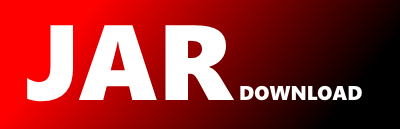
io.sundr.model.NotFluent Maven / Gradle / Ivy
package io.sundr.model;
import java.lang.String;
import java.lang.Class;
import java.lang.SuppressWarnings;
import java.lang.Integer;
import io.sundr.builder.BaseFluent;
import java.lang.Object;
import io.sundr.builder.VisitableBuilder;
import io.sundr.builder.Nested;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class NotFluent> extends BaseFluent{
public NotFluent() {
}
public NotFluent(Not instance) {
this.copyInstance(instance);
}
private VisitableBuilder extends Expression,?> expresion;
protected void copyInstance(Not instance) {
if (instance != null) {
this.withExpresion(instance.getExpresion());
}
}
public Expression buildExpresion() {
return this.expresion!=null?this.expresion.build():null;
}
public A withExpresion(Expression expresion) {
if (expresion==null){ this.expresion = null; _visitables.remove("expresion"); return (A) this;}
VisitableBuilder extends Expression,?> builder = builder(expresion); _visitables.get("expresion").clear();_visitables.get("expresion").add(builder);this.expresion = builder;
return (A) this;
}
public boolean hasExpresion() {
return this.expresion != null;
}
public MultiplyExpresionNested withNewMultiplyExpresion() {
return new MultiplyExpresionNested(null);
}
public MultiplyExpresionNested withNewMultiplyExpresionLike(Multiply item) {
return new MultiplyExpresionNested(item);
}
public A withNewMultiplyExpresion(Object left,Object right) {
return (A)withExpresion(new Multiply(left, right));
}
public NewArrayExpresionNested withNewNewArrayExpresion() {
return new NewArrayExpresionNested(null);
}
public NewArrayExpresionNested withNewNewArrayExpresionLike(NewArray item) {
return new NewArrayExpresionNested(item);
}
public A withNewNewArrayExpresion(Class type,Integer[] sizes) {
return (A)withExpresion(new NewArray(type, sizes));
}
public InstanceOfExpresionNested withNewInstanceOfExpresion() {
return new InstanceOfExpresionNested(null);
}
public InstanceOfExpresionNested withNewInstanceOfExpresionLike(InstanceOf item) {
return new InstanceOfExpresionNested(item);
}
public MethodCallExpresionNested withNewMethodCallExpresion() {
return new MethodCallExpresionNested(null);
}
public MethodCallExpresionNested withNewMethodCallExpresionLike(MethodCall item) {
return new MethodCallExpresionNested(item);
}
public InverseExpresionNested withNewInverseExpresion() {
return new InverseExpresionNested(null);
}
public InverseExpresionNested withNewInverseExpresionLike(Inverse item) {
return new InverseExpresionNested(item);
}
public IndexExpresionNested withNewIndexExpresion() {
return new IndexExpresionNested(null);
}
public IndexExpresionNested withNewIndexExpresionLike(Index item) {
return new IndexExpresionNested(item);
}
public GreaterThanOrEqualExpresionNested withNewGreaterThanOrEqualExpresion() {
return new GreaterThanOrEqualExpresionNested(null);
}
public GreaterThanOrEqualExpresionNested withNewGreaterThanOrEqualExpresionLike(GreaterThanOrEqual item) {
return new GreaterThanOrEqualExpresionNested(item);
}
public A withNewGreaterThanOrEqualExpresion(Object left,Object right) {
return (A)withExpresion(new GreaterThanOrEqual(left, right));
}
public BitwiseAndExpresionNested withNewBitwiseAndExpresion() {
return new BitwiseAndExpresionNested(null);
}
public BitwiseAndExpresionNested withNewBitwiseAndExpresionLike(BitwiseAnd item) {
return new BitwiseAndExpresionNested(item);
}
public A withNewBitwiseAndExpresion(Object left,Object right) {
return (A)withExpresion(new BitwiseAnd(left, right));
}
public MinusExpresionNested withNewMinusExpresion() {
return new MinusExpresionNested(null);
}
public MinusExpresionNested withNewMinusExpresionLike(Minus item) {
return new MinusExpresionNested(item);
}
public A withNewMinusExpresion(Object left,Object right) {
return (A)withExpresion(new Minus(left, right));
}
public LogicalOrExpresionNested withNewLogicalOrExpresion() {
return new LogicalOrExpresionNested(null);
}
public LogicalOrExpresionNested withNewLogicalOrExpresionLike(LogicalOr item) {
return new LogicalOrExpresionNested(item);
}
public A withNewLogicalOrExpresion(Object left,Object right) {
return (A)withExpresion(new LogicalOr(left, right));
}
public NotEqualsExpresionNested withNewNotEqualsExpresion() {
return new NotEqualsExpresionNested(null);
}
public NotEqualsExpresionNested withNewNotEqualsExpresionLike(NotEquals item) {
return new NotEqualsExpresionNested(item);
}
public A withNewNotEqualsExpresion(Object left,Object right) {
return (A)withExpresion(new NotEquals(left, right));
}
public DivideExpresionNested withNewDivideExpresion() {
return new DivideExpresionNested(null);
}
public DivideExpresionNested withNewDivideExpresionLike(Divide item) {
return new DivideExpresionNested(item);
}
public A withNewDivideExpresion(Object left,Object right) {
return (A)withExpresion(new Divide(left, right));
}
public LessThanExpresionNested withNewLessThanExpresion() {
return new LessThanExpresionNested(null);
}
public LessThanExpresionNested withNewLessThanExpresionLike(LessThan item) {
return new LessThanExpresionNested(item);
}
public A withNewLessThanExpresion(Object left,Object right) {
return (A)withExpresion(new LessThan(left, right));
}
public BitwiseOrExpresionNested withNewBitwiseOrExpresion() {
return new BitwiseOrExpresionNested(null);
}
public BitwiseOrExpresionNested withNewBitwiseOrExpresionLike(BitwiseOr item) {
return new BitwiseOrExpresionNested(item);
}
public A withNewBitwiseOrExpresion(Object left,Object right) {
return (A)withExpresion(new BitwiseOr(left, right));
}
public PropertyRefExpresionNested withNewPropertyRefExpresion() {
return new PropertyRefExpresionNested(null);
}
public PropertyRefExpresionNested withNewPropertyRefExpresionLike(PropertyRef item) {
return new PropertyRefExpresionNested(item);
}
public RightShiftExpresionNested withNewRightShiftExpresion() {
return new RightShiftExpresionNested(null);
}
public RightShiftExpresionNested withNewRightShiftExpresionLike(RightShift item) {
return new RightShiftExpresionNested(item);
}
public A withNewRightShiftExpresion(Object left,Object right) {
return (A)withExpresion(new RightShift(left, right));
}
public GreaterThanExpresionNested withNewGreaterThanExpresion() {
return new GreaterThanExpresionNested(null);
}
public GreaterThanExpresionNested withNewGreaterThanExpresionLike(GreaterThan item) {
return new GreaterThanExpresionNested(item);
}
public A withNewGreaterThanExpresion(Object left,Object right) {
return (A)withExpresion(new GreaterThan(left, right));
}
public DeclareExpresionNested withNewDeclareExpresion() {
return new DeclareExpresionNested(null);
}
public DeclareExpresionNested withNewDeclareExpresionLike(Declare item) {
return new DeclareExpresionNested(item);
}
public A withNewDeclareExpresion(Class type,String name) {
return (A)withExpresion(new Declare(type, name));
}
public A withNewDeclareExpresion(Class type,String name,Object value) {
return (A)withExpresion(new Declare(type, name, value));
}
public CastExpresionNested withNewCastExpresion() {
return new CastExpresionNested(null);
}
public CastExpresionNested withNewCastExpresionLike(Cast item) {
return new CastExpresionNested(item);
}
public ModuloExpresionNested withNewModuloExpresion() {
return new ModuloExpresionNested(null);
}
public ModuloExpresionNested withNewModuloExpresionLike(Modulo item) {
return new ModuloExpresionNested(item);
}
public A withNewModuloExpresion(Object left,Object right) {
return (A)withExpresion(new Modulo(left, right));
}
public ValueRefExpresionNested withNewValueRefExpresion() {
return new ValueRefExpresionNested(null);
}
public ValueRefExpresionNested withNewValueRefExpresionLike(ValueRef item) {
return new ValueRefExpresionNested(item);
}
public A withNewValueRefExpresion(Object value) {
return (A)withExpresion(new ValueRef(value));
}
public LeftShiftExpresionNested withNewLeftShiftExpresion() {
return new LeftShiftExpresionNested(null);
}
public LeftShiftExpresionNested withNewLeftShiftExpresionLike(LeftShift item) {
return new LeftShiftExpresionNested(item);
}
public A withNewLeftShiftExpresion(Object left,Object right) {
return (A)withExpresion(new LeftShift(left, right));
}
public TernaryExpresionNested withNewTernaryExpresion() {
return new TernaryExpresionNested(null);
}
public TernaryExpresionNested withNewTernaryExpresionLike(Ternary item) {
return new TernaryExpresionNested(item);
}
public BinaryExpressionExpresionNested withNewBinaryExpressionExpresion() {
return new BinaryExpressionExpresionNested(null);
}
public BinaryExpressionExpresionNested withNewBinaryExpressionExpresionLike(BinaryExpression item) {
return new BinaryExpressionExpresionNested(item);
}
public EqualsExpresionNested withNewEqualsExpresion() {
return new EqualsExpresionNested(null);
}
public EqualsExpresionNested withNewEqualsExpresionLike(Equals item) {
return new EqualsExpresionNested(item);
}
public A withNewEqualsExpresion(Object left,Object right) {
return (A)withExpresion(new Equals(left, right));
}
public EnclosedExpresionNested withNewEnclosedExpresion() {
return new EnclosedExpresionNested(null);
}
public EnclosedExpresionNested withNewEnclosedExpresionLike(Enclosed item) {
return new EnclosedExpresionNested(item);
}
public PreDecrementExpresionNested withNewPreDecrementExpresion() {
return new PreDecrementExpresionNested(null);
}
public PreDecrementExpresionNested withNewPreDecrementExpresionLike(PreDecrement item) {
return new PreDecrementExpresionNested(item);
}
public PostDecrementExpresionNested withNewPostDecrementExpresion() {
return new PostDecrementExpresionNested(null);
}
public PostDecrementExpresionNested withNewPostDecrementExpresionLike(PostDecrement item) {
return new PostDecrementExpresionNested(item);
}
public LambdaExpresionNested withNewLambdaExpresion() {
return new LambdaExpresionNested(null);
}
public LambdaExpresionNested withNewLambdaExpresionLike(Lambda item) {
return new LambdaExpresionNested(item);
}
public NotExpresionNested withNewNotExpresion() {
return new NotExpresionNested(null);
}
public NotExpresionNested withNewNotExpresionLike(Not item) {
return new NotExpresionNested(item);
}
public ThisExpresionNested withNewThisExpresion() {
return new ThisExpresionNested(null);
}
public ThisExpresionNested withNewThisExpresionLike(This item) {
return new ThisExpresionNested(item);
}
public NegativeExpresionNested withNewNegativeExpresion() {
return new NegativeExpresionNested(null);
}
public NegativeExpresionNested withNewNegativeExpresionLike(Negative item) {
return new NegativeExpresionNested(item);
}
public AssignExpresionNested withNewAssignExpresion() {
return new AssignExpresionNested(null);
}
public AssignExpresionNested withNewAssignExpresionLike(Assign item) {
return new AssignExpresionNested(item);
}
public LogicalAndExpresionNested withNewLogicalAndExpresion() {
return new LogicalAndExpresionNested(null);
}
public LogicalAndExpresionNested withNewLogicalAndExpresionLike(LogicalAnd item) {
return new LogicalAndExpresionNested(item);
}
public A withNewLogicalAndExpresion(Object left,Object right) {
return (A)withExpresion(new LogicalAnd(left, right));
}
public PostIncrementExpresionNested withNewPostIncrementExpresion() {
return new PostIncrementExpresionNested(null);
}
public PostIncrementExpresionNested withNewPostIncrementExpresionLike(PostIncrement item) {
return new PostIncrementExpresionNested(item);
}
public RightUnsignedShiftExpresionNested withNewRightUnsignedShiftExpresion() {
return new RightUnsignedShiftExpresionNested(null);
}
public RightUnsignedShiftExpresionNested withNewRightUnsignedShiftExpresionLike(RightUnsignedShift item) {
return new RightUnsignedShiftExpresionNested(item);
}
public A withNewRightUnsignedShiftExpresion(Object left,Object right) {
return (A)withExpresion(new RightUnsignedShift(left, right));
}
public PlusExpresionNested withNewPlusExpresion() {
return new PlusExpresionNested(null);
}
public PlusExpresionNested withNewPlusExpresionLike(Plus item) {
return new PlusExpresionNested(item);
}
public A withNewPlusExpresion(Object left,Object right) {
return (A)withExpresion(new Plus(left, right));
}
public ConstructExpresionNested withNewConstructExpresion() {
return new ConstructExpresionNested(null);
}
public ConstructExpresionNested withNewConstructExpresionLike(Construct item) {
return new ConstructExpresionNested(item);
}
public XorExpresionNested withNewXorExpresion() {
return new XorExpresionNested(null);
}
public XorExpresionNested withNewXorExpresionLike(Xor item) {
return new XorExpresionNested(item);
}
public A withNewXorExpresion(Object left,Object right) {
return (A)withExpresion(new Xor(left, right));
}
public PreIncrementExpresionNested withNewPreIncrementExpresion() {
return new PreIncrementExpresionNested(null);
}
public PreIncrementExpresionNested withNewPreIncrementExpresionLike(PreIncrement item) {
return new PreIncrementExpresionNested(item);
}
public LessThanOrEqualExpresionNested withNewLessThanOrEqualExpresion() {
return new LessThanOrEqualExpresionNested(null);
}
public LessThanOrEqualExpresionNested withNewLessThanOrEqualExpresionLike(LessThanOrEqual item) {
return new LessThanOrEqualExpresionNested(item);
}
public A withNewLessThanOrEqualExpresion(Object left,Object right) {
return (A)withExpresion(new LessThanOrEqual(left, right));
}
public PositiveExpresionNested withNewPositiveExpresion() {
return new PositiveExpresionNested(null);
}
public PositiveExpresionNested withNewPositiveExpresionLike(Positive item) {
return new PositiveExpresionNested(item);
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
NotFluent that = (NotFluent) o;
if (!java.util.Objects.equals(expresion, that.expresion)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(expresion, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (expresion != null) { sb.append("expresion:"); sb.append(expresion); }
sb.append("}");
return sb.toString();
}
protected static VisitableBuilder builder(Object item) {
switch (item.getClass().getName()) {
case "io.sundr.model."+"Multiply": return (VisitableBuilder)new MultiplyBuilder((Multiply) item);
case "io.sundr.model."+"NewArray": return (VisitableBuilder)new NewArrayBuilder((NewArray) item);
case "io.sundr.model."+"InstanceOf": return (VisitableBuilder)new InstanceOfBuilder((InstanceOf) item);
case "io.sundr.model."+"MethodCall": return (VisitableBuilder)new MethodCallBuilder((MethodCall) item);
case "io.sundr.model."+"Inverse": return (VisitableBuilder)new InverseBuilder((Inverse) item);
case "io.sundr.model."+"Index": return (VisitableBuilder)new IndexBuilder((Index) item);
case "io.sundr.model."+"GreaterThanOrEqual": return (VisitableBuilder)new GreaterThanOrEqualBuilder((GreaterThanOrEqual) item);
case "io.sundr.model."+"BitwiseAnd": return (VisitableBuilder)new BitwiseAndBuilder((BitwiseAnd) item);
case "io.sundr.model."+"Minus": return (VisitableBuilder)new MinusBuilder((Minus) item);
case "io.sundr.model."+"LogicalOr": return (VisitableBuilder)new LogicalOrBuilder((LogicalOr) item);
case "io.sundr.model."+"NotEquals": return (VisitableBuilder)new NotEqualsBuilder((NotEquals) item);
case "io.sundr.model."+"Divide": return (VisitableBuilder)new DivideBuilder((Divide) item);
case "io.sundr.model."+"LessThan": return (VisitableBuilder)new LessThanBuilder((LessThan) item);
case "io.sundr.model."+"BitwiseOr": return (VisitableBuilder)new BitwiseOrBuilder((BitwiseOr) item);
case "io.sundr.model."+"PropertyRef": return (VisitableBuilder)new PropertyRefBuilder((PropertyRef) item);
case "io.sundr.model."+"RightShift": return (VisitableBuilder)new RightShiftBuilder((RightShift) item);
case "io.sundr.model."+"GreaterThan": return (VisitableBuilder)new GreaterThanBuilder((GreaterThan) item);
case "io.sundr.model."+"Declare": return (VisitableBuilder)new DeclareBuilder((Declare) item);
case "io.sundr.model."+"Cast": return (VisitableBuilder)new CastBuilder((Cast) item);
case "io.sundr.model."+"Modulo": return (VisitableBuilder)new ModuloBuilder((Modulo) item);
case "io.sundr.model."+"ValueRef": return (VisitableBuilder)new ValueRefBuilder((ValueRef) item);
case "io.sundr.model."+"LeftShift": return (VisitableBuilder)new LeftShiftBuilder((LeftShift) item);
case "io.sundr.model."+"Ternary": return (VisitableBuilder)new TernaryBuilder((Ternary) item);
case "io.sundr.model."+"BinaryExpression": return (VisitableBuilder)new BinaryExpressionBuilder((BinaryExpression) item);
case "io.sundr.model."+"Equals": return (VisitableBuilder)new EqualsBuilder((Equals) item);
case "io.sundr.model."+"Enclosed": return (VisitableBuilder)new EnclosedBuilder((Enclosed) item);
case "io.sundr.model."+"PreDecrement": return (VisitableBuilder)new PreDecrementBuilder((PreDecrement) item);
case "io.sundr.model."+"PostDecrement": return (VisitableBuilder)new PostDecrementBuilder((PostDecrement) item);
case "io.sundr.model."+"Lambda": return (VisitableBuilder)new LambdaBuilder((Lambda) item);
case "io.sundr.model."+"Not": return (VisitableBuilder)new NotBuilder((Not) item);
case "io.sundr.model."+"This": return (VisitableBuilder)new ThisBuilder((This) item);
case "io.sundr.model."+"Negative": return (VisitableBuilder)new NegativeBuilder((Negative) item);
case "io.sundr.model."+"Assign": return (VisitableBuilder)new AssignBuilder((Assign) item);
case "io.sundr.model."+"LogicalAnd": return (VisitableBuilder)new LogicalAndBuilder((LogicalAnd) item);
case "io.sundr.model."+"PostIncrement": return (VisitableBuilder)new PostIncrementBuilder((PostIncrement) item);
case "io.sundr.model."+"RightUnsignedShift": return (VisitableBuilder)new RightUnsignedShiftBuilder((RightUnsignedShift) item);
case "io.sundr.model."+"Plus": return (VisitableBuilder)new PlusBuilder((Plus) item);
case "io.sundr.model."+"Construct": return (VisitableBuilder)new ConstructBuilder((Construct) item);
case "io.sundr.model."+"Xor": return (VisitableBuilder)new XorBuilder((Xor) item);
case "io.sundr.model."+"PreIncrement": return (VisitableBuilder)new PreIncrementBuilder((PreIncrement) item);
case "io.sundr.model."+"LessThanOrEqual": return (VisitableBuilder)new LessThanOrEqualBuilder((LessThanOrEqual) item);
case "io.sundr.model."+"Positive": return (VisitableBuilder)new PositiveBuilder((Positive) item);
}
return (VisitableBuilder)builderOf(item);
}
public class MultiplyExpresionNested extends MultiplyFluent> implements Nested{
MultiplyExpresionNested(Multiply item) {
this.builder = new MultiplyBuilder(this, item);
}
MultiplyBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endMultiplyExpresion() {
return and();
}
}
public class NewArrayExpresionNested extends NewArrayFluent> implements Nested{
NewArrayExpresionNested(NewArray item) {
this.builder = new NewArrayBuilder(this, item);
}
NewArrayBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endNewArrayExpresion() {
return and();
}
}
public class InstanceOfExpresionNested extends InstanceOfFluent> implements Nested{
InstanceOfExpresionNested(InstanceOf item) {
this.builder = new InstanceOfBuilder(this, item);
}
InstanceOfBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endInstanceOfExpresion() {
return and();
}
}
public class MethodCallExpresionNested extends MethodCallFluent> implements Nested{
MethodCallExpresionNested(MethodCall item) {
this.builder = new MethodCallBuilder(this, item);
}
MethodCallBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endMethodCallExpresion() {
return and();
}
}
public class InverseExpresionNested extends InverseFluent> implements Nested{
InverseExpresionNested(Inverse item) {
this.builder = new InverseBuilder(this, item);
}
InverseBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endInverseExpresion() {
return and();
}
}
public class IndexExpresionNested extends IndexFluent> implements Nested{
IndexExpresionNested(Index item) {
this.builder = new IndexBuilder(this, item);
}
IndexBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endIndexExpresion() {
return and();
}
}
public class GreaterThanOrEqualExpresionNested extends GreaterThanOrEqualFluent> implements Nested{
GreaterThanOrEqualExpresionNested(GreaterThanOrEqual item) {
this.builder = new GreaterThanOrEqualBuilder(this, item);
}
GreaterThanOrEqualBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endGreaterThanOrEqualExpresion() {
return and();
}
}
public class BitwiseAndExpresionNested extends BitwiseAndFluent> implements Nested{
BitwiseAndExpresionNested(BitwiseAnd item) {
this.builder = new BitwiseAndBuilder(this, item);
}
BitwiseAndBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endBitwiseAndExpresion() {
return and();
}
}
public class MinusExpresionNested extends MinusFluent> implements Nested{
MinusExpresionNested(Minus item) {
this.builder = new MinusBuilder(this, item);
}
MinusBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endMinusExpresion() {
return and();
}
}
public class LogicalOrExpresionNested extends LogicalOrFluent> implements Nested{
LogicalOrExpresionNested(LogicalOr item) {
this.builder = new LogicalOrBuilder(this, item);
}
LogicalOrBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endLogicalOrExpresion() {
return and();
}
}
public class NotEqualsExpresionNested extends NotEqualsFluent> implements Nested{
NotEqualsExpresionNested(NotEquals item) {
this.builder = new NotEqualsBuilder(this, item);
}
NotEqualsBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endNotEqualsExpresion() {
return and();
}
}
public class DivideExpresionNested extends DivideFluent> implements Nested{
DivideExpresionNested(Divide item) {
this.builder = new DivideBuilder(this, item);
}
DivideBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endDivideExpresion() {
return and();
}
}
public class LessThanExpresionNested extends LessThanFluent> implements Nested{
LessThanExpresionNested(LessThan item) {
this.builder = new LessThanBuilder(this, item);
}
LessThanBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endLessThanExpresion() {
return and();
}
}
public class BitwiseOrExpresionNested extends BitwiseOrFluent> implements Nested{
BitwiseOrExpresionNested(BitwiseOr item) {
this.builder = new BitwiseOrBuilder(this, item);
}
BitwiseOrBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endBitwiseOrExpresion() {
return and();
}
}
public class PropertyRefExpresionNested extends PropertyRefFluent> implements Nested{
PropertyRefExpresionNested(PropertyRef item) {
this.builder = new PropertyRefBuilder(this, item);
}
PropertyRefBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endPropertyRefExpresion() {
return and();
}
}
public class RightShiftExpresionNested extends RightShiftFluent> implements Nested{
RightShiftExpresionNested(RightShift item) {
this.builder = new RightShiftBuilder(this, item);
}
RightShiftBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endRightShiftExpresion() {
return and();
}
}
public class GreaterThanExpresionNested extends GreaterThanFluent> implements Nested{
GreaterThanExpresionNested(GreaterThan item) {
this.builder = new GreaterThanBuilder(this, item);
}
GreaterThanBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endGreaterThanExpresion() {
return and();
}
}
public class DeclareExpresionNested extends DeclareFluent> implements Nested{
DeclareExpresionNested(Declare item) {
this.builder = new DeclareBuilder(this, item);
}
DeclareBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endDeclareExpresion() {
return and();
}
}
public class CastExpresionNested extends CastFluent> implements Nested{
CastExpresionNested(Cast item) {
this.builder = new CastBuilder(this, item);
}
CastBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endCastExpresion() {
return and();
}
}
public class ModuloExpresionNested extends ModuloFluent> implements Nested{
ModuloExpresionNested(Modulo item) {
this.builder = new ModuloBuilder(this, item);
}
ModuloBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endModuloExpresion() {
return and();
}
}
public class ValueRefExpresionNested extends ValueRefFluent> implements Nested{
ValueRefExpresionNested(ValueRef item) {
this.builder = new ValueRefBuilder(this, item);
}
ValueRefBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endValueRefExpresion() {
return and();
}
}
public class LeftShiftExpresionNested extends LeftShiftFluent> implements Nested{
LeftShiftExpresionNested(LeftShift item) {
this.builder = new LeftShiftBuilder(this, item);
}
LeftShiftBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endLeftShiftExpresion() {
return and();
}
}
public class TernaryExpresionNested extends TernaryFluent> implements Nested{
TernaryExpresionNested(Ternary item) {
this.builder = new TernaryBuilder(this, item);
}
TernaryBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endTernaryExpresion() {
return and();
}
}
public class BinaryExpressionExpresionNested extends BinaryExpressionFluent> implements Nested{
BinaryExpressionExpresionNested(BinaryExpression item) {
this.builder = new BinaryExpressionBuilder(this, item);
}
BinaryExpressionBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endBinaryExpressionExpresion() {
return and();
}
}
public class EqualsExpresionNested extends EqualsFluent> implements Nested{
EqualsExpresionNested(Equals item) {
this.builder = new EqualsBuilder(this, item);
}
EqualsBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endEqualsExpresion() {
return and();
}
}
public class EnclosedExpresionNested extends EnclosedFluent> implements Nested{
EnclosedExpresionNested(Enclosed item) {
this.builder = new EnclosedBuilder(this, item);
}
EnclosedBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endEnclosedExpresion() {
return and();
}
}
public class PreDecrementExpresionNested extends PreDecrementFluent> implements Nested{
PreDecrementExpresionNested(PreDecrement item) {
this.builder = new PreDecrementBuilder(this, item);
}
PreDecrementBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endPreDecrementExpresion() {
return and();
}
}
public class PostDecrementExpresionNested extends PostDecrementFluent> implements Nested{
PostDecrementExpresionNested(PostDecrement item) {
this.builder = new PostDecrementBuilder(this, item);
}
PostDecrementBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endPostDecrementExpresion() {
return and();
}
}
public class LambdaExpresionNested extends LambdaFluent> implements Nested{
LambdaExpresionNested(Lambda item) {
this.builder = new LambdaBuilder(this, item);
}
LambdaBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endLambdaExpresion() {
return and();
}
}
public class NotExpresionNested extends NotFluent> implements Nested{
NotExpresionNested(Not item) {
this.builder = new NotBuilder(this, item);
}
NotBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endNotExpresion() {
return and();
}
}
public class ThisExpresionNested extends ThisFluent> implements Nested{
ThisExpresionNested(This item) {
this.builder = new ThisBuilder(this, item);
}
ThisBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endThisExpresion() {
return and();
}
}
public class NegativeExpresionNested extends NegativeFluent> implements Nested{
NegativeExpresionNested(Negative item) {
this.builder = new NegativeBuilder(this, item);
}
NegativeBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endNegativeExpresion() {
return and();
}
}
public class AssignExpresionNested extends AssignFluent> implements Nested{
AssignExpresionNested(Assign item) {
this.builder = new AssignBuilder(this, item);
}
AssignBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endAssignExpresion() {
return and();
}
}
public class LogicalAndExpresionNested extends LogicalAndFluent> implements Nested{
LogicalAndExpresionNested(LogicalAnd item) {
this.builder = new LogicalAndBuilder(this, item);
}
LogicalAndBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endLogicalAndExpresion() {
return and();
}
}
public class PostIncrementExpresionNested extends PostIncrementFluent> implements Nested{
PostIncrementExpresionNested(PostIncrement item) {
this.builder = new PostIncrementBuilder(this, item);
}
PostIncrementBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endPostIncrementExpresion() {
return and();
}
}
public class RightUnsignedShiftExpresionNested extends RightUnsignedShiftFluent> implements Nested{
RightUnsignedShiftExpresionNested(RightUnsignedShift item) {
this.builder = new RightUnsignedShiftBuilder(this, item);
}
RightUnsignedShiftBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endRightUnsignedShiftExpresion() {
return and();
}
}
public class PlusExpresionNested extends PlusFluent> implements Nested{
PlusExpresionNested(Plus item) {
this.builder = new PlusBuilder(this, item);
}
PlusBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endPlusExpresion() {
return and();
}
}
public class ConstructExpresionNested extends ConstructFluent> implements Nested{
ConstructExpresionNested(Construct item) {
this.builder = new ConstructBuilder(this, item);
}
ConstructBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endConstructExpresion() {
return and();
}
}
public class XorExpresionNested extends XorFluent> implements Nested{
XorExpresionNested(Xor item) {
this.builder = new XorBuilder(this, item);
}
XorBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endXorExpresion() {
return and();
}
}
public class PreIncrementExpresionNested extends PreIncrementFluent> implements Nested{
PreIncrementExpresionNested(PreIncrement item) {
this.builder = new PreIncrementBuilder(this, item);
}
PreIncrementBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endPreIncrementExpresion() {
return and();
}
}
public class LessThanOrEqualExpresionNested extends LessThanOrEqualFluent> implements Nested{
LessThanOrEqualExpresionNested(LessThanOrEqual item) {
this.builder = new LessThanOrEqualBuilder(this, item);
}
LessThanOrEqualBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endLessThanOrEqualExpresion() {
return and();
}
}
public class PositiveExpresionNested extends PositiveFluent> implements Nested{
PositiveExpresionNested(Positive item) {
this.builder = new PositiveBuilder(this, item);
}
PositiveBuilder builder;
public N and() {
return (N) NotFluent.this.withExpresion(builder.build());
}
public N endPositiveExpresion() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy