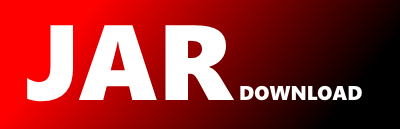
flaskConnexion.model.mustache Maven / Gradle / Ivy
# coding: utf-8
from __future__ import absolute_import
from datetime import date, datetime # noqa: F401
from typing import List, Dict # noqa: F401
from {{modelPackage}}.base_model_ import Model
from {{packageName}} import util
{{#models}}
{{#model}}
class {{classname}}(Model):
"""NOTE: This class is auto generated by the swagger code generator program.
Do not edit the class manually.
"""{{#allowableValues}}
"""
allowed enum values
"""
{{#enumVars}}
{{name}} = {{{value}}}{{^-last}}
{{/-last}}
{{/enumVars}}{{/allowableValues}}
def __init__(self{{#vars}}, {{name}}{{^supportPython2}}: {{datatype}}{{/supportPython2}}={{#defaultValue}}{{{defaultValue}}}{{/defaultValue}}{{^defaultValue}}None{{/defaultValue}}{{/vars}}): # noqa: E501
"""{{classname}} - a model defined in Swagger
{{#vars}}
:param {{name}}: The {{name}} of this {{classname}}. # noqa: E501
:type {{name}}: {{datatype}}
{{/vars}}
"""
self.swagger_types = {
{{#vars}}
'{{name}}': {{{datatype}}}{{#hasMore}},{{/hasMore}}
{{/vars}}
}
self.attribute_map = {
{{#vars}}
'{{name}}': '{{baseName}}'{{#hasMore}},{{/hasMore}}
{{/vars}}
}
{{#vars}}{{#-first}}
{{/-first}}
self._{{name}} = {{name}}
{{/vars}}
@classmethod
def from_dict(cls, dikt){{^supportPython2}} -> '{{classname}}'{{/supportPython2}}:
"""Returns the dict as a model
:param dikt: A dict.
:type: dict
:return: The {{name}} of this {{classname}}. # noqa: E501
:rtype: {{classname}}
"""
return util.deserialize_model(dikt, cls){{#vars}}{{#-first}}
{{/-first}}
@property
def {{name}}(self){{^supportPython2}} -> {{datatype}}{{/supportPython2}}:
"""Gets the {{name}} of this {{classname}}.
{{#description}}
{{{description}}} # noqa: E501
{{/description}}
:return: The {{name}} of this {{classname}}.
:rtype: {{datatype}}
"""
return self._{{name}}
@{{name}}.setter
def {{name}}(self, {{name}}{{^supportPython2}}: {{datatype}}{{/supportPython2}}):
"""Sets the {{name}} of this {{classname}}.
{{#description}}
{{{description}}} # noqa: E501
{{/description}}
:param {{name}}: The {{name}} of this {{classname}}.
:type {{name}}: {{datatype}}
"""
{{#isEnum}}
allowed_values = [{{#allowableValues}}{{#values}}"{{{this}}}"{{^-last}}, {{/-last}}{{/values}}{{/allowableValues}}] # noqa: E501
{{#isContainer}}
{{#isListContainer}}
if not set({{{name}}}).issubset(set(allowed_values)):
raise ValueError(
"Invalid values for `{{{name}}}` [{0}], must be a subset of [{1}]" # noqa: E501
.format(", ".join(map(str, set({{{name}}}) - set(allowed_values))), # noqa: E501
", ".join(map(str, allowed_values)))
)
{{/isListContainer}}
{{#isMapContainer}}
if not set({{{name}}}.keys()).issubset(set(allowed_values)):
raise ValueError(
"Invalid keys in `{{{name}}}` [{0}], must be a subset of [{1}]" # noqa: E501
.format(", ".join(map(str, set({{{name}}}.keys()) - set(allowed_values))), # noqa: E501
", ".join(map(str, allowed_values)))
)
{{/isMapContainer}}
{{/isContainer}}
{{^isContainer}}
if {{{name}}} not in allowed_values:
raise ValueError(
"Invalid value for `{{{name}}}` ({0}), must be one of {1}"
.format({{{name}}}, allowed_values)
)
{{/isContainer}}
{{/isEnum}}
{{^isEnum}}
{{#required}}
if {{name}} is None:
raise ValueError("Invalid value for `{{name}}`, must not be `None`") # noqa: E501
{{/required}}
{{#hasValidation}}
{{#maxLength}}
if {{name}} is not None and len({{name}}) > {{maxLength}}:
raise ValueError("Invalid value for `{{name}}`, length must be less than or equal to `{{maxLength}}`") # noqa: E501
{{/maxLength}}
{{#minLength}}
if {{name}} is not None and len({{name}}) < {{minLength}}:
raise ValueError("Invalid value for `{{name}}`, length must be greater than or equal to `{{minLength}}`") # noqa: E501
{{/minLength}}
{{#maximum}}
if {{name}} is not None and {{name}} >{{#exclusiveMaximum}}={{/exclusiveMaximum}} {{maximum}}: # noqa: E501
raise ValueError("Invalid value for `{{name}}`, must be a value less than {{^exclusiveMaximum}}or equal to {{/exclusiveMaximum}}`{{maximum}}`") # noqa: E501
{{/maximum}}
{{#minimum}}
if {{name}} is not None and {{name}} <{{#exclusiveMinimum}}={{/exclusiveMinimum}} {{minimum}}: # noqa: E501
raise ValueError("Invalid value for `{{name}}`, must be a value greater than {{^exclusiveMinimum}}or equal to {{/exclusiveMinimum}}`{{minimum}}`") # noqa: E501
{{/minimum}}
{{#pattern}}
if {{name}} is not None and not re.search(r'{{{vendorExtensions.x-regex}}}', {{name}}{{#vendorExtensions.x-modifiers}}{{#-first}}, flags={{/-first}}re.{{.}}{{^-last}} | {{/-last}}{{/vendorExtensions.x-modifiers}}): # noqa: E501
raise ValueError("Invalid value for `{{name}}`, must be a follow pattern or equal to `{{{pattern}}}`") # noqa: E501
{{/pattern}}
{{#maxItems}}
if {{name}} is not None and len({{name}}) > {{maxItems}}:
raise ValueError("Invalid value for `{{name}}`, number of items must be less than or equal to `{{maxItems}}`") # noqa: E501
{{/maxItems}}
{{#minItems}}
if {{name}} is not None and len({{name}}) < {{minItems}}:
raise ValueError("Invalid value for `{{name}}`, number of items must be greater than or equal to `{{minItems}}`") # noqa: E501
{{/minItems}}
{{/hasValidation}}
{{/isEnum}}
self._{{name}} = {{name}}{{^-last}}
{{/-last}}
{{/vars}}
{{/model}}
{{/models}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy