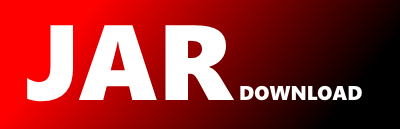
io.swagger.v3.oas.models.Components Maven / Gradle / Ivy
/**
* Copyright 2017 SmartBear Software
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.swagger.v3.oas.models;
import io.swagger.v3.oas.models.callbacks.Callback;
import io.swagger.v3.oas.models.examples.Example;
import io.swagger.v3.oas.models.headers.Header;
import io.swagger.v3.oas.models.links.Link;
import io.swagger.v3.oas.models.media.Schema;
import io.swagger.v3.oas.models.parameters.Parameter;
import io.swagger.v3.oas.models.parameters.RequestBody;
import io.swagger.v3.oas.models.responses.ApiResponse;
import io.swagger.v3.oas.models.security.SecurityScheme;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
/**
* Components
*
* @see "https://github.com/OAI/OpenAPI-Specification/blob/3.0.1/versions/3.0.1.md#componentsObject"
*/
public class Components {
private Map schemas = null;
private Map responses = null;
private Map parameters = null;
private Map examples = null;
private Map requestBodies = null;
private Map headers = null;
private Map securitySchemes = null;
private Map links = null;
private Map callbacks = null;
private java.util.Map extensions = null;
/**
* returns the schemas property from a Components instance.
*
* @return Map<String, Schema> schemas
**/
public Map getSchemas() {
return schemas;
}
public void setSchemas(Map schemas) {
this.schemas = schemas;
}
public Components schemas(Map schemas) {
this.schemas = schemas;
return this;
}
public Components addSchemas(String key, Schema schemasItem) {
if (this.schemas == null) {
this.schemas = new LinkedHashMap<>();
}
this.schemas.put(key, schemasItem);
return this;
}
/**
* returns the responses property from a Components instance.
*
* @return Map<String, ApiResponse> responses
**/
public Map getResponses() {
return responses;
}
public void setResponses(Map responses) {
this.responses = responses;
}
public Components responses(Map responses) {
this.responses = responses;
return this;
}
public Components addResponses(String key, ApiResponse responsesItem) {
if (this.responses == null) {
this.responses = new LinkedHashMap<>();
}
this.responses.put(key, responsesItem);
return this;
}
/**
* returns the parameters property from a Components instance.
*
* @return Map<String, Parameter> parameters
**/
public Map getParameters() {
return parameters;
}
public void setParameters(Map parameters) {
this.parameters = parameters;
}
public Components parameters(Map parameters) {
this.parameters = parameters;
return this;
}
public Components addParameters(String key, Parameter parametersItem) {
if (this.parameters == null) {
this.parameters = new LinkedHashMap<>();
}
this.parameters.put(key, parametersItem);
return this;
}
/**
* returns the examples property from a Components instance.
*
* @return Map<String, Example> examples
**/
public Map getExamples() {
return examples;
}
public void setExamples(Map examples) {
this.examples = examples;
}
public Components examples(Map examples) {
this.examples = examples;
return this;
}
public Components addExamples(String key, Example examplesItem) {
if (this.examples == null) {
this.examples = new LinkedHashMap<>();
}
this.examples.put(key, examplesItem);
return this;
}
/**
* returns the requestBodies property from a Components instance.
*
* @return Map<String, RequestBody> requestBodies
**/
public Map getRequestBodies() {
return requestBodies;
}
public void setRequestBodies(Map requestBodies) {
this.requestBodies = requestBodies;
}
public Components requestBodies(Map requestBodies) {
this.requestBodies = requestBodies;
return this;
}
public Components addRequestBodies(String key, RequestBody requestBodiesItem) {
if (this.requestBodies == null) {
this.requestBodies = new LinkedHashMap<>();
}
this.requestBodies.put(key, requestBodiesItem);
return this;
}
/**
* returns the headers property from a Components instance.
*
* @return Map<String, Header> headers
**/
public Map getHeaders() {
return headers;
}
public void setHeaders(Map headers) {
this.headers = headers;
}
public Components headers(Map headers) {
this.headers = headers;
return this;
}
public Components addHeaders(String key, Header headersItem) {
if (this.headers == null) {
this.headers = new LinkedHashMap<>();
}
this.headers.put(key, headersItem);
return this;
}
/**
* returns the securitySchemes property from a Components instance.
*
* @return Map<String, SecurityScheme> securitySchemes
**/
public Map getSecuritySchemes() {
return securitySchemes;
}
public void setSecuritySchemes(Map securitySchemes) {
this.securitySchemes = securitySchemes;
}
public Components securitySchemes(Map securitySchemes) {
this.securitySchemes = securitySchemes;
return this;
}
public Components addSecuritySchemes(String key, SecurityScheme securitySchemesItem) {
if (this.securitySchemes == null) {
this.securitySchemes = new LinkedHashMap<>();
}
this.securitySchemes.put(key, securitySchemesItem);
return this;
}
/**
* returns the links property from a Components instance.
*
* @return Map<String, Link> links
**/
public Map getLinks() {
return links;
}
public void setLinks(Map links) {
this.links = links;
}
public Components links(Map links) {
this.links = links;
return this;
}
public Components addLinks(String key, Link linksItem) {
if (this.links == null) {
this.links = new LinkedHashMap<>();
}
this.links.put(key, linksItem);
return this;
}
/**
* returns the callbacks property from a Components instance.
*
* @return Map<String, Callback> callbacks
**/
public Map getCallbacks() {
return callbacks;
}
public void setCallbacks(Map callbacks) {
this.callbacks = callbacks;
}
public Components callbacks(Map callbacks) {
this.callbacks = callbacks;
return this;
}
public Components addCallbacks(String key, Callback callbacksItem) {
if (this.callbacks == null) {
this.callbacks = new LinkedHashMap<>();
}
this.callbacks.put(key, callbacksItem);
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Components components = (Components) o;
return Objects.equals(this.schemas, components.schemas) &&
Objects.equals(this.responses, components.responses) &&
Objects.equals(this.parameters, components.parameters) &&
Objects.equals(this.examples, components.examples) &&
Objects.equals(this.requestBodies, components.requestBodies) &&
Objects.equals(this.headers, components.headers) &&
Objects.equals(this.securitySchemes, components.securitySchemes) &&
Objects.equals(this.links, components.links) &&
Objects.equals(this.callbacks, components.callbacks) &&
Objects.equals(this.extensions, components.extensions);
}
@Override
public int hashCode() {
return Objects.hash(schemas, responses, parameters, examples, requestBodies, headers, securitySchemes, links, callbacks, extensions);
}
public java.util.Map getExtensions() {
return extensions;
}
public void addExtension(String name, Object value) {
if (name == null || name.isEmpty() || !name.startsWith("x-")) {
return;
}
if (this.extensions == null) {
this.extensions = new java.util.LinkedHashMap<>();
}
this.extensions.put(name, value);
}
public void setExtensions(java.util.Map extensions) {
this.extensions = extensions;
}
public Components extensions(java.util.Map extensions) {
this.extensions = extensions;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Components {\n");
sb.append(" schemas: ").append(toIndentedString(schemas)).append("\n");
sb.append(" responses: ").append(toIndentedString(responses)).append("\n");
sb.append(" parameters: ").append(toIndentedString(parameters)).append("\n");
sb.append(" examples: ").append(toIndentedString(examples)).append("\n");
sb.append(" requestBodies: ").append(toIndentedString(requestBodies)).append("\n");
sb.append(" headers: ").append(toIndentedString(headers)).append("\n");
sb.append(" securitySchemes: ").append(toIndentedString(securitySchemes)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" callbacks: ").append(toIndentedString(callbacks)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}