMEDIATE(2),
/**
*
* A complete invocation: the function should aggregate the given set of
* inputs to yield a single return value. This style must be used for
* aggregate or window functions that are not decomposable.
*
*
* AGGREGATION_PHASE_INITIAL_TO_RESULT = 3;
*/
AGGREGATION_PHASE_INITIAL_TO_RESULT(3),
/**
*
* Specifies that the inputs of the aggregate or window function are the
* intermediate values of the function, generated previously using
* INITIAL_TO_INTERMEDIATE and possibly INTERMEDIATE_TO_INTERMEDIATE calls.
* This call should combine the intermediate values to yield the final
* return value.
*
*
* AGGREGATION_PHASE_INTERMEDIATE_TO_RESULT = 4;
*/
AGGREGATION_PHASE_INTERMEDIATE_TO_RESULT(4),
UNRECOGNIZED(-1),
;
/**
*
* Implies `INTERMEDIATE_TO_RESULT`.
*
*
* AGGREGATION_PHASE_UNSPECIFIED = 0;
*/
public static final int AGGREGATION_PHASE_UNSPECIFIED_VALUE = 0;
/**
*
* Specifies that the function should be run only up to the point of
* generating an intermediate value, to be further aggregated later using
* INTERMEDIATE_TO_INTERMEDIATE or INTERMEDIATE_TO_RESULT.
*
*
* AGGREGATION_PHASE_INITIAL_TO_INTERMEDIATE = 1;
*/
public static final int AGGREGATION_PHASE_INITIAL_TO_INTERMEDIATE_VALUE = 1;
/**
*
* Specifies that the inputs of the aggregate or window function are the
* intermediate values of the function, and that the output should also be
* an intermediate value, to be further aggregated later using
* INTERMEDIATE_TO_INTERMEDIATE or INTERMEDIATE_TO_RESULT.
*
*
* AGGREGATION_PHASE_INTERMEDIATE_TO_INTERMEDIATE = 2;
*/
public static final int AGGREGATION_PHASE_INTERMEDIATE_TO_INTERMEDIATE_VALUE = 2;
/**
*
* A complete invocation: the function should aggregate the given set of
* inputs to yield a single return value. This style must be used for
* aggregate or window functions that are not decomposable.
*
*
* AGGREGATION_PHASE_INITIAL_TO_RESULT = 3;
*/
public static final int AGGREGATION_PHASE_INITIAL_TO_RESULT_VALUE = 3;
/**
*
* Specifies that the inputs of the aggregate or window function are the
* intermediate values of the function, generated previously using
* INITIAL_TO_INTERMEDIATE and possibly INTERMEDIATE_TO_INTERMEDIATE calls.
* This call should combine the intermediate values to yield the final
* return value.
*
*
* AGGREGATION_PHASE_INTERMEDIATE_TO_RESULT = 4;
*/
public static final int AGGREGATION_PHASE_INTERMEDIATE_TO_RESULT_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static AggregationPhase valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static AggregationPhase forNumber(int value) {
switch (value) {
case 0: return AGGREGATION_PHASE_UNSPECIFIED;
case 1: return AGGREGATION_PHASE_INITIAL_TO_INTERMEDIATE;
case 2: return AGGREGATION_PHASE_INTERMEDIATE_TO_INTERMEDIATE;
case 3: return AGGREGATION_PHASE_INITIAL_TO_RESULT;
case 4: return AGGREGATION_PHASE_INTERMEDIATE_TO_RESULT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
AggregationPhase> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public AggregationPhase findValueByNumber(int number) {
return AggregationPhase.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.substrait.proto.Algebra.getDescriptor().getEnumTypes().get(0);
}
private static final AggregationPhase[] VALUES = values();
public static AggregationPhase valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private AggregationPhase(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:substrait.AggregationPhase)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy