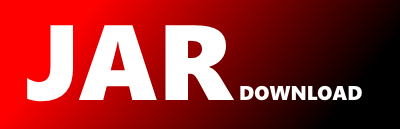
io.sundr.model.DeclareFluent Maven / Gradle / Ivy
package io.sundr.model;
import io.sundr.builder.VisitableBuilder;
import java.util.Optional;
import java.lang.SuppressWarnings;
import io.sundr.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.function.Predicate;
import io.sundr.builder.BaseFluent;
import java.util.Iterator;
import java.util.Collection;
import java.lang.Object;
import java.util.List;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class DeclareFluent> extends BaseFluent{
public DeclareFluent() {
}
public DeclareFluent(Declare instance) {
this.copyInstance(instance);
}
private ArrayList properties = new ArrayList();
private Optional value = Optional.empty();
protected void copyInstance(Declare instance) {
if (instance != null) {
this.withProperties(instance.getProperties());
this.withValue(instance.getValue());
}
}
public A addToProperties(int index,Property item) {
if (this.properties == null) {this.properties = new ArrayList();}
PropertyBuilder builder = new PropertyBuilder(item);
if (index < 0 || index >= properties.size()) { _visitables.get("properties").add(builder); properties.add(builder); } else { _visitables.get("properties").add(index, builder); properties.add(index, builder);}
return (A)this;
}
public A setToProperties(int index,Property item) {
if (this.properties == null) {this.properties = new ArrayList();}
PropertyBuilder builder = new PropertyBuilder(item);
if (index < 0 || index >= properties.size()) { _visitables.get("properties").add(builder); properties.add(builder); } else { _visitables.get("properties").set(index, builder); properties.set(index, builder);}
return (A)this;
}
public A addToProperties(io.sundr.model.Property... items) {
if (this.properties == null) {this.properties = new ArrayList();}
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("properties").add(builder);this.properties.add(builder);} return (A)this;
}
public A addAllToProperties(Collection items) {
if (this.properties == null) {this.properties = new ArrayList();}
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("properties").add(builder);this.properties.add(builder);} return (A)this;
}
public A removeFromProperties(io.sundr.model.Property... items) {
if (this.properties == null) return (A)this;
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("properties").remove(builder); this.properties.remove(builder);} return (A)this;
}
public A removeAllFromProperties(Collection items) {
if (this.properties == null) return (A)this;
for (Property item : items) {PropertyBuilder builder = new PropertyBuilder(item);_visitables.get("properties").remove(builder); this.properties.remove(builder);} return (A)this;
}
public A removeMatchingFromProperties(Predicate predicate) {
if (properties == null) return (A) this;
final Iterator each = properties.iterator();
final List visitables = _visitables.get("properties");
while (each.hasNext()) {
PropertyBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildProperties() {
return properties != null ? build(properties) : null;
}
public Property buildProperty(int index) {
return this.properties.get(index).build();
}
public Property buildFirstProperty() {
return this.properties.get(0).build();
}
public Property buildLastProperty() {
return this.properties.get(properties.size() - 1).build();
}
public Property buildMatchingProperty(Predicate predicate) {
for (PropertyBuilder item: properties) { if(predicate.test(item)){ return item.build();} } return null;
}
public boolean hasMatchingProperty(Predicate predicate) {
for (PropertyBuilder item: properties) { if(predicate.test(item)){ return true;} } return false;
}
public A withProperties(List properties) {
if (this.properties != null) { _visitables.get("properties").clear();}
if (properties != null) {this.properties = new ArrayList(); for (Property item : properties){this.addToProperties(item);}} else { this.properties = null;} return (A) this;
}
public A withProperties(io.sundr.model.Property... properties) {
if (this.properties != null) {this.properties.clear(); _visitables.remove("properties"); }
if (properties != null) {for (Property item :properties){ this.addToProperties(item);}} return (A) this;
}
public boolean hasProperties() {
return properties != null && !properties.isEmpty();
}
public PropertiesNested addNewProperty() {
return new PropertiesNested(-1, null);
}
public PropertiesNested addNewPropertyLike(Property item) {
return new PropertiesNested(-1, item);
}
public PropertiesNested setNewPropertyLike(int index,Property item) {
return new PropertiesNested(index, item);
}
public PropertiesNested editProperty(int index) {
if (properties.size() <= index) throw new RuntimeException("Can't edit properties. Index exceeds size.");
return setNewPropertyLike(index, buildProperty(index));
}
public PropertiesNested editFirstProperty() {
if (properties.size() == 0) throw new RuntimeException("Can't edit first properties. The list is empty.");
return setNewPropertyLike(0, buildProperty(0));
}
public PropertiesNested editLastProperty() {
int index = properties.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last properties. The list is empty.");
return setNewPropertyLike(index, buildProperty(index));
}
public PropertiesNested editMatchingProperty(Predicate predicate) {
int index = -1;
for (int i=0;i value) {
if (value == null || !value.isPresent()) { this.value = Optional.empty(); } else { this.value = value; } return (A) this;
}
public A withValue(Expression value) {
if (value == null) { this.value = Optional.empty(); } else { this.value = Optional.of(value); } return (A) this;
}
public Optional getValue() {
return this.value;
}
public boolean hasValue() {
return value != null && value.isPresent();
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
DeclareFluent that = (DeclareFluent) o;
if (!java.util.Objects.equals(properties, that.properties)) return false;
if (!java.util.Objects.equals(value, that.value)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(properties, value, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (properties != null && !properties.isEmpty()) { sb.append("properties:"); sb.append(properties + ","); }
if (value != null) { sb.append("value:"); sb.append(value); }
sb.append("}");
return sb.toString();
}
public class PropertiesNested extends PropertyFluent> implements Nested{
PropertiesNested(int index,Property item) {
this.index = index;
this.builder = new PropertyBuilder(this, item);
}
PropertyBuilder builder;
int index;
public N and() {
return (N) DeclareFluent.this.setToProperties(index,builder.build());
}
public N endProperty() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy