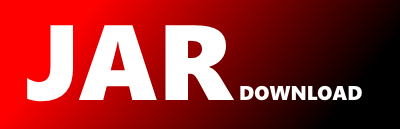
apex.api.mustache Maven / Gradle / Ivy
{{>licenseInfo}}
{{#operations}}
public class {{classname}} {
{{classPrefix}}Client client;
public {{classname}}({{classPrefix}}Client client) {
this.client = client;
}
public {{classname}}() {
this.client = new {{classPrefix}}Client();
}
public {{classPrefix}}Client getClient() {
return this.client;
}
{{#operation}}
/**
* {{summary}}
* {{notes}}
{{#allParams}}
* @param {{paramName}} {{description}}{{#required}} (required){{/required}}{{^required}} (optional{{#defaultValue}}, default to {{{.}}}{{/defaultValue}}){{/required}}
{{/allParams}}
{{#returnType}}
* @return {{{returnType}}}
{{/returnType}}
* @throws Swagger.ApiException if fails to make API call
*/
public {{#returnType}}{{{returnType}}}{{/returnType}}{{^returnType}}void{{/returnType}} {{operationId}}({{#hasParams}}Map params{{/hasParams}}) {
{{#allParams}}
{{#required}}
client.assertNotNull(params.get('{{paramName}}'), '{{paramName}}');
{{/required}}
{{/allParams}}
List query = new List();
{{#hasQueryParams}}
// cast query params to verify their expected type
{{/hasQueryParams}}
{{#queryParams}}
{{#isListContainer}}
{{#isCollectionFormatMulti}}
query.addAll(client.makeParams('{{baseName}}', ({{{dataType}}}) params.get('{{paramName}}')));
{{/isCollectionFormatMulti}}
{{^isCollectionFormatMulti}}
query.addAll(client.makeParam('{{baseName}}', ({{{dataType}}}) params.get('{{paramName}}'), '{{collectionFormat}}'));
{{/isCollectionFormatMulti}}
{{/isListContainer}}
{{^isListContainer}}
query.addAll(client.makeParam('{{baseName}}', ({{{dataType}}}) params.get('{{paramName}}')));
{{/isListContainer}}
{{^hasMore}}
{{/hasMore}}
{{/queryParams}}
List form = new List();
{{#hasFormParams}}
// cast form params to verify their expected type
{{/hasFormParams}}
{{#formParams}}
{{#isListContainer}}
{{#isCollectionFormatMulti}}
form.addAll(client.makeParams('{{baseName}}', ({{{dataType}}}) params.get('{{paramName}}')));
{{/isCollectionFormatMulti}}
{{^isCollectionFormatMulti}}
form.addAll(client.makeParam('{{baseName}}', ({{{dataType}}}) params.get('{{paramName}}'), '{{collectionFormat}}'));
{{/isCollectionFormatMulti}}
{{/isListContainer}}
{{^isListContainer}}
form.addAll(client.makeParam('{{baseName}}', ({{{dataType}}}) params.get('{{paramName}}')));
{{/isListContainer}}
{{/formParams}}
{{#returnType}}return ({{{returnType}}}) {{/returnType}}client.invoke(
'{{httpMethod}}', '{{path}}',{{#bodyParam}}
({{{dataType}}}) params.get('{{paramName}}'){{/bodyParam}}{{^bodyParam}} ''{{/bodyParam}},
query, form,
new Map{{#hasPathParams}}{
{{#pathParams}}
'{{baseName}}' => ({{{dataType}}}) params.get('{{paramName}}'){{#hasMore}},{{/hasMore}}
{{/pathParams}}
}{{/hasPathParams}}{{^hasPathParams}}(){{/hasPathParams}},
new Map{{#hasHeaderParams}}{
{{#headerParams}}
'{{baseName}}' => ({{{dataType}}}) params.get('{{paramName}}'){{#hasMore}},{{/hasMore}}
{{/headerParams}}
}{{/hasHeaderParams}}{{^hasHeaderParams}}(){{/hasHeaderParams}},
{{#hasProduces}}
new List{ {{#produces}}'{{{mediaType}}}'{{#hasMore}}, {{/hasMore}}{{/produces}} },
{{/hasProduces}}
{{^hasProduces}}
new List(),
{{/hasProduces}}
{{#hasConsumes}}
new List{ {{#consumes}}'{{{mediaType}}}'{{#hasMore}}, {{/hasMore}}{{/consumes}} },
{{/hasConsumes}}
{{^hasConsumes}}
new List(),
{{/hasConsumes}}
{{#hasAuthMethods}}
new List { {{#authMethods}}'{{name}}'{{#hasMore}}, {{/hasMore}}{{/authMethods}} },
{{/hasAuthMethods}}
{{^hasAuthMethods}}
new List(),
{{/hasAuthMethods}}
{{#returnType}}
{{{returnType}}}.class
{{/returnType}}
{{^returnType}}
null
{{/returnType}}
);
}
{{/operation}}
}
{{/operations}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy