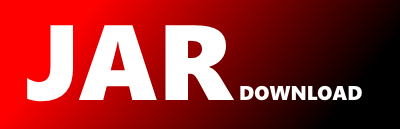
io.sundr.dsl.internal.graph.NodeContext Maven / Gradle / Ivy
/*
* Copyright 2016 The original authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.sundr.dsl.internal.graph;
import io.sundr.codegen.model.TypeDef;
import io.sundr.dsl.internal.utils.GraphUtils;
import io.sundr.dsl.internal.utils.TypeDefUtils;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
public class NodeContext {
private final TypeDef item;
private final List path;
private final Set visited;
private final Set all;
public static class Builder {
private TypeDef item;
private List path = new ArrayList();
private Set visited = new HashSet();
private Set all = new LinkedHashSet();
public Builder withItem(TypeDef item) {
this.item = item;
return this;
}
public Builder withPath(List path) {
this.path = path;
return this;
}
public Builder addToPath(TypeDef clazz) {
List newPath = new ArrayList(path);
newPath.add(clazz);
this.path = newPath;
return this;
}
public Builder withVisited(Set visited) {
this.visited = visited;
return this;
}
public Builder addToVisited(TypeDef clazz) {
Set newVisited = new HashSet(visited);
newVisited.add(clazz);
this.visited = newVisited;
return this;
}
public Builder addToVisited(Collection clazzes) {
Set newVisited = new HashSet(visited);
newVisited.addAll(clazzes);
this.visited = newVisited;
return this;
}
public Builder withAll(Set all) {
this.all = all;
return this;
}
public NodeContext build() {
return new NodeContext(item, path, visited, all);
}
}
public static Builder builder() {
return new Builder();
}
public NodeContext(TypeDef item, List path, Set visited, Set all) {
this.item = item;
this.path = path != null ? Collections.unmodifiableList(path) : Collections.emptyList();
this.visited = visited != null ? Collections.unmodifiableSet(visited) : Collections.emptySet();
this.all = all != null ? Collections.unmodifiableSet(all) : Collections.emptySet();
}
public TypeDef getItem() {
return item;
}
public List getPath() {
return path;
}
public List getPathTypes() {
List pathTypes = new ArrayList();
for (TypeDef c : path) {
pathTypes.add(c);
}
return pathTypes;
}
public Set getVisited() {
return visited;
}
public Set getVisitedTypes() {
Set visitedTypes = new HashSet();
for (TypeDef c : visited) {
visitedTypes.add(c);
}
return visitedTypes;
}
public Set getAll() {
return all;
}
public List getActiveScopes() {
return GraphUtils.getScopes(getPathTypes());
}
public Builder but() {
return builder().withItem(item)
.withPath(new ArrayList(path))
.withVisited(new HashSet(visited))
.withAll(new HashSet(all));
}
public Builder contextOfChild(TypeDef child) {
if (TypeDefUtils.isBeginScope(child)) {
return builder().withItem(child).withAll(new HashSet(all));
}
return but().withItem(child)
.withPath(new ArrayList(path))
.addToPath(item)
.withVisited(new HashSet(visited))
.addToVisited(item)
.withAll(new LinkedHashSet(all));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy