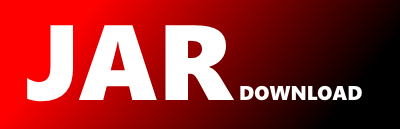
io.sundr.codegen.model.PropertyFluent Maven / Gradle / Ivy
/*
* Copyright 2019 The original authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.sundr.codegen.model;
import io.sundr.builder.Nested;
import io.sundr.builder.Predicate;
import java.util.Collection;
import java.util.List;
public interface PropertyFluent> extends ModifierSupportFluent{
public A addToAnnotations(int index, AnnotationRef item);
public A setToAnnotations(int index, AnnotationRef item);
public A addToAnnotations(AnnotationRef... items);
public A addAllToAnnotations(Collection items);
public A removeFromAnnotations(AnnotationRef... items);
public A removeAllFromAnnotations(Collection items);
/**
* This method has been deprecated, please use method buildAnnotations instead.
* @return The buildable object.
*/
@Deprecated public List getAnnotations();
public List buildAnnotations();
public AnnotationRef buildAnnotation(int index);
public AnnotationRef buildFirstAnnotation();
public AnnotationRef buildLastAnnotation();
public AnnotationRef buildMatchingAnnotation(Predicate predicate);
public Boolean hasMatchingAnnotation(Predicate predicate);
public A withAnnotations(List annotations);
public A withAnnotations(AnnotationRef... annotations);
public Boolean hasAnnotations();
public PropertyFluent.AnnotationsNested addNewAnnotation();
public PropertyFluent.AnnotationsNested addNewAnnotationLike(AnnotationRef item);
public PropertyFluent.AnnotationsNested setNewAnnotationLike(int index, AnnotationRef item);
public PropertyFluent.AnnotationsNested editAnnotation(int index);
public PropertyFluent.AnnotationsNested editFirstAnnotation();
public PropertyFluent.AnnotationsNested editLastAnnotation();
public PropertyFluent.AnnotationsNested editMatchingAnnotation(Predicate predicate);
/**
* This method has been deprecated, please use method buildTypeRef instead.
* @return The buildable object.
*/
@Deprecated public TypeRef getTypeRef();
public TypeRef buildTypeRef();
public A withTypeRef(TypeRef typeRef);
public Boolean hasTypeRef();
public A withPrimitiveRefType(PrimitiveRef primitiveRefType);
public PropertyFluent.PrimitiveRefTypeNested withNewPrimitiveRefType();
public PropertyFluent.PrimitiveRefTypeNested withNewPrimitiveRefTypeLike(PrimitiveRef item);
public A withVoidRefType(VoidRef voidRefType);
public PropertyFluent.VoidRefTypeNested withNewVoidRefType();
public PropertyFluent.VoidRefTypeNested withNewVoidRefTypeLike(VoidRef item);
public A withWildcardRefType(WildcardRef wildcardRefType);
public PropertyFluent.WildcardRefTypeNested withNewWildcardRefType();
public PropertyFluent.WildcardRefTypeNested withNewWildcardRefTypeLike(WildcardRef item);
public A withClassRefType(ClassRef classRefType);
public PropertyFluent.ClassRefTypeNested withNewClassRefType();
public PropertyFluent.ClassRefTypeNested withNewClassRefTypeLike(ClassRef item);
public A withTypeParamRefType(TypeParamRef typeParamRefType);
public PropertyFluent.TypeParamRefTypeNested withNewTypeParamRefType();
public PropertyFluent.TypeParamRefTypeNested withNewTypeParamRefTypeLike(TypeParamRef item);
public String getName();
public A withName(String name);
public Boolean hasName();
public A withNewName(String arg1);
public A withNewName(StringBuilder arg1);
public A withNewName(StringBuffer arg1);
public interface AnnotationsNested extends Nested,AnnotationRefFluent>{
public N and(); public N endAnnotation();
}
public interface PrimitiveRefTypeNested extends Nested,PrimitiveRefFluent>{
public N and(); public N endPrimitiveRefType();
}
public interface VoidRefTypeNested extends Nested,VoidRefFluent>{
public N and(); public N endVoidRefType();
}
public interface WildcardRefTypeNested extends Nested,WildcardRefFluent>{
public N and(); public N endWildcardRefType();
}
public interface ClassRefTypeNested extends Nested,ClassRefFluent>{
public N and(); public N endClassRefType();
}
public interface TypeParamRefTypeNested extends Nested,TypeParamRefFluent>{
public N and(); public N endTypeParamRefType();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy