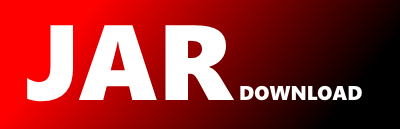
io.sundr.codegen.model.MethodFluentImpl Maven / Gradle / Ivy
/*
* Copyright 2019 The original authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.sundr.codegen.model;
import io.sundr.builder.Nested;
import io.sundr.builder.Predicate;
import io.sundr.builder.VisitableBuilder;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
public class MethodFluentImpl> extends ModifierSupportFluentImpl implements MethodFluent{
private List comments = new ArrayList();
private List annotations = new ArrayList();
private List parameters = new ArrayList();
private String name;
private VisitableBuilder extends TypeRef,?> returnType;
private List arguments = new ArrayList();
private boolean varArgPreferred;
private List exceptions = new ArrayList();
private BlockBuilder block;
public MethodFluentImpl(){
}
public MethodFluentImpl(Method instance){
this.withComments(instance.getComments());
this.withAnnotations(instance.getAnnotations());
this.withParameters(instance.getParameters());
this.withName(instance.getName());
this.withReturnType(instance.getReturnType());
this.withArguments(instance.getArguments());
this.withVarArgPreferred(instance.isVarArgPreferred());
this.withExceptions(instance.getExceptions());
this.withBlock(instance.getBlock());
this.withModifiers(instance.getModifiers());
this.withAttributes(instance.getAttributes());
}
public A addToComments(int index,String item){
if (this.comments == null) {this.comments = new ArrayList();}
this.comments.add(index, item);
return (A)this;
}
public A setToComments(int index,String item){
if (this.comments == null) {this.comments = new ArrayList();}
this.comments.set(index, item); return (A)this;
}
public A addToComments(String... items){
if (this.comments == null) {this.comments = new ArrayList();}
for (String item : items) {this.comments.add(item);} return (A)this;
}
public A addAllToComments(Collection items){
if (this.comments == null) {this.comments = new ArrayList();}
for (String item : items) {this.comments.add(item);} return (A)this;
}
public A removeFromComments(String... items){
for (String item : items) {if (this.comments!= null){ this.comments.remove(item);}} return (A)this;
}
public A removeAllFromComments(Collection items){
for (String item : items) {if (this.comments!= null){ this.comments.remove(item);}} return (A)this;
}
public List getComments(){
return this.comments;
}
public String getComment(int index){
return this.comments.get(index);
}
public String getFirstComment(){
return this.comments.get(0);
}
public String getLastComment(){
return this.comments.get(comments.size() - 1);
}
public String getMatchingComment(Predicate predicate){
for (String item: comments) { if(predicate.apply(item)){return item;} } return null;
}
public Boolean hasMatchingComment(Predicate predicate){
for (String item: comments) { if(predicate.apply(item)){return true;} } return false;
}
public A withComments(List comments){
if (this.comments != null) { _visitables.get("comments").removeAll(this.comments);}
if (comments != null) {this.comments = new ArrayList(); for (String item : comments){this.addToComments(item);}} else { this.comments = new ArrayList();} return (A) this;
}
public A withComments(String... comments){
if (this.comments != null) {this.comments.clear();}
if (comments != null) {for (String item :comments){ this.addToComments(item);}} return (A) this;
}
public Boolean hasComments(){
return comments != null && !comments.isEmpty();
}
public A addNewComment(String arg1){
return (A)addToComments(new String(arg1));
}
public A addNewComment(StringBuilder arg1){
return (A)addToComments(new String(arg1));
}
public A addNewComment(StringBuffer arg1){
return (A)addToComments(new String(arg1));
}
public A addToAnnotations(int index,AnnotationRef item){
if (this.annotations == null) {this.annotations = new ArrayList();}
AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").add(index >= 0 ? index : _visitables.get("annotations").size(), builder);this.annotations.add(index >= 0 ? index : annotations.size(), builder); return (A)this;
}
public A setToAnnotations(int index,AnnotationRef item){
if (this.annotations == null) {this.annotations = new ArrayList();}
AnnotationRefBuilder builder = new AnnotationRefBuilder(item);
if (index < 0 || index >= _visitables.get("annotations").size()) { _visitables.get("annotations").add(builder); } else { _visitables.get("annotations").set(index, builder);}
if (index < 0 || index >= annotations.size()) { annotations.add(builder); } else { annotations.set(index, builder);}
return (A)this;
}
public A addToAnnotations(AnnotationRef... items){
if (this.annotations == null) {this.annotations = new ArrayList();}
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").add(builder);this.annotations.add(builder);} return (A)this;
}
public A addAllToAnnotations(Collection items){
if (this.annotations == null) {this.annotations = new ArrayList();}
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").add(builder);this.annotations.add(builder);} return (A)this;
}
public A removeFromAnnotations(AnnotationRef... items){
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").remove(builder);if (this.annotations != null) {this.annotations.remove(builder);}} return (A)this;
}
public A removeAllFromAnnotations(Collection items){
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").remove(builder);if (this.annotations != null) {this.annotations.remove(builder);}} return (A)this;
}
/**
* This method has been deprecated, please use method buildAnnotations instead.
* @return The buildable object.
*/
@Deprecated public List getAnnotations(){
return build(annotations);
}
public List buildAnnotations(){
return build(annotations);
}
public AnnotationRef buildAnnotation(int index){
return this.annotations.get(index).build();
}
public AnnotationRef buildFirstAnnotation(){
return this.annotations.get(0).build();
}
public AnnotationRef buildLastAnnotation(){
return this.annotations.get(annotations.size() - 1).build();
}
public AnnotationRef buildMatchingAnnotation(Predicate predicate){
for (AnnotationRefBuilder item: annotations) { if(predicate.apply(item)){return item.build();} } return null;
}
public Boolean hasMatchingAnnotation(Predicate predicate){
for (AnnotationRefBuilder item: annotations) { if(predicate.apply(item)){return true;} } return false;
}
public A withAnnotations(List annotations){
if (this.annotations != null) { _visitables.get("annotations").removeAll(this.annotations);}
if (annotations != null) {this.annotations = new ArrayList(); for (AnnotationRef item : annotations){this.addToAnnotations(item);}} else { this.annotations = new ArrayList();} return (A) this;
}
public A withAnnotations(AnnotationRef... annotations){
if (this.annotations != null) {this.annotations.clear();}
if (annotations != null) {for (AnnotationRef item :annotations){ this.addToAnnotations(item);}} return (A) this;
}
public Boolean hasAnnotations(){
return annotations != null && !annotations.isEmpty();
}
public MethodFluent.AnnotationsNested addNewAnnotation(){
return new AnnotationsNestedImpl();
}
public MethodFluent.AnnotationsNested addNewAnnotationLike(AnnotationRef item){
return new AnnotationsNestedImpl(-1, item);
}
public MethodFluent.AnnotationsNested setNewAnnotationLike(int index,AnnotationRef item){
return new AnnotationsNestedImpl(index, item);
}
public MethodFluent.AnnotationsNested editAnnotation(int index){
if (annotations.size() <= index) throw new RuntimeException("Can't edit annotations. Index exceeds size.");
return setNewAnnotationLike(index, buildAnnotation(index));
}
public MethodFluent.AnnotationsNested editFirstAnnotation(){
if (annotations.size() == 0) throw new RuntimeException("Can't edit first annotations. The list is empty.");
return setNewAnnotationLike(0, buildAnnotation(0));
}
public MethodFluent.AnnotationsNested editLastAnnotation(){
int index = annotations.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last annotations. The list is empty.");
return setNewAnnotationLike(index, buildAnnotation(index));
}
public MethodFluent.AnnotationsNested editMatchingAnnotation(Predicate predicate){
int index = -1;
for (int i=0;i();}
TypeParamDefBuilder builder = new TypeParamDefBuilder(item);_visitables.get("parameters").add(index >= 0 ? index : _visitables.get("parameters").size(), builder);this.parameters.add(index >= 0 ? index : parameters.size(), builder); return (A)this;
}
public A setToParameters(int index,TypeParamDef item){
if (this.parameters == null) {this.parameters = new ArrayList();}
TypeParamDefBuilder builder = new TypeParamDefBuilder(item);
if (index < 0 || index >= _visitables.get("parameters").size()) { _visitables.get("parameters").add(builder); } else { _visitables.get("parameters").set(index, builder);}
if (index < 0 || index >= parameters.size()) { parameters.add(builder); } else { parameters.set(index, builder);}
return (A)this;
}
public A addToParameters(TypeParamDef... items){
if (this.parameters == null) {this.parameters = new ArrayList();}
for (TypeParamDef item : items) {TypeParamDefBuilder builder = new TypeParamDefBuilder(item);_visitables.get("parameters").add(builder);this.parameters.add(builder);} return (A)this;
}
public A addAllToParameters(Collection items){
if (this.parameters == null) {this.parameters = new ArrayList();}
for (TypeParamDef item : items) {TypeParamDefBuilder builder = new TypeParamDefBuilder(item);_visitables.get("parameters").add(builder);this.parameters.add(builder);} return (A)this;
}
public A removeFromParameters(TypeParamDef... items){
for (TypeParamDef item : items) {TypeParamDefBuilder builder = new TypeParamDefBuilder(item);_visitables.get("parameters").remove(builder);if (this.parameters != null) {this.parameters.remove(builder);}} return (A)this;
}
public A removeAllFromParameters(Collection items){
for (TypeParamDef item : items) {TypeParamDefBuilder builder = new TypeParamDefBuilder(item);_visitables.get("parameters").remove(builder);if (this.parameters != null) {this.parameters.remove(builder);}} return (A)this;
}
/**
* This method has been deprecated, please use method buildParameters instead.
* @return The buildable object.
*/
@Deprecated public List getParameters(){
return build(parameters);
}
public List buildParameters(){
return build(parameters);
}
public TypeParamDef buildParameter(int index){
return this.parameters.get(index).build();
}
public TypeParamDef buildFirstParameter(){
return this.parameters.get(0).build();
}
public TypeParamDef buildLastParameter(){
return this.parameters.get(parameters.size() - 1).build();
}
public TypeParamDef buildMatchingParameter(Predicate predicate){
for (TypeParamDefBuilder item: parameters) { if(predicate.apply(item)){return item.build();} } return null;
}
public Boolean hasMatchingParameter(Predicate predicate){
for (TypeParamDefBuilder item: parameters) { if(predicate.apply(item)){return true;} } return false;
}
public A withParameters(List parameters){
if (this.parameters != null) { _visitables.get("parameters").removeAll(this.parameters);}
if (parameters != null) {this.parameters = new ArrayList(); for (TypeParamDef item : parameters){this.addToParameters(item);}} else { this.parameters = new ArrayList();} return (A) this;
}
public A withParameters(TypeParamDef... parameters){
if (this.parameters != null) {this.parameters.clear();}
if (parameters != null) {for (TypeParamDef item :parameters){ this.addToParameters(item);}} return (A) this;
}
public Boolean hasParameters(){
return parameters != null && !parameters.isEmpty();
}
public MethodFluent.ParametersNested addNewParameter(){
return new ParametersNestedImpl();
}
public MethodFluent.ParametersNested addNewParameterLike(TypeParamDef item){
return new ParametersNestedImpl(-1, item);
}
public MethodFluent.ParametersNested setNewParameterLike(int index,TypeParamDef item){
return new ParametersNestedImpl(index, item);
}
public MethodFluent.ParametersNested editParameter(int index){
if (parameters.size() <= index) throw new RuntimeException("Can't edit parameters. Index exceeds size.");
return setNewParameterLike(index, buildParameter(index));
}
public MethodFluent.ParametersNested editFirstParameter(){
if (parameters.size() == 0) throw new RuntimeException("Can't edit first parameters. The list is empty.");
return setNewParameterLike(0, buildParameter(0));
}
public MethodFluent.ParametersNested editLastParameter(){
int index = parameters.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last parameters. The list is empty.");
return setNewParameterLike(index, buildParameter(index));
}
public MethodFluent.ParametersNested editMatchingParameter(Predicate predicate){
int index = -1;
for (int i=0;i withNewPrimitiveRefReturnType(){
return new PrimitiveRefReturnTypeNestedImpl();
}
public MethodFluent.PrimitiveRefReturnTypeNested withNewPrimitiveRefReturnTypeLike(PrimitiveRef item){
return new PrimitiveRefReturnTypeNestedImpl(item);
}
public A withVoidRefReturnType(VoidRef voidRefReturnType){
_visitables.get("returnType").remove(this.returnType);
if (voidRefReturnType!=null){ this.returnType= new VoidRefBuilder(voidRefReturnType); _visitables.get("returnType").add(this.returnType);} return (A) this;
}
public MethodFluent.VoidRefReturnTypeNested withNewVoidRefReturnType(){
return new VoidRefReturnTypeNestedImpl();
}
public MethodFluent.VoidRefReturnTypeNested withNewVoidRefReturnTypeLike(VoidRef item){
return new VoidRefReturnTypeNestedImpl(item);
}
public A withWildcardRefReturnType(WildcardRef wildcardRefReturnType){
_visitables.get("returnType").remove(this.returnType);
if (wildcardRefReturnType!=null){ this.returnType= new WildcardRefBuilder(wildcardRefReturnType); _visitables.get("returnType").add(this.returnType);} return (A) this;
}
public MethodFluent.WildcardRefReturnTypeNested withNewWildcardRefReturnType(){
return new WildcardRefReturnTypeNestedImpl();
}
public MethodFluent.WildcardRefReturnTypeNested withNewWildcardRefReturnTypeLike(WildcardRef item){
return new WildcardRefReturnTypeNestedImpl(item);
}
public A withClassRefReturnType(ClassRef classRefReturnType){
_visitables.get("returnType").remove(this.returnType);
if (classRefReturnType!=null){ this.returnType= new ClassRefBuilder(classRefReturnType); _visitables.get("returnType").add(this.returnType);} return (A) this;
}
public MethodFluent.ClassRefReturnTypeNested withNewClassRefReturnType(){
return new ClassRefReturnTypeNestedImpl();
}
public MethodFluent.ClassRefReturnTypeNested withNewClassRefReturnTypeLike(ClassRef item){
return new ClassRefReturnTypeNestedImpl(item);
}
public A withTypeParamRefReturnType(TypeParamRef typeParamRefReturnType){
_visitables.get("returnType").remove(this.returnType);
if (typeParamRefReturnType!=null){ this.returnType= new TypeParamRefBuilder(typeParamRefReturnType); _visitables.get("returnType").add(this.returnType);} return (A) this;
}
public MethodFluent.TypeParamRefReturnTypeNested withNewTypeParamRefReturnType(){
return new TypeParamRefReturnTypeNestedImpl();
}
public MethodFluent.TypeParamRefReturnTypeNested withNewTypeParamRefReturnTypeLike(TypeParamRef item){
return new TypeParamRefReturnTypeNestedImpl(item);
}
public A addToArguments(int index,Property item){
if (this.arguments == null) {this.arguments = new ArrayList();}
PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("arguments").add(index >= 0 ? index : _visitables.get("arguments").size(), builder);this.arguments.add(index >= 0 ? index : arguments.size(), builder); return (A)this;
}
public A setToArguments(int index,Property item){
if (this.arguments == null) {this.arguments = new ArrayList();}
PropertyBuilder builder = new PropertyBuilder(item);
if (index < 0 || index >= _visitables.get("arguments").size()) { _visitables.get("arguments").add(builder); } else { _visitables.get("arguments").set(index, builder);}
if (index < 0 || index >= arguments.size()) { arguments.add(builder); } else { arguments.set(index, builder);}
return (A)this;
}
public A addToArguments(Property... items){
if (this.arguments == null) {this.arguments = new ArrayList();}
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("arguments").add(builder);this.arguments.add(builder);} return (A)this;
}
public A addAllToArguments(Collection items){
if (this.arguments == null) {this.arguments = new ArrayList();}
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("arguments").add(builder);this.arguments.add(builder);} return (A)this;
}
public A removeFromArguments(Property... items){
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("arguments").remove(builder);if (this.arguments != null) {this.arguments.remove(builder);}} return (A)this;
}
public A removeAllFromArguments(Collection items){
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("arguments").remove(builder);if (this.arguments != null) {this.arguments.remove(builder);}} return (A)this;
}
/**
* This method has been deprecated, please use method buildArguments instead.
* @return The buildable object.
*/
@Deprecated public List getArguments(){
return build(arguments);
}
public List buildArguments(){
return build(arguments);
}
public Property buildArgument(int index){
return this.arguments.get(index).build();
}
public Property buildFirstArgument(){
return this.arguments.get(0).build();
}
public Property buildLastArgument(){
return this.arguments.get(arguments.size() - 1).build();
}
public Property buildMatchingArgument(Predicate predicate){
for (PropertyBuilder item: arguments) { if(predicate.apply(item)){return item.build();} } return null;
}
public Boolean hasMatchingArgument(Predicate predicate){
for (PropertyBuilder item: arguments) { if(predicate.apply(item)){return true;} } return false;
}
public A withArguments(List arguments){
if (this.arguments != null) { _visitables.get("arguments").removeAll(this.arguments);}
if (arguments != null) {this.arguments = new ArrayList(); for (Property item : arguments){this.addToArguments(item);}} else { this.arguments = new ArrayList();} return (A) this;
}
public A withArguments(Property... arguments){
if (this.arguments != null) {this.arguments.clear();}
if (arguments != null) {for (Property item :arguments){ this.addToArguments(item);}} return (A) this;
}
public Boolean hasArguments(){
return arguments != null && !arguments.isEmpty();
}
public MethodFluent.ArgumentsNested addNewArgument(){
return new ArgumentsNestedImpl();
}
public MethodFluent.ArgumentsNested addNewArgumentLike(Property item){
return new ArgumentsNestedImpl(-1, item);
}
public MethodFluent.ArgumentsNested setNewArgumentLike(int index,Property item){
return new ArgumentsNestedImpl(index, item);
}
public MethodFluent.ArgumentsNested editArgument(int index){
if (arguments.size() <= index) throw new RuntimeException("Can't edit arguments. Index exceeds size.");
return setNewArgumentLike(index, buildArgument(index));
}
public MethodFluent.ArgumentsNested editFirstArgument(){
if (arguments.size() == 0) throw new RuntimeException("Can't edit first arguments. The list is empty.");
return setNewArgumentLike(0, buildArgument(0));
}
public MethodFluent.ArgumentsNested editLastArgument(){
int index = arguments.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last arguments. The list is empty.");
return setNewArgumentLike(index, buildArgument(index));
}
public MethodFluent.ArgumentsNested editMatchingArgument(Predicate predicate){
int index = -1;
for (int i=0;i();}
ClassRefBuilder builder = new ClassRefBuilder(item);_visitables.get("exceptions").add(index >= 0 ? index : _visitables.get("exceptions").size(), builder);this.exceptions.add(index >= 0 ? index : exceptions.size(), builder); return (A)this;
}
public A setToExceptions(int index,ClassRef item){
if (this.exceptions == null) {this.exceptions = new ArrayList();}
ClassRefBuilder builder = new ClassRefBuilder(item);
if (index < 0 || index >= _visitables.get("exceptions").size()) { _visitables.get("exceptions").add(builder); } else { _visitables.get("exceptions").set(index, builder);}
if (index < 0 || index >= exceptions.size()) { exceptions.add(builder); } else { exceptions.set(index, builder);}
return (A)this;
}
public A addToExceptions(ClassRef... items){
if (this.exceptions == null) {this.exceptions = new ArrayList();}
for (ClassRef item : items) {ClassRefBuilder builder = new ClassRefBuilder(item);_visitables.get("exceptions").add(builder);this.exceptions.add(builder);} return (A)this;
}
public A addAllToExceptions(Collection items){
if (this.exceptions == null) {this.exceptions = new ArrayList();}
for (ClassRef item : items) {ClassRefBuilder builder = new ClassRefBuilder(item);_visitables.get("exceptions").add(builder);this.exceptions.add(builder);} return (A)this;
}
public A removeFromExceptions(ClassRef... items){
for (ClassRef item : items) {ClassRefBuilder builder = new ClassRefBuilder(item);_visitables.get("exceptions").remove(builder);if (this.exceptions != null) {this.exceptions.remove(builder);}} return (A)this;
}
public A removeAllFromExceptions(Collection items){
for (ClassRef item : items) {ClassRefBuilder builder = new ClassRefBuilder(item);_visitables.get("exceptions").remove(builder);if (this.exceptions != null) {this.exceptions.remove(builder);}} return (A)this;
}
/**
* This method has been deprecated, please use method buildExceptions instead.
* @return The buildable object.
*/
@Deprecated public List getExceptions(){
return build(exceptions);
}
public List buildExceptions(){
return build(exceptions);
}
public ClassRef buildException(int index){
return this.exceptions.get(index).build();
}
public ClassRef buildFirstException(){
return this.exceptions.get(0).build();
}
public ClassRef buildLastException(){
return this.exceptions.get(exceptions.size() - 1).build();
}
public ClassRef buildMatchingException(Predicate predicate){
for (ClassRefBuilder item: exceptions) { if(predicate.apply(item)){return item.build();} } return null;
}
public Boolean hasMatchingException(Predicate predicate){
for (ClassRefBuilder item: exceptions) { if(predicate.apply(item)){return true;} } return false;
}
public A withExceptions(List exceptions){
if (this.exceptions != null) { _visitables.get("exceptions").removeAll(this.exceptions);}
if (exceptions != null) {this.exceptions = new ArrayList(); for (ClassRef item : exceptions){this.addToExceptions(item);}} else { this.exceptions = new ArrayList();} return (A) this;
}
public A withExceptions(ClassRef... exceptions){
if (this.exceptions != null) {this.exceptions.clear();}
if (exceptions != null) {for (ClassRef item :exceptions){ this.addToExceptions(item);}} return (A) this;
}
public Boolean hasExceptions(){
return exceptions != null && !exceptions.isEmpty();
}
public MethodFluent.ExceptionsNested addNewException(){
return new ExceptionsNestedImpl();
}
public MethodFluent.ExceptionsNested addNewExceptionLike(ClassRef item){
return new ExceptionsNestedImpl(-1, item);
}
public MethodFluent.ExceptionsNested setNewExceptionLike(int index,ClassRef item){
return new ExceptionsNestedImpl(index, item);
}
public MethodFluent.ExceptionsNested editException(int index){
if (exceptions.size() <= index) throw new RuntimeException("Can't edit exceptions. Index exceeds size.");
return setNewExceptionLike(index, buildException(index));
}
public MethodFluent.ExceptionsNested editFirstException(){
if (exceptions.size() == 0) throw new RuntimeException("Can't edit first exceptions. The list is empty.");
return setNewExceptionLike(0, buildException(0));
}
public MethodFluent.ExceptionsNested editLastException(){
int index = exceptions.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last exceptions. The list is empty.");
return setNewExceptionLike(index, buildException(index));
}
public MethodFluent.ExceptionsNested editMatchingException(Predicate predicate){
int index = -1;
for (int i=0;i withNewBlock(){
return new BlockNestedImpl();
}
public MethodFluent.BlockNested withNewBlockLike(Block item){
return new BlockNestedImpl(item);
}
public MethodFluent.BlockNested editBlock(){
return withNewBlockLike(getBlock());
}
public MethodFluent.BlockNested editOrNewBlock(){
return withNewBlockLike(getBlock() != null ? getBlock(): new BlockBuilder().build());
}
public MethodFluent.BlockNested editOrNewBlockLike(Block item){
return withNewBlockLike(getBlock() != null ? getBlock(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
MethodFluentImpl that = (MethodFluentImpl) o;
if (comments != null ? !comments.equals(that.comments) :that.comments != null) return false;
if (annotations != null ? !annotations.equals(that.annotations) :that.annotations != null) return false;
if (parameters != null ? !parameters.equals(that.parameters) :that.parameters != null) return false;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
if (returnType != null ? !returnType.equals(that.returnType) :that.returnType != null) return false;
if (arguments != null ? !arguments.equals(that.arguments) :that.arguments != null) return false;
if (varArgPreferred != that.varArgPreferred) return false;
if (exceptions != null ? !exceptions.equals(that.exceptions) :that.exceptions != null) return false;
if (block != null ? !block.equals(that.block) :that.block != null) return false;
return true;
}
public class AnnotationsNestedImpl extends AnnotationRefFluentImpl> implements MethodFluent.AnnotationsNested,Nested{
private final AnnotationRefBuilder builder;
private final int index;
AnnotationsNestedImpl(int index,AnnotationRef item){
this.index = index;
this.builder = new AnnotationRefBuilder(this, item);
}
AnnotationsNestedImpl(){
this.index = -1;
this.builder = new AnnotationRefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.setToAnnotations(index, builder.build());
}
public N endAnnotation(){
return and();
}
}
public class ParametersNestedImpl extends TypeParamDefFluentImpl> implements MethodFluent.ParametersNested,Nested{
private final TypeParamDefBuilder builder;
private final int index;
ParametersNestedImpl(int index,TypeParamDef item){
this.index = index;
this.builder = new TypeParamDefBuilder(this, item);
}
ParametersNestedImpl(){
this.index = -1;
this.builder = new TypeParamDefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.setToParameters(index, builder.build());
}
public N endParameter(){
return and();
}
}
public class PrimitiveRefReturnTypeNestedImpl extends PrimitiveRefFluentImpl> implements MethodFluent.PrimitiveRefReturnTypeNested,Nested{
private final PrimitiveRefBuilder builder;
PrimitiveRefReturnTypeNestedImpl(PrimitiveRef item){
this.builder = new PrimitiveRefBuilder(this, item);
}
PrimitiveRefReturnTypeNestedImpl(){
this.builder = new PrimitiveRefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.withPrimitiveRefReturnType(builder.build());
}
public N endPrimitiveRefReturnType(){
return and();
}
}
public class VoidRefReturnTypeNestedImpl extends VoidRefFluentImpl> implements MethodFluent.VoidRefReturnTypeNested,Nested{
private final VoidRefBuilder builder;
VoidRefReturnTypeNestedImpl(VoidRef item){
this.builder = new VoidRefBuilder(this, item);
}
VoidRefReturnTypeNestedImpl(){
this.builder = new VoidRefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.withVoidRefReturnType(builder.build());
}
public N endVoidRefReturnType(){
return and();
}
}
public class WildcardRefReturnTypeNestedImpl extends WildcardRefFluentImpl> implements MethodFluent.WildcardRefReturnTypeNested,Nested{
private final WildcardRefBuilder builder;
WildcardRefReturnTypeNestedImpl(WildcardRef item){
this.builder = new WildcardRefBuilder(this, item);
}
WildcardRefReturnTypeNestedImpl(){
this.builder = new WildcardRefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.withWildcardRefReturnType(builder.build());
}
public N endWildcardRefReturnType(){
return and();
}
}
public class ClassRefReturnTypeNestedImpl extends ClassRefFluentImpl> implements MethodFluent.ClassRefReturnTypeNested,Nested{
private final ClassRefBuilder builder;
ClassRefReturnTypeNestedImpl(ClassRef item){
this.builder = new ClassRefBuilder(this, item);
}
ClassRefReturnTypeNestedImpl(){
this.builder = new ClassRefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.withClassRefReturnType(builder.build());
}
public N endClassRefReturnType(){
return and();
}
}
public class TypeParamRefReturnTypeNestedImpl extends TypeParamRefFluentImpl> implements MethodFluent.TypeParamRefReturnTypeNested,Nested{
private final TypeParamRefBuilder builder;
TypeParamRefReturnTypeNestedImpl(TypeParamRef item){
this.builder = new TypeParamRefBuilder(this, item);
}
TypeParamRefReturnTypeNestedImpl(){
this.builder = new TypeParamRefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.withTypeParamRefReturnType(builder.build());
}
public N endTypeParamRefReturnType(){
return and();
}
}
public class ArgumentsNestedImpl extends PropertyFluentImpl> implements MethodFluent.ArgumentsNested,Nested{
private final PropertyBuilder builder;
private final int index;
ArgumentsNestedImpl(int index,Property item){
this.index = index;
this.builder = new PropertyBuilder(this, item);
}
ArgumentsNestedImpl(){
this.index = -1;
this.builder = new PropertyBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.setToArguments(index, builder.build());
}
public N endArgument(){
return and();
}
}
public class ExceptionsNestedImpl extends ClassRefFluentImpl> implements MethodFluent.ExceptionsNested,Nested{
private final ClassRefBuilder builder;
private final int index;
ExceptionsNestedImpl(int index,ClassRef item){
this.index = index;
this.builder = new ClassRefBuilder(this, item);
}
ExceptionsNestedImpl(){
this.index = -1;
this.builder = new ClassRefBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.setToExceptions(index, builder.build());
}
public N endException(){
return and();
}
}
public class BlockNestedImpl extends BlockFluentImpl> implements MethodFluent.BlockNested,Nested{
private final BlockBuilder builder;
BlockNestedImpl(Block item){
this.builder = new BlockBuilder(this, item);
}
BlockNestedImpl(){
this.builder = new BlockBuilder(this);
}
public N and(){
return (N) MethodFluentImpl.this.withBlock(builder.build());
}
public N endBlock(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy