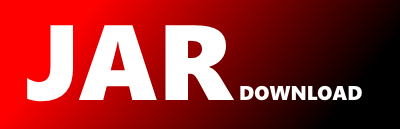
typescript-node.api.mustache Maven / Gradle / Ivy
{{>licenseInfo}}
import localVarRequest = require('request');
import http = require('http');
{{^supportsES6}}
import Promise = require('bluebird');
{{/supportsES6}}
let defaultBasePath = '{{{basePath}}}';
// ===============================================
// This file is autogenerated - Please do not edit
// ===============================================
/* tslint:disable:no-unused-variable */
let primitives = [
"string",
"boolean",
"double",
"integer",
"long",
"float",
"number",
"any"
];
class ObjectSerializer {
public static findCorrectType(data: any, expectedType: string) {
if (data == undefined) {
return expectedType;
} else if (primitives.indexOf(expectedType.toLowerCase()) !== -1) {
return expectedType;
} else if (expectedType === "Date") {
return expectedType;
} else {
if (enumsMap[expectedType]) {
return expectedType;
}
if (!typeMap[expectedType]) {
return expectedType; // w/e we don't know the type
}
// Check the discriminator
let discriminatorProperty = typeMap[expectedType].discriminator;
if (discriminatorProperty == null) {
return expectedType; // the type does not have a discriminator. use it.
} else {
if (data[discriminatorProperty]) {
return data[discriminatorProperty]; // use the type given in the discriminator
} else {
return expectedType; // discriminator was not present (or an empty string)
}
}
}
}
public static serialize(data: any, type: string) {
if (data == undefined) {
return data;
} else if (primitives.indexOf(type.toLowerCase()) !== -1) {
return data;
} else if (type.lastIndexOf("Array<", 0) === 0) { // string.startsWith pre es6
let subType: string = type.replace("Array<", ""); // Array => Type>
subType = subType.substring(0, subType.length - 1); // Type> => Type
let transformedData: any[] = [];
for (let index in data) {
let date = data[index];
transformedData.push(ObjectSerializer.serialize(date, subType));
}
return transformedData;
} else if (type === "Date") {
return data.toString();
} else {
if (enumsMap[type]) {
return data;
}
if (!typeMap[type]) { // in case we dont know the type
return data;
}
// get the map for the correct type.
let attributeTypes = typeMap[type].getAttributeTypeMap();
let instance: {[index: string]: any} = {};
for (let index in attributeTypes) {
let attributeType = attributeTypes[index];
instance[attributeType.baseName] = ObjectSerializer.serialize(data[attributeType.name], attributeType.type);
}
return instance;
}
}
public static deserialize(data: any, type: string) {
// polymorphism may change the actual type.
type = ObjectSerializer.findCorrectType(data, type);
if (data == undefined) {
return data;
} else if (primitives.indexOf(type.toLowerCase()) !== -1) {
return data;
} else if (type.lastIndexOf("Array<", 0) === 0) { // string.startsWith pre es6
let subType: string = type.replace("Array<", ""); // Array => Type>
subType = subType.substring(0, subType.length - 1); // Type> => Type
let transformedData: any[] = [];
for (let index in data) {
let date = data[index];
transformedData.push(ObjectSerializer.deserialize(date, subType));
}
return transformedData;
} else if (type === "Date") {
return new Date(data);
} else {
if (enumsMap[type]) {// is Enum
return data;
}
if (!typeMap[type]) { // dont know the type
return data;
}
let instance = new typeMap[type]();
let attributeTypes = typeMap[type].getAttributeTypeMap();
for (let index in attributeTypes) {
let attributeType = attributeTypes[index];
instance[attributeType.name] = ObjectSerializer.deserialize(data[attributeType.baseName], attributeType.type);
}
return instance;
}
}
}
{{#models}}
{{#model}}
{{#description}}
/**
* {{{description}}}
*/
{{/description}}
export class {{classname}} {{#parent}}extends {{{parent}}} {{/parent}}{
{{#vars}}
{{#description}}
/**
* {{{description}}}
*/
{{/description}}
'{{name}}'{{^required}}?{{/required}}: {{#isEnum}}{{{datatypeWithEnum}}}{{/isEnum}}{{^isEnum}}{{{datatype}}}{{/isEnum}};
{{/vars}}
{{#discriminator}}
static discriminator: string | undefined = "{{discriminator}}";
{{/discriminator}}
{{^discriminator}}
static discriminator: string | undefined = undefined;
{{/discriminator}}
{{^isArrayModel}}
static attributeTypeMap: Array<{name: string, baseName: string, type: string}> = [
{{#vars}}
{
"name": "{{name}}",
"baseName": "{{baseName}}",
"type": "{{#isEnum}}{{{datatypeWithEnum}}}{{/isEnum}}{{^isEnum}}{{{datatype}}}{{/isEnum}}"
}{{#hasMore}},
{{/hasMore}}
{{/vars}}
];
static getAttributeTypeMap() {
{{#parent}}
return super.getAttributeTypeMap().concat({{classname}}.attributeTypeMap);
{{/parent}}
{{^parent}}
return {{classname}}.attributeTypeMap;
{{/parent}}
}
{{/isArrayModel}}
}
{{#hasEnums}}
export namespace {{classname}} {
{{#vars}}
{{#isEnum}}
export enum {{enumName}} {
{{#allowableValues}}
{{#enumVars}}
{{name}} = {{{value}}}{{^-last}},{{/-last}}
{{/enumVars}}
{{/allowableValues}}
}
{{/isEnum}}
{{/vars}}
}
{{/hasEnums}}
{{/model}}
{{/models}}
let enumsMap: {[index: string]: any} = {
{{#models}}
{{#model}}
{{#hasEnums}}
{{#vars}}
{{#isEnum}}
{{#isContainer}}"{{classname}}.{{enumName}}": {{classname}}.{{enumName}}{{/isContainer}}{{#isNotContainer}}"{{datatypeWithEnum}}": {{datatypeWithEnum}}{{/isNotContainer}},
{{/isEnum}}
{{/vars}}
{{/hasEnums}}
{{/model}}
{{/models}}
}
let typeMap: {[index: string]: any} = {
{{#models}}
{{#model}}
"{{classname}}": {{classname}},
{{/model}}
{{/models}}
}
export interface Authentication {
/**
* Apply authentication settings to header and query params.
*/
applyToRequest(requestOptions: localVarRequest.Options): void;
}
export class HttpBasicAuth implements Authentication {
public username: string = '';
public password: string = '';
applyToRequest(requestOptions: localVarRequest.Options): void {
requestOptions.auth = {
username: this.username, password: this.password
}
}
}
export class ApiKeyAuth implements Authentication {
public apiKey: string = '';
constructor(private location: string, private paramName: string) {
}
applyToRequest(requestOptions: localVarRequest.Options): void {
if (this.location == "query") {
(requestOptions.qs)[this.paramName] = this.apiKey;
} else if (this.location == "header" && requestOptions && requestOptions.headers) {
requestOptions.headers[this.paramName] = this.apiKey;
}
}
}
export class OAuth implements Authentication {
public accessToken: string = '';
applyToRequest(requestOptions: localVarRequest.Options): void {
if (requestOptions && requestOptions.headers) {
requestOptions.headers["Authorization"] = "Bearer " + this.accessToken;
}
}
}
export class VoidAuth implements Authentication {
public username: string = '';
public password: string = '';
applyToRequest(_: localVarRequest.Options): void {
// Do nothing
}
}
{{#apiInfo}}
{{#apis}}
{{#operations}}
{{#description}}
/**
* {{&description}}
*/
{{/description}}
export enum {{classname}}ApiKeys {
{{#authMethods}}
{{#isApiKey}}
{{name}},
{{/isApiKey}}
{{/authMethods}}
}
export class {{classname}} {
protected _basePath = defaultBasePath;
protected defaultHeaders : any = {};
protected _useQuerystring : boolean = false;
protected authentications = {
'default': new VoidAuth(),
{{#authMethods}}
{{#isBasic}}
'{{name}}': new HttpBasicAuth(),
{{/isBasic}}
{{#isApiKey}}
'{{name}}': new ApiKeyAuth({{#isKeyInHeader}}'header'{{/isKeyInHeader}}{{^isKeyInHeader}}'query'{{/isKeyInHeader}}, '{{keyParamName}}'),
{{/isApiKey}}
{{#isOAuth}}
'{{name}}': new OAuth(),
{{/isOAuth}}
{{/authMethods}}
}
constructor(basePath?: string);
{{#authMethods}}
{{#isBasic}}
constructor(username: string, password: string, basePath?: string);
{{/isBasic}}
{{/authMethods}}
constructor(basePathOrUsername: string, password?: string, basePath?: string) {
if (password) {
{{#authMethods}}
{{#isBasic}}
this.username = basePathOrUsername;
this.password = password
{{/isBasic}}
{{/authMethods}}
if (basePath) {
this.basePath = basePath;
}
} else {
if (basePathOrUsername) {
this.basePath = basePathOrUsername
}
}
}
set useQuerystring(value: boolean) {
this._useQuerystring = value;
}
set basePath(basePath: string) {
this._basePath = basePath;
}
get basePath() {
return this._basePath;
}
public setDefaultAuthentication(auth: Authentication) {
this.authentications.default = auth;
}
public setApiKey(key: {{classname}}ApiKeys, value: string) {
(this.authentications as any)[{{classname}}ApiKeys[key]].apiKey = value;
}
{{#authMethods}}
{{#isBasic}}
set username(username: string) {
this.authentications.{{name}}.username = username;
}
set password(password: string) {
this.authentications.{{name}}.password = password;
}
{{/isBasic}}
{{#isOAuth}}
set accessToken(token: string) {
this.authentications.{{name}}.accessToken = token;
}
{{/isOAuth}}
{{/authMethods}}
{{#operation}}
/**
* {{¬es}}
{{#summary}}
* @summary {{&summary}}
{{/summary}}
{{#allParams}}
* @param {{paramName}} {{description}}
{{/allParams}}
* @param {*} [options] Override http request options.
*/
public {{nickname}} ({{#allParams}}{{paramName}}{{^required}}?{{/required}}: {{{dataType}}}, {{/allParams}}options: any = {}) : Promise<{ response: http.{{#supportsES6}}IncomingMessage{{/supportsES6}}{{^supportsES6}}ClientResponse{{/supportsES6}}; {{#returnType}}body: {{{returnType}}}; {{/returnType}}{{^returnType}}body?: any; {{/returnType}} }> {
const localVarPath = this.basePath + '{{{path}}}'{{#pathParams}}
.replace('{' + '{{baseName}}' + '}', encodeURIComponent(String({{paramName}}))){{/pathParams}};
let localVarQueryParameters: any = {};
let localVarHeaderParams: any = (Object).assign({}, this.defaultHeaders);
let localVarFormParams: any = {};
{{#allParams}}
{{#required}}
// verify required parameter '{{paramName}}' is not null or undefined
if ({{paramName}} === null || {{paramName}} === undefined) {
throw new Error('Required parameter {{paramName}} was null or undefined when calling {{nickname}}.');
}
{{/required}}
{{/allParams}}
{{#queryParams}}
if ({{paramName}} !== undefined) {
localVarQueryParameters['{{baseName}}'] = ObjectSerializer.serialize({{paramName}}, "{{{dataType}}}");
}
{{/queryParams}}
{{#headerParams}}
localVarHeaderParams['{{baseName}}'] = ObjectSerializer.serialize({{paramName}}, "{{{dataType}}}");
{{/headerParams}}
(Object).assign(localVarHeaderParams, options.headers);
let localVarUseFormData = false;
{{#formParams}}
if ({{paramName}} !== undefined) {
{{#isFile}}
localVarFormParams['{{baseName}}'] = {{paramName}};
{{/isFile}}
{{^isFile}}
localVarFormParams['{{baseName}}'] = ObjectSerializer.serialize({{paramName}}, "{{{dataType}}}");
{{/isFile}}
}
{{#isFile}}
localVarUseFormData = true;
{{/isFile}}
{{/formParams}}
let localVarRequestOptions: localVarRequest.Options = {
method: '{{httpMethod}}',
qs: localVarQueryParameters,
headers: localVarHeaderParams,
uri: localVarPath,
useQuerystring: this._useQuerystring,
{{^isResponseFile}}
json: true,
{{/isResponseFile}}
{{#isResponseFile}}
encoding: null,
{{/isResponseFile}}
{{#bodyParam}}
body: ObjectSerializer.serialize({{paramName}}, "{{{dataType}}}")
{{/bodyParam}}
};
{{#authMethods}}
this.authentications.{{name}}.applyToRequest(localVarRequestOptions);
{{/authMethods}}
this.authentications.default.applyToRequest(localVarRequestOptions);
if (Object.keys(localVarFormParams).length) {
if (localVarUseFormData) {
(localVarRequestOptions).formData = localVarFormParams;
} else {
localVarRequestOptions.form = localVarFormParams;
}
}
return new Promise<{ response: http.{{#supportsES6}}IncomingMessage{{/supportsES6}}{{^supportsES6}}ClientResponse{{/supportsES6}}; {{#returnType}}body: {{{returnType}}}; {{/returnType}}{{^returnType}}body?: any; {{/returnType}} }>((resolve, reject) => {
localVarRequest(localVarRequestOptions, (error, response, body) => {
if (error) {
reject(error);
} else {
{{#returnType}}
body = ObjectSerializer.deserialize(body, "{{{returnType}}}");
{{/returnType}}
if (response.statusCode && response.statusCode >= 200 && response.statusCode <= 299) {
resolve({ response: response, body: body });
} else {
reject({ response: response, body: body });
}
}
});
});
}
{{/operation}}
}
{{/operations}}
{{/apis}}
{{/apiInfo}}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy