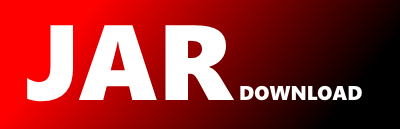
io.sundr.codegen.model.PropertyFluentImpl Maven / Gradle / Ivy
/*
* Copyright 2019 The original authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.sundr.codegen.model;
import io.sundr.builder.Nested;
import io.sundr.builder.Predicate;
import io.sundr.builder.VisitableBuilder;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
public class PropertyFluentImpl> extends ModifierSupportFluentImpl implements PropertyFluent{
private List annotations = new ArrayList();
private VisitableBuilder extends TypeRef,?> typeRef;
private String name;
public PropertyFluentImpl(){
}
public PropertyFluentImpl(Property instance){
this.withAnnotations(instance.getAnnotations());
this.withTypeRef(instance.getTypeRef());
this.withName(instance.getName());
this.withModifiers(instance.getModifiers());
this.withAttributes(instance.getAttributes());
}
public A addToAnnotations(int index,AnnotationRef item){
if (this.annotations == null) {this.annotations = new ArrayList();}
AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").add(index >= 0 ? index : _visitables.get("annotations").size(), builder);this.annotations.add(index >= 0 ? index : annotations.size(), builder); return (A)this;
}
public A setToAnnotations(int index,AnnotationRef item){
if (this.annotations == null) {this.annotations = new ArrayList();}
AnnotationRefBuilder builder = new AnnotationRefBuilder(item);
if (index < 0 || index >= _visitables.get("annotations").size()) { _visitables.get("annotations").add(builder); } else { _visitables.get("annotations").set(index, builder);}
if (index < 0 || index >= annotations.size()) { annotations.add(builder); } else { annotations.set(index, builder);}
return (A)this;
}
public A addToAnnotations(AnnotationRef... items){
if (this.annotations == null) {this.annotations = new ArrayList();}
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").add(builder);this.annotations.add(builder);} return (A)this;
}
public A addAllToAnnotations(Collection items){
if (this.annotations == null) {this.annotations = new ArrayList();}
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").add(builder);this.annotations.add(builder);} return (A)this;
}
public A removeFromAnnotations(AnnotationRef... items){
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").remove(builder);if (this.annotations != null) {this.annotations.remove(builder);}} return (A)this;
}
public A removeAllFromAnnotations(Collection items){
for (AnnotationRef item : items) {AnnotationRefBuilder builder = new AnnotationRefBuilder(item);_visitables.get("annotations").remove(builder);if (this.annotations != null) {this.annotations.remove(builder);}} return (A)this;
}
/**
* This method has been deprecated, please use method buildAnnotations instead.
* @return The buildable object.
*/
@Deprecated public List getAnnotations(){
return build(annotations);
}
public List buildAnnotations(){
return build(annotations);
}
public AnnotationRef buildAnnotation(int index){
return this.annotations.get(index).build();
}
public AnnotationRef buildFirstAnnotation(){
return this.annotations.get(0).build();
}
public AnnotationRef buildLastAnnotation(){
return this.annotations.get(annotations.size() - 1).build();
}
public AnnotationRef buildMatchingAnnotation(Predicate predicate){
for (AnnotationRefBuilder item: annotations) { if(predicate.apply(item)){return item.build();} } return null;
}
public Boolean hasMatchingAnnotation(Predicate predicate){
for (AnnotationRefBuilder item: annotations) { if(predicate.apply(item)){return true;} } return false;
}
public A withAnnotations(List annotations){
if (this.annotations != null) { _visitables.get("annotations").removeAll(this.annotations);}
if (annotations != null) {this.annotations = new ArrayList(); for (AnnotationRef item : annotations){this.addToAnnotations(item);}} else { this.annotations = new ArrayList();} return (A) this;
}
public A withAnnotations(AnnotationRef... annotations){
if (this.annotations != null) {this.annotations.clear();}
if (annotations != null) {for (AnnotationRef item :annotations){ this.addToAnnotations(item);}} return (A) this;
}
public Boolean hasAnnotations(){
return annotations != null && !annotations.isEmpty();
}
public PropertyFluent.AnnotationsNested addNewAnnotation(){
return new AnnotationsNestedImpl();
}
public PropertyFluent.AnnotationsNested addNewAnnotationLike(AnnotationRef item){
return new AnnotationsNestedImpl(-1, item);
}
public PropertyFluent.AnnotationsNested setNewAnnotationLike(int index,AnnotationRef item){
return new AnnotationsNestedImpl(index, item);
}
public PropertyFluent.AnnotationsNested editAnnotation(int index){
if (annotations.size() <= index) throw new RuntimeException("Can't edit annotations. Index exceeds size.");
return setNewAnnotationLike(index, buildAnnotation(index));
}
public PropertyFluent.AnnotationsNested editFirstAnnotation(){
if (annotations.size() == 0) throw new RuntimeException("Can't edit first annotations. The list is empty.");
return setNewAnnotationLike(0, buildAnnotation(0));
}
public PropertyFluent.AnnotationsNested editLastAnnotation(){
int index = annotations.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last annotations. The list is empty.");
return setNewAnnotationLike(index, buildAnnotation(index));
}
public PropertyFluent.AnnotationsNested editMatchingAnnotation(Predicate predicate){
int index = -1;
for (int i=0;i withNewPrimitiveRefType(){
return new PrimitiveRefTypeNestedImpl();
}
public PropertyFluent.PrimitiveRefTypeNested withNewPrimitiveRefTypeLike(PrimitiveRef item){
return new PrimitiveRefTypeNestedImpl(item);
}
public A withVoidRefType(VoidRef voidRefType){
_visitables.get("typeRef").remove(this.typeRef);
if (voidRefType!=null){ this.typeRef= new VoidRefBuilder(voidRefType); _visitables.get("typeRef").add(this.typeRef);} return (A) this;
}
public PropertyFluent.VoidRefTypeNested withNewVoidRefType(){
return new VoidRefTypeNestedImpl();
}
public PropertyFluent.VoidRefTypeNested withNewVoidRefTypeLike(VoidRef item){
return new VoidRefTypeNestedImpl(item);
}
public A withWildcardRefType(WildcardRef wildcardRefType){
_visitables.get("typeRef").remove(this.typeRef);
if (wildcardRefType!=null){ this.typeRef= new WildcardRefBuilder(wildcardRefType); _visitables.get("typeRef").add(this.typeRef);} return (A) this;
}
public PropertyFluent.WildcardRefTypeNested withNewWildcardRefType(){
return new WildcardRefTypeNestedImpl();
}
public PropertyFluent.WildcardRefTypeNested withNewWildcardRefTypeLike(WildcardRef item){
return new WildcardRefTypeNestedImpl(item);
}
public A withClassRefType(ClassRef classRefType){
_visitables.get("typeRef").remove(this.typeRef);
if (classRefType!=null){ this.typeRef= new ClassRefBuilder(classRefType); _visitables.get("typeRef").add(this.typeRef);} return (A) this;
}
public PropertyFluent.ClassRefTypeNested withNewClassRefType(){
return new ClassRefTypeNestedImpl();
}
public PropertyFluent.ClassRefTypeNested withNewClassRefTypeLike(ClassRef item){
return new ClassRefTypeNestedImpl(item);
}
public A withTypeParamRefType(TypeParamRef typeParamRefType){
_visitables.get("typeRef").remove(this.typeRef);
if (typeParamRefType!=null){ this.typeRef= new TypeParamRefBuilder(typeParamRefType); _visitables.get("typeRef").add(this.typeRef);} return (A) this;
}
public PropertyFluent.TypeParamRefTypeNested withNewTypeParamRefType(){
return new TypeParamRefTypeNestedImpl();
}
public PropertyFluent.TypeParamRefTypeNested withNewTypeParamRefTypeLike(TypeParamRef item){
return new TypeParamRefTypeNestedImpl(item);
}
public String getName(){
return this.name;
}
public A withName(String name){
this.name=name; return (A) this;
}
public Boolean hasName(){
return this.name != null;
}
public A withNewName(String arg1){
return (A)withName(new String(arg1));
}
public A withNewName(StringBuilder arg1){
return (A)withName(new String(arg1));
}
public A withNewName(StringBuffer arg1){
return (A)withName(new String(arg1));
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
PropertyFluentImpl that = (PropertyFluentImpl) o;
if (annotations != null ? !annotations.equals(that.annotations) :that.annotations != null) return false;
if (typeRef != null ? !typeRef.equals(that.typeRef) :that.typeRef != null) return false;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
return true;
}
public class AnnotationsNestedImpl extends AnnotationRefFluentImpl> implements PropertyFluent.AnnotationsNested,Nested{
private final AnnotationRefBuilder builder;
private final int index;
AnnotationsNestedImpl(int index,AnnotationRef item){
this.index = index;
this.builder = new AnnotationRefBuilder(this, item);
}
AnnotationsNestedImpl(){
this.index = -1;
this.builder = new AnnotationRefBuilder(this);
}
public N and(){
return (N) PropertyFluentImpl.this.setToAnnotations(index, builder.build());
}
public N endAnnotation(){
return and();
}
}
public class PrimitiveRefTypeNestedImpl extends PrimitiveRefFluentImpl> implements PropertyFluent.PrimitiveRefTypeNested,Nested{
private final PrimitiveRefBuilder builder;
PrimitiveRefTypeNestedImpl(PrimitiveRef item){
this.builder = new PrimitiveRefBuilder(this, item);
}
PrimitiveRefTypeNestedImpl(){
this.builder = new PrimitiveRefBuilder(this);
}
public N and(){
return (N) PropertyFluentImpl.this.withPrimitiveRefType(builder.build());
}
public N endPrimitiveRefType(){
return and();
}
}
public class VoidRefTypeNestedImpl extends VoidRefFluentImpl> implements PropertyFluent.VoidRefTypeNested,Nested{
private final VoidRefBuilder builder;
VoidRefTypeNestedImpl(VoidRef item){
this.builder = new VoidRefBuilder(this, item);
}
VoidRefTypeNestedImpl(){
this.builder = new VoidRefBuilder(this);
}
public N and(){
return (N) PropertyFluentImpl.this.withVoidRefType(builder.build());
}
public N endVoidRefType(){
return and();
}
}
public class WildcardRefTypeNestedImpl extends WildcardRefFluentImpl> implements PropertyFluent.WildcardRefTypeNested,Nested{
private final WildcardRefBuilder builder;
WildcardRefTypeNestedImpl(WildcardRef item){
this.builder = new WildcardRefBuilder(this, item);
}
WildcardRefTypeNestedImpl(){
this.builder = new WildcardRefBuilder(this);
}
public N and(){
return (N) PropertyFluentImpl.this.withWildcardRefType(builder.build());
}
public N endWildcardRefType(){
return and();
}
}
public class ClassRefTypeNestedImpl extends ClassRefFluentImpl> implements PropertyFluent.ClassRefTypeNested,Nested{
private final ClassRefBuilder builder;
ClassRefTypeNestedImpl(ClassRef item){
this.builder = new ClassRefBuilder(this, item);
}
ClassRefTypeNestedImpl(){
this.builder = new ClassRefBuilder(this);
}
public N and(){
return (N) PropertyFluentImpl.this.withClassRefType(builder.build());
}
public N endClassRefType(){
return and();
}
}
public class TypeParamRefTypeNestedImpl extends TypeParamRefFluentImpl> implements PropertyFluent.TypeParamRefTypeNested,Nested{
private final TypeParamRefBuilder builder;
TypeParamRefTypeNestedImpl(TypeParamRef item){
this.builder = new TypeParamRefBuilder(this, item);
}
TypeParamRefTypeNestedImpl(){
this.builder = new TypeParamRefBuilder(this);
}
public N and(){
return (N) PropertyFluentImpl.this.withTypeParamRefType(builder.build());
}
public N endTypeParamRefType(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy