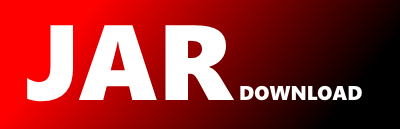
cpprest.modelbase-header.mustache Maven / Gradle / Ivy
{{>licenseInfo}}
/*
* ModelBase.h
*
* This is the base class for all model classes
*/
#ifndef {{modelHeaderGuardPrefix}}_ModelBase_H_
#define {{modelHeaderGuardPrefix}}_ModelBase_H_
{{{defaultInclude}}}
#include "HttpContent.h"
#include "MultipartFormData.h"
#include
#include
{{#modelNamespaceDeclarations}}
namespace {{this}} {
{{/modelNamespaceDeclarations}}
class {{declspec}} ModelBase
{
public:
ModelBase();
virtual ~ModelBase();
virtual void validate() = 0;
virtual web::json::value toJson() const = 0;
virtual void fromJson(web::json::value& json) = 0;
virtual void toMultipart(std::shared_ptr multipart, const utility::string_t& namePrefix) const = 0;
virtual void fromMultiPart(std::shared_ptr multipart, const utility::string_t& namePrefix) = 0;
static web::json::value toJson( const utility::string_t& value );
static web::json::value toJson( const utility::datetime& value );
static web::json::value toJson( std::shared_ptr value );
static web::json::value toJson( std::shared_ptr value );
static web::json::value toJson( int32_t value );
static web::json::value toJson( int64_t value );
static web::json::value toJson( double value );
static web::json::value toJson( bool value );
static int64_t int64_tFromJson(web::json::value& val);
static int32_t int32_tFromJson(web::json::value& val);
static float floatFromJson(web::json::value& val);
static utility::string_t stringFromJson(web::json::value& val);
static utility::datetime dateFromJson(web::json::value& val);
static double doubleFromJson(web::json::value& val);
static bool boolFromJson(web::json::value& val);
static std::shared_ptr fileFromJson(web::json::value& val);
static std::shared_ptr toHttpContent( const utility::string_t& name, const utility::string_t& value, const utility::string_t& contentType = utility::conversions::to_string_t(""));
static std::shared_ptr toHttpContent( const utility::string_t& name, const utility::datetime& value, const utility::string_t& contentType = utility::conversions::to_string_t(""));
static std::shared_ptr toHttpContent( const utility::string_t& name, std::shared_ptr value );
static std::shared_ptr toHttpContent( const utility::string_t& name, const web::json::value& value, const utility::string_t& contentType = utility::conversions::to_string_t("application/json") );
static std::shared_ptr toHttpContent( const utility::string_t& name, int32_t value, const utility::string_t& contentType = utility::conversions::to_string_t("") );
static std::shared_ptr toHttpContent( const utility::string_t& name, int64_t value, const utility::string_t& contentType = utility::conversions::to_string_t("") );
static std::shared_ptr toHttpContent( const utility::string_t& name, double value, const utility::string_t& contentType = utility::conversions::to_string_t("") );
static int64_t int64_tFromHttpContent(std::shared_ptr val);
static int32_t int32_tFromHttpContent(std::shared_ptr val);
static float floatFromHttpContent(std::shared_ptr val);
static utility::string_t stringFromHttpContent(std::shared_ptr val);
static utility::datetime dateFromHttpContent(std::shared_ptr val);
static bool boolFromHttpContent(std::shared_ptr val);
static double doubleFromHttpContent(std::shared_ptr val);
static web::json::value valueFromHttpContent(std::shared_ptr val);
static utility::string_t toBase64( utility::string_t value );
static utility::string_t toBase64( std::shared_ptr value );
static std::shared_ptr fromBase64( const utility::string_t& encoded );
};
{{#modelNamespaceDeclarations}}
}
{{/modelNamespaceDeclarations}}
#endif /* {{modelHeaderGuardPrefix}}_ModelBase_H_ */
© 2015 - 2025 Weber Informatics LLC | Privacy Policy