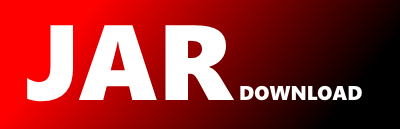
go.api_client.mustache Maven / Gradle / Ivy
{{>partial_header}}
package {{packageName}}
import (
"bytes"
"fmt"
"path/filepath"
"reflect"
"strings"
"net/url"
"github.com/go-resty/resty"
)
type APIClient struct {
}
func (c *APIClient) SelectHeaderContentType(contentTypes []string) string {
if len(contentTypes) == 0 {
return ""
}
if contains(contentTypes, "application/json") {
return "application/json"
}
return contentTypes[0] // use the first content type specified in 'consumes'
}
func (c *APIClient) SelectHeaderAccept(accepts []string) string {
if len(accepts) == 0 {
return ""
}
if contains(accepts, "application/json") {
return "application/json"
}
return strings.Join(accepts, ",")
}
func contains(source []string, containvalue string) bool {
for _, a := range source {
if strings.ToLower(a) == strings.ToLower(containvalue) {
return true
}
}
return false
}
func (c *APIClient) CallAPI(path string, method string,
postBody interface{},
headerParams map[string]string,
queryParams url.Values,
formParams map[string]string,
fileName string,
fileBytes []byte) (*resty.Response, error) {
//set debug flag
configuration := NewConfiguration()
resty.SetDebug(configuration.GetDebug())
request := prepareRequest(postBody, headerParams, queryParams, formParams, fileName, fileBytes)
switch strings.ToUpper(method) {
case "GET":
response, err := request.Get(path)
return response, err
case "POST":
response, err := request.Post(path)
return response, err
case "PUT":
response, err := request.Put(path)
return response, err
case "PATCH":
response, err := request.Patch(path)
return response, err
case "DELETE":
response, err := request.Delete(path)
return response, err
}
return nil, fmt.Errorf("invalid method %v", method)
}
func (c *APIClient) ParameterToString(obj interface{},collectionFormat string) string {
if reflect.TypeOf(obj).String() == "[]string" {
switch collectionFormat {
case "pipes":
return strings.Join(obj.([]string), "|")
case "ssv":
return strings.Join(obj.([]string), " ")
case "tsv":
return strings.Join(obj.([]string), "\t")
case "csv" :
return strings.Join(obj.([]string), ",")
}
}
return fmt.Sprintf("%v", obj)
}
func prepareRequest(postBody interface{},
headerParams map[string]string,
queryParams url.Values,
formParams map[string]string,
fileName string,
fileBytes []byte) *resty.Request {
request := resty.R()
request.SetBody(postBody)
// add header parameter, if any
if len(headerParams) > 0 {
request.SetHeaders(headerParams)
}
// add query parameter, if any
if len(queryParams) > 0 {
request.SetMultiValueQueryParams(queryParams)
}
// add form parameter, if any
if len(formParams) > 0 {
request.SetFormData(formParams)
}
if len(fileBytes) > 0 && fileName != "" {
_, fileNm := filepath.Split(fileName)
request.SetFileReader("file", fileNm, bytes.NewReader(fileBytes))
}
return request
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy