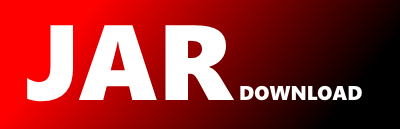
io.sundr.codegen.converters.VariableElementToJavaProperty Maven / Gradle / Ivy
/*
* Copyright 2015 The original authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.sundr.codegen.converters;
import io.sundr.Function;
import io.sundr.codegen.model.JavaProperty;
import io.sundr.codegen.model.JavaPropertyBuilder;
import io.sundr.codegen.model.JavaType;
import io.sundr.codegen.model.JavaTypeBuilder;
import javax.lang.model.element.AnnotationMirror;
import javax.lang.model.element.VariableElement;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.Set;
public class VariableElementToJavaProperty implements Function {
private final Function toType;
public VariableElementToJavaProperty(Function toType) {
this.toType = toType;
}
@Override
public JavaProperty apply(final VariableElement variableElement) {
String name = variableElement.getSimpleName().toString();
boolean isArray = variableElement.asType().toString().endsWith("[]");
JavaType type = new JavaTypeBuilder(toType.apply(variableElement.asType().toString())).withArray(isArray).build();
Set annotations = new LinkedHashSet();
for (AnnotationMirror annotationMirror : variableElement.getAnnotationMirrors()) {
JavaType annotationType = toType.apply(annotationMirror.getAnnotationType().toString());
annotations.add(annotationType);
}
return new JavaPropertyBuilder()
.withName(name)
.withType(type)
.withArray(isArray)
.withAnnotations(annotations)
.withModifiers(variableElement.getModifiers())
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy