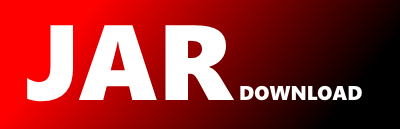
io.sundr.model.ClassRefFluent Maven / Gradle / Ivy
package io.sundr.model;
import io.sundr.builder.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.sundr.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.function.Predicate;
import java.util.Iterator;
import java.util.List;
import java.util.Collection;
import java.lang.Object;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class ClassRefFluent> extends TypeRefFluent{
public ClassRefFluent() {
}
public ClassRefFluent(ClassRef instance) {
this.copyInstance(instance);
}
private String fullyQualifiedName;
private int dimensions;
private ArrayList> arguments = new ArrayList>();
protected void copyInstance(ClassRef instance) {
if (instance != null) {
this.withFullyQualifiedName(instance.getFullyQualifiedName());
this.withDimensions(instance.getDimensions());
this.withArguments(instance.getArguments());
this.withAttributes(instance.getAttributes());
}
}
public String getFullyQualifiedName() {
return this.fullyQualifiedName;
}
public A withFullyQualifiedName(String fullyQualifiedName) {
this.fullyQualifiedName=fullyQualifiedName; return (A) this;
}
public boolean hasFullyQualifiedName() {
return this.fullyQualifiedName != null;
}
public int getDimensions() {
return this.dimensions;
}
public A withDimensions(int dimensions) {
this.dimensions=dimensions; return (A) this;
}
public boolean hasDimensions() {
return true;
}
public A addToArguments(VisitableBuilder extends TypeRef,?> builder) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
_visitables.get("arguments").add(builder);this.arguments.add(builder); return (A)this;
}
public A addToArguments(int index,VisitableBuilder extends TypeRef,?> builder) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
if (index < 0 || index >= arguments.size()) { _visitables.get("arguments").add(builder); arguments.add(builder); } else { _visitables.get("arguments").add(index, builder); arguments.add(index, builder);}
return (A)this;
}
public A addToArguments(int index,TypeRef item) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
VisitableBuilder extends TypeRef,?> builder = builder(item);
if (index < 0 || index >= arguments.size()) { _visitables.get("arguments").add(builder); arguments.add(builder); } else { _visitables.get("arguments").add(index, builder); arguments.add(index, builder);}
return (A)this;
}
public A setToArguments(int index,TypeRef item) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
VisitableBuilder extends TypeRef,?> builder = builder(item);
if (index < 0 || index >= arguments.size()) { _visitables.get("arguments").add(builder); arguments.add(builder); } else { _visitables.get("arguments").set(index, builder); arguments.set(index, builder);}
return (A)this;
}
public A addToArguments(io.sundr.model.TypeRef... items) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
for (TypeRef item : items) { VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("arguments").add(builder);this.arguments.add(builder); }
return (A)this;
}
public A addAllToArguments(Collection items) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
for (TypeRef item : items) { VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("arguments").add(builder);this.arguments.add(builder); }
return (A)this;
}
public A removeFromArguments(VisitableBuilder extends TypeRef,?> builder) {
if (this.arguments == null) return (A)this;
_visitables.get("arguments").remove(builder);this.arguments.remove(builder); return (A)this;
}
public A removeFromArguments(io.sundr.model.TypeRef... items) {
if (this.arguments == null) return (A)this;
for (TypeRef item : items) {
VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("arguments").remove(builder);this.arguments.remove(builder);
} return (A)this;
}
public A removeAllFromArguments(Collection items) {
if (this.arguments == null) return (A)this;
for (TypeRef item : items) {
VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("arguments").remove(builder);this.arguments.remove(builder);
} return (A)this;
}
public A removeMatchingFromArguments(Predicate> predicate) {
if (arguments == null) return (A) this;
final Iterator> each = arguments.iterator();
final List visitables = _visitables.get("arguments");
while (each.hasNext()) {
VisitableBuilder extends TypeRef,?> builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildArguments() {
return build(arguments);
}
public TypeRef buildArgument(int index) {
return this.arguments.get(index).build();
}
public TypeRef buildFirstArgument() {
return this.arguments.get(0).build();
}
public TypeRef buildLastArgument() {
return this.arguments.get(arguments.size() - 1).build();
}
public TypeRef buildMatchingArgument(Predicate> predicate) {
for (VisitableBuilder extends TypeRef,?> item: arguments) { if(predicate.test(item)){ return item.build();} } return null;
}
public boolean hasMatchingArgument(Predicate> predicate) {
for (VisitableBuilder extends TypeRef,?> item: arguments) { if(predicate.test(item)){ return true;} } return false;
}
public A withArguments(List arguments) {
if (arguments != null) {this.arguments = new ArrayList(); for (TypeRef item : arguments){this.addToArguments(item);}} else { this.arguments = null;} return (A) this;
}
public A withArguments(io.sundr.model.TypeRef... arguments) {
if (this.arguments != null) {this.arguments.clear(); _visitables.remove("arguments"); }
if (arguments != null) {for (TypeRef item :arguments){ this.addToArguments(item);}} return (A) this;
}
public boolean hasArguments() {
return arguments != null && !arguments.isEmpty();
}
public ClassRefArgumentsNested addNewClassRefArgument() {
return new ClassRefArgumentsNested(-1, null);
}
public ClassRefArgumentsNested addNewClassRefArgumentLike(ClassRef item) {
return new ClassRefArgumentsNested(-1, item);
}
public ClassRefArgumentsNested setNewClassRefArgumentLike(int index,ClassRef item) {
return new ClassRefArgumentsNested(index, item);
}
public PrimitiveRefArgumentsNested addNewPrimitiveRefArgument() {
return new PrimitiveRefArgumentsNested(-1, null);
}
public PrimitiveRefArgumentsNested addNewPrimitiveRefArgumentLike(PrimitiveRef item) {
return new PrimitiveRefArgumentsNested(-1, item);
}
public PrimitiveRefArgumentsNested setNewPrimitiveRefArgumentLike(int index,PrimitiveRef item) {
return new PrimitiveRefArgumentsNested(index, item);
}
public VoidRefArgumentsNested addNewVoidRefArgument() {
return new VoidRefArgumentsNested(-1, null);
}
public VoidRefArgumentsNested addNewVoidRefArgumentLike(VoidRef item) {
return new VoidRefArgumentsNested(-1, item);
}
public VoidRefArgumentsNested setNewVoidRefArgumentLike(int index,VoidRef item) {
return new VoidRefArgumentsNested(index, item);
}
public TypeParamRefArgumentsNested addNewTypeParamRefArgument() {
return new TypeParamRefArgumentsNested(-1, null);
}
public TypeParamRefArgumentsNested addNewTypeParamRefArgumentLike(TypeParamRef item) {
return new TypeParamRefArgumentsNested(-1, item);
}
public TypeParamRefArgumentsNested setNewTypeParamRefArgumentLike(int index,TypeParamRef item) {
return new TypeParamRefArgumentsNested(index, item);
}
public WildcardRefArgumentsNested addNewWildcardRefArgument() {
return new WildcardRefArgumentsNested(-1, null);
}
public WildcardRefArgumentsNested addNewWildcardRefArgumentLike(WildcardRef item) {
return new WildcardRefArgumentsNested(-1, item);
}
public WildcardRefArgumentsNested setNewWildcardRefArgumentLike(int index,WildcardRef item) {
return new WildcardRefArgumentsNested(index, item);
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ClassRefFluent that = (ClassRefFluent) o;
if (!java.util.Objects.equals(fullyQualifiedName, that.fullyQualifiedName)) return false;
if (dimensions != that.dimensions) return false;
if (!java.util.Objects.equals(arguments, that.arguments)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(fullyQualifiedName, dimensions, arguments, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (fullyQualifiedName != null) { sb.append("fullyQualifiedName:"); sb.append(fullyQualifiedName + ","); }
sb.append("dimensions:"); sb.append(dimensions + ",");
if (arguments != null && !arguments.isEmpty()) { sb.append("arguments:"); sb.append(arguments); }
sb.append("}");
return sb.toString();
}
protected static VisitableBuilder builder(Object item) {
switch (item.getClass().getName()) {
case "io.sundr.model."+"ClassRef": return (VisitableBuilder)new ClassRefBuilder((ClassRef) item);
case "io.sundr.model."+"PrimitiveRef": return (VisitableBuilder)new PrimitiveRefBuilder((PrimitiveRef) item);
case "io.sundr.model."+"VoidRef": return (VisitableBuilder)new VoidRefBuilder((VoidRef) item);
case "io.sundr.model."+"TypeParamRef": return (VisitableBuilder)new TypeParamRefBuilder((TypeParamRef) item);
case "io.sundr.model."+"WildcardRef": return (VisitableBuilder)new WildcardRefBuilder((WildcardRef) item);
}
return (VisitableBuilder)builderOf(item);
}
public class ClassRefArgumentsNested extends ClassRefFluent> implements Nested{
ClassRefArgumentsNested(int index,ClassRef item) {
this.index = index;
this.builder = new ClassRefBuilder(this, item);
}
ClassRefBuilder builder;
int index;
public N and() {
return (N) ClassRefFluent.this.setToArguments(index,builder.build());
}
public N endClassRefArgument() {
return and();
}
}
public class PrimitiveRefArgumentsNested extends PrimitiveRefFluent> implements Nested{
PrimitiveRefArgumentsNested(int index,PrimitiveRef item) {
this.index = index;
this.builder = new PrimitiveRefBuilder(this, item);
}
PrimitiveRefBuilder builder;
int index;
public N and() {
return (N) ClassRefFluent.this.setToArguments(index,builder.build());
}
public N endPrimitiveRefArgument() {
return and();
}
}
public class VoidRefArgumentsNested extends VoidRefFluent> implements Nested{
VoidRefArgumentsNested(int index,VoidRef item) {
this.index = index;
this.builder = new VoidRefBuilder(this, item);
}
VoidRefBuilder builder;
int index;
public N and() {
return (N) ClassRefFluent.this.setToArguments(index,builder.build());
}
public N endVoidRefArgument() {
return and();
}
}
public class TypeParamRefArgumentsNested extends TypeParamRefFluent> implements Nested{
TypeParamRefArgumentsNested(int index,TypeParamRef item) {
this.index = index;
this.builder = new TypeParamRefBuilder(this, item);
}
TypeParamRefBuilder builder;
int index;
public N and() {
return (N) ClassRefFluent.this.setToArguments(index,builder.build());
}
public N endTypeParamRefArgument() {
return and();
}
}
public class WildcardRefArgumentsNested extends WildcardRefFluent> implements Nested{
WildcardRefArgumentsNested(int index,WildcardRef item) {
this.index = index;
this.builder = new WildcardRefBuilder(this, item);
}
WildcardRefBuilder builder;
int index;
public N and() {
return (N) ClassRefFluent.this.setToArguments(index,builder.build());
}
public N endWildcardRefArgument() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy