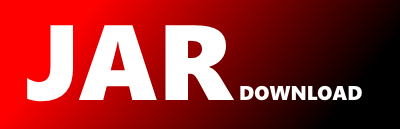
csharp.ApiClient.mustache Maven / Gradle / Ivy
{{>partial_header}}
using System;
using System.Collections;
using System.Collections.Generic;
using System.Globalization;
using System.Text.RegularExpressions;
using System.IO;
{{^netStandard}}
{{^supportsUWP}}
using System.Web;
{{/supportsUWP}}
{{/netStandard}}
using System.Linq;
using System.Net;
using System.Text;
using Newtonsoft.Json;
{{#netStandard}}
using RestSharp.Portable;
using RestSharp.Portable.HttpClient;
{{/netStandard}}
{{^netStandard}}
using RestSharp;
{{/netStandard}}
namespace {{packageName}}.Client
{
///
/// API client is mainly responsible for making the HTTP call to the API backend.
///
{{>visibility}} partial class ApiClient
{
private JsonSerializerSettings serializerSettings = new JsonSerializerSettings
{
ConstructorHandling = ConstructorHandling.AllowNonPublicDefaultConstructor
};
///
/// Allows for extending request processing for generated code.
///
/// The RestSharp request object
partial void InterceptRequest(IRestRequest request);
///
/// Allows for extending response processing for generated code.
///
/// The RestSharp request object
/// The RestSharp response object
partial void InterceptResponse(IRestRequest request, IRestResponse response);
///
/// Initializes a new instance of the class
/// with default configuration.
///
public ApiClient()
{
Configuration = {{packageName}}.Client.Configuration.Default;
RestClient = new RestClient("{{{basePath}}}");
{{#netStandard}}
RestClient.IgnoreResponseStatusCode = true;
{{/netStandard}}
}
///
/// Initializes a new instance of the class
/// with default base path ({{{basePath}}}).
///
/// An instance of Configuration.
public ApiClient(Configuration config)
{
Configuration = config ?? {{packageName}}.Client.Configuration.Default;
RestClient = new RestClient(Configuration.BasePath);
{{#netStandard}}
RestClient.IgnoreResponseStatusCode = true;
{{/netStandard}}
}
///
/// Initializes a new instance of the class
/// with default configuration.
///
/// The base path.
public ApiClient(String basePath = "{{{basePath}}}")
{
if (String.IsNullOrEmpty(basePath))
throw new ArgumentException("basePath cannot be empty");
RestClient = new RestClient(basePath);
{{#netStandard}}
RestClient.IgnoreResponseStatusCode = true;
{{/netStandard}}
Configuration = Client.Configuration.Default;
}
///
/// Gets or sets the default API client for making HTTP calls.
///
/// The default API client.
[Obsolete("ApiClient.Default is deprecated, please use 'Configuration.Default.ApiClient' instead.")]
public static ApiClient Default;
///
/// Gets or sets an instance of the IReadableConfiguration.
///
/// An instance of the IReadableConfiguration.
///
/// helps us to avoid modifying possibly global
/// configuration values from within a given client. It does not guarantee thread-safety
/// of the instance in any way.
///
public IReadableConfiguration Configuration { get; set; }
///
/// Gets or sets the RestClient.
///
/// An instance of the RestClient
public RestClient RestClient { get; set; }
// Creates and sets up a RestRequest prior to a call.
private RestRequest PrepareRequest(
String path, {{^netStandard}}RestSharp.{{/netStandard}}Method method, List> queryParams, Object postBody,
Dictionary headerParams, Dictionary formParams,
Dictionary fileParams, Dictionary pathParams,
String contentType)
{
var request = new RestRequest(path, method);
{{#netStandard}}
// disable ResetSharp.Portable built-in serialization
request.Serializer = null;
{{/netStandard}}
// add path parameter, if any
foreach(var param in pathParams)
request.AddParameter(param.Key, param.Value, ParameterType.UrlSegment);
// add header parameter, if any
foreach(var param in headerParams)
request.AddHeader(param.Key, param.Value);
// add query parameter, if any
foreach(var param in queryParams)
request.AddQueryParameter(param.Key, param.Value);
// add form parameter, if any
foreach(var param in formParams)
request.AddParameter(param.Key, param.Value);
// add file parameter, if any
foreach(var param in fileParams)
{
{{#netStandard}}
request.AddFile(param.Value);
{{/netStandard}}
{{^netStandard}}
{{^supportsUWP}}
request.AddFile(param.Value.Name, param.Value.Writer, param.Value.FileName, param.Value.ContentType);
{{/supportsUWP}}
{{#supportsUWP}}
byte[] paramWriter = null;
param.Value.Writer = delegate (Stream stream) { paramWriter = ToByteArray(stream); };
request.AddFile(param.Value.Name, paramWriter, param.Value.FileName, param.Value.ContentType);
{{/supportsUWP}}
{{/netStandard}}
}
if (postBody != null) // http body (model or byte[]) parameter
{
{{#netStandard}}
request.AddParameter(new Parameter { Value = postBody, Type = ParameterType.RequestBody, ContentType = contentType });
{{/netStandard}}
{{^netStandard}}
request.AddParameter(contentType, postBody, ParameterType.RequestBody);
{{/netStandard}}
}
return request;
}
///
/// Makes the HTTP request (Sync).
///
/// URL path.
/// HTTP method.
/// Query parameters.
/// HTTP body (POST request).
/// Header parameters.
/// Form parameters.
/// File parameters.
/// Path parameters.
/// Content Type of the request
/// Object
public Object CallApi(
String path, {{^netStandard}}RestSharp.{{/netStandard}}Method method, List> queryParams, Object postBody,
Dictionary headerParams, Dictionary formParams,
Dictionary fileParams, Dictionary pathParams,
String contentType)
{
var request = PrepareRequest(
path, method, queryParams, postBody, headerParams, formParams, fileParams,
pathParams, contentType);
// set timeout
{{#netStandard}}RestClient.Timeout = TimeSpan.FromMilliseconds(Configuration.Timeout);{{/netStandard}}
{{^netStandard}}RestClient.Timeout = Configuration.Timeout;{{/netStandard}}
// set user agent
RestClient.UserAgent = Configuration.UserAgent;
InterceptRequest(request);
{{#netStandard}}
var response = RestClient.Execute(request).Result;
{{/netStandard}}
{{^netStandard}}
{{^supportsUWP}}
var response = RestClient.Execute(request);
{{/supportsUWP}}
{{#supportsUWP}}
// Using async method to perform sync call (uwp-only)
var response = RestClient.ExecuteTaskAsync(request).Result;
{{/supportsUWP}}
{{/netStandard}}
InterceptResponse(request, response);
return (Object) response;
}
{{#supportsAsync}}
///
/// Makes the asynchronous HTTP request.
///
/// URL path.
/// HTTP method.
/// Query parameters.
/// HTTP body (POST request).
/// Header parameters.
/// Form parameters.
/// File parameters.
/// Path parameters.
/// Content type.
/// The Task instance.
public async System.Threading.Tasks.Task
© 2015 - 2025 Weber Informatics LLC | Privacy Policy