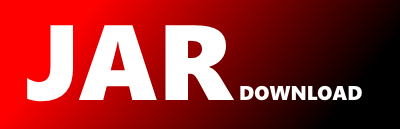
io.sundr.model.BinaryExpressionFluent Maven / Gradle / Ivy
package io.sundr.model;
import java.lang.String;
import java.lang.Class;
import java.lang.SuppressWarnings;
import java.lang.Integer;
import io.sundr.builder.BaseFluent;
import java.lang.Object;
import io.sundr.builder.VisitableBuilder;
import io.sundr.builder.Nested;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class BinaryExpressionFluent> extends BaseFluent{
public BinaryExpressionFluent() {
}
public BinaryExpressionFluent(BinaryExpression instance) {
this.copyInstance(instance);
}
private VisitableBuilder extends Expression,?> left;
private VisitableBuilder extends Expression,?> right;
protected void copyInstance(BinaryExpression instance) {
if (instance != null) {
this.withLeft(instance.getLeft());
this.withRight(instance.getRight());
}
}
public Expression buildLeft() {
return this.left!=null?this.left.build():null;
}
public A withLeft(Expression left) {
if (left==null){ this.left = null; _visitables.remove("left"); return (A) this;}
VisitableBuilder extends Expression,?> builder = builder(left); _visitables.get("left").clear();_visitables.get("left").add(builder);this.left = builder;
return (A) this;
}
public boolean hasLeft() {
return this.left != null;
}
public MultiplyLeftNested withNewMultiplyLeft() {
return new MultiplyLeftNested(null);
}
public MultiplyLeftNested withNewMultiplyLeftLike(Multiply item) {
return new MultiplyLeftNested(item);
}
public A withNewMultiplyLeft(Object left,Object right) {
return (A)withLeft(new Multiply(left, right));
}
public NewArrayLeftNested withNewNewArrayLeft() {
return new NewArrayLeftNested(null);
}
public NewArrayLeftNested withNewNewArrayLeftLike(NewArray item) {
return new NewArrayLeftNested(item);
}
public A withNewNewArrayLeft(Class type,Integer[] sizes) {
return (A)withLeft(new NewArray(type, sizes));
}
public InstanceOfLeftNested withNewInstanceOfLeft() {
return new InstanceOfLeftNested(null);
}
public InstanceOfLeftNested withNewInstanceOfLeftLike(InstanceOf item) {
return new InstanceOfLeftNested(item);
}
public MethodCallLeftNested withNewMethodCallLeft() {
return new MethodCallLeftNested(null);
}
public MethodCallLeftNested withNewMethodCallLeftLike(MethodCall item) {
return new MethodCallLeftNested(item);
}
public InverseLeftNested withNewInverseLeft() {
return new InverseLeftNested(null);
}
public InverseLeftNested withNewInverseLeftLike(Inverse item) {
return new InverseLeftNested(item);
}
public IndexLeftNested withNewIndexLeft() {
return new IndexLeftNested(null);
}
public IndexLeftNested withNewIndexLeftLike(Index item) {
return new IndexLeftNested(item);
}
public GreaterThanOrEqualLeftNested withNewGreaterThanOrEqualLeft() {
return new GreaterThanOrEqualLeftNested(null);
}
public GreaterThanOrEqualLeftNested withNewGreaterThanOrEqualLeftLike(GreaterThanOrEqual item) {
return new GreaterThanOrEqualLeftNested(item);
}
public A withNewGreaterThanOrEqualLeft(Object left,Object right) {
return (A)withLeft(new GreaterThanOrEqual(left, right));
}
public BitwiseAndLeftNested withNewBitwiseAndLeft() {
return new BitwiseAndLeftNested(null);
}
public BitwiseAndLeftNested withNewBitwiseAndLeftLike(BitwiseAnd item) {
return new BitwiseAndLeftNested(item);
}
public A withNewBitwiseAndLeft(Object left,Object right) {
return (A)withLeft(new BitwiseAnd(left, right));
}
public MinusLeftNested withNewMinusLeft() {
return new MinusLeftNested(null);
}
public MinusLeftNested withNewMinusLeftLike(Minus item) {
return new MinusLeftNested(item);
}
public A withNewMinusLeft(Object left,Object right) {
return (A)withLeft(new Minus(left, right));
}
public LogicalOrLeftNested withNewLogicalOrLeft() {
return new LogicalOrLeftNested(null);
}
public LogicalOrLeftNested withNewLogicalOrLeftLike(LogicalOr item) {
return new LogicalOrLeftNested(item);
}
public A withNewLogicalOrLeft(Object left,Object right) {
return (A)withLeft(new LogicalOr(left, right));
}
public NotEqualsLeftNested withNewNotEqualsLeft() {
return new NotEqualsLeftNested(null);
}
public NotEqualsLeftNested withNewNotEqualsLeftLike(NotEquals item) {
return new NotEqualsLeftNested(item);
}
public A withNewNotEqualsLeft(Object left,Object right) {
return (A)withLeft(new NotEquals(left, right));
}
public DivideLeftNested withNewDivideLeft() {
return new DivideLeftNested(null);
}
public DivideLeftNested withNewDivideLeftLike(Divide item) {
return new DivideLeftNested(item);
}
public A withNewDivideLeft(Object left,Object right) {
return (A)withLeft(new Divide(left, right));
}
public LessThanLeftNested withNewLessThanLeft() {
return new LessThanLeftNested(null);
}
public LessThanLeftNested withNewLessThanLeftLike(LessThan item) {
return new LessThanLeftNested(item);
}
public A withNewLessThanLeft(Object left,Object right) {
return (A)withLeft(new LessThan(left, right));
}
public BitwiseOrLeftNested withNewBitwiseOrLeft() {
return new BitwiseOrLeftNested(null);
}
public BitwiseOrLeftNested withNewBitwiseOrLeftLike(BitwiseOr item) {
return new BitwiseOrLeftNested(item);
}
public A withNewBitwiseOrLeft(Object left,Object right) {
return (A)withLeft(new BitwiseOr(left, right));
}
public PropertyRefLeftNested withNewPropertyRefLeft() {
return new PropertyRefLeftNested(null);
}
public PropertyRefLeftNested withNewPropertyRefLeftLike(PropertyRef item) {
return new PropertyRefLeftNested(item);
}
public RightShiftLeftNested withNewRightShiftLeft() {
return new RightShiftLeftNested(null);
}
public RightShiftLeftNested withNewRightShiftLeftLike(RightShift item) {
return new RightShiftLeftNested(item);
}
public A withNewRightShiftLeft(Object left,Object right) {
return (A)withLeft(new RightShift(left, right));
}
public GreaterThanLeftNested withNewGreaterThanLeft() {
return new GreaterThanLeftNested(null);
}
public GreaterThanLeftNested withNewGreaterThanLeftLike(GreaterThan item) {
return new GreaterThanLeftNested(item);
}
public A withNewGreaterThanLeft(Object left,Object right) {
return (A)withLeft(new GreaterThan(left, right));
}
public DeclareLeftNested withNewDeclareLeft() {
return new DeclareLeftNested(null);
}
public DeclareLeftNested withNewDeclareLeftLike(Declare item) {
return new DeclareLeftNested(item);
}
public A withNewDeclareLeft(Class type,String name) {
return (A)withLeft(new Declare(type, name));
}
public A withNewDeclareLeft(Class type,String name,Object value) {
return (A)withLeft(new Declare(type, name, value));
}
public CastLeftNested withNewCastLeft() {
return new CastLeftNested(null);
}
public CastLeftNested withNewCastLeftLike(Cast item) {
return new CastLeftNested(item);
}
public ModuloLeftNested withNewModuloLeft() {
return new ModuloLeftNested(null);
}
public ModuloLeftNested withNewModuloLeftLike(Modulo item) {
return new ModuloLeftNested(item);
}
public A withNewModuloLeft(Object left,Object right) {
return (A)withLeft(new Modulo(left, right));
}
public ValueRefLeftNested withNewValueRefLeft() {
return new ValueRefLeftNested(null);
}
public ValueRefLeftNested withNewValueRefLeftLike(ValueRef item) {
return new ValueRefLeftNested(item);
}
public A withNewValueRefLeft(Object value) {
return (A)withLeft(new ValueRef(value));
}
public LeftShiftLeftNested withNewLeftShiftLeft() {
return new LeftShiftLeftNested(null);
}
public LeftShiftLeftNested withNewLeftShiftLeftLike(LeftShift item) {
return new LeftShiftLeftNested(item);
}
public A withNewLeftShiftLeft(Object left,Object right) {
return (A)withLeft(new LeftShift(left, right));
}
public TernaryLeftNested withNewTernaryLeft() {
return new TernaryLeftNested(null);
}
public TernaryLeftNested withNewTernaryLeftLike(Ternary item) {
return new TernaryLeftNested(item);
}
public BinaryExpressionLeftNested withNewBinaryExpressionLeft() {
return new BinaryExpressionLeftNested(null);
}
public BinaryExpressionLeftNested withNewBinaryExpressionLeftLike(BinaryExpression item) {
return new BinaryExpressionLeftNested(item);
}
public EqualsLeftNested withNewEqualsLeft() {
return new EqualsLeftNested(null);
}
public EqualsLeftNested withNewEqualsLeftLike(Equals item) {
return new EqualsLeftNested(item);
}
public A withNewEqualsLeft(Object left,Object right) {
return (A)withLeft(new Equals(left, right));
}
public EnclosedLeftNested withNewEnclosedLeft() {
return new EnclosedLeftNested(null);
}
public EnclosedLeftNested withNewEnclosedLeftLike(Enclosed item) {
return new EnclosedLeftNested(item);
}
public PreDecrementLeftNested withNewPreDecrementLeft() {
return new PreDecrementLeftNested(null);
}
public PreDecrementLeftNested withNewPreDecrementLeftLike(PreDecrement item) {
return new PreDecrementLeftNested(item);
}
public PostDecrementLeftNested withNewPostDecrementLeft() {
return new PostDecrementLeftNested(null);
}
public PostDecrementLeftNested withNewPostDecrementLeftLike(PostDecrement item) {
return new PostDecrementLeftNested(item);
}
public LambdaLeftNested withNewLambdaLeft() {
return new LambdaLeftNested(null);
}
public LambdaLeftNested withNewLambdaLeftLike(Lambda item) {
return new LambdaLeftNested(item);
}
public NotLeftNested withNewNotLeft() {
return new NotLeftNested(null);
}
public NotLeftNested withNewNotLeftLike(Not item) {
return new NotLeftNested(item);
}
public ThisLeftNested withNewThisLeft() {
return new ThisLeftNested(null);
}
public ThisLeftNested withNewThisLeftLike(This item) {
return new ThisLeftNested(item);
}
public NegativeLeftNested withNewNegativeLeft() {
return new NegativeLeftNested(null);
}
public NegativeLeftNested withNewNegativeLeftLike(Negative item) {
return new NegativeLeftNested(item);
}
public AssignLeftNested withNewAssignLeft() {
return new AssignLeftNested(null);
}
public AssignLeftNested withNewAssignLeftLike(Assign item) {
return new AssignLeftNested(item);
}
public LogicalAndLeftNested withNewLogicalAndLeft() {
return new LogicalAndLeftNested(null);
}
public LogicalAndLeftNested withNewLogicalAndLeftLike(LogicalAnd item) {
return new LogicalAndLeftNested(item);
}
public A withNewLogicalAndLeft(Object left,Object right) {
return (A)withLeft(new LogicalAnd(left, right));
}
public PostIncrementLeftNested withNewPostIncrementLeft() {
return new PostIncrementLeftNested(null);
}
public PostIncrementLeftNested withNewPostIncrementLeftLike(PostIncrement item) {
return new PostIncrementLeftNested(item);
}
public RightUnsignedShiftLeftNested withNewRightUnsignedShiftLeft() {
return new RightUnsignedShiftLeftNested(null);
}
public RightUnsignedShiftLeftNested withNewRightUnsignedShiftLeftLike(RightUnsignedShift item) {
return new RightUnsignedShiftLeftNested(item);
}
public A withNewRightUnsignedShiftLeft(Object left,Object right) {
return (A)withLeft(new RightUnsignedShift(left, right));
}
public PlusLeftNested withNewPlusLeft() {
return new PlusLeftNested(null);
}
public PlusLeftNested withNewPlusLeftLike(Plus item) {
return new PlusLeftNested(item);
}
public A withNewPlusLeft(Object left,Object right) {
return (A)withLeft(new Plus(left, right));
}
public ConstructLeftNested withNewConstructLeft() {
return new ConstructLeftNested(null);
}
public ConstructLeftNested withNewConstructLeftLike(Construct item) {
return new ConstructLeftNested(item);
}
public XorLeftNested withNewXorLeft() {
return new XorLeftNested(null);
}
public XorLeftNested withNewXorLeftLike(Xor item) {
return new XorLeftNested(item);
}
public A withNewXorLeft(Object left,Object right) {
return (A)withLeft(new Xor(left, right));
}
public PreIncrementLeftNested withNewPreIncrementLeft() {
return new PreIncrementLeftNested(null);
}
public PreIncrementLeftNested withNewPreIncrementLeftLike(PreIncrement item) {
return new PreIncrementLeftNested(item);
}
public LessThanOrEqualLeftNested withNewLessThanOrEqualLeft() {
return new LessThanOrEqualLeftNested(null);
}
public LessThanOrEqualLeftNested withNewLessThanOrEqualLeftLike(LessThanOrEqual item) {
return new LessThanOrEqualLeftNested(item);
}
public A withNewLessThanOrEqualLeft(Object left,Object right) {
return (A)withLeft(new LessThanOrEqual(left, right));
}
public PositiveLeftNested withNewPositiveLeft() {
return new PositiveLeftNested(null);
}
public PositiveLeftNested withNewPositiveLeftLike(Positive item) {
return new PositiveLeftNested(item);
}
public Expression buildRight() {
return this.right!=null?this.right.build():null;
}
public A withRight(Expression right) {
if (right==null){ this.right = null; _visitables.remove("right"); return (A) this;}
VisitableBuilder extends Expression,?> builder = builder(right); _visitables.get("right").clear();_visitables.get("right").add(builder);this.right = builder;
return (A) this;
}
public boolean hasRight() {
return this.right != null;
}
public MultiplyRightNested withNewMultiplyRight() {
return new MultiplyRightNested(null);
}
public MultiplyRightNested withNewMultiplyRightLike(Multiply item) {
return new MultiplyRightNested(item);
}
public A withNewMultiplyRight(Object left,Object right) {
return (A)withRight(new Multiply(left, right));
}
public NewArrayRightNested withNewNewArrayRight() {
return new NewArrayRightNested(null);
}
public NewArrayRightNested withNewNewArrayRightLike(NewArray item) {
return new NewArrayRightNested(item);
}
public A withNewNewArrayRight(Class type,Integer[] sizes) {
return (A)withRight(new NewArray(type, sizes));
}
public InstanceOfRightNested withNewInstanceOfRight() {
return new InstanceOfRightNested(null);
}
public InstanceOfRightNested withNewInstanceOfRightLike(InstanceOf item) {
return new InstanceOfRightNested(item);
}
public MethodCallRightNested withNewMethodCallRight() {
return new MethodCallRightNested(null);
}
public MethodCallRightNested withNewMethodCallRightLike(MethodCall item) {
return new MethodCallRightNested(item);
}
public InverseRightNested withNewInverseRight() {
return new InverseRightNested(null);
}
public InverseRightNested withNewInverseRightLike(Inverse item) {
return new InverseRightNested(item);
}
public IndexRightNested withNewIndexRight() {
return new IndexRightNested(null);
}
public IndexRightNested withNewIndexRightLike(Index item) {
return new IndexRightNested(item);
}
public GreaterThanOrEqualRightNested withNewGreaterThanOrEqualRight() {
return new GreaterThanOrEqualRightNested(null);
}
public GreaterThanOrEqualRightNested withNewGreaterThanOrEqualRightLike(GreaterThanOrEqual item) {
return new GreaterThanOrEqualRightNested(item);
}
public A withNewGreaterThanOrEqualRight(Object left,Object right) {
return (A)withRight(new GreaterThanOrEqual(left, right));
}
public BitwiseAndRightNested withNewBitwiseAndRight() {
return new BitwiseAndRightNested(null);
}
public BitwiseAndRightNested withNewBitwiseAndRightLike(BitwiseAnd item) {
return new BitwiseAndRightNested(item);
}
public A withNewBitwiseAndRight(Object left,Object right) {
return (A)withRight(new BitwiseAnd(left, right));
}
public MinusRightNested withNewMinusRight() {
return new MinusRightNested(null);
}
public MinusRightNested withNewMinusRightLike(Minus item) {
return new MinusRightNested(item);
}
public A withNewMinusRight(Object left,Object right) {
return (A)withRight(new Minus(left, right));
}
public LogicalOrRightNested withNewLogicalOrRight() {
return new LogicalOrRightNested(null);
}
public LogicalOrRightNested withNewLogicalOrRightLike(LogicalOr item) {
return new LogicalOrRightNested(item);
}
public A withNewLogicalOrRight(Object left,Object right) {
return (A)withRight(new LogicalOr(left, right));
}
public NotEqualsRightNested withNewNotEqualsRight() {
return new NotEqualsRightNested(null);
}
public NotEqualsRightNested withNewNotEqualsRightLike(NotEquals item) {
return new NotEqualsRightNested(item);
}
public A withNewNotEqualsRight(Object left,Object right) {
return (A)withRight(new NotEquals(left, right));
}
public DivideRightNested withNewDivideRight() {
return new DivideRightNested(null);
}
public DivideRightNested withNewDivideRightLike(Divide item) {
return new DivideRightNested(item);
}
public A withNewDivideRight(Object left,Object right) {
return (A)withRight(new Divide(left, right));
}
public LessThanRightNested withNewLessThanRight() {
return new LessThanRightNested(null);
}
public LessThanRightNested withNewLessThanRightLike(LessThan item) {
return new LessThanRightNested(item);
}
public A withNewLessThanRight(Object left,Object right) {
return (A)withRight(new LessThan(left, right));
}
public BitwiseOrRightNested withNewBitwiseOrRight() {
return new BitwiseOrRightNested(null);
}
public BitwiseOrRightNested withNewBitwiseOrRightLike(BitwiseOr item) {
return new BitwiseOrRightNested(item);
}
public A withNewBitwiseOrRight(Object left,Object right) {
return (A)withRight(new BitwiseOr(left, right));
}
public PropertyRefRightNested withNewPropertyRefRight() {
return new PropertyRefRightNested(null);
}
public PropertyRefRightNested withNewPropertyRefRightLike(PropertyRef item) {
return new PropertyRefRightNested(item);
}
public RightShiftRightNested withNewRightShiftRight() {
return new RightShiftRightNested(null);
}
public RightShiftRightNested withNewRightShiftRightLike(RightShift item) {
return new RightShiftRightNested(item);
}
public A withNewRightShiftRight(Object left,Object right) {
return (A)withRight(new RightShift(left, right));
}
public GreaterThanRightNested withNewGreaterThanRight() {
return new GreaterThanRightNested(null);
}
public GreaterThanRightNested withNewGreaterThanRightLike(GreaterThan item) {
return new GreaterThanRightNested(item);
}
public A withNewGreaterThanRight(Object left,Object right) {
return (A)withRight(new GreaterThan(left, right));
}
public DeclareRightNested withNewDeclareRight() {
return new DeclareRightNested(null);
}
public DeclareRightNested withNewDeclareRightLike(Declare item) {
return new DeclareRightNested(item);
}
public A withNewDeclareRight(Class type,String name) {
return (A)withRight(new Declare(type, name));
}
public A withNewDeclareRight(Class type,String name,Object value) {
return (A)withRight(new Declare(type, name, value));
}
public CastRightNested withNewCastRight() {
return new CastRightNested(null);
}
public CastRightNested withNewCastRightLike(Cast item) {
return new CastRightNested(item);
}
public ModuloRightNested withNewModuloRight() {
return new ModuloRightNested(null);
}
public ModuloRightNested withNewModuloRightLike(Modulo item) {
return new ModuloRightNested(item);
}
public A withNewModuloRight(Object left,Object right) {
return (A)withRight(new Modulo(left, right));
}
public ValueRefRightNested withNewValueRefRight() {
return new ValueRefRightNested(null);
}
public ValueRefRightNested withNewValueRefRightLike(ValueRef item) {
return new ValueRefRightNested(item);
}
public A withNewValueRefRight(Object value) {
return (A)withRight(new ValueRef(value));
}
public LeftShiftRightNested withNewLeftShiftRight() {
return new LeftShiftRightNested(null);
}
public LeftShiftRightNested withNewLeftShiftRightLike(LeftShift item) {
return new LeftShiftRightNested(item);
}
public A withNewLeftShiftRight(Object left,Object right) {
return (A)withRight(new LeftShift(left, right));
}
public TernaryRightNested withNewTernaryRight() {
return new TernaryRightNested(null);
}
public TernaryRightNested withNewTernaryRightLike(Ternary item) {
return new TernaryRightNested(item);
}
public BinaryExpressionRightNested withNewBinaryExpressionRight() {
return new BinaryExpressionRightNested(null);
}
public BinaryExpressionRightNested withNewBinaryExpressionRightLike(BinaryExpression item) {
return new BinaryExpressionRightNested(item);
}
public EqualsRightNested withNewEqualsRight() {
return new EqualsRightNested(null);
}
public EqualsRightNested withNewEqualsRightLike(Equals item) {
return new EqualsRightNested(item);
}
public A withNewEqualsRight(Object left,Object right) {
return (A)withRight(new Equals(left, right));
}
public EnclosedRightNested withNewEnclosedRight() {
return new EnclosedRightNested(null);
}
public EnclosedRightNested withNewEnclosedRightLike(Enclosed item) {
return new EnclosedRightNested(item);
}
public PreDecrementRightNested withNewPreDecrementRight() {
return new PreDecrementRightNested(null);
}
public PreDecrementRightNested withNewPreDecrementRightLike(PreDecrement item) {
return new PreDecrementRightNested(item);
}
public PostDecrementRightNested withNewPostDecrementRight() {
return new PostDecrementRightNested(null);
}
public PostDecrementRightNested withNewPostDecrementRightLike(PostDecrement item) {
return new PostDecrementRightNested(item);
}
public LambdaRightNested withNewLambdaRight() {
return new LambdaRightNested(null);
}
public LambdaRightNested withNewLambdaRightLike(Lambda item) {
return new LambdaRightNested(item);
}
public NotRightNested withNewNotRight() {
return new NotRightNested(null);
}
public NotRightNested withNewNotRightLike(Not item) {
return new NotRightNested(item);
}
public ThisRightNested withNewThisRight() {
return new ThisRightNested(null);
}
public ThisRightNested withNewThisRightLike(This item) {
return new ThisRightNested(item);
}
public NegativeRightNested withNewNegativeRight() {
return new NegativeRightNested(null);
}
public NegativeRightNested withNewNegativeRightLike(Negative item) {
return new NegativeRightNested(item);
}
public AssignRightNested withNewAssignRight() {
return new AssignRightNested(null);
}
public AssignRightNested withNewAssignRightLike(Assign item) {
return new AssignRightNested(item);
}
public LogicalAndRightNested withNewLogicalAndRight() {
return new LogicalAndRightNested(null);
}
public LogicalAndRightNested withNewLogicalAndRightLike(LogicalAnd item) {
return new LogicalAndRightNested(item);
}
public A withNewLogicalAndRight(Object left,Object right) {
return (A)withRight(new LogicalAnd(left, right));
}
public PostIncrementRightNested withNewPostIncrementRight() {
return new PostIncrementRightNested(null);
}
public PostIncrementRightNested withNewPostIncrementRightLike(PostIncrement item) {
return new PostIncrementRightNested(item);
}
public RightUnsignedShiftRightNested withNewRightUnsignedShiftRight() {
return new RightUnsignedShiftRightNested(null);
}
public RightUnsignedShiftRightNested withNewRightUnsignedShiftRightLike(RightUnsignedShift item) {
return new RightUnsignedShiftRightNested(item);
}
public A withNewRightUnsignedShiftRight(Object left,Object right) {
return (A)withRight(new RightUnsignedShift(left, right));
}
public PlusRightNested withNewPlusRight() {
return new PlusRightNested(null);
}
public PlusRightNested withNewPlusRightLike(Plus item) {
return new PlusRightNested(item);
}
public A withNewPlusRight(Object left,Object right) {
return (A)withRight(new Plus(left, right));
}
public ConstructRightNested withNewConstructRight() {
return new ConstructRightNested(null);
}
public ConstructRightNested withNewConstructRightLike(Construct item) {
return new ConstructRightNested(item);
}
public XorRightNested withNewXorRight() {
return new XorRightNested(null);
}
public XorRightNested withNewXorRightLike(Xor item) {
return new XorRightNested(item);
}
public A withNewXorRight(Object left,Object right) {
return (A)withRight(new Xor(left, right));
}
public PreIncrementRightNested withNewPreIncrementRight() {
return new PreIncrementRightNested(null);
}
public PreIncrementRightNested withNewPreIncrementRightLike(PreIncrement item) {
return new PreIncrementRightNested(item);
}
public LessThanOrEqualRightNested withNewLessThanOrEqualRight() {
return new LessThanOrEqualRightNested(null);
}
public LessThanOrEqualRightNested withNewLessThanOrEqualRightLike(LessThanOrEqual item) {
return new LessThanOrEqualRightNested(item);
}
public A withNewLessThanOrEqualRight(Object left,Object right) {
return (A)withRight(new LessThanOrEqual(left, right));
}
public PositiveRightNested withNewPositiveRight() {
return new PositiveRightNested(null);
}
public PositiveRightNested withNewPositiveRightLike(Positive item) {
return new PositiveRightNested(item);
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
BinaryExpressionFluent that = (BinaryExpressionFluent) o;
if (!java.util.Objects.equals(left, that.left)) return false;
if (!java.util.Objects.equals(right, that.right)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(left, right, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (left != null) { sb.append("left:"); sb.append(left + ","); }
if (right != null) { sb.append("right:"); sb.append(right); }
sb.append("}");
return sb.toString();
}
protected static VisitableBuilder builder(Object item) {
switch (item.getClass().getName()) {
case "io.sundr.model."+"Multiply": return (VisitableBuilder)new MultiplyBuilder((Multiply) item);
case "io.sundr.model."+"NewArray": return (VisitableBuilder)new NewArrayBuilder((NewArray) item);
case "io.sundr.model."+"InstanceOf": return (VisitableBuilder)new InstanceOfBuilder((InstanceOf) item);
case "io.sundr.model."+"MethodCall": return (VisitableBuilder)new MethodCallBuilder((MethodCall) item);
case "io.sundr.model."+"Inverse": return (VisitableBuilder)new InverseBuilder((Inverse) item);
case "io.sundr.model."+"Index": return (VisitableBuilder)new IndexBuilder((Index) item);
case "io.sundr.model."+"GreaterThanOrEqual": return (VisitableBuilder)new GreaterThanOrEqualBuilder((GreaterThanOrEqual) item);
case "io.sundr.model."+"BitwiseAnd": return (VisitableBuilder)new BitwiseAndBuilder((BitwiseAnd) item);
case "io.sundr.model."+"Minus": return (VisitableBuilder)new MinusBuilder((Minus) item);
case "io.sundr.model."+"LogicalOr": return (VisitableBuilder)new LogicalOrBuilder((LogicalOr) item);
case "io.sundr.model."+"NotEquals": return (VisitableBuilder)new NotEqualsBuilder((NotEquals) item);
case "io.sundr.model."+"Divide": return (VisitableBuilder)new DivideBuilder((Divide) item);
case "io.sundr.model."+"LessThan": return (VisitableBuilder)new LessThanBuilder((LessThan) item);
case "io.sundr.model."+"BitwiseOr": return (VisitableBuilder)new BitwiseOrBuilder((BitwiseOr) item);
case "io.sundr.model."+"PropertyRef": return (VisitableBuilder)new PropertyRefBuilder((PropertyRef) item);
case "io.sundr.model."+"RightShift": return (VisitableBuilder)new RightShiftBuilder((RightShift) item);
case "io.sundr.model."+"GreaterThan": return (VisitableBuilder)new GreaterThanBuilder((GreaterThan) item);
case "io.sundr.model."+"Declare": return (VisitableBuilder)new DeclareBuilder((Declare) item);
case "io.sundr.model."+"Cast": return (VisitableBuilder)new CastBuilder((Cast) item);
case "io.sundr.model."+"Modulo": return (VisitableBuilder)new ModuloBuilder((Modulo) item);
case "io.sundr.model."+"ValueRef": return (VisitableBuilder)new ValueRefBuilder((ValueRef) item);
case "io.sundr.model."+"LeftShift": return (VisitableBuilder)new LeftShiftBuilder((LeftShift) item);
case "io.sundr.model."+"Ternary": return (VisitableBuilder)new TernaryBuilder((Ternary) item);
case "io.sundr.model."+"BinaryExpression": return (VisitableBuilder)new BinaryExpressionBuilder((BinaryExpression) item);
case "io.sundr.model."+"Equals": return (VisitableBuilder)new EqualsBuilder((Equals) item);
case "io.sundr.model."+"Enclosed": return (VisitableBuilder)new EnclosedBuilder((Enclosed) item);
case "io.sundr.model."+"PreDecrement": return (VisitableBuilder)new PreDecrementBuilder((PreDecrement) item);
case "io.sundr.model."+"PostDecrement": return (VisitableBuilder)new PostDecrementBuilder((PostDecrement) item);
case "io.sundr.model."+"Lambda": return (VisitableBuilder)new LambdaBuilder((Lambda) item);
case "io.sundr.model."+"Not": return (VisitableBuilder)new NotBuilder((Not) item);
case "io.sundr.model."+"This": return (VisitableBuilder)new ThisBuilder((This) item);
case "io.sundr.model."+"Negative": return (VisitableBuilder)new NegativeBuilder((Negative) item);
case "io.sundr.model."+"Assign": return (VisitableBuilder)new AssignBuilder((Assign) item);
case "io.sundr.model."+"LogicalAnd": return (VisitableBuilder)new LogicalAndBuilder((LogicalAnd) item);
case "io.sundr.model."+"PostIncrement": return (VisitableBuilder)new PostIncrementBuilder((PostIncrement) item);
case "io.sundr.model."+"RightUnsignedShift": return (VisitableBuilder)new RightUnsignedShiftBuilder((RightUnsignedShift) item);
case "io.sundr.model."+"Plus": return (VisitableBuilder)new PlusBuilder((Plus) item);
case "io.sundr.model."+"Construct": return (VisitableBuilder)new ConstructBuilder((Construct) item);
case "io.sundr.model."+"Xor": return (VisitableBuilder)new XorBuilder((Xor) item);
case "io.sundr.model."+"PreIncrement": return (VisitableBuilder)new PreIncrementBuilder((PreIncrement) item);
case "io.sundr.model."+"LessThanOrEqual": return (VisitableBuilder)new LessThanOrEqualBuilder((LessThanOrEqual) item);
case "io.sundr.model."+"Positive": return (VisitableBuilder)new PositiveBuilder((Positive) item);
}
return (VisitableBuilder)builderOf(item);
}
public class MultiplyLeftNested extends MultiplyFluent> implements Nested{
MultiplyLeftNested(Multiply item) {
this.builder = new MultiplyBuilder(this, item);
}
MultiplyBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endMultiplyLeft() {
return and();
}
}
public class NewArrayLeftNested extends NewArrayFluent> implements Nested{
NewArrayLeftNested(NewArray item) {
this.builder = new NewArrayBuilder(this, item);
}
NewArrayBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endNewArrayLeft() {
return and();
}
}
public class InstanceOfLeftNested extends InstanceOfFluent> implements Nested{
InstanceOfLeftNested(InstanceOf item) {
this.builder = new InstanceOfBuilder(this, item);
}
InstanceOfBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endInstanceOfLeft() {
return and();
}
}
public class MethodCallLeftNested extends MethodCallFluent> implements Nested{
MethodCallLeftNested(MethodCall item) {
this.builder = new MethodCallBuilder(this, item);
}
MethodCallBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endMethodCallLeft() {
return and();
}
}
public class InverseLeftNested extends InverseFluent> implements Nested{
InverseLeftNested(Inverse item) {
this.builder = new InverseBuilder(this, item);
}
InverseBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endInverseLeft() {
return and();
}
}
public class IndexLeftNested extends IndexFluent> implements Nested{
IndexLeftNested(Index item) {
this.builder = new IndexBuilder(this, item);
}
IndexBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endIndexLeft() {
return and();
}
}
public class GreaterThanOrEqualLeftNested extends GreaterThanOrEqualFluent> implements Nested{
GreaterThanOrEqualLeftNested(GreaterThanOrEqual item) {
this.builder = new GreaterThanOrEqualBuilder(this, item);
}
GreaterThanOrEqualBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endGreaterThanOrEqualLeft() {
return and();
}
}
public class BitwiseAndLeftNested extends BitwiseAndFluent> implements Nested{
BitwiseAndLeftNested(BitwiseAnd item) {
this.builder = new BitwiseAndBuilder(this, item);
}
BitwiseAndBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endBitwiseAndLeft() {
return and();
}
}
public class MinusLeftNested extends MinusFluent> implements Nested{
MinusLeftNested(Minus item) {
this.builder = new MinusBuilder(this, item);
}
MinusBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endMinusLeft() {
return and();
}
}
public class LogicalOrLeftNested extends LogicalOrFluent> implements Nested{
LogicalOrLeftNested(LogicalOr item) {
this.builder = new LogicalOrBuilder(this, item);
}
LogicalOrBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endLogicalOrLeft() {
return and();
}
}
public class NotEqualsLeftNested extends NotEqualsFluent> implements Nested{
NotEqualsLeftNested(NotEquals item) {
this.builder = new NotEqualsBuilder(this, item);
}
NotEqualsBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endNotEqualsLeft() {
return and();
}
}
public class DivideLeftNested extends DivideFluent> implements Nested{
DivideLeftNested(Divide item) {
this.builder = new DivideBuilder(this, item);
}
DivideBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endDivideLeft() {
return and();
}
}
public class LessThanLeftNested extends LessThanFluent> implements Nested{
LessThanLeftNested(LessThan item) {
this.builder = new LessThanBuilder(this, item);
}
LessThanBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endLessThanLeft() {
return and();
}
}
public class BitwiseOrLeftNested extends BitwiseOrFluent> implements Nested{
BitwiseOrLeftNested(BitwiseOr item) {
this.builder = new BitwiseOrBuilder(this, item);
}
BitwiseOrBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endBitwiseOrLeft() {
return and();
}
}
public class PropertyRefLeftNested extends PropertyRefFluent> implements Nested{
PropertyRefLeftNested(PropertyRef item) {
this.builder = new PropertyRefBuilder(this, item);
}
PropertyRefBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endPropertyRefLeft() {
return and();
}
}
public class RightShiftLeftNested extends RightShiftFluent> implements Nested{
RightShiftLeftNested(RightShift item) {
this.builder = new RightShiftBuilder(this, item);
}
RightShiftBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endRightShiftLeft() {
return and();
}
}
public class GreaterThanLeftNested extends GreaterThanFluent> implements Nested{
GreaterThanLeftNested(GreaterThan item) {
this.builder = new GreaterThanBuilder(this, item);
}
GreaterThanBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endGreaterThanLeft() {
return and();
}
}
public class DeclareLeftNested extends DeclareFluent> implements Nested{
DeclareLeftNested(Declare item) {
this.builder = new DeclareBuilder(this, item);
}
DeclareBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endDeclareLeft() {
return and();
}
}
public class CastLeftNested extends CastFluent> implements Nested{
CastLeftNested(Cast item) {
this.builder = new CastBuilder(this, item);
}
CastBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endCastLeft() {
return and();
}
}
public class ModuloLeftNested extends ModuloFluent> implements Nested{
ModuloLeftNested(Modulo item) {
this.builder = new ModuloBuilder(this, item);
}
ModuloBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endModuloLeft() {
return and();
}
}
public class ValueRefLeftNested extends ValueRefFluent> implements Nested{
ValueRefLeftNested(ValueRef item) {
this.builder = new ValueRefBuilder(this, item);
}
ValueRefBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endValueRefLeft() {
return and();
}
}
public class LeftShiftLeftNested extends LeftShiftFluent> implements Nested{
LeftShiftLeftNested(LeftShift item) {
this.builder = new LeftShiftBuilder(this, item);
}
LeftShiftBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endLeftShiftLeft() {
return and();
}
}
public class TernaryLeftNested extends TernaryFluent> implements Nested{
TernaryLeftNested(Ternary item) {
this.builder = new TernaryBuilder(this, item);
}
TernaryBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endTernaryLeft() {
return and();
}
}
public class BinaryExpressionLeftNested extends BinaryExpressionFluent> implements Nested{
BinaryExpressionLeftNested(BinaryExpression item) {
this.builder = new BinaryExpressionBuilder(this, item);
}
BinaryExpressionBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endBinaryExpressionLeft() {
return and();
}
}
public class EqualsLeftNested extends EqualsFluent> implements Nested{
EqualsLeftNested(Equals item) {
this.builder = new EqualsBuilder(this, item);
}
EqualsBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endEqualsLeft() {
return and();
}
}
public class EnclosedLeftNested extends EnclosedFluent> implements Nested{
EnclosedLeftNested(Enclosed item) {
this.builder = new EnclosedBuilder(this, item);
}
EnclosedBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endEnclosedLeft() {
return and();
}
}
public class PreDecrementLeftNested extends PreDecrementFluent> implements Nested{
PreDecrementLeftNested(PreDecrement item) {
this.builder = new PreDecrementBuilder(this, item);
}
PreDecrementBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endPreDecrementLeft() {
return and();
}
}
public class PostDecrementLeftNested extends PostDecrementFluent> implements Nested{
PostDecrementLeftNested(PostDecrement item) {
this.builder = new PostDecrementBuilder(this, item);
}
PostDecrementBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endPostDecrementLeft() {
return and();
}
}
public class LambdaLeftNested extends LambdaFluent> implements Nested{
LambdaLeftNested(Lambda item) {
this.builder = new LambdaBuilder(this, item);
}
LambdaBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endLambdaLeft() {
return and();
}
}
public class NotLeftNested extends NotFluent> implements Nested{
NotLeftNested(Not item) {
this.builder = new NotBuilder(this, item);
}
NotBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endNotLeft() {
return and();
}
}
public class ThisLeftNested extends ThisFluent> implements Nested{
ThisLeftNested(This item) {
this.builder = new ThisBuilder(this, item);
}
ThisBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endThisLeft() {
return and();
}
}
public class NegativeLeftNested extends NegativeFluent> implements Nested{
NegativeLeftNested(Negative item) {
this.builder = new NegativeBuilder(this, item);
}
NegativeBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endNegativeLeft() {
return and();
}
}
public class AssignLeftNested extends AssignFluent> implements Nested{
AssignLeftNested(Assign item) {
this.builder = new AssignBuilder(this, item);
}
AssignBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endAssignLeft() {
return and();
}
}
public class LogicalAndLeftNested extends LogicalAndFluent> implements Nested{
LogicalAndLeftNested(LogicalAnd item) {
this.builder = new LogicalAndBuilder(this, item);
}
LogicalAndBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endLogicalAndLeft() {
return and();
}
}
public class PostIncrementLeftNested extends PostIncrementFluent> implements Nested{
PostIncrementLeftNested(PostIncrement item) {
this.builder = new PostIncrementBuilder(this, item);
}
PostIncrementBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endPostIncrementLeft() {
return and();
}
}
public class RightUnsignedShiftLeftNested extends RightUnsignedShiftFluent> implements Nested{
RightUnsignedShiftLeftNested(RightUnsignedShift item) {
this.builder = new RightUnsignedShiftBuilder(this, item);
}
RightUnsignedShiftBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endRightUnsignedShiftLeft() {
return and();
}
}
public class PlusLeftNested extends PlusFluent> implements Nested{
PlusLeftNested(Plus item) {
this.builder = new PlusBuilder(this, item);
}
PlusBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endPlusLeft() {
return and();
}
}
public class ConstructLeftNested extends ConstructFluent> implements Nested{
ConstructLeftNested(Construct item) {
this.builder = new ConstructBuilder(this, item);
}
ConstructBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endConstructLeft() {
return and();
}
}
public class XorLeftNested extends XorFluent> implements Nested{
XorLeftNested(Xor item) {
this.builder = new XorBuilder(this, item);
}
XorBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endXorLeft() {
return and();
}
}
public class PreIncrementLeftNested extends PreIncrementFluent> implements Nested{
PreIncrementLeftNested(PreIncrement item) {
this.builder = new PreIncrementBuilder(this, item);
}
PreIncrementBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endPreIncrementLeft() {
return and();
}
}
public class LessThanOrEqualLeftNested extends LessThanOrEqualFluent> implements Nested{
LessThanOrEqualLeftNested(LessThanOrEqual item) {
this.builder = new LessThanOrEqualBuilder(this, item);
}
LessThanOrEqualBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endLessThanOrEqualLeft() {
return and();
}
}
public class PositiveLeftNested extends PositiveFluent> implements Nested{
PositiveLeftNested(Positive item) {
this.builder = new PositiveBuilder(this, item);
}
PositiveBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withLeft(builder.build());
}
public N endPositiveLeft() {
return and();
}
}
public class MultiplyRightNested extends MultiplyFluent> implements Nested{
MultiplyRightNested(Multiply item) {
this.builder = new MultiplyBuilder(this, item);
}
MultiplyBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endMultiplyRight() {
return and();
}
}
public class NewArrayRightNested extends NewArrayFluent> implements Nested{
NewArrayRightNested(NewArray item) {
this.builder = new NewArrayBuilder(this, item);
}
NewArrayBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endNewArrayRight() {
return and();
}
}
public class InstanceOfRightNested extends InstanceOfFluent> implements Nested{
InstanceOfRightNested(InstanceOf item) {
this.builder = new InstanceOfBuilder(this, item);
}
InstanceOfBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endInstanceOfRight() {
return and();
}
}
public class MethodCallRightNested extends MethodCallFluent> implements Nested{
MethodCallRightNested(MethodCall item) {
this.builder = new MethodCallBuilder(this, item);
}
MethodCallBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endMethodCallRight() {
return and();
}
}
public class InverseRightNested extends InverseFluent> implements Nested{
InverseRightNested(Inverse item) {
this.builder = new InverseBuilder(this, item);
}
InverseBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endInverseRight() {
return and();
}
}
public class IndexRightNested extends IndexFluent> implements Nested{
IndexRightNested(Index item) {
this.builder = new IndexBuilder(this, item);
}
IndexBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endIndexRight() {
return and();
}
}
public class GreaterThanOrEqualRightNested extends GreaterThanOrEqualFluent> implements Nested{
GreaterThanOrEqualRightNested(GreaterThanOrEqual item) {
this.builder = new GreaterThanOrEqualBuilder(this, item);
}
GreaterThanOrEqualBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endGreaterThanOrEqualRight() {
return and();
}
}
public class BitwiseAndRightNested extends BitwiseAndFluent> implements Nested{
BitwiseAndRightNested(BitwiseAnd item) {
this.builder = new BitwiseAndBuilder(this, item);
}
BitwiseAndBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endBitwiseAndRight() {
return and();
}
}
public class MinusRightNested extends MinusFluent> implements Nested{
MinusRightNested(Minus item) {
this.builder = new MinusBuilder(this, item);
}
MinusBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endMinusRight() {
return and();
}
}
public class LogicalOrRightNested extends LogicalOrFluent> implements Nested{
LogicalOrRightNested(LogicalOr item) {
this.builder = new LogicalOrBuilder(this, item);
}
LogicalOrBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endLogicalOrRight() {
return and();
}
}
public class NotEqualsRightNested extends NotEqualsFluent> implements Nested{
NotEqualsRightNested(NotEquals item) {
this.builder = new NotEqualsBuilder(this, item);
}
NotEqualsBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endNotEqualsRight() {
return and();
}
}
public class DivideRightNested extends DivideFluent> implements Nested{
DivideRightNested(Divide item) {
this.builder = new DivideBuilder(this, item);
}
DivideBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endDivideRight() {
return and();
}
}
public class LessThanRightNested extends LessThanFluent> implements Nested{
LessThanRightNested(LessThan item) {
this.builder = new LessThanBuilder(this, item);
}
LessThanBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endLessThanRight() {
return and();
}
}
public class BitwiseOrRightNested extends BitwiseOrFluent> implements Nested{
BitwiseOrRightNested(BitwiseOr item) {
this.builder = new BitwiseOrBuilder(this, item);
}
BitwiseOrBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endBitwiseOrRight() {
return and();
}
}
public class PropertyRefRightNested extends PropertyRefFluent> implements Nested{
PropertyRefRightNested(PropertyRef item) {
this.builder = new PropertyRefBuilder(this, item);
}
PropertyRefBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endPropertyRefRight() {
return and();
}
}
public class RightShiftRightNested extends RightShiftFluent> implements Nested{
RightShiftRightNested(RightShift item) {
this.builder = new RightShiftBuilder(this, item);
}
RightShiftBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endRightShiftRight() {
return and();
}
}
public class GreaterThanRightNested extends GreaterThanFluent> implements Nested{
GreaterThanRightNested(GreaterThan item) {
this.builder = new GreaterThanBuilder(this, item);
}
GreaterThanBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endGreaterThanRight() {
return and();
}
}
public class DeclareRightNested extends DeclareFluent> implements Nested{
DeclareRightNested(Declare item) {
this.builder = new DeclareBuilder(this, item);
}
DeclareBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endDeclareRight() {
return and();
}
}
public class CastRightNested extends CastFluent> implements Nested{
CastRightNested(Cast item) {
this.builder = new CastBuilder(this, item);
}
CastBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endCastRight() {
return and();
}
}
public class ModuloRightNested extends ModuloFluent> implements Nested{
ModuloRightNested(Modulo item) {
this.builder = new ModuloBuilder(this, item);
}
ModuloBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endModuloRight() {
return and();
}
}
public class ValueRefRightNested extends ValueRefFluent> implements Nested{
ValueRefRightNested(ValueRef item) {
this.builder = new ValueRefBuilder(this, item);
}
ValueRefBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endValueRefRight() {
return and();
}
}
public class LeftShiftRightNested extends LeftShiftFluent> implements Nested{
LeftShiftRightNested(LeftShift item) {
this.builder = new LeftShiftBuilder(this, item);
}
LeftShiftBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endLeftShiftRight() {
return and();
}
}
public class TernaryRightNested extends TernaryFluent> implements Nested{
TernaryRightNested(Ternary item) {
this.builder = new TernaryBuilder(this, item);
}
TernaryBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endTernaryRight() {
return and();
}
}
public class BinaryExpressionRightNested extends BinaryExpressionFluent> implements Nested{
BinaryExpressionRightNested(BinaryExpression item) {
this.builder = new BinaryExpressionBuilder(this, item);
}
BinaryExpressionBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endBinaryExpressionRight() {
return and();
}
}
public class EqualsRightNested extends EqualsFluent> implements Nested{
EqualsRightNested(Equals item) {
this.builder = new EqualsBuilder(this, item);
}
EqualsBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endEqualsRight() {
return and();
}
}
public class EnclosedRightNested extends EnclosedFluent> implements Nested{
EnclosedRightNested(Enclosed item) {
this.builder = new EnclosedBuilder(this, item);
}
EnclosedBuilder builder;
public N and() {
return (N) BinaryExpressionFluent.this.withRight(builder.build());
}
public N endEnclosedRight() {
return and();
}
}
public class PreDecrementRightNested