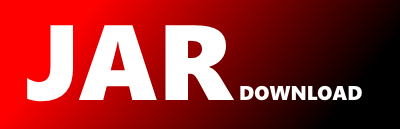
io.substrait.util.Util Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Create a well-defined, cross-language specification for data compute operations
package io.substrait.util;
import java.util.function.Supplier;
public class Util {
static final org.slf4j.Logger logger = org.slf4j.LoggerFactory.getLogger(Util.class);
public static Supplier memoize(Supplier supplier) {
return new Memoizer(supplier);
}
private static class Memoizer implements Supplier {
private boolean retrieved;
private T value;
private Supplier delegate;
public Memoizer(Supplier delegate) {
this.delegate = delegate;
}
@Override
public T get() {
if (!retrieved) {
value = delegate.get();
retrieved = true;
}
return value;
}
}
public static class IntRange {
private final int startInclusive;
private final int endExclusive;
public static IntRange of(int startInclusive, int endExclusive) {
return new IntRange(startInclusive, endExclusive);
}
private IntRange(int startInclusive, int endExclusive) {
this.startInclusive = startInclusive;
this.endExclusive = endExclusive;
}
public int getStartInclusive() {
return startInclusive;
}
public int getEndExclusive() {
return endExclusive;
}
public boolean within(int val) {
return val >= startInclusive && val < endExclusive;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy