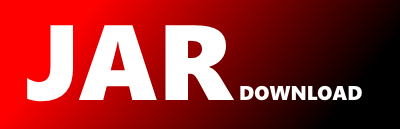
io.sundr.codegen.model.MethodFluent Maven / Gradle / Ivy
/*
* Copyright 2019 The original authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.sundr.codegen.model;
import io.sundr.builder.Nested;
import io.sundr.builder.Predicate;
import java.util.Collection;
import java.util.List;
public interface MethodFluent> extends ModifierSupportFluent{
public A addToComments(int index, String item);
public A setToComments(int index, String item);
public A addToComments(String... items);
public A addAllToComments(Collection items);
public A removeFromComments(String... items);
public A removeAllFromComments(Collection items);
public List getComments();
public String getComment(int index);
public String getFirstComment();
public String getLastComment();
public String getMatchingComment(Predicate predicate);
public Boolean hasMatchingComment(Predicate predicate);
public A withComments(List comments);
public A withComments(String... comments);
public Boolean hasComments();
public A addNewComment(String arg1);
public A addNewComment(StringBuilder arg1);
public A addNewComment(StringBuffer arg1);
public A addToAnnotations(int index, AnnotationRef item);
public A setToAnnotations(int index, AnnotationRef item);
public A addToAnnotations(AnnotationRef... items);
public A addAllToAnnotations(Collection items);
public A removeFromAnnotations(AnnotationRef... items);
public A removeAllFromAnnotations(Collection items);
/**
* This method has been deprecated, please use method buildAnnotations instead.
* @return The buildable object.
*/
@Deprecated public List getAnnotations();
public List buildAnnotations();
public AnnotationRef buildAnnotation(int index);
public AnnotationRef buildFirstAnnotation();
public AnnotationRef buildLastAnnotation();
public AnnotationRef buildMatchingAnnotation(Predicate predicate);
public Boolean hasMatchingAnnotation(Predicate predicate);
public A withAnnotations(List annotations);
public A withAnnotations(AnnotationRef... annotations);
public Boolean hasAnnotations();
public MethodFluent.AnnotationsNested addNewAnnotation();
public MethodFluent.AnnotationsNested addNewAnnotationLike(AnnotationRef item);
public MethodFluent.AnnotationsNested setNewAnnotationLike(int index, AnnotationRef item);
public MethodFluent.AnnotationsNested editAnnotation(int index);
public MethodFluent.AnnotationsNested editFirstAnnotation();
public MethodFluent.AnnotationsNested editLastAnnotation();
public MethodFluent.AnnotationsNested editMatchingAnnotation(Predicate predicate);
public A addToParameters(int index, TypeParamDef item);
public A setToParameters(int index, TypeParamDef item);
public A addToParameters(TypeParamDef... items);
public A addAllToParameters(Collection items);
public A removeFromParameters(TypeParamDef... items);
public A removeAllFromParameters(Collection items);
/**
* This method has been deprecated, please use method buildParameters instead.
* @return The buildable object.
*/
@Deprecated public List getParameters();
public List buildParameters();
public TypeParamDef buildParameter(int index);
public TypeParamDef buildFirstParameter();
public TypeParamDef buildLastParameter();
public TypeParamDef buildMatchingParameter(Predicate predicate);
public Boolean hasMatchingParameter(Predicate predicate);
public A withParameters(List parameters);
public A withParameters(TypeParamDef... parameters);
public Boolean hasParameters();
public MethodFluent.ParametersNested addNewParameter();
public MethodFluent.ParametersNested addNewParameterLike(TypeParamDef item);
public MethodFluent.ParametersNested setNewParameterLike(int index, TypeParamDef item);
public MethodFluent.ParametersNested editParameter(int index);
public MethodFluent.ParametersNested editFirstParameter();
public MethodFluent.ParametersNested editLastParameter();
public MethodFluent.ParametersNested editMatchingParameter(Predicate predicate);
public String getName();
public A withName(String name);
public Boolean hasName();
public A withNewName(String arg1);
public A withNewName(StringBuilder arg1);
public A withNewName(StringBuffer arg1);
/**
* This method has been deprecated, please use method buildReturnType instead.
* @return The buildable object.
*/
@Deprecated public TypeRef getReturnType();
public TypeRef buildReturnType();
public A withReturnType(TypeRef returnType);
public Boolean hasReturnType();
public A withPrimitiveRefReturnType(PrimitiveRef primitiveRefReturnType);
public MethodFluent.PrimitiveRefReturnTypeNested withNewPrimitiveRefReturnType();
public MethodFluent.PrimitiveRefReturnTypeNested withNewPrimitiveRefReturnTypeLike(PrimitiveRef item);
public A withVoidRefReturnType(VoidRef voidRefReturnType);
public MethodFluent.VoidRefReturnTypeNested withNewVoidRefReturnType();
public MethodFluent.VoidRefReturnTypeNested withNewVoidRefReturnTypeLike(VoidRef item);
public A withWildcardRefReturnType(WildcardRef wildcardRefReturnType);
public MethodFluent.WildcardRefReturnTypeNested withNewWildcardRefReturnType();
public MethodFluent.WildcardRefReturnTypeNested withNewWildcardRefReturnTypeLike(WildcardRef item);
public A withClassRefReturnType(ClassRef classRefReturnType);
public MethodFluent.ClassRefReturnTypeNested withNewClassRefReturnType();
public MethodFluent.ClassRefReturnTypeNested withNewClassRefReturnTypeLike(ClassRef item);
public A withTypeParamRefReturnType(TypeParamRef typeParamRefReturnType);
public MethodFluent.TypeParamRefReturnTypeNested withNewTypeParamRefReturnType();
public MethodFluent.TypeParamRefReturnTypeNested withNewTypeParamRefReturnTypeLike(TypeParamRef item);
public A addToArguments(int index, Property item);
public A setToArguments(int index, Property item);
public A addToArguments(Property... items);
public A addAllToArguments(Collection items);
public A removeFromArguments(Property... items);
public A removeAllFromArguments(Collection items);
/**
* This method has been deprecated, please use method buildArguments instead.
* @return The buildable object.
*/
@Deprecated public List getArguments();
public List buildArguments();
public Property buildArgument(int index);
public Property buildFirstArgument();
public Property buildLastArgument();
public Property buildMatchingArgument(Predicate predicate);
public Boolean hasMatchingArgument(Predicate predicate);
public A withArguments(List arguments);
public A withArguments(Property... arguments);
public Boolean hasArguments();
public MethodFluent.ArgumentsNested addNewArgument();
public MethodFluent.ArgumentsNested addNewArgumentLike(Property item);
public MethodFluent.ArgumentsNested setNewArgumentLike(int index, Property item);
public MethodFluent.ArgumentsNested editArgument(int index);
public MethodFluent.ArgumentsNested editFirstArgument();
public MethodFluent.ArgumentsNested editLastArgument();
public MethodFluent.ArgumentsNested editMatchingArgument(Predicate predicate);
public boolean isVarArgPreferred();
public A withVarArgPreferred(boolean varArgPreferred);
public Boolean hasVarArgPreferred();
public A addToExceptions(int index, ClassRef item);
public A setToExceptions(int index, ClassRef item);
public A addToExceptions(ClassRef... items);
public A addAllToExceptions(Collection items);
public A removeFromExceptions(ClassRef... items);
public A removeAllFromExceptions(Collection items);
/**
* This method has been deprecated, please use method buildExceptions instead.
* @return The buildable object.
*/
@Deprecated public List getExceptions();
public List buildExceptions();
public ClassRef buildException(int index);
public ClassRef buildFirstException();
public ClassRef buildLastException();
public ClassRef buildMatchingException(Predicate predicate);
public Boolean hasMatchingException(Predicate predicate);
public A withExceptions(List exceptions);
public A withExceptions(ClassRef... exceptions);
public Boolean hasExceptions();
public MethodFluent.ExceptionsNested addNewException();
public MethodFluent.ExceptionsNested addNewExceptionLike(ClassRef item);
public MethodFluent.ExceptionsNested setNewExceptionLike(int index, ClassRef item);
public MethodFluent.ExceptionsNested editException(int index);
public MethodFluent.ExceptionsNested editFirstException();
public MethodFluent.ExceptionsNested editLastException();
public MethodFluent.ExceptionsNested editMatchingException(Predicate predicate);
/**
* This method has been deprecated, please use method buildBlock instead.
* @return The buildable object.
*/
@Deprecated public Block getBlock();
public Block buildBlock();
public A withBlock(Block block);
public Boolean hasBlock();
public MethodFluent.BlockNested withNewBlock();
public MethodFluent.BlockNested withNewBlockLike(Block item);
public MethodFluent.BlockNested editBlock();
public MethodFluent.BlockNested editOrNewBlock();
public MethodFluent.BlockNested editOrNewBlockLike(Block item);
public interface AnnotationsNested extends Nested,AnnotationRefFluent>{
public N and(); public N endAnnotation();
}
public interface ParametersNested extends Nested,TypeParamDefFluent>{
public N and(); public N endParameter();
}
public interface PrimitiveRefReturnTypeNested extends Nested,PrimitiveRefFluent>{
public N and(); public N endPrimitiveRefReturnType();
}
public interface VoidRefReturnTypeNested extends Nested,VoidRefFluent>{
public N and(); public N endVoidRefReturnType();
}
public interface WildcardRefReturnTypeNested extends Nested,WildcardRefFluent>{
public N and(); public N endWildcardRefReturnType();
}
public interface ClassRefReturnTypeNested extends Nested,ClassRefFluent>{
public N and(); public N endClassRefReturnType();
}
public interface TypeParamRefReturnTypeNested extends Nested,TypeParamRefFluent>{
public N and(); public N endTypeParamRefReturnType();
}
public interface ArgumentsNested extends Nested,PropertyFluent>{
public N and(); public N endArgument();
}
public interface ExceptionsNested extends Nested,ClassRefFluent>{
public N and(); public N endException();
}
public interface BlockNested extends Nested,BlockFluent>{
public N and(); public N endBlock();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy