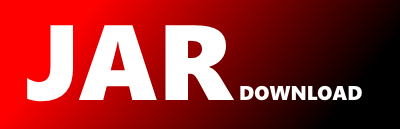
aspnet5.model.mustache Maven / Gradle / Ivy
{{>partial_header}}
using System;
using System.Linq;
using System.IO;
using System.Text;
using System.Collections;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Runtime.Serialization;
using Newtonsoft.Json;
{{#models}}
{{#model}}
namespace {{packageName}}.Models
{
///
/// {{description}}
///
public partial class {{classname}} : {{#parent}}{{{parent}}}, {{/parent}} IEquatable<{{classname}}>
{
///
/// Initializes a new instance of the class.
///
{{#vars}} /// {{#description}}{{description}}{{/description}}{{^description}}{{name}}{{/description}}{{#required}} (required){{/required}}{{#defaultValue}} (default to {{defaultValue}}){{/defaultValue}}.
{{/vars}}
public {{classname}}({{#vars}}{{{datatype}}} {{name}} = null{{#hasMore}}, {{/hasMore}}{{/vars}})
{
{{#vars}}{{#required}}// to ensure "{{name}}" is required (not null)
if ({{name}} == null)
{
throw new InvalidDataException("{{name}} is a required property for {{classname}} and cannot be null");
}
else
{
this.{{name}} = {{name}};
}
{{/required}}{{/vars}}{{#vars}}{{^required}}{{#defaultValue}}// use default value if no "{{name}}" provided
if ({{name}} == null)
{
this.{{name}} = {{{defaultValue}}};
}
else
{
this.{{name}} = {{name}};
}
{{/defaultValue}}{{^defaultValue}}this.{{name}} = {{name}};
{{/defaultValue}}{{/required}}{{/vars}}
}
{{#vars}}
///
/// {{^description}}Gets or Sets {{{name}}}{{/description}}{{#description}}{{{description}}}{{/description}}
/// {{#description}}
/// {{{description}}} {{/description}}
public {{{datatype}}} {{name}} { get; set; }
{{/vars}}
///
/// Returns the string presentation of the object
///
/// String presentation of the object
public override string ToString()
{
var sb = new StringBuilder();
sb.Append("class {{classname}} {\n");
{{#vars}}sb.Append(" {{name}}: ").Append({{name}}).Append("\n");
{{/vars}}
sb.Append("}\n");
return sb.ToString();
}
///
/// Returns the JSON string presentation of the object
///
/// JSON string presentation of the object
public {{#parent}} new {{/parent}}string ToJson()
{
return JsonConvert.SerializeObject(this, Formatting.Indented);
}
///
/// Returns true if objects are equal
///
/// Object to be compared
/// Boolean
public override bool Equals(object obj)
{
if (ReferenceEquals(null, obj)) return false;
if (ReferenceEquals(this, obj)) return true;
if (obj.GetType() != GetType()) return false;
return Equals(({{classname}})obj);
}
///
/// Returns true if {{classname}} instances are equal
///
/// Instance of {{classname}} to be compared
/// Boolean
public bool Equals({{classname}} other)
{
if (ReferenceEquals(null, other)) return false;
if (ReferenceEquals(this, other)) return true;
return {{#vars}}{{#isNotContainer}}
(
this.{{name}} == other.{{name}} ||
this.{{name}} != null &&
this.{{name}}.Equals(other.{{name}})
){{#hasMore}} && {{/hasMore}}{{/isNotContainer}}{{^isNotContainer}}
(
this.{{name}} == other.{{name}} ||
this.{{name}} != null &&
this.{{name}}.SequenceEqual(other.{{name}})
){{#hasMore}} && {{/hasMore}}{{/isNotContainer}}{{/vars}}{{^vars}}false{{/vars}};
}
///
/// Gets the hash code
///
/// Hash code
public override int GetHashCode()
{
// credit: http://stackoverflow.com/a/263416/677735
unchecked // Overflow is fine, just wrap
{
int hash = 41;
// Suitable nullity checks etc, of course :)
{{#vars}}
if (this.{{name}} != null)
hash = hash * 59 + this.{{name}}.GetHashCode();
{{/vars}}
return hash;
}
}
#region Operators
public static bool operator ==({{classname}} left, {{classname}} right)
{
return Equals(left, right);
}
public static bool operator !=({{classname}} left, {{classname}} right)
{
return !Equals(left, right);
}
#endregion Operators
}
{{/model}}
{{/models}}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy