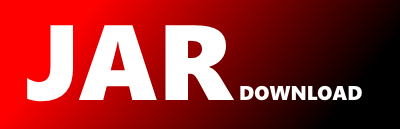
io.sundr.model.LambdaFluent Maven / Gradle / Ivy
package io.sundr.model;
import io.sundr.builder.VisitableBuilder;
import java.util.ArrayList;
import java.lang.String;
import java.util.function.Predicate;
import io.sundr.builder.BaseFluent;
import java.util.List;
import java.util.Collection;
import java.lang.Class;
import java.lang.Object;
import java.lang.SuppressWarnings;
import io.sundr.builder.Nested;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class LambdaFluent> extends BaseFluent{
public LambdaFluent() {
}
public LambdaFluent(Lambda instance) {
this.copyInstance(instance);
}
private List parameters = new ArrayList();
private VisitableBuilder extends Statement,?> statement;
protected void copyInstance(Lambda instance) {
if (instance != null) {
this.withParameters(instance.getParameters());
this.withStatement(instance.getStatement());
}
}
public A addToParameters(int index,String item) {
if (this.parameters == null) {this.parameters = new ArrayList();}
this.parameters.add(index, item);
return (A)this;
}
public A setToParameters(int index,String item) {
if (this.parameters == null) {this.parameters = new ArrayList();}
this.parameters.set(index, item); return (A)this;
}
public A addToParameters(java.lang.String... items) {
if (this.parameters == null) {this.parameters = new ArrayList();}
for (String item : items) {this.parameters.add(item);} return (A)this;
}
public A addAllToParameters(Collection items) {
if (this.parameters == null) {this.parameters = new ArrayList();}
for (String item : items) {this.parameters.add(item);} return (A)this;
}
public A removeFromParameters(java.lang.String... items) {
if (this.parameters == null) return (A)this;
for (String item : items) { this.parameters.remove(item);} return (A)this;
}
public A removeAllFromParameters(Collection items) {
if (this.parameters == null) return (A)this;
for (String item : items) { this.parameters.remove(item);} return (A)this;
}
public List getParameters() {
return this.parameters;
}
public String getParameter(int index) {
return this.parameters.get(index);
}
public String getFirstParameter() {
return this.parameters.get(0);
}
public String getLastParameter() {
return this.parameters.get(parameters.size() - 1);
}
public String getMatchingParameter(Predicate predicate) {
for (String item: parameters) { if(predicate.test(item)){ return item;} } return null;
}
public boolean hasMatchingParameter(Predicate predicate) {
for (String item: parameters) { if(predicate.test(item)){ return true;} } return false;
}
public A withParameters(List parameters) {
if (parameters != null) {this.parameters = new ArrayList(); for (String item : parameters){this.addToParameters(item);}} else { this.parameters = null;} return (A) this;
}
public A withParameters(java.lang.String... parameters) {
if (this.parameters != null) {this.parameters.clear(); _visitables.remove("parameters"); }
if (parameters != null) {for (String item :parameters){ this.addToParameters(item);}} return (A) this;
}
public boolean hasParameters() {
return parameters != null && !parameters.isEmpty();
}
public Statement buildStatement() {
return this.statement!=null?this.statement.build():null;
}
public A withStatement(Statement statement) {
if (statement==null){ this.statement = null; _visitables.remove("statement"); return (A) this;}
VisitableBuilder extends Statement,?> builder = builder(statement); _visitables.get("statement").clear();_visitables.get("statement").add(builder);this.statement = builder;
return (A) this;
}
public boolean hasStatement() {
return this.statement != null;
}
public MethodCallStatementNested withNewMethodCallStatement() {
return new MethodCallStatementNested(null);
}
public MethodCallStatementNested withNewMethodCallStatementLike(MethodCall item) {
return new MethodCallStatementNested(item);
}
public SwitchStatementNested withNewSwitchStatement() {
return new SwitchStatementNested(null);
}
public SwitchStatementNested withNewSwitchStatementLike(Switch item) {
return new SwitchStatementNested(item);
}
public BreakStatementNested withNewBreakStatement() {
return new BreakStatementNested(null);
}
public BreakStatementNested withNewBreakStatementLike(Break item) {
return new BreakStatementNested(item);
}
public DeclareStatementNested withNewDeclareStatement() {
return new DeclareStatementNested(null);
}
public DeclareStatementNested withNewDeclareStatementLike(Declare item) {
return new DeclareStatementNested(item);
}
public A withNewDeclareStatement(Class type,String name) {
return (A)withStatement(new Declare(type, name));
}
public A withNewDeclareStatement(Class type,String name,Object value) {
return (A)withStatement(new Declare(type, name, value));
}
public WhileStatementNested withNewWhileStatement() {
return new WhileStatementNested(null);
}
public WhileStatementNested withNewWhileStatementLike(While item) {
return new WhileStatementNested(item);
}
public ContinueStatementNested withNewContinueStatement() {
return new ContinueStatementNested(null);
}
public ContinueStatementNested withNewContinueStatementLike(Continue item) {
return new ContinueStatementNested(item);
}
public StringStatementNested withNewStringStatement() {
return new StringStatementNested(null);
}
public StringStatementNested withNewStringStatementLike(StringStatement item) {
return new StringStatementNested(item);
}
public A withNewStringStatement(String data) {
return (A)withStatement(new StringStatement(data));
}
public A withNewStringStatement(String data,Object[] parameters) {
return (A)withStatement(new StringStatement(data, parameters));
}
public DoStatementNested withNewDoStatement() {
return new DoStatementNested(null);
}
public DoStatementNested withNewDoStatementLike(Do item) {
return new DoStatementNested(item);
}
public ForeachStatementNested withNewForeachStatement() {
return new ForeachStatementNested(null);
}
public ForeachStatementNested withNewForeachStatementLike(Foreach item) {
return new ForeachStatementNested(item);
}
public BlockStatementNested withNewBlockStatement() {
return new BlockStatementNested(null);
}
public BlockStatementNested withNewBlockStatementLike(Block item) {
return new BlockStatementNested(item);
}
public IfStatementNested withNewIfStatement() {
return new IfStatementNested(null);
}
public IfStatementNested withNewIfStatementLike(If item) {
return new IfStatementNested(item);
}
public LambdaStatementNested withNewLambdaStatement() {
return new LambdaStatementNested(null);
}
public LambdaStatementNested withNewLambdaStatementLike(Lambda item) {
return new LambdaStatementNested(item);
}
public ReturnStatementNested withNewReturnStatement() {
return new ReturnStatementNested(null);
}
public ReturnStatementNested withNewReturnStatementLike(Return item) {
return new ReturnStatementNested(item);
}
public A withNewReturnStatement(Object object) {
return (A)withStatement(new Return(object));
}
public AssignStatementNested withNewAssignStatement() {
return new AssignStatementNested(null);
}
public AssignStatementNested withNewAssignStatementLike(Assign item) {
return new AssignStatementNested(item);
}
public ForStatementNested withNewForStatement() {
return new ForStatementNested(null);
}
public ForStatementNested withNewForStatementLike(For item) {
return new ForStatementNested(item);
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
LambdaFluent that = (LambdaFluent) o;
if (!java.util.Objects.equals(parameters, that.parameters)) return false;
if (!java.util.Objects.equals(statement, that.statement)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(parameters, statement, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (parameters != null && !parameters.isEmpty()) { sb.append("parameters:"); sb.append(parameters + ","); }
if (statement != null) { sb.append("statement:"); sb.append(statement); }
sb.append("}");
return sb.toString();
}
protected static VisitableBuilder builder(Object item) {
switch (item.getClass().getName()) {
case "io.sundr.model."+"MethodCall": return (VisitableBuilder)new MethodCallBuilder((MethodCall) item);
case "io.sundr.model."+"Switch": return (VisitableBuilder)new SwitchBuilder((Switch) item);
case "io.sundr.model."+"Break": return (VisitableBuilder)new BreakBuilder((Break) item);
case "io.sundr.model."+"Declare": return (VisitableBuilder)new DeclareBuilder((Declare) item);
case "io.sundr.model."+"While": return (VisitableBuilder)new WhileBuilder((While) item);
case "io.sundr.model."+"Continue": return (VisitableBuilder)new ContinueBuilder((Continue) item);
case "io.sundr.model."+"StringStatement": return (VisitableBuilder)new StringStatementBuilder((StringStatement) item);
case "io.sundr.model."+"Do": return (VisitableBuilder)new DoBuilder((Do) item);
case "io.sundr.model."+"Foreach": return (VisitableBuilder)new ForeachBuilder((Foreach) item);
case "io.sundr.model."+"Block": return (VisitableBuilder)new BlockBuilder((Block) item);
