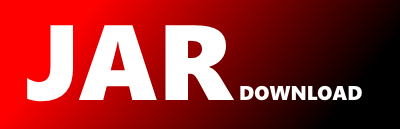
io.sundr.model.MethodCallFluent Maven / Gradle / Ivy
package io.sundr.model;
import java.util.ArrayList;
import java.lang.String;
import java.util.function.Predicate;
import java.util.List;
import java.lang.Class;
import java.lang.SuppressWarnings;
import java.util.Iterator;
import java.lang.Integer;
import io.sundr.builder.BaseFluent;
import java.lang.Object;
import io.sundr.builder.VisitableBuilder;
import java.util.Collection;
import io.sundr.builder.Nested;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class MethodCallFluent> extends BaseFluent{
public MethodCallFluent() {
}
public MethodCallFluent(MethodCall instance) {
this.copyInstance(instance);
}
private String name;
private VisitableBuilder extends Expression,?> scope;
private ArrayList> parameters = new ArrayList>();
private ArrayList> arguments = new ArrayList>();
protected void copyInstance(MethodCall instance) {
if (instance != null) {
this.withName(instance.getName());
this.withScope(instance.getScope());
this.withParameters(instance.getParameters());
this.withArguments(instance.getArguments());
}
}
public String getName() {
return this.name;
}
public A withName(String name) {
this.name=name; return (A) this;
}
public boolean hasName() {
return this.name != null;
}
public Expression buildScope() {
return this.scope!=null?this.scope.build():null;
}
public A withScope(Expression scope) {
if (scope==null){ this.scope = null; _visitables.remove("scope"); return (A) this;}
VisitableBuilder extends Expression,?> builder = builder(scope); _visitables.get("scope").clear();_visitables.get("scope").add(builder);this.scope = builder;
return (A) this;
}
public boolean hasScope() {
return this.scope != null;
}
public MultiplyScopeNested withNewMultiplyScope() {
return new MultiplyScopeNested(null);
}
public MultiplyScopeNested withNewMultiplyScopeLike(Multiply item) {
return new MultiplyScopeNested(item);
}
public A withNewMultiplyScope(Object left,Object right) {
return (A)withScope(new Multiply(left, right));
}
public NewArrayScopeNested withNewNewArrayScope() {
return new NewArrayScopeNested(null);
}
public NewArrayScopeNested withNewNewArrayScopeLike(NewArray item) {
return new NewArrayScopeNested(item);
}
public A withNewNewArrayScope(Class type,Integer[] sizes) {
return (A)withScope(new NewArray(type, sizes));
}
public InstanceOfScopeNested withNewInstanceOfScope() {
return new InstanceOfScopeNested(null);
}
public InstanceOfScopeNested withNewInstanceOfScopeLike(InstanceOf item) {
return new InstanceOfScopeNested(item);
}
public MethodCallScopeNested withNewMethodCallScope() {
return new MethodCallScopeNested(null);
}
public MethodCallScopeNested withNewMethodCallScopeLike(MethodCall item) {
return new MethodCallScopeNested(item);
}
public InverseScopeNested withNewInverseScope() {
return new InverseScopeNested(null);
}
public InverseScopeNested withNewInverseScopeLike(Inverse item) {
return new InverseScopeNested(item);
}
public IndexScopeNested withNewIndexScope() {
return new IndexScopeNested(null);
}
public IndexScopeNested withNewIndexScopeLike(Index item) {
return new IndexScopeNested(item);
}
public GreaterThanOrEqualScopeNested withNewGreaterThanOrEqualScope() {
return new GreaterThanOrEqualScopeNested(null);
}
public GreaterThanOrEqualScopeNested withNewGreaterThanOrEqualScopeLike(GreaterThanOrEqual item) {
return new GreaterThanOrEqualScopeNested(item);
}
public A withNewGreaterThanOrEqualScope(Object left,Object right) {
return (A)withScope(new GreaterThanOrEqual(left, right));
}
public BitwiseAndScopeNested withNewBitwiseAndScope() {
return new BitwiseAndScopeNested(null);
}
public BitwiseAndScopeNested withNewBitwiseAndScopeLike(BitwiseAnd item) {
return new BitwiseAndScopeNested(item);
}
public A withNewBitwiseAndScope(Object left,Object right) {
return (A)withScope(new BitwiseAnd(left, right));
}
public MinusScopeNested withNewMinusScope() {
return new MinusScopeNested(null);
}
public MinusScopeNested withNewMinusScopeLike(Minus item) {
return new MinusScopeNested(item);
}
public A withNewMinusScope(Object left,Object right) {
return (A)withScope(new Minus(left, right));
}
public LogicalOrScopeNested withNewLogicalOrScope() {
return new LogicalOrScopeNested(null);
}
public LogicalOrScopeNested withNewLogicalOrScopeLike(LogicalOr item) {
return new LogicalOrScopeNested(item);
}
public A withNewLogicalOrScope(Object left,Object right) {
return (A)withScope(new LogicalOr(left, right));
}
public NotEqualsScopeNested withNewNotEqualsScope() {
return new NotEqualsScopeNested(null);
}
public NotEqualsScopeNested withNewNotEqualsScopeLike(NotEquals item) {
return new NotEqualsScopeNested(item);
}
public A withNewNotEqualsScope(Object left,Object right) {
return (A)withScope(new NotEquals(left, right));
}
public DivideScopeNested withNewDivideScope() {
return new DivideScopeNested(null);
}
public DivideScopeNested withNewDivideScopeLike(Divide item) {
return new DivideScopeNested(item);
}
public A withNewDivideScope(Object left,Object right) {
return (A)withScope(new Divide(left, right));
}
public LessThanScopeNested withNewLessThanScope() {
return new LessThanScopeNested(null);
}
public LessThanScopeNested withNewLessThanScopeLike(LessThan item) {
return new LessThanScopeNested(item);
}
public A withNewLessThanScope(Object left,Object right) {
return (A)withScope(new LessThan(left, right));
}
public BitwiseOrScopeNested withNewBitwiseOrScope() {
return new BitwiseOrScopeNested(null);
}
public BitwiseOrScopeNested withNewBitwiseOrScopeLike(BitwiseOr item) {
return new BitwiseOrScopeNested(item);
}
public A withNewBitwiseOrScope(Object left,Object right) {
return (A)withScope(new BitwiseOr(left, right));
}
public PropertyRefScopeNested withNewPropertyRefScope() {
return new PropertyRefScopeNested(null);
}
public PropertyRefScopeNested withNewPropertyRefScopeLike(PropertyRef item) {
return new PropertyRefScopeNested(item);
}
public RightShiftScopeNested withNewRightShiftScope() {
return new RightShiftScopeNested(null);
}
public RightShiftScopeNested withNewRightShiftScopeLike(RightShift item) {
return new RightShiftScopeNested(item);
}
public A withNewRightShiftScope(Object left,Object right) {
return (A)withScope(new RightShift(left, right));
}
public GreaterThanScopeNested withNewGreaterThanScope() {
return new GreaterThanScopeNested(null);
}
public GreaterThanScopeNested withNewGreaterThanScopeLike(GreaterThan item) {
return new GreaterThanScopeNested(item);
}
public A withNewGreaterThanScope(Object left,Object right) {
return (A)withScope(new GreaterThan(left, right));
}
public DeclareScopeNested withNewDeclareScope() {
return new DeclareScopeNested(null);
}
public DeclareScopeNested withNewDeclareScopeLike(Declare item) {
return new DeclareScopeNested(item);
}
public A withNewDeclareScope(Class type,String name) {
return (A)withScope(new Declare(type, name));
}
public A withNewDeclareScope(Class type,String name,Object value) {
return (A)withScope(new Declare(type, name, value));
}
public CastScopeNested withNewCastScope() {
return new CastScopeNested(null);
}
public CastScopeNested withNewCastScopeLike(Cast item) {
return new CastScopeNested(item);
}
public ModuloScopeNested withNewModuloScope() {
return new ModuloScopeNested(null);
}
public ModuloScopeNested withNewModuloScopeLike(Modulo item) {
return new ModuloScopeNested(item);
}
public A withNewModuloScope(Object left,Object right) {
return (A)withScope(new Modulo(left, right));
}
public ValueRefScopeNested withNewValueRefScope() {
return new ValueRefScopeNested(null);
}
public ValueRefScopeNested withNewValueRefScopeLike(ValueRef item) {
return new ValueRefScopeNested(item);
}
public A withNewValueRefScope(Object value) {
return (A)withScope(new ValueRef(value));
}
public LeftShiftScopeNested withNewLeftShiftScope() {
return new LeftShiftScopeNested(null);
}
public LeftShiftScopeNested withNewLeftShiftScopeLike(LeftShift item) {
return new LeftShiftScopeNested(item);
}
public A withNewLeftShiftScope(Object left,Object right) {
return (A)withScope(new LeftShift(left, right));
}
public TernaryScopeNested withNewTernaryScope() {
return new TernaryScopeNested(null);
}
public TernaryScopeNested withNewTernaryScopeLike(Ternary item) {
return new TernaryScopeNested(item);
}
public BinaryExpressionScopeNested withNewBinaryExpressionScope() {
return new BinaryExpressionScopeNested(null);
}
public BinaryExpressionScopeNested withNewBinaryExpressionScopeLike(BinaryExpression item) {
return new BinaryExpressionScopeNested(item);
}
public EqualsScopeNested withNewEqualsScope() {
return new EqualsScopeNested(null);
}
public EqualsScopeNested withNewEqualsScopeLike(Equals item) {
return new EqualsScopeNested(item);
}
public A withNewEqualsScope(Object left,Object right) {
return (A)withScope(new Equals(left, right));
}
public EnclosedScopeNested withNewEnclosedScope() {
return new EnclosedScopeNested(null);
}
public EnclosedScopeNested withNewEnclosedScopeLike(Enclosed item) {
return new EnclosedScopeNested(item);
}
public PreDecrementScopeNested withNewPreDecrementScope() {
return new PreDecrementScopeNested(null);
}
public PreDecrementScopeNested withNewPreDecrementScopeLike(PreDecrement item) {
return new PreDecrementScopeNested(item);
}
public PostDecrementScopeNested withNewPostDecrementScope() {
return new PostDecrementScopeNested(null);
}
public PostDecrementScopeNested withNewPostDecrementScopeLike(PostDecrement item) {
return new PostDecrementScopeNested(item);
}
public LambdaScopeNested withNewLambdaScope() {
return new LambdaScopeNested(null);
}
public LambdaScopeNested withNewLambdaScopeLike(Lambda item) {
return new LambdaScopeNested(item);
}
public NotScopeNested withNewNotScope() {
return new NotScopeNested(null);
}
public NotScopeNested withNewNotScopeLike(Not item) {
return new NotScopeNested(item);
}
public ThisScopeNested withNewThisScope() {
return new ThisScopeNested(null);
}
public ThisScopeNested withNewThisScopeLike(This item) {
return new ThisScopeNested(item);
}
public NegativeScopeNested withNewNegativeScope() {
return new NegativeScopeNested(null);
}
public NegativeScopeNested withNewNegativeScopeLike(Negative item) {
return new NegativeScopeNested(item);
}
public AssignScopeNested withNewAssignScope() {
return new AssignScopeNested(null);
}
public AssignScopeNested withNewAssignScopeLike(Assign item) {
return new AssignScopeNested(item);
}
public LogicalAndScopeNested withNewLogicalAndScope() {
return new LogicalAndScopeNested(null);
}
public LogicalAndScopeNested withNewLogicalAndScopeLike(LogicalAnd item) {
return new LogicalAndScopeNested(item);
}
public A withNewLogicalAndScope(Object left,Object right) {
return (A)withScope(new LogicalAnd(left, right));
}
public PostIncrementScopeNested withNewPostIncrementScope() {
return new PostIncrementScopeNested(null);
}
public PostIncrementScopeNested withNewPostIncrementScopeLike(PostIncrement item) {
return new PostIncrementScopeNested(item);
}
public RightUnsignedShiftScopeNested withNewRightUnsignedShiftScope() {
return new RightUnsignedShiftScopeNested(null);
}
public RightUnsignedShiftScopeNested withNewRightUnsignedShiftScopeLike(RightUnsignedShift item) {
return new RightUnsignedShiftScopeNested(item);
}
public A withNewRightUnsignedShiftScope(Object left,Object right) {
return (A)withScope(new RightUnsignedShift(left, right));
}
public PlusScopeNested withNewPlusScope() {
return new PlusScopeNested(null);
}
public PlusScopeNested withNewPlusScopeLike(Plus item) {
return new PlusScopeNested(item);
}
public A withNewPlusScope(Object left,Object right) {
return (A)withScope(new Plus(left, right));
}
public ConstructScopeNested withNewConstructScope() {
return new ConstructScopeNested(null);
}
public ConstructScopeNested withNewConstructScopeLike(Construct item) {
return new ConstructScopeNested(item);
}
public XorScopeNested withNewXorScope() {
return new XorScopeNested(null);
}
public XorScopeNested withNewXorScopeLike(Xor item) {
return new XorScopeNested(item);
}
public A withNewXorScope(Object left,Object right) {
return (A)withScope(new Xor(left, right));
}
public PreIncrementScopeNested withNewPreIncrementScope() {
return new PreIncrementScopeNested(null);
}
public PreIncrementScopeNested withNewPreIncrementScopeLike(PreIncrement item) {
return new PreIncrementScopeNested(item);
}
public LessThanOrEqualScopeNested withNewLessThanOrEqualScope() {
return new LessThanOrEqualScopeNested(null);
}
public LessThanOrEqualScopeNested withNewLessThanOrEqualScopeLike(LessThanOrEqual item) {
return new LessThanOrEqualScopeNested(item);
}
public A withNewLessThanOrEqualScope(Object left,Object right) {
return (A)withScope(new LessThanOrEqual(left, right));
}
public PositiveScopeNested withNewPositiveScope() {
return new PositiveScopeNested(null);
}
public PositiveScopeNested withNewPositiveScopeLike(Positive item) {
return new PositiveScopeNested(item);
}
public A addToParameters(VisitableBuilder extends TypeRef,?> builder) {
if (this.parameters == null) {this.parameters = new ArrayList>();}
_visitables.get("parameters").add(builder);this.parameters.add(builder); return (A)this;
}
public A addToParameters(int index,VisitableBuilder extends TypeRef,?> builder) {
if (this.parameters == null) {this.parameters = new ArrayList>();}
if (index < 0 || index >= parameters.size()) { _visitables.get("parameters").add(builder); parameters.add(builder); } else { _visitables.get("parameters").add(index, builder); parameters.add(index, builder);}
return (A)this;
}
public A addToParameters(int index,TypeRef item) {
if (this.parameters == null) {this.parameters = new ArrayList>();}
VisitableBuilder extends TypeRef,?> builder = builder(item);
if (index < 0 || index >= parameters.size()) { _visitables.get("parameters").add(builder); parameters.add(builder); } else { _visitables.get("parameters").add(index, builder); parameters.add(index, builder);}
return (A)this;
}
public A setToParameters(int index,TypeRef item) {
if (this.parameters == null) {this.parameters = new ArrayList>();}
VisitableBuilder extends TypeRef,?> builder = builder(item);
if (index < 0 || index >= parameters.size()) { _visitables.get("parameters").add(builder); parameters.add(builder); } else { _visitables.get("parameters").set(index, builder); parameters.set(index, builder);}
return (A)this;
}
public A addToParameters(io.sundr.model.TypeRef... items) {
if (this.parameters == null) {this.parameters = new ArrayList>();}
for (TypeRef item : items) { VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("parameters").add(builder);this.parameters.add(builder); }
return (A)this;
}
public A addAllToParameters(Collection items) {
if (this.parameters == null) {this.parameters = new ArrayList>();}
for (TypeRef item : items) { VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("parameters").add(builder);this.parameters.add(builder); }
return (A)this;
}
public A removeFromParameters(VisitableBuilder extends TypeRef,?> builder) {
if (this.parameters == null) return (A)this;
_visitables.get("parameters").remove(builder);this.parameters.remove(builder); return (A)this;
}
public A removeFromParameters(io.sundr.model.TypeRef... items) {
if (this.parameters == null) return (A)this;
for (TypeRef item : items) {
VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("parameters").remove(builder);this.parameters.remove(builder);
} return (A)this;
}
public A removeAllFromParameters(Collection items) {
if (this.parameters == null) return (A)this;
for (TypeRef item : items) {
VisitableBuilder extends TypeRef,?> builder = builder(item); _visitables.get("parameters").remove(builder);this.parameters.remove(builder);
} return (A)this;
}
public A removeMatchingFromParameters(Predicate> predicate) {
if (parameters == null) return (A) this;
final Iterator> each = parameters.iterator();
final List visitables = _visitables.get("parameters");
while (each.hasNext()) {
VisitableBuilder extends TypeRef,?> builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildParameters() {
return build(parameters);
}
public TypeRef buildParameter(int index) {
return this.parameters.get(index).build();
}
public TypeRef buildFirstParameter() {
return this.parameters.get(0).build();
}
public TypeRef buildLastParameter() {
return this.parameters.get(parameters.size() - 1).build();
}
public TypeRef buildMatchingParameter(Predicate> predicate) {
for (VisitableBuilder extends TypeRef,?> item: parameters) { if(predicate.test(item)){ return item.build();} } return null;
}
public boolean hasMatchingParameter(Predicate> predicate) {
for (VisitableBuilder extends TypeRef,?> item: parameters) { if(predicate.test(item)){ return true;} } return false;
}
public A withParameters(List parameters) {
if (parameters != null) {this.parameters = new ArrayList(); for (TypeRef item : parameters){this.addToParameters(item);}} else { this.parameters = null;} return (A) this;
}
public A withParameters(io.sundr.model.TypeRef... parameters) {
if (this.parameters != null) {this.parameters.clear(); _visitables.remove("parameters"); }
if (parameters != null) {for (TypeRef item :parameters){ this.addToParameters(item);}} return (A) this;
}
public boolean hasParameters() {
return parameters != null && !parameters.isEmpty();
}
public ClassRefParametersNested addNewClassRefParameter() {
return new ClassRefParametersNested(-1, null);
}
public ClassRefParametersNested addNewClassRefParameterLike(ClassRef item) {
return new ClassRefParametersNested(-1, item);
}
public ClassRefParametersNested setNewClassRefParameterLike(int index,ClassRef item) {
return new ClassRefParametersNested(index, item);
}
public PrimitiveRefParametersNested addNewPrimitiveRefParameter() {
return new PrimitiveRefParametersNested(-1, null);
}
public PrimitiveRefParametersNested addNewPrimitiveRefParameterLike(PrimitiveRef item) {
return new PrimitiveRefParametersNested(-1, item);
}
public PrimitiveRefParametersNested setNewPrimitiveRefParameterLike(int index,PrimitiveRef item) {
return new PrimitiveRefParametersNested(index, item);
}
public VoidRefParametersNested addNewVoidRefParameter() {
return new VoidRefParametersNested(-1, null);
}
public VoidRefParametersNested addNewVoidRefParameterLike(VoidRef item) {
return new VoidRefParametersNested(-1, item);
}
public VoidRefParametersNested setNewVoidRefParameterLike(int index,VoidRef item) {
return new VoidRefParametersNested(index, item);
}
public TypeParamRefParametersNested addNewTypeParamRefParameter() {
return new TypeParamRefParametersNested(-1, null);
}
public TypeParamRefParametersNested addNewTypeParamRefParameterLike(TypeParamRef item) {
return new TypeParamRefParametersNested(-1, item);
}
public TypeParamRefParametersNested setNewTypeParamRefParameterLike(int index,TypeParamRef item) {
return new TypeParamRefParametersNested(index, item);
}
public WildcardRefParametersNested addNewWildcardRefParameter() {
return new WildcardRefParametersNested(-1, null);
}
public WildcardRefParametersNested addNewWildcardRefParameterLike(WildcardRef item) {
return new WildcardRefParametersNested(-1, item);
}
public WildcardRefParametersNested setNewWildcardRefParameterLike(int index,WildcardRef item) {
return new WildcardRefParametersNested(index, item);
}
public A addToArguments(VisitableBuilder extends Expression,?> builder) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
_visitables.get("arguments").add(builder);this.arguments.add(builder); return (A)this;
}
public A addToArguments(int index,VisitableBuilder extends Expression,?> builder) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
if (index < 0 || index >= arguments.size()) { _visitables.get("arguments").add(builder); arguments.add(builder); } else { _visitables.get("arguments").add(index, builder); arguments.add(index, builder);}
return (A)this;
}
public A addToArguments(int index,Expression item) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
VisitableBuilder extends Expression,?> builder = builder(item);
if (index < 0 || index >= arguments.size()) { _visitables.get("arguments").add(builder); arguments.add(builder); } else { _visitables.get("arguments").add(index, builder); arguments.add(index, builder);}
return (A)this;
}
public A setToArguments(int index,Expression item) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
VisitableBuilder extends Expression,?> builder = builder(item);
if (index < 0 || index >= arguments.size()) { _visitables.get("arguments").add(builder); arguments.add(builder); } else { _visitables.get("arguments").set(index, builder); arguments.set(index, builder);}
return (A)this;
}
public A addToArguments(io.sundr.model.Expression... items) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
for (Expression item : items) { VisitableBuilder extends Expression,?> builder = builder(item); _visitables.get("arguments").add(builder);this.arguments.add(builder); }
return (A)this;
}
public A addAllToArguments(Collection items) {
if (this.arguments == null) {this.arguments = new ArrayList>();}
for (Expression item : items) { VisitableBuilder extends Expression,?> builder = builder(item); _visitables.get("arguments").add(builder);this.arguments.add(builder); }
return (A)this;
}
public A removeFromArguments(VisitableBuilder extends Expression,?> builder) {
if (this.arguments == null) return (A)this;
_visitables.get("arguments").remove(builder);this.arguments.remove(builder); return (A)this;
}
public A removeFromArguments(io.sundr.model.Expression... items) {
if (this.arguments == null) return (A)this;
for (Expression item : items) {
VisitableBuilder extends Expression,?> builder = builder(item); _visitables.get("arguments").remove(builder);this.arguments.remove(builder);
} return (A)this;
}
public A removeAllFromArguments(Collection items) {
if (this.arguments == null) return (A)this;
for (Expression item : items) {
VisitableBuilder extends Expression,?> builder = builder(item); _visitables.get("arguments").remove(builder);this.arguments.remove(builder);
} return (A)this;
}
public A removeMatchingFromArguments(Predicate> predicate) {
if (arguments == null) return (A) this;
final Iterator> each = arguments.iterator();
final List visitables = _visitables.get("arguments");
while (each.hasNext()) {
VisitableBuilder extends Expression,?> builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildArguments() {
return build(arguments);
}
public Expression buildArgument(int index) {
return this.arguments.get(index).build();
}
public Expression buildFirstArgument() {
return this.arguments.get(0).build();
}
public Expression buildLastArgument() {
return this.arguments.get(arguments.size() - 1).build();
}
public Expression buildMatchingArgument(Predicate> predicate) {
for (VisitableBuilder extends Expression,?> item: arguments) { if(predicate.test(item)){ return item.build();} } return null;
}
public boolean hasMatchingArgument(Predicate> predicate) {
for (VisitableBuilder extends Expression,?> item: arguments) { if(predicate.test(item)){ return true;} } return false;
}
public A withArguments(List arguments) {
if (arguments != null) {this.arguments = new ArrayList(); for (Expression item : arguments){this.addToArguments(item);}} else { this.arguments = null;} return (A) this;
}
public A withArguments(io.sundr.model.Expression... arguments) {
if (this.arguments != null) {this.arguments.clear(); _visitables.remove("arguments"); }
if (arguments != null) {for (Expression item :arguments){ this.addToArguments(item);}} return (A) this;
}
public boolean hasArguments() {
return arguments != null && !arguments.isEmpty();
}
public MultiplyArgumentsNested addNewMultiplyArgument() {
return new MultiplyArgumentsNested(-1, null);
}
public MultiplyArgumentsNested addNewMultiplyArgumentLike(Multiply item) {
return new MultiplyArgumentsNested(-1, item);
}
public A addNewMultiplyArgument(Object left,Object right) {
return (A)addToArguments(new Multiply(left, right));
}
public MultiplyArgumentsNested setNewMultiplyArgumentLike(int index,Multiply item) {
return new MultiplyArgumentsNested(index, item);
}
public NewArrayArgumentsNested addNewNewArrayArgument() {
return new NewArrayArgumentsNested(-1, null);
}
public NewArrayArgumentsNested addNewNewArrayArgumentLike(NewArray item) {
return new NewArrayArgumentsNested(-1, item);
}
public A addNewNewArrayArgument(Class type,Integer[] sizes) {
return (A)addToArguments(new NewArray(type, sizes));
}
public NewArrayArgumentsNested setNewNewArrayArgumentLike(int index,NewArray item) {
return new NewArrayArgumentsNested(index, item);
}
public InstanceOfArgumentsNested addNewInstanceOfArgument() {
return new InstanceOfArgumentsNested(-1, null);
}
public InstanceOfArgumentsNested addNewInstanceOfArgumentLike(InstanceOf item) {
return new InstanceOfArgumentsNested(-1, item);
}
public InstanceOfArgumentsNested setNewInstanceOfArgumentLike(int index,InstanceOf item) {
return new InstanceOfArgumentsNested(index, item);
}
public MethodCallArgumentsNested addNewMethodCallArgument() {
return new MethodCallArgumentsNested(-1, null);
}
public MethodCallArgumentsNested addNewMethodCallArgumentLike(MethodCall item) {
return new MethodCallArgumentsNested(-1, item);
}
public MethodCallArgumentsNested setNewMethodCallArgumentLike(int index,MethodCall item) {
return new MethodCallArgumentsNested(index, item);
}
public InverseArgumentsNested addNewInverseArgument() {
return new InverseArgumentsNested(-1, null);
}
public InverseArgumentsNested addNewInverseArgumentLike(Inverse item) {
return new InverseArgumentsNested(-1, item);
}
public InverseArgumentsNested setNewInverseArgumentLike(int index,Inverse item) {
return new InverseArgumentsNested(index, item);
}
public IndexArgumentsNested addNewIndexArgument() {
return new IndexArgumentsNested(-1, null);
}
public IndexArgumentsNested addNewIndexArgumentLike(Index item) {
return new IndexArgumentsNested(-1, item);
}
public IndexArgumentsNested setNewIndexArgumentLike(int index,Index item) {
return new IndexArgumentsNested(index, item);
}
public GreaterThanOrEqualArgumentsNested addNewGreaterThanOrEqualArgument() {
return new GreaterThanOrEqualArgumentsNested(-1, null);
}
public GreaterThanOrEqualArgumentsNested addNewGreaterThanOrEqualArgumentLike(GreaterThanOrEqual item) {
return new GreaterThanOrEqualArgumentsNested(-1, item);
}
public A addNewGreaterThanOrEqualArgument(Object left,Object right) {
return (A)addToArguments(new GreaterThanOrEqual(left, right));
}
public GreaterThanOrEqualArgumentsNested setNewGreaterThanOrEqualArgumentLike(int index,GreaterThanOrEqual item) {
return new GreaterThanOrEqualArgumentsNested(index, item);
}
public BitwiseAndArgumentsNested addNewBitwiseAndArgument() {
return new BitwiseAndArgumentsNested(-1, null);
}
public BitwiseAndArgumentsNested addNewBitwiseAndArgumentLike(BitwiseAnd item) {
return new BitwiseAndArgumentsNested(-1, item);
}
public A addNewBitwiseAndArgument(Object left,Object right) {
return (A)addToArguments(new BitwiseAnd(left, right));
}
public BitwiseAndArgumentsNested setNewBitwiseAndArgumentLike(int index,BitwiseAnd item) {
return new BitwiseAndArgumentsNested(index, item);
}
public MinusArgumentsNested addNewMinusArgument() {
return new MinusArgumentsNested(-1, null);
}
public MinusArgumentsNested addNewMinusArgumentLike(Minus item) {
return new MinusArgumentsNested(-1, item);
}
public A addNewMinusArgument(Object left,Object right) {
return (A)addToArguments(new Minus(left, right));
}
public MinusArgumentsNested setNewMinusArgumentLike(int index,Minus item) {
return new MinusArgumentsNested(index, item);
}
public LogicalOrArgumentsNested addNewLogicalOrArgument() {
return new LogicalOrArgumentsNested(-1, null);
}
public LogicalOrArgumentsNested addNewLogicalOrArgumentLike(LogicalOr item) {
return new LogicalOrArgumentsNested(-1, item);
}
public A addNewLogicalOrArgument(Object left,Object right) {
return (A)addToArguments(new LogicalOr(left, right));
}
public LogicalOrArgumentsNested setNewLogicalOrArgumentLike(int index,LogicalOr item) {
return new LogicalOrArgumentsNested(index, item);
}
public NotEqualsArgumentsNested addNewNotEqualsArgument() {
return new NotEqualsArgumentsNested(-1, null);
}
public NotEqualsArgumentsNested addNewNotEqualsArgumentLike(NotEquals item) {
return new NotEqualsArgumentsNested(-1, item);
}
public A addNewNotEqualsArgument(Object left,Object right) {
return (A)addToArguments(new NotEquals(left, right));
}
public NotEqualsArgumentsNested setNewNotEqualsArgumentLike(int index,NotEquals item) {
return new NotEqualsArgumentsNested(index, item);
}
public DivideArgumentsNested addNewDivideArgument() {
return new DivideArgumentsNested(-1, null);
}
public DivideArgumentsNested addNewDivideArgumentLike(Divide item) {
return new DivideArgumentsNested(-1, item);
}
public A addNewDivideArgument(Object left,Object right) {
return (A)addToArguments(new Divide(left, right));
}
public DivideArgumentsNested setNewDivideArgumentLike(int index,Divide item) {
return new DivideArgumentsNested(index, item);
}
public LessThanArgumentsNested addNewLessThanArgument() {
return new LessThanArgumentsNested(-1, null);
}
public LessThanArgumentsNested addNewLessThanArgumentLike(LessThan item) {
return new LessThanArgumentsNested(-1, item);
}
public A addNewLessThanArgument(Object left,Object right) {
return (A)addToArguments(new LessThan(left, right));
}
public LessThanArgumentsNested setNewLessThanArgumentLike(int index,LessThan item) {
return new LessThanArgumentsNested(index, item);
}
public BitwiseOrArgumentsNested addNewBitwiseOrArgument() {
return new BitwiseOrArgumentsNested(-1, null);
}
public BitwiseOrArgumentsNested addNewBitwiseOrArgumentLike(BitwiseOr item) {
return new BitwiseOrArgumentsNested(-1, item);
}
public A addNewBitwiseOrArgument(Object left,Object right) {
return (A)addToArguments(new BitwiseOr(left, right));
}
public BitwiseOrArgumentsNested setNewBitwiseOrArgumentLike(int index,BitwiseOr item) {
return new BitwiseOrArgumentsNested(index, item);
}
public PropertyRefArgumentsNested addNewPropertyRefArgument() {
return new PropertyRefArgumentsNested(-1, null);
}
public PropertyRefArgumentsNested addNewPropertyRefArgumentLike(PropertyRef item) {
return new PropertyRefArgumentsNested(-1, item);
}
public PropertyRefArgumentsNested setNewPropertyRefArgumentLike(int index,PropertyRef item) {
return new PropertyRefArgumentsNested(index, item);
}
public RightShiftArgumentsNested addNewRightShiftArgument() {
return new RightShiftArgumentsNested(-1, null);
}
public RightShiftArgumentsNested addNewRightShiftArgumentLike(RightShift item) {
return new RightShiftArgumentsNested(-1, item);
}
public A addNewRightShiftArgument(Object left,Object right) {
return (A)addToArguments(new RightShift(left, right));
}
public RightShiftArgumentsNested setNewRightShiftArgumentLike(int index,RightShift item) {
return new RightShiftArgumentsNested(index, item);
}
public GreaterThanArgumentsNested addNewGreaterThanArgument() {
return new GreaterThanArgumentsNested(-1, null);
}
public GreaterThanArgumentsNested addNewGreaterThanArgumentLike(GreaterThan item) {
return new GreaterThanArgumentsNested(-1, item);
}
public A addNewGreaterThanArgument(Object left,Object right) {
return (A)addToArguments(new GreaterThan(left, right));
}
public GreaterThanArgumentsNested setNewGreaterThanArgumentLike(int index,GreaterThan item) {
return new GreaterThanArgumentsNested(index, item);
}
public DeclareArgumentsNested addNewDeclareArgument() {
return new DeclareArgumentsNested(-1, null);
}
public DeclareArgumentsNested addNewDeclareArgumentLike(Declare item) {
return new DeclareArgumentsNested(-1, item);
}
public A addNewDeclareArgument(Class type,String name) {
return (A)addToArguments(new Declare(type, name));
}
public A addNewDeclareArgument(Class type,String name,Object value) {
return (A)addToArguments(new Declare(type, name, value));
}
public DeclareArgumentsNested setNewDeclareArgumentLike(int index,Declare item) {
return new DeclareArgumentsNested(index, item);
}
public CastArgumentsNested addNewCastArgument() {
return new CastArgumentsNested(-1, null);
}
public CastArgumentsNested addNewCastArgumentLike(Cast item) {
return new CastArgumentsNested(-1, item);
}
public CastArgumentsNested setNewCastArgumentLike(int index,Cast item) {
return new CastArgumentsNested(index, item);
}
public ModuloArgumentsNested addNewModuloArgument() {
return new ModuloArgumentsNested(-1, null);
}
public ModuloArgumentsNested addNewModuloArgumentLike(Modulo item) {
return new ModuloArgumentsNested(-1, item);
}
public A addNewModuloArgument(Object left,Object right) {
return (A)addToArguments(new Modulo(left, right));
}
public ModuloArgumentsNested setNewModuloArgumentLike(int index,Modulo item) {
return new ModuloArgumentsNested(index, item);
}
public ValueRefArgumentsNested addNewValueRefArgument() {
return new ValueRefArgumentsNested(-1, null);
}
public ValueRefArgumentsNested addNewValueRefArgumentLike(ValueRef item) {
return new ValueRefArgumentsNested(-1, item);
}
public A addNewValueRefArgument(Object value) {
return (A)addToArguments(new ValueRef(value));
}
public ValueRefArgumentsNested setNewValueRefArgumentLike(int index,ValueRef item) {
return new ValueRefArgumentsNested(index, item);
}
public LeftShiftArgumentsNested addNewLeftShiftArgument() {
return new LeftShiftArgumentsNested(-1, null);
}
public LeftShiftArgumentsNested addNewLeftShiftArgumentLike(LeftShift item) {
return new LeftShiftArgumentsNested(-1, item);
}
public A addNewLeftShiftArgument(Object left,Object right) {
return (A)addToArguments(new LeftShift(left, right));
}
public LeftShiftArgumentsNested setNewLeftShiftArgumentLike(int index,LeftShift item) {
return new LeftShiftArgumentsNested(index, item);
}
public TernaryArgumentsNested addNewTernaryArgument() {
return new TernaryArgumentsNested(-1, null);
}
public TernaryArgumentsNested addNewTernaryArgumentLike(Ternary item) {
return new TernaryArgumentsNested(-1, item);
}
public TernaryArgumentsNested setNewTernaryArgumentLike(int index,Ternary item) {
return new TernaryArgumentsNested(index, item);
}
public BinaryExpressionArgumentsNested addNewBinaryExpressionArgument() {
return new BinaryExpressionArgumentsNested(-1, null);
}
public BinaryExpressionArgumentsNested addNewBinaryExpressionArgumentLike(BinaryExpression item) {
return new BinaryExpressionArgumentsNested(-1, item);
}
public BinaryExpressionArgumentsNested setNewBinaryExpressionArgumentLike(int index,BinaryExpression item) {
return new BinaryExpressionArgumentsNested(index, item);
}
public EqualsArgumentsNested addNewEqualsArgument() {
return new EqualsArgumentsNested(-1, null);
}
public EqualsArgumentsNested addNewEqualsArgumentLike(Equals item) {
return new EqualsArgumentsNested(-1, item);
}
public A addNewEqualsArgument(Object left,Object right) {
return (A)addToArguments(new Equals(left, right));
}
public EqualsArgumentsNested setNewEqualsArgumentLike(int index,Equals item) {
return new EqualsArgumentsNested(index, item);
}
public EnclosedArgumentsNested addNewEnclosedArgument() {
return new EnclosedArgumentsNested(-1, null);
}
public EnclosedArgumentsNested addNewEnclosedArgumentLike(Enclosed item) {
return new EnclosedArgumentsNested(-1, item);
}
public EnclosedArgumentsNested setNewEnclosedArgumentLike(int index,Enclosed item) {
return new EnclosedArgumentsNested(index, item);
}
public PreDecrementArgumentsNested addNewPreDecrementArgument() {
return new PreDecrementArgumentsNested(-1, null);
}
public PreDecrementArgumentsNested addNewPreDecrementArgumentLike(PreDecrement item) {
return new PreDecrementArgumentsNested(-1, item);
}
public PreDecrementArgumentsNested setNewPreDecrementArgumentLike(int index,PreDecrement item) {
return new PreDecrementArgumentsNested(index, item);
}
public PostDecrementArgumentsNested addNewPostDecrementArgument() {
return new PostDecrementArgumentsNested(-1, null);
}
public PostDecrementArgumentsNested addNewPostDecrementArgumentLike(PostDecrement item) {
return new PostDecrementArgumentsNested(-1, item);
}
public PostDecrementArgumentsNested setNewPostDecrementArgumentLike(int index,PostDecrement item) {
return new PostDecrementArgumentsNested(index, item);
}
public LambdaArgumentsNested addNewLambdaArgument() {
return new LambdaArgumentsNested(-1, null);
}
public LambdaArgumentsNested addNewLambdaArgumentLike(Lambda item) {
return new LambdaArgumentsNested(-1, item);
}
public LambdaArgumentsNested setNewLambdaArgumentLike(int index,Lambda item) {
return new LambdaArgumentsNested(index, item);
}
public NotArgumentsNested addNewNotArgument() {
return new NotArgumentsNested(-1, null);
}
public NotArgumentsNested addNewNotArgumentLike(Not item) {
return new NotArgumentsNested(-1, item);
}
public NotArgumentsNested setNewNotArgumentLike(int index,Not item) {
return new NotArgumentsNested(index, item);
}
public ThisArgumentsNested addNewThisArgument() {
return new ThisArgumentsNested(-1, null);
}
public ThisArgumentsNested addNewThisArgumentLike(This item) {
return new ThisArgumentsNested(-1, item);
}
public ThisArgumentsNested setNewThisArgumentLike(int index,This item) {
return new ThisArgumentsNested(index, item);
}
public NegativeArgumentsNested addNewNegativeArgument() {
return new NegativeArgumentsNested(-1, null);
}
public NegativeArgumentsNested addNewNegativeArgumentLike(Negative item) {
return new NegativeArgumentsNested(-1, item);
}
public NegativeArgumentsNested setNewNegativeArgumentLike(int index,Negative item) {
return new NegativeArgumentsNested(index, item);
}
public AssignArgumentsNested addNewAssignArgument() {
return new AssignArgumentsNested(-1, null);
}
public AssignArgumentsNested addNewAssignArgumentLike(Assign item) {
return new AssignArgumentsNested(-1, item);
}
public AssignArgumentsNested setNewAssignArgumentLike(int index,Assign item) {
return new AssignArgumentsNested(index, item);
}
public LogicalAndArgumentsNested addNewLogicalAndArgument() {
return new LogicalAndArgumentsNested(-1, null);
}
public LogicalAndArgumentsNested addNewLogicalAndArgumentLike(LogicalAnd item) {
return new LogicalAndArgumentsNested(-1, item);
}
public A addNewLogicalAndArgument(Object left,Object right) {
return (A)addToArguments(new LogicalAnd(left, right));
}
public LogicalAndArgumentsNested setNewLogicalAndArgumentLike(int index,LogicalAnd item) {
return new LogicalAndArgumentsNested(index, item);
}
public PostIncrementArgumentsNested addNewPostIncrementArgument() {
return new PostIncrementArgumentsNested(-1, null);
}
public PostIncrementArgumentsNested addNewPostIncrementArgumentLike(PostIncrement item) {
return new PostIncrementArgumentsNested(-1, item);
}
public PostIncrementArgumentsNested setNewPostIncrementArgumentLike(int index,PostIncrement item) {
return new PostIncrementArgumentsNested(index, item);
}
public RightUnsignedShiftArgumentsNested addNewRightUnsignedShiftArgument() {
return new RightUnsignedShiftArgumentsNested(-1, null);
}
public RightUnsignedShiftArgumentsNested addNewRightUnsignedShiftArgumentLike(RightUnsignedShift item) {
return new RightUnsignedShiftArgumentsNested(-1, item);
}
public A addNewRightUnsignedShiftArgument(Object left,Object right) {
return (A)addToArguments(new RightUnsignedShift(left, right));
}
public RightUnsignedShiftArgumentsNested setNewRightUnsignedShiftArgumentLike(int index,RightUnsignedShift item) {
return new RightUnsignedShiftArgumentsNested(index, item);
}
public PlusArgumentsNested addNewPlusArgument() {
return new PlusArgumentsNested(-1, null);
}
public PlusArgumentsNested addNewPlusArgumentLike(Plus item) {
return new PlusArgumentsNested(-1, item);
}
public A addNewPlusArgument(Object left,Object right) {
return (A)addToArguments(new Plus(left, right));
}
public PlusArgumentsNested setNewPlusArgumentLike(int index,Plus item) {
return new PlusArgumentsNested(index, item);
}
public ConstructArgumentsNested addNewConstructArgument() {
return new ConstructArgumentsNested(-1, null);
}
public ConstructArgumentsNested addNewConstructArgumentLike(Construct item) {
return new ConstructArgumentsNested(-1, item);
}
public ConstructArgumentsNested setNewConstructArgumentLike(int index,Construct item) {
return new ConstructArgumentsNested(index, item);
}
public XorArgumentsNested addNewXorArgument() {
return new XorArgumentsNested(-1, null);
}
public XorArgumentsNested addNewXorArgumentLike(Xor item) {
return new XorArgumentsNested(-1, item);
}
public A addNewXorArgument(Object left,Object right) {
return (A)addToArguments(new Xor(left, right));
}
public XorArgumentsNested setNewXorArgumentLike(int index,Xor item) {
return new XorArgumentsNested(index, item);
}
public PreIncrementArgumentsNested addNewPreIncrementArgument() {
return new PreIncrementArgumentsNested(-1, null);
}
public PreIncrementArgumentsNested addNewPreIncrementArgumentLike(PreIncrement item) {
return new PreIncrementArgumentsNested(-1, item);
}
public PreIncrementArgumentsNested setNewPreIncrementArgumentLike(int index,PreIncrement item) {
return new PreIncrementArgumentsNested(index, item);
}
public LessThanOrEqualArgumentsNested addNewLessThanOrEqualArgument() {
return new LessThanOrEqualArgumentsNested(-1, null);
}
public LessThanOrEqualArgumentsNested addNewLessThanOrEqualArgumentLike(LessThanOrEqual item) {
return new LessThanOrEqualArgumentsNested(-1, item);
}
public A addNewLessThanOrEqualArgument(Object left,Object right) {
return (A)addToArguments(new LessThanOrEqual(left, right));
}
public LessThanOrEqualArgumentsNested setNewLessThanOrEqualArgumentLike(int index,LessThanOrEqual item) {
return new LessThanOrEqualArgumentsNested(index, item);
}
public PositiveArgumentsNested addNewPositiveArgument() {
return new PositiveArgumentsNested(-1, null);
}
public PositiveArgumentsNested addNewPositiveArgumentLike(Positive item) {
return new PositiveArgumentsNested(-1, item);
}
public PositiveArgumentsNested setNewPositiveArgumentLike(int index,Positive item) {
return new PositiveArgumentsNested(index, item);
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
MethodCallFluent that = (MethodCallFluent) o;
if (!java.util.Objects.equals(name, that.name)) return false;
if (!java.util.Objects.equals(scope, that.scope)) return false;
if (!java.util.Objects.equals(parameters, that.parameters)) return false;
if (!java.util.Objects.equals(arguments, that.arguments)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(name, scope, parameters, arguments, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (name != null) { sb.append("name:"); sb.append(name + ","); }
if (scope != null) { sb.append("scope:"); sb.append(scope + ","); }
if (parameters != null && !parameters.isEmpty()) { sb.append("parameters:"); sb.append(parameters + ","); }
if (arguments != null && !arguments.isEmpty()) { sb.append("arguments:"); sb.append(arguments); }
sb.append("}");
return sb.toString();
}
protected static VisitableBuilder builder(Object item) {
switch (item.getClass().getName()) {
case "io.sundr.model."+"Multiply": return (VisitableBuilder)new MultiplyBuilder((Multiply) item);
case "io.sundr.model."+"NewArray": return (VisitableBuilder)new NewArrayBuilder((NewArray) item);
case "io.sundr.model."+"InstanceOf": return (VisitableBuilder)new InstanceOfBuilder((InstanceOf) item);
case "io.sundr.model."+"MethodCall": return (VisitableBuilder)new MethodCallBuilder((MethodCall) item);
case "io.sundr.model."+"Inverse": return (VisitableBuilder)new InverseBuilder((Inverse) item);
case "io.sundr.model."+"Index": return (VisitableBuilder)new IndexBuilder((Index) item);
case "io.sundr.model."+"GreaterThanOrEqual": return (VisitableBuilder)new GreaterThanOrEqualBuilder((GreaterThanOrEqual) item);
case "io.sundr.model."+"BitwiseAnd": return (VisitableBuilder)new BitwiseAndBuilder((BitwiseAnd) item);
case "io.sundr.model."+"Minus": return (VisitableBuilder)new MinusBuilder((Minus) item);
case "io.sundr.model."+"LogicalOr": return (VisitableBuilder)new LogicalOrBuilder((LogicalOr) item);
case "io.sundr.model."+"NotEquals": return (VisitableBuilder)new NotEqualsBuilder((NotEquals) item);
case "io.sundr.model."+"Divide": return (VisitableBuilder)new DivideBuilder((Divide) item);
case "io.sundr.model."+"LessThan": return (VisitableBuilder)new LessThanBuilder((LessThan) item);
case "io.sundr.model."+"BitwiseOr": return (VisitableBuilder)new BitwiseOrBuilder((BitwiseOr) item);
case "io.sundr.model."+"PropertyRef": return (VisitableBuilder)new PropertyRefBuilder((PropertyRef) item);
case "io.sundr.model."+"RightShift": return (VisitableBuilder)new RightShiftBuilder((RightShift) item);
case "io.sundr.model."+"GreaterThan": return (VisitableBuilder)new GreaterThanBuilder((GreaterThan) item);
case "io.sundr.model."+"Declare": return (VisitableBuilder)new DeclareBuilder((Declare) item);
case "io.sundr.model."+"Cast": return (VisitableBuilder)new CastBuilder((Cast) item);
case "io.sundr.model."+"Modulo": return (VisitableBuilder)new ModuloBuilder((Modulo) item);
case "io.sundr.model."+"ValueRef": return (VisitableBuilder)new ValueRefBuilder((ValueRef) item);
case "io.sundr.model."+"LeftShift": return (VisitableBuilder)new LeftShiftBuilder((LeftShift) item);
case "io.sundr.model."+"Ternary": return (VisitableBuilder)new TernaryBuilder((Ternary) item);
case "io.sundr.model."+"BinaryExpression": return (VisitableBuilder)new BinaryExpressionBuilder((BinaryExpression) item);
case "io.sundr.model."+"Equals": return (VisitableBuilder)new EqualsBuilder((Equals) item);
case "io.sundr.model."+"Enclosed": return (VisitableBuilder)new EnclosedBuilder((Enclosed) item);
case "io.sundr.model."+"PreDecrement": return (VisitableBuilder)new PreDecrementBuilder((PreDecrement) item);
case "io.sundr.model."+"PostDecrement": return (VisitableBuilder)new PostDecrementBuilder((PostDecrement) item);
case "io.sundr.model."+"Lambda": return (VisitableBuilder)new LambdaBuilder((Lambda) item);
case "io.sundr.model."+"Not": return (VisitableBuilder)new NotBuilder((Not) item);
case "io.sundr.model."+"This": return (VisitableBuilder)new ThisBuilder((This) item);
case "io.sundr.model."+"Negative": return (VisitableBuilder)new NegativeBuilder((Negative) item);
case "io.sundr.model."+"Assign": return (VisitableBuilder)new AssignBuilder((Assign) item);
case "io.sundr.model."+"LogicalAnd": return (VisitableBuilder)new LogicalAndBuilder((LogicalAnd) item);
case "io.sundr.model."+"PostIncrement": return (VisitableBuilder)new PostIncrementBuilder((PostIncrement) item);
case "io.sundr.model."+"RightUnsignedShift": return (VisitableBuilder)new RightUnsignedShiftBuilder((RightUnsignedShift) item);
case "io.sundr.model."+"Plus": return (VisitableBuilder)new PlusBuilder((Plus) item);
case "io.sundr.model."+"Construct": return (VisitableBuilder)new ConstructBuilder((Construct) item);
case "io.sundr.model."+"Xor": return (VisitableBuilder)new XorBuilder((Xor) item);
case "io.sundr.model."+"PreIncrement": return (VisitableBuilder)new PreIncrementBuilder((PreIncrement) item);
case "io.sundr.model."+"LessThanOrEqual": return (VisitableBuilder)new LessThanOrEqualBuilder((LessThanOrEqual) item);
case "io.sundr.model."+"Positive": return (VisitableBuilder)new PositiveBuilder((Positive) item);
case "io.sundr.model."+"ClassRef": return (VisitableBuilder)new ClassRefBuilder((ClassRef) item);
case "io.sundr.model."+"PrimitiveRef": return (VisitableBuilder)new PrimitiveRefBuilder((PrimitiveRef) item);
case "io.sundr.model."+"VoidRef": return (VisitableBuilder)new VoidRefBuilder((VoidRef) item);
case "io.sundr.model."+"TypeParamRef": return (VisitableBuilder)new TypeParamRefBuilder((TypeParamRef) item);
case "io.sundr.model."+"WildcardRef": return (VisitableBuilder)new WildcardRefBuilder((WildcardRef) item);
}
return (VisitableBuilder)builderOf(item);
}
public class MultiplyScopeNested extends MultiplyFluent> implements Nested{
MultiplyScopeNested(Multiply item) {
this.builder = new MultiplyBuilder(this, item);
}
MultiplyBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endMultiplyScope() {
return and();
}
}
public class NewArrayScopeNested extends NewArrayFluent> implements Nested{
NewArrayScopeNested(NewArray item) {
this.builder = new NewArrayBuilder(this, item);
}
NewArrayBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endNewArrayScope() {
return and();
}
}
public class InstanceOfScopeNested extends InstanceOfFluent> implements Nested{
InstanceOfScopeNested(InstanceOf item) {
this.builder = new InstanceOfBuilder(this, item);
}
InstanceOfBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endInstanceOfScope() {
return and();
}
}
public class MethodCallScopeNested extends MethodCallFluent> implements Nested{
MethodCallScopeNested(MethodCall item) {
this.builder = new MethodCallBuilder(this, item);
}
MethodCallBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endMethodCallScope() {
return and();
}
}
public class InverseScopeNested extends InverseFluent> implements Nested{
InverseScopeNested(Inverse item) {
this.builder = new InverseBuilder(this, item);
}
InverseBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endInverseScope() {
return and();
}
}
public class IndexScopeNested extends IndexFluent> implements Nested{
IndexScopeNested(Index item) {
this.builder = new IndexBuilder(this, item);
}
IndexBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endIndexScope() {
return and();
}
}
public class GreaterThanOrEqualScopeNested extends GreaterThanOrEqualFluent> implements Nested{
GreaterThanOrEqualScopeNested(GreaterThanOrEqual item) {
this.builder = new GreaterThanOrEqualBuilder(this, item);
}
GreaterThanOrEqualBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endGreaterThanOrEqualScope() {
return and();
}
}
public class BitwiseAndScopeNested extends BitwiseAndFluent> implements Nested{
BitwiseAndScopeNested(BitwiseAnd item) {
this.builder = new BitwiseAndBuilder(this, item);
}
BitwiseAndBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endBitwiseAndScope() {
return and();
}
}
public class MinusScopeNested extends MinusFluent> implements Nested{
MinusScopeNested(Minus item) {
this.builder = new MinusBuilder(this, item);
}
MinusBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endMinusScope() {
return and();
}
}
public class LogicalOrScopeNested extends LogicalOrFluent> implements Nested{
LogicalOrScopeNested(LogicalOr item) {
this.builder = new LogicalOrBuilder(this, item);
}
LogicalOrBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endLogicalOrScope() {
return and();
}
}
public class NotEqualsScopeNested extends NotEqualsFluent> implements Nested{
NotEqualsScopeNested(NotEquals item) {
this.builder = new NotEqualsBuilder(this, item);
}
NotEqualsBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endNotEqualsScope() {
return and();
}
}
public class DivideScopeNested extends DivideFluent> implements Nested{
DivideScopeNested(Divide item) {
this.builder = new DivideBuilder(this, item);
}
DivideBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endDivideScope() {
return and();
}
}
public class LessThanScopeNested extends LessThanFluent> implements Nested{
LessThanScopeNested(LessThan item) {
this.builder = new LessThanBuilder(this, item);
}
LessThanBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endLessThanScope() {
return and();
}
}
public class BitwiseOrScopeNested extends BitwiseOrFluent> implements Nested{
BitwiseOrScopeNested(BitwiseOr item) {
this.builder = new BitwiseOrBuilder(this, item);
}
BitwiseOrBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endBitwiseOrScope() {
return and();
}
}
public class PropertyRefScopeNested extends PropertyRefFluent> implements Nested{
PropertyRefScopeNested(PropertyRef item) {
this.builder = new PropertyRefBuilder(this, item);
}
PropertyRefBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endPropertyRefScope() {
return and();
}
}
public class RightShiftScopeNested extends RightShiftFluent> implements Nested{
RightShiftScopeNested(RightShift item) {
this.builder = new RightShiftBuilder(this, item);
}
RightShiftBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endRightShiftScope() {
return and();
}
}
public class GreaterThanScopeNested extends GreaterThanFluent> implements Nested{
GreaterThanScopeNested(GreaterThan item) {
this.builder = new GreaterThanBuilder(this, item);
}
GreaterThanBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endGreaterThanScope() {
return and();
}
}
public class DeclareScopeNested extends DeclareFluent> implements Nested{
DeclareScopeNested(Declare item) {
this.builder = new DeclareBuilder(this, item);
}
DeclareBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endDeclareScope() {
return and();
}
}
public class CastScopeNested extends CastFluent> implements Nested{
CastScopeNested(Cast item) {
this.builder = new CastBuilder(this, item);
}
CastBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endCastScope() {
return and();
}
}
public class ModuloScopeNested extends ModuloFluent> implements Nested{
ModuloScopeNested(Modulo item) {
this.builder = new ModuloBuilder(this, item);
}
ModuloBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endModuloScope() {
return and();
}
}
public class ValueRefScopeNested extends ValueRefFluent> implements Nested{
ValueRefScopeNested(ValueRef item) {
this.builder = new ValueRefBuilder(this, item);
}
ValueRefBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endValueRefScope() {
return and();
}
}
public class LeftShiftScopeNested extends LeftShiftFluent> implements Nested{
LeftShiftScopeNested(LeftShift item) {
this.builder = new LeftShiftBuilder(this, item);
}
LeftShiftBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endLeftShiftScope() {
return and();
}
}
public class TernaryScopeNested extends TernaryFluent> implements Nested{
TernaryScopeNested(Ternary item) {
this.builder = new TernaryBuilder(this, item);
}
TernaryBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endTernaryScope() {
return and();
}
}
public class BinaryExpressionScopeNested extends BinaryExpressionFluent> implements Nested{
BinaryExpressionScopeNested(BinaryExpression item) {
this.builder = new BinaryExpressionBuilder(this, item);
}
BinaryExpressionBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endBinaryExpressionScope() {
return and();
}
}
public class EqualsScopeNested extends EqualsFluent> implements Nested{
EqualsScopeNested(Equals item) {
this.builder = new EqualsBuilder(this, item);
}
EqualsBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endEqualsScope() {
return and();
}
}
public class EnclosedScopeNested extends EnclosedFluent> implements Nested{
EnclosedScopeNested(Enclosed item) {
this.builder = new EnclosedBuilder(this, item);
}
EnclosedBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endEnclosedScope() {
return and();
}
}
public class PreDecrementScopeNested extends PreDecrementFluent> implements Nested{
PreDecrementScopeNested(PreDecrement item) {
this.builder = new PreDecrementBuilder(this, item);
}
PreDecrementBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endPreDecrementScope() {
return and();
}
}
public class PostDecrementScopeNested extends PostDecrementFluent> implements Nested{
PostDecrementScopeNested(PostDecrement item) {
this.builder = new PostDecrementBuilder(this, item);
}
PostDecrementBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endPostDecrementScope() {
return and();
}
}
public class LambdaScopeNested extends LambdaFluent> implements Nested{
LambdaScopeNested(Lambda item) {
this.builder = new LambdaBuilder(this, item);
}
LambdaBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endLambdaScope() {
return and();
}
}
public class NotScopeNested extends NotFluent> implements Nested{
NotScopeNested(Not item) {
this.builder = new NotBuilder(this, item);
}
NotBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endNotScope() {
return and();
}
}
public class ThisScopeNested extends ThisFluent> implements Nested{
ThisScopeNested(This item) {
this.builder = new ThisBuilder(this, item);
}
ThisBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endThisScope() {
return and();
}
}
public class NegativeScopeNested extends NegativeFluent> implements Nested{
NegativeScopeNested(Negative item) {
this.builder = new NegativeBuilder(this, item);
}
NegativeBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endNegativeScope() {
return and();
}
}
public class AssignScopeNested extends AssignFluent> implements Nested{
AssignScopeNested(Assign item) {
this.builder = new AssignBuilder(this, item);
}
AssignBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endAssignScope() {
return and();
}
}
public class LogicalAndScopeNested extends LogicalAndFluent> implements Nested{
LogicalAndScopeNested(LogicalAnd item) {
this.builder = new LogicalAndBuilder(this, item);
}
LogicalAndBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endLogicalAndScope() {
return and();
}
}
public class PostIncrementScopeNested extends PostIncrementFluent> implements Nested{
PostIncrementScopeNested(PostIncrement item) {
this.builder = new PostIncrementBuilder(this, item);
}
PostIncrementBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endPostIncrementScope() {
return and();
}
}
public class RightUnsignedShiftScopeNested extends RightUnsignedShiftFluent> implements Nested{
RightUnsignedShiftScopeNested(RightUnsignedShift item) {
this.builder = new RightUnsignedShiftBuilder(this, item);
}
RightUnsignedShiftBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endRightUnsignedShiftScope() {
return and();
}
}
public class PlusScopeNested extends PlusFluent> implements Nested{
PlusScopeNested(Plus item) {
this.builder = new PlusBuilder(this, item);
}
PlusBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endPlusScope() {
return and();
}
}
public class ConstructScopeNested extends ConstructFluent> implements Nested{
ConstructScopeNested(Construct item) {
this.builder = new ConstructBuilder(this, item);
}
ConstructBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endConstructScope() {
return and();
}
}
public class XorScopeNested extends XorFluent> implements Nested{
XorScopeNested(Xor item) {
this.builder = new XorBuilder(this, item);
}
XorBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endXorScope() {
return and();
}
}
public class PreIncrementScopeNested extends PreIncrementFluent> implements Nested{
PreIncrementScopeNested(PreIncrement item) {
this.builder = new PreIncrementBuilder(this, item);
}
PreIncrementBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endPreIncrementScope() {
return and();
}
}
public class LessThanOrEqualScopeNested extends LessThanOrEqualFluent> implements Nested{
LessThanOrEqualScopeNested(LessThanOrEqual item) {
this.builder = new LessThanOrEqualBuilder(this, item);
}
LessThanOrEqualBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endLessThanOrEqualScope() {
return and();
}
}
public class PositiveScopeNested extends PositiveFluent> implements Nested{
PositiveScopeNested(Positive item) {
this.builder = new PositiveBuilder(this, item);
}
PositiveBuilder builder;
public N and() {
return (N) MethodCallFluent.this.withScope(builder.build());
}
public N endPositiveScope() {
return and();
}
}
public class ClassRefParametersNested extends ClassRefFluent> implements Nested{
ClassRefParametersNested(int index,ClassRef item) {
this.index = index;
this.builder = new ClassRefBuilder(this, item);
}
ClassRefBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToParameters(index,builder.build());
}
public N endClassRefParameter() {
return and();
}
}
public class PrimitiveRefParametersNested extends PrimitiveRefFluent> implements Nested{
PrimitiveRefParametersNested(int index,PrimitiveRef item) {
this.index = index;
this.builder = new PrimitiveRefBuilder(this, item);
}
PrimitiveRefBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToParameters(index,builder.build());
}
public N endPrimitiveRefParameter() {
return and();
}
}
public class VoidRefParametersNested extends VoidRefFluent> implements Nested{
VoidRefParametersNested(int index,VoidRef item) {
this.index = index;
this.builder = new VoidRefBuilder(this, item);
}
VoidRefBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToParameters(index,builder.build());
}
public N endVoidRefParameter() {
return and();
}
}
public class TypeParamRefParametersNested extends TypeParamRefFluent> implements Nested{
TypeParamRefParametersNested(int index,TypeParamRef item) {
this.index = index;
this.builder = new TypeParamRefBuilder(this, item);
}
TypeParamRefBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToParameters(index,builder.build());
}
public N endTypeParamRefParameter() {
return and();
}
}
public class WildcardRefParametersNested extends WildcardRefFluent> implements Nested{
WildcardRefParametersNested(int index,WildcardRef item) {
this.index = index;
this.builder = new WildcardRefBuilder(this, item);
}
WildcardRefBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToParameters(index,builder.build());
}
public N endWildcardRefParameter() {
return and();
}
}
public class MultiplyArgumentsNested extends MultiplyFluent> implements Nested{
MultiplyArgumentsNested(int index,Multiply item) {
this.index = index;
this.builder = new MultiplyBuilder(this, item);
}
MultiplyBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToArguments(index,builder.build());
}
public N endMultiplyArgument() {
return and();
}
}
public class NewArrayArgumentsNested extends NewArrayFluent> implements Nested{
NewArrayArgumentsNested(int index,NewArray item) {
this.index = index;
this.builder = new NewArrayBuilder(this, item);
}
NewArrayBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToArguments(index,builder.build());
}
public N endNewArrayArgument() {
return and();
}
}
public class InstanceOfArgumentsNested extends InstanceOfFluent> implements Nested{
InstanceOfArgumentsNested(int index,InstanceOf item) {
this.index = index;
this.builder = new InstanceOfBuilder(this, item);
}
InstanceOfBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToArguments(index,builder.build());
}
public N endInstanceOfArgument() {
return and();
}
}
public class MethodCallArgumentsNested extends MethodCallFluent> implements Nested{
MethodCallArgumentsNested(int index,MethodCall item) {
this.index = index;
this.builder = new MethodCallBuilder(this, item);
}
MethodCallBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToArguments(index,builder.build());
}
public N endMethodCallArgument() {
return and();
}
}
public class InverseArgumentsNested extends InverseFluent> implements Nested{
InverseArgumentsNested(int index,Inverse item) {
this.index = index;
this.builder = new InverseBuilder(this, item);
}
InverseBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToArguments(index,builder.build());
}
public N endInverseArgument() {
return and();
}
}
public class IndexArgumentsNested extends IndexFluent> implements Nested{
IndexArgumentsNested(int index,Index item) {
this.index = index;
this.builder = new IndexBuilder(this, item);
}
IndexBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToArguments(index,builder.build());
}
public N endIndexArgument() {
return and();
}
}
public class GreaterThanOrEqualArgumentsNested extends GreaterThanOrEqualFluent> implements Nested{
GreaterThanOrEqualArgumentsNested(int index,GreaterThanOrEqual item) {
this.index = index;
this.builder = new GreaterThanOrEqualBuilder(this, item);
}
GreaterThanOrEqualBuilder builder;
int index;
public N and() {
return (N) MethodCallFluent.this.setToArguments(index,builder.build());
}
public N endGreaterThanOrEqualArgument() {
return and();
}
}
public class BitwiseAndArgumentsNested extends BitwiseAndFluent> implements Nested