case "io.sundr.model."+"If": return (VisitableBuilder)new IfBuilder((If) item);
case "io.sundr.model."+"Lambda": return (VisitableBuilder)new LambdaBuilder((Lambda) item);
case "io.sundr.model."+"Return": return (VisitableBuilder)new ReturnBuilder((Return) item);
case "io.sundr.model."+"Assign": return (VisitableBuilder)new AssignBuilder((Assign) item);
case "io.sundr.model."+"For": return (VisitableBuilder)new ForBuilder((For) item);
}
return (VisitableBuilder)builderOf(item);
}
public class MethodCallStatementNested extends MethodCallFluent> implements Nested{
MethodCallStatementNested(MethodCall item) {
this.builder = new MethodCallBuilder(this, item);
}
MethodCallBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endMethodCallStatement() {
return and();
}
}
public class SwitchStatementNested extends SwitchFluent> implements Nested{
SwitchStatementNested(Switch item) {
this.builder = new SwitchBuilder(this, item);
}
SwitchBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endSwitchStatement() {
return and();
}
}
public class BreakStatementNested extends BreakFluent> implements Nested{
BreakStatementNested(Break item) {
this.builder = new BreakBuilder(this, item);
}
BreakBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endBreakStatement() {
return and();
}
}
public class DeclareStatementNested extends DeclareFluent> implements Nested{
DeclareStatementNested(Declare item) {
this.builder = new DeclareBuilder(this, item);
}
DeclareBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endDeclareStatement() {
return and();
}
}
public class WhileStatementNested extends WhileFluent> implements Nested{
WhileStatementNested(While item) {
this.builder = new WhileBuilder(this, item);
}
WhileBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endWhileStatement() {
return and();
}
}
public class ContinueStatementNested extends ContinueFluent> implements Nested{
ContinueStatementNested(Continue item) {
this.builder = new ContinueBuilder(this, item);
}
ContinueBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endContinueStatement() {
return and();
}
}
public class StringStatementNested extends StringStatementFluent> implements Nested{
StringStatementNested(StringStatement item) {
this.builder = new StringStatementBuilder(this, item);
}
StringStatementBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endStringStatement() {
return and();
}
}
public class DoStatementNested extends DoFluent> implements Nested{
DoStatementNested(Do item) {
this.builder = new DoBuilder(this, item);
}
DoBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endDoStatement() {
return and();
}
}
public class ForeachStatementNested extends ForeachFluent> implements Nested{
ForeachStatementNested(Foreach item) {
this.builder = new ForeachBuilder(this, item);
}
ForeachBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endForeachStatement() {
return and();
}
}
public class BlockStatementNested extends BlockFluent> implements Nested{
BlockStatementNested(Block item) {
this.builder = new BlockBuilder(this, item);
}
BlockBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endBlockStatement() {
return and();
}
}
public class IfStatementNested extends IfFluent> implements Nested{
IfStatementNested(If item) {
this.builder = new IfBuilder(this, item);
}
IfBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endIfStatement() {
return and();
}
}
public class LambdaStatementNested extends LambdaFluent> implements Nested{
LambdaStatementNested(Lambda item) {
this.builder = new LambdaBuilder(this, item);
}
LambdaBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endLambdaStatement() {
return and();
}
}
public class ReturnStatementNested extends ReturnFluent> implements Nested{
ReturnStatementNested(Return item) {
this.builder = new ReturnBuilder(this, item);
}
ReturnBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endReturnStatement() {
return and();
}
}
public class AssignStatementNested extends AssignFluent> implements Nested{
AssignStatementNested(Assign item) {
this.builder = new AssignBuilder(this, item);
}
AssignBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endAssignStatement() {
return and();
}
}
public class ForStatementNested extends ForFluent> implements Nested{
ForStatementNested(For item) {
this.builder = new ForBuilder(this, item);
}
ForBuilder builder;
public N and() {
return (N) LambdaFluent.this.withStatement(builder.build());
}
public N endForStatement() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy